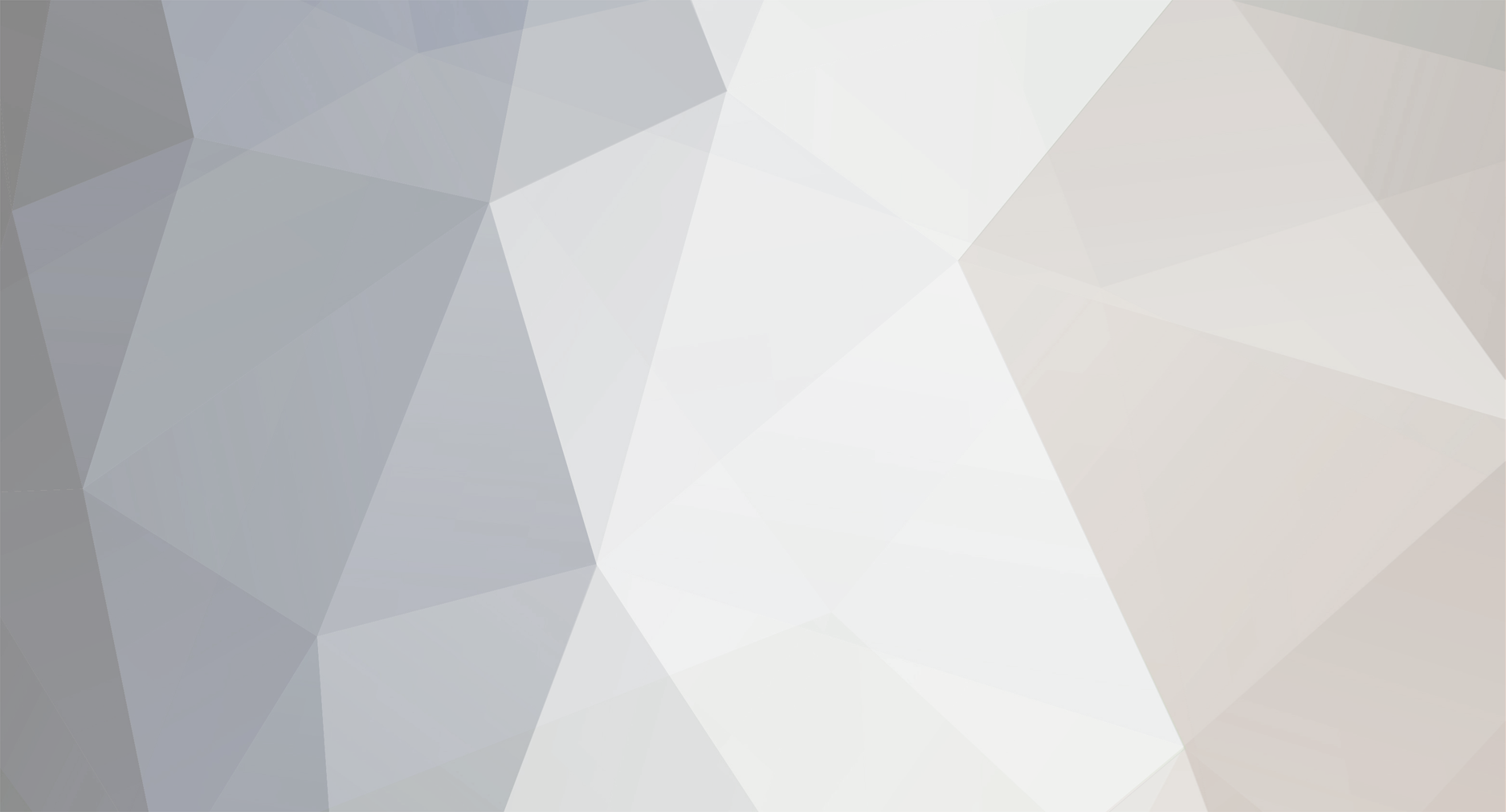
Alex
Staff Alumni-
Posts
2,467 -
Joined
-
Last visited
Everything posted by Alex
-
You want to echo the keys of the array? Or the names of the variables? In this case they would be the same.. echo $key . ':';
-
That's happening because the page needs to load all the images and such on the page before that function is called. jQuery has a way around this.. Ex: $(document).ready(function(){ //Code here } Try jQuery.
-
Thanks. I never even thought about the define problem. There are some other things in there that could be optimized as well, but it was written fast and I never went back to clean it up.
-
$q1 = "INSERT INTO banning (`user`, `type`, `until`, `note`) VALUES ('$user', '$bantype', '$stamp', '$note')"; $q2 = "UPDATE `users` SET `banned`='1' WHERE `login`='$user'"; mysql_query($q1); mysql_query($q2);
-
You shouldn't be using JPEG format images if you don't want that to occur. This isn't a code issue. Idealy you should be using PNG or another lossless image format. JPEG isn't lossless so every time it's processed it tends to get messed up.
-
That doesn't look right.
-
An Image class that I wrote quickly has the ability to do exactly what you want (and a lot more). here's the class: class Image { var $image, $ext, $file; public function __construct($im=NULL) { define('CENTER', 0, true); define('CENTER_LEFT', 1, true); define('CENTER_RIGHT', 2, true); define('TOP_CENTER', 3, true); define('TOP_LEFT', 4, true); define('TOP_RIGHT', 5, true); define('BOTTOM_CENTER', 6, true); define('BOTTOM_LEFT', 7, true); define('BOTTOM_RIGHT', 8, true); if(empty($im)) return true; $info = @getimagesize($im); $backtrace = debug_backtrace(); if(!$info) return die('<b>Fatal error:</b> \'' . $im . '\' is not a valid image resource on <b>line ' . $backtrace[0]['line'] . ' [' . $backtrace[0]['file'] . ']</b>'); $ex = explode('/', $info['mime']); $this->ext = $ex[1]; $func = 'imagecreatefrom' . $this->ext; $this->image = @$func($im); $this->file = $im; return true; } public function setImage($im) { $info = @getimagesize($this->file); $backtrace = debug_backtrace(); if(!$info) return die('<b>Fatal error:</b> \'' . $im . '\' is not a valid image resource on <b>line ' . $backtrace[0]['line'] . ' [' . $backtrace[0]['file'] . ']</b>'); $ex = explode('/', $info['mime']); $this->ext = $ex[1]; $func = 'imagecreatefrom' . $this->ext; $this->image = @$func($im); $this->file = $im; return true; } public function resize($width, $height, $porportional=FALSE, $percent=NULL, $max=NULL) { $backtrace = debug_backtrace(); if(empty($this->image)) return die('<b>Fatal error:</b> Invalid call to Image()->resize, no image is set on <b>line ' . $backtrace[0]['line'] . ' [' . $backtrace[0]['file'] . ']</b>'); $info = Array(imagesx($this->image), imagesy($this->image)); if($porportional) { if(empty($max) && empty($percent)) return false; if(empty($max)) { $new_width = $info[0] * ($percent/100); $new_height = $info[1] * ($percent/100); } else { if($info[0] < $max && $info[1] < $max) return false; $new_width = ($info[0] > $info[1]) ? $max : ($info[0]/$info[1]) * $max; $new_height = ($info[0] > $info[1]) ? ($info[1]/$info[0]) * $max : $max; } } else { $new_width = $width; $new_height = $height; } $new_image = imagecreatetruecolor($new_width, $new_height); imagecopyresampled($new_image, $this->image, 0, 0, 0, 0, $new_width, $new_height, $info[0], $info[1]); $this->image = $new_image; return true; } public function crop($width, $height, $x, $y, $position=NULL) { $backtrace = debug_backtrace(); if(empty($this->image)) return die('<b>Fatal error:</b> Invalid call to Image()->crop, no image is set on <b>line ' . $backtrace[0]['line'] . ' [' . $backtrace[0]['file'] . ']</b>'); $info = Array(imagesx($this->image), imagesy($this->image)); if($width > $info[0] || $height > $info[1]) return false; if(!empty($position) && $position >= 0 && $position <= { switch($position) { case CENTER: $x = ($info[0] - $width)/2; $y = ($info[1] - $height)/2; break; case CENTER_LEFT: $x = 0; $y = ($info[1] - $height)/2; break; case CENTER_RIGHT: $x = ($info[0] - $height); $y = ($info[1] - $height)/2; break; case TOP_CENTER: $x = ($info[0] - $width)/2; $y = 0; break; case TOP_LEFT: $x = 0; $y = 0; break; case TOP_RIGHT: $x = ($info[0] - $width); $y = 0; break; case BOTTOM_CENTER: $x = ($info[0] - $width)/2; $y = ($info[1] - $height); break; case BOTTOM_LEFT: $x = 0; $y = ($info[1] - $height); break; case BOTTOM_RIGHT: $x = ($info[0] - $width); $y = ($info[1] - $height); break; default: return false; break; } } $new_image = imagecreatetruecolor($width, $height); imagecopyresampled($new_image, $this->image, 0, 0, $x, $y, $width, $height, $width, $height); $this->image = $new_image; return true; } public function save($loc, $compression=0) { $backtrace = debug_backtrace(); if(empty($this->image)) return die('<b>Fatal error:</b> Invalid call to Image()->save, no image is set on <b>line ' . $backtrace[0]['line'] . ' [' . $backtrace[0]['file'] . ']</b>'); $func = 'image' . $this->ext; return $func($this->image, $loc, $compression); } public function output() { $backtrace = debug_backtrace(); if(empty($this->image)) return die('<b>Fatal error:</b> Invalid call to Image()->output, no image is set on <b>line ' . $backtrace[0]['line'] . ' [' . $backtrace[0]['file'] . ']</b>'); header('Content-type: image/' . $this->ext); $func = 'image' . $this->ext; $func($this->image); } } You're gonna wanna do something like this: //Include my class $image = new Image('path/to/image'); $image->resize(null, null, true, null, 150); $image->save('path/to/new/location.ext');
-
I actually made a class that does exactly this. It's pretty good for image manipulation. It could have more, but for my purposes it's more than enough. <?php class Image { var $image, $ext, $file; public function __construct($im=NULL) { define('CENTER', 0, true); define('CENTER_LEFT', 1, true); define('CENTER_RIGHT', 2, true); define('TOP_CENTER', 3, true); define('TOP_LEFT', 4, true); define('TOP_RIGHT', 5, true); define('BOTTOM_CENTER', 6, true); define('BOTTOM_LEFT', 7, true); define('BOTTOM_RIGHT', 8, true); if(empty($im)) return true; $info = @getimagesize($im); $backtrace = debug_backtrace(); if(!$info) return die('<b>Fatal error:</b> \'' . $im . '\' is not a valid image resource on <b>line ' . $backtrace[0]['line'] . ' [' . $backtrace[0]['file'] . ']</b>'); $ex = explode('/', $info['mime']); $this->ext = $ex[1]; $func = 'imagecreatefrom' . $this->ext; $this->image = @$func($im); $this->file = $im; return true; } public function setImage($im) { $info = @getimagesize($this->file); $backtrace = debug_backtrace(); if(!$info) return die('<b>Fatal error:</b> \'' . $im . '\' is not a valid image resource on <b>line ' . $backtrace[0]['line'] . ' [' . $backtrace[0]['file'] . ']</b>'); $ex = explode('/', $info['mime']); $this->ext = $ex[1]; $func = 'imagecreatefrom' . $this->ext; $this->image = @$func($im); $this->file = $im; return true; } public function resize($width, $height, $porportional=FALSE, $percent=NULL, $max=NULL) { $backtrace = debug_backtrace(); if(empty($this->image)) return die('<b>Fatal error:</b> Invalid call to Image()->resize, no image is set on <b>line ' . $backtrace[0]['line'] . ' [' . $backtrace[0]['file'] . ']</b>'); $info = Array(imagesx($this->image), imagesy($this->image)); if($porportional) { if(empty($max) && empty($percent)) return false; if(empty($max)) { $new_width = $info[0] * ($percent/100); $new_height = $info[1] * ($percent/100); } else { if($info[0] < $max && $info[1] < $max) return false; $new_width = ($info[0] > $info[1]) ? $max : ($info[0]/$info[1]) * $max; $new_height = ($info[0] > $info[1]) ? ($info[1]/$info[0]) * $max : $max; } } else { $new_width = $width; $new_height = $height; } $new_image = imagecreatetruecolor($new_width, $new_height); imagecopyresampled($new_image, $this->image, 0, 0, 0, 0, $new_width, $new_height, $info[0], $info[1]); $this->image = $new_image; return true; } public function crop($width, $height, $x, $y, $position=NULL) { $backtrace = debug_backtrace(); if(empty($this->image)) return die('<b>Fatal error:</b> Invalid call to Image()->crop, no image is set on <b>line ' . $backtrace[0]['line'] . ' [' . $backtrace[0]['file'] . ']</b>'); $info = Array(imagesx($this->image), imagesy($this->image)); if($width > $info[0] || $height > $info[1]) return false; if(!empty($position) && $position >= 0 && $position <= { switch($position) { case CENTER: $x = ($info[0] - $width)/2; $y = ($info[1] - $height)/2; break; case CENTER_LEFT: $x = 0; $y = ($info[1] - $height)/2; break; case CENTER_RIGHT: $x = ($info[0] - $height); $y = ($info[1] - $height)/2; break; case TOP_CENTER: $x = ($info[0] - $width)/2; $y = 0; break; case TOP_LEFT: $x = 0; $y = 0; break; case TOP_RIGHT: $x = ($info[0] - $width); $y = 0; break; case BOTTOM_CENTER: $x = ($info[0] - $width)/2; $y = ($info[1] - $height); break; case BOTTOM_LEFT: $x = 0; $y = ($info[1] - $height); break; case BOTTOM_RIGHT: $x = ($info[0] - $width); $y = ($info[1] - $height); break; default: return false; break; } } $new_image = imagecreatetruecolor($width, $height); imagecopyresampled($new_image, $this->image, 0, 0, $x, $y, $width, $height, $width, $height); $this->image = $new_image; return true; } public function save($loc, $compression=0) { $backtrace = debug_backtrace(); if(empty($this->image)) return die('<b>Fatal error:</b> Invalid call to Image()->save, no image is set on <b>line ' . $backtrace[0]['line'] . ' [' . $backtrace[0]['file'] . ']</b>'); $func = 'image' . $this->ext; return $func($this->image, $loc, $compression); } public function output() { $backtrace = debug_backtrace(); if(empty($this->image)) return die('<b>Fatal error:</b> Invalid call to Image()->output, no image is set on <b>line ' . $backtrace[0]['line'] . ' [' . $backtrace[0]['file'] . ']</b>'); header('Content-type: image/' . $this->ext); $func = 'image' . $this->ext; $func($this->image); } } For your case you could do something like.. <?php require_once 'image.class.php'; //My Class $im = new Image('/path/to/image'); $im->resize(width, height); //Or if you want to do it by a % proportionally.. $im->resize(null, null, true, %); $im->size('someplace.png/jpg/gif'); There's also a lot of other options included in the class. For example you can crop (which also offers different location, CENTER_LEFT, CENTER_RIGHT, .. 9 in total). Here's the class with some documentation/examples I wrote for it: http://pastesite.com/10199
-
The source of the file must be in a string, not in an array, file() returns an array.
-
I think this will work: /\<a href="member.php?u=(.+?)"\>(.+?)\<\/a\>/
-
At first glance I see 2 things: 1. You're missing a double quote: $query_rstcomments = "SELECT comment FROM comments; should be: $query_rstcomments = "SELECT comment FROM comments"; 2. For a line break "\n" to work it must be wrapped in double quotes, otherwise it'll just be read as a string. So.. $comment = $comment . '\n' . $rec['comment']; Should be: $comment = $comment . "\n" . $rec['comment'];
-
For that it's a bit different, you'd store the selected value and do something like this: Storing: $selected = mysql_real_escape_string($_POST['select-menu']); //Query Then for displaying it with the selected one: //Query.. $items = Array('Menu Option 1', 'Menu Option 2', 'Menu Option 3'); foreach($items as $item) { $menu .= ($row['selected'] == $item) ? "<option value='$item' selected='selected'>$item</option>" : "<option value='$item'>$item</option>"; } Then $menu would contain the contents of the <select..></select>.
-
You can store 1 for a checked box and 0 for an unchecked box. So.. Inserting: $checkbox = (isset($_POST['some-check-box'])) ? 1 : 0; //Insert $checkbox into mysql.. Getting back from the database: //Query etc... $checkbox = ($row['checkbox'] == 0) ? NULL : 'checked="checked"'; echo "<input type='checkbox' name='whatever' $checkbox />";
-
You're probably going to want to store each genetic trait in a separate element of an array. You can build in the string at the end. To do probability you can do something like this: if(rand(0,2) == 0) { //1/3 Chance }
-
What are you expecting/trying to get?
-
[SOLVED] Fatal error: Function name must be a string
Alex replied to heldenbrau's topic in PHP Coding Help
$headline = headingsafe($_POST['headline']); -
edit: nvm, use array_search()
-
Like this? $arr = Array( 'key1' => 'value', 'key2' => 'value'); echo implode(', ', array_keys($arr)); Output: key1, key2 Edit: That's easier, lol.
-
header('Location: ..'); is used for redirecting and include() is not, include is used for including other files, not re-routing at all.
-
It's regex (Regular Expressions)
-
Basically yes, but you don't need the "s on the CURLOPT_URL, it's a constant not a string. $Something = CURLOPT_URL;
-
[SOLVED] how to use require() problem
Alex replied to southampton_web_designer's topic in PHP Coding Help
You can use file_exists() to confirm that the file exists in the location you're requesting it from. -
<?php $limit = 45; for($i = 1;$i <= $limit;$i++) { if(!empty($_POST['txtRow' . $i])) { //etc.. } } ?>
-
This isn't a PHP question, it's html/css. <span style="color: #FF7113;">Colored Text</span>
-
Np, just remember to press the "topic solved" button on the bottom left.