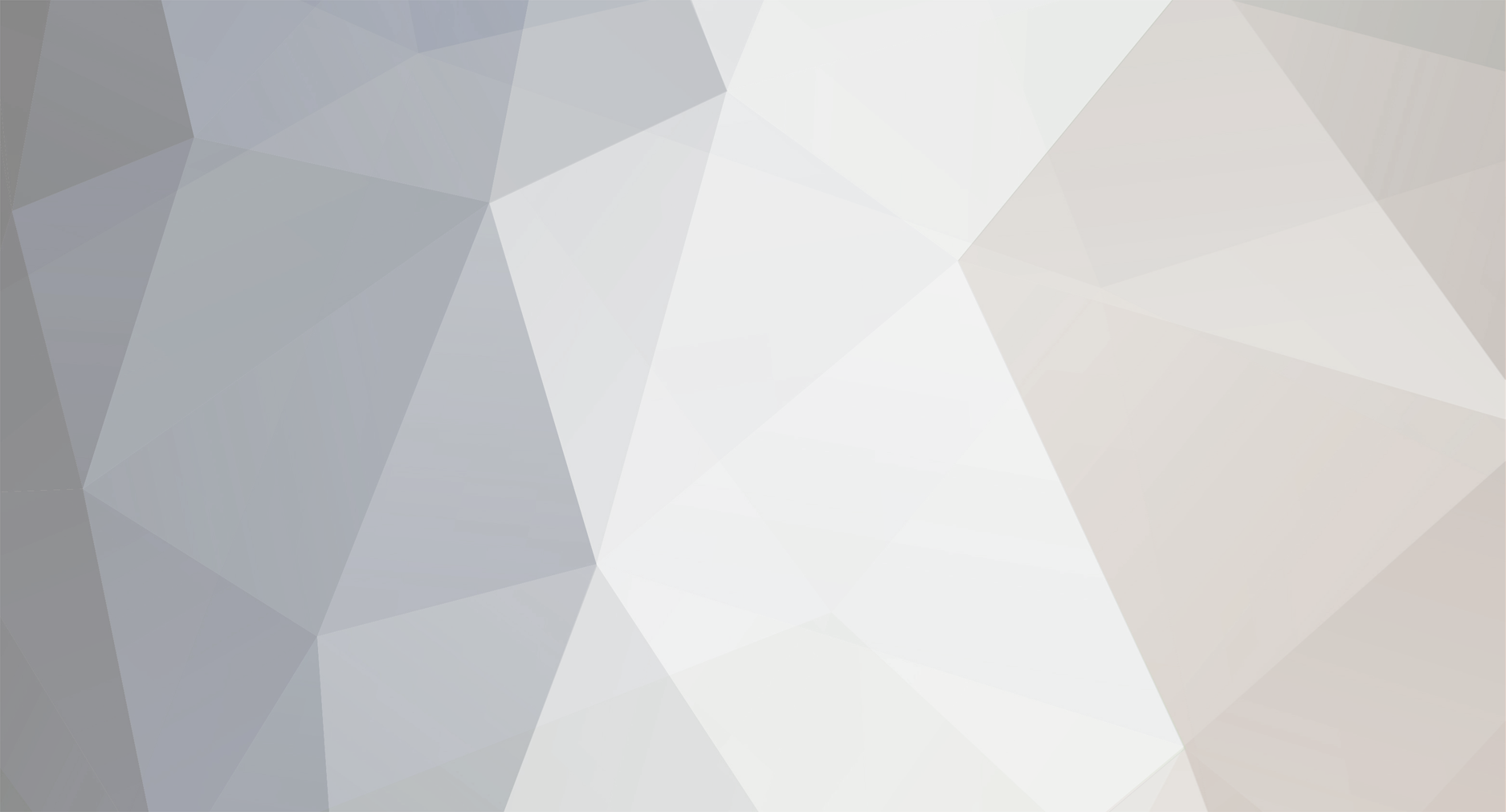
JAY6390
Members-
Posts
825 -
Joined
-
Last visited
Everything posted by JAY6390
-
$result = preg_replace('/<\?(?!php)/', '<?php', $subject);
-
Just change the two / to be ~ and then change the two )-( to read )/(
-
ha yeah I like to keep my groups just so I know where everything is tbh, you are right though it was unnecessary. Love the one liner!
-
<?php $str = "R1,R2-5,R7"; $parts = explode(',', $str); $out = array(); $regex = '~^([^\d]+)(\d+)-([^\d]*)(\d+)$~'; foreach($parts as $part) { if(preg_match($regex, $part, $matches)) { $range = range($matches[2], $matches[4]); foreach($range as $number) { $out[] = $matches[1] . $number; } } else { $out[] = $part; } } echo '<pre>' . print_r($out, true) . '</pre>'; ?> That's how I would do it. Should work for your last example too
-
[^\d]+ will match everything before the numbers
-
You aren't going to be solving this using regex, at least not all of it. You're going to need to explode the values using the , then us a regex to match the 2-5, then use a for loop to iterate over the values and add them correctly to the array
-
are you wanting the addslashes() function?
-
You can just put the forward slash since you're using # as your delimiter
-
$abc = "I drink water. I drink vodka too."; $regex = '~\b(water|vodka)\b~i'; if(preg_match($regex, $abc)) { // Found a match }
-
I'm pretty sure from this quote that its part of a full page of text
-
Surely that would match the data between all tags on a page, not just the specific data in the span tag that the OP requested? I'm assuming the string given is only part of the page, not the whole thing
-
Note that in my regex I used ~ ~ as the start/end delimiters, instead of / That is why you are getting an error. While using the DOM Document is great, it's probably overkill for your example
-
~<span id="TotalDue">([^<]+)</span>~ The value will be stored in capture group 1
-
I was being sarcastic It's not me that needs to know how to fix the error lol
-
... but that involves reading ...
-
This isn't related to regex at all! This is because you're outputting content before your cookies are set, which you can't do. There must be a million articles on why this error happens
-
haha I know that feeling
-
$output = preg_replace('~^[^\da-z]+~i', '', $input); That will replace any characters at the start of a string up until the first letter or number only
-
You would be better off using parse_url <?php $text = 'http://www.somedomain.com/blah/blah/blah'; $parts = parse_url($text); echo $parts['scheme'] . '://' . $parts['host'] . '/'; For the regex version, this will do it <?php $text = 'http://www.somedomain.com/blah/blah/blah'; $result = preg_replace('~^(.*?//.*?/).*$~', '$1', $text); echo $result;
-
If you're wanting to restrict it to just text either side as the "tags" you could use function getTextBetweenTags($string, $start, $end) { $start = preg_quote($start, '~'); $end = preg_quote($end, '~'); $pattern = "~$start(.*?)$end~"; if(preg_match($pattern, $string, $matches)) { return $matches[1]; } return false; } $html = 'this is a bunch of nothing _MARKER_OBJ = [ get this crap ]'; $content = getTextBetweenTags($html, '_MARKER_OBJ = [', ']'); echo $content; That doesn't require you to escape the start/end tags as it escapes all regex syntax characters using preg_quote
-
This is why my solution stops at 99
-
Ah OK, I skipped that post sorry. my regex above should work
-
Also, if you really want to do it via regex...you could use this, although personally I find it a bit overkill ~^(1[89]|[2-9][0-9])$~
-
Why would you use regex for this? Surely it's easier to just use regular php $age = (int) $_POST['age']; if($age >= 18 && $age <= 99) { // Age is correct } else { // Age is incorrect }