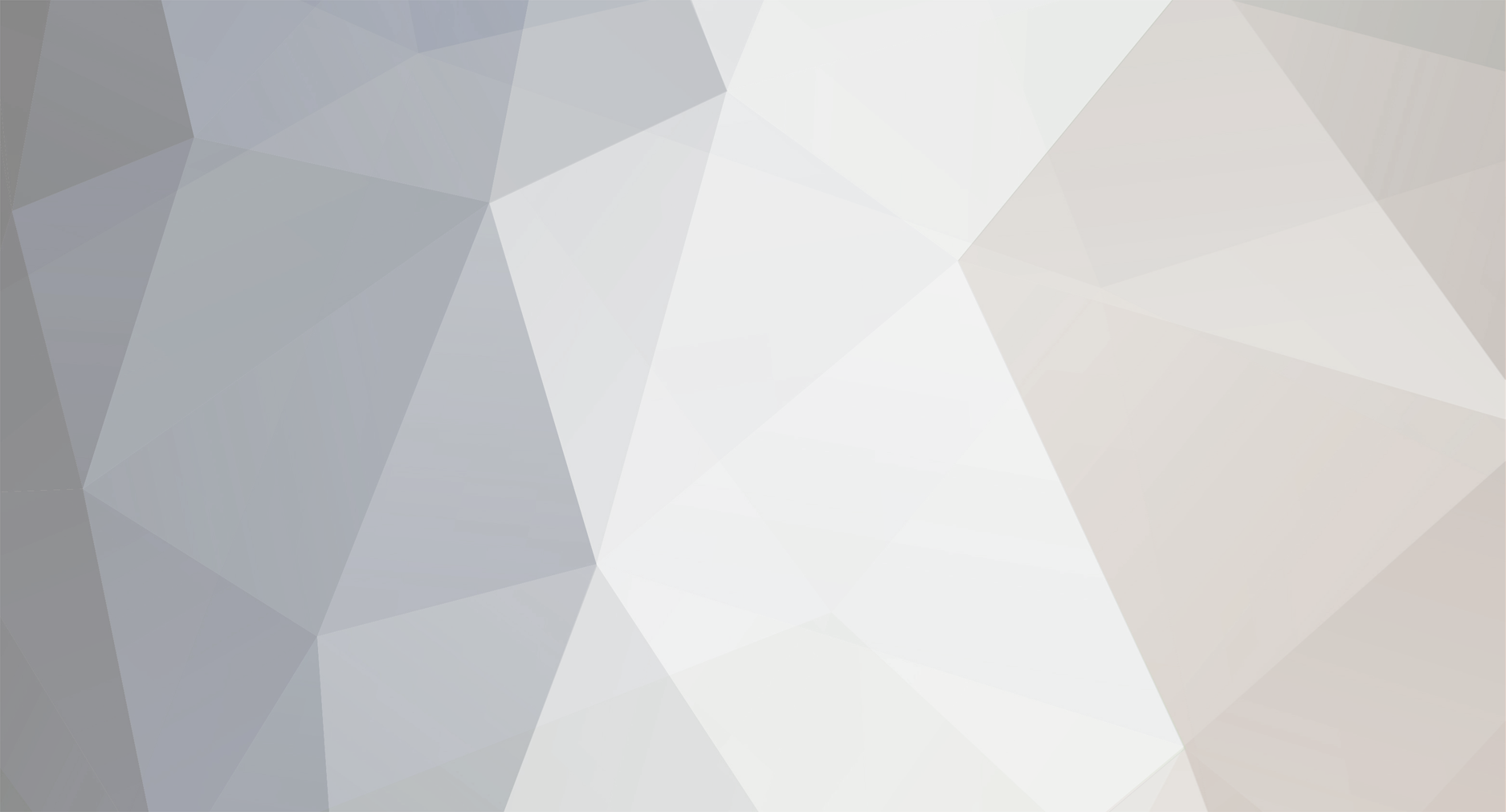
jcbones
-
Posts
2,653 -
Joined
-
Last visited
-
Days Won
8
Posts posted by jcbones
-
-
Try this, and let me know.
<html> <head> </head> <body> <?php // Define db details //db details mysql_connect(DB_HOST, DB_USER, DB_PASSWORD) or trigger_error(mysql_error()); mysql_select_db(DB_DATABASE) or trigger_error(mysql_error()); $tbl_name="prospects"; //your table name // How many adjacent pages should be shown on each side? $adjacents = 3; /* First get total number of rows in data table. If you have a WHERE clause in your query, make sure you mirror it here. */ $query = "SELECT COUNT(*) as num FROM $tbl_name"; $total_pages = mysql_fetch_array(mysql_query($query)); $total_pages = $total_pages['num']; /* Setup vars for query. */ $targetpage = "view_prospect.php"; //your file name (the name of this file) $limit = 5; //how many items to show per page $page = (isset($_GET['page'])) ? (int)$_GET['page'] : 1; $start = ($page - 1) * $limit; //first item to display on this page $queryLimit = 'LIMIT ' . $start . ',' . $limit; /* Get data. */ // $sql = "SELECT prospect_id FROM $tbl_name LIMIT $start, $limit"; // $result = mysql_query($sql); /* Setup page vars for display. */ if ($page == 0) $page = 1; //if no page var is given, default to 1. $prev = $page - 1; //previous page is page - 1 $next = $page + 1; //next page is page + 1 $lastpage = ceil($total_pages/$limit); //lastpage is = total pages / items per page, rounded up. $lpm1 = $lastpage - 1; //last page minus 1 /* Now we apply our rules and draw the pagination object. We're actually saving the code to a variable in case we want to draw it more than once. */ $pagination = ""; if($lastpage > 1) { $pagination .= "<div class=\"pagination\">"; //previous button if ($page > 1) $pagination.= "<a href=\"$targetpage?page=$prev\">« previous</a>"; else $pagination.= "<span class=\"disabled\">« previous</span>"; //pages if ($lastpage < 7 + ($adjacents * 2)) //not enough pages to bother breaking it up { for ($counter = 1; $counter <= $lastpage; $counter++) { if ($counter == $page) $pagination.= "<span class=\"current\">$counter</span>"; else $pagination.= "<a href=\"$targetpage?page=$counter\">$counter</a>"; } } elseif($lastpage > 5 + ($adjacents * 2)) //enough pages to hide some { //close to beginning; only hide later pages if($page < 1 + ($adjacents * 2)) { for ($counter = 1; $counter < 4 + ($adjacents * 2); $counter++) { if ($counter == $page) $pagination.= "<span class=\"current\">$counter</span>"; else $pagination.= "<a href=\"$targetpage?page=$counter\">$counter</a>"; } $pagination.= "..."; $pagination.= "<a href=\"$targetpage?page=$lpm1\">$lpm1</a>"; $pagination.= "<a href=\"$targetpage?page=$lastpage\">$lastpage</a>"; } //in middle; hide some front and some back elseif($lastpage - ($adjacents * 2) > $page && $page > ($adjacents * 2)) { $pagination.= "<a href=\"$targetpage?page=1\">1</a>"; $pagination.= "<a href=\"$targetpage?page=2\">2</a>"; $pagination.= "..."; for ($counter = $page - $adjacents; $counter <= $page + $adjacents; $counter++) { if ($counter == $page) $pagination.= "<span class=\"current\">$counter</span>"; else $pagination.= "<a href=\"$targetpage?page=$counter\">$counter</a>"; } $pagination.= "..."; $pagination.= "<a href=\"$targetpage?page=$lpm1\">$lpm1</a>"; $pagination.= "<a href=\"$targetpage?page=$lastpage\">$lastpage</a>"; } //close to end; only hide early pages else { $pagination.= "<a href=\"$targetpage?page=1\">1</a>"; $pagination.= "<a href=\"$targetpage?page=2\">2</a>"; $pagination.= "..."; for ($counter = $lastpage - (2 + ($adjacents * 2)); $counter <= $lastpage; $counter++) { if ($counter == $page) $pagination.= "<span class=\"current\">$counter</span>"; else $pagination.= "<a href=\"$targetpage?page=$counter\">$counter</a>"; } } } //next button if ($page < $counter - 1) $pagination.= "<a href=\"$targetpage?page=$next\">next »</a>"; else $pagination.= "<span class=\"disabled\">next »</span>"; $pagination.= "</div>\n"; } function findColor($n) { return ($n % 2 == 0) ? '#0026FF' : '#0094FF'; } // Create function to display the news entries function print_prospectEntry($arrEntry) { // convert \n linebreaks to HTML formatted <br> breaks $arrEntry['comments'] = str_replace("\n", '<br>', $arrEntry['comments']); $i = 1; ?> <table width="100%" border="0" cellspacing="0" cellpadding="0"> <tr style="background-color:<?php echo findColor($i++); ?>"> <td width="10"></td> <td width="300">Representative:</td> <td style="padding: 5px;"> <?php echo $arrEntry['representative']; ?> </td> </tr> <tr style="background-color:<?php echo findColor($i++); ?>"> <td width="10"></td> <td width="300">Company Name:</td> <td style="padding: 5px;"> <a href="prospect.php?prospect_id=<?php echo $arrEntry['prospect_id']; ?>"><?php echo $arrEntry['company_name']; ?></a> </td> </tr> <tr style="background-color:<?php echo findColor($i++); ?>"> <td width="10"></td> <td width="300">Phone Number:</td> <td style="padding: 5px;"> <?php echo $arrEntry['phone']; ?> </td> </tr> <tr style="background-color:<?php echo findColor($i++); ?>"> <td width="10"></td> <td width="300">First Name:</td> <td style="padding: 5px;"><?php echo $arrEntry['firstname']; ?> </td> </tr> <tr style="background-color:<?php echo findColor($i++); ?>"> <td width="10"></td> <td width="300">Last Name:</td> <td style="padding: 5px;"> <?php echo $arrEntry['lastname']; ?> </td> </tr> <tr style="background-color:<?php echo findColor($i++); ?>"> <td width="10"></td> <td width="300">Last Contacted:</td> <td style="padding: 5px;"> <?php echo $arrEntry['last_contact']; ?> </td> </tr> <tr style="background-color:<?php echo findColor($i++); ?>"> <td width="10"></td> <td width="300">Comments:</td> <td style="padding: 5px;"> <?php echo $arrEntry['comments']; ?> </td> </tr> </table> <br> <br> <?php } // Get all news entries $prospectEntries = mysql_query('SELECT * FROM prospects ORDER BY prospect_id DESC ' . $queryLimit) or trigger_error(mysql_error()); while ($prospectEntry = mysql_fetch_array($prospectEntries)) { // Your while loop here print_prospectEntry($prospectEntry); // when printing here, it does 5 results per page like I want, however it just prints the same record //echo $row; } ?> <?php echo $pagination; ?> </body> </html>
-
I would put an insert query on the thank you page. This would be on a table called printed_coupons. 2 fields, coupon_id and user.
$sql = "INSERT INTO `printed_coupons`(`coupon_id`,`user`) VALUES ('$id','$user')"; mysql_query($sql);
at the top of the print page, I would put this.
<?php $sql = "SELECT `coupon_id` FROM `printed_coupons` WHERE `coupon_id` = '$coupon_id' AND `user` = '$user'"; $result = mysql_query($sql); if(mysql_num_rows($result) > 0) { die('You have already printed this coupon'); } ?>
-
That error means your query failed.
-
Clickatell is down, and has been for about a month. None of my SMS is getting through. I'm getting ID's, but the service is returning routing errors. All they will say is "Shared short code services will be restored shortly".
Just saying, you may want to try a different service.
-
<?php define("STR_RELATIVE_PATH", ""); require STR_RELATIVE_PATH . "_includes/_database.php"; db_connect(); db_select(); $strQuery //Rename column to the column you are searching, and the value is self explanatory. = "SELECT * FROM `tblServices` WHERE `column` = 'value'"; //You don't need an ORDER BY if you are only pulling one row. $queryGetServices = db_query($strQuery); ?>
-
If you are really worried about the "Drop table", then deny "drop" to the database.
For a public site, I usually only allow INSERT, SELECT, and UPDATE. DELETE is for admin's only, and works on a seperate mysql user and password.
-
One last hint:
You are using a black background:
if (isset($_POST['header'])) { $header = intval($_POST['header']); echo '<span style="color:white;">You choose: ' . $header . '</span>; // outputs: 1, 2 or 3 //.. }
-
class link_parser { function __construct() { $this->debug = new Debug(); } }
Edit: Proof reading, makes you less of an idiot. :doh:
-
I first thought maybe your server had globals turned off, so the variables were always empty.
Then I saw what all of us missed. (!calc) is not defined or set, so it will always validate to false.
I went ahead, and defined the variables also, and since I did that, I'll go ahead and give it to you also.
<html> <body> <?php if (isset($_POST['submit'])) { $score_1 = (isset($_POST['score_1'])) ? $_POST['score_1'] : ''; $score_2 = (isset($_POST['score_2'])) ? $_POST['score_2'] : ''; $score_3 = (isset($_POST['score_3'])) ? $_POST['score_3'] : ''; if (($score_1 == "") || ($score_2 =="") || ($score_3 =="")) { echo("<font face=\"Tahoma\" size=\"2\" color=\"#FF0000\"><b>Oops, you forgot to supply some information. Please correct the problem below.</b></font><br>"); } elseif ((!ereg("[0-9]",$score_1)) || (!ereg("[0-9]",$score_2) || (!ereg("[0-9]",$score_3)))) //missing 4 )'s in this statement. { echo("<font face=\"Tahoma\" size=\"2\" color=\"#FF0000\"><b>Please restrict your input to only numbers.</b></font><br>"); } else { $avg=($score_1 + $score_2 + $score_3)/3; //missed semi-colon; if ($avg >= 29) { echo $total = 0; //missed semi-colon; } else { $total = ((29 - $avg) * (7/10)); //didn't need the echo here, just the variable. } print("The freegumpher handicap is:". $total); //removed bracket } from here. } } ?> <form method="post" action=""> Enter your three most <i>recent</i> Freegumpher scores to calculate Handicap:<br/> <br/> <input type="text" name="score_1" size=8 <input type="text" name="score_2" size=8 <input type="text" name="score_3" size=8 /><br /><br/> <input type="submit" name="submit" value="Calculate Handicap"/> </form> </body> </html>
-
If you would like, I have an upload function that also makes sure the physical image size is correct, and to your settings. It returns the image if everything worked, and an error if it doesn't.
I've included it, just in case you might want to use it. I went ahead and put in a mysql connection, and a query. You must edit this portion to work with your setup, or take it out if you already have that part.
function uploadImage($setWidth, $setHeight) { $filepath = 'upload/'; //edit this for your file path. $content = NULL; if($_FILES["file"]["size"] > 900000) { $content = "*File is to large, submit a smaller image!"; } elseif(empty($_FILES["file"]["name"])) { $content = "*No File Uploaded!"; } else { if ((($_FILES["file"]["type"] == "image/gif") || ($_FILES["file"]["type"] == "image/jpeg") || ($_FILES["file"]["type"] == "image/pjpeg") || ($_FILES["file"]["type"] == "image/png") || ($_FILES["file"]["type"] == "image/jpg") || ($_FILES["file"]["type"] == "image/x-png")) && ($_FILES["file"]["size"] < 900000)) { $image = str_replace(' ', '_', $_FILES['file']['name']); if ($_FILES["file"]["error"] > 0) { $content = "*Return Code: " . $_FILES["file"]["error"] . "<br />"; } elseif (file_exists($filepath . $image)) { $content .= '*Image exists: ' . $image ; } else { $uploadedfile = $_FILES["file"]["tmp_name"]; $size = getimagesize($uploadedfile); $type = $size['mime']; $width = $size[0]; $height = $size[1]; $filename = $filepath.$image; if($height > $setHeight || $width > $setWidth) { $newwidth=$setWidth; $newheight=($height/$width)*$setWidth; $tmp=imagecreatetruecolor($newwidth,$newheight); if($size[2] == IMAGETYPE_GIF) { $src = imagecreatefromgif($uploadedfile); imagecopyresampled($tmp,$src,0,0,0,0,$newwidth,$newheight,$width,$height); imagegif($tmp,$filename,100); } elseif($size[2] == IMAGETYPE_JPEG) { $src = imagecreatefromjpeg($uploadedfile); imagecopyresampled($tmp,$src,0,0,0,0,$newwidth,$newheight,$width,$height); imagejpeg($tmp,$filename,100); } elseif($size[2] == IMAGETYPE_PNG) { $src = imagecreatefrompng($uploadedfile); imagecopyresampled($tmp,$src,0,0,0,0,$newwidth,$newheight,$width,$height); imagepng($tmp,$filename,9); } $content .= "*Image has been resized to $newwidth X $newheight<br/>"; imagedestroy($src); imagedestroy($tmp); } else { move_uploaded_file($uploadedfile, $filename); } return $filename; } } else { $content .= "*Invalid file"; } } return $content; } define("DB_SERVER", "localhost"); define("DB_USER", "root"); define("DB_PASS", ""); define("DB_NAME", "test"); mysql_connect(DB_SERVER, DB_USER, DB_PASS); mysql_select_db(DB_NAME); if(isset($_POST['submit'])) { $image = uploadImage(150,150); if(substr($image,0,1) != '*') { $sql = "UPDATE `table` SET `image` = '$image' WHERE `user` = '$username'"; if(mysql_query($sql)) { echo 'Profile image updated!'; } else { echo 'Profile image failed to update!'; } } else { echo $image; } }
-
You are wanting this input hidden correct?
<input type="hidden" name="Report_Message" id="Report_Message" value="<?php echo $row['Report_Message']; ?>" /> <!-- OR --> <input style="display:none;" type="text" name="Report_Message" id="Report_Message" value="<?php echo $row['Report_Message']; ?>" />
-
Cleaned up all parse errors.
<html> <body> <?php if ($submit) { if (($score_1 == "") || ($score_2 =="") || ($score_3 =="") || (!$calc)) { echo("<font face=\"Tahoma\" size=\"2\" color=\"#FF0000\"><b>Oops, you forgot to supply some information. Please correct the problem below.</b></font><br>"); } elseif ((!ereg("[0-9]",$score_1)) || (!ereg("[0-9]",$score_2) || (!ereg("[0-9]",$score_3)))) //missing 4 )'s in this statement. { echo("<font face=\"Tahoma\" size=\"2\" color=\"#FF0000\"><b>Please restrict your input to only numbers.</b></font><br>"); } } else { $avg=($score_1 + $score_2 + score_3)/3; //missed semi-colon; if ($avg >= 29) { echo $total = 0; //missed semi-colon; } else { $total = ((29 - $avg) * (7/10)); //didn't need the echo here, just the variable. } print("The freegumpher handicap is:". $total); //removed bracket } from here. } ?> <form method="post" action="freegumph.php"> Enter your three most <i>recent</i> Freegumpher scores to calculate Handicap:<br/> <br/> <input type="text" name="score_1" size=8 <input type="text" name="score_2" size=8 <input type="text" name="score_3" size=8 /><br /><br/> <input type="submit" name="submit" value="Calculate Handicap"/> </form> </body> </html>
Now, the function ereg is depreciated, and will no longer be supported in php6. You will be fine, as long as you don't update to that version.
-
http://dev.mysql.com/doc/refman/5.1/en/adding-users.html
I'm correct in that this is user accounts for the database, and not for a website, or other program?
If so, you will need root access to the database (which you aren't likely to get on a shared server).
-
Hidden form field
<input type="hidden" name="Report_Message" id="Report_Message" value="<?php echo $row['Report_Message']; ?>" />
Or
<form action="whatever.php"> <div style="display:none;"> <input type="text" name="Report_Message" id="Report_Message" value="<?php echo $row['Report_Message']; ?>" /> </div> <input type="submit" value="Submit"> </form>
-
I'm not real sure how your filing structure is laid out. You could generate a new folder for each user, then save the image inside of that. You would know the file path is the users name. This style is an easy solution, but only if you don't have 100's of users. As a lot of different users would bloat the file system.
My suggestion would be to create a directy for profiles inside of your current image directory, then use the users name as the image name. This way, if they uploaded a new file, it would overwrite the old file, and in doing so would help with the housekeeping. If you do it this way, you would only need to know the image name, and you have that, it is the users name. The file path would be the same for every user, so you know what that is to.
The sql then becomes very easy to do.
<?php $sql = "UPDATE `user` SET `image` = '$image' WHERE `name` = '$username'"; ?> //example of image tag. <img src="http://mysite.com/images/profiles/<?php echo $user; ?>.jpg" alt="user profile image" />
-
Number 1. You are not saving your image to a file. Maybe you don't want to, but there is no reason to upload if you aren't going to do that.
Number 2. You are outputting binary code without telling the browser what it is. As the browser only displays things as it is told to do so, it will just display the raw binary data.
Try this: (Un-tested)
<?php if( isset($_POST['submit']) ) { header('Content-type: image/jpeg'); include('SimpleImage.php'); $image = new SimpleImage(); $image->load($_FILES['uploaded_image']['tmp_name']); $image->resizeToWidth(150); $image->output(); } else { ?> <form action="upload.php" method="post" enctype="multipart/form-data"> <input type="file" name="uploaded_image" /> <input type="submit" name="submit" value="Upload" /> </form> <?php } ?>
-
Not unless you want a lot of tables.
while($condition) { //this loop will run until the condition is met //inserting tables inside this loop will create //a new table each time the loop runs. }
BTW, <<<Print is a heredoc, and doesn't actually print to the page. http://www.phpf1.com/tutorial/php-heredoc-syntax.html
-
Export your database into CSV files (1 table per file).
Edit: Upload file to the server.
Use this command to import.
LOAD DATA INFILE 'table.csv' REPLACE INTO TABLE `table` FIELDS TERMINATED BY ',' IGNORE 1 LINES
-
Very limited info, gets very limited help.
file.php
$sql = "SELECT * FROM `table` WHERE `timestamp` = NOW()"; $result = mysql_query($sql); if(mysql_num_rows($result) > 0) { while($r = mysql_fetch_assoc($result)) { //Select data, then use: } //then mail. mail($to,$subject,$message,$headers); }
crontab quick-reference http://adminschoice.com/crontab-quick-reference
0 * * * * curl --silent --compressed http://mysite.com/file.php
-
This should get you started.
$dbhost = 'localhost'; $dbuser = '***************'; $dbpass = '***************'; $dbname = 'OnlineSurvey'; $tableval = 'survey'; $conn = mysql_connect($dbhost, $dbuser, $dbpass) or die ('Error connecting to database'); mysql_select_db($dbname); @mysql_select_db("$dbname") or die("Error: Unable to select database"); $query="SELECT * FROM tableval"; $result=mysql_query($query); echo '<TABLE CELLPADDING="0" CELLSPACING="0" BORDER="0" WIDTH="800">'; while($row=mysql_fetch_array($result)){ $fname=$row[fullname]; $gender=$row[gender]; $answer1=$row[answer1]; $answer2=$row[answer2]; $answer3=$row[answer3]; $answer4=$row[answer4]; $answer5=$row[answer5]; $answer6=$row[answer6]; $answer7=$row[answer7]; $answer8=$row[answer8]; $answer9=$row[answer9]; $comments=$row[comments]; echo <<<Print <TR> <TD><b>Respondent's Name:</b> {$fname}</TD> </TR> <TR> <TD> </TD> </TR> <TR> <TD><b>Respondent's Gender:</b> {$gender}</TD> </TR> <TR> <TD> </TD> </TR> <TR> <TD><b>Answers</b></TD> </TR> <TR> <TD><b>1</b> {$answer1}</TD> </TR> etc. Print; } echo '</table>';
My first function
in PHP Coding Help
Posted