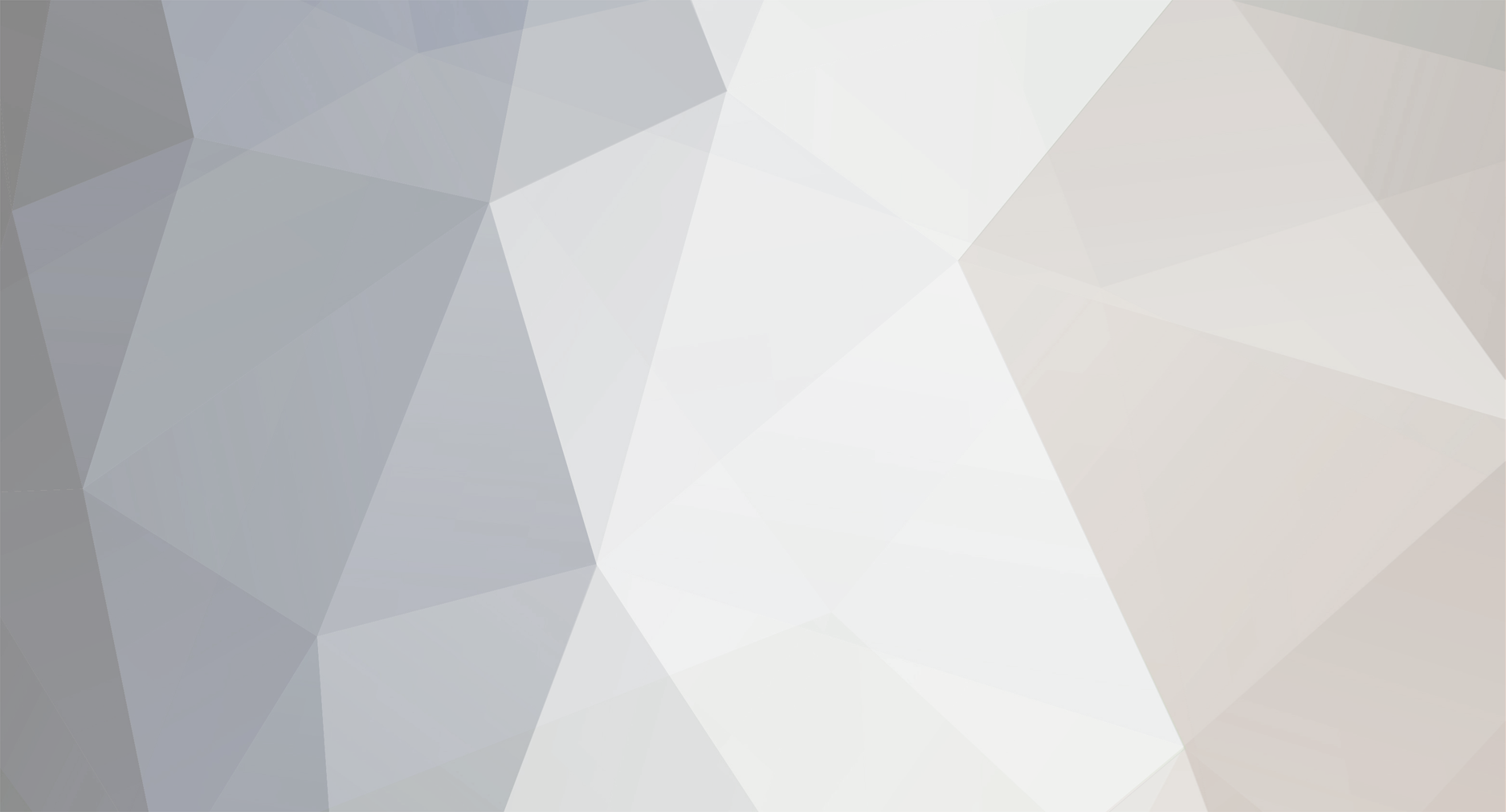
stevieontario
Members-
Posts
108 -
Joined
-
Last visited
Everything posted by stevieontario
-
Hello Freaks, Much as I love puzzles, this one has me stumped. I'm trying to loop through this array: [Machines] => Array ( [Machine] => Array ( [0] => Array ( [MachineName] => Machine1 [Type] => STAMPER [Outputs] => Array ( [Output] => Array ( [0] => Array ( [Hour] => 1 [Widgets] => 55 ) [1] => Array ( [Hour] => 2 [Widgets] => 54 ) ) ) [Capabilities] => Array ( [Capability] => Array ( [0] => Array ( [Hour] => 1 [Widgets] => 100 ) [1] => Array ( [Hour] => 2 [Widgets] => 100 ) ) ) I want to echo Machine1's output and capability for each of the two hours shown, each hour on the same line, like so: MachineName Type Hour Output Capability Machine1 STAMPER 1 55 100 Machine1 STAMPER 2 54 100 I have written a php script to do this, but so far I can't figure out how to grab both the outputs AND capabilities and put them on the same line. Here's what I've written: <?php foreach ($machine as $k=>$v){ if ($k == "MachineName"){ $unit = $v;} if ($k == "Type"){ $type = $v; } //begin grabbing outputs and capabilities// { if ($k == "Outputs"){ foreach($v as $outputs) { foreach($outputs as $k=>$v) { foreach ($v as $kk=>$vv){ if($kk=="Hour"){$hourout = $vv;} {if (array_key_exists("Widgets", $v)){$widgets = $vv;} else {$widgets = 0;}} } echo "<pre>"; echo "$unit $hourout $electricity"; echo "</pre>"; }}}//end of outputs loop// }} ?> This script can get the outputs, no problem. But going back in and getting the matching capabilities for that hour? I'm just stumped. Am I trying to do something impossible?
-
thanks again everyone. Barand, using isset() might just solve my problem. Newbie question (VERY newbie) though: can I mix object oriented syntax in with conventional? i.e. I have been denoting arrays using $k => $v syntax
-
thanks everyone. How about if a value is just empty? I neglected to view the source of the XML table. In source files that work, here's how the XML looks: <Output> <Hour>1</Hour> <Energy>0</Energy> </Output> ... and here's how it looks when I'm getting that error: <Output> <Hour>1</Hour> </Output> i.e., there is no Energy data. Not even a tag. Somehow, that translates into "N/A" on the web table. How do I handle this? My script is looking for some data in the Energy tag, but there isn't even an Energy tag.
-
sorry, should have said php does not know what to do when I tell it to divide "N/A" by some number.
-
Afternoon Freaks, I have a script that dies at this point: if ($a != 0) $ratio = $a/$b; My script is supposed to process a bunch of XML, almost all of which is numerical (digits). However, at one point in the XML file, the value that $a represents is "N/A". The script dies when it tries to execute line 128, i.e. at $ratio = $a/b; The error message reads: "Fatal error: Unsupported operand types in /[path/file.php] on line 128" My layman's guess is that php doesn't know what to do when I tell it to divide some number by "N/A". Any ideas?
-
Afternoon freaks, I'm trying to customize a free bit of calculator code I got from the web. There's a regex in it that appears designed to prevent users from entering anything but digits in the input fields. However, it does allow "garbage" in, e.g. 55f x 45e = 2475 How can I redo the regex so that it only allows digits and one decimal? The regex uses eregi, which the PHP manual says has been deprecated. I don't think that's the main issue though. The code containing the regex is here: if(!eregi("[0-9]", $number1)){ echo("Number 1 MUST be numbers!"); exit; ... and this is the entire code: <?php // Calculator Script v1 // Copyright (C) 2007 RageD // Define to make this all one document $page = $_GET['page']; // Defining the "calc" class class calc { var $number1; var $number2; function add($number1,$number2) { $result =$number1 + $number2; echo("The sum of $number1 and $number2 is $result<br><br>"); echo("$number1 + $number2 = $result"); exit; } function subtract($number1,$number2) { $result =$number1 - $number2; echo("The difference of $number1 and $number2 is $result<br><br>"); echo("$number1 - $number2 = $result"); exit; } function divide($number1,$number2) { $result =$number1 / $number2; echo("$number1 divided by $number2 is $result<br><br>"); echo("$number1 ÷ $number2 = $result"); exit; } function multiply($number1,$number2) { $result =$number1 * $number2; echo("The product of $number1 and $number2 is $result<br><br>"); echo("$number1 x $number2 = $result"); exit; } } $calc = new calc(); ?> <TITLE>PHP Calculator v1</TITLE> <form name="calc" action="?page=calc" method="POST"> Number 1: <input type=text name=value1><br> Number 2: <input type=text name=value2><br> Operation: <input type=radio name=oper value="add">Addition <input type=radio name=oper value="subtract">Subtraction <input type=radio name=oper value="divide">Division <input type=radio name=oper value="multiply">Multiplication</input><br> <input type=submit value="Calculate"> </form> <?php if($page == "calc"){ $number1 = $_POST['value1']; $number2 = $_POST['value2']; $oper = $_POST['oper']; if(!$number1){ echo("You must enter number 1!"); exit; } if(!$number2){ echo("You must enter number 2!"); exit; } if(!$oper){ echo("You must select an operation to do with the numbers!"); exit; } if(!eregi("[0-9]", $number1)){ echo("Number 1 MUST be numbers!"); exit; } if(!eregi("[0-9]", $number2)){ echo("Number 2 MUST be numbers!"); exit; } if($oper == "add"){ $calc->add($number1,$number2); } if($oper == "subtract"){ $calc->subtract($number1,$number2); } if($oper == "divide"){ $calc->divide($number1,$number2); } if($oper == "multiply"){ $calc->multiply($number1,$number2); } } ?> I tried amending the regex inside the quotes to this: [\d.] ... but no dice.
-
FOR loop keeps iterating over last item
stevieontario replied to stevieontario's topic in PHP Coding Help
thanks everyone -- yes I wrote the loop so it would endlessly repeat. I've rewritten, everything is good. Thanks again! -
FOR loop keeps iterating over last item
stevieontario replied to stevieontario's topic in PHP Coding Help
... and how I became an "advanced member" is beyond me -- unless I automatically got promoted due to seniority (I think I joined this group a few years ago)... -
Morning Freaks, I can't get the following For loop to stop iterating over the final item. As you can see, I want to put the results of a select MySQL query into another table in the same database. <?php for ($i=1000; $i >= 1000; $i++) $sql = "SELECT columnB, columnC from table1 where columnA = '$i'"; $result = mysql_query($sql, $cxn) or die (mysql_error()); while ( $row = mysql_fetch_assoc($result)){ extract($row); } $sqlIns = "insert into table2 (columnB,columnC) values ('$columnB', '$columnC' )"; $t1query = mysql_query($sqlIns); error_reporting(E_ALL); } mysql_close($cxn); ?> columnA is table1's id field, auto-increment. Table1 has 2000 rows; so the maximum value of columnA is 2000. What happens is the loop just keeps executing, and adding row upon row of the columnB and columnC values where columnA = 2000. I have to kill the darn query to get out of it. I suspect I should be using a break command somewhere, but where?
-
yes, read it and implemented it and it worked. Thanks very much. However, I have discovered a new problem -- more accurately, clarified my original problem. And that is my HTML table definition. It does not appear to allow for the addition of more than one row of data. I want six rows. I suspect I'll need to write a php function that adds HTML table rows dynamically, which also means I need to learn more about HTML tables. Back to my reading!
-
mj, thanks -- however please accept my humble apology for having led you astray by omitting a line of code in my original post. Cut and paste sloppiness on my part. The line is "$foutput = number_format($output);" -- it should have appeared just before the block of code about which you said "Not sure what this block is for since none of these variables are used." Hopefully my adding it will explain what that code block is for. Here's how the original code should have looked: </head> <table border="1"> <tr> <th>Fuel type</th> <th>Number of units</th> <th>Output</th> <th>Capability factor</th> <th>Pollution, in tons<sub>2</sub> emitted</th> </tr> <?php $db_hostname = "localhost"; $db_name = "dbname"; $db_username = "joeblow"; $db_password = "password"; $cxn = mysql_connect($db_hostname,$db_username,$db_password) or die ("Could not connect: " . mysql_error()); mysql_select_db($db_name); $sql = "SELECT output, fuel, numunits, capabilityfactor, pollution FROM db1 GROUP BY fuel ORDER BY sum(output) DESC"; $result = mysql_query($sql, $cxn) or die ("sorry, try again"); while ( $row = mysql_fetch_assoc($result)) { extract($row); $foutput = number_format($output); $capfactor = number_format($capabilityfactor,2)*100; if ($fuel == "fuel1") $fuel1_total = $output; if ($fuel == "fuel2") {$fuel2_total = $output; $fuel2_pollution = $pollution;} if ($fuel == "fuel3") {$fuel3_total = $output; $fuel3_pollution = $pollution;} if ($fuel == "fuel4") $fuel4_total = $output; if ($fuel == "fuel5") $fuel5_total = $output; if ($fuel == "fuel6") {$fuel6_total = $output; $fuel6_pollution = $pollution;} $waste = number_format($pollution); } ?> <tr> <td><?php echo $fuel; ?></td> <td><?php echo $numunits; ?></td> <td><?php echo $foutput; ?></td> <td><?php echo $capfactor; ?></td> <td><?php echo $waste; ?></td> </tr> </table> <body> </body> </html>
-
Afternoon Freaks, I'm trying to display the results of a MySQL query in an HTML table, unsuccessfully. The query returns six rows, but my HTML table only returns one (besides the header row). Here's the code: </head> <table border="1"> <tr> <th>Fuel type</th> <th>Number of units</th> <th>Output</th> <th>Capability factor</th> <th>Pollution, in tons<sub>2</sub> emitted</th> </tr> <?php $db_hostname = "localhost"; $db_name = "dbname"; $db_username = "joeblow"; $db_password = "password"; $cxn = mysql_connect($db_hostname,$db_username,$db_password) or die ("Could not connect: " . mysql_error()); mysql_select_db($db_name); $sql = "SELECT output, fuel, numunits, capabilityfactor, pollution FROM db1 GROUP BY fuel ORDER BY sum(output) DESC"; $result = mysql_query($sql, $cxn) or die ("sorry, try again"); while ( $row = mysql_fetch_assoc($result)) { extract($row); $capfactor = number_format($capabilityfactor,2)*100; if ($fuel == "fuel1") $fuel1_total = $output; if ($fuel == "fuel2") {$fuel2_total = $output; $fuel2_pollution = $pollution;} if ($fuel == "fuel3") {$fuel3_total = $output; $fuel3_pollution = $pollution;} if ($fuel == "fuel4") $fuel4_total = $output; if ($fuel == "fuel5") $fuel5_total = $output; if ($fuel == "fuel6") {$fuel6_total = $output; $fuel6_pollution = $pollution;} $waste = number_format($pollution); } ?> <tr> <td><?php echo $fuel; ?></td> <td><?php echo $numunits; ?></td> <td><?php echo $foutput; ?></td> <td><?php echo $capfactor; ?></td> <td><?php echo $waste; ?></td> </tr> </table> <body> </body> </html> What I get is the html table with the right header row but the only data row is the one corresponding to fuel6. Something tells me the problem is with the positioning of the PHP tags, but I've tried all sorts of combinations and they usually produce an error that indicates I'm missing a curly bracket or the ?> tag. I'm stumped. Anybody have an idea what I'm doing wrong?
-
array structure problem -- probably elementary
stevieontario replied to stevieontario's topic in PHP Coding Help
You see, my problem is I have no idea what I'm doing. I got stuck on the subquery (for whatever reason) and that was what had me hung up. So I took it out and grouped by var1 and PRESTO! Problem solved! Thanks everyone! -
array structure problem -- probably elementary
stevieontario replied to stevieontario's topic in PHP Coding Help
Zanus, thanks. I'll study your suggestion, though I don't see the difference between the result I get from my extract and your first statement. You correctly assumed that var1 is an auto-incremented field, and I was thinking along the lines of your foreach statement. Let me fiddle around with it and see what happens. Abra, thanks as well. I tried using a subquery, but it wouldn't let me get more than one group of var1s ("subquery returns more than one row"). -
Afternoon Freaks, I'm trying to add up certain variables inside an array, and keep going around in circles. The array is the result of a mysql SELECT query. I select the contents of a database table, then extract them in php using the following code: while ( $row = mysql_fetch_assoc($result)) { extract($row); } var_dump($row) outputs the following: What I want is to add up all the var2 values for each var1. e.g., in the case of var1=1193, this would be 799 + 725+ 818. Of course, there are nearly 2,000 var1s (each one with multiple var2s), and I want my computer to do all the work. So I'm trying to isolate each var1 so I can figure out some loop to do this operation. I've tried a bunch of funny little tricks with min(), but keep going in circles. Any ideas?
-
"INSERT command denied to user" -- two connections to two DBs
stevieontario replied to stevieontario's topic in MySQL Help
Well, every time I decide to avoid learning critical subjects (like MySQL access rights), those same issues catch me down the road. Thanks everybody for your help, and I'll read up/practice the mysqli thing. Steve -
"INSERT command denied to user" -- two connections to two DBs
stevieontario replied to stevieontario's topic in MySQL Help
okay, I just managed to get the script to stop giving me that error: I put the full name of db2 into the query $query2. So, instead of $query2 = "insert into db2.table1 (field1, field2, etc.) I put $query2 = "insert into mainuser_db2.table1 (field1, field2, etc.) and presto -- data is inserted, no fatal error. I don't know why that worked, since I did not give the full DB name of db1 for the SELECT query ($query1). I thought that since I used the same $db_hostname for both connections I wouldn't have to get particular about the DB names. -
"INSERT command denied to user" -- two connections to two DBs
stevieontario replied to stevieontario's topic in MySQL Help
Pika -- when I first login to the host's cPanel and load phpMyAdmin, localhost is the first item that appears over the tabs ("Databases", "SQL", "Status", etc.) in the main window. -
"INSERT command denied to user" -- two connections to two DBs
stevieontario replied to stevieontario's topic in MySQL Help
okay, so by "dump" you meant "display." I thought you meant get rid of them! It's purely bizarre. From the top of the tree (i.e., at the localhost level), it says I have all privileges to the DB in question. But from the level of the DB in question, I can't even do the SHOW GRANTS -- access denied! -
"INSERT command denied to user" -- two connections to two DBs
stevieontario replied to stevieontario's topic in MySQL Help
fenway, thanks. But what do you mean, dump the grants? I checked out on "the net" and couldn't find anything that didn't involve transferring DBs from one host to another. -
"INSERT command denied to user" -- two connections to two DBs
stevieontario replied to stevieontario's topic in MySQL Help
thanks Juddster. Well there are definitely user access issues -- legitimate or not is hard to say at this time. I thought that as the account owner I had global access privileges, but that's obviously not the case. Weird, because I can, from the host's cPanel, create new DBs and grant access. But then I can't insert to that table, even from the host's phpMyAdmin. Pika, don't know if that answers your 2nd question. From phpMyAdmin, I clicked on the relevant DB then tried an insert query. Access denied but this time to the global user (who I thought was me). -
"INSERT command denied to user" -- two connections to two DBs
stevieontario replied to stevieontario's topic in MySQL Help
Pikachu2000, thanks. When I contacted the host they took my username and password, and put data into the table just to test. I saw what they inserted, and assumed that proves that the user has all the privileges. And now as I tried the same thing from the host's phpMyAdmin, I am still being denied access. I'll check again. Again, thanks, -
Morning Freaks, PHP Version 5.2.15 MySql server version 5.1.54-log MySQL client version: 5.1.54 I'm trying to run a query (from php) which involves two DBs and (I believe) two connections. I cannot get past this error when I launch the script: Here's the code: $db_hostname = "localhost"; $db_name = "db1"; $db_username = "user1"; $db_password = "password1"; $cxn = mysql_connect($db_hostname,$db_username,$db_password, true) or die ("Could not connect: " . mysql_error()); mysql_select_db($db_name, $cxn); $db_name2 = "db2"; $db_username2 = "user2"; $db_password2 = "password2"; $cxn2 = mysql_connect($db_hostname,$db_username2,$db_password2, true) or die ("Could not connect: " . mysql_error()); mysql_select_db($db_name2, $cxn2); $query1 = "SELECT sourceid as id2, carname, fuel, output, capability, capabilityfactor, generated, version as hour FROM performance, sourceinfo where performance.sourceid=sourceinfo.id and fuel like 'gasoline%'"; $result = mysql_query($query1, $cxn) or die("<b>A fatal MySQL error occured</b>.\n<br />Query: " . $query1 . "<br />\nError: (" . mysql_errno() . ") " . mysql_error()); while ( $row = mysql_fetch_assoc($result)) { extract($row); $query2 = "insert into db2.table1 (id2, carname, fuel, output, capability, capabilityfactor, generated, hour) values ('$id2', '$carname', '$fuel', '$output', '$capability', '$capabilityfactor', '$generated', '$hour')"; $result1 = mysql_query($query2, $cxn2) or die("<b>A fatal MySQL error occured</b>.\n<br />Query: " . $query2 . "<br />\nError: (" . mysql_errno() . ") " . mysql_error()); } I set the fourth parameters in the mysql_connect functions because that's what "sources" -- books and online info -- said to do in the case of multiple connections. I also checked with my host -- they tell me I have all the privileges to those DBs/tables. Any thoughts on how/why I'm screwing this up? Thanks,
-
Evening Freaks, I am trying to identify gaps in a column that holds hourly versions of files -- i.e., this column contains only numbers between 1 and 24. Rows are added to this table every hour, so that this column -- called Version -- would, if this were a perfect world, always contain repeating sequences of 24 rows. The Version column would always contain numbers in sequence, 1 to 24, and repeating until the end of time. Of course in the real world, rows sometimes don't get added. I want to find the instances where there is a gap, and then manually add the rows. Any idea on what kind of query would help me find these gaps? Thanks in advance, Steve