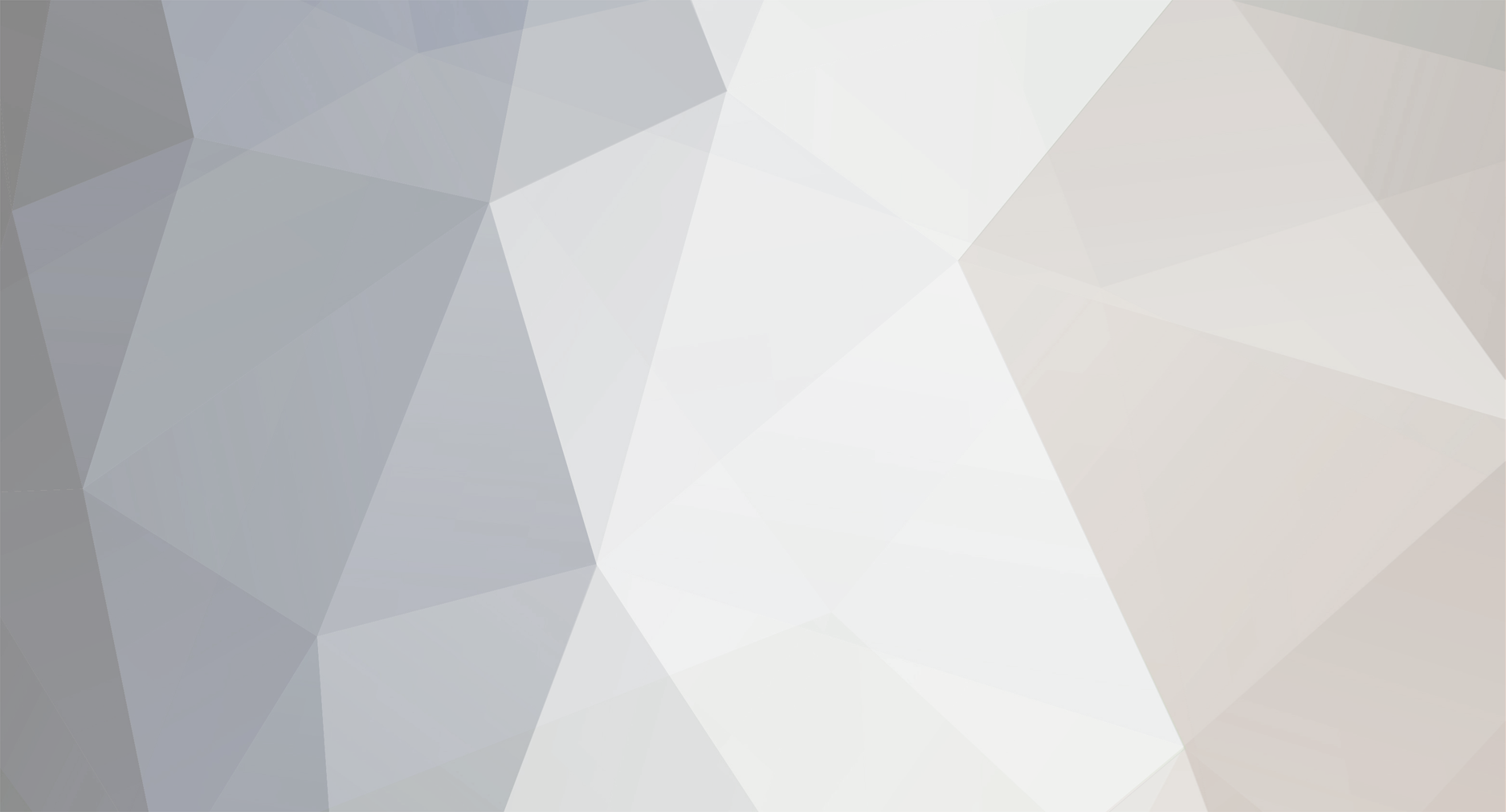
An7hony
Members-
Posts
79 -
Joined
-
Last visited
Everything posted by An7hony
-
thanks i'll give it a try
-
The user will click on the first button (.Applybutton) which opens a bootstrap modal with the ID ApplyModal. From then on an apply button (.SendButton) will send the job id that is passed in the first button press $_POST['jobid'] to the database. The problem: The JSON is displaying correctly in the console however the $_POST['jobid'] data is being ignored in the second button press on the modal when inserting into the database. $_POST['send'] is just being used to recognise the button press. //Open modal button pressed $(".Applybutton").click(function() { var element = $(this); var ID = $(this).attr("id"); var dataString = 'jobid='+ ID; $.ajax({ type: "POST", dataType: "json", url: "actions/ApplyData.php", data: dataString, cache: false, success: function(data) { console.log(JSON.stringify(data)); $('#ApplyModal').modal('show'); } }); return false; }); //Send application button pressed on modal $(".SendButton").click(function() { var dataString = 'send=101'; $.ajax({ type: "POST", dataType: "json", url: "actions/ApplyData.php", data: dataString, cache: false, success: function(data) { console.log(JSON.stringify(data)); } }); return false; }); ApplyData.php <?php session_start(); header('Content-type: application/json'); include '../includes/connection.php'; $candidateEmail = mysqli_real_escape_string($con, $_SESSION['email']); $covering_letter = mysqli_real_escape_string($con, $_POST['coveringletter']); $response_object = array('status' => 'success'); if (isset($_POST['jobid'])) { $message = $_POST['jobid']; } if (isset($_POST['send'])) { $message = "Successfully Applied."; $querySend = mysqli_query($con, "INSERT INTO applied_jobs (candidate_email, job_id) VALUES ('$candidateEmail','".$_POST['jobid']."')"); } $response_object['message'] = $message; print json_encode($response_object); ?> Any ideas?
-
Below is an example of how the script used to work. The values were stored in a cell like: 6, 7, 5, 8 but know they are stored like: id | people_id | people_interests | --------------------------------------------- 1 | 44 | 2 | 2 | 44 | 3 | How do i change this to show checked values from the new table structure: <?php $query="select people_interests from People where people_id='{$_GET['id']}'"; $row=mysql_fetch_object(mysql_query($query)); $interests_array=split(",",$row->people_interests); $qt=mysql_query("SELECT interest_no, interest FROM Interests"); $checked=""; while($interests=mysql_fetch_array($qt)){ if(in_array($interests['interest_no'],$interests_array)){$checked="checked";} else{$checked="";} echo "<label class=checkboxclm><input type=checkbox name=people_interests[] value='$interests[interest_no]' $checked> $interests[interest]</label>"; } ?> Thanks!
-
I am using datatables.net and want to include a SUM() on this query below: $sQuery = " SELECT SQL_CALC_FOUND_ROWS * FROM (SELECT p.people_id, p.people_firstName, p.people_surname, p.people_address, p.people_town, p.people_county, p.people_postcode, SUM(r.relationships_id) FROM People p LEFT JOIN Relationships r ON p.people_id = r.people_id) sel $sWhere $sOrder $sLimit "; This is only returning one row (should return around 2k records in the database) I have tried adding GROUP BY $sQuery = " SELECT SQL_CALC_FOUND_ROWS * FROM (SELECT p.people_id, p.people_firstName, p.people_surname, p.people_address, p.people_town, p.people_county, p.people_postcode, SUM(r.relationships_id) FROM People p LEFT JOIN Relationships r ON p.people_id = r.people_id GROUP BY r.relationships_id) sel $sWhere $sOrder $sLimit "; Although this is throwing a JSON format error Below is my full code: <?php $aColumns = array('people_id', 'people_firstName', 'people_surname', 'people_address', 'people_town', 'people_county', 'people_postcode', 'relationships_id', 'people_id'); /* Indexed column (used for fast and accurate table cardinality) */ $sIndexColumn = "people_id"; /* DB table to use */ $sTable = "People"; /* * Paging */ $sLimit = ""; if ( isset( $_GET['iDisplayStart'] ) && $_GET['iDisplayLength'] != '-1' ) { $sLimit = "LIMIT ".intval( $_GET['iDisplayStart'] ).", ". intval( $_GET['iDisplayLength'] ); } /* * Ordering */ $sOrder = ""; if ( isset( $_GET['iSortCol_0'] ) ) { $sOrder = "ORDER BY "; for ( $i=0 ; $i<intval( $_GET['iSortingCols'] ) ; $i++ ) { if ( $_GET[ 'bSortable_'.intval($_GET['iSortCol_'.$i]) ] == "true" ) { $sOrder .= "`".$aColumns[ intval( $_GET['iSortCol_'.$i] ) ]."` ". ($_GET['sSortDir_'.$i]==='asc' ? 'asc' : 'desc') .", "; } } $sOrder = substr_replace( $sOrder, "", -2 ); if ( $sOrder == "ORDER BY" ) { $sOrder = ""; } } /* * Filtering * NOTE this does not match the built-in DataTables filtering which does it * word by word on any field. It's possible to do here, but concerned about efficiency * on very large tables, and MySQL's regex functionality is very limited */ $sWhere = ""; if ( isset($_GET['sSearch']) && $_GET['sSearch'] != "" ) { $sWhere = "WHERE ("; for ( $i=0 ; $i<count($aColumns) ; $i++ ) { $sWhere .= "`".$aColumns[$i]."` LIKE '%".mysql_real_escape_string( $_GET['sSearch'] )."%' OR "; } $sWhere = substr_replace( $sWhere, "", -3 ); $sWhere .= ')'; } /* Individual column filtering */ for ( $i=0 ; $i<count($aColumns) ; $i++ ) { if ( isset($_GET['bSearchable_'.$i]) && $_GET['bSearchable_'.$i] == "true" && $_GET['sSearch_'.$i] != '' ) { if ( $sWhere == "" ) { $sWhere = "WHERE "; } else { $sWhere .= " AND "; } $sWhere .= "`".$aColumns[$i]."` LIKE '%".mysql_real_escape_string($_GET['sSearch_'.$i])."%' "; } } $sQuery = " SELECT SQL_CALC_FOUND_ROWS * FROM (SELECT p.people_id, p.people_firstName, p.people_surname, p.people_address, p.people_town, p.people_county, p.people_postcode, r.relationships_id FROM People p LEFT JOIN Relationships r ON p.people_id = r.people_id) sel $sWhere $sOrder $sLimit "; // http://datatables.net/forums/discussion/2774/sql-join-two-tables/p1 $rResult = mysql_query( $sQuery, $gaSql['link'] ) or die(mysql_error()); /* Data set length after filtering */ $sQuery = " SELECT FOUND_ROWS() "; $rResultFilterTotal = mysql_query( $sQuery, $gaSql['link'] ) or die(mysql_error()); $aResultFilterTotal = mysql_fetch_array($rResultFilterTotal); $iFilteredTotal = $aResultFilterTotal[0]; /* Total data set length */ $sQuery = " SELECT COUNT(`".$sIndexColumn."`) FROM $sTable "; $rResultTotal = mysql_query( $sQuery, $gaSql['link'] ) or die(mysql_error()); $aResultTotal = mysql_fetch_array($rResultTotal); $iTotal = $aResultTotal[0]; /* * Output */ $output = array( "sEcho" => intval($_GET['sEcho']), "iTotalRecords" => $iTotal, "iTotalDisplayRecords" => $iFilteredTotal, "aaData" => array() ); while ( $aRow = mysql_fetch_array( $rResult ) ) { $row = array(); for ( $i=0 ; $i<count($aColumns) ; $i++ ) { if ( $aColumns[$i] == "relationships_id" ) { /* Special output formatting for 'version' column */ $row[] = ($aRow[ $aColumns[$i] ]=="") ? '0' : $aRow[ $aColumns[$i] ]; } else if ( $aColumns[$i] != ' ' ) { /* General output */ $row[] = $aRow[ $aColumns[$i] ]; } } $output['aaData'][] = $row; } echo json_encode( $output ); ?> Can any one help?
-
going to try this create a new field in Carer_Project_SubGroup called CPC_CatGroup. Update all CPC_Project=1 with CPC_CatGroup=50 and then run: insert into Carer_Category_Subgroup (CCatS_Carer) select (50) from Carer_Project_SubGroup where Carer_Project_SubGroup.CPC_Project=1 AND Carer_Project_SubGroup.CPC_CatGroup=50
-
think i have it insert into table2 (col1, col2, col3) select (1, 'norman', 'US') from Table1 t1 where t1.id=1 and t1.name = 'norman' and t1.country = 'US'
-
hello vinny i need to insert a value into table 1 where table2 value = 1 can you help?
-
looking at it i'll try this: SELECT a.CPC_Project, b.CCatS_Category FROM Carer_Project_SubGroup as a, Carer_Category_Subgroup as b Where a.CPC_Carer = b.CCatS_Carer AND a.CPC_Project = '1'; INSERT INTO Carer_Category_Subgroup (CCatS_Category) VALUES ('50');
-
I have some mysql UPDATE t1 LEFT JOIN t2 ON t2.id = t1.id SET t1.col1 = newvalue WHERE t2.id IS NULL; I have used the above example and it works well. I Know need to join and insert. Have Tried the below INSERT INTO Carer_Category_Subgroup LEFT JOIN Carer_Project_SubGroup ON Carer_Project_SubGroup.CPC_Carer = Carer_Category_Subgroup.CCatS_Carer SET Carer_Category_Subgroup.CCatS_Category = '50' WHERE Carer_Project_SubGroup.CPC_Project = '1'; but get: You have an error in your SQL syntax; check the manual that corresponds to your MySQL server version for the right syntax to use near 'LEFT JOIN Carer_Project_SubGroup ON Carer_Project_SubGroup.CPC_Carer = Carer_Cat' at line 1" Can't seem to get this to work?
-
Brilliant. Made my day!
-
if i change $merchdata to $merchdata[] $merchData[] = array($EventFees_item2=>$EventFees_item_qty); and then: $fields = ''; $merchData = $merchData[0]; foreach($merchData as $col => $val) { if ($count++ != 0) $fields .= ', '; $fields .= "`$col` = $val"; } ++$count; i get INSERT INTO `EventSignUps` SET ordered_dateStamp = NOW(), people_id = , event_id = 9, event_total = , order_auth = '1', payment_type = , `Ridders` = ; if i change it to: $merchData = $merchData[1]; i get INSERT INTO `EventSignUps` SET ordered_dateStamp = NOW(), people_id = , event_id = 9, event_total = , order_auth = '1', payment_type = , `Walkers` = ; Does anyone know how to get INSERT INTO `EventSignUps` SET ordered_dateStamp = NOW(), people_id = , event_id = 9, event_total = , order_auth = '1', payment_type = , `Walkers` =, `Ridders` = ;
-
<?php $count = 0; $query2 = "SELECT EventFees_id, EventFees_item, EventFees_fee, EventFees_event FROM EventFees WHERE EventFees_event = '{$_GET['id']}'"; $result2 =mysql_query($query2) or die(mysql_error()); while(list($EventFees_id, $EventFees_item, $EventFees_fee, $EventFees_event) = mysql_fetch_array($result2, MYSQL_NUM)) { $EventFees_item2 = str_replace(' ', '', $EventFees_item); $EventFees_item_qty = mysql_real_escape_string($_POST[$EventFees_item2 = str_replace(' ', '', $EventFees_item)]); $merchData = array($EventFees_item2=>$EventFees_item_qty); ++$count; } $fields = ''; foreach($merchData as $col => $val) { if ($count++ != 0) $fields .= ', '; $col = mysql_real_escape_string($col); $val = mysql_real_escape_string($val); $fields .= "`$col` = $val"; } $query = "INSERT INTO `EventSignUps` SET ordered_dateStamp = NOW(), people_id = $people_id, event_id = $event_id, event_total = $event_total, order_auth = '1', payment_type = $payment_type, $fields;"; ?> produces : INSERT INTO `EventSignUps` SET ordered_dateStamp = NOW(), people_id = , event_id = 9, event_total = , order_auth = '1', payment_type = , , `Runners` = ; I need $merchData = array($EventFees_item2=>$EventFees_item_qty); to provide 2 records. Currently its only showing results for 1 Should look like: INSERT INTO `EventSignUps` SET ordered_dateStamp = NOW(), people_id = , event_id = 9, event_total = , order_auth = '1', payment_type = , `Walkers` = , `Runners` = ; I'm going somewhere wrong in the while loop. Its counting 2, but only showing results for 1 ?
-
Think this has worked $q = "SELECT username FROM users WHERE NOT EXISTS (SELECT candidate_name FROM applied_jobs WHERE candidate_name = username)";
-
Hi Guys I am trying to match the usernames from 2 tables but then only show the usernames that are not matched (the rows: users and candidate_name both hold usernames) $query1 = "SELECT users FROM users"; $query2 = "SELECT candidate_name FROM applied_jobs"; Can anyone teach me how to do this? Thanks guys
-
thanks you set me on the right path, i added a ++$count; to do the bit you suggested
-
Hi i am trying to pass values from a cart to a database using foreach. This is my code: $columns = array('order_id', 'order_item_id', 'order_product_name', 'order_product_dimensions', 'order_product_options', 'order_product_quantity', 'order_product_price', 'order_product_subtotal', 'order_product_vat', 'order_product_delivery','order_product_total','order_product_sku','order_product_image_name','order_product_image_type','order_product_image_path','order_product_image_size','order_product_artwork_link','order_product_artwork_name','order_product_artwork_type','order_product_artwork_path','order_product_artwork_size','order_firstname','order_lastname','order_companyname','order_email','order_tel','order_billing_address','order_billing_address2','order_billing_town','order_billing_county','order_billing_postcode','order_shipping_address','order_shipping_address2','order_shipping_town','order_shipping_county','order_shipping_postcode','order_status','order_username'); $data = array_fill_keys($columns, 'NULL'); foreach($data as $key => $value) { $data[$key] = empty($_POST[$key]) ? 'NULL' : "'".mysql_escape_string($_POST[$key])."'"; $query = "INSERT INTO orders (id, order_created_at, ".implode(", ",$columns).") VALUES (null, NOW(), ".implode(",",$data).')'; } Problem is posting 2 or more products into the database. How do i split the values so it enters each product into the database?
-
i'll take a look thanks.
-
without the brackets it pulls the correct result. With the brakets it doesnt pull the right number. I was just hoping there was a way to write this where i didn't have to write out the col_1 !='' or col_2 !='' or col_3 !='' or col_4 !='' or col_5 !='' or col_6 !='' or col_7. Was thinking of a loop col_$i !='' And a way of finding out how many cells was in the table i.e col_1 to col_10?
-
Hi Guys how do i streamline this code and generally make it better? <?php $query2 = "SELECT id, col_1, col_2, col_3, col_4, col_5, col_6, col_7, col_8, col_9, col_10 FROM prices WHERE product = '".$product_name."' AND col_1 !='' or col_2 !='' or col_3 !='' or col_4 !='' or col_5 !='' or col_6 !='' or col_7 !='' or col_8 !='' or col_9 !='' or col_10 !='' ORDER BY id"; $res2 =mysql_query($query2) or die(mysql_error()); $num_rows = mysql_num_rows($res2); $i=1; while($row = mysql_fetch_array($res2)){ while($i<=$num_rows) { echo "<li><a rel=\"nofollow\" class=\"opt\" href=\"#\">".$row[$i]."<span>".$row[$i]."</span></a></li>"; $i++; } } ?>
-
this worked: function getOtherProject($worker) { $output = ''; $query2 = "SELECT company_project FROM projects WHERE username='$worker'"; $res = mysql_query($query2); while($a = mysql_fetch_array($res)) { $output .= "company_project = '".$a["company_project"]."' or "; } return $output; }
-
if i use return it shows the, 'and company_project = 'HTML'' but not the full statement? function getOtherProject($worker) { $query2 = "SELECT company_project FROM projects WHERE username='$worker'"; $res = mysql_query($query2); while($a = mysql_fetch_array($res)) { return "company_project = '".$a["company_project"]."' or "; } }
-
Can anyone suggest a solution? function getOtherProject($worker) { $query2 = "SELECT company_project FROM projects WHERE username='$worker'"; $res = mysql_query($query2); while($a = mysql_fetch_array($res)) { echo "company_project = '".$a["company_project"]."' or "; } } getOtherProject($session->username); When called here reads: company_project = 'HTML' or company_project = 'Coder' or $query1 = "SELECT username, company, company_line_manager, firstname, lastname, level FROM work_users WHERE level = '4' and "; $query2 = getOtherProject($session->username); $query3 = "company_project = 'abc123'"; $query = $query1.$query2.$query3; $res = mysql_query($query, $cid); echo $query; [/code] When called here the function getOtherProject, get ignored: SELECT username, company, company_line_manager, firstname, lastname, level FROM work_users WHERE level = '4' and company_project = 'abc123'