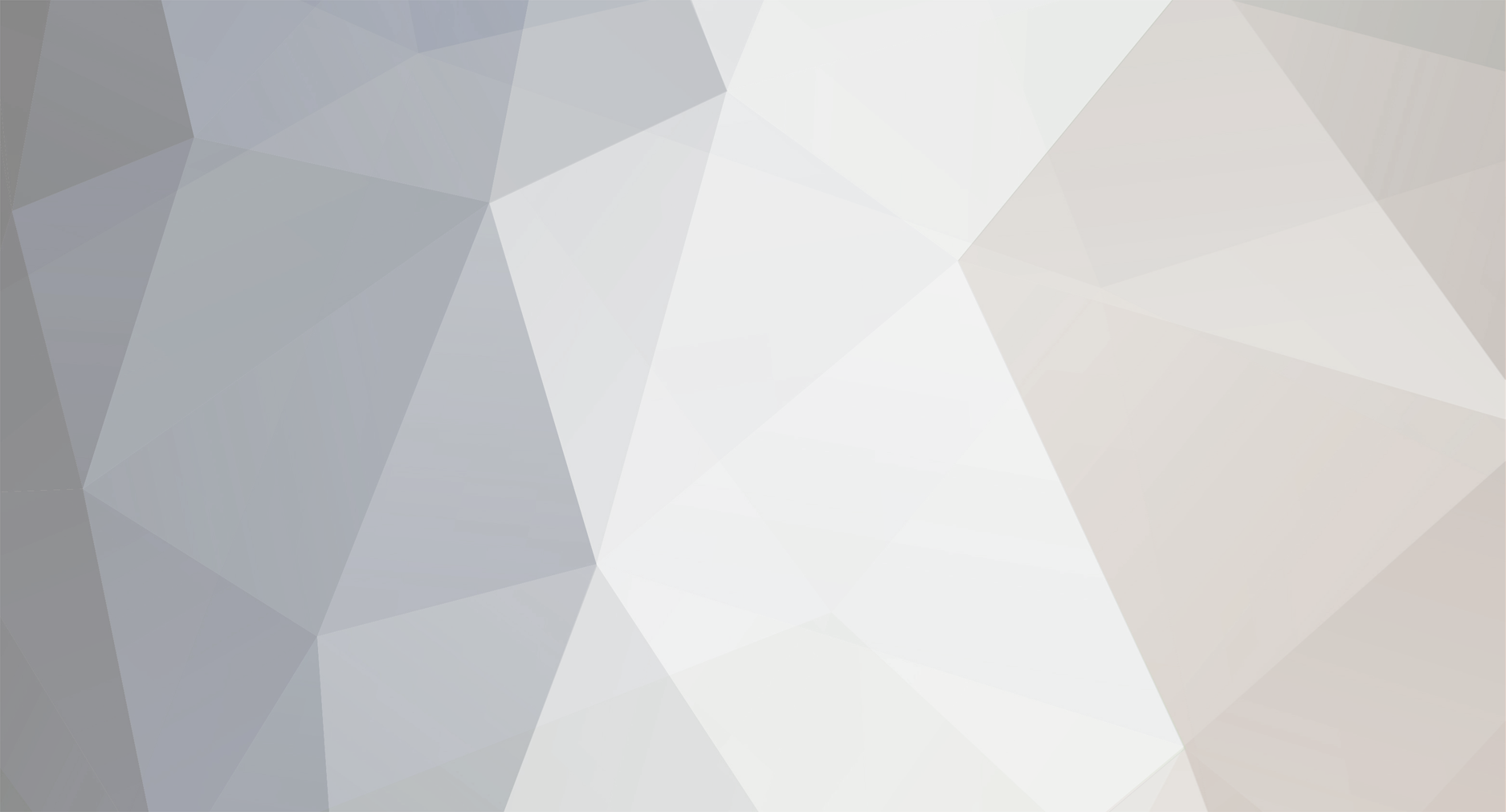
w3evolutions
Members-
Posts
39 -
Joined
-
Last visited
Never
Everything posted by w3evolutions
-
You can also use Apache mod_re-write to accomplish this.
-
There is a lot that can go into creating your own custom E-commerce application. There are a bunch out there that you can use as pre-built applications. OSCommerce, Zend cart, X-cart... There are also tutorials http://www.phpwebcommerce.com/. I would take a browse around google.
-
Try making your code a little more structured, it helps to read. Also, mysql_real_escape_string() on $_POST vars. foreach($_POST as $key=>$value) { $_POST[$key] = mysql_real_escape_string($value); } $school = $_POST['school']; $room = $_POST['room']; $operatingsystem = $_POST['operatingsystem']; $project = $_POST['projector']; $whiteboard = $_POST['whiteboard']; if($school == "ALL") { $where_school = ""; $school_count = "0"; } else { $where_school = " WHERE `school` = '$school'"; $school_count = "1"; } if($room == "ALL") { $where_room = ""; $room_count = "0"; } else { if($school_count == "0") { $where_room = " WHERE `room` = '$room'"; } else { $where_room = " AND `room` = '$room'"; } $room_count = "1"; } if($project == "ALL") { $where_project = ""; $project_count = "0"; } else { if($school_count == "0" && $room_count == "0") { $where_project = " WHERE `projector` = '$project'"; } else { $where_project = " AND `projector` = '$project'"; } $project_count = "1"; } if($whiteboard == "ALL") { $where_whiteboard = ""; $whiteboard_count = "0"; } else { if($school_count == "0" && $room_count == "0" && $project_count == "0") { $where_whiteboard = " WHERE `whiteboard` = '$whiteboard'"; } else { $where_whiteboard = " AND `whiteboard` = '$whiteboard'"; } $whiteboard_count = "1"; } if($operatingsystem == "ALL") { $where_operatingsystem = ""; $operatingsystem_count = "0"; } else { if($school_count == "0" && $room_count == "0" && $project_count == "0" && $whiteboard_count == "0") { $where_operatingsystem = " WHERE (`operatingsystem`= '$operatingsystem' OR 'operatingsystem2'= '$operatingsystem' OR" . " 'operatingsystem3'= '$operatingsystem' OR 'operatingsystem4'= '$operatingsystem' OR 'operatingsystem5'= '$operatingsystem'" . " OR 'operatingsystem6' = '$operatingsystem')"; } else { $where_operatingsystem = " AND (`operatingsystem`= '$operatingsystem' OR 'operatingsystem2'= '$operatingsystem' OR" . " 'operatingsystem3'= '$operatingsystem' OR 'operatingsystem4'= '$operatingsystem' OR 'operatingsystem5'= '$operatingsystem'". " OR 'operatingsystem6' = '$operatingsystem')"; } $operatingsystem_count = "1"; }
-
Can you post the rest of the code, and the outcome of the entire SQL statement when you do ones of these searches? Please.
-
If you are trying to upload to the same directroy or a derectory below the current php file you are in you can just use it's name: // Edit upload location here $result = 0; $target_path = getcwd()."/lgl-up/". basename( $_FILES['myfile']['name']); // This can also just be "lgl-up/" . basename( $_FILES['myfile']['name']); if(@move_uploaded_file($_FILES['myfile']['tmp_name'], $target_path)) { $result = 1; } sleep(1); try that.
-
Variables within variables - Quick Question
w3evolutions replied to Ifoundyoufondoo's topic in PHP Coding Help
I thought you were talking about a variable variable. Ex: $bar = "Hello"; $foo = "bar"; echo($$foo); // will display Hello What you are dealing with is simple array keys, and yes that is one way to do it as Ken2k7 stated. if you are using echo() to list the entire td element you can also do it this way: echo('<td class="cell" align="center" height="19">'.$row[$_SESSION['name']].'</td>'); same difference. -
session_register is being DEPRECATED soon so I would suggest just doing it the way you have in index.php. $_SESSION['myusername'] = $_POST['myusername']; Also this line on index.php $_SESSION['myusername']=$_POST['myusername']; tells php to reset the session variable. try this: if(isset($_POST)) && !empty($_POST['myusername'])) { $_SESSION['myusername']=$_POST['myusername']; }
-
Image resize proportionally and watermarking
w3evolutions replied to iceman023's topic in PHP Coding Help
There are many PHP libraries that can help you easily manipulate images with the GD library. I would suggest reading up on ImageMagick http://php.net/ImageMagick. -
The script you have will redirect to $MM_redirectLoginSuccess = "welcome to.php" if they are a valid user, or to $MM_redirectLoginSuccess = $_SESSION['PrevUrl'], which I'm assuming is the previous URL. You have to change the value of $MM_redirectLoginSuccess for each user based on who they login as. What are the page names for each user (you don't have to list them all just as an example)?
-
There are many times in programing where there is a need to have an object that creates other object or maybe there needs to be a set of instruction or data manipulation that needs to occur before an object gets created. This is called an object factory. Factory is a design pattern that controls the creation of other objects from one entry point. (not the formal definition, but mainly what it is used for.) Example: function do_some_work() { // Possibly manipulate some data, or connect to a database and retrieve information... .... } class ObjectFactory { public function create($classname="") { if(!empty($classname)) { // Do some work $work = do_some_work(); return new $classname($work); } } ....... } class Test { public function __construct($args="") { .... } ...... } $factory = new ObjectFactory(); $test = $factory->create("Test"); The issue with this object factory is that it is not generalized. It also will pass $work to ever object that it creates which might not be what is needed. By the nature of this object you can't create both static (Singleton), and instantiatable (using the reserved word new) objects. There are a few ways this can be accomplished. Example: function do_some_work() { // Possibly manipulate some data, or connect to a database and retrieve information... .... } class ObjectFactory { public static function create($classname="") { // Do some work $work = do_some_work(); if(!empty($classname)) { switch($classname) { case "Singleton": return Singleton::getInstance($work); break; case "Instantiable": return new Instantiable($work); break; ....... } } } ............. } class Singleton { public static function getInstance($args="") { .... } .... } class Instantiable { public function __construct($args="") { .... } } $factory = new ObjectFactory(); $test = $factory::create("Instantiable"); The issue with this class is first the ObjectFactory is an instantiable class itself, therefore taking up more memory and coding. Second, every time a new object needs to be created it must be placed into the switch() statement. Third, again it will pass $work to all of the objects it creates (or returns). On the other hand her is an example of a general Factory object. Example: abstract class ObjectFactory { public function create($classname="") { if(!empty($classname)) { $reflection = new ReflectionClass($classname); if($reflection->isInstantiable()) { return new $class_name($args); } else { return call_user_func(array($classname,"getInstance")); } } } ....... } class Test { public function __construct() { return; } public function sayHi($string="") { echo("Hi: ".$string); return; } } class TestSingleton { public static function getInstance() { ..... } public function sayHi($string="") { echo("Hi: ".$string); return; } } // Will print Hi: Have a nice day. // on the screen ObjectFactory::create("Test")->sayHi("Have a nice day."); // Uses the instantiable Object ObjectFactory::create("TestSingleton")->sayHi("again from a singleton."); // Uses the Singleton Object. This example uses PHP5 reflection class. A very handy class to reverse engineering object. It is also an abstract class which means it can not be instantiated. It allows for object chaining and leaves it to the returning class to "do work". by chaining ->sayHi("again from a singleton."); Lastly, it solves both cases of returning a new instance of an object that is instantiatable and the instance of a singleton object, and gives a standardized way of creating other objects. This is a brief overview of an abstract factory object.
-
Ken2k7 I don't understand what you mean by a singleton is not defined as a class? A singleton is an object so it has to have a class. All I'm saying is you don't have to save a singleton into a variable. Yes you have to call getInstance() every time, but you don't keep creating references to that singleton every time. Also the object registry mentioned by ionik is also a good example. I only use a registry to put objects and variables into a global scope, and since you can call the singleton object from anywhere in the application framework there is no need to place it into a registry, but you can if needed. If you use the method of calling the singleton as I have stated ionik there is no need to worry about references or repeating properties you will always only get the original object.
-
The wonderful & symbol or reference symbol. In order to understand what a reference is one first needs to question how PHP handles variables and their values. PHP variables and variable content are separate entities. You can have more than 1 variable point to the same content location. This is done via reference. Like so: $a = 0; $b =& $a; This means that both $a and $b point to the same content. This does NOT mean that $a points to $b or vice versa (php.net). This means that if I change $a, $b will also change and vice versa. Example: $a = 0; $b =& $a; $b = $b+1; // same as $a = $a+1; echo($a); // $a = 1; the best way to think of it is by drawing this diagram: This reflects that there are 2 different variable names, that point (the term point in php means reference) the same value. What is the real formal definition of a reference? References in PHP are a means to access the same variable content by different names (php.net). This means by creating a reference in PHP you effectively create another "name" that points to the same value. With this in mind lets take a look at PHP5's object model. PHP references are very useful in PHP OOP, or at least from the OOP I have done with PHP. References allow you to iterate though Arrays / Objects easier, help with recursion, allow you to follow good naming conventions and coding practices, and most of all save memory. Although creating a reference does create a new variable in memory and does consume memory it does NOT make a copy of the value. This can be good and bad. There are some instances where you want a copy of the object to be made, and there are instances where you do not. PHP5 automatically passes object by reference. This is good, as we don't (or usually don't) want a copy of the current object we are working with, we want the current instance. OK, I understand references but what is a Singleton? The term singleton is a design pattern. A design pattern in a example of a piece of code (or an object) that gives building block to solves or helps to solve a complex situations (help from wikipedia.org). The singleton design pattern states that the a singleton object can only ever be instantiated once, there can only ever be one running copy of this object. Why would I need this? In OOP there are times when you only need, or only want (more for security or memory purposes) to instantiate a class once. For instance, a database object should only ever be instantiated once. Even if you need to connect to different databases or read and write to a database you still only ever need one database connection. A well designed (and abstracted) database object(s) will allow you to connect to different types of databases no matter where you are in the system or what type of database you are connecting to, and it will allow you to make multiple connections, or queries when generating a page. So, now what do I do? The interesting thing about the singleton design pattern is there is only ever one instance of the object...... Doesn't that sound like a pointer? Not exactly but it can be used as one. For instance: Incorrect way! class Singleton { public static function getInstance() { .......... } public function sayHi() { echo("Hello there!"); return; } .......... } $newSingelton = Singelton::getInstance(); // BAD BAD BAD $newSingleton->sayHi(); ..... What you are actually doing here is creating an instance of the class Singleton and then creating a reference to the object you just created. WHAT!!???!! Remember PHP5 passes (returns) objects by reference. When you save a singleton object into a variable you create a reference to that value ... that is what a reference is and that is how PHP5 passes objects around. So what is the correct way? Correct Way!! class Singleton { public static function getInstance() { .......... } public function sayHi() { echo("Hello there!"); return; } .......... } Singleton::getInstance()->sayHi(); // CORRECT WAY !!! .... Now that is something interesting. Having singleton objects is a MUST in good application frameworks. It avoids a lot of confusion in debugging and saves a lot of memory (especially in large applications). Because the nature of the Singleton pattern there is no need to save the object into a variable as there is only ever one reference to the object itself, and when you call it's static getInstace() method it returns the object itself, this is where object chaining comes in handy. Effectively the two code examples above do exactly the same thing, the only difference is there is more overhead saving the object to a variable, and if your working with many objects, passing them to other objects, polymorphism, and other design patterns it's a good programming practice to have standardized coding, naming conventions, and to save memory. I hope you enjoyed this article!!