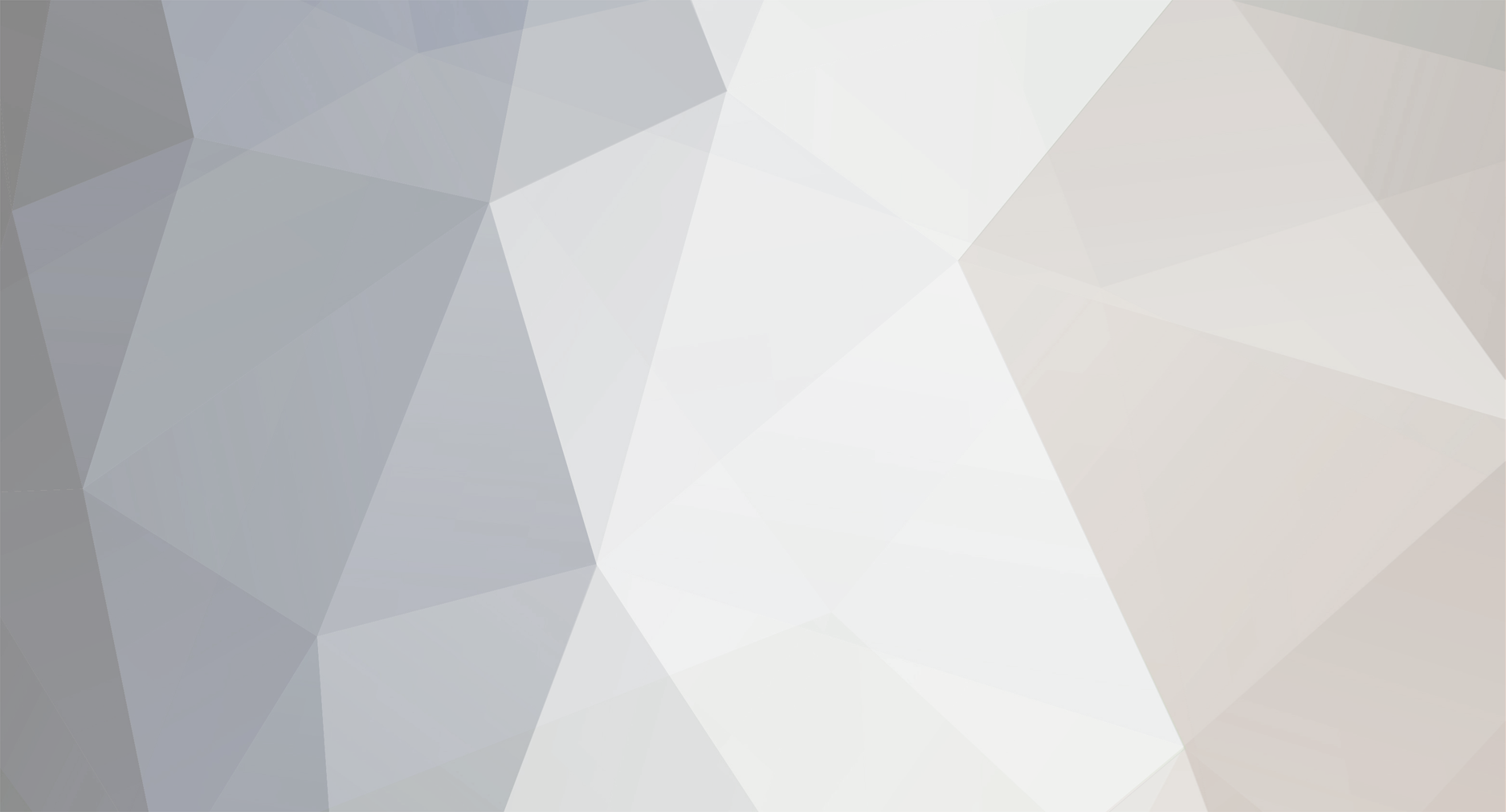
newbtophp
Members-
Posts
631 -
Joined
-
Last visited
Everything posted by newbtophp
-
Thanks, both of you I'll be using the INT type cast, for sure.
-
<?php $file = file_get_contents("http://omars.gmu.edu/items/show/2536"); preg_match("/<div class=\"element-text\">http:\/\/([a-z0-9\/\.]*)<\/div>/i", $file, $matches); echo $matches[0]; ?>
-
Which is the most suitable function, for validing the id (id is an auto increment in sql), is_int, ctype_digit, or is_numeric? <?php //always numbers... $id = $_GET['id']; if(is_int($id)){ //proceed... } if(ctype_digit($id)){ //proceed... } if(is_numeric($id)){ //proceed... } ?>
-
I've been inserting the referer information like this: mysql_query("INSERT INTO site_referrals (referer_id, referer_date, referer_ip, referer_type) VALUES ('$id', '$referer_date', '$referer_ip', 'in')"); But now was wondering how would i echo the total number of referalls this week? I was thinking something such as: <?php $id = 1; $sql = mysql_query("SELECT * FROM site_referalls WHERE referer_id = $id"); $i=0; while($row = mysql_fetch_array($sql)){ $todaysweek = (int)date('W', time()); $week = (int)date('W', $row['referer_date']); if($week == $todaysweek){ $i++; } } echo "Total Referalls this Week: ".$i; ?> :-\
-
solved thanks to jl5501!
-
OK i've managed to progress: Code: <?php function count_word_occurrences($str, $case_sensitive = true) { $str = $case_sensitive ? $str : strtolower($str); $occurrences = array(); foreach(str_word_count($str, 1) as $word){ $word = $case_sensitive ? $word : strtolower($word); if(!in_array($word, array_keys($occurrences))){ $occurrences[$word] = preg_match_all("~\b$word\b~", $str, $matches); } } arsort($occurrences); return array_slice(array_keys($occurrences), 0, 3); } function count_c($arr, $find) { $count = 0; foreach($arr as $words) { $count += preg_match("~\b$find\b~", $words); } return $count; } $oldarray = array('php coding united kingdom', 'united kingdom rocks', 'php is the best', 'united kingdom uses php', 'united kingdom coding', 'php has a united kingdom tld'); $newarray = array('coding', 'rocks', 'is the best', 'uses', 'coding', 'has a tld'); $titles = array(); foreach($newarray as $title) { $titles[$title] = count_c($oldarray, $title); } foreach($titles as $k=>$v) { if($v > 1) { $realtitles[] = $k; } else { $others[] = $k; } } echo "<br><br><b>titles</b>: "; print_r($realtitles); echo "<br><br><b>others</b>: "; print_r($others); ?> Now it gives: Titles: Array ( [0] => coding ) Others: Array ( [0] => rocks [1] => is the best [2] => uses [3] => has a tld ) Just need to figure out how to display it in the following format: Title: coding php coding united kingdom, united kindom coding Title: others united kingdom rocks, php is the best, united kingdom uses php, php has a united kingdom tld If the $title is in one of the values in $oldarray then echo that value below the title, if the title is not or has occured 1 time then it's echo'd under the title "others".
-
anyone? please
-
print_r($titles) produces: Array ( [coding] => 2 [rocks] => 1 [is the best] => 1 [uses] => 1 [has a tld] => 0 ) Im trying to make it so: if [$titles] is 2 or above then it becomes a title, otherwise its placed under a global title called "others", then under each title the words from $oldarray are categorized based on if the title is included within the words, if the title doesn't then its placed under "others". Current code: <?php function count_word_occurrences($str, $case_sensitive = true) { $str = $case_sensitive ? $str : strtolower($str); $occurrences = array(); foreach(str_word_count($str, 1) as $word){ $word = $case_sensitive ? $word : strtolower($word); if(!in_array($word, array_keys($occurrences))){ $occurrences[$word] = preg_match_all("~\b$word\b~", $str, $matches); } } arsort($occurrences); return array_slice(array_keys($occurrences), 0, 3); } function count_c($arr, $find) { $count = 0; foreach($arr as $words) { $count += preg_match("~\b$find\b~", $words); } return $count; } $oldarray = array('php coding united kingdom', 'united kingdom rocks', 'php is the best', 'united kingdom uses php', 'united kingdom coding', 'php has a united kingdom tld'); $newarray = array('coding', 'rocks', 'is the best', 'uses', 'coding', 'has a tld'); $titles = array(); foreach($newarray as $title) { $titles[$title] = count_c($oldarray, $title); } print_r($titles); ?> Would Output: Title: coding php coding united kingdom, united kindom coding Title: others united kingdom rocks, php is the best, united kingdom uses php, php has a united kingdom tld :-\
-
Well i was given a task to rearrange/format words too related keywords, a visitor would submit a list of keywords and can also submit keywords to ignore (optionally - in another textarea), then it would sort the keywords by two word phrase; top recurring keywords which would give the output. When i was assigned the task this example was given: INPUT KEYWORDS (Via $_POST ->new line per word): real property Miami Miami real property for sale Miami homes for sale homes in Miami new homes in Miami Miami houses for sale Miami houses houses in Miami real property Los Vegas Los Vegas real property for sale Los Vegas homes for sale homes in Los Vegas new homes in Los Vegas Los Vegas houses for sale Los Vegas houses houses in Los Vegas IGNORED KEYWORDS (Via $_POST ->new line per word): Miami Los Vegas OUTPUT (comma separated && new line per word): real property,real property Miami real property,real property Los Vegas real property,Miami real property for sale real property,Bevery Hills real property for sale homes,homes in Miami homes,homes in Los Vegas homes,new homes in Miami homes,new homes in Los Vegas houses,Miami houses for sale houses,Miami houses houses,Los Vegas houses for sale houses,Los Vegas houses houses,houses in Miami houses,houses in Los Vegas The trouble, is i need your help to think of how I could achieve, this ie. the methodology behind this?. I've already started it but im getting to the point where im lost on how to get the output (like the example above). My code: <?php if(isset($_POST['submit'])){ if(!empty($_POST['keywords'])){ $words = str_replace(array("\r\n", "\r"), "\n", $_POST['keywords']); //words array $wordsArray = explode("\n", $words); $words2 = str_replace(array("\r\n", "\r"), "\n", $_POST['ignorewords']); //ignore words array $wordsArray2 = explode("\n", $words2); //remove found ignore'd words for words array foreach(array_intersect($wordsArray, $wordsArray2) as $match) { foreach(array_keys($wordsArray, $match) as $key) { unset($wordsArray[$key]); } } $comma_separated = implode(",", $wordsArray); $words = array_count_values(str_word_count($comma_separated, 1)); arsort($words); $words = array_slice($words, 0, 2); //this gives the top 2 recurring words. print_r($words); } } ?> All help is appreciated. :-\
-
Can you write the full code for me? I dont know the function, so .. :i function replace_br($input){ $text = str_replace("<br />","", $input[1]); $ret = '<div class="code"><pre class="brush: php;">'.$text.'</pre></div>'; return $ret; } $turtorial = stripslashes(nl2br($foresp['turtorial'])); $turtorial = preg_replace_callback("/\\[ code\\](.+?)\[\/code\]/is", "replace_br", $turtorial); echo $turtorial;
-
I've already tried but the values need to be a filename (unless i've missed anything). In my case the words are submitted via $_POST['words']
-
*sigh* Perhaps a problem connecting to your db?, are you sure you have the correct db info...
-
Hi, I'm in a bit of trouble. If I have a list of words each on a separate line: Example Words: something say phpfreaks us all something help php php arithmetic php functions How can i place each word within a variable; (like with foreach)? So at the end in this foreach if i echo that variable it should echo 5 words. Also how would i retrieve the top recurring word from the words? (like for e.g. in this case its 'php' since that word is mentioned the most) :-\
-
Solved thanks RussellReal!
-
I'm having some trouble, im using the img bbcode on a forum page and a user page, the forum page layout is different too the user page, theirfore they have different widths etc. Currently the user can simply embed any image within the img bbcode, if the image is huge the webpage and layout will literally expload. (out of the width, destroying the layout). So i decided to use a image resize function to limit the image to a max width. If the image is on topic.php the max width is: 599px If the image is on user.php the max width is: 376px Heres the function: function scaleimage($location){ if($_SERVER['PHP_SELF'] == "user.php"){ $maxw=376; } else if($_SERVER['PHP_SELF'] == "topic.php"){ $maxw=599; } $maxh=200; $img = @getimagesize($location[1]); if($img){ $w = $img[0]; $h = $img[1]; $dim = array('w','h'); foreach($dim AS $val){ $max = "max{$val}"; if(${$val} > ${$max} && ${$max}){ $alt = ($val == 'w') ? 'h' : 'w'; $ratio = ${$alt} / ${$val}; ${$val} = ${$max}; ${$alt} = ${$val} * $ratio; } } $w = round($w); $h = round($h); return("<img src='{$location[1]}' width='{$w}' height='{$h}'>"); } } Usage: $Text = preg_replace_callback("/\[img\](.+?)\[\/img\]/", "scaleimage", $Text); The trouble is i have to currently define the height, how would i set it so it decides for it self and makes sure the height is within the proportion of the max width for the particular files; (topic.php and user.php), but staying appropriate at the same time? :-\
-
I managed to figure it out on my own in the end
-
I have a txt file containing str_replace's, such as: $message = str_replace(":icon_wtf:", "<img src=\"../images/smilies/icon_wtf.gif\">", $message); $message = str_replace(":icon_yay:", "<img src=\"../images/icon_yay.gif\">", $message); $message = str_replace("blank", "<img src=\"../images/icon_wtf.gif\">", $message); $message = str_replace("", "<img src=\"../images/icon_.gif\">", $message); But I want to; with php rearrange all the str_replaces. So they will turn from: $message = str_replace(":icon_wtf:", "<img src=\"../images/smilies/icon_wtf.gif\">", $message); Too: $message = str_replace("<img src=\"../images/smilies/icon_wtf.gif\">", ":icon_wtf:" $message); :-\ All help is greatly appreciated, im assuming i'd need to use preg_replace_callback? and fwrite, just need help to proceed.. My attempt: <?php $file = file_get_contents("replaces.txt"); $file = preg_replace('#str_replace\(".*", ".*"#', 'str_replace("$2", "$1"', $file); $fp = fopen('replaces.txt', 'w'); fwrite($fp, $file); fclose($fp); ?>
-
Im stuck; basically i have a review system, where an author uploads something and another user can review it, then the author can respond to that review, and their can only be 1 author response per user review. I know the concept behind it just unsure how to proceed :-\ <?php include("conn.php"); //the upload id $id = clean($_GET['id']); //this is the reviewer $user = $_SESSION['username']; $result = mysql_fetch_array(mysql_query("select * from portal_main where id = '$id'")); //this is the author $author = $result['user']; //this would be the query to select a review $querytoselectareview = mysql_query("SELECT review FROM portal_reviews WHERE id = '$id' AND response='0'"); //this would be the query to select a author response $querytoselectaresponse = mysql_query("SELECT review FROM portal_reviews WHERE id = '$id' AND response='1'"); //now how would i check if the author can respond to the review?, or if the author cant etc. ?>
-
I've got alittle problem, the username is defined correctly via $_POST etc. But can't get the character length to validate, it proceed without paying any notice. I want to username to be valid if its between 3->15 characters long (3 as minimum and 15 as maximum) Heres my attempt using strlen(): elseif(!strlen($user) >= 3 && strlen($user) <= 15){ echo 'Username must be atleast 3 characters, and no longer than 15!'; } I then tried doing it with regex: elseif(!preg_match("/^\d{3,15}$/", $user)){ echo 'Username must be atleast 3 characters, and no longer than 15!'; } :-\
-
I get the following errors: Notice: Undefined index: username In the file func.php; in the first line of the auto_logout() function which is this line: $username = $_SESSION['username']; And this error: Notice: Undefined index: user_slashed In the file func.php; in the second line of the auto_logout() function which is this line: $user_slashed = $_SESSION['user_slashed']; :-\
-
I've added this: session_start() ; require("func.php"); $logged_in = inc_online(); auto_logout(); update(); if($logged_in == 'yes') { //protected stuff.... } But still no progess
-
Still the same problem it logs me out upon page load even though the function aint been declared. Besides auto_logout(); only occurs upon a fixed period of time.
-
I'm having abit of trouble, logging in and out, sometimes when i login it keeps me logged in for a few hours then auto logs out, sometimes once i log in it logs me back out when viewing a protected page, and sometimes when i click logout (run logout.php) it does'nt log me out (and keeps me signed in). Logout.php: <?php require("func.php"); $logged_in = inc_online(); auto_logout(); update(); if($logged_in == 'yes') { $time = time(); $online_query = mysql_query("UPDATE ".$users_table." SET user_online = '0', user_time = '$time', user_lastlog = '$time' WHERE user_username = '".addslashes($_SESSION['username'])."'"); $ip = $_SERVER['REMOTE_ADDR']; $sql = mysql_query("select userid from site_remember where userip = '$ip'"); $num = mysql_num_rows($sql); if ($num > 0) { $sql = mysql_query("DELETE FROM `site_remember` WHERE userip = '".$ip."'"); } echo "You have logged out sucessfully"; } else { print "You cannot logout if your not logged in!<br /><br><a href=\"login.php\">Login</a>"; } ?> func.php: <?php // online function function inc_online() { $ip = $_SERVER['REMOTE_ADDR']; $sql = mysql_query("SELECT `userid` FROM `site_remember` WHERE `userip` = '$ip'"); $num = mysql_num_rows($sql); if ($num > 0) { $row = mysql_fetch_array($sql); $userid = $row['userid']; $sql2 = mysql_query("SELECT `user_password`, `user_username`, `user_level`, `user_slashed` FROM `site_users` WHERE `user_id` = '$userid'"); $row2 = mysql_fetch_array($sql2); $username = $row2['user_username']; $password = $row2['user_password']; $user_level = $row2['user_level']; $user_slashed = $row2['user_slashed']; $time = time(); mysql_query("UPDATE `site_users` SET `user_online` = '1' WHERE `user_username` = '$username'"); mysql_query("UPDATE `site_users` SET `user_time` = '$time' WHERE `user_username` = '$username'"); mysql_query("UPDATE `site_users` SET `user_ip` = '$ip' WHERE `user_username` = '$username'"); $_SESSION['user_id'] = $userid; $_SESSION['username'] = $username; $_SESSION['user_level'] = $user_level; $_SESSION['password'] = $password; $_SESSION['user_slashed'] = $user_slashed; } if (isset($_SESSION['user_id']) && isset($_SESSION['username']) && isset($_SESSION['password']) && isset($_SESSION['user_level']) && isset($_SESSION['user_slashed'])) { $sql44 = mysql_query("select user_id, user_password, user_username, user_slashed, user_level from site_users where user_ip = '$ip'"); if ($sql44) { $row2 = mysql_fetch_array($sql44); $username = $row2['user_username']; $password = $row2['user_password']; $user_level = $row2['user_level']; $user_slashed = $row2['user_slashed']; $_SESSION['user_id'] = $userid; $_SESSION['username'] = $username; $_SESSION['user_level'] = $user_level; $_SESSION['password'] = $password; $_SESSION['user_slashed'] = $user_slashed; $logged_in = "yes"; } else { $logged_in = "no"; } } else { $logged_in = "no"; } return $logged_in; } // auto logout function auto_logout() { $username = $_SESSION['username']; $user_slashed = $_SESSION['user_slashed']; if (isset($username)) { $offline = 500; $current = time(); $offline = ($current-$offline); $time = time(); $sql_query = mysql_query("SELECT `user_id` FROM `site_users` WHERE `user_time` >= '$offline' AND `user_online` = '1' AND `user_slashed` = '$user_slashed'"); if (mysql_num_rows($sql_query) == 0) { session_destroy(); mysql_query("UPDATE `site_users` SET `user_online` = '0' AND `user_lastlog` = '$time' WHERE user_slashed = '$user_slashed'"); } else { mysql_query("UPDATE `site_users` SET `user_time` = '$time' WHERE `user_slashed` = '$user_slashed'"); } } } ?> Login.php <?php require("func.php"); $logged_in = inc_online(); auto_logout(); update(); if($logged_in == 'yes'){ print "You are already logged in!<br /><a href=\"logout.php\">Logout?</a>"; } else { if ($_GET['pg'] == '') { ?> <form action="?pg=login" method="post" enctype="multipart/form-data"> Username:<br> <input name="username" type="text" class="textfield3" /> <br> <br> Password:<br> <input name="password" type="password" class="textfield3" /> <br> <br> <input type="hidden" name="auto" value="" /> <input name="Submit" type="submit" class="button" value="Login" /> </form> <?php } elseif ($_GET['pg'] == 'login') { $username = clean($_POST['username']); $password = clean($_POST['password']); $auto = $_POST['auto']; if (!$username) { echo "Fill in username"; } elseif (!$password) { echo "Fill in password"; } else{ $username = clean($username); $password = clean($password); $pass = md5($password); $sql = mysql_query("SELECT user_id, user_password, user_username, user_slashed, user_level FROM ".$users_table." WHERE `user_slashed` = '".$username."' and `user_password` = '".$pass."' and (`user_verified` = 'Y' || `user_verified` = 'B')"); $sql1 = mysql_query("SELECT user_verified FROM ".$users_table." WHERE `user_slashed` = '".$username."' and `user_password` = '".$pass."' and `user_verified` = 'B'"); $u_v=mysql_fetch_array($sql1); $time = time(); $online_query = mysql_query("UPDATE ".$users_table." SET user_online = '1' WHERE user_username = '$username'"); $online_query2 = mysql_query("UPDATE ".$users_table." SET user_time = '$time' WHERE user_username = '$username'"); mysql_query("UPDATE ".$users_table." SET user_lastlog = '$time' WHERE user_username = '$username'"); $row = mysql_fetch_array($sql); $user_id = $row['user_id']; $username = $row['user_username']; $password = $row['user_password']; $user_level = $row['user_level']; $user_slashed = $row['user_slashed']; $user_ip = $_SERVER['REMOTE_ADDR']; if ($auto == 'yes') { $sql = mysql_query("select userid from site_remember where userip = '$user_ip'"); $num = mysql_num_rows($sql); if ($num == 0) { $auto_query = mysql_query("INSERT INTO `site_remember` ( `userip` , `userid` ) VALUES ('".$user_ip."', '".$user_id."')"); } } mysql_query("UPDATE `site_users` SET `user_ip` = '$user_ip' WHERE `user_username` = '$username'"); // Get them logged in $_SESSION['user_id'] = $user_id; $_SESSION['username'] = $username; $_SESSION['user_level'] = $user_level; $_SESSION['user_level1'] = $user_level; $_SESSION['password'] = $password; $_SESSION['user_slashed'] = $user_slashed; echo "You are not logged in"; if ($auto == 'yeS') { print "<br />You have also enabled auto login. You will be logged in automaticlly now until u click log out."; } } } } if(@$_SESSION['username']){ echo "You will be logged in until you close your browser or click on log out."; } ?> When logged in and i access a protected page it logs me back out, its usually when i have the following code on the protected page: require("func.php"); $logged_in = inc_online(); auto_logout(); update(); if($logged_in == 'yes') { //protected stuff.... } Can anyone, help identify the problem? :-\ Thanks.
-
I've got pagination links and they look a mess - especially if the topic/thread is very large, so im wanting to contain the links within a dropdown, and then make the dropdown selected value the current page. Heres the code: <?php $sql = mysql_query("SELECT `post_id` FROM `site_posts` WHERE `post_topic` = '" . $topic_id . "'"); $d=0; $f=0; $g=1; print "Page: "; while($order3=mysql_fetch_array($sql)) { if($f%$limit==0) { if ($start == $d) { print " $g |"; } else { //$g = page number print " <a href='view_topic.php?start=$d&id=$topic_id'>$g</a> |"; } $g++; } $d=$d+1; $f++; } ?> I tried myself, it works however it doesnt make the default selected value the current page. <?php $sql = mysql_query("SELECT `post_id` FROM `site_posts` WHERE `post_topic` = '" . $topic_id . "'"); $d=0; $f=0; $g=1; ?> <FORM NAME="nav"><DIV> <?php print "Page Select: "; ?> <SELECT NAME="SelectURL" onChange= "document.location.href= document.nav.SelectURL.options[document.nav.SelectURL.selectedIndex].value"> <?php while($order3=mysql_fetch_array($sql)) { if($f%$limit==0) { if ($start == $d) { print " $g |"; } else { //print " <a href='view_topic.php?start=$d&id=$topic_id'>$g</a> |"; ?> <option value="view_topic.php?start=<?php echo $d; ?>&nav=<?php echo $g; ?>&id=<?php echo $topic_id; ?>" <?php if($_GET['nav'] == $g){ echo 'SELECTED'; } ?>><?php echo $g; ?></option> <?php } $g++; } $d=$d+1; $f++; } ?> </SELECT><DIV> </FORM> :-\
-
Solved thanks to russellreal