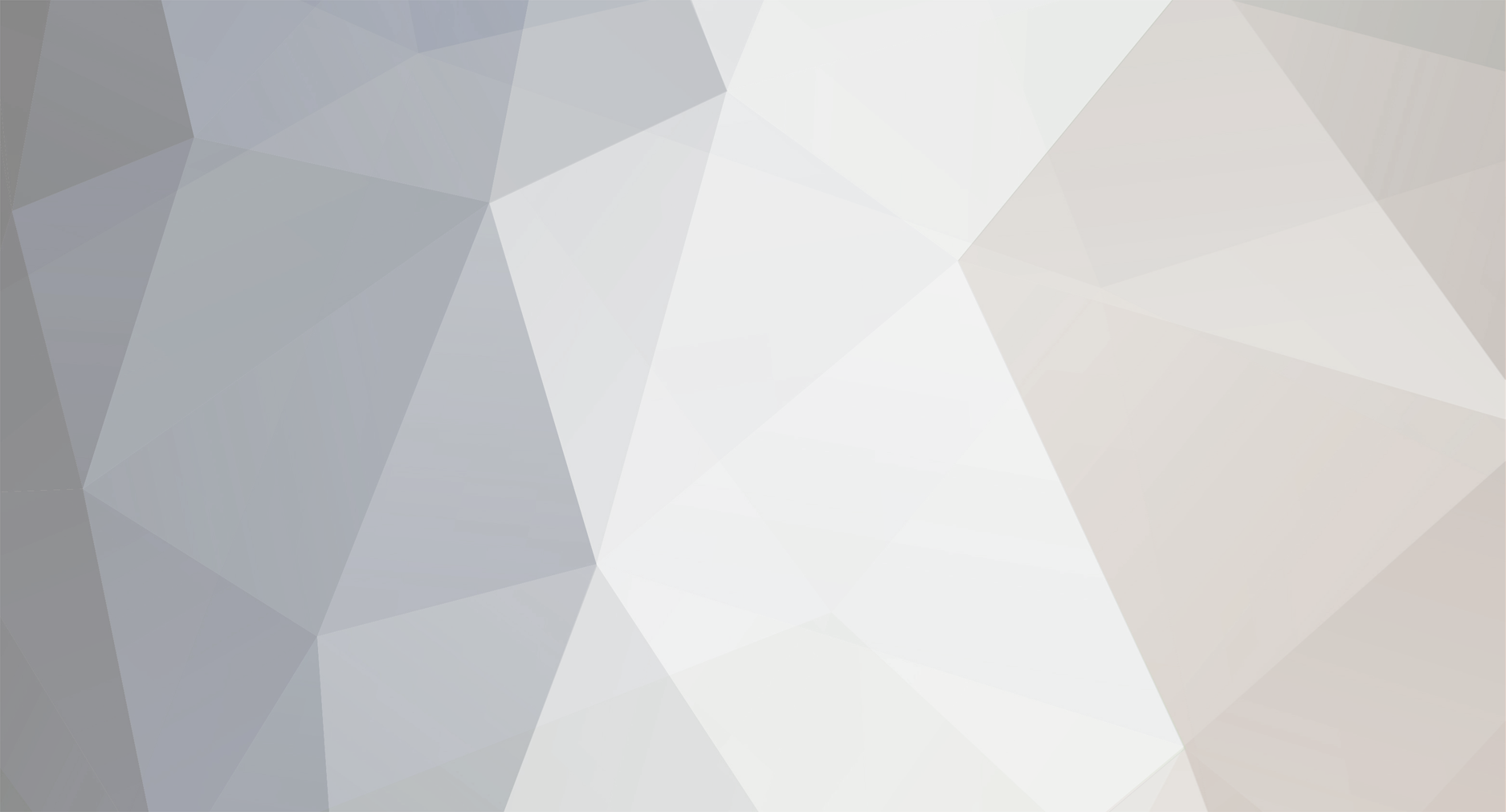
ReeceSayer
Members-
Posts
97 -
Joined
-
Last visited
Everything posted by ReeceSayer
-
Hi all, I'm just wondering if there's an easier way of doing what accomplishing the following: I have a value in my database which represents a selection in a drop down menu, i want to read it from the database and have it automatically selected depending on the stored data. I have the following working but just wondered if there was an easier way to get the same result: <?php //database connection $query = "SELECT id FROM `tablename` WHERE username='$username'"; $result = mysql_query($query); $row = mysql_fetch_object($result); $id = $row->id; $id = (int)$id; ?> <select name="id"> <option value="">Select your option...</option> <option value="1" <?php if (($id - 1) === 0) { echo 'selected="selected"'; }?>>Selection 1</option> <option value="2"<?php if (($id - 2) === 0) { echo 'selected="selected"'; }?>>Selection 2</option> </select> Sorry if it's not very clear, i'll explain best i can if anyone can help. Thanks
-
Creating Links & Including Variables Simple XML
ReeceSayer replied to ReeceSayer's topic in PHP Coding Help
Thanks a lot. The links will be valid as they'll point to the users profile id. I'll give it a try tomorrow when i'm back doing work. Thanks, Reece -
Hi guys, I'm currently in the process of making a simple social networking type site for a uni project and have basically everything i want except the ability to use AJAX to link to my profiles in the search bar. I have this code to generate my XML: <?php // create simplexml object $xml = new SimpleXMLElement('<rss version="2.0"></rss>'); // add channel information $xml->addChild('channel'); $xml->channel->addChild('title', 'The Social Network'); $xml->channel->addChild('link', 'http://reecesayer.com/projects/template/XML/news.rss'); $xml->channel->addChild('description', 'example'); // query database for article data include("phpfunctions.php"); db_connect(); $link = "www.reecesayer.com/projects/template/testprofile.php?id="; $select='SELECT email, id FROM users'; $result = mysql_query($select) or die(mysql_error()); while ($row = mysql_fetch_assoc($result)) { // add item element for each article $item = $xml->channel->addChild('item'); $item->addChild('email', $row['email']); $item->addChild('link', $row['id']); //I wanted to add a link before the $row['id'] } // save the xml to a file $xml->asXML('profiles.xml'); header ('Location: http://reecesayer.com/projects/template/XML/profiles.xml'); ?> I need to add a partial URL (http://reecesayer.com/projects/template/testprofile.php?id=) before the row is called so that when the email is searched it provides a link to the profile depending upon the id (http://reecesayer.com/projects/template/testprofile.php?id= Is there an easier way to do this or am i going the long way around? I'm only storing the data in XML as i saw a tutorial for a livesearch script on another site. Cheers, Reece
-
Issue updating database and redirecting
ReeceSayer replied to HDFilmMaker2112's topic in PHP Coding Help
This probably isn't right but i'll try and answer for the sake of trying to learn myself: this line: $update_approved_sql='UPDATE application SET approved="y" WHERE id="$id"'; //Change it to this $update_approved_sql="UPDATE application SET approved='y' WHERE id='$id'"; -
Operator value != when it seems to be ==
ReeceSayer replied to ReeceSayer's topic in PHP Coding Help
Ahhhh Sh*t sorry for wasting your time Not a clue why i haven't already noticed that. -
Hi, This ones stumped me a little as i've done this previously and it seemed to work fine: I have a form to add with two password boxes, the IDs are different as they should be but the type is the same: <form action="<?php echo $PHP_SELF;?>" method="post"> <p>New Password: <input type="password" id="newpassword" name="newpassword" /></p><br /> <p>Confirm Password: <input type="password" id="confirmpassword" name="confirmpassword" /></p><br /> <p><input type="submit" name="submit" value="submit"></p> </form> I have this code to check if they are both equal then md5 them and add them to the database: // Check if button name "submit" is active, do this if(isset($_POST['submit'])) { //variable to identify user $email = ($_SESSION['email']); //post variable from form $newpassword = $_POST['newpassword']; $password = $_POST['confirmpassword']; //if passwords are not equal if($password != $confirmpassword) { echo "The passwords do not match<br />"; //debugging - to see if they are equal or not - remove afterwards echo $password . "<br />"; echo $newpassword . "<br />"; } //if passwords are equal if($newpassword == $password) { //use md5 so they match when logging in $password = md5($password); $newpassword = md5($newpassword); $sql= "UPDATE users SET password='$newpassword' WHERE username='$email'"; $result = mysql_query($sql); if($result) { echo "Congratulations You have successfully changed your password"; } } } I've tried to explain my thinking as best i can in the code, the debugging part at the top shows that both values seem to be identical yet i'm being thrown into the not equal loop and they aren't adding to the database. Can anyone explain what i'm doing wrong here? I imagine its only minor but its thrown me. Cheers
-
Cheers, I'll give first one a go before attempting the zend solution as i've never used a framework before. Thanks again.
-
I'm still a learner too so this might not be right but: //enter into btree ---------------------------------------------- $sql_plevel='select nlevel from btree where uniqueid = '.$formjoinedunder; $abc = mysql_query($sql_plevel , $db) $result_plevel = mysql_fetch_assoc($abc) or die(mysql_error()); $plevel = $result_plevel['nlevel'] + 1; $insert_btree = 'INSERT INTO btree (uniqueid,nlevel,pside) VALUES ('$reguniqueid', '$plevel', '$formposition')'; mysql_query($insert_btree , $db) or die(mysql_error()); Not sure you needed the .$value. in the query? I've looked at some i've done before and they just have the values as they are above. Can always try it. Cheers
-
Yeah, it's definitely not the best, took them about 15mins to sort out the issue too. I've managed to sort out the code to write to an xml file: <?php $xml_shell = '<?xml version="1.0"?><?xml-stylesheet type="text/xsl" href="style.xlst"?><grandparent></grandparent>'; include("phpfunctions.php"); db_connect(); $xml_grandparent = new SimpleXMLElement( $xml_shell ); //select all from users table $select="SELECT title, link, description FROM news"; $result = mysql_query($select) or die(mysql_error()); while( $row = mysql_fetch_assoc($result) ) { $xml_parent = $xml_grandparent->addChild( 'parent' ); foreach( $row as $column => $value ) { $xml_parent->addChild( $column, $value ); } } header( 'Content-type: text/xml; charset=UTF-8' ); // write the xml out to a document fwrite( $xml_grandparent->asXML("news.xml")); ?> Just wondering how i'd do a properly formed RSS, i could replace grandparent with channel and parent with item... but still not sure how i'd go about getting it into an rss file. Cheers
-
Hi again, I've just been on the phone to hostgator tech support and they said that he had to do a file permissions re-write for my site (files: 644 folders:755), this solved the issue, i've seen quite a dew of these that seem to be unresolved on the forum so thought i'd post the solution. I have another problem with simpleXML but if i need help i'll post it as a new topic later. Reece EDIT: Sorry xyph i was writing this as you'd posted. Cheers
-
Apparently it's not solved. I went straight in made the changes and made put them live but i get an internal server error. So to double check i put the file in xampp and it works perfectly. So is there anything i need to turn on in my php config to allow me to do this? I've only had a live host for a couple of months so i'm not too sure. Cheers
-
I don't mind being wrong if i'm learning from it Edd, i think you might also need code at the top of those specific pages otherwise people could type the url and go straight to it. Something along these lines worked for me: session_start(); if(!isset($_SESSION['if']) || !isset($_SESSION['FirstName']) ||!isset($_SESSION['LastName']) || $_SESSION['type'] != 1) { header("Location: logout.php"); exit(); } Basically if the session type is not set to one then they shouldn't be on the page so it sends it back with the header. Sorry if i'm over complicating what you needed.
-
Brilliant cheers, I did google it but it just came up with a bunch of tutorials for basically what i'd already done. I'll mark as solved. Thanks
-
Hi, I've written some code to take information from an SQL database and write it out in the RSS format (Although it doesn't validate). The problem is i'd like the page to have the .rss (or .xml) file extension, I'm not sure if there's any advantages in having this but thought i'd ask. I've got the following code: <?php header('Content-type: text/xml'); print '<?xml version="1.0"?>'; print '<rss version="2.0">'; print '<channel>'; include("phpfunctions.php"); db_connect(); //select all from users table $select="SELECT title, link, description FROM news"; $result = mysql_query($select) or die(mysql_error()); //If nothing is returned display error no records if (mysql_num_rows($result) < 1) { die("No records"); } //loop through the results and write each as a new item while ($row = mysql_fetch_assoc($result)) { $item_title = $row["title"]; $item_link = $row["link"]; $item_desc = $row["description"]; print '<item>'; print '<title>' . $item_title . '</title>'; print '<link>' . $item_link . '</link>'; print '<description>' . $item_desc . '</description>'; print '</item>'; } print '</channel>'; print '</rss>'; ?> This seems to work fine as i get what i expect and i'm assuming i can do the same to output .xml but is there a way to have it in a proper .rss / .xml file so that an aggregator or someone could read this properly. Cheers, Reece
-
Never actually posted a solution on here before so please give me a break if i'm not right Firstly, as xyph said, add (if you don't already have one) a column to the table that stores the membership type (memberid). Then try something like this below: //Create query $qry="SELECT * FROM users WHERE username='$login' AND password='$EncryptedPassword'"; $result=mysql_query($qry); //Check whether the query was successful or not if($result) { if(mysql_num_rows($result) == 1) { //Login Successful session_regenerate_id(); $member = mysql_fetch_assoc($result); $_SESSION['SESS_MEMBER_ID'] = $member['id']; $_SESSION['SESS_FIRST_NAME'] = $member['FirstName']; $_SESSION['SESS_LAST_NAME'] = $member['LastName']; $_SESSION['SESS_LAST_NAME'] = $member['memberid']; session_write_close(); } else { //Login failed header("location: login-failed.php"); exit(); } } else { die("Query failed"); } $row = mysql_fetch_object($result); //if the member has an id equal to 0 send them to the member page if($row->memberid == 0){ header("Location: ./member/index.php"); exit(); } //if the member has an id equal to 1 send them to the admin page if($row->memberid == 1){ header("Location: ./admin/index.php"); exit(); } I had something similar to that working for me. Hope this helps rather than confusing you. Reece
-
Hi Buddski, Brilliant suggestion. From being able to see the array i could see that the variables had the ID appended on the end. The issue is solved for now. Thanks!
-
Hi again, Still having the same problem. Anyone got any ideas on this or could point me to a better way of doing it if there is one. Regards
-
Hi guys, It's running now with both of you're solutions in place. However, when submitting the form it wipes everything in the database except for the id (primary key). and i get an undefined index error on line 82 which is my foreach statement. Any ideas on this? Cheers
-
Hi Shawn, Appreciate the quick reply - I'll post the rest of the code below: <section id="Results"> <CENTER> <?php $users = "SELECT * FROM users"; $listusers = mysql_query($users); ?> <table width="500" border="0" cellspacing="1" cellpadding="0"> <form name="form1" method="POST" action="<?php echo $_SERVER['PHP_SELF']; ?>"> <tr> <td align="center"><strong>ID</strong></td> <td align="center"><strong>First Name</strong></td> <td align="center"><strong>Last Name</strong></td> <td align="center"><strong>Email</strong></td> <td align="center"><strong>Password</strong></td> <td align="center"><strong>Admin ID</strong></td> </tr> <?php while($rows=mysql_fetch_array($listusers)) { ?> <tr> <td align="center"><input type="hidden" name="id" value="<? echo $rows['id']; ?>" /><? echo $rows['id']; ?></td> <td align="center"><input name="First Name<? echo $rows['id']; ?>" type="text" id="firstname" value="<? echo $rows['firstname']; ?>"></td> <td align="center"><input name="Last Name<? echo $rows['id']; ?>" type="text" id="lastname" value="<? echo $rows['lastname']; ?>"></td> <td align="center"><input name="Email<? echo $rows['id']; ?>" type="text" id="email" value="<? echo $rows['email']; ?>"></td> <td align="center"><input name="Password<? echo $rows['id']; ?>" type="text" id="password" value="<? echo $rows['password']; ?>"></td> <td align="center"><input name="adminid<? echo $rows['id']; ?>"type="checkbox" id="adminid" value="1" <?php if ($rows['adminid'] ==1) { echo "checked";} else {} ?>></td> </tr> <?php } ?> <tr> <td colspan="4" align="center"> <input type='hidden' name='Submit' /> <input type="Submit" name="Submit" value="Submit"></td> </tr> </form> </table> <?php // Check if button name "Submit" is active, do this if($_SERVER['REQUEST_METHOD'] == "POST") { foreach($_POST['id'] as $id) { $update="UPDATE users SET firstname='" . $_POST["firstname".$id] . "', lastname='" . $_POST["lastname".$id] . "', email='" . $_POST["email".$id] . "', password='" . $_POST["password".$id] . "', adminid='" . $_POST["adminid".$id] . "' WHERE id='" . $id . "'"; $result = mysql_query($update); echo "Updated"; } } ?> </CENTER> </section> There are a couple other things above it but thought i'd post just this to save you trawling through endless code. Cheers again for taking a look.
-
Hi, I've been looking at this for a couple of hours and it's killing me a little inside. I've butchered a few pieces of code from various sources together and come up with this. I understand how it's supposed to work (or i'm pretty sure i do) but the foreach statement is giving me the following error: Warning: Invalid argument supplied for foreach() /document/etc/etc on line 80 Obviously the offending statement is this one: <?php // Check if button name "Submit" is active, do this if($_SERVER['REQUEST_METHOD'] == "POST") { foreach($_POST['id'] as $id) { $update="UPDATE users SET firstname='" . $_POST["firstname".$id] . "', lastname='" . $_POST["lastname".$id] . "', email='" . $_POST["email".$id] . "', password='" . $_POST["password".$id] . "', adminid='" . $_POST["adminid".$id] . "' WHERE id='" . $id . "'"; $result = mysql_query($update); echo "Updated"; } } ?> Not a clue what the problem is atm. If someone could either tell me or point me in the right direction i'd appreciate it/ Reece
-
Adam, Really do appreciate your response so thanks. You've answered pretty much everything. Just one thing though. When i mention click tracking i didn't mean as such tracking my traffic. I meant, would it be possible to see how many times people viewed a particular job / clicked a particular link. As you said about being spammed, i know this is an issue for candidates, I currently work for a job board which is why i wanted to do this project in particular, Hopefully i'll be able to code something where if there aren't a certain number of jobs (say 5 for example) in a candidates chosen location / category, then the email won't get sent to that candidate. I'm not actually planning on the site taking off / being used for a lot i just wanted to showcase something with a real life application, A little more complex than an e-commerce site, I think anyway. When I get cracking i'll post some links up to see what people think and so i can get feedback on the usability of the site. Thanks again, Reece
-
Hi, I've been out of touch with the PHP stuff for nearly 18 months now but as i've started my final year at university i wanted to do a worthwhile project that could showcase my skills and i'm looking for your thoughts (as professionals) on the viability of my initial ideas. I want to create a Job board (for Graduate jobs) using PHP, MYSQL, JQuery etc. essentially whatever I can to get the job done but would like to hear suggestions about the following: [*] Using sessions to have separate logins and back ends for Candidates & Recruiters [*] Automatic emails at set intervals for both candidates and recruiters - Cron Jobs? [*] Need ideas on database tables (I saw a post further down which i'll take a look at) [*] Click tracking etc. Is there a way i can create something to handle this? And how accurate would it be? [*] I would need some sort of reporting, should i use open source software or create something myself [*] Creating a Search function - Is this easy? Or should i just use the google one [*] Would this kind of site be ok hosted? Or would it suffer problems with query speed etc? Sorry for all of the questions, i have a lot more but don't want to overload the page. I'm not asking for people to link to me everything i'm sure google would be able to help me out there but i just want to know if there are specific things i should look into etc or if this type of project is too much for me to handle. I look forward to hearing your feedback. Also if there is a more appropriate forum (I didn't see one), Could a mod move the thread? Cheers, Reece
-
Hi, i was unsure whether to place this in here or the apache web server thread so feel free to move it if need be. I'm not too sure what the problem is myself, i've followed a basic tutorial on another site to create a carousel. When i export the carousel to a html file it works perfectly. however when i load it or even include it into XAMPP it doesn't load. I've never used flash on the server before but am trying to make my site to showcase my work using the carousel as navigation. Would be grateful for any help. Thanks
-
Thanks alot that tutorial has done just fine. Sorry for posting such a simple query
-
Thanks alot, ive never done paging before, i wasn't even sure what it was called. I'll have a read through