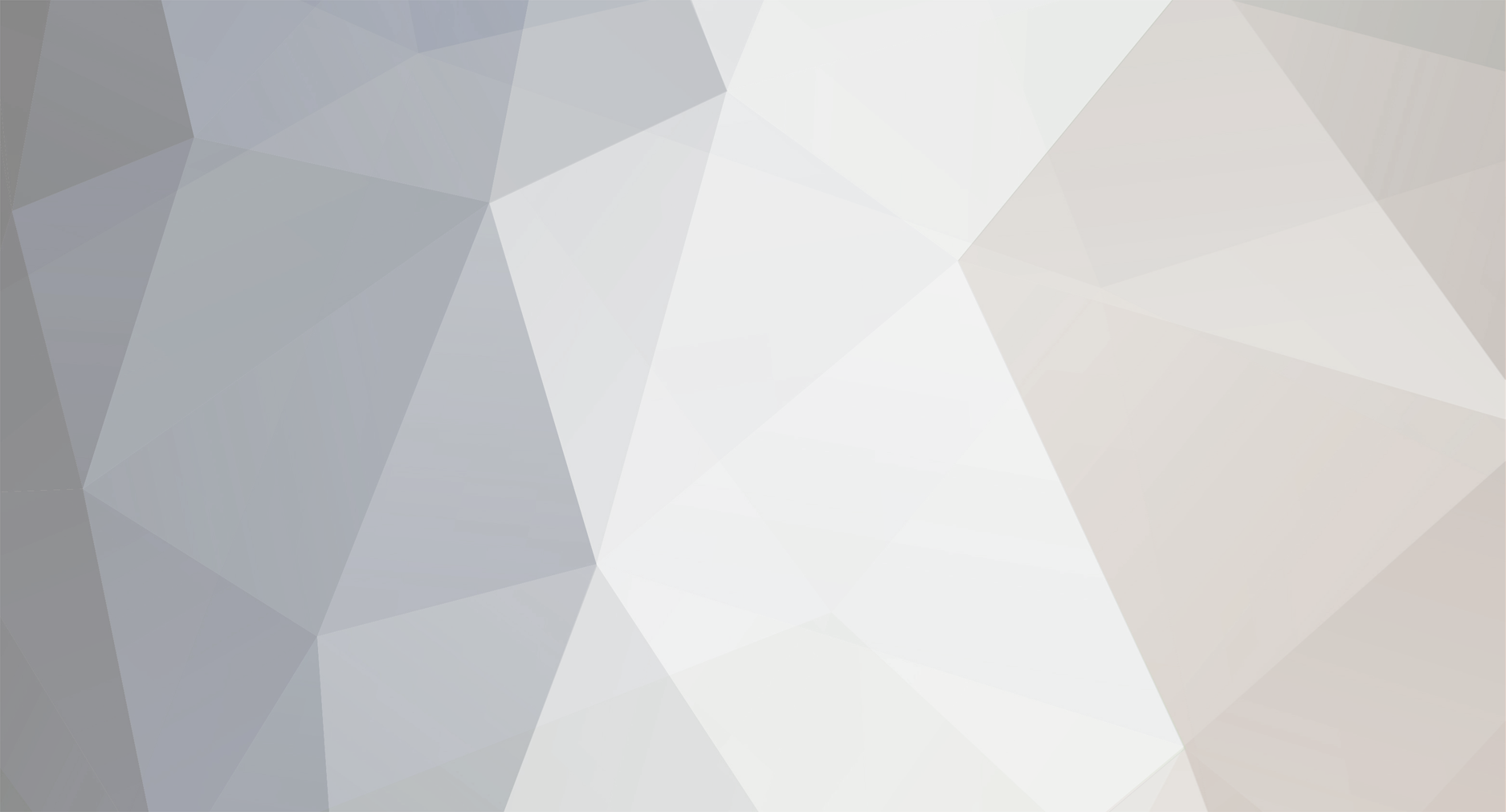
kirkh34
Members-
Posts
80 -
Joined
-
Last visited
Everything posted by kirkh34
-
does anyone have a simple script that could insert data into a table up to a few hundred rows using integers that increase until the number of rows is reached?
-
i have two tables of user messages that when put in the trash, receive a date 2 weeks ahead of the current time in the trash_expire column, i am trying to make an if statement, if now is greater than the expiration, delete the row....i cannot get this right i have tried for days $sql = mysql_query("SELECT * FROM message_sent WHERE message_trash = 1 UNION SELECT * FROM message_rec WHERE message_trash = 1") or die (mysql_error()); while ($row = mysql_fetch_array($sql)) { $trash_expire = $row['trash_expire']; $trash_expire = strtotime($trash_expire); $now = strtotime("now"); if ($trash_expire < $now) { $sql = mysql_query("DELETE FROM message_rec WHERE trash_expire < '$now'") or die (mysql_error()); $sql = mysql_query("DELETE FROM message_sent WHERE trash_expire < '$now'") or die (mysql_error()); } } //close while
-
this code kind of works, i don't know if i have an excess of code or if there is anything simpler than this, the problems are... if a row trash_date reaches 14 days old, it deletes all the rows in the table, also... when the message_trash is marked to "1" in the row then the trash_date is updated for the current time, i have a message_date that is the date of the creation of the message, but when the trash_date is updated, the message_date is updated also... i don't understand this at all because message_date it totally left out of all this.. so my two probs 1. when a trash_date in a single row reaches 14 days old, all of the rows in the table are deleted instead of the single row 2. when a trash_date is updated to the current time, it also updates the message_date ////////////////////////////// /////////////////////////////// //AUTOMATIC MESSAGE TRASH DELETE ////////////////////////////// /////////////////////////////// $sql = mysql_query("SELECT * FROM message_sent WHERE message_trash = 1 UNION SELECT * FROM message_rec WHERE message_trash = 1") or die (mysql_error()); while ($row = mysql_fetch_array($sql)) { $trash_date = $row['trash_date']; $trash_date = strtotime($trash_date); $twoweeks = strtotime('-14 days'); if ($trash_date < $twoweeks) { $sql = mysql_query("DELETE FROM message_rec WHERE trash_date > $twoweeks") or die (mysql_error()); $sql = mysql_query("DELETE FROM message_sent WHERE trash_date > $twoweeks") or die (mysql_error()); } } //close while ////////////////////////////// /////////////////////////////// //^^^^^^^^^^^^^^^^^^^^^^^^^^^^^// //AUTOMATIC MESSAGE TRASH DELETE ////////////////////////////// ///////////////////////////////
-
the message_date is the "timestamp"
-
how could you update a table automatically if a date of a row is a certain amount of days old? The date in my tables are displayed like this, date("Y-m-d H:i:s") this code isn't working, is this the correct way to use operators in a query? $twoweeks = strtotime('-14 days'); $twoweeks2 = date("Y-m-d H:i:s",$twoweeks); $sql = mysql_query("DELETE FROM message_rec WHERE message_date < $twoweeks2")
-
anyone? i am stumped
-
here's the full php section <?php $sql3 = mysql_query("SELECT * FROM message_rec WHERE to_user ='$id' AND message_trash = '1' UNION SELECT * FROM message_sent WHERE from_user ='$id' AND message_trash = '1' ORDER BY message_date DESC") or die (mysql_error()); while ($row = mysql_fetch_array($sql3)) { $message_id = $row["message_id"]; $message_content = $row["message_content"]; $message_date = $row["message_date"]; $message_title = $row["message_title"]; $to_user = $row["to_user"]; // the id of the session user in the message_rec table OR the id of the receiver in the message_sent table $from_user =$row["from_user"]; // the id of the receiver in the message_rec table OR the id of the sender (session user) in the message_sent table $message_read = $row["message_read"]; if ($to_user != $id) /// if the message was a sent message /// i want the $id to be = to the person it was sent to (so the pic display below will have the correct id) { $id = $row["to_user"]; } //close if $sql4 = mysql_query("SELECT * FROM mymembers WHERE id='$id'") or die (mysql_error()); while ($row = mysql_fetch_array($sql4)) { $firstname = $row["firstname"]; $lastname = $row["lastname"]; } $position= 30; // Define how many character you want to display. $title = substr($message_title, 0, $position); $content = substr($message_content, 0, $position); /// pic display $check_pic = "memberFiles/$id/image01.jpg"; $default_pic = "memberFiles/0/image01.jpg"; if (file_exists($check_pic)) { $pic_id = "<img src=\"$check_pic\" width=\"50px\" />"; // forces picture to be 100px wide and no more } else { $pic_id = "<img src=\"$default_pic\" width=\"50px\" />"; // forces default picture to be 100px wide and no more } $envelope_read = "images/read.gif"; $envelope_unread = "images/unread.gif"; if ($message_read == '0'){ $envelope = "<p style=color:#000;>Unread</p>"; } else { $envelope = "<p style=color:#9f9f9f;>Read</p>"; } if($from_id = $id){ $message_type = "SENT TO";} else{ $message_type = "RECEIVED BY";} echo "<tr>"; echo "<td>"; echo "<a href='profile.php?id=$to_user'>$pic_id</a>"; echo "</td>"; echo "<td>"; echo "<a href='profile.php?id=$to_user'>$firstname $lastname</a>"; echo "</td>"; echo "<td>"; echo "$message_type"; echo "</td>"; echo "<td>"; echo "<a href='message.php?id=$message_id&user=$id'><p id='title'>$title</p></a>"; echo "<p id='content'>$content</p>"; echo "</td>"; echo "<td>"; if (date("d, m, y", time()) != date("d, m, y", strtotime($message_date)) ) { print date('h:i A', strtotime($message_date)); } else { print "<p id='date'>"; print date('m/d/y', strtotime($message_date)); print "</p>"; print date('h:i A', strtotime($message_date)); } echo "</td>"; echo "<td>"; echo "<a href='outbox.php?id=$message_id'>Delete</a>"; echo "</td>"; echo "</tr>"; } //close while ?>
-
im trying to display messages that have been put in the trash by users, sent and received... the message info comes from the message_rec and message_sent tables. the member info comes from a mymembers table....the message info displays but the the picture of the person it was sent to or received from along with the name is not displaying... also the the link does not have the correct id... i seem to have a problem with the query to the mymembers table.. it is displaying the ID of the first person in the row for all messages it seems, i've tried to look for something simple but i've been looking at it for so long, all of it is kind of not making any sense <?php $sql3 = mysql_query("SELECT * FROM message_rec WHERE to_user ='$id' AND message_trash = '1' UNION SELECT * FROM message_sent WHERE from_user ='$id' AND message_trash = '1' ORDER BY message_date DESC") or die (mysql_error()); while ($row = mysql_fetch_array($sql3)) { $message_id = $row["message_id"]; $message_content = $row["message_content"]; $message_date = $row["message_date"]; $message_title = $row["message_title"]; $to_user = $row["to_user"]; // the id of the session user in the message_rec table OR the id of the receiver in the message_sent table $from_user =$row["from_user"]; // the id of the receiver in the message_rec table OR the id of the sender (session user) in the message_sent table $message_read = $row["message_read"]; if ($to_user != $id) /// if the message was a sent message /// i want the $id to be = to the person it was sent to (so the pic display below will have the correct id) { $id = $row["to_user"]; } //close if $sql4 = mysql_query("SELECT * FROM mymembers WHERE id='$id'") or die (mysql_error()); while ($row = mysql_fetch_array($sql4)) { $firstname = $row["firstname"]; $lastname = $row["lastname"]; } $position= 30; // Define how many character you want to display. $title = substr($message_title, 0, $position); $content = substr($message_content, 0, $position); /// pic display $check_pic = "memberFiles/$id/image01.jpg"; $default_pic = "memberFiles/0/image01.jpg"; if (file_exists($check_pic)) { $pic_id = "<img src=\"$check_pic\" width=\"50px\" />"; // forces picture to be 100px wide and no more } else { $pic_id = "<img src=\"$default_pic\" width=\"50px\" />"; // forces default picture to be 100px wide and no more }
-
i have two tables which hold message data for sent and received messages, im trying to display messages that have been put in the trash, how do i access two tables at once to display the message data, the code i have doesn't work of course but i have both queries with the tables i need the data from <?php $sql = mysql_query("SELECT * FROM message_rec WHERE to_user ='$id' AND message_trash = '1' ORDER BY message_date DESC") or die (mysql_error()); $sql = mysql_query("SELECT * FROM message_sent WHERE from_user ='$id' AND message_trash = '1' ORDER BY message_date DESC") or die (mysql_error()); while ($row = mysql_fetch_array($sql)) { $message_id = $row["message_id"]; $message_content = $row["message_content"]; $message_date = $row["message_date"]; $message_title = $row["message_title"]; $to_id = $row["to_user"]; $message_read = $row["message_read"];
-
well the submit is under isset().. it's the next part down that's not working, if i leave an empty title or body it still says "message has been sent" and data is put into the table as blanks
-
hello, i have a compose message file with a form that inserts data into tables, i'm trying to figure out how to make an error appear if they have left a title or body blank, i'm not sure why this isn't working, here is the code <?php session_start(); include_once "scripts/connect_to_mysql.php"; $logout = ""; $login_table = ""; $message_title= $_POST['message_title']; $message_content= $_POST['message_content']; $to_user= $_POST['to_user']; $from_user= $_POST['from_user']; if (isset ($_POST['submit'])){ if((!$message_title) || (!$message_content)){ if (!$message_title){ $msgToUser = "You need a title!"; include_once "msgToUser.php"; } if(!$message_content){ $msgToUser = "You need a body!"; include_once "msgToUser.php"; } } } else { $sql = mysql_query("INSERT INTO message_rec (to_user, message_content, message_title) VALUES('$to_user','$message_content','$message_title')") or die (mysql_error()); $sql = mysql_query("INSERT INTO message_sent (from_user, message_content, message_title) VALUES('$from_user','$message_content','$message_title')") or die (mysql_error()); $msgToUser = "Your message has been sent"; include_once "msgToUser.php"; } ?>
-
hello, i'm creating a pm system where users can exchange messages, i have a message_id, to_id, from_id in my table of "messages"... everything works fine, one message is put into the database with it's unique id, my problem is... when say a sender deletes one of his sent messages, the receivers message is also deleted... i do not want this.. i've been trying to figure out how to set it up so that doesn't happen...2 copies of messages in the table? how can i go about doing this so each message, sent or received, is the users own... thanks
-
nvm, figured it out, it is funny how you can let something so simple end up really consfusing, thanks
-
well im a php noob and a little rusty on all of this, can someone give me an example of what kind of query i should have? i think i can understand once i see it
-
this page is an inbox of links to messages i have a $firstname and $lastname that displays next to a picture and they are both links to a user profile... i use the same $from_id to link to their profile and it works just fine... i am trying to use this $from_id variable to pull from a different table to obtain their first and last name, the problem is... it shows the first and last name of the user who has sent the latest message for all of the users... i can't figure it out... all the other users links to their profile works just fine with the $from_id variable but for some reason it won't pull individual first and last names....maybe it's because i'm pulling from a different table? i may be doing this wrong but i cannot figure it out...help is appreciated <?php session_start(); include_once "scripts/connect_to_mysql.php"; if (!isset($_SESSION['id'])) header('Location: /index.php'); $user = $_SESSION['id']; if (isset($_GET['id'])) { $delete_id = $_GET['id']; $sql = mysql_query("DELETE FROM messages WHERE message_id ='$delete_id'") or die (mysql_error()); } $sql = mysql_query("SELECT * FROM messages WHERE to_user ='$user'") or die (mysql_error()); while ($row = mysql_fetch_array($sql)) { $from_id = $row["from_user"]; }//close while $sql = mysql_query("SELECT * FROM mymembers WHERE id ='$from_id'") or die (mysql_error()); while ($row = mysql_fetch_array($sql)) { $firstname = $row["firstname"]; $lastname = $row["lastname"]; } //close while ?> <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <meta http-equiv="Content-Type" content="text/html; charset=utf-8" /> <title>Untitled Document</title> <style type="text/css"> #customers { font-family:"Trebuchet MS", Arial, Helvetica, sans-serif; border-collapse:collapse; padding: 0px; } #customers td, #customers th { width: auto; font-size:.8em; border-bottom:1px solid #98bf21; padding:5px 20px 5px 0px; } #customers th { font-size:1.1em; text-align:center; padding-top:5px; padding-bottom:4px; background-color:#A7C942; color:#ffffff; width: auto; } #content{ color: #999; margin: 0 0 0 0; } #title{ margin: 0 0 0 0 ; } #date{ color: #999; margin: 0; } </style> </head> <body> <table id="customers"> <tr> <th>Inbox</th> </tr> <tr> <td></td> <td>Name</td> <td></td> <td>Subject</td> <td>Date</td> <td> </td> </tr> <?php $sql = mysql_query("SELECT * FROM messages WHERE to_user ='$user'") or die (mysql_error()); while ($row = mysql_fetch_array($sql)) { $message_id = $row["message_id"]; $message_content = $row["message_content"]; $message_date = $row["message_date"]; $message_title = $row["message_title"]; $from_id = $row["from_user"]; $message_read = $row["message_read"]; $position= 30; // Define how many character you want to display. $title = substr($message_title, 0, $position); $content = substr($message_content, 0, $position); $check_pic = "memberFiles/$from_id/image01.jpg"; $default_pic = "memberFiles/0/image01.jpg"; if (file_exists($check_pic)) { $from_user = "<img src=\"$check_pic\" width=\"50px\" />"; // forces picture to be 100px wide and no more } else { $from_user = "<img src=\"$default_pic\" width=\"50px\" />"; // forces default picture to be 100px wide and no more } $envelope_read = "images/read.gif"; $envelope_unread = "images/unread.gif"; if ($message_read == '0'){ $envelope = "<img src=\"$envelope_unread\" width=\"25px\" />"; } else { $envelope = "<img src=\"$envelope_read\" width=\"25px\" />"; } echo "<tr>"; echo "<td>"; echo "<a href='profile.php?id=$from_id'>$from_user</a>"; echo "</td>"; echo "<td>"; echo "<a href='profile.php?id=$from_id'>$firstname $lastname</a>"; echo "</td>"; echo "<td>"; echo "$envelope"; echo "</td>"; echo "<td>"; echo "<a href='message.php?id=$message_id&user=$user'><p id='title'>$title</p></a>"; echo "<p id='content'>$content</p>"; echo "</td>"; echo "<td>"; if (date("d, m, y", time()) == date("d, m, y", strtotime($message_date)) ) { print date('h:i A', strtotime($message_date)); } else { print "<p id='date'>"; print date('m/d/y', strtotime($message_date)); print "</p>"; print date('h:i A', strtotime($message_date)); } echo "</td>"; echo "<td>"; echo "<a href='inbox.php?id=$message_id'>Delete</a>"; echo "</td>"; echo "</tr>"; } //close while print "$from_id"; ?> </table> </body> </html>
-
awesome! thanks a lot, the times are correct, the were correct in the database the whole time but the other way showed the time hours off...i wonder why..oh well this works great thanks again
-
yeah that worked but it does the same thing as date('d,n,y', time()), it shows the time but it's showing like 1:20pm instead of 10:20pm but i forgot to mention, the formula isn't working right, i have it so if today is equal to the date of the message, then it will output just the time, if it isn't equal to today it will just output the month day and year but it's backwards, it won't if i change it to != then it does output just the time like i want but it's hours off, confusing
-
well i can't seem to get it right here, it sounded like it was gonna work aright but... if i try to print strftime('%F'); to see what it says.. nothing shows up, so i thought that wasn't gonna work so i tried to do date('d,n,y', time() to match up which seems like the same thing but it doesn't work either, it just shows the month day and year, and it should just show the time since it's the same day, if i change the == to != to reverse it, it shows just the time but it's totally wrong, it's like 4 hours off, im pretty confused lol
-
ahh i see, thanks a lot guys, i should've researched the meanings of all the symbols for outputting time on php.net lol
-
im trying to display the current time if it's the same day but if it's not the same day then just the day... i dont know how to match what mysql has to the current time because the current time from the function is from seconds from 1970 (i think thats what it is i forget) code: echo "<td>"; if (strftime('%a, %b %d, %Y', time()) == strftime('%a, %b %d, %Y', strtotime($message_date)) ) { print strftime('I:%M %p', strtotime($message_date)); } else { print strftime('%a, %b %d, %Y', strtotime($message_date)); } echo "</td>";
-
i have a messaging system where i'd like to display the date and time of a message.... how can i take 0000-00-00 00:00:00 and pull from it to display the words, like sunday, april 25, 2010 6:00 pm, is there any easy way to do this?
-
okay, well it sounds easier that way, i did a tutorial on a user system and messaging system and he had multiple databases... but i just got it to work by accessing the messages db just for the id, then pulling from mymembers and then back to pulling from messages for the table, so idk, you are right it would prob be easier w/ just one db oops i noticed something, i've been saying db when i just mean tables in the db, lol i am a noooob, anyway i got it to work
-
hello, im setting up a messaging system where users can create a profile and exchange messages... im on the inbox page where i'm listing the messages with the name, a thumbnail of the sender, the subject, and date. i have two databases right now, one with the member info including their name and origin and so on, i have another database for the messages... in the db "messages" it has a "from_user" and "to_user", when you go t your inbox it matches the session id to the "to_user". I am trying to list the persons name in the table of who it's from... but "from_user" is just an id of the user... i need to access the db of mymembers that has the name matched with the id... i'm not sure how to access both databases... , here's the code <?php session_start(); include_once "scripts/connect_to_mysql.php"; $user= $_SESSION['id']; ?> <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <meta http-equiv="Content-Type" content="text/html; charset=utf-8" /> <title>Untitled Document</title> <style type="text/css"> #customers { font-family:"Trebuchet MS", Arial, Helvetica, sans-serif; width:100%; padding: 0px; } #customers td, #customers th { font-size:1em; border-top:1px solid #98bf21; border-bottom:1px solid #98bf21; padding:3px 7px 2px 7px; } #customers th { font-size:1.1em; text-align:left; padding-top:5px; padding-bottom:4px; background-color:#A7C942; color:#ffffff; } #customers tr.alt td { color:#000000; background-color:#EAF2D3; } </style> </head> <body> <table id="customers"> <tr> <th>Inbox</th> </tr> <tr> <td>Picture</td> <td>Name</td> <td>Subject</td> <td>Date</td> </tr> <?php $sql = mysql_query("SELECT * FROM messages WHERE to_user ='$user'") or die (mysql_error()); while ($row = mysql_fetch_array($sql)) { $from_id = $row["from_user"]; $check_pic = "memberFiles/$from_id/image01.jpg"; $default_pic = "memberFiles/0/image01.jpg"; if (file_exists($check_pic)) { $from_user = "<img src=\"$check_pic\" width=\"50px\" />"; // forces picture to be 100px wide and no more } else { $from_user = "<img src=\"$default_pic\" width=\"50px\" />"; // forces default picture to be 100px wide and no more } echo "<tr>"; echo "<td>"; echo "$from_user"; echo "</td>"; echo "<td>"; echo "$firstname"; echo "</td>"; echo "<td>"; echo $row["message_title"]; echo "</td>"; echo "<td>"; echo $row["message_title"]; echo "</td>"; echo "</tr>"; } //close while ?> </table> </body> </html>
-
<?php session_start(); include_once "scripts/connect_to_mysql.php"; $from_user= $_SESSION['id']; $to_user= $_GET['id']; $sql = mysql_query("SELECT * FROM myMembers WHERE id='$to_user'"); while($row = mysql_fetch_array($sql)){ $firstname = $row["firstname"]; } ?> <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <meta http-equiv="Content-Type" content="text/html; charset=utf-8" /> <title>Message to <?php echo "$firstname"; ?></title> <form name="message" action="messageck.php" method="post"> Title: <input type="text" name="message_title"><br> Message:<br /> <textarea rows="20" cols="50" name="message_content"></textarea> <?php echo '<input type="hidden" name="to_user" value="'.$to_user.'"><br>'; ?> <?php echo '<input type="hidden" name="from_user" value="'.$from_user.'"><br>'; ?> <input type="submit" value="Submit"> </form> </body> </html> <?php session_start(); $message_title= $_POST['message_title']; $message_content= $_POST['message_content']; $to_user= $_POST['to_user']; $from_user= $_POST['from_user']; if (isset ($_POST['message_title'])){ include_once "scripts/connect_to_mysql.php"; if((!$message_title) || (!$message_content)){ if(!$message_title){ $msgToUser = "You need a title!"; include_once "msgToUser.php"; } if(!$message_content){ $msgToUser = "You need a body!"; include_once "msgToUser.php"; } } } else { $sql = mysql_query("INSERT INTO messages (to_user, from_user, message_content, message_title) VALUES('$to_user','$from_user','$message_content','$message_title')") or die (mysql_error()); $msgToUser = "Your message has been sent"; include_once "msgToUser.php"; } ?>
-
hello, i'm trying to use the php mail function and when i do i get a 500 internal server error message, is there anything i can do to fix this or is it out of my hands?