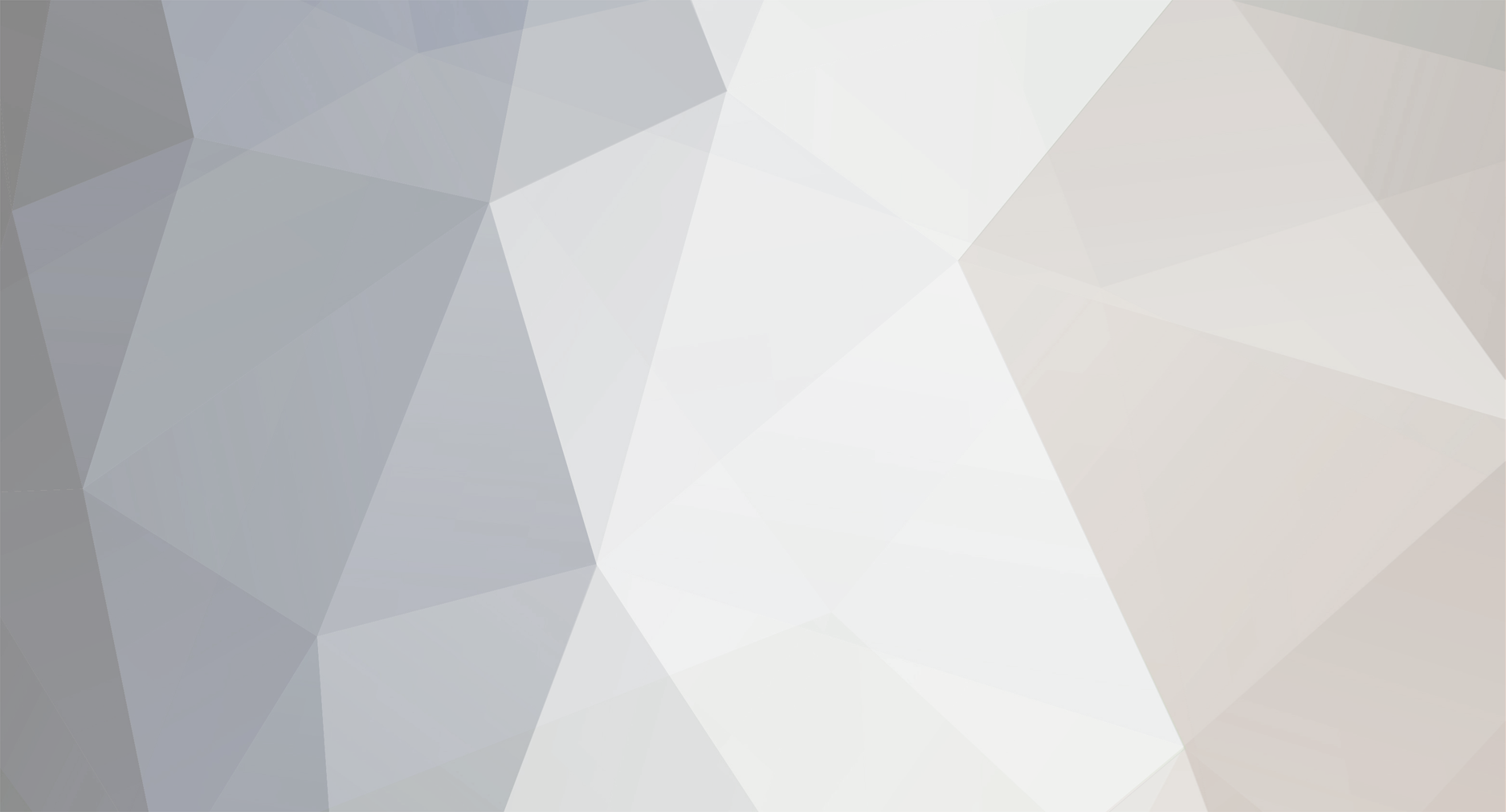
mikr
Members-
Posts
32 -
Joined
-
Last visited
Profile Information
-
Gender
Not Telling
mikr's Achievements

Newbie (1/5)
0
Reputation
-
If I understand you right, you have a video table with the following fields: name desc videoid comments And you want comments to contain a comma delimited list of user id and comments. So, what if the user's comments contain commas? Having worked with csv situations, I always aim to avoid them when possible, so here's a suggestion... Why don't you create one new table (that's all you need) with the following columns: id, datetime, fk_video_id, user_id, comments Then you can have multiple comments for each video, and you can timestamp em and it makes it easier to delete unwanted messages.
-
First, a minor point on the html. You've named the input and the select both "aNumber[]", and as your comments indicate, select ought to be named "select[]". Second, I thought I'd walk through your code and tell you what it tells me. <?php $i = 0; // initialize $i // for every element $key in the array $_POST["aNumber"], add 1 to $i foreach ($_POST["aNumber"] as $key) $i++; echo $i; // so $i = count($_POST["aNumber"]) $j = 0; while ($j<=$i) { // loop $i times. // append the arrays $_POST["aNumber"], $_POST["select"], and $_POST["extOp"] // togther, with inner comma's and set $content. $content = $_POST["aNumber"] . "," . $_POST["select"] . "," . $_POST["extOp"]; $j++; } echo $content; // echo $content which will probably echo... // array(),array(),array() or thereabouts ?> Now, some suggestions... <?php $content = array(); for ($i = 0; $i < count($_POST["aNumber"]); $i++) { $content[] = $_POST["aNumber"][$i] . "," . $_POST["select"][$i] . "," . $_POST["extOp"][$i]; } // at this point, I'm a little unclear as to what you want to do with the extra rows. // if I understand you, you just want to add them in a comma seperated form to the $content // string. So... $content = implode(",",$content); echo $content; ?> Ok, some points. First, two reasons your while loop didn't work. First, you were appending the the arrays together and not their individual elements (see where I add [$i] after each name). Second, you were setting $content each time through the loop, thus wiping out all previous values of $content. I decided to make $content an array (initially) so that I could then implode the final results into a string. Also, your foreach loop can be replaced by a call to the function count() which returns the number of elements in an array. Hope all this helps! Good luck.
-
Do you still have some of the older forms that worked? If you do, then try them out and see if they still work. If they do, try to compare them with what you've got now. Otherwise, my other suggestion is to check if sendmail_form is set: <?php print ini_get("sendmail_from"); ?> If it isn't set, try setting it before executing mail() without the fourth variable. <?php ini_set("sendmail_from", "fromaddress@host.com"); ?> At some point this may be a server problem, and if it is, the question becomes whether you have access to the server or not. If you don't, you'll have to take it up with the owner. If you do, you may try looking at the sendmail logs and seeing what they say.
-
In the past, did you have a header containing a From in it? The php manual suggests that if sendmail_from is not set in php.ini and a custom From header is missing, sendmail may fail. Try adding as a fourth variable, "From: yourself@yourwebsite.com\r\n" .
-
Like Ken2k7 said, I assumed you would change the variables as they properly should be changed. So, assuming that, and you are still having trouble... You need to figure out if it is something you are doing, or something wrong with the server. So you start small. Don't use any variables, just attempt to use mail... <?php mail('me@wherever.com', 'Test Subject', 'Test message'); ?> Replace me@whataever.com with your email address, of course. So, if that works, then replace 'Test Subject' with your $subject variable and test again, then 'Test message' with your $body variable. You may be running afoul of mail in some manner.
-
Ooh. Good catch. That's embarrassing. That's what I get for not running code through php -l...
-
It would be as simple as: <?php if (mail($to, $subject, $message)) { echo "Mailer accepted mail."; } else { echo "Mailer did not accept mail."; } ?>
-
Taking what Ken2k7 said into account, and assuming that $v is an array containing fieldnames, the following would work (I added 'Date' and 'Time' to the array since they were both header names and field names according to your code). <?php $v = array('Date', 'Time', 'Height', 'Weight'); // To print table header of Date and Time echo " <table width='120%' border='1' cellspacing='0' cellpadding='0'>"; echo "<tr> // To print table header for the selected variables ($var) foreach ($v as $var) { echo "<th bgcolor='#CCCCCC'>$var</th>"; } // <- no problem with this echo "</tr>"; while ($row = mysql_fetch_array($result)) { foreach ($v as $var) { echo '<tr><td align=center>' . $row[$var] . '</td></tr>'; } } //end while ?>
-
Perhaps your server can't handle the format of sending to "NAME <EMAIL ADDR>" (are you running on Windows?). It sounds like it's executing the line after mail() properly. If so, then something isn't right about your mail() command. Some folks seem to suggest there needs to be a space between the above NAME and '<'. Maybe that's the problem? I assume you've changed $to_name and $to_email for the purpose of this discussion and that they are properly filled out. You could try checking if mail() returned true or false. True, just because the mail was accepted for delivery, it does not mean the mail will actually reach you, however, it will tell you if the problem is between you and your mailer, or your mailer and the world.
-
Since simplexml_load_file() is read only, that shouldn't be a problem, should it? However, just for fun, supposing you still want to lock it, you don't have to lock the file itself. Let's say your file is whatever.xml. Then, if you want to be sure you are the only one reading whatever.xml (but you can't use fopen/flock), you can use fopen/flock on whatever.xml.lock. Essentially, you are creating your own lock system using filenames. So, when you are about to read the xml file, you first attempt to fopen/flock the xml file with a ".lock" extension. If you can do that, then you go ahead. If not, then someone else has a lock. There are two downsides. If a process dies before the lock file is deleted, you are stuck having to ftp in (or whatever) and delete it by hand. Also, you have to police your own locking and unlocking.
-
If I understand you right, you are saying that when the user isn't logged in, it won't submit the data to the db (which is good), but it also won't redirect to a new page (which is bad). A couple possibilities. If there is a blank line above your php code in that file, the header will fail to work. You can't output any html (even a blank line) before the header, else the header will be ignored. Second, Location requires an absolute address. Some clients allow a relative one (such as you are using), but those following the standards will not. You can usually use $_SERVER['HTTP_HOST'], $_SERVER['PHP_SELF'] and dirname() to make an absolute URI from a relative one yourself. Capitalization is also important. Capitalize the L. Hope all that helps.
-
Here's an approach that uses regular expressions. May not be what you need, but may help you in your quest. <?php $data = "textFileNameHere.txt"; $contents = file_get_contents($data); $res = array(); preg_match_all('/\*start\*(.*?)\*stop\*/',$contents,$res,PREG_PATTERN_ORDER); $res = $res[1]; print_r($res); // prints an array of matches ?>
-
I grabbed this from the php documentation: <?php $fp = fopen("/tmp/lock.txt", "w+"); if (flock($fp, LOCK_EX)) { // do an exclusive lock fwrite($fp, "Write something here\n"); flock($fp, LOCK_UN); // release the lock } else { echo "Couldn't lock the file !"; } fclose($fp); ?>
-
Why don't you reshow your code as it now stands and a short comment on expected behavior. Otherwise, I'm not sure if by getting the error worked out, you mean you removed the semi-colon from the while statement or not.
-
Is there a possibility that you aren't setting $id correctly? You may wish to echo $sql to find out what the SELECT statement looks like. If you have access to something like phpMyAdmin, you could do what I often do, which is grab that select statement that you just echoed and try it in phpMyAdmin. Another possibility is that one of your fields is a reserved keyword for mysql. I often hedge against that by using back-quotes (` - usually under the tilda on your keyboard) around all fieldnames (ie `id`,`Playername`,`Password` ...). Other than that, more info on what you're experiencing would be helpful.