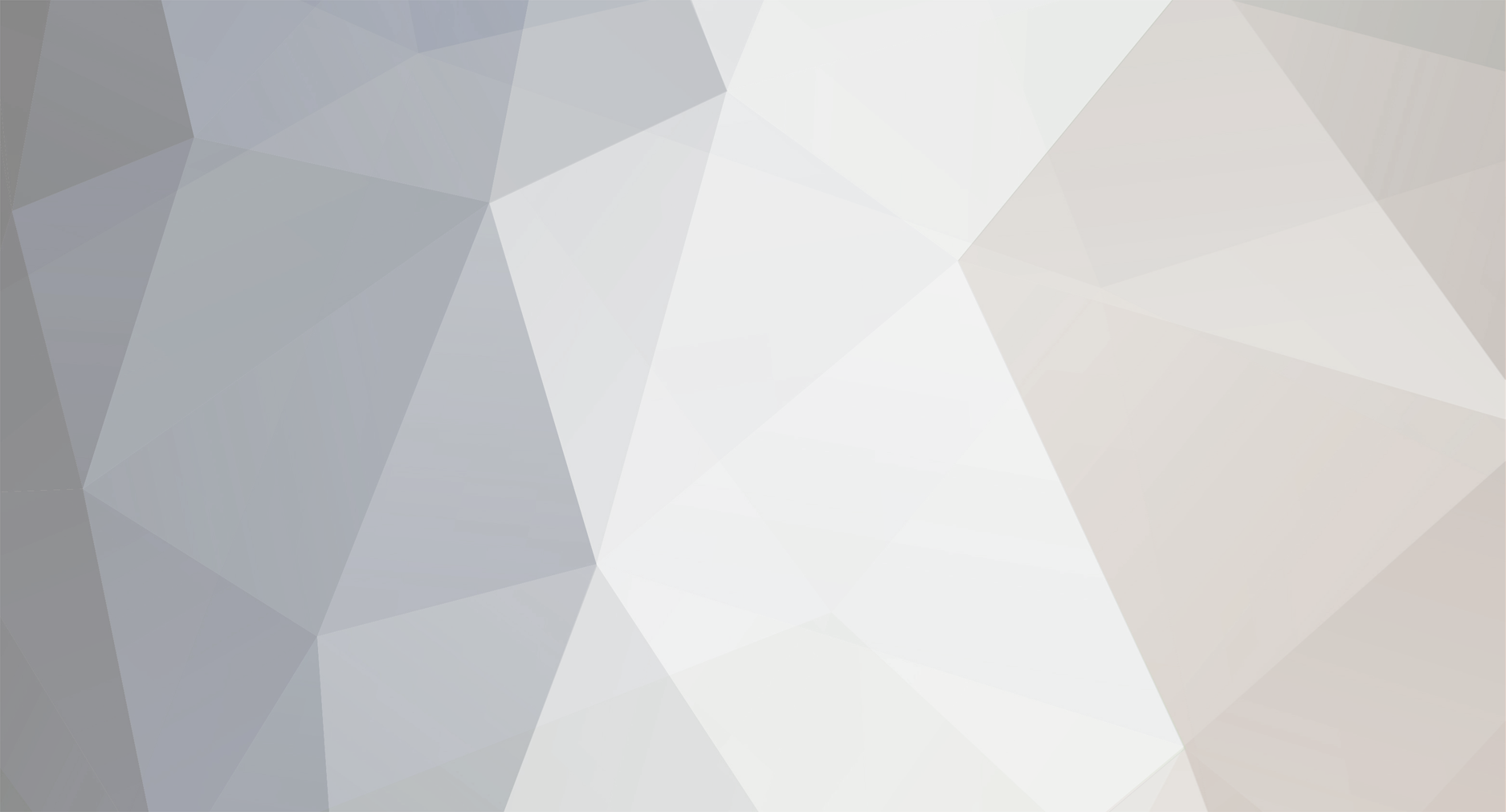
RopeADope
-
Posts
157 -
Joined
-
Last visited
Posts posted by RopeADope
-
-
Hi all. I've got a little function to generate a simple form depending on the input. What I'd like to do is alias the field names that get output to make them a little more human readable. However, I'm not entirely sure how to do that with the output that I get.
The function....
function build_form($table_name){ $result=mysql_query("SELECT * FROM $table_name"); $num=mysql_num_rows($result); $i=1; echo "<table>"; while ($i < mysql_num_fields($result)) { $fields=mysql_fetch_field($result,$i); echo "<tr><td>" . $fields->name . "</td><td><input type=\"text\" size=\"30\" name=\"" . $fields->name . "\" /></td></tr>"; $i++; }; echo "</table>"; };
Q: Where would I have to modify the output from mysql_fetch_field?
My modified function...
function build_form($table_name){ $result=mysql_query("SELECT * FROM $table_name"); $num=mysql_num_rows($result); $i=1; echo "<table>"; while ($i < mysql_num_fields($result)) { $fields=mysql_fetch_field($result,$i); $field_name=str_replace("_"," ",$fields->name); echo "<tr><td>" . $field_name . "</td><td><input type=\"text\" size=\"30\" name=\"" . $field_name . "\" /></td></tr>"; $i++; }; echo "</table>"; };
Not sure if that will work, but am I heading in the right direction? Any help is much appreciated!
-
Despite the hilarious title, I do have a real quandary. I'm currently using a MySQL db, myisam engine. I want to cascade CRUD's across several tables. My assumption is that I would use triggers to do that. Is this assumption correct?
OR
Would a procedure be better for cascading CRUD's across multiple tables?
EDIT: Minus the "R" from the acronym. I realize there's no point in cascading retrievals.....
-
You question is quite ambiguous,
so my answer is
$user = ""; $pass = ""; if(Auth::validate_login($user,$pass)){ header("Location: Failed.html"); }
What does the "::" do? Also, I plan to have a function under the Auth class that calls another class with a query method to check the $user and $pass against a database. Will I still be able to do that using your method?
-
Nobody? Really?
-
Great stuff, getting closer. I can round corners and change the shape of the box and everything, but what is the element that controls how the button looks? like if you wanted to change that button into a custom graphic like the one above. Say instead of using classes just to insert the rule so that ALL php elements of this type will look like this.
1) Define "looks".
2) If you put the <link> tag with all of it's proper attributes(i.e. <link rel="stylesheet" type="text/css" href="master.css" media="screen" />), you can copy and paste(or use php includes) to include the .css file on every page...in which case it'd apply your custom style to every page.
-
Yes, you should be able to alter it with CSS. There are several properties you could assign to the select and option tags to mimic the dropdown in the pic you posted. It may not be exactly the same, but you can get it pretty close. You'll wind up with a master.css(<-arbitrary name) file with some code in it that looks something like this...
select{ background-color:gray; font:10px arial; etc.... } option{ background-color:gray; font:10px arial; etc.... }
I'm not 100% sure off hand but you may even be able to leave the select tag alone and only do it with the option tags since they show by default. The select tag is more just a container so you don't have option tags all over the place.
Here's some links that should at least get you started:
http://www.outfront.net/tutorials_02/adv_tech/funkyforms5.htm
-
You should be able to modify the style via CSS. Just edit the select and li tags in the .css file.
-
The class:
class Auth{ function validate_login($user,$pass){ if(ctype_alnum($user)){ return true; }else{ return false; } } }
Q1: (new to OOPHP) As you can see, I just have it checking an input to make sure it contains only alphanumeric characters. My question is, how do I work with the returned result from the if() statement(if at all possible)? I basically want to have an automatic denial followed by a kickback to the login screen if the if() statement returns false. My assumption is I should have a class/function somewhere called "location" that I can call to redirect to different pages and such. Thougts?
-
@salath
-So could I modify the expression to simply be {^A-Za-z0-9} and forgo the quotes all together, or would it have to be "{^A-Za-z0-9}" ?
@cags:
-That function does exactly what I was looking for, lol. The overwhelming consensus from the search results I've seen is to use preg_match since ereg is deprecated. So ctype_alnum() would return false if any non-alphanumeric characters or a blank was detected?
-
My match statement
if(preg_match('"[^A-Za-z0-9]"', $user)){ echo "Your login contains invalid characters."; }
Q1: I couldn't get this to work until I put single AND double quotes around the expression. Why is that?
Q2: Please clarify my understanding of this statement if I'm wrong. "if $user contains characters other than what's in the expression, echo 'Your login contains invalid characters.'"
Q3: The end goal is to check a username from a $_POST variable and if it contains any non-alphanumeric characters, kickback to the login. Will preg_match catch characters like \n \r : ; etc.?
-
use jquery ..very simple
jQuery and other libraries are kind of my last choice. I realize it'd be easier, but what's the point of putting it on your own website if you can't do it yourself? I want to at least attempt to make the effect myself.
-
I have a problem that seems somewhat complicated. I have an simple mouseover/mouseout image swap on my site. What I'd like to have it do, is onmouseover(fade out src image) and onmouseout(fade in src image). I've seen a lot of code for doing swaps and such, but nothing quite like what I'm looking for. Can anyone provide a snippet to get me started or a link to a tutorial?(P.S. I hardly know any javascript...I can do mouseovers and that's about it)
-
Not at all
Plus it isn't my design, it was on some free wordpress template site
There is the problem, lol. This is just my checklist, some of it may have already been covered.
- Change Forum White Pages: A Forum Directory to an image. I assume its always going to say that so there's no need for text
- Upon visiting the page, it looks like one of those generic page holders
- IMO, you should change the inner div backgrounds to white, or a light gray, with white text. Light text on a dark background is almost always tough to read because of the staunch contrast
- The point of the site isn't obvious(I think someone noted this)
All in all, its functional...but unimpressive.
- Change Forum White Pages: A Forum Directory to an image. I assume its always going to say that so there's no need for text
-
on some of the pages like the car rentals where the countries are listed there is also way to much white-space
For the countries, my suggestions would be to put clickable images of the countries that have some minor change on mouseover. Or if you're feeling froggy, put in an image map of Africa, and have the clickable countries change on mouseover. IMO, I'd go with the image map. Slightly more work but the affect will be much stronger.
-
The white writing on the green health bar is blinding. I'd change the font for the header as well(Level 80 Human Mage), something a little more relative to the game maybe. Look into sIFR as well, it handles font smoothing. Last thing, the "Specific Stats" box drops around 15-20px when you click "Ranged", for the sake of consistency I'd suggest making it stay one fixed size. All in all, very nice site. Good colors, well proportioned, and the item mouseovers are very nice as well.
-
For the most part, I second xcandiottix's summary. My first suggestion would be if you're going to have a small site footprint, add a background image(that follows the theme) to break up all of the white space. The only other note I have is on the car rental section. I'm not sure I'd agree with red and yellow as that may somewhat painful to read. I'd suggest either a light gray/darker gray color alternation, or as xcandiottix suggested but using a light yellow/darker yellow alternation.
-
I indeed hit a wall. I'm not sure exactly what comments I am/was expecting but something to the tune of "you're on/off the right track". The bullet points I put are what I've surmised from looking around, but I wasn't sure if I was right on those points or if there were other big points I was missing.
The text, like I noted, is semi-pointless. Its really just so I can see how things will exactly fall. I plan on doing some marker style icons for my top bullet points, then maybe a word cloud for the sub points, and I need to throw in some color. I'll repost once I have some more time into it...then hopefully I can get a "pat on the head" haha.
-
In short, I'm AWFUL with designing my own sites. Designing sites for others I can do no problem, but I'm ridiculously critical of my personal site. So I did some research and looked at the commonalities of the websites of professional web designers and here's what I came up with...
- Big images
- Simple color schemes
- Less content, more space
This is what I've seen the most of per cssremix.com so that's what I've tried to mimic with my site. As the title suggests, there's only around an hour of coding into it including graphics. The words are semi-pointless, more there just to test the layout, and please ignore the hover events as I haven't taken the time to change them yet.
http://chad-scribner.com/sandbox/active/
SIDE NOTE: Is there a way to implement sIFR without being able to edit the .fma file that the documentation suggests? I'd really like to have nice clean writing on this but I have nothing to edit flash files with.
- Big images
-
Just a thought, I'm still a PHP novice, but what if you had the passwords in the database, and just temporarily granted access to certain directories based on their login credentials, that way you'd leave the htpasswd files in tact, but still allow users access. Basically have your application change directory permissions on login, then lock them down on logout(this may be less efficient but I'm positive). This would make your application the only method of accessing the files instead of possibly opening up holes via constant htpasswd modification.
On a side note, if you wanted to go the route of allowing users to mod their htpasswd files, I'd assume it'd be safe as long as the traffic is encrypted and the update method was failsafe(e.g. trim unneeded chars, etc).
-
Ok here's my simplified task...connect and select a db. My thought is that connect and select should be in the same class because you wouldn't need to connect to MySQL and not do anything, so selecting a db would be inherent. With that said, should the connect and select functions be combined into one?
<?php class connect { var $host; var $dbuser; var $dbpass; var $db; //Set variables for db connection function connect($host,$dbuser,$dbpass) { $this->host=$host; $this->dbuser=$dbuser; $this->dbpass=$dbpass; mysql_connect($host,$dbuser,$dbpass)or die('No connection'); } function select($db) { $this->db=$db; mysql_select_db($db)or die('No database selected'); } } ?>
-
You might want to at least get a grasp of functional programming before eve attempting OOP.
The classes connect() & clean_input syntactically incorrect and will not function.
auth_user() should probably just return true or false. Making it redirect to specific pages makes it completely tied to the current application.
Yeah I'm realizing that now...I need to start simpler. I understand the concept(I think). A class is a blueprint that has properties and the methods work with those properties(right?). I'm going to give it another go but make it much simpler...
-
Wouldn't it be easier to set up the usernames and passwords in a database?
-
Ok not sure if I made it better or worse but here it is.
<?php class auth { var $username; var $password; function auth_user($username,$password){ $sql=mysql_query("SELECT * FROM users WHERE username='$username' AND password='$password'"); $result=mysql_result($sql); $num=mysql_num_rows($result); if($num==1) { header('Location:main.php'); }else{ header('Location:index.php'); }; }; }; class connect{ $host="localhost"; $dbuser="main_user"; $dbpass="password"; $dbname="main_db"; mysql_connect($host, $dbuser, $dbpass)or die('Error establishing connection!'); mysql_select_db($dbname)or die('Error connecting to the database!'); } class clean_input{ $regex="'/^[A-Za-z0-9]+$/'"; //Expression to match $repex=""; //Replace unmet characters with nothing, i.e. remove them from the string preg_replace($regex, $repex, $username); preg_replace($regex, $repex, $password); } ?>
-
Cleaning user input & making a database connection has nothing at all to do with user authentication and should be in there own classes.
Your auth_user() method is completely floored. mysql_result() returns either a result resource if the query succeeds (this is not the same as finding a result) or false otherwise. It will never return 1.
Gotcha. I'll revise and re-post.
Get/display data
in PHP Coding Help
Posted
Hi all. The following is a function to get and display data. However, I've hit a wall in my progress. It's only printing out one field. I need to have it print out every retrieved field. Any help is appreciated.
I realized that (I think) I need the $display_fields var to be concatenated with the $i variable so there's a unique var name for each field retrieved from the mysql_fetch_field line. Any ideas?