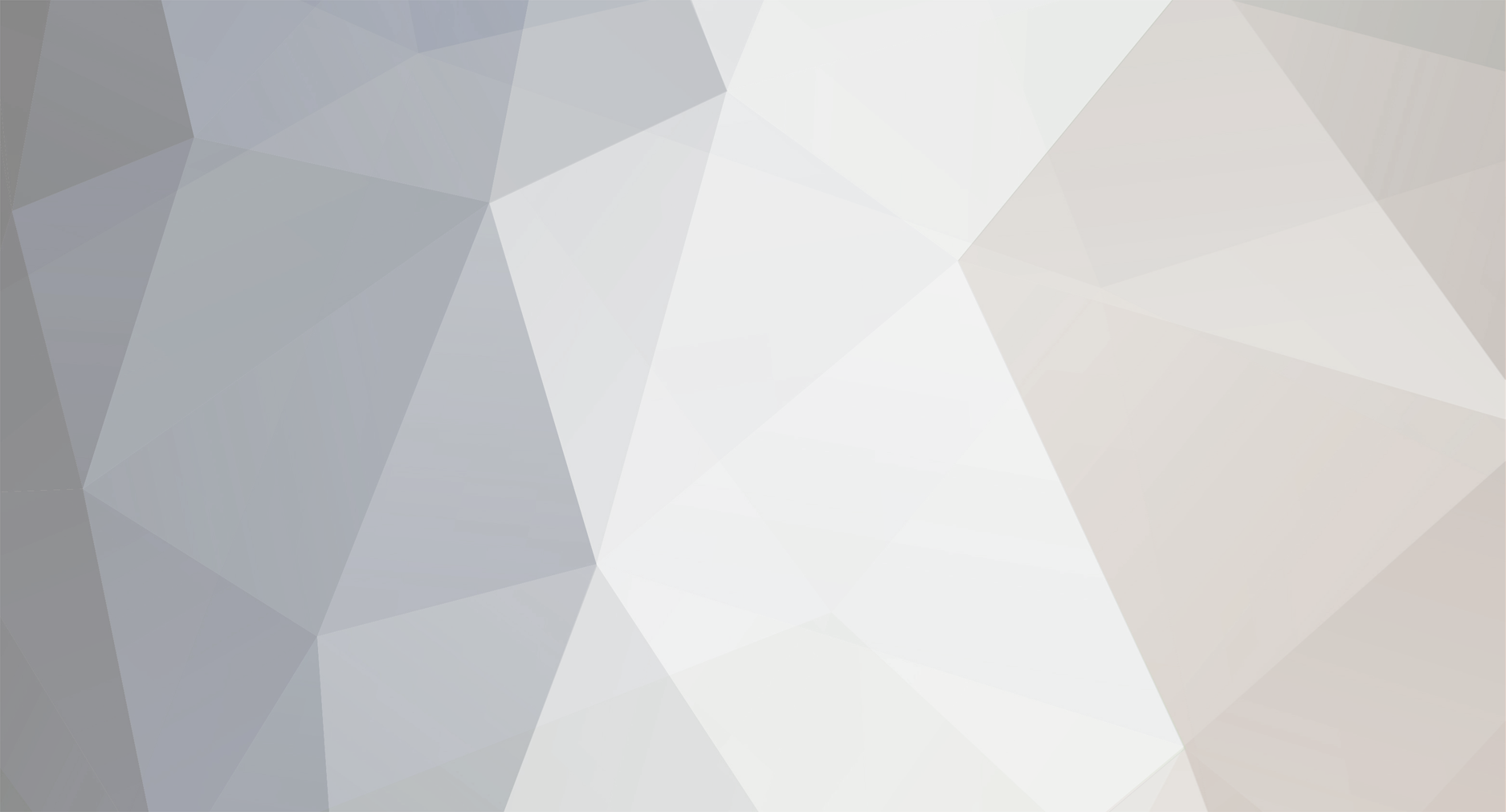
unknown1
-
Posts
128 -
Joined
-
Last visited
Posts posted by unknown1
-
-
Anyone know of any good tutorials on how to create a php whois look up script??
or can someone explain how its done?
Thanks in advance!!
-
I have created a script for my listing site and what the script does is
asks the user to add a line of code to his/her site to verify that they are the owner of the sites...
The script is working fine but I need some advice on how to rewrite it so that it only redirects after
both JavaScript alerts are completed or after all conditions have been met.
Thanks in advance!!
I have fix this issue and now notice that the script is sending out 2 alerts per test.
e.g.
Script checks 1st Url for verification code and then give message Validation code was found! \n Your site has been successfully verified. but it give the message twice
then the script check url two and find that the verification code is not found and send alert
Validation code was not found! \n Please make sure you have entered the correct \n validation code and that its on the index page of your site but also gives message twice.
Any ideas of how I can fix this... I'm sure once again I'm missing something easy.
<?php session_start(); include("config.php"); $sql_site = ("SELECT * FROM sit_details_tmp WHERE `email` = '{$_SESSION['EMAIL']}'"); $site_con = mysql_query($sql_site, $conn) or die(mysql_error()); $numrows = mysql_num_rows($site_con); if($numrows > 0){ while($row = mysql_fetch_array($site_con)) { $sql_ver = ("SELECT Url FROM sit_details_tmp WHERE `email` = '{$_SESSION['EMAIL']}'"); $site_ver = mysql_query($sql_ver, $conn) or die(mysql_error()); $rs_ver = mysql_fetch_array($site_ver); do { $validationURL="$row[url]"; $htmlString=file_get_contents($validationURL); $var_code = "$row[ver_code]"; if (eregi($var_code, $htmlString)) { $ver_update=("UPDATE `sit_details_tmp` SET `id_verification` = '2' WHERE `email` = '$_SESSION[email]' AND `Url` = '$row[url]'") or die(mysql_error()); if (!mysql_query($ver_update,$conn)) { die('Error: ' . mysql_error()); } echo '<script language=javascript>'; echo 'alert("Validation code was found! \n Your site has been successfully verified.");'; echo '</script>'; echo '<SCRIPT language="JavaScript"> <!-- window.location="my_account.php"; //--> </SCRIPT> '; }else{ echo"$var_code"; echo '<script language=javascript>'; echo 'alert("Validation code was not found! \n Please make sure you have entered the correct \n validation code and that its on the index page of your site.");'; echo '</script>'; echo '<SCRIPT language="JavaScript"> <!-- window.location="my_account.php"; //--> </SCRIPT> '; } }while(mysql_fetch_array($site_ver)); } }else{ echo"User not logged."; } ?>
-
I have created a script for my listing site and what the script does is
asks the user to add a line of code to his/her site to verify that they are the owner of the sites...
The script is working fine but I need some advice on how to rewrite it so that it only redirects after
both JavaScript alerts are completed or after all conditions have been met.
Thanks in advance!!
<?php session_start(); include("config.php"); $sql_site = ("SELECT * FROM sit_details_tmp WHERE `email` = '{$_SESSION['EMAIL']}'"); $site_con = mysql_query($sql_site, $conn) or die(mysql_error()); $numrows = mysql_num_rows($site_con); if($numrows > 0){ while($row = mysql_fetch_array($site_con)) { $sql_ver = ("SELECT Url FROM sit_details_tmp WHERE `email` = '{$_SESSION['EMAIL']}'"); $site_ver = mysql_query($sql_ver, $conn) or die(mysql_error()); $rs_ver = mysql_fetch_array($site_ver); do { $validationURL="$row[url]"; $htmlString=file_get_contents($validationURL); $var_code = "$row[ver_code]"; if (eregi($var_code, $htmlString)) { $ver_update=("UPDATE `sit_details_tmp` SET `id_verification` = '2' WHERE `email` = '$_SESSION[email]' AND `Url` = '$row[url]'") or die(mysql_error()); if (!mysql_query($ver_update,$conn)) { die('Error: ' . mysql_error()); } echo '<script language=javascript>'; echo 'alert("Validation code was found! \n Your site has been successfully verified.");'; echo '</script>'; echo '<SCRIPT language="JavaScript"> <!-- window.location="my_account.php"; //--> </SCRIPT> '; }else{ echo"$var_code"; echo '<script language=javascript>'; echo 'alert("Validation code was not found! \n Please make sure you have entered the correct \n validation code and that its on the index page of your site.");'; echo '</script>'; echo '<SCRIPT language="JavaScript"> <!-- window.location="my_account.php"; //--> </SCRIPT> '; } }while(mysql_fetch_array($site_ver)); } }else{ echo"User not logged."; } ?>
-
I have now fixed the original issues but now have a new one.
The script is now working and doing what it should... but I need some advice on how to rewrite it so that it only redirects after both JavaScript alerts are completed or after all conditions have been met.
<?php session_start(); include("config.php"); $sql_site = ("SELECT * FROM sit_details_tmp WHERE `email` = '{$_SESSION['EMAIL']}'"); $site_con = mysql_query($sql_site, $conn) or die(mysql_error()); $numrows = mysql_num_rows($site_con); if($numrows > 0){ while($row = mysql_fetch_array($site_con)) { $sql_ver = ("SELECT Url FROM sit_details_tmp WHERE `email` = '{$_SESSION['EMAIL']}'"); $site_ver = mysql_query($sql_ver, $conn) or die(mysql_error()); $rs_ver = mysql_fetch_array($site_ver); do { $validationURL="$row[url]"; $htmlString=file_get_contents($validationURL); $var_code = "$row[ver_code]"; if (eregi($var_code, $htmlString)) { $ver_update=("UPDATE `sit_details_tmp` SET `id_verification` = '2' WHERE `email` = '$_SESSION[email]' AND `Url` = '$row[url]'") or die(mysql_error()); if (!mysql_query($ver_update,$conn)) { die('Error: ' . mysql_error()); } echo '<script language=javascript>'; echo 'alert("Validation code was found! \n Your site has been successfully verified.");'; echo '</script>'; echo '<SCRIPT language="JavaScript"> <!-- window.location="my_account.php"; //--> </SCRIPT> '; }else{ echo"$var_code"; echo '<script language=javascript>'; echo 'alert("Validation code was not found! \n Please make sure you have entered the correct \n validation code and that its on the index page of your site.");'; echo '</script>'; echo '<SCRIPT language="JavaScript"> <!-- window.location="my_account.php"; //--> </SCRIPT> '; } }while(mysql_fetch_array($site_ver)); } }else{ echo"User not logged."; } ?>
-
-
Thanks guys you're awesome!!!
-
Yes, exactly like that. Then it will update only for that email and that url, not for ALL urls. The way to include arrays inside a string is like this:
$sql_site = ("SELECT * FROM sit_details_tmp WHERE `email` = '{$_SESSION['EMAIL']}' ");
The {} characters "protect" the array and make sure it will be interpreted properly. If you just use $row for example, then it MIGHT work, but it's much safer to use the {} and also to put single quotes around the 'Url' bit inside the [].
In my script I already have $sql_site = ("SELECT * FROM sit_details_tmp WHERE `email` = '$_SESSION['EMAIL']' "); and it is cycling through the users Url ok... what I mean by updates all sites is all sites that belong to that user. So that part is ok... The problem is if the user has 3 sites
and has to verify all of them the script cycles through the $row and $row[ver_code] for that user... and if the user puts the code on site but not the other the query $ver_update=("UPDATE `sit_details_tmp` SET `id_verification` = '2' WHERE `email` = '".$_SESSION."'") or die(mysql_error()); updates all sites that belong to that users email and not just the the one site that contains the verification code.
$ver_update=("UPDATE `sit_details_tmp` SET `id_verification` = '2' WHERE `email` = '".$_SESSION[email]."' AND eregi($var_code,$htmlString) ") or die(mysql_error());
Error: You have an error in your SQL syntax; check the manual that corresponds to your MySQL server version for the right syntax to use near '
-
If you are allowing more than one site for one email address then you'll need to store the site in the database as well as the email. Then when you update it, you check the site as well as the email.
I do have email and url in the same table... I'm not sure I understand what your saying.
or do you mean add
AND Url = $row[url];
or something like that??
Oh and the site and email is already in the table what the script is doing is checking if $row; aka site Url has $row['ver_code']; and ver_code contains the code that they enter on the index page.... if the site has ver_code then updates table.
-
I have created a script for my listing site and this script does is asks user to add a line of code to his/her site to verify that they are the owner of the sites...
<?php session_start(); include("config.php"); $sql_site = ("SELECT * FROM sit_details_tmp WHERE `email` = '$_SESSION[email]' "); $site_con = mysql_query($sql_site, $conn) or die(mysql_error()); $numrows = mysql_num_rows($site_con); if($numrows > 0){ while($row = mysql_fetch_array($site_con)) { $partnersURL="$row[url]"; $htmlString=file_get_contents($partnersURL); $var_code = "$row[ver_code]"; if (eregi($var_code, $htmlString)) { $ver_update=("UPDATE `sit_details_tmp` SET `id_verification` = '2' WHERE `email` = '".$_SESSION[email]."'") or die(mysql_error()); if (!mysql_query($ver_update,$conn)) { die('Error: ' . mysql_error()); } echo '<script language=javascript>'; echo 'alert("Validation code was found! \n Your site has been successfully verified.");'; echo '</script>'; echo '<SCRIPT language="JavaScript"> <!-- window.location="my_account.php"; //--> </SCRIPT> '; }else{ echo"$var_code"; echo '<script language=javascript>'; echo 'alert("Validation code was not found! \n Please make sure you have entered the correct \n validation code and that its on the index page of your site.");'; echo '</script>'; echo '<SCRIPT language="JavaScript"> <!-- window.location="my_account.php"; //--> </SCRIPT> '; } } }else{ echo"User not logged."; } ?>
The script is working fine with one big flaw.
Say a user goes to verify the code put on her/her sites they add the code to one site but not the other and hit verify...
the script will update all sites as verified and not just the site that actually has the code.
I assume the problem is in the line
$ver_update=("UPDATE `sit_details_tmp` SET `id_verification` = '2' WHERE `email` = '".$_SESSION."'") or die(mysql_error());
If anyone has an idea of how I could fix this it sure would be appreciated.
-
Try this
echo '<div id="rightcol">'; echo '<SCRIPT type="text/javascript" language="JavaScript" src="http://xslt.alexa.com/site_stats/js/s/a?url=www.source2.co.uk">'; echo '</SCRIPT>'; echo '</div>';
This code should work fine if not change all the " " to ' ' and the ' ' to " "
e.g. echo "</div>";
-
That won't work either, lol. It's actually pretty simple; just remove the single quotes from within your string:
echo '<div id="rightcol"><SCRIPT type="text/javascript" language="JavaScript" src="http://xslt.alexa.com/site_stats/js/s/a?url=www.source2.co.uk"></SCRIPT></div>';
echo '<div id="rightcol">'; echo '<SCRIPT type="text/javascript" language="JavaScript" src="http://xslt.alexa.com/site_stats/js/s/a?url=www.source2.co.uk">'; echo '</SCRIPT>'; echo '</div>';
This code will work fine but you're right in saying that if you remove the "" and add ' ' in it's place it will also work. or even '" . ' ' . "
-
Try something like the following
$sql_site = ("SELECT * FROM table WHERE `id` = '$_SESSION[id]' "); $site_con = mysql_query($sql_site, $conn) or die(mysql_error()); $numrows = mysql_num_rows($site_con); if($numrows > 0){ while($row = mysql_fetch_array($site_con)) { echo "$row['row name']"; }
-
Try this
echo '<div id="rightcol">';
echo '<SCRIPT type="text/javascript" language="JavaScript" src="http://xslt.alexa.com/site_stats/js/s/a?url=www.source2.co.uk">'; '</SCRIPT>';
echo '</div>';
This code will work fine.
-
Just clean up the form input tags
e.g.
$keywords = $_POST['keywords'];
$keywords = stripslashes($keywords);
$keywords = strip_tags($keywords);
htmlspecialchars(addslashes($_POST[$keywords]));
-
does that table not have a primary key? just use the primary key when updating. IE
if (eregi($var_code, $htmlString)) { $ver_update=("UPDATE `sit_details_tmp` SET `id_verification` = '2' WHERE `id` = '".row['id']."'") or die(mysql_error()); if (!mysql_query($ver_update,$conn)) { die('Error: ' . mysql_error()); }
by the way you need to encase associative array keys with single quotes
$array[key];//wrong $array['key'];//right
Ok I have figured out that the script is cycling through the urls fine but updating all sites
to verified and not just the one site that is actually has been verified.
*** not all sites in table but all site for that user***
I want it to work so that it checks all the users urls one by one and updates one by one
now with
ver_update=("UPDATE `sit_details_tmp` SET `id_verification` = '2' WHERE `id` = '".row['id']."'") or die(mysql_error());
it's updating all listings that the user has at once and not only the one that have the verification code.
If you understand already... sorry. I just assume you figured the issues was updating all urls in the table and not just the users ones.
Anyways, if you have any ideas it sure would help.
Thanks!!
-
I do have a primary key but in table one it's site_id and in the user table it's id_user
and the id fields don't match so I'm not sure I follow on how to do what your saying.
I used
WHERE `email` = '$_SESSION[email]'
cause when the user registers I register the session value email and that way I know the information I'm pulling belongs to the right user.
The only matching fields I have between the two table is email
-
Ok I have figured out that the script is cycling through the urls fine but updating all sites
to verified and not just the one site that is actually has been verified.
I assume that it's in the following code but not sure how else I could write it cause
the matching rows in this and the user table are email so that is the only way I can think of to do it.
$ver_update=("UPDATE `table` SET `id_verification` = '2' WHERE `email` = '".$_SESSION[email]."'") or die(mysql_error());
This is the full script... it works fine but validates all url when it should only update the ones that contain the validation code.
<?php session_start(); include("config.php"); $sql_site = ("SELECT * FROM sit_details_tmp WHERE `email` = '$_SESSION[email]' "); $site_con = mysql_query($sql_site, $conn) or die(mysql_error()); $numrows = mysql_num_rows($site_con); if($numrows > 0){ while($row = mysql_fetch_array($site_con)) { $partnersURL="$row[url]"; $htmlString=file_get_contents($partnersURL); $var_code = "$row[ver_code]"; if (eregi($var_code, $htmlString)) { $ver_update=("UPDATE `sit_details_tmp` SET `id_verification` = '2' WHERE `email` = '".$_SESSION[email]."'") or die(mysql_error()); if (!mysql_query($ver_update,$conn)) { die('Error: ' . mysql_error()); } echo '<script language=javascript>'; echo 'alert("Validation code was found! \n Your site has been successfully verified.");'; echo '</script>'; echo '<SCRIPT language="JavaScript"> <!-- window.location="my_account.php"; //--> </SCRIPT> '; }else{ echo"$var_code"; echo '<script language=javascript>'; echo 'alert("Validation code was not found! \n Please make sure you have entered the correct \n validation code and that its on the index page of your site.");'; echo '</script>'; echo '<SCRIPT language="JavaScript"> <!-- window.location="my_account.php"; //--> </SCRIPT> '; } } }else{ echo"User not logged."; } ?>
-
Good plan. Thanks!!!
-
Hello all, I need a little help if someone has some extra time.
I have created a script that checks for a line of code on an external url and if it's on the page give a success message and if not found give error alert...
The issue I'm having is if the person have more than one site they want to verify and they have already verified the code on one... when they go to verify the other sites it updates automatic because it was found on the first one.
because I use $row and it can contain more than one url
I assume my script is only checking the 1st url on the list and not the others...
session_start(); include("config.php"); $sql_site = ("SELECT * FROM table WHERE `email` = '$_SESSION[email]' "); $site_con = mysql_query($sql_site, $conn) or die(mysql_error()); $numrows = mysql_num_rows($site_con); if($numrows > 0){ while($row = mysql_fetch_array($site_con)) { $sql_cat = "SELECT Cate_Details FROM `category` WHERE idCategory = '". $row["idCategory"]."'"; $rec_cat = mysql_query($sql_cat) or die(mysql_error()); $rs_cat = mysql_fetch_array($rec_cat); $sql_stat = "SELECT staDetails FROM `site_status` WHERE idStatus = '". $row["idStatus"]."'"; $rec_stat = mysql_query($sql_stat) or die(mysql_error()); $rs_stat = mysql_fetch_array($rec_stat); $row[url] = $row[url]++; $partnersURL="$row[url]"; $htmlString=file_get_contents($partnersURL); if (eregi('46546546546', $htmlString)) { $ver_update=("UPDATE `table` SET `id_verification` = '2' WHERE `email` = '".$_SESSION[email]."'") or die(mysql_error()); if (!mysql_query($ver_update,$conn)) { die('Error: ' . mysql_error()); } echo '<script language=javascript>'; echo 'alert("Validation code was found! \n Your site has been successfully verified.");'; echo '</script>'; echo '<SCRIPT language="JavaScript"> <!-- window.location="my_account.php"; //--> </SCRIPT> '; }else{ echo '<script language=javascript>'; echo 'alert("Validation code was not found! \n Please make sure you have entered the correct \n validation code and that its on the index page of your site.");'; echo '</script>'; echo '<SCRIPT language="JavaScript"> <!-- window.location="my_account.php"; //--> </SCRIPT> '; } } }else{ echo"User not logged."; }
-
Got that working... Grrrrrrr stupid little issues.
Anyways my biggest issues is counting from date('m.d.y') to 90 days from that time.
e.g.
$date = date('m.d.y');
if($date == date('m.d.y') + 30){
I know this wont work but does anyone know what I can do to make it work.
}
Thanks!!
-
oh ic it's because i did add a format date("m.d.y") just had date() so it was showing in the database as 00-00-0000 =) lol
Any idea of how I would go about counting 30 days from the date("m.d.y")??
Want to set a script up so it will change a listing back to regular after 90 days
Still showing up as 00-00-000 in database any ideas
$date = DATE("m.d.y"); $insetDate = "INSERT INTO sit_details_tmp (rDate) VALUES ('$date')";
works fine on one script but when added to my other page doesn't work.
-
oh ic it's because i did add a format date("m.d.y") just had date() so it was showing in the database as 00-00-0000 =) lol
Any idea of how I would go about counting 30 days from the date("m.d.y")??
Want to set a script up so it will change a listing back to regular after 90 days
-
yes I know that mysql has date time function but I'm having an issue submitting
it to the database.
e.g.
$sql = "insert into table (date) VALUES ('????????')";
I don't understand how to use the function.
when I try something like
$sql = "insert into table (date) VALUES (date())";
I get an error even when I create a var like $date = date() I still get and error or 00-00-0000
in database.
can someone please elaborate on how to use these when submitting to a database...
Thanks in advance!
-
Hello all, I was hoping someone could help me figure out the logic behind a script that
deletes a listing automatically after a 90 day time frame.
I have no clue on this so any input would be helpful.
e.g. how would I say
if(todays date == todays date + 90){
}
Not familiar with php date function so if someone could help that would be great.
Thanks in advance.
[SOLVED] checking dates
in PHP Coding Help
Posted
This will take todays date and add 90 days
$today=date('m/d/y');
$theFuture=date('Y-m-d',strtotime($today.' + 90 days'));
echo $theFuture;