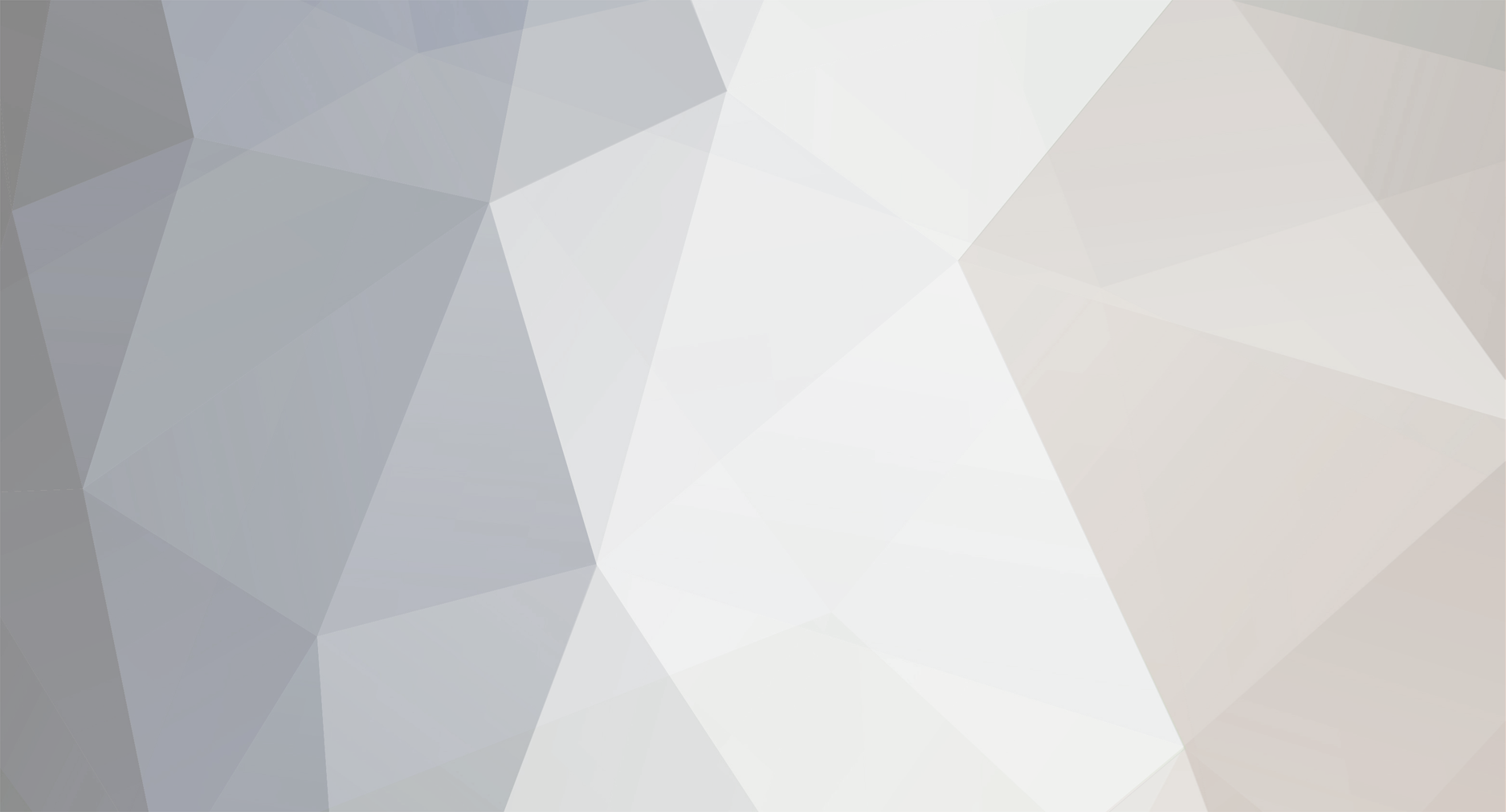
Fergal Andrews
-
Posts
65 -
Joined
-
Last visited
Posts posted by Fergal Andrews
-
-
hi unemployment,
you shouldn't need to restart the system, but you probably need to restart MySQL so that it takes the updated system time.
Fergal
-
Hi unemployment,
As far as I am aware MySQL uses the system time. "date_default_timezone_set" sets the timezone for PHP's date functions but will not alter the system time, so it will not affect MySQL.
Fergal
-
hi fmohrmann,
I think Nightslyr is correct about the load order of the javascript and html.
You might be better using jquery to do this. You can check the dom has loaded before the javascript is read.
It is off-topic for a php forum to go into a lot of detail about javascript but here is an example of some jquery that will show and hide divs. You should be able to adapt this to your needs.
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01//EN" "http://www.w3.org/TR/html4/strict.dtd" > <html lang="en"> <head> <title>test</title> <script src="http://ajax.googleapis.com/ajax/libs/jquery/1.4/jquery.min.js" type="text/javascript"></script> <script type="text/javascript"> $(document).ready(function() { // by default show the first gallery $('div.gallery').hide(); $('#div1').show(); $('a.button').click(function() { // get the numeric id of the link var idNum = this.id.substr(6,this.id.length); // create the id for the gallery div var galleryDivId = 'div' + idNum; // add the hide class to all galleries $('div.gallery').hide(); // show the chosen gallery $('#' + galleryDivId).show(); }) }) </script> <style type="text/css"> div.gallery { border: 1px solid #000; padding: 30px; width: 70px; } </style> </head> <body> <div class="gallery" id="div1"> test 1 </div> <div class="gallery" id="div2"> test 2 </div> <div class="gallery" id="div3"> test 3 </div> <div class="gallery" id="div4"> test 4 </div> <a href="#" class="button" id="button1">gallery 1</a> <a href="#" class="button" id="button2">gallery 2</a> <a href="#" class="button" id="button3">gallery 3</a> <a href="#" class="button" id="button4">gallery 4</a> </body> </html>
Hope this helps,
Fergal
-
Hi brown2005,
I think MySQL's FULLTEXT indexing and searching could be what you need. I'm not sure if you have the access to the database to alter tables in order to index them for FULLTEXT searches but if you do then this could help you achieve what you want. FULLTEXT searches allow you to use multiple search words and other advanced options.
Here are a couple of URL's about FULLTEXT :
http://www.petefreitag.com/item/477.cfm
http://onlamp.com/pub/a/onlamp/2003/06/26/fulltext.html
Hope that helps,
Fergal
-
Hi maraza,
How are you viewing the content? Is it possible that the content is actually correct but the viewing medium is causing the problem, such as a web page that does not have the correct content type or character set specified?
Fergal
-
Hi xuniter,
The problem is with the form action. You're missing an end quote:
<form action="dologin.php method="post">
should be
<form action="dologin.php" method="post">
Also, headers need to be sent before anything is output to the page. It is best to move your php code containing the header command to the top of the page before <html>, etc. There's no need to use an else command. If the condition is not met then the code will just continue. If it is met then the header will redirect to the new page.
Cheers,
Fergal
<?php if (isset($_SESSION['user'])) { header("Location: index.php"); } ?> <html> <head> <title>Basic CMS | Admin area Login</title>
-
Hi billy_111,
Your form inputs have no value so the form is returning an empty array. Try something like: <input type="text" name="author[]" id="author1" value="author1" /> <input type="text" name="author[]" id="author2" value="author2" /> <input type="text" name="author[]" id="author3" value="author3" /> <input type="text" name="author[]" id="author4" value="author4" /> ... ... ... <input type="text" name="author[]" id="author10" value="author10" />
Fergal
-
Hi csckid,
You use the $_FILES array to access uploaded files. When a file is uploaded it is written to a temporary directory. You then need to move it to its correct location. You access the file using $_FILES['myfile'] , where 'myfile' is the name you gave the file field in your form:
$tempName = $_FILES['myfile']['tmp_name']; $uploadedFilename = $_FILES['myfile']['name']; $directory = '/uploadedFiles'; move_uploaded_file($tempName, $directory . '/' . $uploadedFilename);
Do a var_dump of $_FILES['myfile'] to see all the array variables you can access.
all the best,
Fergal
-
Hi mattspriggs28,
There are a number of ways to prevent reading of files but allow writing to a directory. You can do it with server configuration but directory permissions is usually easier. If your hosting is unix/linux then check this link for information on how to change the permissions. You can often do this through your ftp software.
http://www.perlfect.com/articles/chmod.shtml
Also, stick a blank index.html file in there so that it will display instead of a list of files if a user goes directly to the directory url.
all the best,
Fergal
-
Hi viperjts10,
I ran your code and the redirection worked for me.
The only thing i added was a class to create the session object with methods is_logged_in() and logout() both of which returned true.
I'm not sure why you are having problems but it must be something in the code preceeding the snippets you posted.
The full code I used is below.
All the best,
Fergal
<?php class session { function is_logged_in() { return true; } function logout() { return true; } } $session = new session(); if($session->is_logged_in()) { /* Kill session variables */ $session->logout(); //$_SESSION = array(); // reset session array redirect_to('index.php'); //echo "<meta http-equiv=\"Refresh\" content=\"0;url=index.php\">"; } function redirect_to($location = NULL) { if ($location != NULL) { header("Location: {$location}"); exit; } }
-
Hi MyCode,
You could use this:
function createSubstring($string)
{
$pos = strpos($string, ":");
$substring = substr($string, $pos - 1, strlen($string));
return $substring;
}
all the best,
Fergal
-
Hi Chud37,
I haven't tested the code but you have $_POSt instead of $_POST in your if statement.
All the best,
Fergal
-
Hi,
I'm trying to create a PDF using the PdfLib extension. I am having great difficulty finding out how to change the colour of fonts. The only info I can find on font colours relates to the block plugin for PdfLib and I don't think the client has that on their server.
Can anyone tell me how to do this?
Thanks in advance,
Fergal
-
Hi thorpe,
thanks for the reply.
The job is for a bank who are paranoid about security, which I guess is understandable. They are also worried about the fact that there will be a lot of use. PHP's session handling can have problems when subjected to heavy traffic.
Cheers,
Fergal
-
Hi,
I am writing a session management class for a project I am working on at the moment. The live server has a very restrictive environment, PHP4, Oracle 9i, no cache available, no writing to files on the server and no usage of PHP sessions.
Consequently, I am writing a class to manage sessions as the project involves a big online form over several pages. I've written the class which uses a cookie to store an ID and writes 'session' data to the database.
However, I need to handle users that have disabled cookies. I was thinking the best solution would be to write the id to a hidden form field, but there might be some security concerns about that. Does anyone have a better solution for this problem?
Many thanks,
Fergal
-
Ok, I see what you mean, but the form code you have supplied has no way of submitting the form so the data is not going to be saved. If this is going to be done by javascript in the popup code then that's ok, if unreliable.
-
Hi Justin,
Using a hyperlink to a php page will not submit form data, so when your link goes to info.php there is no form data. The SQL statement therefore will be
SELECT * FROM info WHERE vipcode=''
which will return no results unless you have a record with a null code value. The conditional statement
if (!$row[levelofproject] || !$row[name] || !$row[id]){
therefore fails and nothing is output to the screen.
All the best,
Fergal
You need to use a proper method of submitting the form, either a submit input or javascript and put a form action with info.php as the value in the form tag.
Fergal
-
Well, in terms of security sessions are better but they do die as soon as the sesssion is over. With login data, I wouldn't use cookies, I'd use sessions.
-
Hi Iluvatar13,
If you just want to disable the form and stop the user's journey through the shopping cart, then nothing you do will HTML or Javascript will affect the PHP.
If you don't need anything from the form, then why show it to the user in the first place? Would it not be better to bring them to a different page and show them the required message instead? You could do this with javascript or PHP.
Fergal
-
Hi corrupshun,
Cookies and Sessions are useful for different things. The advantage of cookies is that they can persist after the user has closed their browser. Generally, sessions are more reliable because the user can turn off cookies so you can't depend on the data they contain, though very few people actually do that. Some complex server environments where you may have many load balanced servers and proxy servers can make the use of sessions unreliable. I have done quite a bit of work for the BBC and within their complex server environment, session data can get lost. It really comes down to a judgement call as to which suits the job you are doing best. If you are in doubt then both is the safest option.
Fergal
-
Hi bullbree,
The Notice you are getting is because your first usage of pfrontimage is within a conditional statement.
You can fix this by either putting @ before
@$pfrontimage = mysql_real_escape_string($_POST['pfrontimage']);
which will supress the Notice or by declaring the variable earlier in your code before it is used.
$pfrontimage = '';
I've only had a quick look at your code but I can't understand why your are doing a mysql_real_escape_string on pfrontimage if it is a file. Do you have two form fields named pfrontimage?
If not and I could be wrong, but you seem to be using the same variable as a string and a file in different parts of your code.
-
Hi dbradbury,
sorry for not following this up but I've been really busy lately.
How did you sort it and well done for doing so?
Fergal
-
sorry d, my tiredness is getting in the way this evening. Try changing 'image/jpg' to 'image/jpeg'
-
Sorry, I should have spotted the schoolboy error in the modifed script I gave you (it's been a long day!). It was echoing the path to the file and not the data. Try this on your local file and then the remote one.
<?php
if(isset($_GET['player']))
{
$idpic = mysql_query("SELECT * FROM phpbb_attachments WHERE post_msg_id='$player'");
$countid = mysql_num_rows($idpic);
if($countid!=0)
{
$row = mysql_fetch_assoc($idpic);
$file = './forum/files/'.$row['physical_filename'];
if (file_exists($file))
{
$data = readfile($file);
header('Content-Type: image/jpg');
echo $data;
}
}
}
?>
PHP can only modify divs declared before the modifier
in PHP Coding Help
Posted
Hi Frederik,
Your problem is a bit confusing alright. Could it be something to do with the css? If the 'galleries_hidden' class sets the divs to display: none then they may not be visible to the javascript. I'm just guessing here.
Fergal