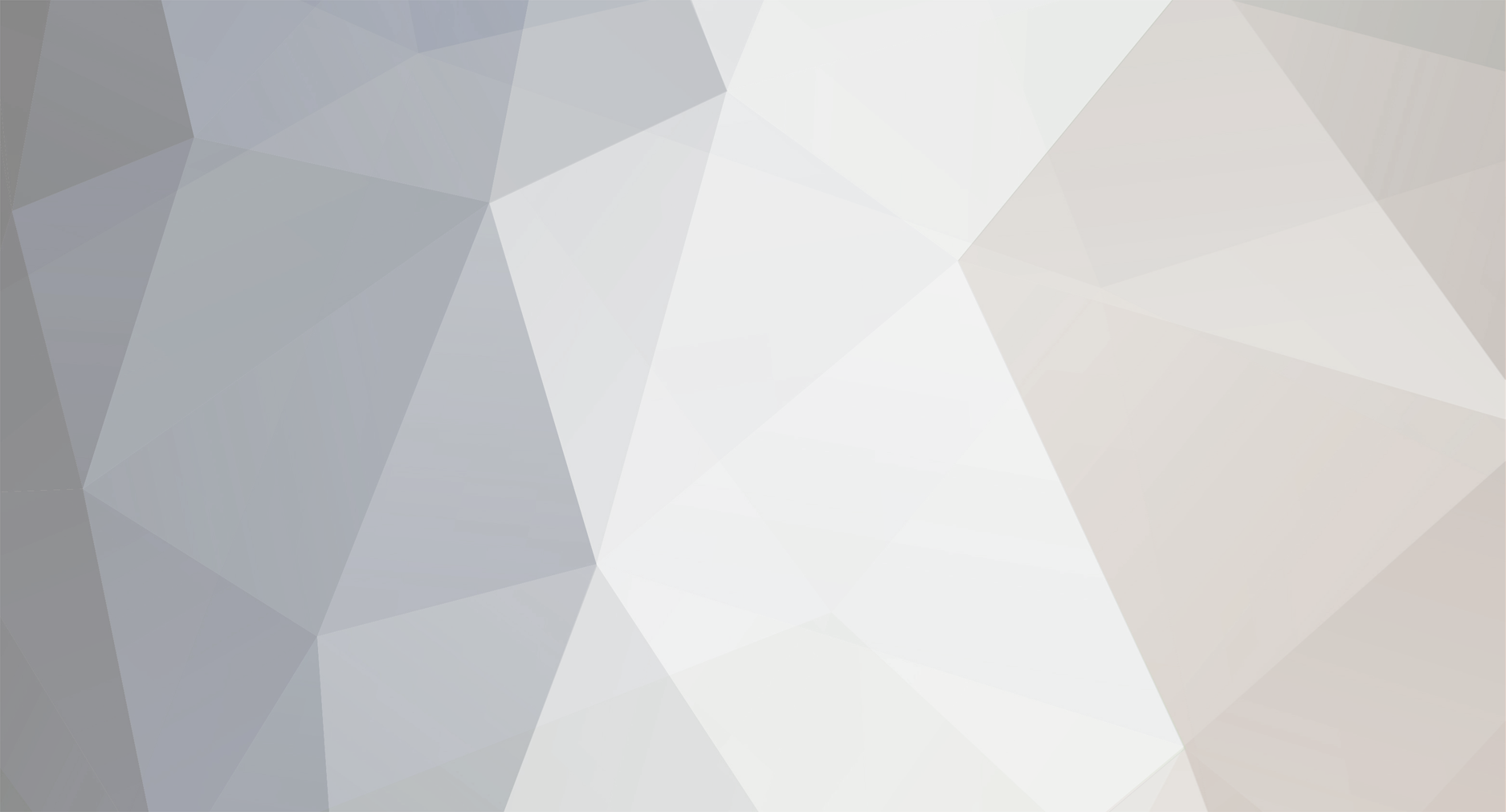
kaiman
Members-
Posts
104 -
Joined
-
Last visited
Everything posted by kaiman
-
Hi Everyone, I am working on implementing a blog comment system using the query below to display comments. My question is what is the best way to do a row count for the id column with this query or do I need to do a second db query to accomplish this? The purpose of this is to echo out the number of comments for that post, before displaying them. Any help is appreciated. Thanks in advance, kaiman <?php // get url variables $post_id = mysql_real_escape_string($_GET['id']); include ("../../scripts/includes/nl2p.inc.php"); // connects to server and selects database include ("../../scripts/includes/dbconnect.inc.php"); // table name $tbl_name3="blog_comments"; // select info from comments database $result3 = mysql_query ("SELECT count(*) FROM $table_name3 WHERE id='$post_id' ORDER BY id DESC LIMIT 1") or trigger_error("A mysql error has occurred!"); if (mysql_num_rows($result3) > 0 ) { while($row = mysql_fetch_array($result3)) { extract($row); // display number of comments // display date $row_date = strtotime($row['date']); putenv("TZ=America/Denver"); echo "<p class=\post\">On ".date('F, jS, Y', $row_date)." "; // display commenter name if($row['url'] == "") { echo $row['name']." wrote:</p>\n"; } else { echo "<a href=\"".$row['url']."\" target=\"_blank\">".$row['name']."</a> wrote:</p>\n"; } // display content $comments = $row['comment']; echo nl2p($comments); } } else { echo "<p class=\"large_spacer\">No Comments</p>"; } ?>
-
@ mjdamato Thanks again for the suggestions and input. That is the answer I was looking for. I will use hidden input fields to get the post id and add it to the comments and categories tables. Thanks for the advice on using mysql_real_escape_string and url's. I typically use it on any form fields going into a db, but will also use it when I pass url's to prevent sql injection there as well. I am not totally sure by looking at your code that I understand how to do the join, but let me get the rest of this sorted out and I will post back if I have issues related to the last part. Thanks again, kaiman
-
@mjdamato Thanks for the reply and helping me spell out more clearly what I am looking for. Your advice on using two tables for categories is helpful. I was thinking of just having articles belong to one category, but your suggestion may make me rethink that scenario... What I am ultimately trying to do with the categories part is build a link list in the sidebar (like wordpress does) that lists the categories for users to choose from and then when they click on one of the category links it will take them to a page that lists all articles/posts in that category. Sorry about the confusion on the second part related to get, post and joins. What I am trying to determine is what is the best way to get the id of the post into the other two tables in the db so that when a user views a particular post that the comments and category related to that post show up. Your code examples more or less answered the query part, but how would I capture the id of the post from the first table and use it to display the correct comments from the outset? Should I use a hidden field in the comments form something like this: $post_id = mysql_real_escape_string($_GET['id']); <input type="hidden" value="<?php $post_id ?>" name="article_id" /> And then insert it into the comments table so that the id (or post_id) and article_id are the same? Like I said this is my first time doing this, so I am wondering if this is the best/safest way to do this? Thanks again for your help, kaiman T
-
* BUMP * Please let me know if I'm not being clear... Thanks!
-
Hi Everyone, I am working on developing a blog/comment system for my website. I have 3 tables, the first a blog post table with a row/primary key of id that is auto incremented as each new article is created via a form. The second table is a comments table with a row of article_id and the third table is a category table with an article_id row as well. From the main blog page I have links that will take users to each individual blog post using $_GET like so: http://www.mydomain.com/blog/article/?id={$row['id']} From here I would like to be able to display the comment for that particular article below the article and also have a sidebar with links to blog categories which will then display each post according to whether they are in that category or not. My question is two-fold: First, what is the best way to pull the id field from the blog/post table, form, or url and enter it into the article_id rows of the second two tables? Should I use a hidden field, the URL id via $_GET, using a join, or what? Secondly: What is the best way to compare and display those rows so that the appropriate comments are being shown for the correct article, etc. This is my first time doing this so I am grateful for any help or suggestions. Thanks, kaiman
-
@ BlueSkyIS Maybe I'm a bit dense but I'm not sure I am following you on your first point. Can you elaborate with an example? BTW, thanks for the info on the OS line carriage differences, I thought it was something like that. kaiman
-
Hi all, I am working on debugging a function that converts single breaks in a form text area field into HTML break tags and double breaks into paragraph tags before sticking them into a MySQL DB, so that they can be retrieved and properly displayed on another page. The script seems to work fine for the most part except it seems to be trimming the text around the break tags off. My assumption is in the way that I am trimming the string, but I am not sure. Here is my code: <?php // turns line breaks in forms into HTML <br> <br/> or <p></p> tags function nl2p($string, $line_breaks = true, $xml = true) { // remove existing HTML formatting to avoid double tags $string = str_replace(array('<p>', '</p>', '<br>', '<br/>'), '', $string); // convert single line breaks into <br> or <br/> tags if ($line_breaks == true) return '<p>'.preg_replace(array("/([\n]{2,})/i", "/([^>])\n([^<])/i"), array("</p>\n<p>", '<br'.($xml == true ? '/' : '').'>'), trim($string)).'</p>'; // convert double line breaks into <p></p> tags else return '<p>'.preg_replace("/([\n]{1,})/i", "</p>\n<p>", trim($string)).'</p>'; } ?> <?php // example uses $text = "Hello. My name is Kai."; echo nl2p($text) . "\n"; $text = "Your IP is: {$_SERVER["REMOTE_ADDR"]}"; echo nl2p($text) . "\n"; ?> Here is what it is returning: <p>Hello.</p> <p>My name is Kai.</p> <p>Your IP is<br/>23.123.123.123</p> And here is what it should be returning (notice the missing : and first number of the IP address in the code above): <p>Hello.</p> <p>My name is Kai.</p> <p>Your IP is:<br/>123.123.123.123</p> Also, I remember reading somewhere about Mac, Linux, and Windows OS using different break tags could this have something to do with it (I am on Mac OS X), and how would I implement that into this script. Any help or suggestions are appreciated. Thanks, kaiman
-
parse error when making a form remember last user input
kaiman replied to pandu's topic in PHP Coding Help
Try: <?php if (isset($_POST['synonymsearch'])) echo { $_POST ['synonymsearch']; } ?> -
@MMDE Ah, yes I see, thanks for pointing that out. @ BK87 Thanks mysql_fetch_array worked. @ thorpe Okay, thanks for the alternative method... Sarcasm on the mysql error noted. I tend to use error logs to review problems instead. This is really what the message should say: trigger_error("A useless error message displayed to hackers who want to learn the directory paths on the server!"); Problem solved, kaiman
-
Hi again everyone, I am trying to compare a php variable to the value of a row in mysql, but keep getting the following error: Wrong perameter count for mysql_result() What syntax would I use to accomplish this? Here is my code: // connects to server and selects database. include ("../includes/dbconnect.inc.php"); // table name $table_name = "availability"; // split up date into 3 separate fields $date = "12/25/2010"; $date_split = explode("/", $date); $month = $date_split[0]; $day = $date_split[1]; $year = $date_split[2]; // check if earth room is already reserved $taken = "Reserved"; $sql = "SELECT earth_room FROM $table_name WHERE month='$month' AND day='$day' AND year='$year' LIMIT 1"; $result = mysql_query($sql) or trigger_error("A mysql error has occurred!"); $row = mysql_result($result); if($row == $taken){ echo "Room Already Reserved"; } else{ echo "Room Available"; } Thanks for the help, kaiman
-
@ spinsfil That worked the charm. Thanks for the help! Cheers, kaiman
-
Okay, I'm back already with another question: Is it possible to use strtotime to display the date like this(?): <?php $get_date = strtotime($_GET['month'],$_GET['day'],$_GET['year']); echo date('F, j, Y', $get_date); ?> Or: <?php $row_date = strtotime($row['month'],$row['day'],$row['year']); echo date('F, j, Y', $row_date); ?> From this script: // get url variables $month = $_GET['month']; $year = $_GET['year']; $day_num = $_GET['day']; // connects to server and selects database. include ("../includes/dbconnect.inc.php"); // table name $table_name = "availability"; // query database for events $result = mysql_query ("SELECT * FROM $table_name WHERE month='$month' AND year='$year' AND day='$day_num' LIMIT 1") or die(mysql_error()); if (mysql_num_rows($result) > 0 ) { while($row = mysql_fetch_array($result)) { extract($row); echo "<h1>Availability for ".$row['month'] . "/" . $row['day'] . "/" . $row['year'] . "</h1>"; echo " <ul>"; echo " <li>Earth Room: " . $row['earth_room'] . "</li>"; echo " <li>Air Room: " . $row['air_room'] . "</li>"; echo " <li>Fire Room: " . $row['fire_room'] . "</li>"; echo " <li>Water Room: " . $row['water_room'] . "</li>"; echo " </ul>"; } } else { echo "<h1>Availability for ".$_GET['month'] . "/" . $_GET['day'] . "/" . $_GET['year'] . "</h1>"; echo " <ul>"; echo " <li>Currently no reservations.</li>"; echo " </ul>"; } I have my date broken down into 3 rows like this: `day` varchar(2) NOT NULL default '', `month` varchar(2) NOT NULL default '', `year` varchar(4) NOT NULL default '', Currently when I run strtotime it gives me this warning and then returns the Epoch date - December, 31, 1969: Warning: strtotime() expects at most 2 parameters, 3 given Is there any way around this or do I need to adjust my DB rows somehow? Any help is appreciated. Thanks again, kaiman
-
@ PFMaBiSmAd Right you are! Thanks for looking over my shoulder while I stumble through this. Regards, kaiman
-
@ shlumph - okay that resolved the MySQL error - thanks! @ solon - yep that is what I was looking for, that seems to be working, but the issue I am having now is that it doesn't seem to be echoing out the row info from the db. One days there is no reservations it prints out: Currently no reservations. As expected. However, on days where I have entered information into the DB for testing it prints out empty rows such as: Current availability for 12/25/2010 Earth Room: Air Room: Fire Room: Water Room: Here is my DB structure: CREATE TABLE `availability` ( `id` int(11) NOT NULL auto_increment, `day` varchar(2) NOT NULL default '', `month` varchar(2) NOT NULL default '', `year` varchar(4) NOT NULL default '', `earth_room` varchar(25) NOT NULL default 'Available', `air_room` varchar(25) NOT NULL default 'Available', `fire_room` varchar(25) NOT NULL default 'Available', `water_room` varchar(25) NOT NULL default 'Available', PRIMARY KEY (`id`) ) ENGINE=MyISAM DEFAULT CHARSET=latin1 AUTO_INCREMENT=1 ; And here is my working code: // get url variables $month = $_GET['month']; $year = $_GET['year']; $day_num = $_GET['day']; // connects to server and selects database. include ("../includes/dbconnect.inc.php"); // table name $table_name = "availability"; // query database for events $result = mysql_query ("SELECT id FROM $table_name WHERE month='$month' AND year='$year' AND day='$day_num' LIMIT 1") or die(mysql_error()); if (mysql_num_rows($result) > 0 ) { while($row = mysql_fetch_array($result)) { extract($row); echo "<h1>Availability for ".$_GET['month'] . "/" . $_GET['day'] . "/" . $_GET['year'] . "</h1>"; echo " <ul>"; echo " <li>Earth Room: " . $row['earth_room'] . "</li>"; echo " <li>Air Room: " . $row['air_room'] . "</li>"; echo " <li>Fire Room: " . $row['fire_room'] . "</li>"; echo " <li>Water Room: " . $row['water_room'] . "</li>"; echo " </ul>"; } } else { echo "<h1>Availability for ".$_GET['month'] . "/" . $_GET['day'] . "/" . $_GET['year'] . "</h1>"; echo " <ul>"; echo " <li>Currently no reservations.</li>"; echo " </ul>"; } Any ideas? Thanks for the continued help, kaiman
-
I am working on a room availability calendar that has links for each day. When you click on a link it passes the day month and year in the URL to another page like this: echo "<td class=\"today\"> <a href=\"status.php?month=$month&day=$day_num&year=$year\">$day_num</a> </td>\n"; I can see that the correct information is being passed through the URL like this: .../status.php?month=12&day=9&year=2010 Then the information is supposed to be passed to the MySQL query, but here is my question: How do I do this? I have a DB table set up, but the query is currently returning a blank page. Here is my current query: // connects to server and selects database. include ("../includes/dbconnect.inc.php"); // table name $table_name = "availability"; // query database for events $result = mysql_query ("SELECT id FROM $table_name WHERE month=$month AND year=$year AND day=$day_num LIMIT 1") or die(); if (mysql_num_rows($result) > 0 ) { while($row = mysql_fetch_array($result)) { extract($row); echo "<h1>Current availability for ".$row['month'] . "/" . $row['day'] . "/" . $row['year'] . "</h1>"; echo " <ul>"; echo " <li>Earth Room: " . $row['earth_room'] . "</li>"; echo " <li>Air Room: " . $row['air_room'] . "</li>"; echo " <li>Fire Room: " . $row['fire_room'] . "</li>"; echo " <li>Water Room: " . $row['water_room'] . "</li>"; echo " </ul>"; } } else { echo " <ul>"; echo " <li>Currently no reservations.</li>"; echo " </ul>"; } Any help is appreciated. Thanks, kaiman
-
Okay, I've been looking at this far too long... please help!
kaiman replied to kaiman's topic in PHP Coding Help
thorpe, it's just like me to over complicate it. Thanks for the help, that worked! kaiman -
Hi All, I am trying to call a javascript pop-up window via a link by echoing out the html via php but keep getting parse errors: Parse error: syntax error, unexpected ')', expecting ',' or ';' Here is the code in question: echo "<td class=\"today\"> <a href=\"javascript:statusWindow('status.php?month=".$month."&day=".$day."&year=$year');\">"$day_num</a> </td>\n"; And here is the javascript function: <script type="text/javascript"> function statusWindow(url){ status_popupWin = window.open(url, 'status', 'resizable=yes, scrollbars=yes, toolbar=no,width=400,height=400'); status_popupWin.opener = self; } </script> Any help is appreciated. Thanks, kaiman
-
issues with highlighting current date in php calendar
kaiman replied to kaiman's topic in PHP Coding Help
Nevermind, I realized I was comparing two negatives in my if statement and it was always returning positive. Also forgot the month and year details. FYI for anyone who cares - here is my working code: while( $day_num <= $days_in_month ){ if($day_num == date('d') && $month == date('n') && $year == date('Y')) { // display today's date echo "<td class=\"today\"> $day_num </td>"; $day_num++; $day_count++; } else{ echo "<td> $day_num </td>"; $day_num++; $day_count++; } -
Hi everyone, I have a basic calendar that I am trying to change to highlight the current date. I have got the current date highlighted using an if else statement inside a while clause, however, the issue arises when I use the forward/previous links to go to a different month - the same day is highlighted on those months as well. Here is the code snippet in question (the entire code is below): while( $day_num <= $days_in_month ) { if(date('d') != $day_num) { echo "<td> $day_num </td>"; $day_num++; $day_count++; } // display today's date else { echo "<td class=\"today\"> $day_num </td>"; $day_num++; $day_count++; } My question is how do I get this calendar to display the current date, just on the current month. I assume there is something I am missing when it comes to handling the date(s) correctly, but what? Any help is appreciated. Thanks, kaiman Full code: // gets today's date $date = time () ; // puts the day, month, and year in seperate variables $day = date('d', $date) ; $month = (int) ($_GET['month'] ? $_GET['month'] : date('m')); $year = (int) ($_GET['year'] ? $_GET['year'] : date('Y')); // generate the first day of the month $first_day = mktime(0,0,0,$month, 1, $year) ; // get the month name $current_month = date('F', $first_day) ; // determine what day of the week the first day of the month falls on $day_of_week = date('D', $first_day) ; // determine blank days before start of month switch($day_of_week){ case "Sun": $blank = 0; break; case "Mon": $blank = 1; break; case "Tue": $blank = 2; break; case "Wed": $blank = 3; break; case "Thu": $blank = 4; break; case "Fri": $blank = 5; break; case "Sat": $blank = 6; break; } // determine how many days are in the current month $days_in_month = cal_days_in_month(0, $month, $year) ; // determine next month $next_month_link = '<a href="?month='.($month != 12 ? $month + 1 : 1).'&year='.($month != 12 ? $year : $year + 1).'">>></a>'; // determine previous month $previous_month_link = '<a href="?month='.($month != 1 ? $month - 1 : 12).'&year='.($month != 1 ? $year : $year - 1).'"><<</a>'; // start building the table echo " <table border=0 width=294>\n"; echo " <tr>\n"; // display month and year echo " <th> $previous_month_link </th>\n"; echo " <th colspan=4> $current_month $year </th>\n"; echo " <th> $next_month_link </th>\n"; echo " </tr>\n"; // display days of week echo " <tr>\n"; echo " <td width=42>S</td>\n"; echo " <td width=42>M</td>\n"; echo " <td width=42>T</td>\n"; echo " <td width=42>W</td>\n"; echo " <td width=42>T</td>\n"; echo " <td width=42>F</td>\n"; echo " <td width=42>S</td>\n"; echo " </tr>\n"; // counts the days in the week $day_count = 1; echo " <tr>\n"; // display blank days while ( $blank > 0 ) { echo " <td></td>\n"; $blank = $blank-1; $day_count++; } // sets the first day of the month to 1 $day_num = 1; // count the days in the month and display them while( $day_num <= $days_in_month ) { if(date('d') != $day_num) { echo "<td> $day_num </td>"; $day_num++; $day_count++; } // display today's date else { echo "<td class=\"today\"> $day_num </td>"; $day_num++; $day_count++; } // make sure we start a new row every week if ($day_count > 7) { echo " </tr>\n"; echo " <tr>\n"; $day_count = 1; } } // finish calendar while ( $day_count >1 && $day_count <=7 ) { echo " <td>\n"; echo " </td>\n"; $day_count++; } echo " </tr>\n"; echo " </table>\n";
-
display xml pubDate from mysql database with php
kaiman replied to kaiman's topic in PHP Coding Help
No go. There must be some other error that this statement is causing: I tried it as: date, 'date', and `date` Thanks again, kaiman -
display xml pubDate from mysql database with php
kaiman replied to kaiman's topic in PHP Coding Help
Okay now I am getting a mysql error: <b>Warning</b>: mysql_fetch_array(): supplied argument is not a valid MySQL result resource in <b>/home/mysite/public_html/projects/rft/rss/index.php</b> on line <b>14</b><br /> supposedly on line 14 which is this line: while ($row=mysql_fetch_array($result)){ Here is my complete code: <?php header('content-type: text/xml'); // connects to server and selects database. include ("dbconnect.inc.php"); // table name $tbl_name="news"; // select info from database $sql="SELECT *, UNIX_TIMESTAMP('date', '%W, %M %e, %Y %k:%s %p') as date_added FROM $tbl_name ORDER BY date DESC LIMIT 0, 10"; $result=mysql_query($sql); while ($row=mysql_fetch_array($result)){ // display xml page echo '<?xml version="1.0" encoding="UTF-8"?> <rss version="2.0"> <channel> <title>My Site</title> <description>My Site News Feed</description> <link>http://www.mysite.com/</link> <copyright>Copyright '.date('Y').' My Site</copyright> <language>en-us</language> <lastBuildDate>'.$row['date_added'].'</lastBuildDate> <managingEditor>'.$row['author'].'</managingEditor> <pubDate>'.$row['date_added'].'</pubDate> <webMaster>news@mysite.com</webMaster>'; echo '<item>\n <title>'.$row['title'].'</title> <description><![CDATA[<p> '.$row['text'].' </p>]]></description> <link>http://www.mysite.com/news/</link> <managingEditor>'.$row['author'].'</managingEditor> <pubDate>'.$row['date_added'].'</pubDate> </item>'; } echo '</channel> </rss>'; ?> -
display xml pubDate from mysql database with php
kaiman replied to kaiman's topic in PHP Coding Help
Okay, I will give it a whirl and let you know whether it works. Thanks again, kaiman -
display xml pubDate from mysql database with php
kaiman replied to kaiman's topic in PHP Coding Help
@ JAY6390 Please excuse my ignorance, but I haven't used UNIX_TIMESTAMP in this instance before (I am not a professional developer). Could I use something like this? SELECT *, UNIX_TIMESTAMP(`date`, '%W, %M %e, %Y, %k:%s %p') as `pubdate` FROM $tbl_name ORDER BY date DESC LIMIT 0, 10 And then just return this? $row['date']; Thanks again, kaiman -
display xml pubDate from mysql database with php
kaiman replied to kaiman's topic in PHP Coding Help
@ JAY6390 I did something similar to your recommendation and changed the value of date to something else, but that did not seem to work. @ spfoonnewb I am afraid that if I change my date database field to UNIX_TIMESTAMP versus datetime it will blow up 3 other scripts that are running off of that database table for my news and comments page. Also, another issue that is occuring is that Safari can't seem to handle this file as a feed when it is saved as a php file versus an xml file. Is there a way to save it as a xml file and get php to recognize it? i.e. feed.php > feed.php.xml? Thanks for the continued help, kaiman -
display xml pubDate from mysql database with php
kaiman replied to kaiman's topic in PHP Coding Help
I am still looking for help. I tried using the DATE_FORMAT function in mysql but without any success. Here is what I now have: <?php header('content-type: text/xml'); // connects to server and selects database. include ("dbconnect.inc.php"); // table name $tbl_name="news"; // select info from database $sql="SELECT *, DATE_FORMAT('date', '%W, %M %e, %Y, %k:%s %p') as date FROM $tbl_name ORDER BY date DESC LIMIT 0, 10"; $result=mysql_query($sql); while ($row=mysql_fetch_array($result)){ // display xml page echo '<?xml version="1.0" encoding="UTF-8"?> <rss version="2.0"> <channel> <title>My Site</title> <description>My Site News Feed</description> <copyright>Copyright '.date('Y').' My Site</copyright> <language>en-us</language> <link>http://www.mysite.com/</link>'; echo '<item>\n <title>'.$row['title'].'</title> <description><![CDATA[<p> '.$row['text'].' </p>]]></description> <link>http://www.mysite.com/news/</link> <pubDate>'.$row['date'].'</pubDate> <managingEditor>'.$row['author'].'</managingEditor> </item>'; } echo '</channel> </rss>'; ?>