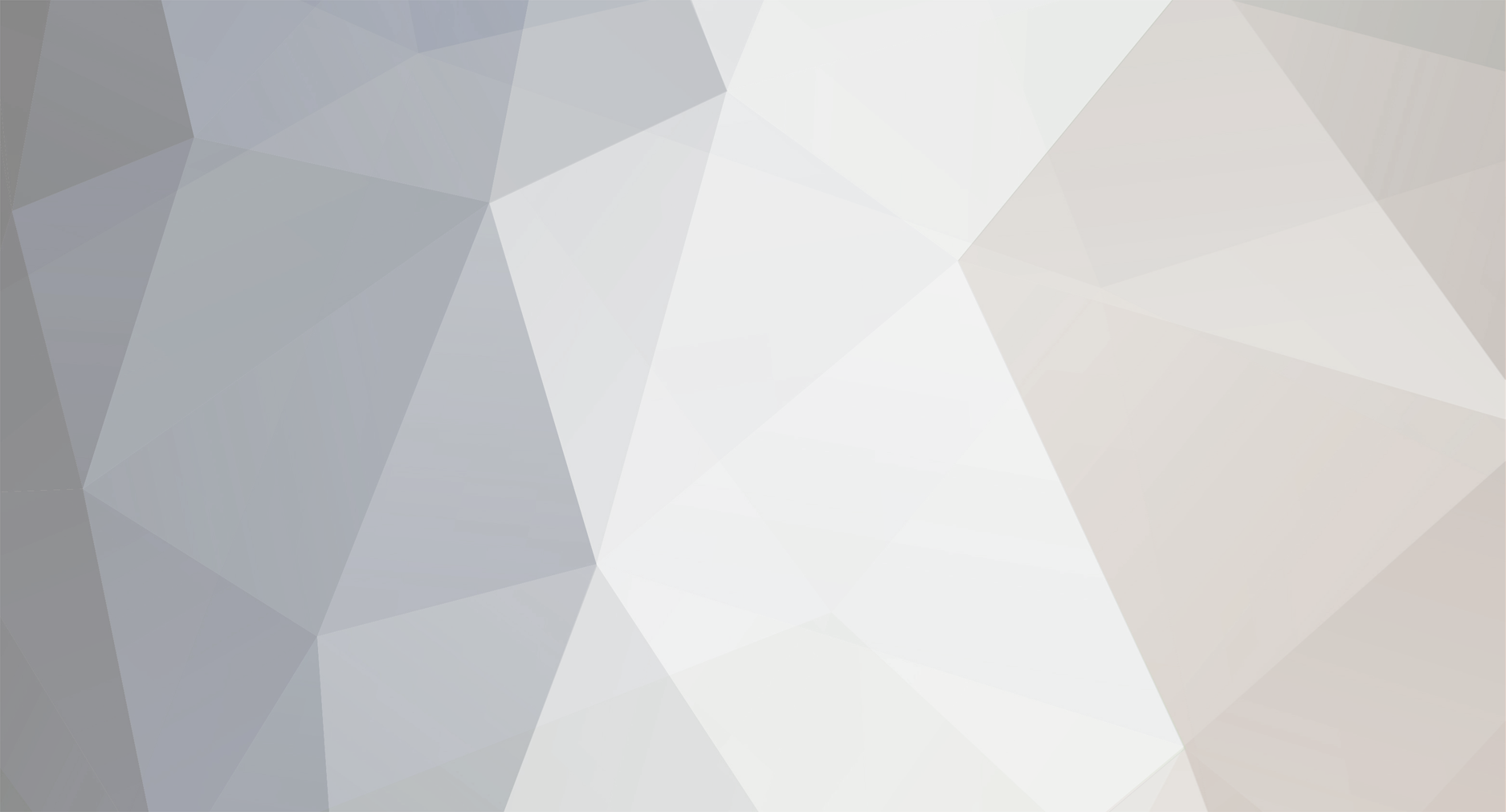
Cetanu
-
Posts
395 -
Joined
-
Last visited
Never
Posts posted by Cetanu
-
-
I get this error message after logging in:
Can't connect to local MySQL server through socket '/var/run/mysqld/mysqld.sock' (2)The script for logging in:
<?php session_start(); if(!isset($_POST['login'])){ echo "<h3>Log In</h3><br/> <form action='../index.php' method='post'> <label for='name'>Username</label> <input type='text' name='name' id='name' maxlength='16'/> <br/> <label for='pass'>Password</lable> <input type='password' name='pass' id='pass'/> <br/> <input type='submit' name='login' value='Log In'/><br/> </form>"; } else{ include "includes/db.php"; $user = protect($_POST['name']); $pass = protect($_POST['pass']); if($user && $pass) { $pass = md5($pass); //compare the encrypted password $sql="SELECT id,username FROM `d9_users` WHERE `username`='$user' AND `password`='$pass'"; $query=mysql_query($sql) or die(mysql_error()); if(mysql_num_rows($query) > 0) { $row = mysql_fetch_assoc($query); $_SESSION['id'] = $row['id']; $_SESSION['username'] = $user; header('Location: http://d9fans.freezoka.com'); } else { echo "Username - Password Combination Incorrect."; echo "<a href=\"../index.php\"> Try Again!</a>"; } } else { echo "<script type=\"text/javascript\"> alert(\"You need to gimme a username AND password!\"); window.location=\"../index.php\"</script>"; } } ?>
The script for what happens after you log in:
<?php include "includes/db.php"; $result = mysql_query("SELECT * FROM d9_users WHERE username='{$_SESSION['username']}'") or die(mysql_error()); while($row = mysql_fetch_array( $result )) { if($row['species'] == "Non Human"){ echo "<img src='images/nhdisplay.png' class='center' alt='Non Human'/><br/>"; } if($row['species'] == "Human"){ echo "<img src='images/hdisplay.png' class='center' alt='Human'/><br/>"; } echo "Hey there, ".$_SESSION['username']."<br/>"; } ?>
-
Can you write $_POST to a txt file? I was wondering if that could be the error.
-
I like the design. My only things are that the header and footer seem slightly too big. The header could be a little smaller, and in the footer to make it smaller couldn't you just display those links inline? Then they won't be tall and obtrusive, they'll be small and compact.
Holy crap, the XHTML AND CSS are valid. That's a first for me! Very good job.
Other than that, I really like it! Nice job.
-
The new code that won't write to my file is....:
<?php if(!isset($_POST['submit'])){ echo " <h2>Contact the Admin</h2> <form action='contact.php' method='post'> Name: <input type='text' maxlength='20' name='name'/><br/> Email: <input type='text' name='email'/><br/> Subject: <input type='text' maxlength='50' name='subj'/><br/> Body: <br/> <textarea cols='50' rows='5' name='body'></textarea><br/> Date: <input type='text' value='".date("D, M d, Y")."' readonly='readonly' name='date'/><br/> <input type='submit' name='submit' value='Contact'/> | <input type='reset' value='Clear'/> </form>"; } else{ if(!$_POST['name'] || !$_POST['email'] || !$_POST['subj'] || !$_POST['body'] || !$_POST['date']){ echo "<strong>Fill in all required fields. <a href='contact.php' title='Back'>Try again.</a></strong>"; } $contactfile = fopen("contact.txt" , "w+"); fwrite($contactfile , "Name: ".$_POST['name']."<br/> Email: ".$_POST['email']."<br/> Subject: ".$_POST['subj']."<br/> Body: ".$_POST['body']."<br/> Date: ".$_POST['date']."<br/><br/><br/>"); fclose($contactfile); echo "<script>alert('Successful.'); location='contact.php';</script>"; } $filec = fopen("contact.txt" , "w+"); while(!feof($filec)){ echo fgets($filec); } fclose($filec); echo "</p>"; ?>
-
*shakes head*
I'm so stupid sometimes.
-
I have a simple question, will
h3:first letter{ color: #fff; }
Work? Right now I am trying that and it won't work for me.
-
Nevermind, I just modified the width of <div id="whole'></div>
-
Well that helped that problem, the next problem is that now the page extends to the right past the normal width so that there is just empty space. I'm looking into that now, but it you have any ideas, help?
-
I set it to w+ and it wasn't working... :-\
-
Same error. :'(
I FEEL LIKE A RETARD.
mysql_query("INSERT INTO shop (item_name, item_description, price, quantity, species)VALUES('$name', '$description', $price, $quant, '$species'") or die(mysql_error());
That was was what threw the error. I dunno HOW we didn't notice that there wasn't the required first ) to end the VALUES part. The correct syntax is:
mysql_query("INSERT INTO shop (item_name, item_description, price, quantity, species)VALUES('$name', '$description', $price, $quant, '$species')") or die(mysql_error());
Thanks for your help.
-
INSERT INTO shop (item_name, item_description, price, quantity, species) VALUES('Netgun' , 'A ranged weapon that ensnares and kills enemies in its metal net. Attack +5 Honor -10 ' , 1800 , 1 , 'Predator'
-
Oooooh yeah, I'm such an idiot! I forgot all about the integers not needing '.
I've removed them, but still get an error message.
-
After removing the {} it echoed my query back to me (because I removed mysql_query() )
But then, when I put the mysql_query back on, it gives me an error again.
This is the query when it's echoed
INSERT INTO shop (item_name, item_description, price, quantity, species) VALUES('Netgun' , 'A ranged weapon that ensnares and kills enemies in its metal net. Attack +5 Honor -10 ' , '1800' , '1 ' , 'Predator' -
It looks a little too....plain/basic for me. I like websites that showcase design elements, but this site seems plain (as I said before
). It's hard to explain, maybe the content could be displayed differently...maybe more than one font could be used.
In your CSS one error was present:
for <h3> you wrote paddin rather than padding.
Also, mailto: is used a lot, but I've heard that that is a lure for spam bots. Perhaps mail () could be used? Or just your email address and no mailto:
Well, it's okay, I just don't like the design.
-
Okay.
By the way, new error:
You have an error in your SQL syntax; check the manual that corresponds to your MySQL server version for the right syntax to use near 'A ranged weapon that ensnares and kills enemies in a metal net. Attack +5 Honor ' at line 2>_> I'll go echo it into a variable.
UPDATE:
$query = mysql_query("INSERT INTO shop (item_name, item_description, price, quantity, species) VALUES('{$name}' , '{$description}' , '{$price}' , '{$quant}' , '{$species}'") or die(mysql_error()); echo $query;
Yields nothing, just the error, which has changed back to the first one.
-
Oh, simple enough, then. I'll do that.
You have an error in your SQL syntax; check the manual that corresponds to your MySQL server version for the right syntax to use near '' at line 2 -
It tells me there's an error anyway after I take out the `````
I dunno how to USE mysql_real_escape_string() what goes in the () part?
-
For some reason, it's telling me that this is wrong:
<?php if($_SESSION['username']=="Admin" || $_SESSION['user_id']=="Chris P" || $_SESSION['user_id']=="Cetanu"){ echo "<br/><br/><form action='shop.php' method='post'> <strong>Adding an Item</strong><br/><br/> Name: <input type='text' maxlength='30' name='name'/><br/> Description:<br/> <textarea rows='5' cols='50' name='description'>Be sure to remember the +attack, -attack, etc.</textarea><br/> Quantity: <input type='text' name='quant'/><br/> Price: <input type='text' name='price'/> <br/> <select name='species'> <option value='Predator'>Predator</option> <option value='Alien'>Alien</option> <option value='Marine'>Marine</option> </select><br/> <input type='submit' name='add' value='Confirm Add'/> | <input type='reset' value='Reset'/> </form> "; if(isset($_POST['add'])){ if(!$_POST['name'] || !$_POST['description'] || !$_POST['price'] || !$_POST['quant'] || !$_POST['species']){ echo "<script>alert('Try Again, and fill in all fields.'); location='shop.php';</script>"; } include "db.php"; mysql_query("INSERT INTO shop (item_name, item_description, price, quantity, species) VALUES(`'{$_POST['name']}'` , `'{$_POST['description']}'` , `'{$_POST['price']}'` , `'{$_POST['quant']}'` , `'{$_POST['species']}'`") or die(mysql_error()); echo "<script>alert('Item Added');</script>"; } } else{ echo ""; } ?>
When I enter my information it tells me there is an error in my syntax, but I've done this before and it usually works fine.
-
The design isn't exactly appealing, quite frankly there is too much to distract the eye on the home page from the search box, and the results page from the search results.
The colour scheme is too complex (too many colours), also, the choice of colour would be better suited for an audience of babies, mums etc. It's the colours you would usually find on toys designed for young children.
I don't really care about what other people have been searching for, when I hit the site it wasn't obvious what these links were, it seems as if you were trying to play up to the search engines, this just screamed link farm to me. Also, you have explicit links in your recently searched box, this is a massive no no.
Also, why do I want my search term shared with the rest of the world? This seems a little backwards to me.
I guess the issues boil down to the same problem at the end of the day, when creating a service that competes directly with Google, what are your USPs? Becuase if you don't have at least one that will blow my socks off, you can be pretty sure it won't be successful.
Just my 2c
I agree with all of that ^.
The cube seemed like those blocks babies play with, the colors screamed maternity ward, and my eyes went all over the place with those colored links, not to mention, times new roman normally makes websites a little boring for some reason.
AND, in the search results where it says "Latest Keyword" (one of the major downfalls of the site) it says XXX and PORN in the links. Niiiiiiiiiiiiiiiiiice. The colors appeal to moms and the keywords appeal to creepy old guys sitting in their shacks.
-
-
Oh, thanks for enlightening me as to why all of the fopens I saw had "r"
It fixed the error, but now it's not writing to my file.
-
Okay. ...
Didn't work, all it did was move the sidebar all the way over with the text. They still overlap, too.
-
I want to have it so that members can ask for help by submitting a query and having their posted values inserted into a file. It won't work, when I click Contact it says this:
ERRORThe requested URL could not be retrieved
While trying to retrieve the URL: http://d9fans.freezoka.com/contact.php
The following error was encountered:
Zero Sized Reply
Squid did not receive any data for this request.
Your cache administrator is webmaster.
Code:
<?php if(!isset($_POST['submit'])){ echo " <h2>Contact the Admin</h2> <form action='contact.php' method='post'> Name: <input type='text' maxlength='20' name='name'/><br/> Email: <input type='text' name='email'/><br/> Subject: <input type='text' maxlength='50' name='subj'/><br/> Body: <br/> <textarea cols='50' rows='5' name='body'></textarea><br/> Date: <input type='text' value='".date("D, M d, Y")."' readonly='readonly' name='date'/><br/> <input type='submit' name='submit' value='Contact'/> | <input type='reset' value='Clear'/> </form>"; } else{ if(!$_POST['name'] || !$_POST['email'] || !$_POST['subj'] || !$_POST['body'] || !$_POST['date']){ echo "<strong>Fill in all required fields. <a href='contact.php' title='Back'>Try again.</a></strong>"; } $contactfile = fopen("contact.txt"); fwrite($contactfile , "Name: ".$_POST['name']."<br/> Email: ".$_POST['email']."<br/> Subject: ".$_POST['subj']."<br/> Body: ".$_POST['body']."<br/> Date: ".$_POST['date']."<br/><br/><br/>"); fclose($contactfile); echo "<script>alert('Successful.'); location='contact.php';</script>"; } $filec = fopen("contact.txt"); while(!feof($filec)){ echo fgets($filec); } fclose($filec); ?>
-
Heh.
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Strict//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-strict.dtd"> <html xmlns="http://www.w3.org/1999/xhtml" lang="en" xml:lang="en"> <head> <title>D9 Fans</title> <link rel="stylesheet" type="text/css" href="style.css" /> </head> <body> <div id="whole"> <div id="nav"> <img src="/images/d9fans.png" class="head" alt="D9Fans"/> <ul> <li><a href="register.php">Register</a></li> <li><a href="contact.php">Contact</a></li> <li><a href="#" class="selected">Home</a></li> </ul> </div> <div id="whole"> <div class="clear"></div> <div id="sidebar"> <fieldset> <legend><img src="images/d9header.png" alt="MNU Classified"/></legend> Test Form! <form> <input type="text" name="uname"/><br/> <input type="password" name="password"/><br/> <input type="submit" name="submit" value="Log In"/> </form> </fieldset> <div class="clear"></div> <br/> <fieldset> <legend><img src="images/d9header.png" alt="MNU Classified"/></legend> The news could go here. Maybe user info. I dunno, do YOU? </fieldset> </div> <p> District 9 is a movie that came out in August of 2009. It focuses around an extraterrestrial race (derogatory name: prawns) that has become stranded on Earth. The main characters are Christopher (prawn) and Wilkes (MNU Personnel)...BLAHBLAHBLAHBLAHBLAH. <br/> |<br/> | <br/> | <br/> |<br/> </p> </div> <div class="clear"></div> <a href="http://mythscape.freezoka.com/"><img src="/images/cetnet.png" alt="Cetanu Network!" class="center"/></a> </div> </body> </html>
Connecting Error
in PHP Coding Help
Posted
I used that to connect for registering and it worked...