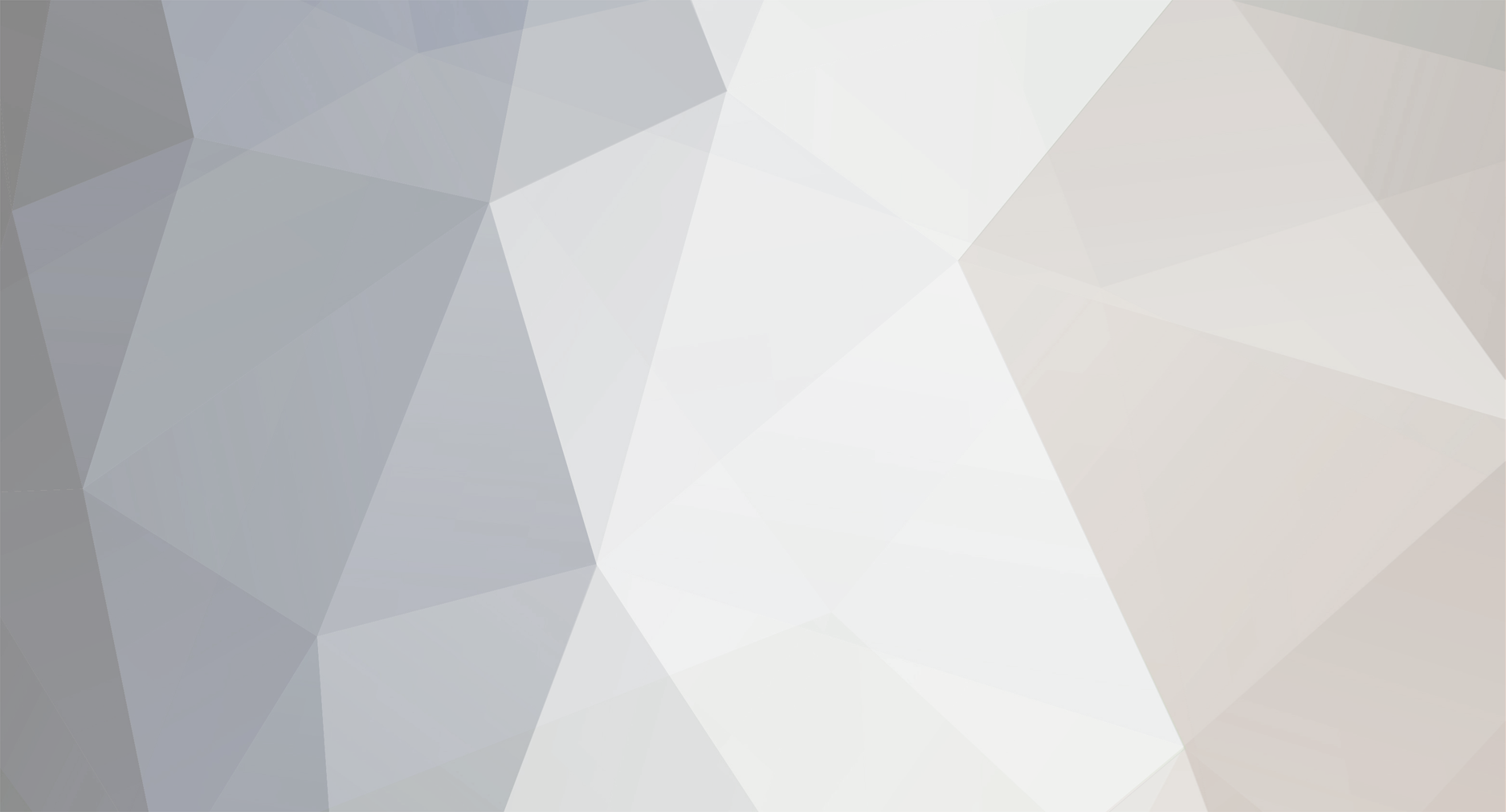
3raser
Members-
Posts
815 -
Joined
-
Last visited
Everything posted by 3raser
-
<?php $words = array( 'fuck','shit', 'dick', 'cunt', 'twat', 'motherfucker', 'asshole', 'damn', 'bitch', 'bitching', 'fucking', 'fuckin', 'shitting', 'shittin', 'whore', 'whores', 'slut', 'sluts', 'bullshit', 'ass', 'bitches'); //string to search $string = $sentence; //amount in the array $x = count($words); for($i = 0; $i < $x; ++$i) { if(strpos($string, $words[$i]) === false) { ++$i; } else { //holds the number of bad words found ++$b; ++$i; } } if(!$b) { //echo "No bad words found, yippe!"; } else { $_SESSION['message'] = "Your sentence contains curse words. Please remove the foul language."; die(header("location: test.php")); } ?> This will count them as individual words.
-
Ah, didn't see that. Thanks
-
Why $_SESSION['id']? I looked in his login.php and it showd $_SESSION['user']
-
Thanks, this is much appreciated. I thought by adding one, that would fix the problem with $i. I forgot about for loops for some reason. :/
-
So you want their session ID to be added into about_me? To use the code below, make sure you add another column called session_id, or anything of your choice. <?php session_start(); if (!isset($_SESSION['user'])) { header("Location: login.php"); } include ('dbc.php'); if ($_POST['Submit'] == 'Post') $nick_name = $_POST['nick_name']; $descript = $_POST['descript']; $aim = $_POST['aim']; $cell = $_POST['cell']; $school = $_POST['school']; $music = $_POST['music']; $aspire = $_POST['aspire']; $city = $_POST['city']; mysql_query("INSERT INTO about_me (nick_name, descript, aim, cell, school, music, aspire, city) VALUES (null, '$nick_name', '$descript', '$aim', '$cell', '$school', '$music', '$aspire', '$city', '". $_SESSION['user'] ."')") or die(mysql_error()); echo("Profile Updated Successfully!"); exit; ?>
-
I'm trying to expand my knowledge on some more str type functions, and I've always wanted to learn implode and explode, but I was just too lazy. Anyways, for some reason, if I type in: salad,pizza,apples - the first value in the array, salad, doesn't show up. o.O <?php $favorite_food = $_POST['favorite_food']; if(!$favorite_food) { ?> <form action="words.php" method="POST"> <input type="text" name="favorite_food"> <input type="submit"> </form> <?php } else { $ex = explode(",", $favorite_food); $amount = count($ex) + 1; while($i < $amount) { echo $ex[$i]."<br/>"; ++$i; } } ?> I know arrays start at 0, so thats why I added 1 to the count.
-
Didn't see post above. I recommend using his way, less code. Tested, and works. <?php //bad words 0 1 2 $words = array('asshhfghh', 'gfhfghfgh', 'fuck'); //string to search $string = 'Blah blah blah ass blah blah fuck blah blah shit!'; //amount in the array $x = count($words) + 1; while($i < $x) { if(strpos($string, $words[$i]) === false) { ++$i; } else { //holds the number of bad words found ++$b; ++$i; } } if(!$b) { echo "No bad words found, yippe!"; } else { echo "Bad words found."; } ?>
-
$to_censor = array("f", "a", "e", "r", "c"); $new_censor = array("****", "****", "****", "****", "****"); $new = str_replace($to_censor, $new_censor, $description); Is it possible to do this without having to add a new **** in $new_censor when I add another censored word in $to_censor
-
I don't think thats the reason. If that were the reason, the text wouldn't be displayed on index.php
-
Just fixed it as you posted.
-
I can't remember how many times this has happened to me, yet I still forget..... -.- Thanks! EDIT: Still doesn't display. <.< How the hell do I manage to make so many bugs... D;
-
EDIT Sorry, fixed it. <?php $words = array('ass','fuck','shit'); $string = 'Blah blah blah ass blah blah fuck blah blah shit!'; $validate = explode(" ", $string); if(in_array($words)) { echo "No cursing please."; } else { //no cussing found } ?>
-
My error below returns (echos) that there is no data under the table sites to display. But, there is. Why is it doing this? class_l.php <?php class displaySites { function grabSites() { $query = mysql_query("SELECT title,description,votes,dates,comments,out,in FROM sites ORDER BY id DESC LIMIT 100"); if(mysql_num_rows($query) > 0) { while($fetch = mysql_fetch_assoc($query)) { echo " <div id='post-1' class='post'> <h2 class='title'><a href='#'>". $fetch['title'] ."</a></h2> <h3 class='date'>Submitted on ". $fetch['date'] ."</h3> <div class='entry'> <p>". nl2br(stripslashes($fetch['description'])) ."</p> </div> <p class='meta'><a href='#'>View</a></p> <div class='hr'> </div> </div> "; } } else { echo " <div id='post-1' class='post'> <h2 class='title'><a href='#'>Oh NOEZ!</a></h2> <h3 class='date'>". date("M-d-Y") ."</h3> <div class='entry'> <p>There are currently no sites to display!</p> </div> <p class='meta'>View</p> <div class='hr'> <hr /> </div> </div> "; } } } class register { function createAccount() { $username = mysql_real_escape_string($_POST['username']); $password = md5(sha1(sha1($_POST['password']))); $name = mysql_real_escape_string($_POST['title']); $description = mysql_real_escape_string($_POST['description']); if(isset($username) && isset($password) && isset($name) && isset($description)) { if(!$username || !$password || !$name || !$description) { echo '<table border="0"><form action="register.php" method="POST"> <tr><td>Username</td><td><input type="text" name="username" maxlength="20"></td></tr> <tr><td>Password</td><td><input type="text" name="password" maxlength="30"></td></tr> <tr><td>Site Name</td><td><input type="text" name="title" maxlength="25"></td></tr> <tr><td>Site Description</td><td><textarea cols="40" rows="18" name="description" maxlength="750"></textarea></td></tr> <tr><td>Finish!</td><td><input type="submit"></td></tr> </form></table>'; } else { $query = mysql_query("SELECT * FROM sites WHERE username = '$username' LIMIT 1"); if(mysql_num_rows($query) > 0) { echo "Sorry, an account with this username already exists."; } else { $date = date("M-d-Y"); $ip = $_SERVER['REMOTE_ADDR']; //create their account mysql_query("INSERT INTO sites VALUES (null, '$username', '$password', '$name', '$description', 0, 0, 0, '$date', '$ip', 0)"); echo "You have successfully registered your site on NovaTops! <a href='login.php'>Login</a>"; } } } else { echo "One or more of the variables are not set."; } } } class login { function verifyLogin() { $username = mysql_real_escape_string($_POST['username']); $password = md5(sha1(sha1($_POST['password']))); if(isset($username) && isset($password)) { if(!$username || !$password) { echo "<table><form action='login.php' method='POST'> <tr><td>Username</td><td><input type='text' name='username' maxlength='20'></td></tr> <tr><td>Password</td><td><input type='password' name='password' maxlength='30'></td></tr> <tr><td>Finished?</td><td><input type='submit'></td></tr> </form></table>"; } else { $query = mysql_query("SELECT * FROM sites WHERE username = '$username' AND password = '$password'LIMIT 1"); if(mysql_num_rows($query) > 0) { echo "Congratulations! You have successfully logged in! <a href='index.php'>Home</a>"; $_SESSION['user'] = $username; } else { echo "It seems you've typed in the wrong username and/or password. <a href='login.php'>Try Again</a>"; } } } else { echo "One or more of the variables aren't set."; } } } ?> index.php <?php include_once('includes/config.php'); include_once('class_l.php'); ?> <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Strict//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-strict.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <meta http-equiv="content-type" content="text/html; charset=utf-8" /> <title><?php echo $title; ?></title> <meta name="keywords" content="" /> <meta name="description" content="" /> <link href="styles.css" rel="stylesheet" type="text/css" /> </head> <body> <div id="wrapper"> <div class="top_text"><h1><a href="#"><?php echo $site_name; ?></a></h1> <h2><a href="#"><?php echo $slogan; ?></a></h2></div> <div id="content"> <div id="blog"> <div id="post-1" class="post"> <h2 class="title"><a href="#">Welcome to NovaTops!</a></h2> <h3 class="date">Created on April 06, 2011</h3> <div class="entry"> <p>Hello there! If your viewing this message right now, your on the awesome site called NovaTops! NovaTops allows you to register your site in our database, and then the site's script automatically starts to rank your site. The higher your rank, the more likely your site's going to get a ton of views!</p> </div> <div class="hr"> <hr /> </div> </div> <!-- end #post-1 --> <?php $displaySites = new displaySites(); $displaySites->grabSites(); ?> </div> <!-- end #blog --> <?php include_once('includes/navigation.php'); ?> <!-- end #sidebar --> <div style="clear: both; height: 1px;"></div> </div> <!-- end #content --> <div id="footer"> <p>Copyright © 2011. Design by <a href="http://www.flashtemplatesdesign.com/" title="Free Flash Templates">Free Flash Templates</a></p> <p><a href="#">Privacy Policy</a> | <a href="#">Terms of Use</a> | <a href="http://validator.w3.org/check/referer" title="This page validates as XHTML 1.0 Transitional"><abbr title="eXtensible HyperText Markup Language">XHTML</abbr></a> | <a href="http://jigsaw.w3.org/css-validator/check/referer" title="This page validates as CSS"><abbr title="Cascading Style Sheets">CSS</abbr></a></p> </div> </div> <!-- end #wrapper --> </body> </html>
-
"simple" calculations using database values
3raser replied to xwishmasterx's topic in PHP Coding Help
Something like this? $total = table_col1 + table_col2 + table_col3; echo $total; EDIT: if(table_col1 + table_col2 + table_col3 == 20) { echo "adds up to 20 exactly"; } else { echo "does not equal"; } -
Also: echo "<table class=roung bgcolor='#000000'><tr><TD><img src='uploaded/". $li2[picname] ."' width='760' height='540'></td></tr></table>"; Use that instead of echo "<table class=roung bgcolor='#000000'><tr><TD><img src=uploaded/$li2[picname] width='760' height='540'></td></tr></table>";
-
A bit confused on what your asking, hope this helps. Mind putting it in [*PHP] tags to make it easier to read? if ($page=="P8"&$view>0) Shouldn't that be this: if ($page=="P8" && $view>0) Also, to display the image, wouldn't you need to call it via header? header("Content-type:image/jpeg"); <?php if ($_FILES) { $username = $_SESSION['user']; $date =time(); $name = $_FILES["myfile"]["name"]; $type = $_FILES["myfile"]["type"]; $size = $_FILES["myfile"]["size"]; $temp = $_FILES["myfile"]["tmp_name"]; $error = $_FILES["myfile"]["error"]; $hs=$_POST['High Score']; $g=$_POST['Glitch']; $f=$_POST['Funny']; $o=$_POST['Other']; $tags = new Array(); foreach($_POST['tag'] as $tag){ if($tag){ tags[] = $tag; } } $tag = implode(',',$tags); if ($error > 0 ) die("Error uploading file! Code $error."); else { if ($type =="image/jpeg") { include 'connect.inc'; move_uploaded_file($temp,"uploaded/".$name); mysql_query("INSERT INTO images VALUES('','$name','','$username','$date','0','0','0','0','0','0','0','0')"); $uploadreport='1'; header("Content-type:image/jpeg"); } else { } } } else { } ?>
-
Plus you're not echoing the result. Try: <?php class findName { private $name; public function setName($sname='') { if(!$sname) { $this->name = "There is no name to return."; } else { $this->name=$sname; } } public function getName() { return $this->name; } } $n = new findName(); $n->setName(); echo $n->getName() . "<br>\n"; ?> Ken Thanks to both, much appreciated.
-
New code, still no output: <?php class findName { private $name; public function setName($sname) { if(!$sname) { $this->name = "There is no name to return."; } else { $this->name=$sname; } } public function getName() { return $this->name; } } $n = new findName(); $n->setName(); $n->getName(); ?>
-
I just decided to start up on OOP, and it seems quite fun (yes, I find programming fun). But, I'm having trouble on this test OOP page. This is suppose to return the private variable $name, and if it's empty, it's suppose to echo the no name to return message. Code: <?php class findName { private $name; public function setName($sname) { if(!$sname) { $this->name = "There is no name to return."; } else { $this->name=$sname; } } public function getName() { return $this->name; } } $n = new findName(); $n->getName(); ?> If there is an easier way to do this, please let me now!
-
Hm, it seems it's not because of the redirection. I removed the header function, and made a success message. It showed up, but it seems the reason is because of the $_SESSION. How come the session isn't creating?
-
Thanks, I'll give it a try soon. But a little off-topic here, why does this say this is a loop? It checks once then creates a session, refreshes their page, and it should check within the function that they are an admin. My error, for google chrome: This webpage has a redirect loop The webpage at http://localhost/admin.php has resulted in too many redirects. Clearing your cookies for this site or allowing third-party cookies may fix the problem. If not, it is possibly a server configuration issue and not a problem with your computer. admin.php: <?php include_once('includes/config.php'); include_once('functions.php'); if(!$_SESSION['admin']) { $content = AccountRelated($_COOKIE['user'], null, 6); } else { $content = "Welcome to the Administrator Control Panel."; } ?> <html> <head> <link rel="stylesheet" type="text/css" href="style/style.css" /> <title><?php echo $title; ?></title> </head> <body> <div class="logo"><a href="index.php"><img src="style/images/logo.png" border="0"></a></div> <center> <div class="background"> <div class="container"> <?php echo $content; ?> </div> </div> </center> </body> </html> function (last bit of code) <?php function AccountRelated($username, $password, $query_type) { if($query_type == 1) { $set_query = mysql_query("SELECT COUNT(d.username), u.date, u.username FROM uploads d, users u WHERE d.username = '$username' AND u.username = '$username' LIMIT 1") or die(mysql_error()); //user must not exist if(mysql_num_rows($set_query) == 0) { $content_return = 'Sorry, no information was found'; } else { $grab = mysql_fetch_assoc($set_query); //login information if($grab['COUNT(d.username)'] > 0) { $welcome_return = "You have uploaded ". $grab['COUNT(d.username)'] ." files. You've registered on ". $grab['u.date'] ."!"; } else { $welcome_return = "You have uploaded 0 files. You've registered on ".$grab['date'] . "!"; } } } elseif($query_type == 2) { $set_query = mysql_query("SELECT title,views,downloads,description,username,date FROM uploads LIMIT 20"); if(mysql_num_rows($set_query) == 0) { $content_return = "Sorry, there are currently no files uploaded to view."; } else { //display all files while($row = mysql_fetch_assoc($set_query) == 0) { echo $row['title']."<br/>"; } } } elseif($query_type == 3) { $username = mysql_real_escape_string($_POST['username']); $password = sha1(sha1(md5($_POST['password']))); if(!$username || !$password) { $return_content = "All fields are required! <table><form action='register.php' method='POST'> <tr><td>Username</td><td><input type='text' name='username' maxlength='20'></td></tr> <tr><td>Password</td><td><input type='text' name='password' maxlength='30'</td></tr> <tr><td><input type='submit' value='Register'></td></tr> </form></table>"; } else { $set_query = mysql_query("SELECT username FROM users WHERE username = '$username' LIMIT 1"); if(mysql_num_rows($set_query) == 0) { $return_content = "You have successfully registered the account ". $username ." with the password ". $_POST['password'] ."! <a href='login.php'>Login now</a>!"; mysql_query("INSERT INTO users VALUES (null, '$username', '$password', 0, 0, '". date("M-d-Y") ."', '". $_SERVER['REMOTE_ADDR'] ."')") or die(mysql_error()); } else { $return_content = "An account with this username already exists."; } } return $return_content; } elseif($query_type == 4) { $username = mysql_real_escape_string($_POST['username']); $password = sha1(sha1(md5($_POST['password']))); if(!$username || !$password) { $return_content = "<table><form action='login.php' method='POST'> <tr><td>Username</td><td><input type='text' name='username' maxlength='20'></td></tr> <tr><td>Password</td><td><input type='text' name='password' maxlength='30'</td></tr> <tr><td><input type='submit' value='Login'></td></tr> </form></table>"; } else { $set_query = mysql_query("SELECT * FROM users WHERE username = '$username' AND password = '$password' LIMIT 1"); if(mysql_num_rows($set_query) == 0) { $return_content = "Hmm, it seems you've submitted the wrong username and/or password! <a href='login.php'>Try Again</a>"; } else { $return_content = "You have successfully logged in! <a href='index.php'>Home</a>"; setcookie('user', $username, time()+31556926); } } return $return_content; } elseif($query_type == 5) { //lets verify if they are banned or not $set_query = mysql_query("SELECT banned FROM users WHERE username = '$username' AND banned = 1 LIMIT 1"); if(mysql_num_rows($set_query) > 0) { $return_content = "Sorry, you account has been banned. Until you are unbanned, your account no longer has the option to upload files."; } else { //uploading files if(!$_FILES['file'] || !$_POST['title'] || !$_POST['description']) { $return_content = "<table><form action='upload.php' method='POST' enctype='multipart/form-data'> <tr><td>Title</td><td><input type='text' name='title' maxlength='25'></td></tr> <tr><td>Password (Optional)</td><td><input type='password' maxlength='15'></td></tr> <tr><td>Description</td><td><textarea name='description' rows='15' cols='35' maxlength='250'></textarea></td></tr> <tr><td>Choose File</td><td><input type='file' name='file'></td></tr> <tr><td><input type='submit' value='Upload'></td></tr> </form></table>"; } else { if($_FILES['file']['error'] > 0) { $content_return = "OOPS! Something went wrong! Make sure you have selected a file to upload, or try again later."; } elseif(strlen($_FILES['file']['name']) > 25) { $content_return = "The file name cannot be larger than 25 characters! Please go back and manually change the file name, and try uploading again."; } else { //lets get the required information to submit to the database $title = mysql_real_escape_string($_POST['title']); $password = mysql_real_escape_string($_POST['password']); $description = mysql_real_escape_string($_POST['description']); $date = date("M-d-Y"); $ip = $_SERVER['REMOTE_ADDR']; //lets get the file extension $extension = end(explode('.', $_FILES['file']['name'])); //insert the data into the database mysql_query("INSERT INTO uploads VALUES (null, '". $_COOKIE['user'] ."', '$password', '$title', '$description', '$extension', 0, 0, 0, '$date', '$ip')"); //file uploaded successfully, lets move it to the files directory move_uploaded_file($_FILES['file']['tmp_name'], "files/". mysql_insert_id() .".". $extension); $return_content = "File uploaded successfully! <a href='myfiles.php'>My Files</a>"; } } } return $return_content; } elseif($query_type == 6) { //lets verify they are an admin $set_query = mysql_query("SELECT status FROM users WHERE username = '$username' AND status = 2 LIMIT 1"); if(mysql_num_rows($set_query) > 0) { $_SESSION['is_admin'] = $username; header("location:admin.php"); } else { $content = "You are not an admin! This page is available to admins only."; } return $content; } else { //nothing to process } } ?>
-
I'm working on a file uploading project, and I want to let admins restrict the files uploaded to certain files only. Should this be saved in the database, or should I make a file that saves an array? If I do it via the file method, how would I update an array, say allowed.php? Example: How could I change <?php $allowed = array('zip', 'png'); ?> To <?php $allowed = array('gif', 'png'); ?>
-
I do: file:///C:/wamp/www/functions.php And tried: http://localhost/ EDIT: Somehow a few files were missing, but I fixed this.
-
(I looked at the appropriate forums to put this in, but it seems I couldn't find a forum section specifically for this problem) Yesterday PHP on WAMP was working perfectly. So I shutdown the computer, go to sleep, and wake up and turn WAMP on. But for some odd reason, when none of my PHP files are loading properly. It just shows all the code as if it were a .txt file. Any reasons for this?