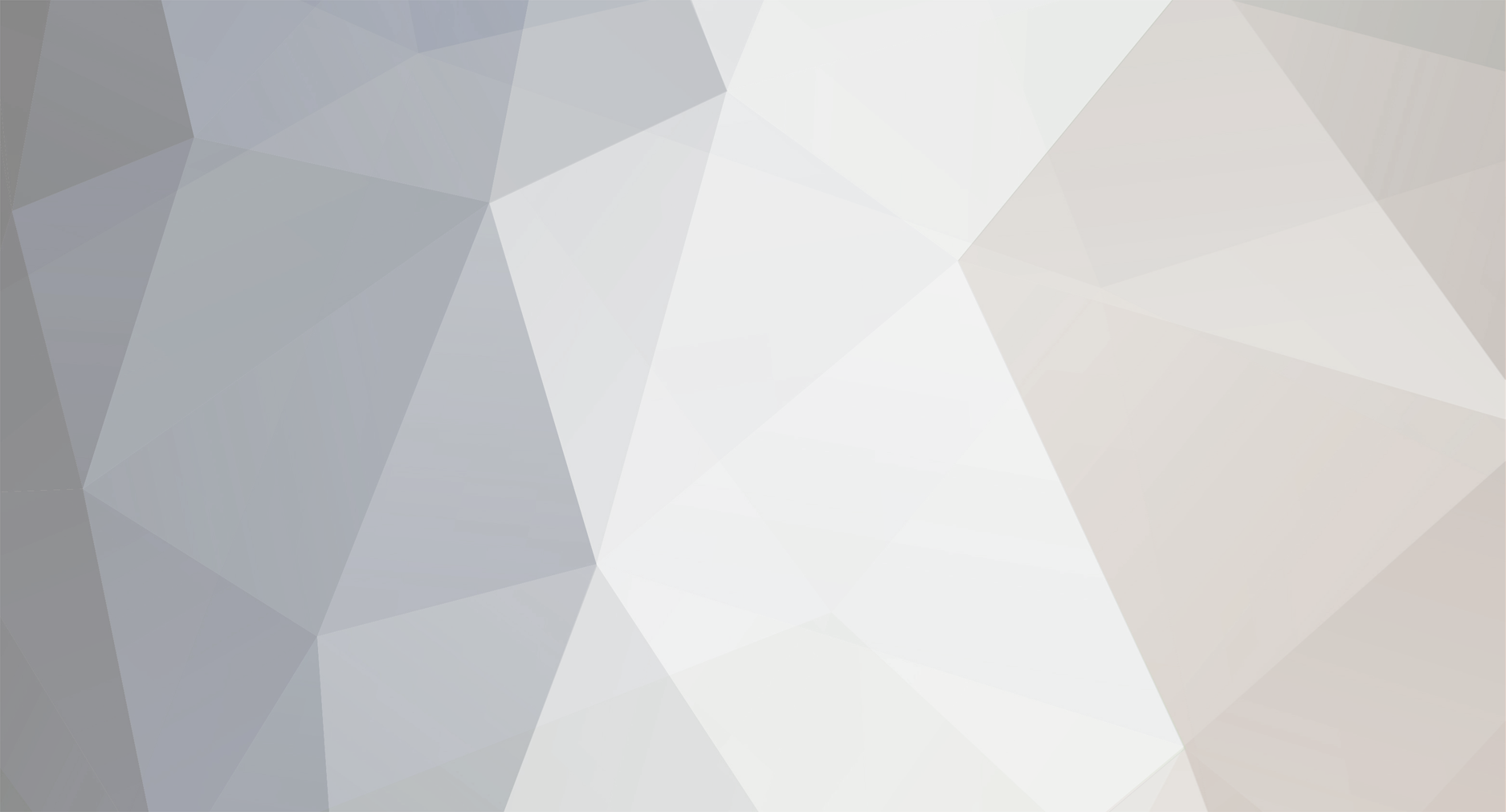
gamblor01
Members-
Posts
73 -
Joined
-
Last visited
Everything posted by gamblor01
-
Showing and disabling specific data from mysql
gamblor01 replied to Darkwoods's topic in PHP Coding Help
D'oh! Thanks for spotting that MadTechie. I have fixed it now (and also added the <?php ?> tags for syntax highlighting). Note however, that I might need to "cougth" at your typo too. -
Showing and disabling specific data from mysql
gamblor01 replied to Darkwoods's topic in PHP Coding Help
I believe what you are asking about is a simple OR statement? You cannot do it as you have written though...it's not like you can test whether id is in the set '2,5' (though you could do this in a WHERE clause like 'AND NOT IN (2,5)'). The == operator will expect exactly one value on both sides. Therefore this statement is incorrect: <?php if ($id == '2,5') { // do stuff } ?> You need to test each value individually with an OR statement (written || ), so the following should work: <?php if ($id == 2 || $id == 5) { // do stuff } ?> If you want to print every row EXCEPT rows 2 and 5 then you can either negate the OR statement, or you can distribute the negation over the entire statement (using DeMorgan's Law) to obtain an AND (&&) statement. Both of the if tests below would be acceptable to print everything except for rows 2 and 5: <?php if (!($id == 2 || $id == 5)) ?> <?php if ($id != 2 && $id != 5) ?> So if you want to print every row except 2 and 5 in a table called foo (let's assume it only has 2 columns: id and name), then I would expect the code to look something like this: <?php $result = mysql_query("SELECT * FROM foo"); while($row = mysql_fetch_array($result)) { $id = $row['id']; // don't print rows 2 and 5 if ($id !=2 && $id !=5) { $name = $row['name']; echo "$id - $name"; } } ?> -
So I figured out how to do it, though some may claim it's a bit of a hack. Basically what I wound up doing is having AJAX populate a div tag in a "parent" HTML document (see the stateChanged() function and you will see that it specifically writes the data to the "myTable" div tag). The idea is that the entire HTML content (the table and everything) is printed by a script that is invoked within the parent document. Certainly if someone had more content to write to the page then this form and table then that could be static content contained outside of the myTable div tag -- and therefore only this small portion of the page's content would be reloaded. Initially, the div tag contains a single space character, and I use the body onload option to populate the page initially. Whenever a button is pushed, it invokes the AJAX code to reload the page -- the showTable function is called and I pass the child page (getTable.php) the appropriate arguments. Depending on what arguments are passed to getTable.php it will either add a row to the table, delete it, or "do nothing". In all three cases it prints the table afterwards into the myTable div tag, replacing what was previously there. One thing I learned from this whole experience (which I think is pretty neat) was that the "child" page can invoke scripts that are written in the parent page as if the entire document was all one large, single HTML file. Nifty! This particular implementation is for adding and deleting "users" from a MySQL database. The code simply adds or deletes users (rows) from the database. The PHP scripts I have show the table and displays a form that allows one to add new rows to it. This is just one implementation -- I'm sure there are others and I'm sure that there is a better way to implement parts or all of this. If anyone has any suggestions, I would love to know. I particularly believe that the HTTP GET request is not implemented well. The AJAX code invokes a PHP script and passes it parameters by appending to the end of the string such as: getTable.php?action=delete?newusername=12 Normally when I do a GET or POST through a form then I can use $_GET or $_POST in the backend script to retrieve the values. Inside of getTable.php I have to do a bunch of substrings in order to parse the string...using $_GET doesn't seem to work for some reason (I'm not savvy enough yet to know why). So here is the "parent" page that I wrote. Note that I have trimmed down some of the code because I have some session data going on, checking for admin rights (only admins can add/delete users), and so on. I wouldn't recommend using this for some sort of secure user administration, but I do think it's a good example: <html> <head> <script type="text/javascript"> var xmlhttp; function showTable(str,user,newpw1,newpw2,admin) { xmlhttp=GetXmlHttpObject(); if (xmlhttp==null) { alert ("Sorry but your browser does not support AJAX or Javascript has been disabled"); return; } // we always want to call getTable var url="getTable.php"; url=url+"?action="+str; if (str=="add") { // we're adding so append new user info in GET request url=url+"?newusername="+user; url=url+"?newpassword="+newpw1; url=url+"?newpassword2="+newpw2; url=url+"?admin="+admin; } else if (str=="delete") { var doAction = confirm("Are you sure you want to delete id #"+user+"?"); if (doAction == true) { // we're deleting so append userID in the GET request url=url+"?newusername="+user; } else { // not deleting so reset the url url = "getTable.php?action=nothing" } } // execute the fully formed request xmlhttp.onreadystatechange=stateChanged; xmlhttp.open("GET",url,true); xmlhttp.send(null); } function stateChanged() { if (xmlhttp.readyState==4) { document.getElementById("myTable").innerHTML=xmlhttp.responseText; } } function GetXmlHttpObject() { if (window.XMLHttpRequest) { // code for IE7+, Firefox, Chrome, Opera, Safari return new XMLHttpRequest(); } if (window.ActiveXObject) { // code for IE6, IE5 return new ActiveXObject("Microsoft.XMLHTTP"); } return null; } </script> </head> <body onload="showTable('first',null,null,null,null)"> <div id="myTable"> </div> </body> </html> And then here is the "child" script, getTable.php: <?php ob_start(); $host="localhost"; // Host name $username="root"; // Mysql username $password="xxx"; // Mysql password $db_name="test"; // Database name $tbl_name="users"; // Table name // Connect to server and select database. mysql_connect("$host", "$username", "$password")or die(mysql_error()); mysql_select_db("$db_name")or die("cannot select DB"); // get the value of action $action = $_GET['action']; if (substr($action,0,3) == "add") { // get the rest of the values -- start by stripping off "add?" $action = substr($action, 4); // newusername is first so strip it and then get the first "?" $action = substr($action, 12); // newusername= is 12 chars $pos = strpos($action, "?"); $newusername = substr($action, 0, $pos); $action = substr($action, ++$pos); // now get the newpassword value $action = substr($action, 12); // newpassword= is 12 chars $pos = strpos($action, "?"); $newpassword = substr($action, 0, $pos); $action = substr($action, ++$pos); // now get the newpassword2 value $action = substr($action, 13); // newpassword2= is 13 chars $pos = strpos($action, "?"); $newpassword2 = substr($action, 0, $pos); $action = substr($action, ++$pos); // now get the admin value $action = substr($action, 6); $admin = $action; // To protect MySQL injection $newusername = stripslashes($newusername); $newpassword = stripslashes($newpassword); $newpassword2 = stripslashes($newpassword2); $newusername = mysql_real_escape_string($newusername); $newpassword = mysql_real_escape_string($newpassword); $newpassword2 = mysql_real_escape_string($newpassword2); // check if the username already exists $sql="SELECT * FROM $tbl_name WHERE username='$newusername'"; $result=mysql_query($sql); // mysql_num_row counts table rows $count=mysql_num_rows($result); // If result matched $myusername table rows must be 1 row if($count==1) { echo "<center>"; echo "<p><font color=\"red\">User already exists</p></font>"; echo "</center>"; } else if ( $newpassword == $newpassword2) { $encrypted_mypassword = md5($newpassword); // check for admin authority if ($admin == "true") { $sql="INSERT INTO $tbl_name (username, password, isadmin) VALUES('$newusername', '$encrypted_mypassword', 1)"; } else { $sql="INSERT INTO $tbl_name (username, password, isadmin) VALUES('$newusername', '$encrypted_mypassword', 0)"; } mysql_query($sql); } else if ($newpassword != $newpassword2) { echo "<center>"; echo "<p><font color=\"red\">Passwords do not match</font></p>"; echo "</center>"; } } else if (substr($action, 0, 6) == "delete") { // get the correct user to be deleted using substr // delete?newusername= is 19 characters $delUser = substr($action, 19); // protect against MySQL injection $delUser = stripslashes($delUser); $delUser = mysql_real_escape_string($delUser); $sql="DELETE FROM members WHERE id=" . $delUser; mysql_query($sql); } // if the value of action was anything else then ignore it // and print the table without adding or deleting $sql = "SELECT * FROM members ORDER BY id"; $result = mysql_query($sql); // write a link back to the login_success page: ?> <form> <table width="300" border="0" align="center" cellpadding="0" cellspacing="1" bgcolor="#CCCCCC"> <tr> <td> <table width="100%" border="0" cellpadding="3" cellspacing="1" bgcolor="#FFFFFF"> <tr> <td colspan="3"><strong>Add a user</strong></td> </tr> <tr> <td width="78">Username</td> <td width="6">:</td> <td width="294"><input name="newusername" type="text" id="newusername" value=""></td> </tr> <tr> <td>Password</td> <td>:</td> <td><input type="password" name="newpassword" id="newpassword" value=""></td> </tr> <tr> <td>Repeat Password</td> <td>:</td> <td><input type="password" name="newpassword2" id="newpassword2" value=""></td> </tr> <tr> <td>Create as admin?</td> <td> </td> <td><input type="checkbox" name="admin" value=""></td> </tr> <tr> <td> </td> <td> </td> <td><input type="button" name="submit" value="Add User" onClick="showTable('add',this.form.newusername.value,this.form.newpassword.value,this.form.newpassword2.value,this.form.admin.checked);"></td> </tr> </table> </td> </tr> </table> <br><br> <?php // write the rest of the form echo "<center>\n"; // draw the table echo "<table border=1>\n"; echo "<tr bgcolor=\"#000000\">\n"; echo "<th><font color=\"#ffffff\">id</font></th>\n"; echo "<th><font color=\"#ffffff\">username</font></th>\n"; echo "<th><font color=\"#ffffff\">password</font></th>\n"; echo "<th><font color=\"#ffffff\">isadmin</font></th>\n"; echo "<td><font color=\"#ffffff\"> </font></th>\n"; echo "</tr>\n"; $rownum = 0; while ($row = mysql_fetch_array($result)) { if ($rownum % 2 == 0) { $color="#ffffff"; } else { $color="#cfd9c3"; } echo "<tr bgcolor=\"$color\">\n"; echo "<td>" . $row['id'] . "</td>\n"; echo "<td>" . $row['username'] . "</td>\n"; echo "<td>" . $row['password'] . "</td>\n"; echo "<td>" . $row['isadmin'] . "</td>\n"; echo "<td><input type=\"button\" value=\"Delete User\" onClick=\"showTable('delete'," . $row['id'] . ", null, null, null);\"></td>\n"; echo "</tr>"; $rownum++; } echo "</table>\n"; echo "</form>\n"; echo "</center>\n"; ob_end_flush(); ?> Oh -- and I almost forgot. My table in MySQL was created using something like this: CREATE TABLE users ( id integer not null auto_increment, username varchar(32) unique, password varchar(64), isadmin tinyint, primary key(id) );
-
Cool thanks! I'll check it out. I see that there is a defect in the code though -- it only wants to delete the last row added. But I'm sure I can tweak things and get it to work. I'll try anyway!
-
Hi all, I am not sure how much work this would be to accomplish, but right now I have a PHP script that is pulling users from a MySQL table and printing it in an HTML table. I currently have a button at the end of each row that I want to make a delete button. Essentially, administrative users should be able to view a complete list of all of the members and if desired, click the button on one of the rows to delete a member from the table. I could send a POST back to the server with the id of the row selected, delete the row in MySQL, and then refresh the page. However, it would be more desirable to just click the button and have the table automatically updated through ajax. This is basically the same way that gmail behaves. If I click on a message and select the delete button then the selected row(s) is/are deleted but the whole page doesn't refresh. I'm not really sure how to do this however -- it seems like I would need to pull the entire HTML for the table through ajax. Any ideas on how to accomplish this?
-
Hi everyone, I read through the w3 schools section for CSS, because I'm not very good with them. I am just playing around with things and I wanted to know how to properly setup a page so that, for example, I have 2 different "boxes". Let's suppose that I have a navigation menu on the left side of my page with hyperlinks it in. I want to define certain styles for the links in that navigation menu that are different than the ones defined in the body of the page. How would I accomplish this? For example, suppose I want the following in the "navigation" bar: a:link {color:#000000} a:visited {color:#000000} but then for my main page I want: a:link {color:#cfcfcf} a:visited {color:#cfcfcf} I'm looking at using ids or classes here, though I am not really sure what the most appropriate choice is. The w3 school's tutorial does not really clarify this to any extent: http://www.w3schools.com/css/css_id_class.asp Do I just do something like this: #menu { a:link {color:#000000} a:visited {color:#000000} } #content { a:link {color:#cfcfcf} a:visited {color:#cfcfcf} } and then later in my HTML code do: <div id="menu"> <p>Visit <a href="www.google.com">www.google.com</a> to search for some stuff!</p> </div> <div id="content"> <p>Lots of content <a href="www.google.com">here!</a></p> </div> Is this the correct syntax? It doesn't seem to work when I try it so I'm guessing the answer is no. Ideally I would like to be able to specify all sorts of different formatting/colors/decoration/etc. for one section of a page, and change some or all of those in another section of the page. Thus, I would want some div tag with one id that has definitions for the a:* tags, the p tag, the hr tag, and so on -- and another div tag with a different id that has completely different definitions. Can anyone explain to me how this is done? If you can recommend a good CSS book or online tutorial that is fantastic as well. The w3 tutorial is OK but I need more.
-
Thanks laffin! Great info. I changed all of my pages over to use the isset() function instead of session_is_registered() and it works perfectly. FYI you have a typo in your code block...it says "is_set($_SESSION)" instead of "isset($_SESSION")" -- but I knew what you meant. Thanks everyone...I know have this working where I can add users or change passwords -- and both have error checking and everything works great! Looks like I have my work cut out for me. I have some pages to design.
-
I am going to bring this one back from the dead since I think it is an interesting and valid question. I have a question of my own about how to handle this stuff though. I had previously worked on some Perl/CGI stuff about 5 years ago in an internship. It used a MySQL backend and what we found was that we were constantly opening and closing handles to the database -- which caused a severe performance hit. So we eventually defined two new functions: 1. db_connect() -- connects to the database 2. get_db_handle() -- if the dbh == -1 then we call db_connect() and return the handle. Else we simply return the handle already stored This sped up performance SIGNIFICANTLY in the application. One page was drastically reduced from like 26 seconds to fully load to about 2 seconds. Granted, these database connections were being constantly created and torn down within a loop (i.e. code in the same file...the same function even). So it is a bit different to PHP which is only tearing down the connection when the user navigates to another page. I guess as long as your users aren't sitting there constantly between PHP pages, your code should be more than responsive. I still have the following question though: 1. What is the best way to maintain database connections. Should I just copy and paste the same mysql_connect() code into every PHP page that I write? I was thinking about defining two functions like I discussed above...one to get the db connection and another to create it (if it doesn't exist). Then, whenever I need the db connection in my code I can just do something like: $con = getDBConnection(); Instead of typing code like this over and over again: $host="localhost"; // Host name $username="root"; // Mysql username $password="topsecret!"; // Mysql password // Connect to server and select database. mysql_connect("$host", "$username", "$password")or die("cannot connect"); Is that the preferred way to handle this? Clearly typing out multiple lines of code again and again is much more error prone than typing a single line. I am just trying to figure out the good "rule of thumb" type stuff for MySQL connections in my PHP code. Thanks!
-
Cool...looks like I got things working. If you look at main_login.php in the tutorial from my first post, it does an HTTP POST in the form. The action is to call checklogin.php. Inside of checklogin.php I can save away the username in the session info (once I have verified the correct password) like so: // If result matched $myusername and $mypassword, table row must be 1 row if($count==1) { // save username away in the session $_SESSION['myusername'] = $myusername; // Register $myusername, $mypassword and redirect to file "login_success.php" session_register("myusername"); session_register("mypassword"); header("location:login_success.php"); } else { echo "Wrong Username or Password"; } And it works now! Later I can retrieve the username just by checking $_SESSION['myusername']. I'm not sure what the difference is between setting a variable in $_SESSION vs. calling session_register() though. If anyone knows I guess that would be my last question on this for now. According to the manual, session_register() is deprecated and using $_SESSION is now preferred. Apparently they are supposed to be equivalent: http://php.net/manual/en/function.session-register.php That's strange because you can see that the original code uses this line: session_register("myusername"); and yet I wasn't able to pull the value of $_SESSION['myusername'] until I explicitly set it using $_SESSION['myusername'] = $myusername; Thus, in my experience the two calls: session_register("myusername"); and $_SESSION['myusername'] = $myusername; Are NOT equivalent! So can someone answer the following: 1. Is assignment via $_SESSION['key'] = value and session_register() supposed to be equivalent? 2. Could I change the "protection" at the top of each page from this: <?php session_start(); if(!session_is_registered(myusername)){ header("location:main_login.php"); } ?> to this? <?php session_start(); if(!$_SESSION['myusername']){ header("location:main_login.php"); } ?> Are those equivalent?
-
Thanks teamatomic...I'll try some of your suggestions out today and see how it goes. Just FYI, the reason why I wanted to allow one user create another user is: a) Just to verify that I can actually write something useful...it's just a test I wanted to do b) I would eventually like to create a pages (or pages) that offer "admin" access. Essentially I want the content management to be built into the page, so I can create a page for someone, and then they can update the content contained in that page without having any knowledge of PHP or HTML (or maybe they just have a very basic understanding of HTML). Being able to create users is just a step in that direction. But I'll try out your suggestions right now and see how it goes. I guess I misunderstood the point of session_start(). It was my understanding that it was only supposed to be called on the page that started the session, but I guess you have to use it on every page contained in the session? If so, then the function name is a bit of a misnomer -- I'm not starting a session but continuing an existing one! In any case, thanks for the help so far.
-
Hi folks, I have a few random questions surrounding a PHP login application and passing session data around between pages. I started by following this tutorial, which seemed pretty good: http://www.phpeasystep.com/phptu/6.html This isn't my first time using MySQL or PHP, so I was able to get things up and running within minutes. But then I decided to try adding some real functionality. For example, I wanted the "login_success.php" page to have a few functions on it: 1. Be able to change the user's password 2. Be able to create a new user 3. Logout I got the logout to work just fine -- I can either display a "logout" confirmation page (with a link to go back to the main login screen), or just perform a redirect back to the main PHP page (main_login.php). Either one works. What I need help with is performing password changes and creating users. So let's take a look at my concerns with each one: 1. changing passwords: - I should only be able to change the current user's password. Therefore, I need to know the username in the current session. - A user should only be able to reach this page if they successfully logged in (and therefore have a valid session). 2. adding users - Again, a user should only be able to reach this page if they successfully logged in (and I will handle "admin" users exclusively having access to this page later). I see that the login_success.php page from the above tutorial has this code at the top: <? session_start(); if(!session_is_registered(myusername)){ header("location:main_login.php"); } ?> So my questions are: 1. Should I use this same code at the top of every page I wish to prevent "unauthorized" access to (i.e. the user needs to be logged in)? If not, how to protect pages like that? 2. What is the best way to pass session data around between pages? I looked at this tutorial: http://php.net/manual/en/function.session-start.php but the SID variable doesn't seem to work in my environment (PHP Version 5.2.6-3ubuntu4.5) like that page shows. Instead I have to save the result of session_id() to a variable. But should I be passing the session variable around between pages like this? Let's say I have a form in adduser.php which has 2 fields (new password and confirm password). Then the submit button calls adduser2.php?sessionIDHere. Then of course, the adduser2.php page will compare the two passwords, create a connection to mysql, and update the members table. Is this the best way to be changing pages (just simple <a href> hyperlinks)? It doesn't seem like it because then my session ID is up in the location bar of the browser. I have seen redirects done like this: header("location:someOtherPage.php"); Is it possible to pass session data around around using the header function? Sorry, I know there is a lot going on here, but it's really just the two questions...sort of.
-
Thanks garethp -- your expression worked like a champ! I know you're anchoring to the beginning of the expression but I'll have to really sit down and look it over at some point to truly understand what the heck it's doing.
-
Hi everyone, I have a regex that is looking for URLs in a line of text (actually I have several but only one is giving me problems that I know of). The idea is that I want to find strings of the form something <dot> something <dot> (2|3 characters). This will allow me to find things like: a.b.cd www.google.com hello.world.tv So here is the current line of code I am using: preg_match('/.+\..+\.([a-zA-Z]{2}|[a-zA-Z]{3})/i', $msg) The problem is that it is matching strings such as "forever...or" and I see why, I just don't know how to avoid it. Is there any way that I can specify in my regex to match everything of that form EXCEPT a string that contains "..." in it? Or do I simply need to rewrite the entire regular expression in a different way? Thanks!
-
Now THAT is the slick solution that I was looking for! It works beautifully...thank you!
-
Well the data isn't random, but the data is being pulled by a SQL query that pulls from one of many possible tables. Each table has a different number of rows in it, and it depends on which table the user selects. If they select TableA then I need to print 30 rows on a page. If they select table B then it's 7 rows, and so on. So the generic PHP code generating this page is just taking the rows returned and going through a loop to generate the HTML table. The idea is that the user would then be allowed to mark some of the rows as "questionable" and I need to know which rows those are. If I can't do this with checkboxes I suppose I could do this with a text both where they simply enter the row numbers that they think are questionable. Then I just pass that string when the form is submitted and I break it into separate tokens using comma/space/semicolon/colon/whatever as a delimiter. I might just code this up to use a text box where you enter the rows in a list for now (just to get it working) but ultimately I would really like to get it working with checkboxes. So far I don't really know how to do this without a gigantic if statement that has the same amount of conditions as the number of rows in the largest table. That sounds like a bad design. I'm just trying to figure out if there is something better.
-
I have a page that will be generating unpredictable numbers of checkboxes (well unpredictable until the time the HTML is generated). It's a data mining application and depending on the selection made by the user, they will presented with a table that may have 10 rows, 42, or 100. My vision is to have a checkbox on each row, and allow the user to select any of the rows they want to throw out of the training set for my classifier. Thus, I need to be able to submit the form to a php page and determine which checkboxes were selected because those are the data values that I need to throw out. I know that if I had a form with a set number of fields this would be really easy. But because this can have any number of fields, I'm not sure how to tell which boxes are checked and which ones are not. Is this possible? Thoughts?
-
[SOLVED] dynamic updates without reloading page
gamblor01 replied to gamblor01's topic in Javascript Help
See reply #7 above...it has the full code for convert.php -- it's just a simple if-else structure. @dpacmittal: It works!!!! Passing the object as an argument -- brilliant! If I should somehow randomly run into you on the street one day I owe you a beer! -
[SOLVED] dynamic updates without reloading page
gamblor01 replied to gamblor01's topic in Javascript Help
Thank you! Finally -- the buttons get updated! Seems there is still a logic error somewhere in here though. When I click on the "one" button for the first time, nothing happens. Then I click it again and it changes to "uno". Then I click on "two" and it changes to "uno". Then I click on "three" and it changes to "dos". You can keep clicking and eventually they will all change to the same value if you click things in a certain order. Any thoughts on that? -
[SOLVED] dynamic updates without reloading page
gamblor01 replied to gamblor01's topic in Javascript Help
Ah, well the GETT typo was actually my fault for typing in the code instead of pasting it from my file. I was trying to use POST at the time and pass my argument in the send() function, which didn't work either. I have switched back to using GET and I setup the string concatenation just like you mentioned. So keep the exact same convert.php that I posted above, and then I have the following (in what I call foo.php): <html> <script type="text/javascript"> function sendRequest(myMsg) { var xmlhttp; if (window.XMLHttpRequest) { // code for IE7+, Firefox, Chrome, Opera, Safari xmlhttp=new XMLHttpRequest(); } else if (window.ActiveXObject) { // code for IE6, IE5 xmlhttp=new ActiveXObject("Microsoft.XMLHTTP"); } else { alert("Your browser does not support XMLHTTP!"); } xmlhttp.onreadystatechange=function() { if(xmlhttp.readyState==4) { return xmlhttp.responseText; } } xmlhttp.open("GET","convert.php?message="+myMsg,true); xmlhttp.send(null); } </script> <body> <?php echo "<table>"; echo "<tr>"; echo "<td align=\"center\"><input type=\"button\" value=\"one\" onClick=\"this.value=sendRequest('one');\"></td>"; echo "</tr>"; echo "<tr>"; echo "<td align=\"center\"><input type=\"button\" value=\"two\" onClick=\"this.value=sendRequest('two');\"></td>"; echo "</tr>"; echo "<tr>"; echo "<td align=\"center\"><input type=\"button\" value=\"three\" onClick=\"this.value=sendRequest('three');\"></td>"; echo "</tr>"; echo "</table>"; ?> </body> </html> It doesn't work. When I click on a button it changes the text of the button to "undefined". Can you try it and see what happens on your server? Mine doesn't work. -
[SOLVED] dynamic updates without reloading page
gamblor01 replied to gamblor01's topic in Javascript Help
Ok I almost have it working. It's definitely invoking my PHP script but I'm not sure that I am passing it a value properly, because it seems to be missing the value that I am passing. So let's take a really simple example, and if we can get this working then I should be able to get my app working no problem. In this example, I have 3 buttons with text values "one", "two", and "three". My plan is that when I click on the button it simply changes the text on the button to "uno", "dos", or "tres". So I create a simple table with buttons in PHP: echo "<table>"; echo "<tr>"; echo "<td align=\"center\"><input type=\"button\" value=\"one\" onClick=\"this.value=sendRequest('one');\"></td>"; echo "</tr>"; echo "<tr>"; echo "<td align=\"center\"><input type=\"button\" value=\"two\" onClick=\"this.value=sendRequest('two');\"></td>"; echo "</tr>"; echo "<tr>"; echo "<td align=\"center\"><input type=\"button\" value=\"three\" onClick=\"this.value=sendRequest('three');\"></td>"; echo "</tr>"; echo "</table>"; And I create the following function in javascript: <script type="text/javascript"> function sendRequest(myMsg) { var xmlhttp; if (window.XMLHttpRequest) { // code for IE7+, Firefox, Chrome, Opera, Safari xmlhttp=new XMLHttpRequest(); } else if (window.ActiveXObject) { // code for IE6, IE5 xmlhttp=new ActiveXObject("Microsoft.XMLHTTP"); } else { alert("Your browser does not support XMLHTTP!"); } xmlhttp.onreadystatechange=function() { if(xmlhttp.readyState==4) { return xmlhttp.responseText; } } xmlhttp.open("GETT","convert.php?message=myMsg",true); xmlhttp.send(null); } </script> Then I have the following in convert.php: <?php $val = $_GET["message"]; if ($val == "one") { echo "uno"; } else if ($val == "two") { echo "dos"; } else if ($val == "three") { echo "tres"; } ?> This example doesn't work and I'm not sure why yet. I'm guess it's either in the way I am invoking the convert.php script or the way I am returning the value in my javascript code. Something in there is broken. If you can identify what that would be fantastic! -
[SOLVED] dynamic updates without reloading page
gamblor01 replied to gamblor01's topic in Javascript Help
@dpacmittal: I don't follow your second block of code there at all. The first one makes sense however. In fact, it almost seems to be working properly. I am writing code like this: echo "<td align=\"center\"><input type=\"button\" value=\"No Value\" onClick=\"this.value=sendRequest('$msg');\"></td>"; I wrote sendRequest() like so: function sendRequest(myMsg) { mineData.php?message=myMsg; } All the php script does is echo some output. I have tested calling the script by typing the url into a browser and it works. So the page is rendering all of the buttons properly, but when I click on a button it doesn't seem to do anything. I tried adding in the following line to the sendRequest() function: alert('hello world'); It's not working though. I would expect that when I click the button the alert will pop up saying 'hello world' but it does not. I'm also looking for some files to be creating in my /tmp directory by the call to mineData.php and they are not. So clearly, clicking on the button is not invoking the javascript function. Any idea why not? UPDATE: If I have the function ONLY show the alert then it works fine, and I get the pop-up. Adding the call to my php script back in causes the button to stop working again. I must not be using the correct syntax to invoke that page. I also tried returning that value but it didn't work. Any idea what I am doing wrong? I'm so close! I'm thinking the problem is that I cannot invoke my PHP script unless I use an xmlhttp object, which goes back to the AJAX stuff. Is that correct? -
[SOLVED] dynamic updates without reloading page
gamblor01 replied to gamblor01's topic in Javascript Help
Cool thanks. I was actually hoping the term AJAX woudn't come up because I have never looked into it yet, but I guess it's time! I did a quick google search and found the following tutorial: http://www.w3schools.com/Ajax/ajax_intro.asp It's a great tutorial and all, but I still have one question. In my description above I mentioned that I wanted a button for every row in the table. The problem is that there is no fixed number of rows. It may be 7, it may be 37, it may even be 104. In the sample code they provided, they hardcoded "document.myForm.time.value" into the xmlhttp.onreadystatechange function: xmlhttp.onreadystatechange=function() { if(xmlhttp.readyState==4) { document.myForm.time.value=xmlhttp.responseText; } } Let's suppose that my table has 100 rows. Does this mean that I need to generate 100 different functions like this: xmlhttp.onreadystatechange1=function() { if(xmlhttp.readyState==4) { document.myForm.button1.value=xmlhttp.responseText; } } xmlhttp.onreadystatechange2=function() { if(xmlhttp.readyState==4) { document.myForm.button2.value=xmlhttp.responseText; } } ... If so, I suppose I can generate this with a loop in PHP; it's not hard to create a loop that echoes out these few lines (with the counter in it to change the button number each time). I'm just curious if there is perhaps a better method so that I could have just a single onreadystatechange function. In this manner I would just click on a button, the browser would request the response from the server, and then when the response came back...is there some way to know specifically which button performed the action so that I can apply the change to that button? I'm wondering if this is saving me anything though. It is starting to sound like I would have to generate one large onreadystatechange function that contains an if statement with 100 different possible cases. Any thoughts? -
Hi everyone, I am relatively new to PHP (though well versed in shell scripts, Java, and C/C++) and unfortunately the search function here seems to be down at the moment, so this may be answered already but I just cannot find it. I was wondering if anyone has a good tutorial on dynamically updating content within a page, without having to reload the entire page. For example, on Facebook if 3 of my friends post new updates while I am sitting on the homepage, Facebook will display a message that says "3 new updates". This message just appears to be "magically" inserted (i.e. the entire page is not refreshing in my browser). If I click it, instead of reloading the entire homepage, it again dynamically inserts the content into the page instead of reloading the entire home.php. For my particular example, I plan on having a table in my page. The idea that the last column would contain a button, and the user could click on the button to analyze content in the rest of the table (it's a data mining application). I want this to kick off some action in the background (for example: I want to run a shell script), and then when that shell script finishes I would like to dynamically update the column so that it no longer shows the button, but instead it shows the output of my shell script. Can anyone tell me how to do this, or direct me to a good how-to?