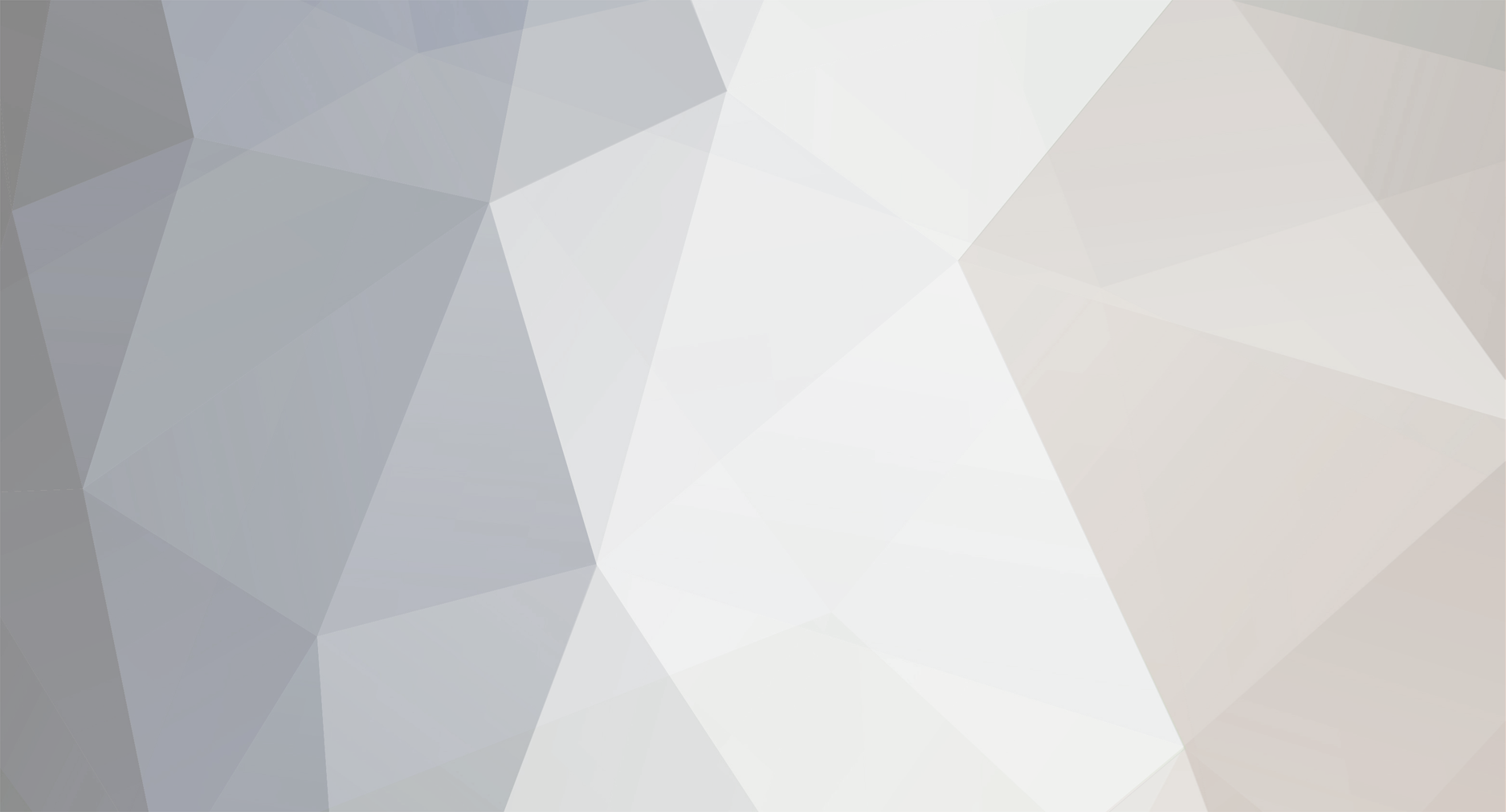
khr2003
-
Posts
112 -
Joined
-
Last visited
Never
Posts posted by khr2003
-
-
did you use coockies? if so check this php function http://php.net/manual/en/function.setcookie.php, you can incerease the time paramater as much as you like. Otherwise you have to post the code for us to look
-
It really depends on the way you are learning php and if you have previous experience with other programming languages (or at least you are good at maths).
like anything else in the world, practicing the main key to learn php. For a start try to download light open source programs that really do basic operations, then try to add something to it. Forum like this one and many others exist so people can exchange experiences, ask questions and get answers so do not be afraid to ask (or search if you think your question is simple).
If you can master small scripts then large scripts will not be an issue since they follow the same logic and apply similar principles only on a large scale.
hope this helps
-
my mistake. I concentrated on the php code and forgot the html part
-
I think premiso solution should work fine:
look at this code:
$i = '0'; echo '<table>'; foreach ( $listPilots as $Pilot ) { echo '<tr><td>$pilot</td></tr>'; // if number of pilots is divisable by four (or any other number you choose) without a reminder then close the table and start a new one if (($i%4) == 0) { // start new table echo '</table><table> } echo '</table>
-
option tags should be included in <select name="NAME"></select> tags. In order to retrive the value of the option selected you can just use the name of the select as a reference.
like this:
echo $_POST['NAME'];
or any other that suites your application
-
why don't you include it as a simple javascript code without the echo command.
-
you can do it this way:
while($row = mysql_fetch_array($result)) { // extract row values for an easier use of vars extract($row); // this will generate a multi-dimensional array // look at the format of the array, you can modify it as you need $array[$field1][$field2] = $field3; } // print the result, just checking echo nl2br(print_r($array,1));
-
Are you asking for an ftp server that transfer file across different servers and not just a basic upload/download ftp script?
if that is the case, I would ask why?
-
one way is to include it into a function like this:
function header($title) { echo '<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8" /> <title>.'$title'.</title> <link href="master.css" rel="stylesheet" type="text/css" /> </head> <body>'; }
then you can use the function anywhere you like:
header('This is page One');
header('This is page Two');
header('This is page Three');
and so on
-
if the date values are stored in timestamp format then you can just use a simple subtract operation in php to get the difference then you can convert the timestamp to a human-readable format. Otherwise, convert the date to timestamp, do the subtraction then convert back to a human-readable date.
To see numerous examples on what I have just explained just google "convert to time stamp php" "time difference php"
-
what are you trying to achieve here? what is the end result will be?
-
check the content of the_permalink function. it is probable that it is returning a correct value
-
on this line:
while($category->parent_id <= 0) {
are you trying to compare values bigger than zero or less than zero. the statement shows that you want to loop all values less or equal to zero
-
why not put these two line within the function rather than outside it:
$ini_array = parse_ini_file("configuration.ini", true); $febskip = $ini_array['feb']['skip'];
variables within function are local. so you might want to try this:
<?PHP function create_month_array($month, $year) { $ini_array = parse_ini_file("configuration.ini", true); $febskip = $ini_array['feb']['skip']; $curDay = mktime(0,0,0,$month,1,$year); $numDays = (int) date('t', $curDay); switch($month){ case 2: $skip = array($febskip); break; } $pass = array(); $days = array(); for ($i = 1, $prev = 1; $i <= $numDays; ++$i, $curDay = strtotime('+1 day', $curDay)) { if (date('N', $curDay) >= 6) { $days[$i] = null; } else if (in_array($i, $skip)) { $prev = !$prev; $days[$i] = null; } else if (in_array($i, $pass)) { $days[$i] = null; } else { $prev = $days[$i] = (int) !$prev; } } echo $febskip; var_dump($febskip); //var_dump($days); }
-
This problem because of two php functions conflicting. If "magic_quotes_gpc" is enables (assuming that you are using php version less than 5.3.0) then you should not use mysql_real_esacpe_string when inserting data into database.
you can use this code to over come this issue:
if(!get_magic_quotes_gpc()) { $text= mysql_real_escape_string($text); }
-
try this code:
<?php include ('E:\domains\s\domain\user\private\mysql_connect.php'); mysql_select_db("pix", $con); $result = mysql_query("SELECT * FROM mpora); while($row = mysql_fetch_assoc($result)) { echo '<div class="panel">'; echo '<div class="inside">'; echo $row['embed_url']; echo '<h2>' . $row['heading'] . '</h2>'; echo '<p>' . $row['caption'] . '<p>'; echo '</div>'; echo '</div>'; } mysql_close($con); ?>
also try to update your php version
-
Your question is related to javascript more than it does to php coding. if you google populate menus I think you will find some ready made scripts on the two or even three levels menus.
-
Put the code for the word display within the while statment of the query and not outside it.
like this:
<?php mysql_connect("localhost", "Master", "pword"); mysql_select_db("db"); $letter = htmlentities($_GET['search']); $user = mysql_query("SELECT * FROM Stacks WHERE keywords LIKE '$letter%' ORDER BY keywords")or die (mysql_error()); $words_output = array(); while($rowz = mysql_fetch_array($user)){ $keyword = $rowz['keywords']; $name = $rowz['name']; $bad = mysql_query("SELECT * FROM Stacks WHERE keywords LIKE '$letter%' ORDER BY keywords")or die (mysql_error()); $num_rows = mysql_num_rows($bad)or die (mysql_error()); if ($num_rows == 0) { echo "<font face='Courier New' font size=18px font color=#FF9900>$letter</font><br /><br />"; echo "<font face='Courier New' font size=3px font color=#FBB917>No Stacks</font><br>"; } else { if(!in_array($keyword,$words_output)) { $words_output[] = $keyword; echo "<font face='Courier New' font size=18px font color=#FF9900>$letter</font><br /><br />"; echo "<font face='Courier New' font size=3px font color=#FBB917><a href='stack.php?search=$keyword&submit=Go!' style='text-decoration: none';>$keyword</a></font><br>"; } } } ?>
-
both script work fine in my PC. I don't think it is a php problem. Try changing the encoding from the browser itself and see what happens.
-
I think it is hard to determine the difference without knowing the contents of the file templates.txt. My guess is that there is a space or some other letters that cange the value of Sha1.
Since your are looking for a string within a text why don't you use strstr function. This operator "==" requires both sides of the equation to be exactly the same while strstr function searches a whole text for one word.
check it out here http://php.net/manual/en/function.strstr.php
-
hi
you can add an array that grabs the values of the foreach loop and then read it somewhere else, like this:
<?php foreach ($products as &$value) { $partnumber = '<PartNumber>'.$value["sku"].'</PartNumber>'; $array[$value] .= $value; } // then outside the loop echo $array[The value of the array to be printed]; ?>
-
Try this:
<head> <title>FotoSnap.net</title> <meta http-equiv="content-type" content="text/html; charset=UTF-8" /> <meta name="description" content="description" /> <meta name="keywords" content="keywords" /> <meta name="author" content="author" /> <link rel="stylesheet" type="text/css" href="style.css" /> </head> <body> <div id="container"> <div id="top"> <div id="header"> </div> <div id="menu"> <a href="#">Upload</a><a href="#">*News</a><a href="#">About</a><a href="#">Contact</a><a href="#">Register</a> </div> </div> <div id="body"> <center> <div class="post"> <h1>Upload a photo!.</h1> <p> <?php //define a maxim size for the uploaded images in Kb define ("MAX_SIZE","100"); //This function reads the extension of the file. It is used to determine if the file is an image by checking the extension. function getExtension($str) { $i = strrpos($str,"."); if (!$i) { return ""; } $l = strlen($str) - $i; $ext = substr($str,$i+1,$l); return $ext; } //This variable is used as a flag. The value is initialized with 0 (meaning no error found) and it will be changed to 1 if an errro occures. If the error occures the file will not be uploaded. $errors=0; //checks if the form has been submitted if(isset($_POST['Submit'])) { //reads the name of the file the user submitted for uploading for ($i = 0; $i < count($_FILES['image']['name']; $i++){ $image=$_FILES['image']['name'][$i]; //if it is not empty if ($image) { //get the original name of the file from the clients machine $filename = stripslashes($_FILES['image']['name'][$i]); //get the extension of the file in a lower case format $extension = getExtension($filename); $extension = strtolower($extension); //if it is not a known extension, we will suppose it is an error and will not upload the file, otherwize we will do more tests if (($extension != "jpg") && ($extension != "jpeg") && ($extension != "png") && ($extension != "gif") && ($extension != "bmp") && ($extension != "tif") && ($extension != "tiff")) { //print error message echo '<h1>Wrong file format!, !</h1>'; $errors=1; } else { //get the size of the image in bytes //$_FILES['image']['tmp_name'] is the temporary filename of the file in which the uploaded file was stored on the server $size=filesize($_FILES['image']['tmp_name'][$i]); //compare the size with the maxim size we defined and print error if bigger if ($size > MAX_SIZE*1024) { echo '<h1>You have exceeded the size limit!</h1>'; $errors=1; } //we will give an unique name, for example the time in unix time format $image_name=time().'.'.$extension; //the new name will be containing the full path where will be stored (images folder) $newname="images/".$image_name; //we verify if the image has been uploaded, and print error instead $copied = copy($_FILES['image']['tmp_name'][$i], $newname); if (!$copied) { echo '<h1>Copy unsuccessfull!</h1>'; $errors=1; }}}} //If no errors registred, print the success message if(isset($_POST['Submit']) && !$errors) { if ($image) { echo "<h1>Image Uploaded Successfully!</h1>"; echo "<textarea rows=\"2\" cols=\"40\">http://fotosnap.net/images/$image_name</textarea></form>"; } else { echo "<h1>Error: You did not select a image to upload!</h1>"; } } } ?> <!--next comes the form, you must set the enctype to "multipart/frm-data" and use an input type "file" --> <form name="newad" method="post" enctype="multipart/form-data" action=""> <table> <tr><td><input type="file" name="image[]"></td></tr> <tr><td><input type="file" name="image[]"></td></tr> <tr><td><input type="file" name="image[]"></td></tr> <tr><td><input type="file" name="image[]"></td></tr> </table> <br /> <br /> <table> <tr><td><input name="Submit" type="submit" value="Upload image"></td></tr> </table> </form> </p> </div> </center> <div class="post"> <h1>FotoSnap.net</h1> <p>Welcome to FotoSnap!, FotoSnap is a free any easy way to host photo's for everybody, With no limit on trafic!.</p> <p>FotoSnap is here to provide a easy way to upload and share photos across the web.</p> </div> <div class="post"> <h1>What images do we support?</h1> <p> <ul> <li>.jpg</li> <li>.bmp</li> <li>.gif</li> <li>.tif</li> <p>And more soon!</p> </ul> </p> </div> <div class="post"> <h1>Rules:</h1> <p> You cannot upload the following type of images: <ul> <li>Pornography</li> <li>Images that do not belong to you</li> <li>Images showing harm being inflicted on humans/animals.</li> <p>Breaking these rules will result in the image being deleted by a administrator!.</p> </ul> </p> </div> </div> <div id="footer"> <p class="right"><a href="http://templates.lanmust.org">Custom design by</a> <a href="http://webdesign.lanmust.org">Mapers</a></p><p>Copyright © 2009 <a href="#">FotoSnap.net</a></p> <center><p><a rel="license" href="http://creativecommons.org/licenses/by-nc-nd/2.0/uk/"><img alt="Creative Commons License" style="border-width:0" src="http://i.creativecommons.org/l/by-nc-nd/2.0/uk/80x15.png" /></a><br /><a xmlns:cc="http://creativecommons.org/ns#" href="FotoSnap.net" property="cc:attributionName" rel="cc:attributionURL"></a><a rel="license" href="http://creativecommons.org/licenses/by-nc-nd/2.0/uk/"></a></p></center> </div> </div> </body>
-
Google does not, and can not, read your source code rather google reads the whole page, so yes; if you include navigation in all pages (and you should - professionally speaking) google will crawl your website the same way if you had a navigation in everypage
-
array_search help
in PHP Coding Help
Posted
it is because you code is placed outside the foreach loop, and you are echoing the wrong variable. Also are you searching for $array in $lines or otherwise? in any way try this: