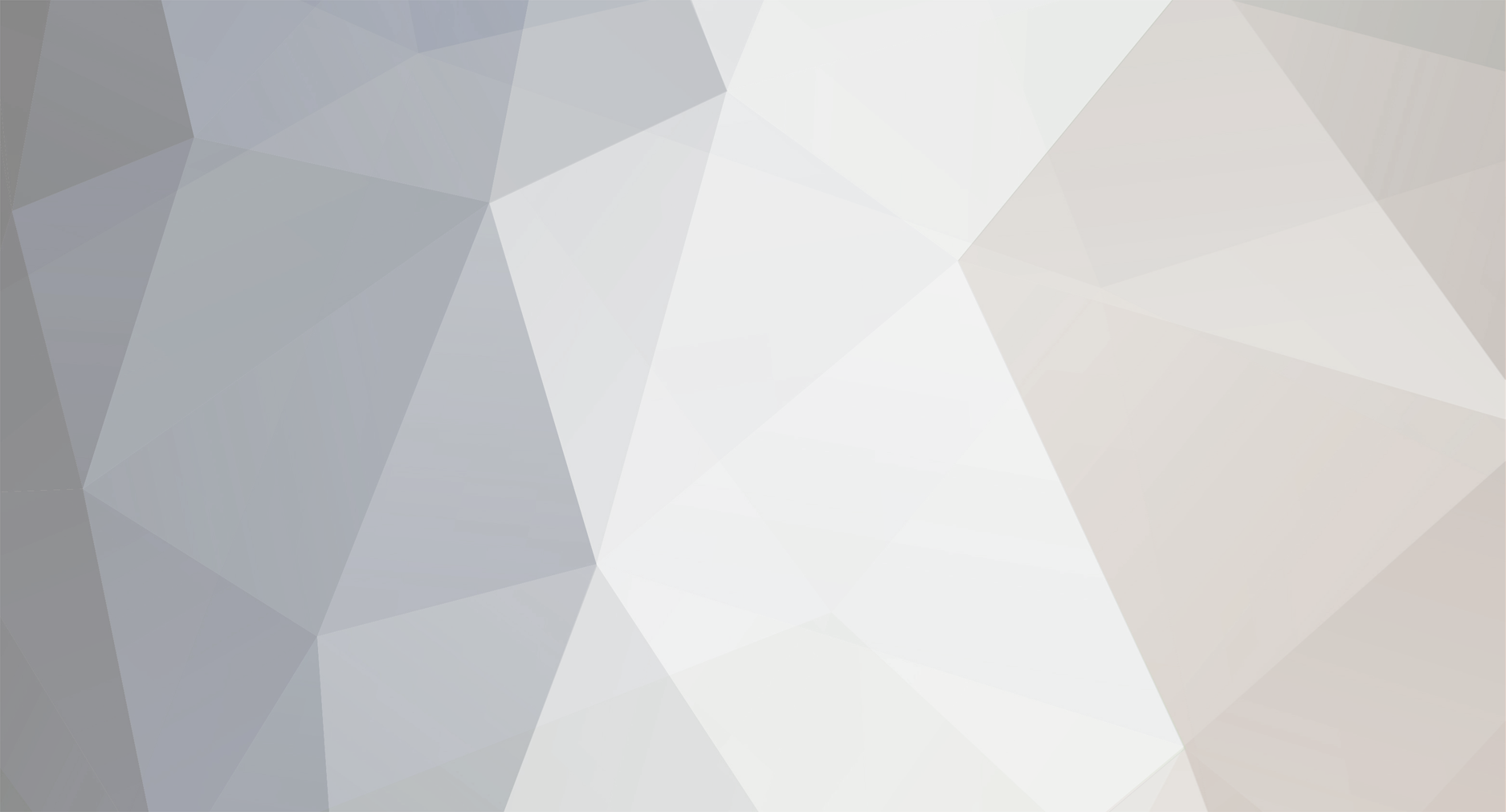
spenceddd
Members-
Posts
107 -
Joined
-
Last visited
Never
Everything posted by spenceddd
-
Could somebody please explain this drop down driven page to me?
spenceddd replied to spenceddd's topic in PHP Coding Help
Yeah sure here they are: buildfileList5: <?php function buildFileList5($theFolder) { // Execute code if the folder can be opened, or fail silently if ($contents = @ scandir($theFolder)) { // initialize an array for matching files $found = array(); // Create an array of file types $fileTypes = array('jpg','jpeg','gif','png'); // Traverse the folder, and add filename to $found array if type matches $found = array(); foreach ($contents as $item) { $fileInfo = pathinfo($item); if (array_key_exists('extension', $fileInfo) && in_array($fileInfo['extension'],$fileTypes)) { $found[] = $item; } } // Check the $found array is not empty if ($found) { // Sort in natural, case-insensitive order, and populate menu natcasesort($found); foreach ($found as $filename) { echo "<option value='$filename'>$filename</option>\n"; } } } } ?> populateDropDownList: <?php /* 1. Query the database and get the ID and name FROM the table PortfolioItems */ mysql_select_db($database_db, $dbc); $query_PortfolioItems = "SELECT id, name FROM PortfolioItems"; $PortfolioItems = mysql_query($query_PortfolioItems, $dbc) or die(mysql_error()); $row_PortfolioItems = mysql_fetch_assoc($PortfolioItems); $totalRows_PortfolioItems = mysql_num_rows($PortfolioItems); /* 2. If the form was submitted then get the ID from the name text box * This is used for the form location, from and leadership **/ $colname_chosen_pItem = "-1"; if (isset($_POST['name'])) { $colname_chosen_pItem = (get_magic_quotes_gpc()) ? $_POST['name'] : addslashes($_POST['name']); /*if(get_magic_quotes_gpc()){ $colname_chosen_pItem = $_POST['name'] }else{ $colname_chosen_pItem =addslashes($_POST['name'] }*/ } mysql_select_db($database_db, $dbc); $query_chosen_pItem = sprintf("SELECT * FROM PortfolioItems WHERE id = %s", $colname_chosen_pItem); $chosen_pItem = mysql_query($query_chosen_pItem, $dbc) or die(mysql_error()); $row_chosen_pItem = mysql_fetch_assoc($chosen_pItem); $totalRows_chosen_pItem = mysql_num_rows($chosen_pItem); //not sure if the term id is meant to be used for the sprintf function ?> Thanks Spenec -
Could somebody please explain this drop down driven page to me?
spenceddd replied to spenceddd's topic in PHP Coding Help
I have now successfuly integrated the above code into my form, though in taking my form forward I am stuck again. It's a bit frustrating as the answers are right in front of me with the existing code. I just need to apply the same functionality to some new UI items. Specifically the image chooser drop down. Now I just need the image chooser drop down box to populate a table cell with an image when an item from the image chooser drop down list is chosen. Since the form already effectively does this with the portfolio item drop down menu at the top of the page I know it's just a case of understanding the code and reapplying it. The new image chooser drop down box in question needs to: # read it's list contents from a folder (which it already does) # when a list item is selected and the form is submitted it needs to send the item (plus a path string) to the database - I cannot do this in conjunction with the feature above Although the table cell is populated with the chosen image from the drop down I know that this is just a case of returning whatever is sent from the portfolio top drop down list at the top of the page using the 'post' function. Below is the script for the entire form to help explain: <?php include('connectToDB.php'); include('populateDropDownList.php'); include('buildFileList5.php'); ?> <html> <head> <script type="text/javascript"> function chkFrm(el){ if(el.selectedIndex == 0){ alert("Please choose an option"); return false } else{ el.form.submit(); } } </script> </head> <body> <h1>Select Existing Item: </h1> <form action"" method="POST" >Existing Item: <select name="name" onChange="chkFrm(this)"> <option value="Choose" <?php if (!(strcmp("Choose", $_POST['name']))) {echo "SELECTED";} ?>>Choose Portfolio Items</option> <?php do { ?> <option value="<?php echo $row_PortfolioItems['id']?>"<?php if (!(strcmp($row_PortfolioItems['id'], $_POST['name']))) {echo "SELECTED";} ?>><?php echo $row_PortfolioItems['name']?></option> <?php } while ($row_PortfolioItems = mysql_fetch_assoc($PortfolioItems)); $rows = mysql_num_rows($PortfolioItems); if($rows > 0) { mysql_data_seek($PortfolioItems, 0); $row_PortfolioItems = mysql_fetch_assoc($PortfolioItems); } ?> </select> </form> <form action="addToPortfolioItems.php" method="post"> <p> Name: <input name="name" type="text" value="<?php echo $row_chosen_pItem['name']; ?>" size="25"> </p> <p> Short Description: <textarea name="shortDesc" cols="40" rows="4"><?php echo $row_chosen_pItem['shortDesc']; ?></textarea> </p> <p> Long Description: <textarea name="longDesc" cols="40" rows="10"><?php echo $row_chosen_pItem['longDesc']; ?></textarea> </p> <p>Image01: <select name="img01"> <option value= "Choose" <?php if (!(strcmp("Choose", $_POST['img01']))) {echo "SELECTED";} ?>> <option value= <?php echo $row_chosen_pItem['img01']; ?>> Choose image</option> <? buildFileList5('uploaded'); ?> </select> </p> <table width="24" border="1"> <tr> <td><?php echo $row_chosen_pItem['longDesc']; ?> </td> <td> </td> </tr> <tr> <td><img src="<?php echo $row_chosen_pItem['img02']; ?>" width="250" height="250"></td> <td><?php echo $row_chosen_pItem['img02']; ?> </td> </tr> <tr> <td> </td> <td> </td> </tr> <tr> <td> </td> <td> </td> </tr> <tr> <td> </td> <td> </td> </tr> </table> <p> </p> <p>Related Item01 <select name="relatedItem01" > <option value="" <?php if (!(strcmp("Choose", $_POST['relateItem01']))) {echo "SELECTED";} ?>>Choose Item</option> <?php do { ?> <option value="<?php echo $row_PortfolioItems['id']?>"<?php if (!(strcmp($row_PortfolioItems['id'], $_POST['relateItem01']))) {echo "name";} ?>><?php echo $row_PortfolioItems['name']?></option> <?php } while ($row_PortfolioItems = mysql_fetch_assoc($PortfolioItems)); $rows = mysql_num_rows($PortfolioItems); if($rows > 0) { mysql_data_seek($PortfolioItems, 0); $row_PortfolioItems = mysql_fetch_assoc($PortfolioItems); } ?> </select> <p>Related Item02 <select name="relateItem02" > <option value="Choose" <?php if (!(strcmp("Choose", $_POST['relateItem01']))) {echo "SELECTED";} ?>>Choose Item</option> <?php do { ?> <option value="<?php echo $row_PortfolioItems['id']?>"<?php if (!(strcmp($row_PortfolioItems['id'], $_POST['relateItem02']))) {echo "name";} ?>><?php echo $row_PortfolioItems['name']?></option> <?php } while ($row_PortfolioItems = mysql_fetch_assoc($PortfolioItems)); $rows = mysql_num_rows($PortfolioItems); if($rows > 0) { mysql_data_seek($PortfolioItems, 0); $row_PortfolioItems = mysql_fetch_assoc($PortfolioItems); } ?> </select> <p>Related Item03 <select name="relateItem03" > <option value="Choose" <?php if (!(strcmp("Choose", $_POST['relateItem03']))) {echo "SELECTED";} ?>>Choose Item</option> <?php do { ?> <option value="<?php echo $row_PortfolioItems['id']?>"<?php if (!(strcmp($row_PortfolioItems['id'], $_POST['relateItem01']))) {echo "name";} ?>><?php echo $row_PortfolioItems['name']?></option> <?php } while ($row_PortfolioItems = mysql_fetch_assoc($PortfolioItems)); $rows = mysql_num_rows($PortfolioItems); if($rows > 0) { mysql_data_seek($PortfolioItems, 0); $row_PortfolioItems = mysql_fetch_assoc($PortfolioItems); } ?> </select> <p>Keywords: <input name="keywords" type="text" value="<?php echo $row_chosen_pItem['keywords']; ?>" size="50"> <p>Skills: <input name="skills" type="text" value="<?php echo $row_chosen_pItem['skills']; ?>" size="50"> <p><input type="submit"/> </form> <h1>Upload an image file</h1> <form enctype="multipart/form-data" action="uploaderAndResize.php" method="POST"><input type="file" name="myfile"> <p> <input name="Submit" type='submit' value="upload" > <?echo $heldVariable?> </form> <h1>Upload a movie file</h1> <form enctype="multipart/form-data" action="uploaderAndResize.php" method="POST"><input type="file" name="myfile"> <p> <input name="Submit" type='submit' value="upload" > <?echo $heldVariable?> </form> <h1>Upload a download file</h1> <form enctype="multipart/form-data" action="uploaderAndResize.php" method="POST"><input type="file" name="myfile"> <p> <input name="Submit" type='submit' value="upload" > <?echo $heldVariable?> </form> </body> </html> <?php ?> This link shows the form in action in its current state: http://www.spencercarpenter.co.uk/portfolioAppFiles/simpleForm.php I hope I have explained this properly and will very much appreciate some help. Spencer -
Could somebody please explain this drop down driven page to me?
spenceddd replied to spenceddd's topic in PHP Coding Help
Great! Thanks again for your help. I am working through it now hopefully I will have some success! -
Could somebody please explain this drop down driven page to me?
spenceddd replied to spenceddd's topic in PHP Coding Help
I am still quite stuck on understanding this code. Could anyopne please tell me how the 'get_magic_quotes_gpc()' function works in the context of this code? Also I don't understand '?', addslashes() or %s. Again thanks for any guidance on this... Spencer -
Could somebody please explain this drop down driven page to me?
spenceddd replied to spenceddd's topic in PHP Coding Help
Ah yes thanks for pointing it out. -
Could somebody please explain this drop down driven page to me?
spenceddd replied to spenceddd's topic in PHP Coding Help
Thanks very much for that I will try and work it out. -
Hi, I am a total newbie at php. I have manged to setup my database and a form which writes and reads info from it, though probably a bit sketchily. I have been struggling like hell to get all the fields (well any actually) of my html form to update when a user picks a item from the dropdown list. What I am trynig to achieve is that when a user picks an item from the DD the form finds the info from that row and then fills in all the fields pertaining to that the chosen DD item. It looks like I have found some code lots of which is baffling me. If anyone could leave some comments on it exlpaining what the code is doing I would be very grateful. <?php //error_reporting(E_ALL); require_once('db.php'); ?> <?php mysql_select_db($database_db, $db); $query_seminars = "SELECT id, attend FROM seminar"; $seminars = mysql_query($query_seminars, $db) or die(mysql_error()); $row_seminars = mysql_fetch_assoc($seminars); $totalRows_seminars = mysql_num_rows($seminars); $colname_chosen_seminar = "-1"; if (isset($_POST['attend'])) { $colname_chosen_seminar = (get_magic_quotes_gpc()) ? $_POST['attend'] : addslashes($_POST['attend']); } mysql_select_db($database_db, $db); $query_chosen_seminar = sprintf("SELECT * FROM seminar WHERE id = %s", $colname_chosen_seminar); $chosen_seminar = mysql_query($query_chosen_seminar, $db) or die(mysql_error()); $row_chosen_seminar = mysql_fetch_assoc($chosen_seminar); $totalRows_chosen_seminar = mysql_num_rows($chosen_seminar); ?> <!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=iso-8859-1"> <title>Untitled Document</title> <script type="text/javascript"> function chkFrm(el){ if(el.selectedIndex == 0){ alert("Please choose an option"); return false } else{ el.form.submit(); } } </script> </head> <body> <form name="form1" method="post" action=""> <p> <select name="attend" onchange="chkFrm(this)"> <option value="Choose" <?php if (!(strcmp("Choose", $_POST['attend']))) {echo "SELECTED";} ?>>Choose Seminar</option> <?php do { ?> <option value="<?php echo $row_seminars['id']?>"<?php if (!(strcmp($row_seminars['id'], $_POST['attend']))) {echo "SELECTED";} ?>><?php echo $row_seminars['attend']?></option> <?php } while ($row_seminars = mysql_fetch_assoc($seminars)); $rows = mysql_num_rows($seminars); if($rows > 0) { mysql_data_seek($seminars, 0); $row_seminars = mysql_fetch_assoc($seminars); } ?> </select> </p> <p>Location <input name="textfield" type="text" value="<?php echo $row_chosen_seminar['location']; ?>"> <br> From <input name="textfield" type="text" value="<?php echo $row_chosen_seminar['from']; ?>"> <br> Leader <input name="textfield" type="text" value="<?php echo $row_chosen_seminar['leadership']; ?>"> </p> </form> </body> </html> <?php mysql_free_result($seminars); mysql_free_result($chosen_seminar); ?> Thanks for any help on this...