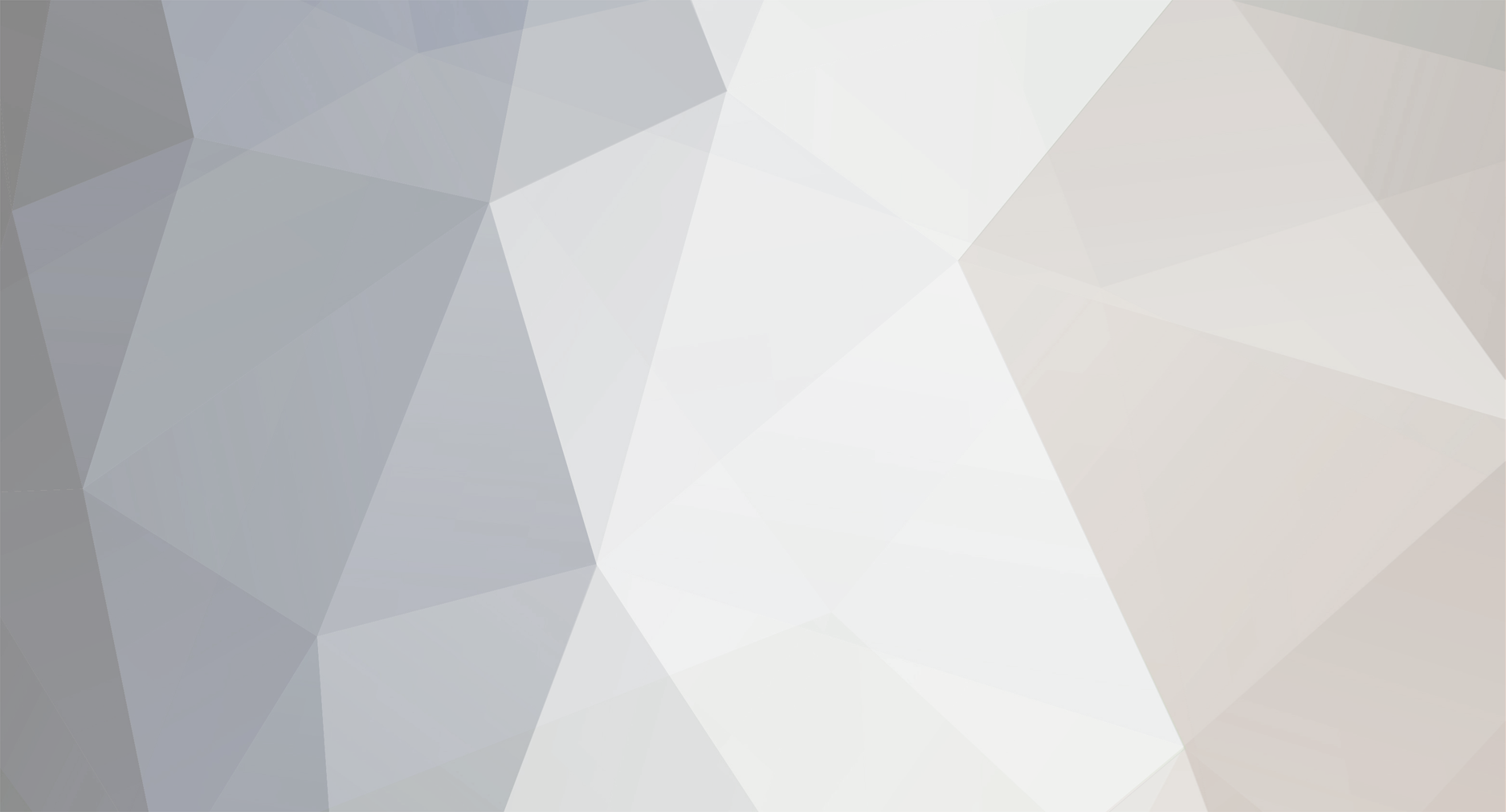
scheda
Members-
Posts
20 -
Joined
-
Last visited
Never
Profile Information
-
Gender
Not Telling
scheda's Achievements

Member (2/5)
0
Reputation
-
I'm having a rough time getting an AS3 application working with a PHP script. The PHP script takes POST variables and then sends an email using SMTP. When running it through a standard HTML form, the PHP side works perfectly. However, when I send the exact same POST variables through AS3 to the script, I get this error. Failed to set sender: phpemailtesting@gmail.com [sMTP: Failed to write to socket: not connected (code: -1, response: )] So, I'm not really sure what the issue is here... Here's some code that might help. //print_r($_POST); require_once('Mail-1.2.0/Mail.php'); $from = isset($_POST['emailfrom']) ? $_POST['emailfrom'] : ''; $to = isset($_POST['emailsubject']) ? $_POST['emailto'] : ''; $bcc = isset($_POST['bcc'])? $_POST['bcc'] : ''; $subject = isset($_POST['emailsubject']) ? $_POST['emailsubject'] : ''; $body = isset($_POST['emailbody']) ? $_POST['emailbody'] : ''; $ssl = isset($_POST['ssl']) ? $_POST['ssl'].'://' : ''; $smtp_server = isset($_POST['smtpserver']) ? $_POST['smtpserver'] : ''; $password = isset($_POST['emailpassword']) ? $_POST['emailpassword'] : ''; $port = isset($_POST['port']) ? $_POST['port'] : ''; $success_counter = 0; $fail_counter = 0; $bigerror; if($from!='' && $to!='' && $subject!='' && $body!='' && $smtp_server!='' && $password!='' && $port!='') { if($bcc!='') { $bcc_array = explode(',',$bcc); } $host = $ssl . $smtp_server; $smtp = Mail::factory('smtp', array('host'=>$host, 'port'=>$port, 'auth'=>true, 'username'=>$from, 'password'=>$password)); $header = array('From'=>$from, 'To'=>$to, 'Subject'=>$subject); $mail = $smtp->send($to, $header, $body); if (PEAR::isError($mail)) { $bigerror = $mail->getMessage(); $fail_counter++; //echo("<p>Message failed".$to." :<br />" . $mail->getMessage() . "</p>"); } else { $success_counter++; //echo("<p>Message successfully sent to <b>". $to ."</b>!</p>"); } for($counter=0; $counter<count($bcc_array) && $counter<=99; $counter++) { $header = array('From'=>$from, 'To'=>$bcc_array[$counter], 'Subject'=>$subject); $mail = $smtp->send($bcc_array[$counter], $header, $body); if (PEAR::isError($mail)) { $bigerror = $mail->getMessage(); //echo("<p>Message failed".$bcc_array[$counter]." :<br />" . $mail->getMessage() . "</p>"); $fail_counter++; } else { //echo("<p>Message successfully sent to <b>". $bcc_array[$counter] ."</b>!</p>"); $success_counter++; } } //echo "<br /><br />Successful emails: " . $success_counter; //echo "<br />Failed emails: " . $fail_counter; echo $success_counter.",".$fail_counter.",".$bigerror; } else { echo "error"; //echo '<p>Some information is missing, please try again!</p>'; } Then, the AS if anybody's interested in seeing it. //Create loader to load emailer.php var loader:URLLoader = new URLLoader(); var request:URLRequest = new URLRequest("http://tendollartools.com/testmail/emailer.php"); //Create POST variables var variables:URLVariables = new URLVariables(); variables.emailfrom = preferences["smtpLogin"]; variables.emailto = preferences["smtpLogin"]; variables.smtpserver = preferences["smtpServer"]; variables.emailpassword = preferences["smtpPassword"]; variables.ssl = preferences["smtpSSL"]; variables.port = preferences["smtpPort"]; variables.bcc = emails; variables.emailsubject = preferences["emailSubject"]; variables.emailbody = preferences["emailBody"]; //Set method to POST and set variables as the vars to send to emailer.php request.method = URLRequestMethod.POST; request.data = variables; //Load emailer.php and run the onEmailComplete function when finished loading loader.dataFormat = URLLoaderDataFormat.TEXT; loader.addEventListener(Event.COMPLETE, onEmailComplete); loader.load(request); The preferences object has all the correct information in it... so this is where I'm confused. Why will the exact same POST information work from an HTML form, but not when sent through AS?
-
I'm having a little trouble piecing together the exact formula I need to figure this out. I am getting results from the Google Search API, which comes in groups of 8 - which makes counting kind of annoying. Anyway, I'm parsing through the results to find a user defined URL, so the URL might be located on page 2 (results 9-16) in the second spot, making it result #10 in the total results. So what I need to do, is come up with a way to extrapolate the position number. I considered having a variable that counted every time a row was found that didn't have the URL in it, but that wasn't working properly, so I'm back to the real math. Any help here would be much obliged.
-
Okay, so I keep crashing my server while trying to get a simple script to run. Firstly, here's the code. <?php $dbhost = 'localhost'; $dbuser = 'root'; $dbpass = ''; $conn = mysql_connect($dbhost, $dbuser, $dbpass) or die('Error connecting to mysql'); $dbname = 'businessletters'; mysql_select_db($dbname); $files = getFiles("parseme.txt"); for($i=0;$i<200;$i++) { $file = getReadFile($files[$i][0]); insertIntoDb($files[$i][1], $file); //echo "Inserted successfully - " . $files[$i][0] . "<br />"; } function getFiles($file) { $fh = fopen($file, "rb"); $data = fread($fh, filesize($file)); fclose($fh); $dataArr = explode("\n", $data); for($i=0;$i<count($dataArr);$i++) { $dataArr[$i] = explode(",", $dataArr[$i]); } return($dataArr); } function getReadFile($file) { $fh = fopen($file, "rb"); $data = fread($fh, filesize($file)); fclose($fh); return $data; } function insertIntoDb($title, $body) { $sql = "insert into letters values('', '".mysql_real_escape_string($title)."', '".mysql_real_escape_string($body)."')\n"; //mysql_query($sql) or die("Error inserting"); echo $sql; } mysql_close(); ?> And now, here's what it's supposed to do. Open and read File A - this file has file names and titles in a CSV file. File,Title is the format. Then the loop starts. Here I would like to do everything in one run, which could be done by counting the records in the CSV file, which I can do with count(), but even 200 is too much. I have 600. Anyway, it goes through the CSV file and opens every file referenced and pulls out the content. Then it creates a simple SQL statement where the content is entered into the database. And the problem is that Apache keeps crashing on me... There must be a better way for me to do this without having my server crash on me!
-
Oh that works perfectly. Thank you for clarifying!
-
I'm getting a syntax error in this code. Basically I'm printing out a form, and depending on the value of an option, I want "checked" to be printed out. I was getting a syntax error by using the non-shorthand version of an if statement too. Any ideas why? '<input type="radio" name="position" value="1" '.(get_option("shPosition") == 1 ? echo "checked" .' /> Top <input type="radio" name="position" value="0" '.(get_option("shPosition") == 0 ? echo "checked" .' /> Bottom'
-
Exactly, that's what I'm after. Sorry, should have clarified!
-
I'm having trouble with this regex... \<span class="yls-rs-listing-title" id="yls-rs-listing-title-\.*\">\.*\</span>\ Here's a sample string that it should be finding it in. <a rel="rel:Link" href="http://local.yahoo.com/info-14094857-curry-phillip-dvm-spca-of-north-brevard-wellness-titusville;_ylt=Aqjid9bbdVokS3CoB.iHLzCHNcIF;_ylv=3?csz=Titusville%2C+FL+32780" class="fn org yltiefix " name="14094857"><span class="yls-rs-listing-title" id="yls-rs-listing-title-14094857" property="dc:title" content="Curry, Phillip DVM - SPCA of North Brevard Wellness">Curry, Phillip DVM - SPCA of North Brevard Wellness</span></a> Any idea why this isn't working?
-
Here it is. preg_match_all('<a href="(*)register.php" class=l>',$html,$links);
-
Hi, So I'm having some trouble with regex... of course... Here's what's happening. I'm building a Google scraper. It goes out, does a search for forums register pages, and grabs the links to them all. I have everything working, I just need the regex to grab the proper links. In my head I see the script grabbing all links that have register.php inside the href area. This is what my regex looks like right now. <a href="(*)register.php" class=l> However, I get this error when it runs. Warning: preg_match_all() [function.preg-match-all]: Compilation failed: nothing to repeat at offset 9 in C:\wamp\www\forumfinder.php on line 33 I'm guessing it has to do with the quotes I have in there. Any thoughts on how to fix this? Thank you in advance!
-
Yep, that did it. Thanks!
-
First of all, here's the code I'm having trouble with. $vals[1][1] = preg_match("input name=\"authenticity_token\" type=\"hidden\" value=\"(*)\"", $twit_source); What I'm trying to do is extract a token from the value of the input field. It's on the page three times, but I only need it once. I want to store it in $vals[1][1]. I'm getting this error. Warning: preg_match() [function.preg-match]: Delimiter must not be alphanumeric or backslash in C:\wamp\www\twitter-poster\index.php on line 11 Thanks for any help you can lend.
-
SimpleXML, Base64_encode, and form submission issue.
scheda replied to scheda's topic in PHP Coding Help
Well, that would explain it then! Changing the XML is easy, so I'll just do that instead. Thanks! -
Hey all, Should be relatively easy, but my brain is fizzled at the moment, so I can't see the issue here. I'm putting a >> on the lines in question. <?php include("functions.php"); if (isset($_POST['title'])) { //set vars $title = $_POST['title']; $description = $_POST['description']; $keywords = $_POST['keywords']; $adsense_bar = base64_encode($_POST['adsense-bar']); $adsense_box = base64_encode($_POST['adsense-box']); $adsense_square = base64_encode($_POST['adsense-square']); $article_title = $_POST['article-tite']; $article_content = base64_encode($_POST['article-content']); //edit file $xml -> title = $title; $xml -> description = $description; $xml -> keywords = $keywords; >>$xml -> adsense-bar = $adsense_bar; >>$xml -> adsense-box = $adsense_box; >>$xml -> adsense-square = $adsense_square; $xml -> article -> title = $article_title; $xml -> article -> body = $article_content; //save file file_put_contents('data.xml', $xml->asXml()); } header("Location: admin.php"); ?> When running those lines, I'm getting this error. Of course the script breaks at that point and goes no further. If I comment out line 18, line 19 spouts the error. Again for 20. Any idea what could be causing this? (And yes - I should be doing this in OO, but this is a quick run through to make sure I can get everything to work before real production)
-
Yeah! That was it. Thanks a ton. I guess this was one of those things where I just need to do the actual math myself and see where that takes me. Thanks again.
-
I'm working on a web statistics program and have run into a little snafoo with a function. I think it's just because my brain has stopped working for the day - but if I could get another pair of eyes on it, that'd be great. Here's the function. //get hits per day in a comma seperated list public function get_daily_hits($days) { $daily_hits = array(); $ip = $_SERVER['REMOTE_ADDR']; $time = time(); $num = 0; for($i = $days; $i > 0; $i--) { $days_in_seconds = $i * 86400; $b = $days_in_seconds + 86400; foreach($this -> xml -> hit as $a) { if ($a -> ip != $ip && $a -> time > ($time - $days_in_seconds) && $a -> time < $b) {//FIX THIS LINE! $num++; } } array_push($daily_hits, $num); $num = 0; } $daily_hits = implode(',', $daily_hits); return $daily_hits; } The if statement in the foreach is where I'm having trouble. The function is supposed to create a comma delineated string of how many hits were registered on each day for the number of days entered as an argument. So, as you can see in the if statement, what I'm trying to do is... 1. Weed out the admin's IP 2. Start the search at the end of the day being searched 3. End the search at the beginning of the day. Included below is a sample XML file that I'm trying to pull from. <?xml version="1.0"?> <visits> <hit> <id>0</id> <ip>97.101.183.201</ip> <referrer>http://www.google.com</referrer> <time>1254013189</time> </hit> <hit> <id>1</id> <ip>127.0.0.1</ip> <referrer>http://www.google.com</referrer> <time>1253926689</time> </hit> </visits> It's being read just fine, but the function is just displaying a comma delimited string of 0's - which doesn't match up with the data in the XML file. Thanks in advance for any help you can lend!