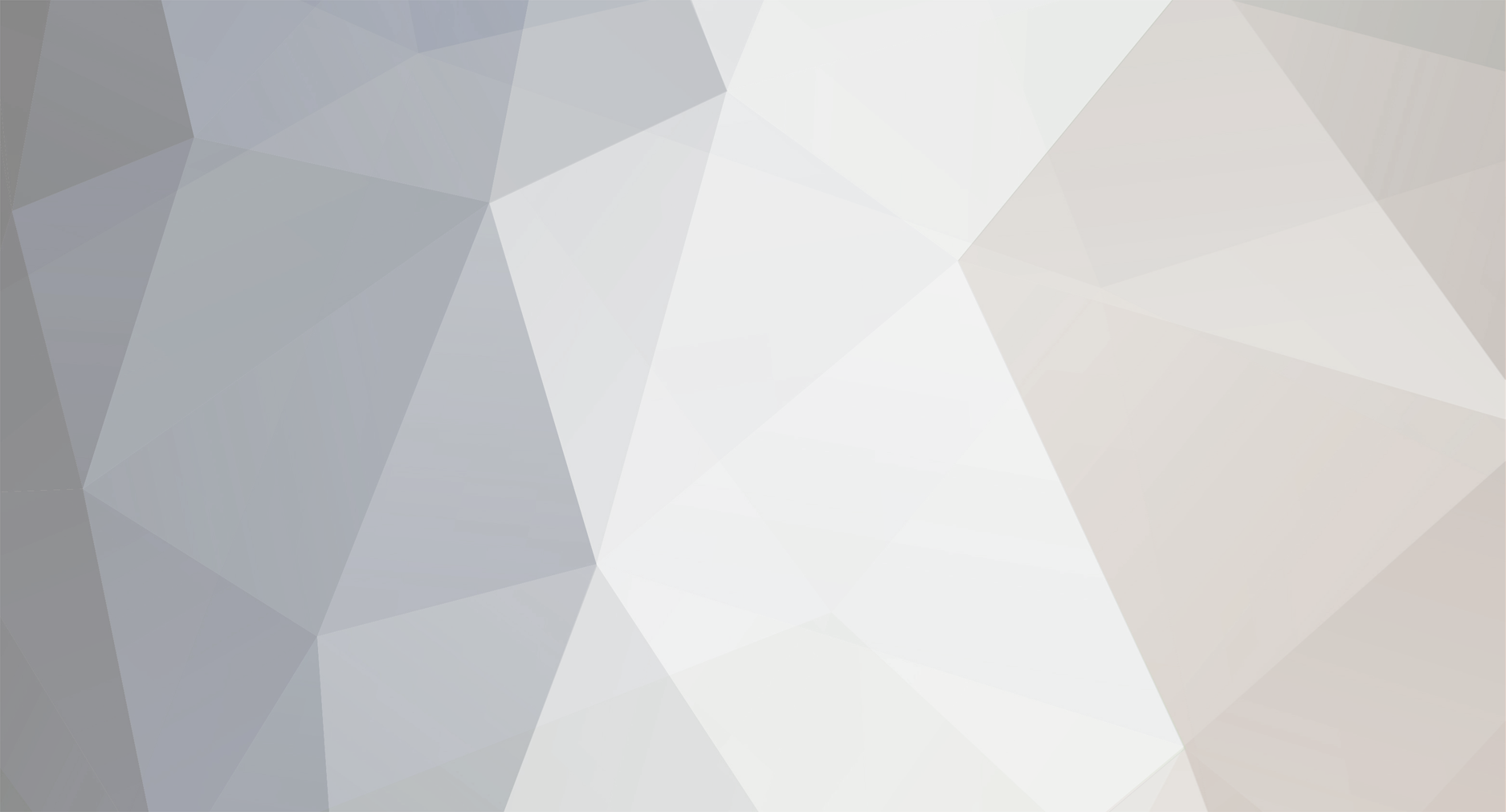
Iconate
New Members-
Posts
9 -
Joined
-
Last visited
Never
Everything posted by Iconate
-
I have read into it, and in order to have a proper function call from a button, you need to have something of this format <form action="<?=$_SERVER['PHP_SELF'];?>" method="post"> <input type="button" name="submit" value="Submit"> </form> then have php at the top of your page <?php if(isset($_POST['submit'])) { foo(); }?> The only problem is, in my code, I have to cut my function short to insert the button I want. I do not know if thats the issue, but my function is not being called. My MySQL statement works, no issue there. <?php //all php code above, DB connections etc... function dataInsert() //Function trying to be called { mysql_query("INSERT INTO b18_3420450_cal.dates (event_id, arena, date, end_date) VALUES ('6', '1', '2009-05-05 11:00:00', '2009-05-05 12:00:00')") or die(mysql_error()); } if(isset($_POST['book'])) // calling dataInsert if book is submitted { dataInsert(); } function timeCompare($tabletime) { global $result; global $q; $available = true; while ($row = mysql_fetch_array($result)) { $qtime = substr($row['date'], 11, 16); if ($tabletime == $qtime) { echo "<td>TAKEN</td>"; $available = false; break; } } if ($available) { echo "<td>"; echo "AVAILABLE - "; ?> <form action="<?=$_SERVER['php_SELF'];?>" method="post"> <input type="submit" name="book" value=" Book Now "> </form> <?php echo "</td>"; } if (mysql_num_rows($result) != 0) { mysql_data_seek($result,0); } } echo "<table border='1'>"; echo "<tr>"; echo "<th>Time</th>"; echo "<th>Availablility for ". $weekday .", ". $q ."</th>"; echo "</tr>"; echo "<tr>"; echo "<td>08:00</td>"; timeCompare("08:00:00"); echo "<tr>"; ... Is what I did legal? Is there a way around it if its not? Because I need the timeCompare function to determine the Availability of the indicated time, if available a user could click the button to sign up for that time
-
My table is being created which is good, however I know my function isn't being called because I created a mysql_query guaranteed to give me an error, and it does not.
-
Ok well I still need to have a PHP function called in a button form. I looked around on the net, and many examples were posed like the following However I have trouble understanding how I can incorporate this into my file. Would it look something like this? <?php ini_set ("display_errors", "1"); error_reporting(E_ALL); $q=$_GET["q"]; $con = mysql_connect('----', '----', '----'); if (!$con) { die('Could not connect: ' . mysql_error()); } mysql_select_db("----", $con); $a = $q[0]; $q = substr($q, 1, 11); $sql="SELECT * FROM dates WHERE arena = '".$a."' AND date LIKE '%".$q."%'"; $result = mysql_query($sql) or die(mysql_error()); $weekday = date('l', strtotime($q)); function dataInsert() //Function I wanna call { //MySQL stuff } if(isset($_POST['book'])) { dataInsert(); } function timeCompare($tabletime) { global $result; global $q; $available = true; while ($row = mysql_fetch_array($result)) { $qtime = substr($row['date'], 11, 16); if ($tabletime == $qtime) { echo "<td>TAKEN</td>"; $available = false; break; } } if ($available) { echo "<td>"; echo "AVAILABLE - "; ?> <form action="<?=$_SERVER['PHP_SELF'];?>" method="post"> //This button is still created <input type="button" name="book" value=" Book Now "> </form> <?php echo "</td>"; } if (mysql_num_rows($result) != 0) { mysql_data_seek($result,0); } } echo "<table border='1'>"; echo "<tr>"; echo "<th>Time</th>"; echo "<th>Availablility for ". $weekday .", ". $q ."</th>"; echo "</tr>"; echo "<tr>"; echo "<td>08:00</td>"; timeCompare("08:00:00"); echo "<tr>"; ... echo "</table>"; mysql_close($con); ?>
-
Hmm tried that, now my table doesn't render. But I am planning to use MySQL in the function, so I am staying away from javascript in this file However what you said makes sense, and I guess alert() is a javascript function, Im just looking for a way to indicate to me that the function is being called
-
Ive been trying to make a simple function call through a button, and to make sure its working properly, I want to display an alert, but I have been having difficulty thus far; function dataInsert() { echo "alert('TEST')"; } function timeCompare($tabletime) { global $result; global $q; $available = true; while ($row = mysql_fetch_array($result)) { $qtime = substr($row['date'], 11, 16); if ($tabletime == $qtime) { echo "<td>TAKEN</td>"; $available = false; break; } } if ($available) { echo "<td>"; echo "AVAILABLE - "; echo '<input type=button name="book" value=" BOOK NOW " onClick="dataInsert()"/>'; //This is supposed to call the function, and display the alert message echo "</td>"; } if (mysql_num_rows($result) != 0) { mysql_data_seek($result,0); } } To be honest, Im not sure if I am supposed to be echoing the alert or not, as well whether or not im supposed to be using single quotes or double quotes. I have messed around with both, and no luck. Any help and additional knowledge would be great
-
[SOLVED] $result is not FALSE, yet errors still
Iconate replied to Iconate's topic in PHP Coding Help
That fixed everything Thank you very much, you guys have been great help. (also gotta figure out how to mark topics as solved ) EDIT Yeah, I know this, I am just getting this aspect to work first. I have other global variables that were defined outside the function which I need to use. -
Hello, I have a feeling this error may be caused by scope issue, but I continuously get the error "Notice: Undefined variable: result in ... on line 38 Warning: mysql_fetch_array(): supplied argument is not a valid MySQL result resource in ... on line 38" I added on the error tags to the mysql_query and I don't get any issues. Note that it is a function too, and I only call it once. I also tried using MYSQL_ASSOC as a parameter for mysql_fetch_array(), but that didn't work at all $result = mysql_query($sql) or die(mysql_error()); function timeCompare() { while ($row = mysql_fetch_array($result)) { echo "<td>". $row["date"] ."</td>"; } } echo "<table border='1'>"; echo "<tr>"; echo "<th>Time</th>"; echo "<th>Availablility</th>"; echo "</tr>"; echo "<tr>"; echo "<td>08:00</td>"; timeCompare(); echo "<tr>"; For now I am just trying to the while loop working before I move on to anything else. I know result cant be wrong, because I had a while loop following my headers before, so the rows were dynamic, now I need the elements in the table to be dynamic, which is why I have the function call.
-
*facepalm* Well it works now heh, thanks, so much for my stupid arbitrary test names.....
-
Hey everyone, I have been working on a project which requires me to use PHP/MySQL, and I have been teaching myself along the way, but I am currently having some function calling issues, and I am not sure if its just syntactical or what. Essentially Im just trying to create a table, but some of the columns are dynamic, my solution to that is to have function calls, but first I need to figure out how to actually do them properly <?php function print() { echo "<table border='1'>"; echo "<tr>"; echo "<th>Time</th>"; echo "<th>Availablility</th>"; echo "</tr>"; echo "<tr><td>08:00</td></tr>"; echo "<tr><td>09:00</td></tr>"; echo "<tr><td>10:00</td></tr>"; echo "</tr>"; echo "</table>"; } $q=$_GET["q"]; $con = mysql_connect('....'); if (!$con) { die('Could not connect: ' . mysql_error()); } mysql_select_db("....", $con); $a = $q[0]; $q = substr($q, 1, 11); $sql="SELECT * FROM dates WHERE arena = '".$a."' AND date LIKE '%".$q."%'"; $result = mysql_query($sql); $dbtime = "NULL"; print(); mysql_close($con); ?> When I run this along with my other files, the page does not display what is in the "print()" function, but if I just take what is in that function and hardcode it, it does get displayed. Thanks, sorry if this is such a trivial error