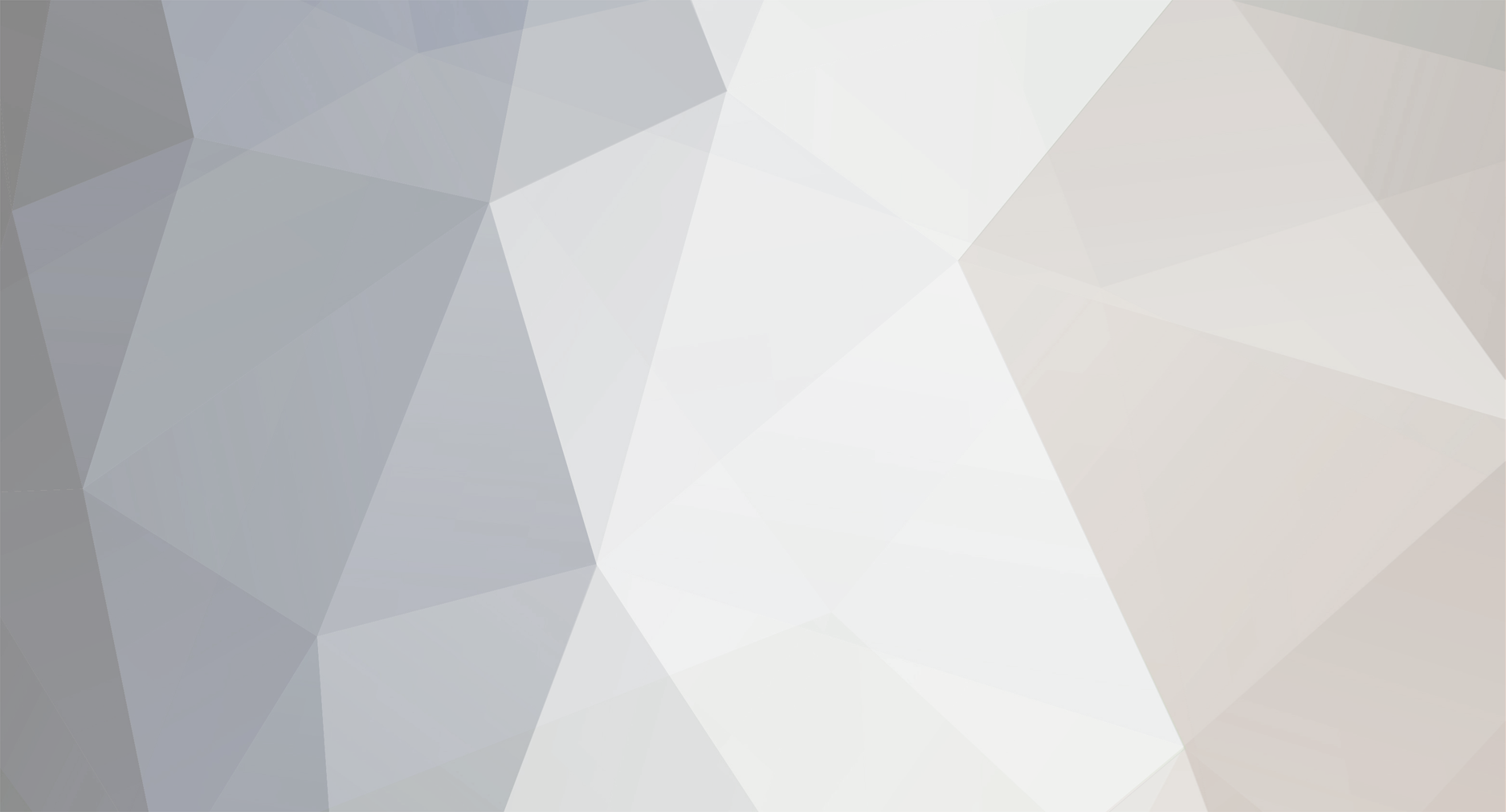
Alex
Staff Alumni-
Posts
2,467 -
Joined
-
Last visited
Everything posted by Alex
-
Is the key supposed to always correspond to the value? If so couldn't you just store the key only then get the value using that key? If you insist in storing it within 2 values (kinda defeats the purpose of an array). You can do this: $arr = array( 'G'=>'Good', 'B'=>'BAD', 'O'=>'OK' ); $a = array_rand($arr); $b = $arr[$a];
-
I don't think it's possible to do that with str_replace. To get the variables that will be replaced you can make a simple function like this: function replacedWords($string, $filter) { foreach($filter as $word) { if(stripos($string, $word) === false){}else { $replaced[] = $word; } } return $replaced; } $filter = array("just","very short", "long", "two words", "other"); $str1 = "This is just a sample text, a very short one"; $replaced = replacedWords($str1, $filter); $str2 = str_replace($filter, "", $str1); print_r($replaced);// Array ( [0] => just [1] => very short )
-
Cool. Thanks for letting us know.. More seriously, what you actually asking?
-
It would've been nice if you gave us the error you get. I looked over it fast and I saw this: $result = 'mysql_query($sql)'; Should be: $result = mysql_query($sql); If it still doesn't work post your errors. Edit: Owait.. All of this needs to be changed: $username = 'stripslashes($username)'; $password = 'stripslashes($password)'; $username = 'mysql_real_escape_string($username)'; $password = 'mysql_real_escape_string($password)'; to: $username = stripslashes($username); $password = stripslashes($password); $username = mysql_real_escape_string($username); $password = mysql_real_escape_string($password);
-
You should have it as an INT. Then when getting it back from the database you can do something like: $row['price'] = (empty($row['price'])) ? 'price unavailable' : $row['price']; If the row is empty, when fetching it it'll set $row['price'] to price unavailable for echoing it out later
-
This might help: http://groups.google.com/group/ffmpeg-php/browse_thread/thread/965d68325844073a
-
Mine will work fine, it only shows the last couple because that's what I put it to do, instead just remove those echos, and put print_r($parts); when you run it on the full string it'll show the array that contains all the parts.
-
Something like: switch($_GET['page']) { case 1: $content = 'content 1'; break; case 2: $content = 'content 2'; break; default: $content = 'Default content..'; break; } echo $content;
-
Assuming all the spacers are 7 characters long (as they are in your example) You can use this: $string = '|ACTIV|gvnE8HEsyivRyirwE|TRIME|wpxFGswFxmJdcJF|CLAS1|gUGJxfJd|CLAS2|gUGJxfJd|SEVER|'; $split = str_split($string); $parts = Array(); $j = -1; for($i = 0;$i < count($split)-7;$i++) { if($split[$i] == '|'){ $i += 7; $j++;} $parts[$j] .= $split[$i]; } echo $parts[0] . '<br />'; //gvnE8HEsyivRyirwE echo $parts[1] . '<br />'; //wpxFGswFxmJdcJF echo $parts[2] . '<br />'; //gUGJxfJd echo $parts[3] . '<br />'; //gUGJxfJd You could probably also use Regex, I might post a regex solution in a few minutes.
-
I am a little confused. So does this mean >= and => are interchangeable? No, => is used in arrays. >= would be used in a conditional statement.
-
Here's a little bit of a better explanation: $array = Array('something' => 'value'); echo $array['something']; // 'value' Key => Value, Key is the name by which you'll be accessing that element of the array. And the value is well, the value of that element.
-
They pretty much covered it. => in arrays key => value. -> Is used (not only in PHP) as a pointer. Example: class->method();
-
He was very talented, but he did have another side. And he was scheduled to have a tour starting July, 50 shows; he was supposed to make over $400,000,000.
-
Function to skip strtolower() if variable contain "wikipedia.org"
Alex replied to ArizonaJohn's topic in PHP Coding Help
if(strpos($site, 'wikipedia.org') === false) { $site = strtolower($site); } -
I'm looking for a good VPS host. I don't want to jump into something that I won't like. Anyone have any suggestions? The one good host that was recommended to me doesn't except paypal and I can't use the paypal secure card feature until July 7th because I had to create a new paypal account..
-
What other language are you using to make it besides php/mysql? I made a 'chat box' using Ajax/Php/MySQL, if it's done efficiently and correctly there shouldn't be any issues.
-
There are so many more advantages of using a MySQL database over a txt file-storage system. First off it will be faster. Second off I'm sure you're going to want to limit chat displayed, that's much easier with MySQL. Google 'MySql vs text file' you'll find tons of reasons to use a database over a text file.
-
[SOLVED] How to display nothing if $row result is empty
Alex replied to rmelino's topic in PHP Coding Help
while ($row = @mysql_fetch_assoc($result)){ echo (empty($row['name'])) ? NULL : '<p>Name: ' . $row['name'] . '</p>' . "\n"; echo (empty($row['address'])) ? NULL : '<p>Address: ' . $row['address'] . '</p>' . "\n"; echo (empty($row['email'])) ? NULL : '<p>Email: ' . $row['email'] . '</p>' . "\n"; } -
Because arrays start at indexing at 0, not 1. Say you have: $array = Array('number1', 'number2', 'number3'); $array[0] = number1 $array[1] = number2 $array[2] = number3 There's 3 elements, but the highest index is 2.
-
I found this online, that you can use to get the full, current URL: function selfURL() { $s = empty($_SERVER["HTTPS"]) ? '' : ($_SERVER["HTTPS"] == "on") ? "s" : ""; $protocol = strleft(strtolower($_SERVER["SERVER_PROTOCOL"]), "/").$s; $port = ($_SERVER["SERVER_PORT"] == "80") ? "" : (":".$_SERVER["SERVER_PORT"]); return $protocol."://".$_SERVER['SERVER_NAME'].$port.$_SERVER['REQUEST_URI']; } function strleft($s1, $s2) { return substr($s1, 0, strpos($s1, $s2)); } $currentURL = selfURL();
-
if (result) { Should be: if ($result) {
-
Make sure they have a value: <?php if(isset($_POST['fname'])) echo $_POST["fname"]; ?> <?php if(isset($_POST['age'])) echo $_POST["age"]; ?>
-
Edit: Is it really necessary to use those classes? If not you can just use: $result = mysql_query('SELECT id, title FROM table LIMIT 10'); while($row = mysql_fetch_assoc($result)) { echo '<p><a href="/page/?item=' . $row['id'] . '">' . $row['title'] . '</a></p>'; }
-
Thanks, I thought about that a little later that I was foolish because I didn't test it with more than one '>' in the text. And figured out why I was wrong, just too lazy to edit my post. Thanks for the help; I'm trying to get better at regex.