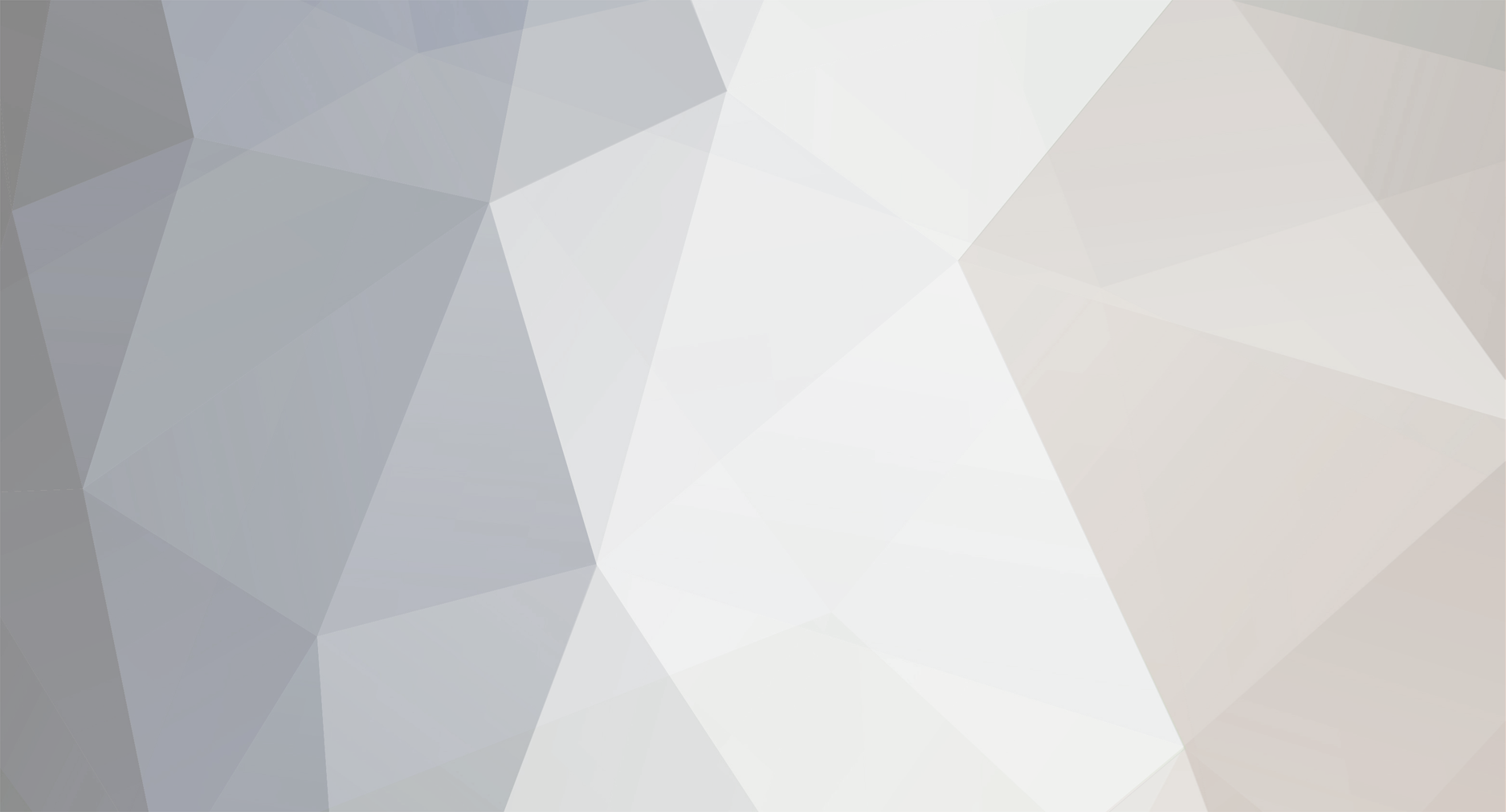
russthebarber
Members-
Posts
62 -
Joined
-
Last visited
Everything posted by russthebarber
-
Hi, I've been trying for several hours now to backup a table to a .sql file using php. The php code seems to be running but nothing at all is happening and no file is created in my "backups" folder. Can anyone help show me where I am going wrong? Thanks. Code below: <?php $dbhost = "localhost"; $dbuser = "myusername"; $dbpass = "mypassword"; $dbname = "mydbname"; $dbtable = "divs"; //Connect to MySQL Server mysql_connect($dbhost, $dbuser, $dbpass); //Select Database mysql_select_db($dbname) or die(mysql_error()); $backupFile = 'backups/backupTest.sql'; $query = "SELECT * INTO OUTFILE '$backupFile' FROM $dbtable"; $result = mysql_query($query); echo $backupFile; ?>
-
Lots of good tips and advice. Thanks very much. I need to have a good think about which way to go.
-
I can only assume my explaination of what I need is extremely bad, so I'll start from scratch. Let's say I have a project with 100 different database tables. Every table has a class with various methods for doing specific tasks in that table. The tables have many fields. At the moment I am having to physically type in the name of the fields at the top of my class...see code above. I am also then physically typing every field name once again when declaring the public variables. This is obviusly time consuming and very bad practice. I am looking for a better way of doing this in a loop, so regardless of which database table I am writing the class for, I just enter the name of the table and my code does the rest. I hope that is clearer.
-
What ManiacDan has suggested is maybe what I'm looking for. This is an example of the kind of thing I want to do...obviously this code is completely wrong and won't work but will hopefully show which direction I want to go in. protected static $db_fields=array('id', 'field1', 'field2', 'field3', 'field4', 'field5'); function writeAttributes() { for($i = 0; $i < count($db_fields); $i++) { public $$db_fields[$i]; } } //looking for the function to return the following: //public $id; //public $field1; //public $field2; //public $field3; //public $field4; //public $field5;
-
I'm looking for a more efficient way of declaring variables within a class. What I normally use is a class which is actually a database object. Every time I make a new table in my database I make a new class and new attributes to match the fields in the db table. Looks something like this: protected static $table_name="myTable"; protected static $db_fields=array('id', 'name', 'address', 'tel_number', 'fax_number'); public $name; public $address; public $tel_number; public $fax_number; //etc. etc. At the moment I am manually adding the attribute, everytime I add a field to the db table. I'm guessing there should be a way to loop through the $db_fields array and add an attribute in the loop? Can anyone help me with the syntax for this. Is there also a way that I don't have to list the fields everytime in $db_fields....can $db_fields not just look at the relevant db table and pull out the field names in some kind of clever method?
-
$_SERVER['PHP_SELF'] - safari showing as firefox
russthebarber replied to russthebarber's topic in PHP Coding Help
Thanks. I have just seen that if you look right to the end of the returned string, you get more specific browser info. Will have to research what all the other stuff means. -
I am just setting up a db to catch user info on my site. After some testing all is working except that when I use Safari, my log wrongly shows that I am using Firefox. I have been scanning the web for a solution but all workarounds I have found seem to be very out of date. Can anyone help with this? here is the code I am using: //logs user info to db $browser = $_SERVER['HTTP_USER_AGENT']; $time = $_SERVER['REQUEST_TIME']; $refer = $_SERVER['HTTP_REFERER']; $page = $_SERVER['PHP_SELF']; $pageQuery = $_SERVER['QUERY_STRING']; $ip = $_SERVER['REMOTE_ADDR'];
-
that works perfectly...thanks again for the good advice!
-
Thanks for the replies. I think Pikachu2000 may have the answer....I'll look into that. Basically this array will store what a user inputs, but I want to return an error and warn the user if he inputs the same thing twice.
-
php string variable in a html form value parameter ?
russthebarber replied to Frank74's topic in PHP Coding Help
You need to echo the variable like this: <input type="text" name="EmitterFirstName" value="<?php echo $EmitterFirstName ?>"/> -
How can I check if an array contains two items that are the same? For example ("apples", "bananas", "oranges") returns false But ("apples", "bananas", "bananas") returns true
-
using php id without going back to server
russthebarber replied to russthebarber's topic in Javascript Help
thorpe, I see what you mean. Thanks. Maybe I could even put the php array information into javascript arrays when the page is created and then do the whole thing in javascript. -
using php id without going back to server
russthebarber replied to russthebarber's topic in Javascript Help
I'm pretty sure the problem is that my explaination is not good enough so I'll try and explain another way: I already have rows information from my database table stored in an array called "$entries" when the page first loads. Therefore I don't need to get anything from the server or reload the page at all to list the information that I need. I go through the array in a php foreach loop and list out my row info onto an html table. <?php foreach($entries as $entry) { ?> <tr> <td><?php echo ucwords($entry->firstName); ?></td> <td><?php echo ucwords($entry->lastName); ?></td> <td><?php echo ucwords($entry->pseudonym); ?></td> <td><?php echo ucwords($entry->company); ?></td> <td><?php echo $entry->tel; ?></td> <td><?php echo $entry->mobile; ?></td> <td><?php echo $entry->email; ?></td> <td><?php echo ucwords($entry->town); ?></td> <td><?php echo ucwords($entry->country); ?></td> <td><img id="<?php echo $entry->id; ?>" src="../images/edit.gif" border=0></td> </tr> <?php } ?> On each row you can see on the last line that an edit button is created. If the user clicks the edit button for id=15 for example, I want to then list out in a separate window (javascript / jquery) the row information for id=15. As this information is already in my php arry "$entries", there must be a way of doing this without reloading the page or using ajax. Anyone know the answer? -
using php id without going back to server
russthebarber replied to russthebarber's topic in Javascript Help
Shame this post has been moved to the ajax section as I am not sure I need ajax or anything very complicated for this. All my rows information is stored already in an array. I was thinking that somehow the foreach loop could assign an id to each edit button and then when clicked the id could be used to show a particular row. -
I have a table that lists rows of data from a mysql database using a foreach loop in php. Each row has an edit button. Normally I would click on the edit button and this would go to a page where the specific row could be edited. Something like this: <td><a href="edit_entry.php?id=<?php echo $entry->id; ?>"><img src="../images/edit.gif" border=0></a></td> This time, however, what I want is that all this is done without going to another page. I can use javascript and/or jQuery to open a window on my page with a form for editing, but I am not sure how it would be known which id edit button was clicked. Does anyone know of a sensible way to do this. maybe something in the php foreach loop?
-
I have this working now using commas instead of AND SET. Thanks anyway.
-
I am trying to use a loop to enter values from several arrays into a mysql table. i am pretty sure that my syntax must be wrong here. i have also tried without using "SET" on every line. can anyone help? for($index = 0; $index < $repeatTimes; $index++) { $sql2 = "UPDATE table SET weekday = '$weekDayPhpArr[$index]' AND SET monthday = '$monthDayPhpArr[$index]' AND SET month = '$monthPhpArr[$index]' AND SET year = '$yearPhpArr[$index]' AND SET veranstaltung = '$veranstaltungPhpArr[$index]' AND SET musik = '$musikPhpArr[$index]' AND SET dj_id = '$djIdPhpArr[$index]' AND SET dj_name = '$djNamePhpArr[$index]' AND SET dj_info = '$djInfoPhpArr[$index]' AND SET handy = '$djHandyPhpArr[$index]' AND SET email = '$djEmailPhpArr[$index]' AND SET geld = '$geldPhpArr[$index]' AND SET gebucht = '$gebuchtPhpArr[$index]' WHERE id = '$idPhpArr[$index]'"; $result2 = mysql_query($sql2); }
-
I am pretty confused as to if php is running on my machine or not. I have installed XAMPP on a mac (snow leopard). I can set up databases, run localhost/php myadmin all OK. Here's the strange thing....I have a flash AS3 file that gets data from a mysql db through a php file. When XAMPP has not been started this AS3 file, as expected, won't work, but when XAMPP is on the file runs OK. This would indicate to me that PHP is set up and running. The strange thing is that when I try to upload something to that db from the flash file, it won't work. I tried a simple html/php form setup to upload something to the db and that flips out my browser completely (it keeps opening new windows until I force quit it). Anyone have any idea of why my AS3 file works and gets the data through the php file but all other indications are that php is not working???
-
Dan, Thanks for getting back to me. I really feel quite strongly about this as forums are such a great way for people to learn and it's so important for people to use them in the most useful way possible. But I really feel we are going around in circles a bit. So, I'm stopping after this. No I haven't! I haven't missed any of your points. You gave me some great advice. Have you missed my point? Here it is again.... This was great answer to my question: $foo = "bar"; ${$foo} = "test"; echo $bar; That's my point. If I wanted to ask about optimising speed for my database I would have done. I asked about variables inside of variables. Thanks again for the tips but I'm sorry that you can't take my point on board that answering my question was what was needed the most and any other help or advice would have been thankfully received afterwards. In fact it was thankfully received.
-
Don't agree, sorry. If someone asks me how to repair a tyre on their bike....I do not suggest that they drive a car instead as it's quicker. I can only say how I would have answered this if it were a topic closer to my area of expertise: Here's how you put variables inside of variables but be warned it's not a good way of working in general. If you want more on that I'd be happy to help.
-
Thanks for all the tips, people. I will bear all this info in mind and can learn much from it. I don't want to seem ungrateful for the tips but it really would be nice if the question asked was the priority to answer. I see this so often in forums that everybody is very keen to tell the newbie he is doing it all wrong that they ignore the actual question. Like I say, I am grateful for the advice but please bear this in mind. Thank you tomfmason for actually answering the question asked.
-
These variables already have values that are stored in a mysql table. What I am looking for is the correct syntax to use to reference these variable names. I don't care if I use an array or not but the problem is still the same...... I am confused with the syntax that will enable me to use the variable or array reference inside the name of another variable. There may be 100 variables to enter into the mysql table but I am trying to avaoid listing all 500 like this: $sql= "UPDATE `bobtracks`.`charts` SET `d01track_artist` = '$d01artist' WHERE `charts`.`chart_no` = '$chart_no'"; $result = mysql_query($sql); $sql= "UPDATE `bobtracks`.`charts` SET `d02track_artist` = '$d02artist' WHERE `charts`.`chart_no` = '$chart_no'"; $result = mysql_query($sql); $sql= "UPDATE `bobtracks`.`charts` SET `d03track_artist` = '$d03artist' WHERE `charts`.`chart_no` = '$chart_no'"; $result = mysql_query($sql); ...etc.
-
If I have a for loop with a variable called $counter, how can I use the value of this inside a variable name. For example: If I want a bunch of variables called $d1name, $d2name, $d3name ...etc. what's the correct syntax to use? The following gives me a string but not a variable name: $myVariable = "$"."d".$counter."name";
-
basic function problem - connect to mysql
russthebarber replied to russthebarber's topic in PHP Coding Help
thanks. That makes sense. -
Hi, I have just started using PHP again after a long break and am very rusty. I am having a few problems with some basic things: I have defined a function to connect to my MySQL database and it doesn't work. My $hostname, $username, $password variables are stored in a separate php file which I am including first and the standard mysql_connect function works ok, but I wanted to put it inside another user defined function called "condb" to make things quicker later. my function looks like this: function condb(){ mysql_connect($hostname, $username, $password) OR DIE ('Unable to connect to database! Please try again later.'); } and I am trying to run it like this: condb(); Unfortunately it is not working. Probably a basic error but any help appreciated. Thanks