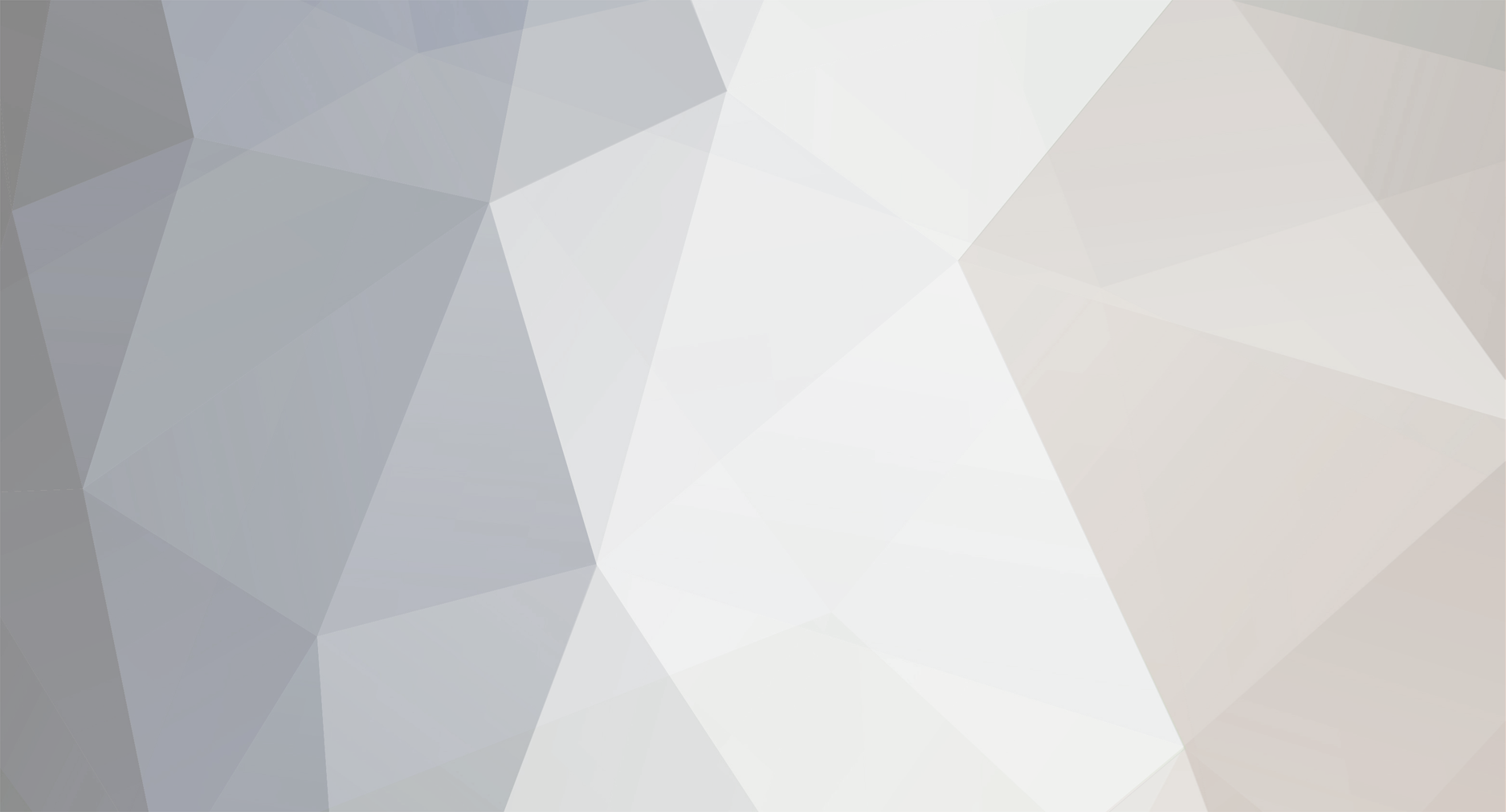
Johns3n
Members-
Posts
72 -
Joined
-
Last visited
Everything posted by Johns3n
-
Of course! However it is quite long! But it should be well commented so not so difficult to work out ^^ <?php // Selects the Blog Posts table $tbl_name="". $db_prefix ."posts"; // Defines how many adjacent pages should be shown on each side on the pagination module $adjacents = 3; // Gets the total number of rows in data table. $query = "SELECT COUNT(*) as num FROM $tbl_name"; $total_pages = mysql_fetch_array(mysql_query($query)); $total_pages = $total_pages[num]; // The file which should include the Pagination Module (Always the name of the file this code appears in ex. Index.php) $targetpage = "index.php"; // Defines the number of Blog Posts to show on each page $limit = 4; $page = $_GET['page']; if($page) // Defines the first item to display on this page $start = ($page - 1) * $limit; else // If no page variable is given, set start to 0 $start = 0; // Get Blog Post data from MySQL database $sql = "SELECT * FROM $tbl_name ORDER BY id DESC LIMIT $start, $limit"; $result = mysql_query($sql); // Setup variables for Pagination Module. (DO NOT EDIT THESE!) if ($page == 0) $page = 1; // If no page var is given, default to 1. $prev = $page - 1; // Previous page is page - 1 $next = $page + 1; // Next page is page + 1 $lastpage = ceil($total_pages/$limit); // Lastpage is = total pages / items per page, rounded up. $lpm1 = $lastpage - 1; // Last page minus 1 // Apply the variables and output the pagination Module. // (We're actually saving the code to a variable in case we want to draw it more than once.) $pagination = ""; if($lastpage > 1) { $pagination .= "<div class=\"pagination\">"; // Previous Button if ($page > 1) $pagination.= "<a href=\"$targetpage?page=$prev\">« Previous</a>"; else $pagination.= "<span class=\"disabled\">« Previous</span>"; // Pages if ($lastpage < 7 + ($adjacents * 2)) { for ($counter = 1; $counter <= $lastpage; $counter++) { if ($counter == $page) $pagination.= "<span class=\"current\">$counter</span>"; else $pagination.= "<a href=\"$targetpage?page=$counter\">$counter</a>"; } } // Enough pages are detected to start hiding some in the Pagination Module elseif($lastpage > 5 + ($adjacents * 2)) { // Close to beginning in Pagination Module: hide only later pages if($page < 1 + ($adjacents * 2)) { for ($counter = 1; $counter < 4 + ($adjacents * 2); $counter++) { if ($counter == $page) $pagination.= "<span class=\"current\">$counter</span>"; else $pagination.= "<a href=\"$targetpage?page=$counter\">$counter</a>"; } $pagination.= "..."; $pagination.= "<a href=\"$targetpage?page=$lpm1\">$lpm1</a>"; $pagination.= "<a href=\"$targetpage?page=$lastpage\">$lastpage</a>"; } // In the middle of the Pagination Module: Hide some pages front and some back elseif($lastpage - ($adjacents * 2) > $page && $page > ($adjacents * 2)) { $pagination.= "<a href=\"$targetpage?page=1\">1</a>"; $pagination.= "<a href=\"$targetpage?page=2\">2</a>"; $pagination.= "..."; for ($counter = $page - $adjacents; $counter <= $page + $adjacents; $counter++) { if ($counter == $page) $pagination.= "<span class=\"current\">$counter</span>"; else $pagination.= "<a href=\"$targetpage?page=$counter\">$counter</a>"; } $pagination.= "..."; $pagination.= "<a href=\"$targetpage?page=$lpm1\">$lpm1</a>"; $pagination.= "<a href=\"$targetpage?page=$lastpage\">$lastpage</a>"; } // Close to end of the Pagination Module: Hide only earliere pages else { $pagination.= "<a href=\"$targetpage?page=1\">1</a>"; $pagination.= "<a href=\"$targetpage?page=2\">2</a>"; $pagination.= "..."; for ($counter = $lastpage - (2 + ($adjacents * 2)); $counter <= $lastpage; $counter++) { if ($counter == $page) $pagination.= "<span class=\"current\">$counter</span>"; else $pagination.= "<a href=\"$targetpage?page=$counter\">$counter</a>"; } } } // Next Button if ($page < $counter - 1) $pagination.= "<a href=\"$targetpage?page=$next\">Next »</a>"; else $pagination.= "<span class=\"disabled\">Next »</span>"; $pagination.= "</div>\n"; } ?> <?php $num_query = "SELECT ". $db_prefix ."posts.id,". $db_prefix ."posts.title,". $db_prefix ."posts.content,". $db_prefix ."posts.date,". $db_prefix ."posts.author, COUNT(". $db_prefix ."comments.id) AS numComments FROM ". $db_prefix ."comments INNER JOIN ". $db_prefix ."posts ON ". $db_prefix ."comments.post_id = ". $db_prefix ."posts.id GROUP BY ". $db_prefix ."posts.id, ". $db_prefix ."posts.title,". $db_prefix ."posts.content,". $db_prefix ."posts.date,". $db_prefix ."posts.author"; $num_result = mysql_query($num_query); // This is where the HTML output of our Blog Posts is generated while($row = mysql_fetch_array($result)) { echo "<div class='post_entry'>"; echo "<div class='post_title'><h2><a href='singlepost.php?itemid=" . $row['id'] . "' title='" . $row['title'] . "'>" . $row['title'] . "</a></h2></div>"; echo "<div class='post_author'><p>Written by: " . $row['author'] . "</p></div>"; echo "<div class='post_date'><p>Posted: " . $row['date'] . "</p></div>"; echo "<div class='post_entry_content'><p>" . $row['content'] . "</p></div>"; echo "<div class='post_comments'><a href='singlepost.php?itemid=" . $row['id'] . "#comments' title='Click here to view comments'>"; while($num_row = mysql_fetch_assoc($num_result)) { echo "". $num_row['numComments'] ." Comments</a>"; } echo "</div>"; } mysql_close($con); ?> P.S There is a config.php file declared in the header of the file, so if you wondering about a connection string! I can assure you it's there ^^
-
Problem with calling mySQL queries into meta tags
Johns3n replied to Johns3n's topic in PHP Coding Help
Come to think of it.. you probably dont need a loop as I am presuming you only have 1 row in the parameters table otherwise you will have more than 1 doctype, title etc.. so you can just do if (mysql_num_rows($params) > 0) { $site_params = mysql_fetch_assoc($params); //echo your stuff here } Right you are! There is only one row in the parameters table! I inserted the code you suggested, however with the same result as the with the "while" loop, it renders the page, but the everything in the echo's are still missing in the source code. Sorry for being this pain! -
Problem with calling mySQL queries into meta tags
Johns3n replied to Johns3n's topic in PHP Coding Help
For some strange reason error reporting is not working for me at all! Tried turning it on multiple times It is indeed a typo and the missing "<" is present in the file im editting, so the problem isn't there But thanks for pointing it out As for the config.php: <?php // Connection string $con = mysql_connect(localhost,*******,********); // If no connection is made generate a error warning if (!$con) { die('Ohh no! Could not connect: ' . mysql_error()); } // Selects the Database mysql_select_db(*******, $con); $db_prefix = 'lork_'; ?> However I 99% sure the problem isn't in the config.php file either since it has worked perfectly on other pages in the project to call mySQL queries -
the initial query? Would you mean the "Comment Counter" query or the "Blog posts" query? Seeing I already have one COUNT and GROUP BY in the "Comment Counter" query, it would make it hard adding another set of those, especially since the query it self works as intended when I user the statement ". $num_row['numComments'] ." defined by the AS in the "Comment Counter" query.
-
Problem with calling mySQL queries into meta tags
Johns3n replied to Johns3n's topic in PHP Coding Help
I did try running my SQL statement through the DB earliere and it did return the values I needed then But I did try to do as you said, however it did nothing -
Hello PHPFreaks! I am nearly done with my project which is a small Blog CMS, however I have run into a couple of problems that I have posted to this forum, in the hopes that you users might be able to assist me with! This might seem like a complete newbie question, but interestingly enough that is exactly what I am ^^ However regardless of the difficulty of the problem, it is a problem that has been giving me a headache for the past few hours, so I was hoping that there were some PHPfreaks what might be of assistance and seeing where my problem(s) lie, as I am afraid I have stared myself blind to the problem. At first I thought it was possible to just use a MySQL query to call some values into some metatags! It might be that simple and I just made a simple mistake, but really hoping you can help me! I supplied the code in question below ?php include("config.php"); ?> <?php $params = mysql_query("SELECT * FROM ". db_prefix ."parameters"); while($site_params = mysql_fetch_assoc($params)) { echo "<!DOCTYPE html PUBLIC '-//W3C//DTD XHTML 1.0 Transitional//EN' 'http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd'>"; echo "<html xmlns='http://www.w3.org/1999/xhtml'>"; echo "<head>"; echo "<meta name='Keywords' content='" . $site_params['site_keywords'] . "' />"; echo "<meta name='Description' content='" . $site_params['site_description'] . "' />"; echo "<title>" . $site_params['site_title'] . "</title>"; } ?> <meta name="Robots" content="ALL" /> <meta http-equiv="Content-Type" content="text/html; charset=utf-8" /> <link rel="alternate" title="RSS" href="*********/rss.php" type="application/rss+xml" /> <link href="default.css" rel="stylesheet" type="text/css" media="all" /> </head> <body> The page it self, renders perfectly, however everything in the echo tags are simply left out of the code. In advance thanks! (P.S I am rookie at both PHP and SQL, so when if you need to explain something to me, do not take it I know things for granted xD)
-
Hello PHPFreaks! I am nearly done with my project which is a small Blog CMS, however I have run into a couple of problems that I have posted to this forum, in the hopes that you users might be able to assist me with! I have written a SQL query that counts the amount of comments, that is "tied" to a Blog Post entry. The blog post entries are generated through a "while" a loop, since I found a way to paginate them if I ran it through a while statement. However, when I need to get my second SQL statement (the one that counted the amound of comments) into the while loop, I thought it was most prudent to just make another while loop inside it, however when I ran the script I could see that was not the case! Since it generated this: (Blog post nr. 2 has 2 comments tied to it and Blog post nr. 1 has 1 comment tied to it in the Database by the way!) BLOG POST NR. 2 BLAH BLAH BLAH (Blog post content) 1 Comments2 Comments BLOG POST NR. 1 BLAH BLAH BLAH (Blog post content) So this is what the page output for me! The Blog post entries are shown 100% correct, however the "Comment Counter" is the only thing that is off! And I think it might have something to do with while in while statement, but below I supplied the PHP code I wrote! and I hope that you can help me solve this little mystery. <?php $num_query = "SELECT ". $db_prefix ."posts.id,". $db_prefix ."posts.title,". $db_prefix ."posts.content,". $db_prefix ."posts.date,". $db_prefix ."posts.author, COUNT(". $db_prefix ."comments.id) AS numComments FROM ". $db_prefix ."comments INNER JOIN ". $db_prefix ."posts ON ". $db_prefix ."comments.post_id = ". $db_prefix ."posts.id GROUP BY ". $db_prefix ."posts.id, ". $db_prefix ."posts.title,". $db_prefix ."posts.content,". $db_prefix ."posts.date,". $db_prefix ."posts.author"; $num_result = mysql_query($num_query); // This is where the HTML output of our Blog Posts is generated while($row = mysql_fetch_array($result)) { echo "<div class='post_entry'>"; echo "<div class='post_title'><h2><a href='singlepost.php?itemid=" . $row['id'] . "' title='" . $row['title'] . "'>" . $row['title'] . "</a></h2></div>"; echo "<div class='post_author'><p>Written by: " . $row['author'] . "</p></div>"; echo "<div class='post_date'><p>Posted: " . $row['date'] . "</p></div>"; echo "<div class='post_entry_content'><p>" . $row['content'] . "</p></div>"; echo "<div class='post_comments'><a href='singlepost.php?itemid=" . $row['id'] . "#comments' title='Click here to view comments'>"; while($num_row = mysql_fetch_assoc($num_result)) { echo "". $num_row['numComments'] ." Comments</a>"; } echo "</div>"; } mysql_close($con); ?> In advance thanks! (P.S I am rookie at both PHP and SQL, so when if you need to explain something to me, do not take it I know things for granted xD)
-
Okay, since im 100% that the mysql is correct, il post the entire file that i am working in right now so that you may get a better understanding of how it works.. <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <meta name="Description" content="INSERT DISCRIPTION OF YOUR WEBSITE HERE, THIS DISCRIPTION IS ALSO USED FOR YOUR WEBSITE WHEN LISTED IN GOOGLE SEARCH RESULTS" /> <meta name="Keywords" content="INSERT YOUR WEBSITE KEYWORDS HERE SEPERATED BY COMMAS" /> <meta name="Robots" content="ALL" /> <meta http-equiv="Content-Type" content="text/html; charset=utf-8" /> <link rel="alternate" title="RSS" href="http://www.prankstar.dk/johns3n/lork/rss.php" type="application/rss+xml" /> <link href="default.css" rel="stylesheet" type="text/css" media="all" /> <title>TITLE OF YOUR WEBSITE</title> </head> <body> <div class="wrapper"> <div class="posts"> <img src="gfx/logo.jpg" alt="Lörk Logo" /> <!-- Insert Posts Start --> <?php include("config.php"); ?> <?php // Selects the Blog Posts table $tbl_name="". $db_prefix ."posts"; // Defines how many adjacent pages should be shown on each side on the pagination module $adjacents = 3; // Gets the total number of rows in data table. $query = "SELECT COUNT(*) as num FROM $tbl_name"; $total_pages = mysql_fetch_array(mysql_query($query)); $total_pages = $total_pages[num]; // The file which should include the Pagination Module (Always the name of the file this code appears in ex. Index.php) $targetpage = "index.php"; // Defines the number of Blog Posts to show on each page $limit = 4; $page = $_GET['page']; if($page) // Defines the first item to display on this page $start = ($page - 1) * $limit; else // If no page variable is given, set start to 0 $start = 0; // Get Blog Post data from MySQL database $sql = "SELECT * FROM $tbl_name ORDER BY id DESC LIMIT $start, $limit"; $result = mysql_query($sql); // Setup variables for Pagination Module. (DO NOT EDIT THESE!) if ($page == 0) $page = 1; // If no page var is given, default to 1. $prev = $page - 1; // Previous page is page - 1 $next = $page + 1; // Next page is page + 1 $lastpage = ceil($total_pages/$limit); // Lastpage is = total pages / items per page, rounded up. $lpm1 = $lastpage - 1; // Last page minus 1 // Apply the variables and output the pagination Module. // (We're actually saving the code to a variable in case we want to draw it more than once.) $pagination = ""; if($lastpage > 1) { $pagination .= "<div class=\"pagination\">"; // Previous Button if ($page > 1) $pagination.= "<a href=\"$targetpage?page=$prev\">« Previous</a>"; else $pagination.= "<span class=\"disabled\">« Previous</span>"; // Pages if ($lastpage < 7 + ($adjacents * 2)) { for ($counter = 1; $counter <= $lastpage; $counter++) { if ($counter == $page) $pagination.= "<span class=\"current\">$counter</span>"; else $pagination.= "<a href=\"$targetpage?page=$counter\">$counter</a>"; } } // Enough pages are detected to start hiding some in the Pagination Module elseif($lastpage > 5 + ($adjacents * 2)) { // Close to beginning in Pagination Module: hide only later pages if($page < 1 + ($adjacents * 2)) { for ($counter = 1; $counter < 4 + ($adjacents * 2); $counter++) { if ($counter == $page) $pagination.= "<span class=\"current\">$counter</span>"; else $pagination.= "<a href=\"$targetpage?page=$counter\">$counter</a>"; } $pagination.= "..."; $pagination.= "<a href=\"$targetpage?page=$lpm1\">$lpm1</a>"; $pagination.= "<a href=\"$targetpage?page=$lastpage\">$lastpage</a>"; } // In the middle of the Pagination Module: Hide some pages front and some back elseif($lastpage - ($adjacents * 2) > $page && $page > ($adjacents * 2)) { $pagination.= "<a href=\"$targetpage?page=1\">1</a>"; $pagination.= "<a href=\"$targetpage?page=2\">2</a>"; $pagination.= "..."; for ($counter = $page - $adjacents; $counter <= $page + $adjacents; $counter++) { if ($counter == $page) $pagination.= "<span class=\"current\">$counter</span>"; else $pagination.= "<a href=\"$targetpage?page=$counter\">$counter</a>"; } $pagination.= "..."; $pagination.= "<a href=\"$targetpage?page=$lpm1\">$lpm1</a>"; $pagination.= "<a href=\"$targetpage?page=$lastpage\">$lastpage</a>"; } // Close to end of the Pagination Module: Hide only earliere pages else { $pagination.= "<a href=\"$targetpage?page=1\">1</a>"; $pagination.= "<a href=\"$targetpage?page=2\">2</a>"; $pagination.= "..."; for ($counter = $lastpage - (2 + ($adjacents * 2)); $counter <= $lastpage; $counter++) { if ($counter == $page) $pagination.= "<span class=\"current\">$counter</span>"; else $pagination.= "<a href=\"$targetpage?page=$counter\">$counter</a>"; } } } // Next Button if ($page < $counter - 1) $pagination.= "<a href=\"$targetpage?page=$next\">Next »</a>"; else $pagination.= "<span class=\"disabled\">Next »</span>"; $pagination.= "</div>\n"; } ?> <?php $amount = "SELECT ". $prefix ."posts.id,". $prefix ."posts.title,". $prefix ."posts.content,". $prefix ."posts.date,". $prefix ."posts.author, COUNT(". $prefix ."comments.id) AS numComments FROM ". $prefix ."comments INNER JOIN ". $prefix ."posts ON ". $prefix ."comments.post_id = ". $prefix ."posts.id GROUP BY ". $prefix ."posts.id, ". $prefix ."posts.title,". $prefix ."posts.content,". $prefix ."posts.date,". $prefix ."posts.author"; $num = mysql_query($amount,$con); // This is where the HTML output of our Blog Posts is generated while($row = mysql_fetch_array($result)) { echo "<div class='post_entry'>"; echo "<div class='post_title'><h2><a href='singlepost.php?itemid=" . $row['id'] . "' title='" . $row['title'] . "'>" . $row['title'] . "</a></h2></div>"; echo "<div class='post_author'><p>Written by: " . $row['author'] . "</p></div>"; echo "<div class='post_date'><p>Posted: " . $row['date'] . "</p></div>"; echo "<div class='post_entry_content'><p>" . $row['content'] . "</p></div>"; echo "<div class='post_comments'><a href='singlepost.php?itemid=" . $row['id'] . "#comments' title='Click here to view comments'>" . $num['numComments'] . " Comments</a>"; echo "</div>"; } mysql_close($con); ?> <!-- Insert Pagination Module --> <?=$pagination?> <!-- Call Posts End --> </div> </div> </body> </html>
-
Okay thanks for the advice i tweaked it abit around and finally got the MySQL query to output the right numbers in PHPmyadmin, however now the only problem is i can't make it show... the mysql query is 100% correct now.. just a matter of showing the data now code atm: <?php $amount = mysql_query("SELECT ". $prefix ."posts.id,". $prefix ."posts.title,". $prefix ."posts.content,". $prefix ."posts.date,". $prefix ."posts.author, COUNT(". $prefix ."comments.id) AS numComments FROM ". $prefix ."comments INNER JOIN ". $prefix ."posts ON ". $prefix ."comments.post_id = ". $prefix ."posts.id GROUP BY ". $prefix ."posts.id, ". $prefix ."posts.title,". $prefix ."posts.content,". $prefix ."posts.date,". $prefix ."posts.author", $con); // This is where the HTML output of our Blog Posts is generated while($row = mysql_fetch_array($result)) { echo "<div class='post_entry'>"; echo "<div class='post_title'><h2><a href='singlepost.php?itemid=" . $row['id'] . "' title='" . $row['title'] . "'>" . $row['title'] . "</a></h2></div>"; echo "<div class='post_author'><p>Written by: " . $row['author'] . "</p></div>"; echo "<div class='post_date'><p>Posted: " . $row['date'] . "</p></div>"; echo "<div class='post_entry_content'><p>" . $row['content'] . "</p></div>"; echo "<div class='post_comments'><a href='singlepost.php?itemid=" . $row['id'] . "#comments' title='Click here to view comments'>" . $amount['numComments'] . " Comments</a>"; echo "</div>"; } mysql_close($con); ?>
-
soo this would be correct? However it still doesn't work Sorry to be this incredible pain! <?php $amount = mysql_query("SELECT ". $db_prefix ."posts.id, ". $db_prefix ."comments.post_id, count(". $db_prefix ."comments.post_id) as numComments WHERE ". $db_prefix ."posts.id = ". $db_prefix ."comments.post_id", $con); $num_rows = mysql_num_rows($amount); // This is where the HTML output of our Blog Posts is generated while($row = mysql_fetch_array($result)) { echo "<div class='post_entry'>"; echo "<div class='post_title'><h2><a href='singlepost.php?itemid=" . $row['id'] . "' title='" . $row['title'] . "'>" . $row['title'] . "</a></h2></div>"; echo "<div class='post_author'><p>Written by: " . $row['author'] . "</p></div>"; echo "<div class='post_date'><p>Posted: " . $row['date'] . "</p></div>"; echo "<div class='post_entry_content'><p>" . $row['content'] . "</p></div>"; echo "<div class='post_comments'><a href='singlepost.php?itemid=" . $row['id'] . "#comments' title='Click here to view comments'>" . $row['numComments'] . " Comments</a>"; echo "</div>"; } mysql_close($con); ?>
-
$amount = mysql_query("SELECT ". $db_prefix ."posts.id, ". $db_prefix ."comments.post_id, WHERE ". $db_prefix ."posts.id = ". $db_prefix ."comments.post_id" count(". $db_prefix ."comments.post_id) as numComments", $con); Would this be an appropriate position for the where clause?
-
Tried that and didn't work But aren't we forgetting a kind of "WHERE post.id=comments.post_id" somewhere? I could be wrong? I have posted my DB structure as you mentioned about 2 posts up code so far: <?php $amount = mysql_query("SELECT ". $db_prefix ."posts.id, ". $db_prefix ."comments.post_id, count(". $db_prefix ."comments.post_id) as numComments", $con); $num_rows = mysql_num_rows($amount); // This is where the HTML output of our Blog Posts is generated while($row = mysql_fetch_array($result)) { echo "<div class='post_entry'>"; echo "<div class='post_title'><h2><a href='singlepost.php?itemid=" . $row['id'] . "' title='" . $row['title'] . "'>" . $row['title'] . "</a></h2></div>"; echo "<div class='post_author'><p>Written by: " . $row['author'] . "</p></div>"; echo "<div class='post_date'><p>Posted: " . $row['date'] . "</p></div>"; echo "<div class='post_entry_content'><p>" . $row['content'] . "</p></div>"; echo "<div class='post_comments'><a href='singlepost.php?itemid=" . $row['id'] . "#comments' title='Click here to view comments'>" . $row['numComments'] . " Comments</a>"; echo "</div>"; } mysql_close($con); ?>
-
I worked abit around with code that mikesta707 provided, I got the page rendered, unfortunaly it still doesn't show the correct number of comment posts, again! im very sorry! I am abit of a idiot when it comes to PHP, so any help is appreciated! <?php $amount = mysql_query("SELECT ". $db_prefix ."posts.id, ". $db_prefix ."comments.post_id, count(". $db_prefix ."comments.post_id) as numComments", $con); $num_rows = mysql_num_rows($amount); // This is where the HTML output of our Blog Posts is generated while($row = mysql_fetch_array($result)) { echo "<div class='post_entry'>"; echo "<div class='post_title'><h2><a href='singlepost.php?itemid=" . $row['id'] . "' title='" . $row['title'] . "'>" . $row['title'] . "</a></h2></div>"; echo "<div class='post_author'><p>Written by: " . $row['author'] . "</p></div>"; echo "<div class='post_date'><p>Posted: " . $row['date'] . "</p></div>"; echo "<div class='post_entry_content'><p>" . $row['content'] . "</p></div>"; echo "<div class='post_comments'><a href='singlepost.php?itemid=" . $row['id'] . "#comments' title='Click here to view comments'>" . $num_rows . " Comments</a>"; echo "</div>"; } mysql_close($con); ?>
-
Indeed! $result is all of my posts, and works without a problem i just need a small "by" script that counted the comments for each blog post If it's any help my db structure: comments{ id post_id comment_name comment_content comment_date } posts{ id title content date author } Hope this helps?
-
thanks both of you! Would be any assistance if i posted, my DB structure? Because i would really like some help with this since i think i got over my head here
-
Hello PHPfreaks I am having some trouble with the mysql_num_rows, i was written a small script that displays the total number of comments that was connected with a blog post, i got it to succesfully display a number, but unfortunally it took the total number of comments, instead of the numbers actually matching with that blog post. Please bear in mind, i am a complete novice at PHP. So please execuse me, if my question is complete idiotic one! here is the code that i use: <?php $amount = mysql_query("SELECT ". $db_prefix ."posts.id, ". $db_prefix ."comments.post_id "."FROM ". $db_prefix ."posts, ". $db_prefix ."comments "."WHERE ". $db_prefix ."posts.id = ". $db_prefix ."comments.post_id", $con); $num_rows = mysql_num_rows($amount); // This is where the HTML output of our Blog Posts is generated while($row = mysql_fetch_array($result)) { echo "<div class='post_entry'>"; echo "<div class='post_title'><h2><a href='singlepost.php?itemid=" . $row['id'] . "' title='" . $row['title'] . "'>" . $row['title'] . "</a></h2></div>"; echo "<div class='post_author'><p>Written by: " . $row['author'] . "</p></div>"; echo "<div class='post_date'><p>Posted: " . $row['date'] . "</p></div>"; echo "<div class='post_entry_content'><p>" . $row['content'] . "</p></div>"; echo "<div class='post_comments'><a href='singlepost.php?itemid=" . $row['id'] . "#comments' title='Click here to view comments'>" . $num_rows . " Comments</a>"; echo "</div>"; } mysql_close($con); ?> So in advance, thanks for your help!
-
Can't seem to get error reporting to work, still just shows a blank page when i run it Im using my brothers mySQL database and ftp server to test it out.. I've included the whole file now, since what i read about error reporting it only shows the error if the error code snippet is above it: <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <meta name="Robots" content="NONE" /> <meta http-equiv="Content-Type" content="text/html; charset=utf-8" /> <link href="cms.css" rel="stylesheet" type="text/css" media="all" /> <title>Lörk CMS - Installing!</title> </head> <body> <?php error_reporting(E_ALL); //This gets all the data from the form $hostname=$_POST['hostname']; $databasename=$_POST['databasename']; $db_username=$_POST['db_username']; $db_password=$_POST['db_password']; $prefix=$_POST['prefix']; $username=$_POST['username']; $password=$_POST['password']; $realname=$_POST['realname']; $con = mysql_connect($hostname,$db_username,$db_password); if (!$con) { die('Ohh no! Could not connect: ' . mysql_error() 'Are you sure that you wrote the correct data in the installer?'); } // Selects the Database mysql_select_db($databasename,$con); // Create tables $posts = "CREATE TABLE ". $prefix ."posts ( id int(3) not null auto_increment, title varchar(250), content text, date varchar(250), author varchar(250) )"; // Execute query to create tables mysql_query($posts,$con); $users = "CREATE TABLE ". $prefix ."users ( id int(3) not null auto_increment, username varchar(250), password varchar(250), realname varchar(250) )"; // Execute query to create tables mysql_query($users,$con); // Writes the admin user into the database mysql_query("INSERT INTO ".$prefix."users VALUES ('$id', '$username', '$password', '$realname')"); // Close the mySQL connection mysql_close($con); // Write the config.php file $filename = 'config.php'; $configs = "<?php // Connection string $con = mysql_connect("$hostname","$db_username","$db_password"); // If no connection is made generate a error warning if (!$con) { die('Ohh no! Could not connect: ' . mysql_error() 'Please check the Connection String, in the insertpost.php file!'); } // Selects the Database mysql_select_db("$databasename", $con); $db_prefix = "$prefix" ?> \n"; // Make sure that config.php is writeable if (is_writable($filename)) { // Open config.php if (!$handle = fopen($filename, 'a')) { echo "Cannot open file ($filename)"; exit; } // Write content to config.php if (fwrite($handle, $configs) === FALSE) { echo "Cannot write to file ($filename)"; exit; } // If it works! Yeah for us! echo "Success! You have now installed Lörk! Happy Bloggin!"; fclose($handle); } else { // If not! That sucks echo "The file $filename is not writable"; } ?> </body> </html>
-
Didn't know where such a thing as a error reporting! thanks for pointing that out to me! Maybe that could have saved myself a headache! ^^ il try and get back if i can't work it out by then! Thanks again!
-
Thanks for the swift answer mate I took a look at the things you pointed out and tried to apply them to where else i might see a similar problem, i tried running the script once again, but it still fails on me, so there for i turn to you again! The new and updated code: <?php //This gets all the data from the form $hostname=$_POST['hostname']; $databasename=$_POST['databasename']; $db_username=$_POST['db_username']; $db_password=$_POST['db_password']; $prefix=$_POST['prefix']; $username=$_POST['username']; $password=$_POST['password']; $realname=$_POST['realname']; $con = mysql_connect($hostname,$db_username,$db_password); if (!$con) { die('Ohh no! Could not connect: ' . mysql_error() 'Are you sure that you wrote the correct data in the installer?'); } // Selects the Database mysql_select_db($databasename,$con); // Create tables $posts = "CREATE TABLE "$prefix"posts ( id int(3) not null auto_increment, title varchar(250), content text, date varchar(250), author varchar(250) )"; // Execute query to create tables mysql_query($posts,$con); $users = "CREATE TABLE "$prefix"users ( id int(3) not null auto_increment, username varchar(250), password varchar(250), realname varchar(250) )"; // Execute query to create tables mysql_query($users,$con); // Writes the admin user into the database mysql_query("INSERT INTO ".$prefix."users VALUES ('$id', '$username', '$password', '$realname')"); // Close the mySQL connection mysql_close($con); // Write the config.php file $filename = 'config.php'; $configs = "<?php // Connection string $con = mysql_connect("$hostname","$db_username","$db_password"); // If no connection is made generate a error warning if (!$con) { die('Ohh no! Could not connect: ' . mysql_error() 'Please check the Connection String, in the insertpost.php file!'); } // Selects the Database mysql_select_db("$databasename", $con); $db_prefix = "$prefix" ?> \n"; // Make sure that config.php is writeable if (is_writable($filename)) { // Open config.php if (!$handle = fopen($filename, 'a')) { echo "Cannot open file ($filename)"; exit; } // Write content to config.php if (fwrite($handle, $configs) === FALSE) { echo "Cannot write to file ($filename)"; exit; } // If it works! Yeah for us! echo "Success! You have now installed Lörk! Happy Bloggin!"; fclose($handle); } else { // If not! That sucks echo "The file $filename is not writable"; } ?> again! Thanks alot for your time! as im starting to turn grey haired here! ^^
-
Hey all at PHP freaks! First off! Thanks for solving my previous problem very quickly and swiftly! Sure helped me alot! Now i'm once again in a position where i need the help of people who are alot more talented with PHP than me! (I only took it up, half a year ago, before that it was strictly HTML, CSS) I have created a little CMS blog system which works perfectly! Save for one little file! The installer file i have created! When i try to run it on a server, it just does nothing for me! Which means there is a mistake somewhere xD But I have spent like 1 hour looking over it and couldn't find it! Therefor i was hoping that one of you, could donate a little time to look over my installer code, and maybe see the problem? as i am afraid that i stared myself blind Here is the code: <?php //This gets all the data from the form $hostname=$_POST['hostname']; $databasename=$_POST['databasename']; $db_username=$_POST['db_username']; $db_password=$_POST['db_password']; $prefix=$_POST['prefix']; $username=$_POST['username']; $password=$_POST['password']; $realname=$_POST['realname']; $con = mysql_connect("" . $hostname . "","" . $db_username . "","" . $db_password . ""); if (!$con) { die('Ohh no! Could not connect: ' . mysql_error() 'Are you sure that you wrote the correct data in the installer?'); } // Selects the Database mysql_select_db("" . $databasename . "", $con); // Create tables $posts = "CREATE TABLE '".$prefix."'posts ( id int(3) not null auto_increment, title varchar(250), content text, date varchar(250), author varchar(250) )"; $users = "CREATE TABLE '".$prefix."'users ( id int(3) not null auto_increment, username varchar(250), password varchar(250), realname varchar(250) )"; // Execute query to create tables mysql_query($posts,$users,$con); // Writes the admin user into the database mysql_query("INSERT INTO '".$prefix."'users VALUES ('$id', '$username', '$password', '$realname')"); // Close the mySQL connection mysql_close($con); // Write the config.php file $filename = 'config.php'; $configs = "<?php // Connection string $con = mysql_connect('" . $hostname . "','" . $db_username . "','" . $db_password . "'); // If no connection is made generate a error warning if (!$con) { die('Ohh no! Could not connect: ' . mysql_error() 'Please check the Connection String, in the insertpost.php file!'); } // Selects the Database mysql_select_db('" . $databasename . "', $con); $db_prefix = " . $prefix . " ?> \n"; // Make sure that config.php is writeable if (is_writable($filename)) { // Open config.php if (!$handle = fopen($filename, 'a')) { echo "Cannot open file ($filename)"; exit; } // Write content to config.php if (fwrite($handle, $configs) === FALSE) { echo "Cannot write to file ($filename)"; exit; } // If it works! Yeah for us! echo "Success! You have now installed Lörk! Happy Bloggin!"; fclose($handle); } else { // If not! That sucks echo "The file $filename is not writable"; } ?> Again! Thank you alot for your time to gelp out a huge noob at PHP!
-
Thanks both you I realized that I made a typo david91, but was aware of that But thanks again It works flawlessly now I might make a new post later about how to secure a login box from SQL injection attacks.. but that will be another time, since i just need this working at the moment But again thanks This is now solved
-
Hello everyone @ PHPfreaks.com I recently began coding PHP and learned quite alot by own and doing some Google searches (yes, Google is our friend!) But unfortunaly, I have come across a problem which I wasn't able to find a solution to by myself (Maybe I just don't know what to search for probaly) But I saw my only way out to finally join a PHP community, and now I hope you can help with me problem. I made a small CMS system for a client, it's nothing much, but does it job.. but unfortunaly I have some issues with SQL and the $GET_ function, this is the code that is causing my headaches: $result = mysql_query("SELECT * FROM nybygninger WHERE id='"$_GET['itemid']"'"); while($row = mysql_fetch_array($result)) { echo "" . $row['name'] . ""; I made a page called Single.php which always has this after is it's URL ?item=idnumber refering to the post i need. But the above, but when i try to do with the top mentioned code string, the webpage just goes blank for me! So was hoping that you guys could help me see where i go wrong? ^^ Thanks in advance! - Kenneth (P.S Sorry if I posted in the wrong forum)