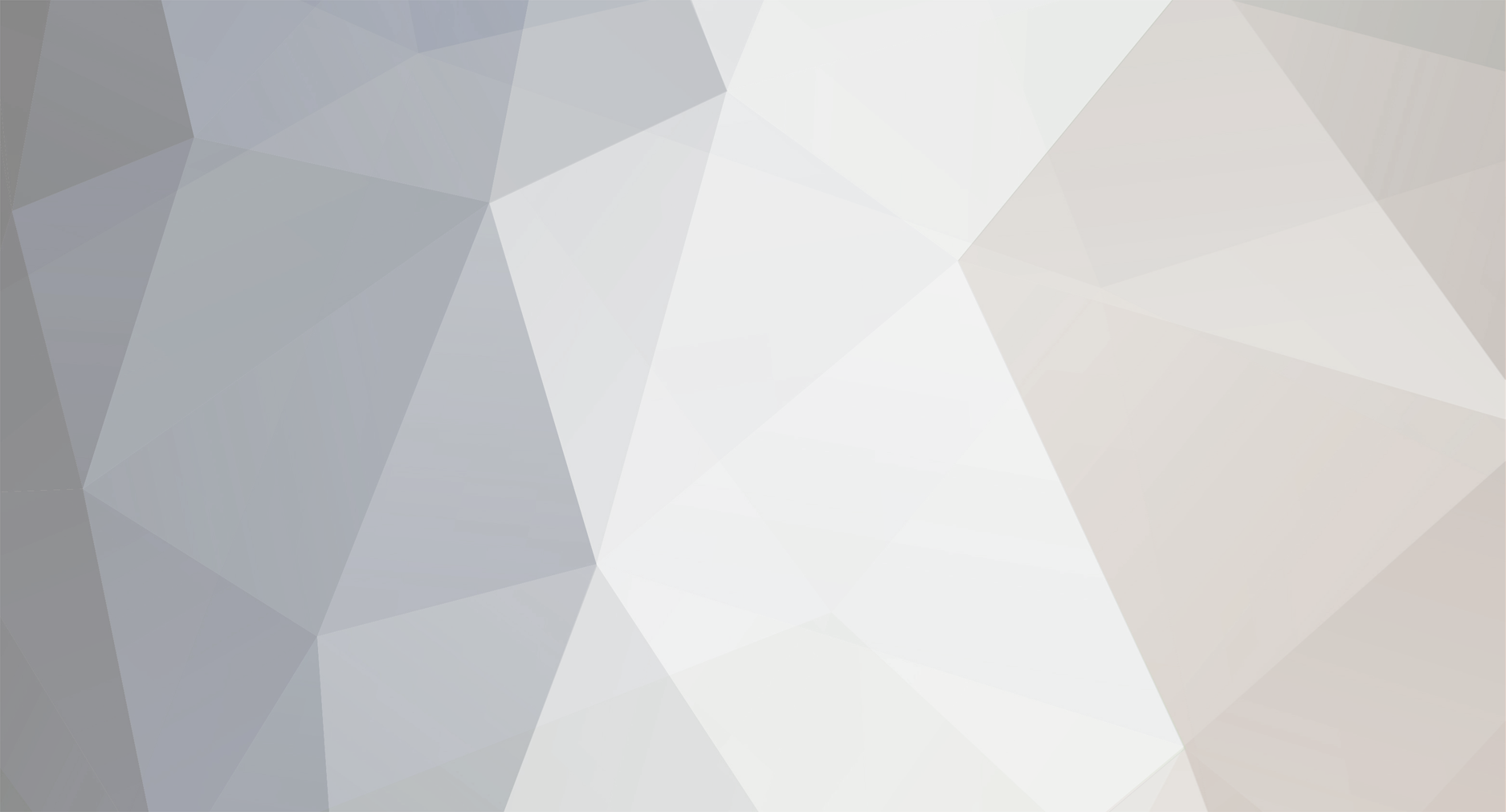
matthew9090
-
Posts
135 -
Joined
-
Last visited
Never
Posts posted by matthew9090
-
-
<?php
error_reporting(E_ALL);
$name = $_POST['name'];
$file = $_POST['file'];
$moved = move_uploaded_file($_POST['file']['tmp_name'], "upload/");
if ($moved) {
echo "moved!";
}
else {
echo "did not move!";
}
echo $name . "<br />" . $file . "<p>";
if (strlen($name)>10)
{
echo "name too long";
}
else
{
//private stuff
-
move_uploaded_file($_POST['file']['tmp_name'], "upload/"); doesn't work and if i echo the tmp name it comes up with the first letter of the uploaded file.
please help!
-
when i view the html of the page it comes up with the embed src as nothing
-
I have got a flash file that is stored in the database. So I fetch it and put it in a variable then put the variable in the flash embed code where the src is but the flash comes up blank.
$file = "SELECT * FROM table WHERE column='$name'"; $file2 = mysql_query($sqlfile); $file3 = @mysql_fetch_assoc($file2); $file4 = $file3['gamefile']; //skip some code to html embed section <object width='550' height='400'> <param name='movie' value='valuename'> <embed src='$file4' width='550' height='400'> </embed> </object>
then the page comes up with a white box which when you hover over it says 'file not loaded'.
-
its called pagination, search for it.
-
you need to use pagination
http://www.phpfreaks.com/tutorial/basic-pagination
it's better to use a database for it.
-
here is the full code:
<?php //get data $button = $_GET['submit']; $search = $_GET['search']; $s = $_GET['s']; if (!$s) $s = 0; $e = 10; $next = $s + $e; $prev = $s - $e; if (strlen($search)<=2) echo "Must be greater then 3 chars"; else { echo "<br /><table><tr><td><a href = index.php></a></td><td><form action='search.php' method='GET'><a href ='index.php'><img src='logo.png' align='top' height='28' width='60'></img></a><input type='text' value='' size='50' name='search' value='$search'> <input type='submit' name='submit' value='Search'></form></td></tr></table>"; //connect to database mysql_connect("localhost","root",""); mysql_select_db("test"); //explode out search term $search_exploded = explode(" ",$search); foreach($search_exploded as $search_each) { //construct query $x++; if ($x==1) $construct .= "keywords LIKE '%$search_each%'"; else $construct .= " OR keywords LIKE '%$search_each%'"; } //echo outconstruct $constructx = "SELECT * FROM search WHERE $construct"; $construct = "SELECT * FROM search WHERE $construct LIMIT $s,$e"; $run = mysql_query($constructx); $foundnum = mysql_num_rows($run); $run_two = mysql_query("$construct"); if ($foundnum==0) echo "No results found for <b>$search</b>"; else { echo "<table bgcolor='#0000FF' width='100%' height='1px'><br /></table><table bgcolor='#f0f7f9' width='100%' height='10px'><tr><td><div align='right'><b>$foundnum</b> result(s) found for <b>$search.</b></div></td></tr></table><p>"; while ($runrows = mysql_fetch_assoc($run_two)) { //get data $title = $runrows['title']; $desc = $runrows['description']; $url = $runrows['url']; echo "<table width='300px'> <h4><a href='http://$url'><b>$title</b></a><br /> $desc<br> <font color='00CC00'>$url</font></table></h4> "; } ?> <table width='100%'> <tr> <td> <div align="center"> <?php if (!$s<=0) echo "<a href='search.php?search=$search&s=$prev'>Prev</a>"; $i =1; for ($x=0;$x<$foundnum;$x=$x+$e) { echo " <a href='search.php?search=$search&s=$x'>$i</a> "; $i++; } if ($s<$foundnum-$e) echo "<a href='search.php?search=$search&s=$next'>Next</a>"; } } ?> </div> </td> </tr> </table>
-
you could use the implode function to turn it into string then str_replace then explode into array again.
e.g
<?php $array = //array implode(" " . $array); str_replace($replace, $array); explode(" " . $array); ?>
it should work, just wrote it of the top of my head.
-
I've looked at loads of other scripts that highlight the page but none of them work on mine . Here's part of my code:
$i =1; for ($x=0;$x<$foundnum;$x=$x+$e) { echo " <a href='search.php?search=$search&s=$x'>$i</a> "; $i++;
-
in my site search some of the results come out still with html. i have used the strip tags function but how would i use the preg_match function to get rid of the "> still left over?
-
thanks it worked!
-
i have made my own website suggestion box but when i start typing in on my websites search box google chrome automatically suggests other words and you can't see underneath. I don't want this to happen to other people viewing my website. Is there any sort of code i an put on my website to stop this?
-
code so far
<?php include('simple_html_dom.php'); ?> <script type="text/javascript"> function redirect(){ window.location.href = "index.html"; } </script> <?php $s = $_GET["s"]; if ($s == null) echo "you have not searched for anything" . '<script type="text/javascript">window.onload = setTimeout("redirect()", 3000);</script>'; else echo "you searched for $s<hr>"; $urls[1] = 'http://url.com/'; $urls[2] = 'http://url2.com'; foreach ($urls as $url) { $html = file_get_html($url); strip_tags($html); preg_match("/<body>(.*)<\/body>/s", $html, $body); preg_match('@^(?:http://)?([^/]+)@i', $html, $matches); $htmls_split = split('<', $body[1]); foreach ($htmls_split as $line) { if (preg_match("/$s.*/", $line, $matches)) { if (strlen($s) == 0) die(); else str_replace($s, "<b>$s</b>", $matches[0]); echo "<p>$matches[0]"; } } } ?>
the str_replace doesn't do anything at the moment. :'(
please help!
-
were about do I put that in the script?
-
no it defiantly retrieves the html and displays the results i just need to hightlight the searched words within the results
-
i am making a search engine to search my site and the code is going well but i don't know how to find your search within the results and put it in bold (like google does). I use a thing called http://simplehtmldom.sourceforge.net/ for getting the html of my website.
<?php include('simple_html_dom.php'); ?> <?php $s = $_GET["s"]; if ($s == null) echo "you have not searched for anything"; else echo "you searched for $s<hr>"; $urls[0] = 'http://url.com/'; $urls[1] = 'http://url2.com'; foreach ($urls as $url) { $html = file_get_html($url); strip_tags($html); preg_match("/<body>(.*)<\/body>/s", $html, $body); $htmls_split = split('<', $body[1]); foreach ($htmls_split as $line) { if (preg_match("/$s.*/", $line, $matches)) { echo "<p>$matches[0]"; } } } ?>
-
if you need to parse an HTML page, it's probably worth looking at simplehtmldom, which makes the process much much easier: http://simplehtmldom.sourceforge.net/
it comes up with an error for this line:
$html = file_get_html('http://www.google.com/');
Fatal error: Call to undefined function file_get_html() in C:\wamp\www\usearch\submit_url.php on line 12
-
i am making a search engine and i need it so when you submit your url it scrolls the page for any keywords and descriptions and puts that into the database
-
i am making a search engine and i need help because i need it so you submit your url then it automatically scrolls it for the description and keywords then puts it in the database. my html code:
<form action="submit_url.php" method="get"> <input type="text" name="url" value="url" /> <input type="submit" name="submit" value="submit" /> </form>
php code so far:
<html> <head> <title>submitting url: <?php $url = $_GET['url']; echo $url; ?></title> <link href="style.css" rel="stylesheet" type="text/css" /> </head> <body> <?php echo "Submitting <b>$url</b>"; $url = fopen("http://knexideas.co.cc", "r") or exit("Unable to open"); while (!feof($url)) { fgetc($url); } fclose($url); ?> </body> </html>
all it does is reads the website if you put 'echo $url' at the bottom it just reads and prints the web page.
-
it comes up with this in the database:
id name file_name type_of_file
1 file [bLOB - 11 Bytes] Array[type]
2 another file [bLOB - 11 Bytes] Array[type]
the insert script:
<?php //connection stuff $sql="INSERT INTO upload (name, file_name, type_of_file, size) VALUES ('$_POST[name]','$_FILES[file][name]','$_FILES[file][type]')"; if (!mysql_query($sql,$con)) { die('Error: ' . mysql_error()); } echo "Idea name: " . $_POST["name"] . "<br />"; echo "Upload: " . $_FILES["file"]["name"] . "<br />"; echo "Type: " . $_FILES["file"]["type"] . "<br />"; echo "Size: " . ($_FILES["file"]["size"] / 1024) . " Kb<br />"; echo "<b>Upload sucessful!</b>"; mysql_close($con) ?>
-
im not a proffesional but if you want to transfer it over i think you have to create a seperate page called calender and a page with the form on the the data goes to the calender.
e.g:
form.html
<form action="myform.php" method="post">
myform.php
//code here
but i think there is another way to do it on the same page:
<form action="<? echo $_SERVER['PHP_SELF']; ?>" method="post" ENCTYPE="multipart/form-data">
//code down here
or something like that
-
i've found a pagination script of the internet but the image comes up blank. I've stored it in a largeblob. When i check the html it says 'array[name]' as the image pz help me heres the code:
<?php //connection stuff here $page = 1; if ( isset( $_GET['page'] ) ) { $page = (int) $_GET['page']; } $query = mysql_query("SELECT COUNT(*) FROM `upload`",$link); list( $total ) = mysql_fetch_row( $query ); $total = ceil( $total / $perPage ); $start = ( $perPage * ( $page - 1 ) ); $limit = ''; $show = true; if ( $total > 1 ) { $limit = " LIMIT {$start}, {$perPage}"; } else { $show = false; } $query = mysql_query("SELECT * FROM `upload` ORDER BY `id`{$limit}"); while( $row = mysql_fetch_assoc( $query ) ) { echo "<img src=\"{$row['file_name']}\" alt=\"{$row['name']}\" title=\"{$row['name']}\" />"; } if ( $show ) { $prev = $page - 1; $next = $page + 1; if ( $prev > 0 ) { echo "<a href=\"{$thispage}?page={$prev}\">[PREV]</a>"; } if ( $next < $total ) { echo "<a href=\"{$thispage}?page={$next}\">[NEXT]</a>"; } } ?>
-
i am trying to put a perl variable in html but i don't know how to.
e.g. my $variable = #the code
print qq(
#variable needs to be displayed here
#html
\n);
-
i am designing a browser game at the moment. ipw.matthew9090.x10hosting.com i am still working on it.
i need help with the layout of this page and the others:
ipw.matthew9090.x10hosting.com/player_overview.php
or
ipw.matthew9090.x10hosting.com/ranking.php
or any other
move_uploaded_file not working
in PHP Coding Help
Posted
that doesn't work it comes up with 'did not move'