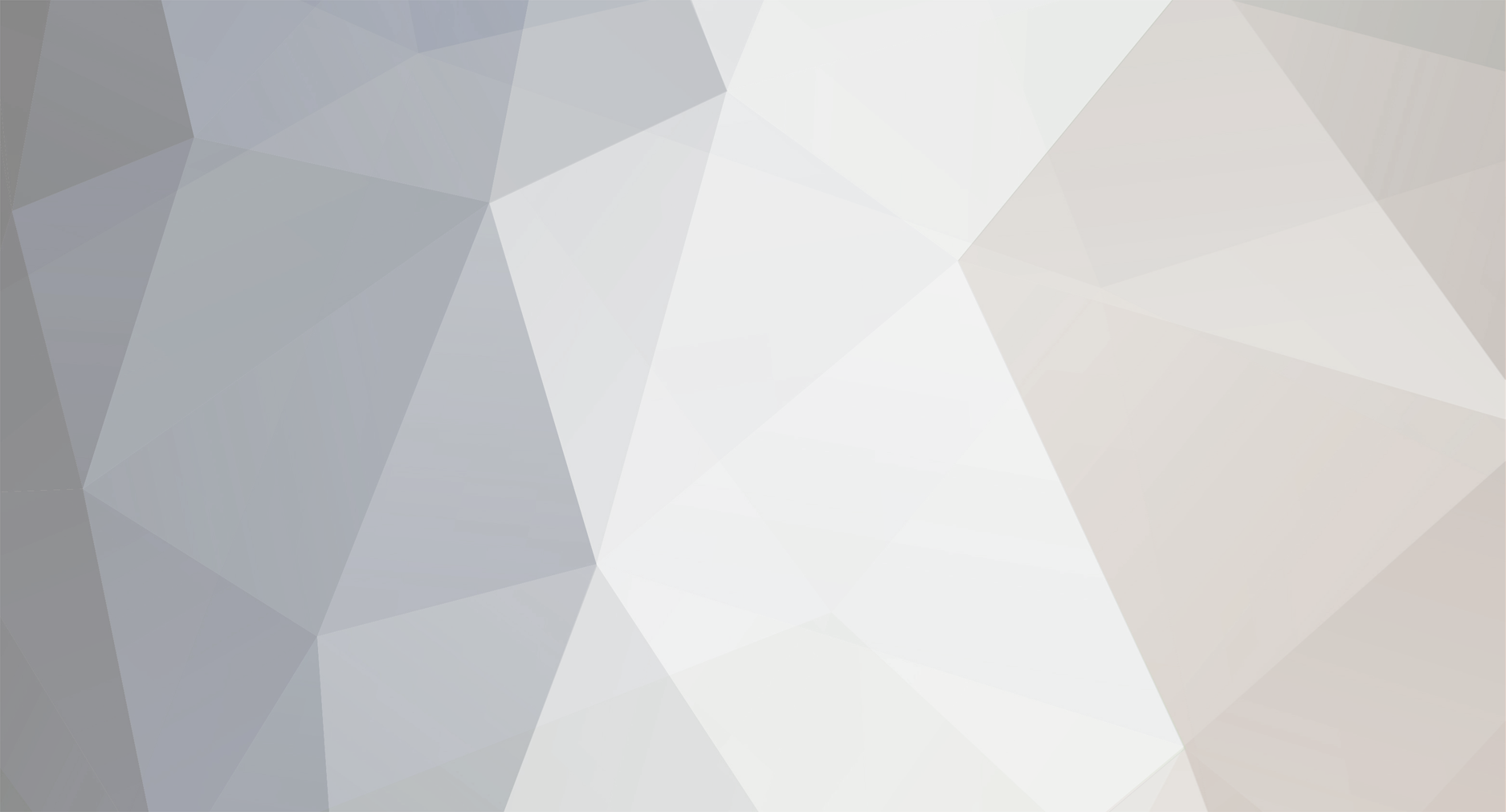
TGWSE_GY
Members-
Posts
255 -
Joined
-
Last visited
Never
Everything posted by TGWSE_GY
-
PHP Arrays - Adding Elements when conditions are met in a loop.
TGWSE_GY replied to TGWSE_GY's topic in PHP Coding Help
Thanks RussellReal works like a charm I also wanted to post my working code in hopes that it helps someone else someday <?php //Get config file include('content/conf/config.site.php'); //Get directory $directory = $abs_site_root . $dir_content; $dir = dir("$directory"); $filearray = array(0 => "seed"); //List files in images directory while (($file = $dir->read()) !== false) { if($file != "."){ if($file != ".."){ $search = ".php"; $replace = ""; $file = str_replace($search, $replace, $file); $filearray[] = $file; } } } $dir->close(); ?> -
I am wanting to create an array on the fly, when two if conditions are true then I can add the file name to the array. As it stands right now it adds nothing to the array and I think it may have to do with array_push(). Here is the code, any thoughts? <?php //Get config file include('content/conf/config.site.php'); //Get directory $directory = $abs_site_root . $dir_content; $dir = dir("$directory"); $count = 1; $filearray = array(0 => "seed"); //List files in images directory while (($file = $dir->read()) !== false) { if($file != "."){ if($file != ".."){ $search = ".php"; $replace = ""; $count ++; $file = str_replace($search, $replace, $file); array_push($filearray, $count, $file); } } } $dir->close(); ?> Thanks guys!!!
-
MDanz php.ini is the config file that dictates what intrinsic functions are turned on or off. It works in the similar way of a .ini file in windows for a lot of applications, where you set properties for the application to run with. The same thing with php if you set url_fopen=1 in your php.ini(where your php compiler is installed on the server) it will turn it on. However I personally do not recomend using url_fopen because of security risks. I would suggest using cURL http://us3.php.net/manual/en/curl.examples-basic.php
-
A better question would be how do I ensure my data is not broken when posting to the db?
-
What are replace querys?
-
yes there is slashes in the db now
-
Just checked and I don't have magic quotes enabled.
-
Now when things are being returned from the db where there was comma there is a forward slash now that I am using mysql_real_escape_string(). What am I doing wrong. here is my new code <?php mysql_connect($dbhost, $dbuser, $dbpass) or die(mysql_error()); mysql_select_db($dbname) or die(mysql_error()); $identity = $_POST['identity']; if($identity == "1"){ $description = mysql_real_escape_string($_POST['accommodations']); $query = "UPDATE accommodations SET column2='$description' WHERE id=1"; mysql_query($query) or die(mysql_error()); header("Location: http://www.innonmainmanasquan.com/admin/index.php?section=3"); }elseif($identity=="2"){ $content = mysql_real_escape_string($_POST['content']); $promotitle = mysql_real_escape_string($_POST['promotitle']); $promo = mysql_real_escape_string($_POST['promo']); $address1 = mysql_real_escape_string($_POST['address1']); $address2 = mysql_real_escape_string($_POST['address2']); $phone = mysql_real_escape_string($_POST['phone']); $query = "UPDATE `home` SET content='$content', promotitle='$promotitle', promo='$promo', address1='$address1', address2='$address2', phone='$phone' WHERE id= '1'"; mysql_query($query) or die(mysql_error()); header("Location: http://www.innonmainmanasquan.com/admin/index.php?section=1"); }elseif($identity=="3"){ $north = mysql_real_escape_string($_POST['north']); $south = mysql_real_escape_string($_POST['south']); $west = mysql_real_escape_string($_POST['west']); $query = "UPDATE `directions` SET north='$north', south='$south', west='$west' WHERE id='1'"; mysql_query($query) or die(mysql_error()); header("Location: http://www.innonmainmanasquan.com/admin/index.php?section=3"); }elseif($identity=="4"){ $content = mysql_real_escape_string($_POST['description']); $phone1 = mysql_real_escape_string($_POST['phone1']); $phone2 = mysql_real_escape_string($_POST['phone2']); $email = mysql_real_escape_string($_POST['email']); $specialtitle = mysql_real_escape_string($_POST['specialtitle']); $special = mysql_real_escape_string($_POST['special']); $query = "UPDATE `reservations` SET content='$content', phone1='$phone1', phone2='$phone2', email='$email', specialtitle='$specialtitle', special='$special' WHERE id='1'"; mysql_query($query) or die(mysql_error()); header("Location: http://www.innonmainmanasquan.com/admin/index.php?section=4"); }elseif($identity=="5"){ $dinner = mysql_real_escape_string($_POST['dinner']); $lunch = mysql_real_escape_string($_POST['lunch']); $brunch = mysql_real_escape_string($_POST['brunch']); $query = "UPDATE `restaurant` SET dinner='$dinner', lunch='$lunch', brunch='$brunch' WHERE id='1'"; mysql_query($query) or die(mysql_error()); header("Location: http://www.innonmainmanasquan.com/admin/index.php?section=5"); }elseif($identity != 1){ if($identity != 2){ if($identy != 3){ if($identity != 4){ if($identity !=5 ){ $description = $_POST['description']; $cost = $_POST['cost']; $query = "UPDATE `rooms` SET description='$description' cost='$cost' WHERE roomnumber='$identity'"; mysql_query($query) or die(mysql_error()); header("Location: http://www.innonmainmanasquan.com/admin/index.php?section=$identity"); } } } } } ?>
-
Ok thanks
-
Thanks PFMaBiSmAdj thanks for the pointer I had it that way but I needed the nested if statements to test. Thanks thorpe I was just always told to you use addslashes() and stripslashes() when do mysql and it has always worked up until this point. And I wasn't "THINKING" that it wasnt working I knew it wasnt because special characters where being converted to question marks (?). So what is the reverse of the real_escape_string() will strip slashes still work to remove them? Thanks
-
Hi guys, I am working on this script and it doesn't seem to be adding slashes to the content before it inserts into the database and it is taking special characters like ' and changing them to ? can someone help me please? Here is my code <?php $dbhost = "***********"; $dbuser = "***********"; $dbpass = "***********"; $dbname = "innonmainnj"; $tblrooms = "rooms"; $tbllocations = "directions"; $tblhome = "home"; $tblaccommodations = "accommodations"; $tblreservations = "reservations"; $tblrestaurant = "restaurant"; mysql_connect($dbhost, $dbuser, $dbpass) or die(mysql_error()); mysql_select_db($dbname) or die(mysql_error()); $identity = $_POST['identity']; if($identity == "1"){ $description = addslashes($_POST['accommodations']); $query = "UPDATE accommodations SET column2='$description' WHERE id=1"; mysql_query($query) or die(mysql_error()); header("Location: http://www.innonmainmanasquan.com/admin/index.php?section=3"); }elseif($identity=="2"){ $content = addslashes($_POST['content']); echo $content; die(); $promotitle = addslashes($_POST['promotitle']); $promo = addslashes($_POST['promo']); $address1 = addslashes($_POST['address1']); $address2 = addslashes($_POST['address2']); $phone = addslashes($_POST['phone']); $query = "UPDATE `home` SET content='$content', promotitle='$promotitle', promo='$promo', address1='$address1', address2='$address2', phone='$phone' WHERE id= '1'"; mysql_query($query) or die(mysql_error()); header("Location: http://www.innonmainmanasquan.com/admin/index.php?section=1"); }elseif($identity=="3"){ $north = addslashes($_POST['north']); $south = addslashes($_POST['south']); $west = addslashes($_POST['west']); $query = "UPDATE `directions` SET north='$north', south='$south', west='$west WHERE id='1'"; mysql_query($query) or die(mysql_error()); header("Location: http://www.innonmainmanasquan.com/admin/index.php?section=3"); }elseif($identity=="4"){ $content = addslashes($_POST['description']); $phone1 = addslashes($_POST['phone1']); $phone2 = addslashes($_POST['phone2']); $email = addslashes($_POST['email']); $specialtitle = addslashes($_POST['specialtitle']); $special = addslashes($_POST['special']); $query = "UPDATE `reservations` SET content='$content', phone1='$phone1', phone2='$phone2', email='$email', specialtitle='$specialtitle', special='$special' WHERE id='1'"; mysql_query($query) or die(mysql_error()); header("Location: http://www.innonmainmanasquan.com/admin/index.php?section=4"); }elseif($identity=="5"){ $dinner = addslashes($_POST['dinner']); $lunch = addslashes($_POST['lunch']); $brunch = addslashes($_POST['brunch']); $query = "UPDATE `restaurant` SET dinner='$dinner', lunch='$lunch', brunch='$brunch' WHERE id='1'"; mysql_query($query) or die(mysql_error()); header("Location: http://www.innonmainmanasquan.com/admin/index.php?section=5"); }elseif($identity != 1){ if($identity != 2){ if($identy != 3){ if($identity != 4){ if($identity !=5 ){ $description = $_POST['description']; $cost = $_POST['cost']; $query = "UPDATE `rooms` SET description='$description' cost='$cost' WHERE roomnumber='$identity'"; mysql_query($query) or die(mysql_error()); header("Location: http://www.innonmainmanasquan.com/admin/index.php?section=$identity"); } } } } } ?> Thanks Again.
-
okay here is my code <?php $dbhost = "mysql.hmtotc.com"; $dbuser = "thegayestever"; $dbpass = "emit098nice054"; $dbname = "innonmainnj"; $tblrooms = "rooms"; $tbllocations = "directions"; $tblhome = "home"; $tblaccommodations = "accommodations"; $tblreservations = "reservations"; $tblrestaurant = "restaurant"; mysql_connect($dbhost, $dbuser, $dbpass) or die(mysql_error()); mysql_select_db($dbname) or die(mysql_error()); $indentity = $_POST['indentity']; if($indentity == "accommodations"){ $description = addslashes($_POST['accommodations']); $query = "UPDATE accommodations SET description='$description' WHERE id= '1'"; mysql_query($query) or die(mysql_error()); header("Location: http://www.innonmainmanasquan.com/admin/index.php?section=3"); }elseif($indentity=="home"){ $content = addslashes($_POST['content']); $promotitle = addslashes($_POST['promotitle']); $promo = addslashes($_POST['promo']); $address1 = addslashes($_POST['address1']); $address2 = addslashes($_POST['address2']); $phone = addslashes($_POST['phone']); $query = "UPDATE `home` SET content='$content', promotitle='$promotitle', promo='$promo', address1='$address1', address2='$address2', phone='$phone' WHERE id= '1'"; mysql_query($query) or die(mysql_error()); header("Location: http://www.innonmainmanasquan.com/admin/index.php?section=1"); }elseif($indentity=="locations"){<br /> $north = addslashes($_POST['north']); $south = addslashes($_POST['south']); $west = addslashes($_POST['west']); $query = "UPDATE `directions` SET north='$north', south='$south', west='$west WHERE id='1'"; mysql_query($query) or die(mysql_error()); header("Location: http://www.innonmainmanasquan.com/admin/index.php?section=3"); }elseif($indentity=="reservations"){ $content = addslashes($_POST['description']); $phone1 = addslashes($_POST['phone1']); $phone2 = addslashes($_POST['phone2']); $email = addslashes($_POST['email']); $specialtitle = addslashes($_POST['specialtitle']); $special = addslashes($_POST['special']); $query = "UPDATE `reservations` SET content='$content', phone1='$phone1', phone2='$phone2', email='$email', specialtitle='$specialtitle', special='$special' WHERE id='1'"; mysql_query($query) or die(mysql_error()); header("Location: http://www.innonmainmanasquan.com/admin/index.php?section=4"); }elseif($indentity=="restaurant"){ $dinner = addslashes($_POST['dinner']); $lunch = addslashes($_POST['lunch']); $brunch = addslashes($_POST['brunch']); $query = "UPDATE `restaurant` SET dinner='$dinner', lunch='$lunch', brunch='$brunch' WHERE id='1'"; mysql_query($query) or die(mysql_error()); header("Location: http://www.innonmainmanasquan.com/admin/index.php?section=5"); }elseif(is_numeric($identity)){ $description = $_POST['description']; $cost = $_POST['cost']; $query = "UPDATE `rooms` SET description='$description' cost='$cost' WHERE roomnumber='$identity'"; mysql_query($query) or die(mysql_error()); header("Location: http://www.innonmainmanasquan.com/admin/index.php?section=$identity"); } ?> whats happening is that it is not updating the fields, however it is taking symbols like ' that already exist in the table-collumn and changing it to a ? any ideas? Thanks
-
Ok still not sure what was wrong but now it is working, I will look into it and post the solution later. Thanks Guys you are all always such a great help!!
-
Yup it is
-
okay I have a form that is suppose to display contents retrieved from the database. However all the other forms work but this one. Could someone point me in the right direction please? home.php <?php $dbhost = "**********"; $dbuser = "*********"; $dbpass= "**********"; ?> <?php $dbtbl = "home"; $dbname = "innonmainnj"; ?> <?php $conn = mysql_connect($dbhost, $dbuser, $dbpass) or die(mysql_error()); ?> <?php mysql_select_db($dbname) or die(mysql_error()); ?> <?php $query = "SELECT * FROM `$dbtbl` WHERE id = '1'"; ?> <?php $result = mysql_query($query) or die(mysql_error()); ?> <?php if(mysql_num_rows($result) == 1){$returned = mysql_fetch_assoc($result);}else{echo "Table accommodations is not valid, please contact system administrator"; die();} ?> <div id="form_container"> <h1><a>Home Page</a></h1> <form id="form_108881" class="appnitro" method="post" action="proccess.form.php"> <div class="form_description"> <h2>Home Page</h2> <p>You are now editing your Home Page</p> </div> <ul > <li id="li_1" > <label class="description" for="element_1">Home Content </label> <div> <textarea id="element_1" name="element_1" class="element textarea medium"><?php echo stripslashes($returned['content']); ?></textarea> </div> </li> <li id="li_2" > <label class="description" for="element_2">Promo Title </label> <div> <input id="element_2" name="element_2" class="element text large" type="text" maxlength="255" value="<?php echo stripslashes($returned['promotitle']); ?>"/> </div> </li> <li id="li_3" > <label class="description" for="element_3">Promo </label> <div> <input id="element_3" name="element_3" class="element text large" type="text" maxlength="255" value="<?php echo stripslashes($returned['promo']); ?>"/> </div> </li> <li id="li_4" > <label class="description" for="element_4">Address Line 1 </label> <div> <input id="element_4" name="element_4" class="element text large" type="text" maxlength="255" value="<?php echo stripslashes($returned['address1']); ?>"/> </div> </li> <li id="li_5" > <label class="description" for="element_5">Address Line 2 </label> <div> <input id="element_5" name="element_5" class="element text large" type="text" maxlength="255" value="<?php echo stripslashes($returned['address2']); ?>"/> </div> </li> <li id="li_6" > <label class="description" for="element_6">Phone Number </label> <div> <input id="element_6" name="element_6" class="element text medium" type="text" maxlength="255" value="<?php echo stripslashes($returned['phone']); ?>"/> </div> </li> <li class="buttons"> <input type="hidden" name="form_id" value="108881" /> <input id="saveForm" class="button_text" type="submit" name="submit" value="Submit" /> </li> </ul> </form> <div id="footer"> inkControl CMS © <?php echo date(Y); ?> <a href="http://www.visualrealityink.com">Visual Reality ink.</a> </div> </div> Thanks
-
OH DEAR GOD I can't believe I missed that, thanks.
-
Ok I have my index.php file <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <meta http-equiv="Content-Type" content="text/html; charset=utf-8" /> <title>Untitled Document</title> <link rel="stylesheet" type="text/css" href="view.css" media="all"> </head> <body> <table id="content" align="center" width="750" height="100%" cellspacing="0" cellpadding="0"> <tr valign="top"> <td align="left" width="200" bgcolor="#FFFFFF"> <br /> <br /> <br /> <a href="index.php?section=home">Edit Home</a><br /><hr /> <a href="index.php?section=locations">Edit Locations</a><br /><hr /> <a href="index.php?section=accommodations">Edit Accommodations</a><br /><hr /> <a href="index.php?section=reservations">Edit Reservations</a><br /><hr /> <a href="index.php?section=restaurant">Edit Restaurant</a><br /><hr /> <a href="index.php?section=201">Edit Room 201</a><br /><hr /> <a href="index.php?section=202">Edit Room 202</a><br /><hr /> <a href="index.php?section=203">Edit Room 203</a><br /><hr /> <a href="index.php?section=204">Edit Room 204</a><br /><hr /> <a href="index.php?section=205">Edit Room 205</a><br /><hr /> <a href="index.php?section=206">Edit Room 206</a><br /><hr /> <a href="index.php?section=207">Edit Room 207</a><br /><hr /> <a href="index.php?section=301">Edit Room 301</a><br /><hr /> <a href="index.php?section=302">Edit Room 302</a><br /><hr /> <a href="index.php?section=303">Edit Room 303</a><br /><hr /> <a href="index.php?section=304">Edit Room 304</a><br /><hr /> <a href="index.php?section=305">Edit Room 305</a> </td> <td align="center"> <?php include('contcond.php'); ?> </td> </tr> </table> </body> </html> Then I have contcond.php <?php $section = "1"; if (isset($_GET['section'])) { $section = $_GET['section']; } if($section="home"){ include('home.php'); }elseif($section="locations"){ include('locations.php'); }elseif($section="accommodations"){ include('accommodations.php'); }elseif($section="reservations"){ include('reservations.php'); }elseif($section="restaurant"){ include('restaurant.php'); }elseif($section="201"){ include('rooms.php'); }elseif($section="202"){ include('rooms.php'); }elseif($section="203"){ include('rooms.php'); }elseif($section="204"){ include('rooms.php'); }elseif($section="205"){ include('rooms.php'); }elseif($section="206"){ include('rooms.php'); }elseif($section="207"){ include('rooms.php'); }elseif($section="301"){ include('rooms.php'); }elseif($section="302"){ include('rooms.php'); }elseif($section="303"){ include('rooms.php'); }elseif($section="304"){ include('rooms.php'); }elseif($section="305"){ include('rooms.php'); }else{ include('welcome.php'); } ?> Here is a link to it http://www.innonmainmanasquan.com/admin/index.php Its not reloading the pages based off of the section it is just going to home.php no matter what. This is driving me absolutly nuts because I have done this 100's of times before and it has never not worked. Can someone point me in the right direction to what the issue could be. Thanks
-
[SOLVED] Parse Error when combining HTML and PHP
TGWSE_GY replied to TGWSE_GY's topic in PHP Coding Help
Thanks BrickTop that was it man -
Ok I get this error: Parse error: syntax error, unexpected T_INCLUDE in /home/.valerieheater/jcnh74/innonmainmanasquan.com/dev/index.php on line 30 when I run this file can anyone tell me why? <html> <head> <title>Inn On Main Manasquan - A Unique Jersey Shore Bed And Breakfast</title> <META NAME="description" content="Inn On Main Manasquan NJ Bed and Breakfast Romantic Getaways Spring Lake New Jersey and lodging at the NJ shore."> <META NAME="KEYWORDS" CONTENT="manasquan bed and breakfast, spring lake bed and breakfast, manasquan nj lodging, spring lake nj lodging, manasquan new jersey, spring lake new jersey, manasquan, spring lake, new jersey, NJ, bed and breakfast, lodging, new jersey lodging, NJ lodging, NJ bed and breakfast, vacation, jersey shore, jersey vacation, inn, new jersey inn, jersey shore vacation, accommodations, new jersey travel, spring lake inn, new jersey inn, NJ inn, jersey coast bed and breakfast, jersey coast, romantic vacation, romantic getaway"> <meta http-equiv="Content-Type" content="text/html; charset=iso-8859-1"> <link href="style.css" rel="stylesheet" type="text/css"> <style type="text/css"> <!-- .style2 { font-size: 16px; font-weight: bold; } .style3 { font-size: 14px; font-weight: bold; } .style4 {color: #003366} .style5 { color: #003399; font-weight: bold; } --> </style> </head> <body bgcolor="#FFFFFF" leftmargin="0" topmargin="0" marginwidth="0" marginheight="0"> <?php $page = "home"; include('get.info.php'); <--------------This Line here! ?> <div class="main"> <table width="100%" border="0" cellspacing="0" cellpadding="0"> <tr> <td align="center"> <table id="Table_01" width="762" height="551" border="0" cellpadding="0" cellspacing="0"> <tr> <td> <a href="index.php"><img src="images/Manasquan-Inn-Logo.gif" width="412" height="54" alt="home" border="0"></a></td> <td colspan="4" background="images/Jersey-Shore-Nav-Background.jpg" valign="middle"><div class="nav"><a href="location-north.php">Location</a> | <a href="accommodation.php">Rooms</a> | <a href="reservation.php">Reservations</a></div></td> </tr> <tr> <td colspan="5"> <img src="images/Jersey-Bed-Breakfast-Establ.gif" width="762" height="25" alt=""></td> </tr> <tr> <td colspan="5" width="762" height="259" valign="top" background="images/innonmain_center_bed.jpg"><div class="center"><strong>The Inn On Main</strong>: <?php echo $retured['content']; ?> <br> <span class="style5">Summer Bailout Promotion</span> - Stay Saturday & Sunday, Get 30% off Monday! <em>Subject to availability</em> <br> </div><div class="center3"><span class="style2">Inn On Main</span><br> 152 Main Street<br>Manasquan, New Jersey<br> <span class="style3">(732) 528-0099</span></div></td> </tr> <tr> <td colspan="5"> <img src="images/Jersey-Shore-Inn-Bottom-Sli.gif" width="762" height="25" alt=""></td> </tr> <tr> <td colspan="2" background="images/Bottom-Left.gif" width="762" height="187" valign="top"><div class="bottom"> <p><img src="images/fruit_plate_mixx.jpg" width="142" height="106" hspace="10" vspace="5" border="1" align="left"><strong>Mixx American Bistro </strong><br> Enjoy Mixx with its menu of eclectic dishes…or traditional American fare, made interesting. You will find yourself wanting more, frequenting it like the locals do. Chef Tricia Tracey shops local farms for ingredients, imparting that Jersey flavor to the pot. For reservations, call 732.292.9292.<br> <br> <br> <a href="restaurant.php">Click Here for More Info »</a> </p> </div></td> <td colspan="3" background="images/Bed-Breakfast-Manasquan.gif" width="289" height="187" valign="top"><div class="bottom"> <p><img src="images/Inn-On-Main-Bed.jpg" width="130" height="106" hspace="10" vspace="5" align="left" class="packpic"><strong>Our Rooms</strong><br> Spacious bed and bath areas. Plantation-style ceiling fans buzz above. Notch-up the evening from our lamp selection or the glow of the fireplace.<br> <a href="inn-packages.php">Click Here for Info »</a></p> </div></td> </tr> <tr> <td> <img src="images/spacer.gif" width="412" height="1" alt=""></td> <td> <img src="images/spacer.gif" width="61" height="1" alt=""></td> <td> <img src="images/spacer.gif" width="34" height="1" alt=""></td> <td> <img src="images/spacer.gif" width="136" height="1" alt=""></td> <td> <img src="images/spacer.gif" width="119" height="1" alt=""></td> </tr> </table> <table width="100%" border="0" cellspacing="0" cellpadding="5"> <tr> <td align="center"><span class="style4">Inn On Main - 152 Main Street - Manasquan, NJ - (732) 528-0099 - </span><a href="mailto:info@innonmainmanasquan.com"><span class="style4">info@innonmainmanasquan.com</span></a><br> <br><a href="http://www.tripadvisor.com/"><img src="http://www.tripadvisor.com/img/triplogo162w.gif" border="0" alt="TripAdvisor.com"></a> <span class="bottom"><br> Read unbiased opinions about <a href="http://www.tripadvisor.com/">hotels</a> and <a href="http://www.tripadvisor.com/">vacations</a> at TripAdvisor.</span> <br> <img src='http://counter.dreamhost.com/cgi-bin/Count.cgi?df=jcnh74-countername.dat&pad=F&ft=0&dd=C&istrip=T'></td> </tr> </table> </td> </tr> </table> </div> </body> </html>
-
HAHAHAHAHAHAHAHAHAHAHAHAHAHAHAHHAHA there was a space before my <?php tag. Thanks
-
Warning: Cannot modify header information - headers already sent by (output started at /home/.valerieheater/jcnh74/soundreview.net/admin/classes/forms/process_changes.php:1) in /home/.valerieheater/jcnh74/soundreview.net/admin/classes/forms/process_changes.php on line 60
-
Ok there is only one place in my script that I use headers, every time it reaches the header statement I get an error that the headers are already sent. <?php //This script will process the updates to the article that is designated by the sectionid passed from the previouse php page in the form. //Then we will take this and store it into the correct table of the database. Remember we always call our database configuration at the start. //Get database connectiong config include('phpcontent/config.php'); $id = $_POST['id']; $date = date(mdo); /************************************************************EDIT LINES STARTING HERE**************************************************/ $tblnews = "sndreview_news"; $tblreviews = "sndreview_reviews"; $tblfeatured = "sndreview_featured"; $tblindustry = "sndreview_industry"; /************************************************************STOP EDITING HERE ********************************************************/ //Get the entries from the form. $section = $_POST['section']; $new_title = addslashes($_POST['title']); $new_article = $_POST['article']; $summary = addslashes($_POST['summary']); $search = "src=\"http://www.soundreview.net/images/"; $replace = "src=\"images/"; $title = str_replace($search, $replace, $new_title); $article = str_replace($search, $replace, $new_article); $article = addslashes($article); switch ($section) { case 0: //News mysql_query("UPDATE `$tblnews` SET title='$title', article='$article', summary='$summary', date='$date' WHERE id='$id'") or die(mysql_error()); $sectionid = "news"; break; case 1: //Industry $tbl = $tblindustry; mysql_query("INSERT INTO `$tblindustry` ( title , article , summary , date ) VALUES ('$title', '$article', '$summary', '$date')") or die(mysql_error()); $sectionid = "radio"; break; case 2: //Reviews $tbl = $tblreviews; mysql_query("INSERT INTO `$tblreviews` ( title , article , summary , date ) VALUES ('$title', '$article', '$summary', '$date')") or die(mysql_error()); $sectionid = "reviews"; break; case 3: //Featured $tbl = $tblfeatured; mysql_query("INSERT INTO `$tblfeatured` ( featured , date ) VALUES ('$article', '$date')") or die(mysql_error()); $sectionid = "featured"; break; case 4: $tbl = "sndreview_photos"; mysql_query("INSERT INTO `$tbl` ( article , date ) VALUE ('$article', '$date')") or die(mysql_error()); break; } header("Location: http://www.soundreview.net/admin/classes/forms/maineditor.php?section=$sectionid"); ?> Is there anyway to test if the header has already been sent and the clear it and send the new one? This same exact code works on several other domains without fail. And YES I AM SURE! that the headers are not being sent anywhere else in my script. Thanks
-
Ok removed the or die, and I have checked, checked again, and rechecked again and the data is in the data base is absolutly correct. so why is the second record being repeated? I am absolutely sure that it is all right.
-
[SOLVED] UPDATE Statement not working but returning no errors
TGWSE_GY replied to TGWSE_GY's topic in MySQL Help
onedumbcoder was right, it just needed to be rewritten IDK what the problem was possibly something in cache. Thanks guys! -
Ok guys this one is a strange one. I know that this is not a mysql issue because mysql is not outputting any errors. I believe it is in the while loop, however I have never seen a while loop act like this. here is a link to the page http://www.jamsmagazine.com/index2.php?section=news and here is my code <p><img src="images/jams-magazine-news.gif" width="150" height="20"></p> <?php //Get database connectiong config include('config.php'); $query = "SELECT * FROM jamsmag_news ORDER BY id ASC LIMIT 4"; $result = mysql_query($query) or die(mysql_error()); //Get article from db $count = "0"; while ($row = mysql_fetch_assoc($result) or die(mysql_error())) { $title = $row['title']; $article = $row['article']; echo $title; echo $article; echo $count; $count = $count + 1; } ?> Now it is repeating the second news article "No more Gorillaz studio albums" is being repeated. whats more the first article is not displaying the counter echoed. What is causing this odd behavior any ideas, there are only 4 records in the news table so this makes no sense. Thanks :facewall: :facewall: