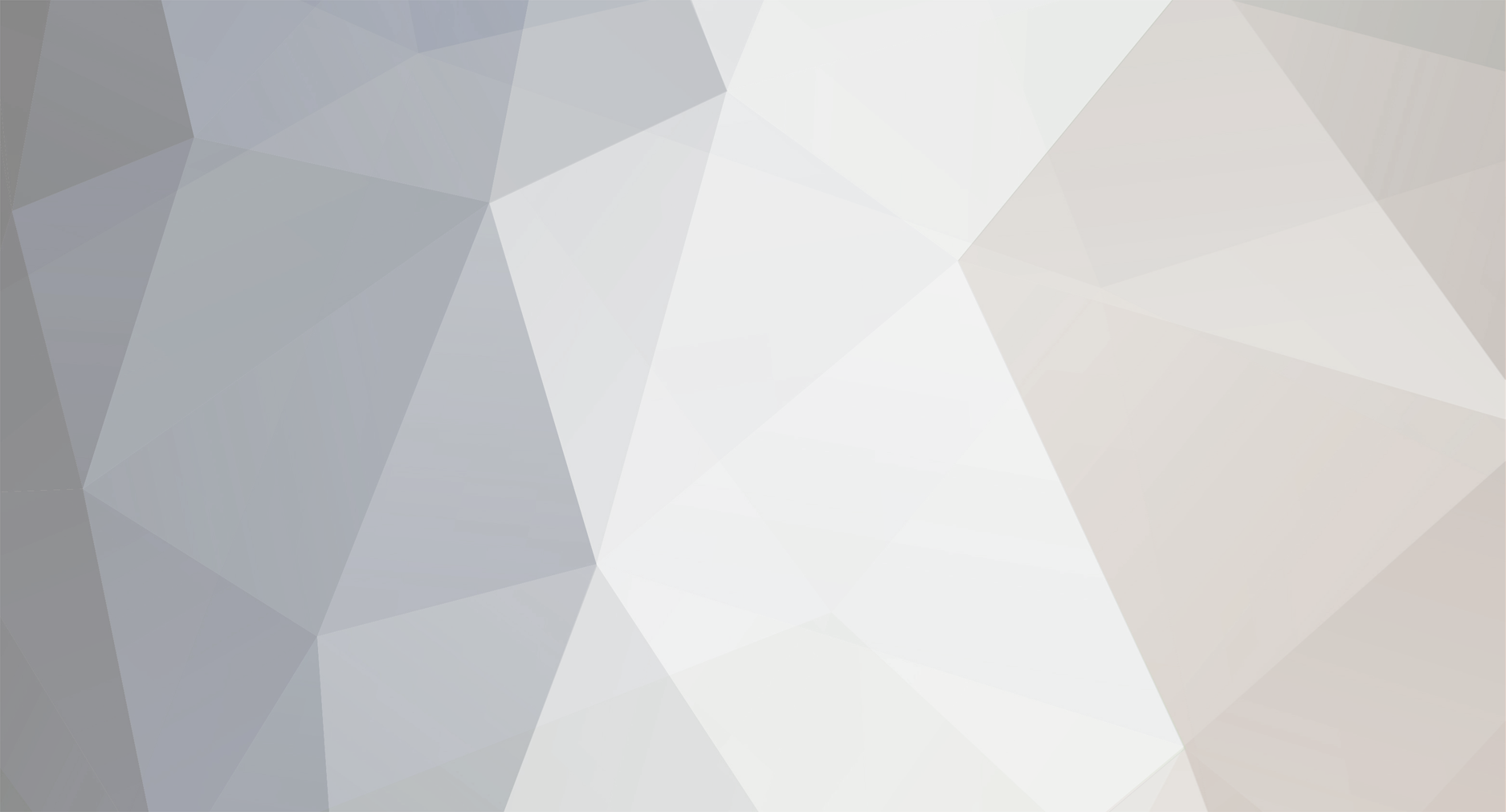
mystery_man
Members-
Posts
15 -
Joined
-
Last visited
Never
Everything posted by mystery_man
-
oops - use $weapon_array = array(); instead of $weapon_array = new array();. I didn't test the code, but that is the basic idea and should get you moving in the right direction, along with that good explanation from Nightslyr.
-
mmm... pipe dream indeed - just burst my bubble :'( I should have said: 1. It groups all of the commonly used db functions you commonly use together in one place 2. It is reasonably generic, and provides a bit of abstraction between databases as far as simple functionality is concerned
-
I suggest storing your XML data in pure xml format, meaning 'weapondata.xml', and looking like this: <?xml version='1.0' standalone='yes'?> <weapons> <weapon> <name>Eastwind Katana</name> <basebonus>2</basebonus> </weapon> <weapon> <name>Robin Bow</name> <basebonus>2</basebonus> </weapon> <weapon> <name>Blue Ice Staff</name> <basebonus>2</basebonus> </weapon> </weapons> Now to access that file in php using simpleXML (code not tested): <?php //create a variable to store the xml data you're going to import from the file (in the same dir. as your script): $xml = simplexml_load_file('weapondata.xml'); //this creates a simpleXML object that can be worked with like so: //create an empty array: $weapon_array = new array(); //for each weapon/item in $xml: foreach($xml->weapon as $weapon){ //get the info from the simpleXML object: $name = $weapon->name; $bonus = $weapon->basebonus; //add them to the weapon array: $weapon_array[$name] = $bonus; //here having the weapon name as the key of the array, //and the base bonus thing as the value. } //and then printing the data: echo "<h3>Weapon data:</h3><br /><br />"; foreach($weapon_array as $name=>$bonus){ echo "Name: {$name}. Bonus: {$bonus}<br />"; } ?>
-
I would suggest looking at OOP and other commonly used design patterns to get a good understanding of what techniques are currently being used, and which ones work well. It does not neccasarilly imply looking at the code itself (although it is also important), but also understanding the concepts behind these techniques. Reccomendation: Their is an course on Lynda.com called "PHP: Beyond the basics" by Kevin Skoglund which was my Eureka in php, me only having started about 6 months ago. This is a really great resource, as it not only teaches and shows cool concepts, but also opens your mind to how things might be done better.
-
Oh I got carried away... security. Just make sure all your data going into your database are escaped. If you use 'model' classes for your data objects (eg users, cars, photos, whatever) knowing how to update/create themselves using, for example, $user->save(); or $user->create(); , escape all the attributes in the create/save functions prior to insertion.
-
It is a very good idea to have a Class that provides an abstraction layer for your database functions. I always use one when working on any dynamic site, here are the two main pro's: 1. It provides all the commonly used database functions you would need 2. It is generic, for MS SQL or other databases, just change the insides of the functions, and you have abstraction for easy reuse If you have one for MySQL, PostGre, SQLite, MS SQL etc, your application is only working with the 'Database' class, and it cares not what goes on under the hood of it. It makes it super easy to port apps to other databases. Here is the idea of what I use. "//@" explains my code. <?php //=======MySQL Connection details============ //@ these can be kept in a config file, especially if there is plenty of other 'config' data $host = "localhost"; $database = "dbname"; $user = "username"; $password = "password"; //@ keep your different settings at hand while in development - it saves me some time. /* ====Local Settings $host = "localhost"; $database = "dbname"; $user = "username"; $password = "password"; ====development server settings $host = "localhost"; $database = "dbname"; $user = "username"; $password = "password"; ====production server settings $host = "localhost"; $database = "dbname"; $user = "username"; $password = "password"; */ //===================== //Database Constants defined('DB_HOST') ? null : define("DB_HOST", $host); defined('DB_NAME') ? null : define("DB_NAME", $database); defined('DB_USER') ? null : define("DB_USER", $user); defined('DB_PASS') ? null : define("DB_PASS", $password); class MySQLDatabase{ //attributes private $connection; public $last_query; private $magic_quotes_active; private $real_escape_string_exists; public function __construct(){ //@ open the connection at construction, so it is available from the start $this->open_connection(); $this->magic_quotes_active = get_magic_quotes_gpc(); $this->real_escape_string_exists = function_exists( "mysql_real_escape_string" ); } public function open_connection(){ $this->connection = mysql_connect(DB_HOST, DB_USER, DB_PASS); //open connection if(!$this->connection){ die("Error opening MySQL Database connection: ". mysql_error()); }else{ $db_select = mysql_select_db(DB_NAME, $this->connection); //select database if (!$db_select){ die("Database selection failed: " . mysql_error()); } } } public function close_connection(){ if(isset($this->connection)){ mysql_close($this->connection); unset($this->connection); } } //@ to do a simple query, and return the resultset public function query($sql){ $this->last_query = $sql; //set last query $result = mysql_query($sql, $this->connection); if (!$result) { //check for error //output the last query - usefull for debugging $output = "Last Query ".$this->last_query."<br>"; $output .= "Database query failed: " . mysql_error() . "<br /><br />"; die( $output ); } return $result; } //@ this is some rather tricky, it just returns the escaped paramater public function escape_value($value) { if($this->real_escape_string_exists) { // undo any magic quote effects so mysql_real_escape_string can do the work. PHP v4.3.0 or higher if( $this->magic_quotes_active ){ $value = stripslashes( $value ); } $value = mysql_real_escape_string($value); } else{ // if magic quotes aren't already on then add slashes manually, before PHP v4.3.0 if( !$this->magic_quotes_active ){ $value = addslashes( $value ); } // if magic quotes are active, then the slashes already exist } return $value; } //database neutral methods public function fetch_array($result){ return mysql_fetch_array($result); } public function num_rows($result){ return mysql_num_rows($result); } public function get_last_id(){ return mysql_insert_id($this->connection); // get the last id inserted over the current db connection } public function affected_rows(){ return mysql_affected_rows($this->connection); } public function insert_id() { // get the last id inserted over the current db connection return mysql_insert_id($this->connection); } } //@ make the $database and $db variables available for use. $database = new MySQLDatabase(); $db =& $database; ?> This approach becomes really effective when using an OOP design, in which you have clas modelling each of your data objects, which each in turn has a table. You can build the models inteligently so that they 'know how to' construct, update and create themselves (in and from the their tables in the DB) by making use of the database class. It works for me! Regards
-
Designing a photo gallery with Plug-in feature question
mystery_man replied to OOP's topic in Application Design
This is something I have been pondering on myself. I have found it difficult to imagine how to build an interface for adding modules/plugins on the fly, as I also want to use such functionality for a project I am working on. Here are some usefull questions and my opinions on them: 1. What should the modules be able to do? Most probably, this will encompass Create-Read-update-delete (CRUD, and I use these terms very generically) functions, I think most other operations can be performed provided that these 4 features are presented and available. Eg, if some reporting needs to be done on existing data, the READ function should be all that is needed. The other 3 'umbrella' functions will obviously be more complex to work with. 2. How can the modules interface with the core of the application? This can be achieved by using some kind of API to provide the CRUD generically to modules that will make use of these functions. This is how, for example, facebook enables developers to build their own facebook apps. The API is developed, documented and provided to developers by facebook to use in their applications they build for facebook. From an OOP perspective (for an application much simpler then facebook!), I would think that the API would take the shape of a class/model that provides abstraction between the functionality of the CRUD and how they are implemented (the business logic and all the code behind them). Example: a) You have a DB table for your photos. b) You have a class, 'PhotoObject' which models a photo, and: - contains attributes like id, name, description, filename, size, author - contains CRUD functions and other operations that is related to working with the photos. c) You have an API implementation in the form of a class, 'myAPI', that: - uses functionality of the PhotoObject and other Classes used in the application - provides the CRUD functionality that is generic enough to be reused by modules that are still to be written (but we know they will need CRUD functions, thus we have an idea of what the API class should look like). After writing a module, the API will probably be altered. 3. How can the core be desinged to simplify the interfacing with modules? I assume the main goals in achieving the right kind of environment for a 'plugin system' is to build the framework of the app in such a way that it is very modular, and that the different VMC layers is effectively separated from each other. I also assume that this 'API' will be similar to controllers - and come to think of it, a Controller in the VMC model acts sort of like an API anyway - but it will be more generic in design and more 'transparent' in terms of usage. These are my thoughts, although I am by no means an expert on application design - yet. I hope this at least gives some ideas of what to look for. Regards -
oh, And here is my PHP code I used: $wsdl = "http://ww2.merseta.org.za/webservices/sampleservice1.asmx?WSDL"; $params = array('levyNo' => 'L630729640'); //test data $namespace = "http://schemas.xmlsoap.org/soap/envelope/"; $soapAction = "http://tempuri.org/OrgInfo"; $methodname = "OrgInfo"; $client = new nusoap_client($wsdl); //set encoding $client->soap_defencoding = 'UTF-8'; $client->decode_utf8 = false; $result = $client->call($methodname, $params, $namespace, $soapAction);
-
this might also be usefull for you: http://www.w3schools.com/php/php_arrays.asp
-
Hi Kryllster As I understand: 1. check the name of the weapon, and find its corresponding $base_bonus 2. Assosiate that $base_bonus to something for future use. It is done like so, using arrays: <?php //create the weapon array with the name of the weapon $weapon = array('name'=>'weapon name'); //declare the $base_bonus as 0, innitially $base_bonus = 0; //evaluate the base_bonus (this is a tedious way of doing it, especially when using a config file, but that is not the issue you raised) // level 1 weapons if($weapon['name'] == "Eastwind Katana" || $weapon == "Robin Bow" || $weapon == "Blue Ice Staff"){ $base_bonus = 2; } // level 2 weapons if($weapon['name'] == "Aldonian Spatha" || $weapon == "Cobalt Bow" || $weapon == "Fire Staff"){ $base_bonus = 4; } //level 3 weapons if($weapon['name'] == "Hitzman Claymore" || $weapon == "Fire Bow" || $weapon == "Plaz Staff"){ $base_bonus = 6; } // starter weapon if($weapon['name'] == "Big Club"){ $base_bonus = 2; } //assign the $base_bonus the the weapon array as well, seeing as that is determined now. $weapon['base_bonus'] = $base_bonus; //to access the weapon array keys: $name = $weapon['name']; $base_bonus = $weapon['base_bonus']; ?> Hope this helps
-
Hi Ed Assuming that your 'expiry_date' field is of type DATE in the database: 1. Get the current date in the script in a SQL-acceptable format in $this_var 2. Add 5 months to $this_var 3. SQL logic: select all records where the date is SMALLER than $this_var (within 5 months of the given date) Now for the code: <?php //You can use this function to get the date function get_my_date(){ //get the current date plus five months $date = strtotime("+ 5 months", time()); //format the time for SQL (at least MySQL is happy with this) $time = strftime("%Y-%m-%d %H:%M:%S",$date); return $time; //looks like "2010-02-03 14:30:24" if today is "2009-09-03 14:30:24" } //using the function, get the time: $date = get_my_date(); //here is your SQL $sql = "SELECT * FROM <tablename> WHERE expiry_date < '{$date}'"; //the rest of your script ... ?> I Hope this is helpfull!
-
Consuming a .net web service with php code
mystery_man replied to klaibert26's topic in PHP Coding Help
Also, this might be helpfull: http://forums.devnetwork.net/viewtopic.php?f=1&t=102203&p=548118&hilit=nusoap&sid=dec6140f170c5bad8c646ad25a0be356#p548118 -
Consuming a .net web service with php code
mystery_man replied to klaibert26's topic in PHP Coding Help
Hi There I have also been having a problem trying to do exactly what you are doing. My problem is not that I cannot connect, but in passing the params, so my connection code is working. Check out my code, it might help you http://www.phpfreaks.com/forums/index.php/topic,267646.0.html -
get the date after the given date -- please check this out
mystery_man replied to louis_coetzee's topic in PHP Coding Help
I had my fair share of trouble with the php date stuff. Try this - it might help you in the right direction: //get the current time, or whatever time you're workign with as timestamp $date = time(); //Get tomorrow's date $day_after = strtotime("tomorrow",$date); //get yesterday's date $day_previous = strtotime("yesterday",$date); //then use strftime to format the date as you want it. $day_after_formatted = strftime("%Y-%m-%d", $day_after); $day_previous_formatted = strftime("%Y-%m-%d", $day_previous); -
Hi Guys I need some urgent help on web services using NuSoap here from the southen tip of the 'dark continent', South africa. (And yes, pity me - yesterday I found a production web server of a certain ISP in SA running PHP 4.2.9!! ) I am busy with a website that needs to connect to a .net web service in order to validate some information against a MS SQL database, but that is completely transparent as the 2 web service methods does all the DB work. Not having worked with web services before, I did some googling and stumbled upon NuSoap, which seems to be able to do the job. The fact that NuSoap is a standalone library you can just add onto your code is of importance, as I cannot tamper with the PHP extensions on the production server, and I don't think the server is even has the SOAP extension running. Ok, I can connect to the web service, send the request and get the response, but it doesnt like what is sent, as what is being sent by my NuSoap Implementation is probably uncosure. Here is the details of the one method exposed by the web service. The wsdl stuff is here: http://ww2.merseta.org.za/webservices/sampleservice1.asmx?WSDL //The following is the sample SOAP 1.1 request for one of the methods POST /webservices/sampleservice1.asmx HTTP/1.1 Host: ww2.merseta.org.za Content-Type: text/xml; charset=utf-8 Content-Length: length SOAPAction: "http://tempuri.org/OrgInfo" <?xml version="1.0" encoding="utf-8"?> <soap:Envelope xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:xsd="http://www.w3.org/2001/XMLSchema" xmlns:soap="http://schemas.xmlsoap.org/soap/envelope/"> <soap:Body> <OrgInfo xmlns="http://tempuri.org/"> <levyNo>string</levyNo> </OrgInfo> </soap:Body> </soap:Envelope> //The following is the sample SOAP 1.1 response HTTP/1.1 200 OK Content-Type: text/xml; charset=utf-8 Content-Length: length <?xml version="1.0" encoding="utf-8"?> <soap:Envelope xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:xsd="http://www.w3.org/2001/XMLSchema" xmlns:soap="http://schemas.xmlsoap.org/soap/envelope/"> <soap:Body> <OrgInfoResponse xmlns="http://tempuri.org/"> <OrgInfoResult> <xsd:schema>schema</xsd:schema>xml</OrgInfoResult> </OrgInfoResponse> </soap:Body> </soap:Envelope> Here is my php code using NuSoap: //include the nuSoap lib require_once('nuSoap/nusoap.php'); //construct the client object, using WSDL $client = new nusoap_client("http://ww2.merseta.org.za/webservices/sampleservice1.asmx?WSDL", true); //set encoding (it defaults to some ISO-... encoding) $client->soap_defencoding = 'UTF-8'; $client->decode_utf8 = false; //if there is a problem, do the moaning... $err = $client->getError(); if ($err) { echo '<h2>Constructor error</h2><pre>' . $err . '</pre>'; echo '<h2>Debug</h2><pre>' . htmlspecialchars($client->getDebug(), ENT_QUOTES) . '</pre>'; exit(); } // This is some test data that should work fine. $params = array( 'levyNo' => "L630729640", ); //cal the method $result = $client->call('OrgInfo', $params,'http://tempuri.org', "http://tempuri.org/OrgInfo"); //output of operation if ($client->fault) { echo '<h2>Fault (Expect - The request contains an invalid SOAP body)</h2><pre>'; print_r($result); echo '</pre>'; } else { $err = $client->getError(); if ($err) { echo '<h2>Error</h2><pre>' . $err . '</pre>'; } else { echo '<h2>Result</h2><pre>'; print_r($result); echo '</pre>'; } } //echo the output and debug stuff echo '<h2>Request</h2><pre>' . htmlspecialchars($client->request, ENT_QUOTES) . '</pre>'; echo '<h2>Response</h2><pre>' . htmlspecialchars($client->response, ENT_QUOTES) . '</pre>'; echo '<h2>Debug</h2><pre>' . htmlspecialchars($client->getDebug(), ENT_QUOTES) . '</pre>'; ok, now this is what I am getting back from the server: Error XML error parsing SOAP payload on line 1: Empty document Request POST /webservices/sampleservice1.asmx HTTP/1.0 Host: ww2.merseta.org.za User-Agent: NuSOAP/0.7.3 (1.114) Content-Type: text/xml; charset=UTF-8 SOAPAction: "http://tempuri.org/OrgInfo" Content-Length: 433 <?xml version="1.0" encoding="UTF-8"?> <SOAP-ENV:Envelope xmlns:SOAP-ENV="http://schemas.xmlsoap.org/soap/envelope/" xmlns:xsd="http://www.w3.org/2001/XMLSchema" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:SOAP-ENC="http://schemas.xmlsoap.org/soap/encoding/" xmlns:ns8389="http://tempuri.org"> <SOAP-ENV:Body> <OrgInfo xmlns="http://tempuri.org/"> <levyNo>L630729640</levyNo> </OrgInfo> </SOAP-ENV:Body> </SOAP-ENV:Envelope> Response HTTP/1.1 200 OK Connection: close Date: Wed, 02 Sep 2009 09:14:31 GMT Server: Microsoft-IIS/6.0 MicrosoftOfficeWebServer: 5.0_Pub X-Powered-By: ASP.NET X-AspNet-Version: 2.0.50727 Cache-Control: private, max-age=0 Content-Type: text/xml; charset=utf-8 Content-Length: 386 Procedure or function 'RetrieveUserInfo' expects parameter '@levyNo', which was not supplied. <?xml version="1.0" encoding="utf-8"?><soap:Envelope xmlns:soap="http://schemas.xmlsoap.org/soap/envelope/" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:xsd="http://www.w3.org/2001/XMLSchema"> <soap:Body> <OrgInfoResponse xmlns="http://tempuri.org/" /> </soap:Body> </soap:Envelope> I do not know how to get my request look more like the sample request that was provided, as I think this descrepancy is causing problems. There might be some dumb @ss Sh!t I in my code, I don't know anything about web services, please bear with me. Any help, advice or suggestions will be very much appreciated. Thank you.