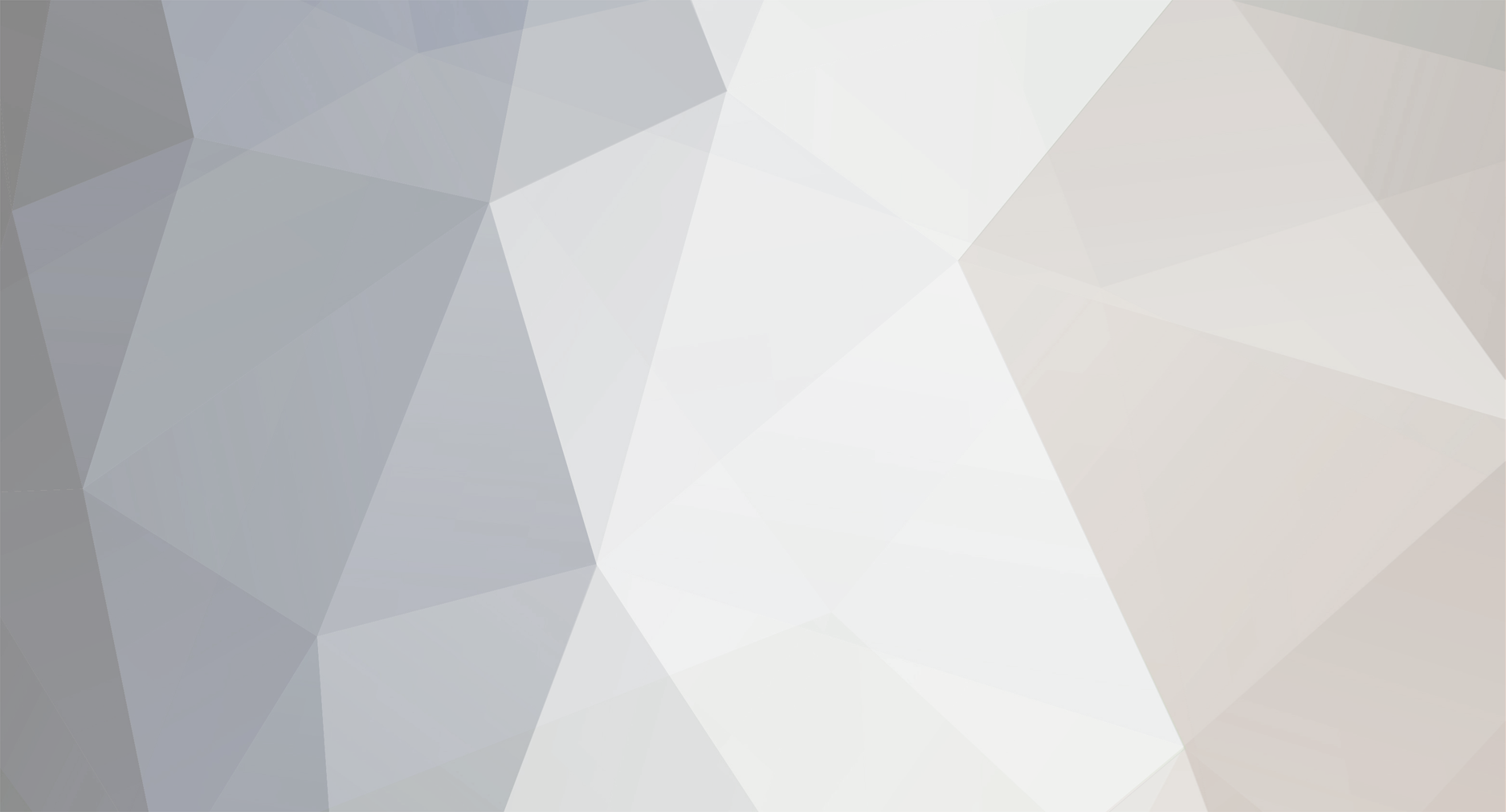
GetPutDelete
-
Posts
70 -
Joined
-
Last visited
Never
Posts posted by GetPutDelete
-
-
Ok, you're trying to get the contents of a variable (URL variables are listed after the question mark (?) and separated by (&). To echo these variables use
<?php echo $_GET['page']; ?>
An example using multiple variables in the URL...
(URL is http://example.com/?my_name=bob&fav_color=blue&age=23)
<?php echo 'My name is ' . $_GET['my_name'] . ' my favourite color is ' . $_GET['fav_color'] . ' and I am ' . $_GET['age'] . ' years old.'; ?>
I hope that helps.
-
For apostrophes when you insert the data to the database you should use mysql_real_escape_string() http://php.net/manual/en/function.mysql-real-escape-string.php This will add slashes to the quotes ' and " to make them database friendly, strings will then be displayed like (Hello, GetPutDelete\'s awesome!) Then when you output the string use stripslashes() http://php.net/manual/en/function.stripslashes.php to remove the slashes to output (Hello, GetPutDelete's awesome).
-
Use the function nl2br() http://php.net/manual/en/function.nl2br.php On the form data when you save it to the database. This converts the new lines in the from the textarea to HTML BR tags so that when it comes out of the database the page displays the new line correctly.
-
Do you mean getting the user to login to their email through your site and then grabbing the contents of their address book?
If so and you want to write it yourself then look no further than some IMAP classes, if you don't then I think I came across one a while back after a google search, but if I remember correctly they charged for their service. Personally I'd just write it myself.
-
1. Look at the parameters under "What" http://php.net/manual/en/function.phpinfo.php This enables you to specify what you would like to have returned from phpinfo()
-
As far as I know that's not possible with phpMA alone. Now if you were writing a script to assign AI values then that would be different... Sorry dude, hope someone else has heard of a way.
-
Click "Empty" and it will clear the table and also reset the increment.
-
No problem, don't forget to set the thread to solved.
-
Headers are designed to work before there is any output on the site. The error you are getting is typical of there being HTML before the header function. You should check that PHP isn't echo'ing anything prior to this (that includes PHP error messages too!).
-
If you're looking to specify a special favicon location in the HEAD then just do it the same way you would in HTML
<?php echo '<head>...
-
Are you sure the database login details are correct?
-
Here you go...
<?php if(isset($_POST['user']) && isset($_POST['vanaparool']) && isset($_POST['uusparool'])) { $username = $_POST['user']; $password = $_POST['vanaparool']; $password2 = $_POST['uusparool']; $con = mysql_connect("localhost","baasiksutaja","parool"); if (!$con) { die('Could not connect: ' . mysql_error()); } // vastus mysql_select_db(baasinimi); $uuendamine = mysql_query("SELECT * FROM testtable WHERE (username='$username' AND password='$password')"); while($row = mysql_fetch_array($uuendamine)) { echo "Your " . $row['username'] . " password is updated!" ; } // uuendamine mysql_select_db(baasinimi); mysql_query("UPDATE testtable set password='$password2'"); mysql_close($con); } ?> <form method="post" action=""> <table width="80%" border="0" align="center" cellpadding="3" cellspacing="3" class="forms"> <tr> <td width="31%">Username:</td> <td width="69%"><input name="user" type="text" id="user"></td> </tr> <tr> <td width="31%">Old password:</td> <td width="69%"><input name="vanaparool" type="password" id="vanaparool"></td> </tr> <tr> <td>New password:</td> <td width="69%"><input name="uusparool" type="password" id="uusparool"></td> </tr> </table> <p align="center"> <input name="uuenda" type="submit" id="uuenda" value="Update"> </p> </form>
The form now posts to itself. When the page loads again it checks for POST data and if there is some it runs the code to update the users details.
-
Where do you want them to be redirected to? You could just check in the database that a user with that email address and key exists, and if not bring up a message saying there was an error.
-
I'm a little confused with what you are asking, do you want to POST the form to the same page and have the same page process the data?
-
Look at the link in my second post.
-
No because all you need to do is have a cleanup script run each time a user loads the page. So the last user leaves and the chat is now empty, now when the next user comes on the script determines that he too hasn't been active for over 10 seconds and so removes him from the db. This all should happen prior to the page being displayed so that once the house keeping is complete the new user gets an accurate view of what's going on in the chat.
Anyway that's enough PHPing for me tonight, good luck with your project.
-
The problem you have though is getting the script to execute once the user has left the chat in order to delete the data from the db. Really the only way to be able to maintain accurate info on who is in chat and who is not is to use the ajax example I gave you.
The problem with your last idea is that once the user has left no script has been executed in order to bring the db up to date.
-
Ok no problem, no not like that. What is the name of the file that takes the HTML from the editor that your parents use and then edits the existing HTML file with those changes? And if you paste the code in here I'll do it for you.
-
Take a look at the zip library I sent you.
-
<?php if(mysql_num_rows(mysql_query("select * from players where player_id='" . $_SESSION['player_id'] . "' and player_captain='player' limit 0, 1")) == 1) { //Redirect to player site header('Location: example.com'); } ?>
And do the same for the captain one as well.
-
Correct, if the user keeps their other windows open and closes the chat window then the ajax is no longer updating the database, therefore the user gets labelled as being out of the chat.
-
When I said "should" I mean you should develop it to do that.
-
Unless you have PHP set to expire sessions after a certain amount of time the user should never get signed out unless the window is closed. If you did get signed out the ajax chat should kick in to display a message with a link prompting the user to refresh the page.
-
It's displaying the last result because the query is overwriting the variables each time, to get around this you could turn the variables into an array, for example $role[1] = bla, $position[1] = bla ect, then for the next row $role[2] = bla... ect.
That way after the loop is finished you will have each row and its data assigned to an array.
Free address lookup API based on postcode?
in Other Libraries
Posted
Questions in the title really, has anyone heard of one?