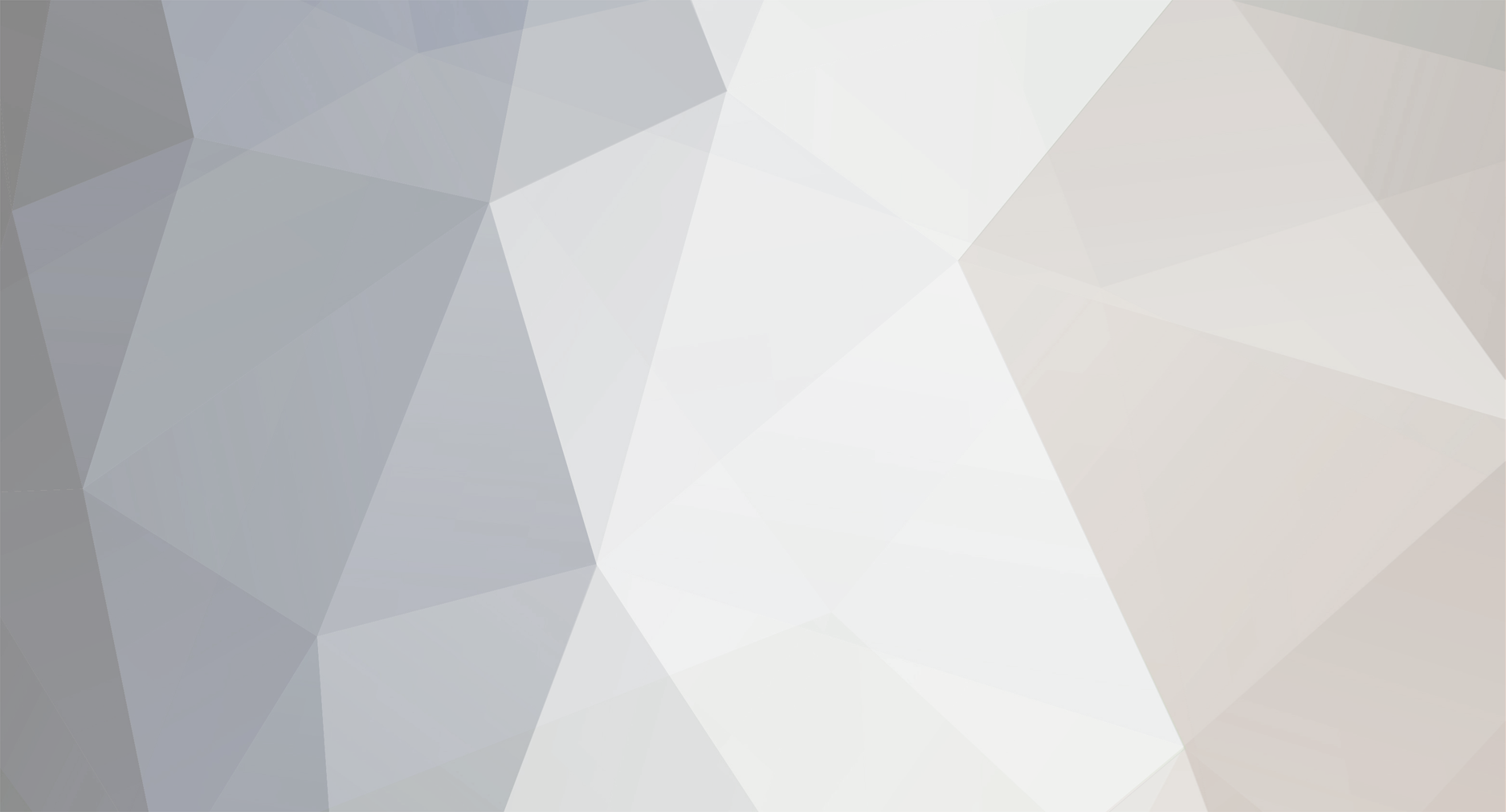
xjermx
Members-
Posts
35 -
Joined
-
Last visited
Never
Everything posted by xjermx
-
I am trying to implement an email style messaging feature into a PHP application. I have a 'users' table with a primary key that auto increments, and my plan is to do two mail tables, mail1 has the fields mail1_id, message_text, from_user, timestamp (mail1_id is the primary key and autoincrements, from_user is a foreign key that will relate back to the users table) mail2 has the fields mail2_id, to_user, link_to_mail1 (mail2_id is primary key and autoincrements, link_to_mail1 is intended to provide relationship between the table records) I'm a newbie, so I'm assuming this is a descent setup. I've done it this way so that if a user sends a message to multiple other users, it will minimize repetition in the table, especially of the message_text field. My question is, how do I insert data into this arrangement from a PHP application? I'm familiar with basic INSERT, but obviously I need to know what mail1_id ends up being, in order to populate the link_to_mail1 field. I could probably use an INSERT, QUERY, INSERT scheme to do it, but I can't help but feel like there is a better way of doing this. Any suggestions? Thanks! (edit: I'm assuming that this should be here in MySQL instead of in PHP?)
-
I have a page with some buttons on it, currently using html <submit> that I am using to link to another PHP page. I am interested in having a set of functions represented on page 1 by buttons. In this case, something like "Create a new message", "Read messages for me", "View messages that I have sent". I want each of these functions to be supported on page 2. I'm aware that I can likely do this with functions and switch or if statements, but my question is this: What is the best case recommendation for implementing the buttons on page 1 and then getting the $whichaction variable or value over to page 2 so that page knows which function to execute?
-
Thanks for the responses. I tried what BillyBob and PFMaBiSmAd suggested. I stuck return $gNames; // return value here at the tail end of my function Here is part is my code again, with a few more printed debug lines: <?php print "<h5>Debug line 1. Top of program.</h5>"; $returnVal = myfunction1(); //collect data here $rowCheck = mysql_num_rows($returnVal); print $rowCheck; while ($row = mysql_fetch_assoc($returnVal)){ print "<h5>Debug line 2. Start of WHILE</h5>"; echo is_array($row) ? 'Array<br>' : 'not an Array<br>'; print_r($row); foreach ($row as $col=>$val){ // if ($col == 'name') { print " $val, \n"; // } } // end foreach foreach ($row as$val){ print " $val, \n"; } // end foreach }// end while function myfunction1() { global $gNames; $db = mysql_connect("blahblahblah", "myuser", "mypass") or die ("Error connecting to database."); mysql_select_db("databasename", $db) or die ("Couldn't select the database."); $result = mysql_query("SELECT * FROM characters WHERE whoschar='1'",$db); // modified to only show for user 1 $gNames = $result; $rowCheck = mysql_num_rows($result); print "<h5>Debug line 3. inside MYFUNCTION1</h5>"; if($rowCheck > 0){ echo 'You do have characters, they should be listed here..<br>'; while ($row = mysql_fetch_assoc($result)){ print "<h5>Debug line 4. inside MYFUNCTION1, inside WHILE</h5>"; echo is_array($row) ? 'Array<br>' : 'not an Array<br>'; //debugging print_r($row); //debugging echo "<br>"; foreach ($row as $col=>$val){ if ($col == 'name') { print " $val, \n"; } } // end foreach }// end while print "<br><br>"; } else { echo 'You have no characters. You should create one.'; } return $gNames; // return value here } /////////////////////////////// end function ?> Note that I'm not seeing "Debug Line 2", which means that it is not getting into the while statement there at the top.
-
I've tried re-reading and re-re-reading the available info on arrays, but I'm just not understanding what I'm doing wrong, or how I can effectively troubleshoot it. My question is this: How do I do a mysql query inside a function, and then let the main page, or another function on the main page use the array that the query produces? Here is some code: <?php myfunction1(); $rowCheck = mysql_num_rows($gNames); print $rowCheck; while ($row = mysql_fetch_assoc($gNames)){ echo is_array($row) ? 'Array<br>' : 'not an Array<br>'; print_r($row); foreach ($row as $col=>$val){ // if ($col == 'name') { print " $val, \n"; // } } // end foreach foreach ($row as$val){ print " $val, \n"; } // end foreach }// end while function myfunction1() { global $gNames; $db = mysql_connect("blahblahblah", "myname", "mypass") or die ("Error connecting to database."); mysql_select_db("databasename", $db) or die ("Couldn't select the database."); $result = mysql_query("SELECT * FROM characters WHERE whoschar='1'",$db); // modified to only show for user 1 $gNames = $result; $rowCheck = mysql_num_rows($result); if($rowCheck > 0){ echo 'You do have characters, they should be listed here..<br>'; while ($row = mysql_fetch_assoc($result)){ echo is_array($row) ? 'Array<br>' : 'not an Array<br>'; //debugging print_r($row); //debugging echo "<br>"; foreach ($row as $col=>$val){ if ($col == 'name') { print " $val, \n"; } } // end foreach }// end while print "<br><br>"; } else { echo 'You have no characters. You should create one.'; } } /////////////////////////////// end function ?> Basically, the query goes just fine inside the function, it displays its data, it works like a dream. But I cannot seem to be able to pass it off to the main part of the page. the rowCheck in the main part returns "3", indicating that it has data in it, but I can't touch/view it. Is there a trick that I don't know, or something basic that I'm overlooking/not understanding?
-
How to prevent users from viewing certain pages?
xjermx replied to Smudly's topic in PHP Coding Help
As someone else may have pointed out, using a login system and $_SESSION variables may do this for you. I can provide some simple code examples if you like. -
OMG. Fixed. So simple. Thanks so much.
-
Thanks for the responses. I should provide more clarification, and there may be a completely different (better) way to do what I'm trying to do. When a user hits the page, there is no value set for "charname". So function2 is implemented. In function 2 there is an input box to enter a name (charname), and a submit box, but also a box to navigate away to another menu, in the event that the user changes their mind and doesn't want to put in a name. Normally when they DO enter a name, the program behaves normally, taking that value, and running function1, which is normally a mySQL insert or somesuch. However, what's happening is that when someone decides "Nah, I don't wanna put in a name", and hits the Back to Game Menu button, the POST data has a value for charname as "" (blank). It then gives that you function1 which throws the blank value into sql. What I'm really trying to do is simply create a "Nah I changed my mind, get me outta here" button. I know I could do this with a simple a href= link back to another page, but I wanted to do it with form stuff if possible.
-
Newbie question: I have the following code. <?php if (filter_has_var(INPUT_POST, "charname")) { function1(); } else { function2(); } function function1() { print "<h2>Function 1</h2>"; } function function2() { print "<h2>Function 2</h2>"; print <<< HERE <form method = "post" action = ""> Please enter a name for your new character: <input type="text" name="charname" value="" /> <form method = "post" action = ""> <input type="submit" value="Create" /> <br> HERE; print <<< HERE <form method = "post" action = "game_playmenu.php"> <input type="submit" value="Back to Game Menu" /> </form> HERE; } ?> What I'm trying to do here is this. Suppose you've arrived at the page and decide that you *don't* want to enter a character name, so you click the Back to Game Menu button.. What seems to happen is that it gives 'charname' a value (""), and then hits function1. I want it to just exit directly back to the game menu. How can I modify my existing code to do this? Thanks!
-
Hi, total newbie here. Working on teaching myself PHP and codeigniter, largely through a cargo cult style of learning. I've spent a few days banging head against the wall trying to do something that I think is relatively simple. I have an array which I'll pulled via a function in my model from a mysql database. $data = $this->smoke_model1->list_users(); print_r($data); gives me: I am trying to fiddle with how that array is setup. Ultimately I'm trying to pass the data to a view, display the data with checkboxes and a submit button, and then based on which are checked, pass them back to the model to be deleted from the mysql table. I've successfully passed the data to the view, and gotten it to display, but I think that I need to tweak the array - the lack of key names is throwing me off. I've managed to make the following code work for testing: $data['multi'] = array( array('name'=> 'xid[]','id'=>'78','name2'=>'Bob Barker','ip'=>'5465465465','value'=>'hi my name is Bob'), array('name'=> 'xid[]','id' => '17','name2'=>'Joe Smith','ip'=>'255.255.255.0','value' => 'Hi my name is Joe'), array('name'=> 'xid[]','id' => '14', 'name2' => 'Sam Turner','ip' =>'127.0.0.1','value'=> "Hi my name is Sam"), ); because I can use foreach ($multi as $var) and make calls to $var and $var['name'] etc. Hence, my assumption is that if I can plug the values from my sql table into an array that has proper key names, then I can use them in the view. Is there a simpler way to do this?
-
I've thrown myself into the pool, so to speak, and am using some reference material, but a large dose of reverse engineering to teach myself PHP. My ultimate goal is to be able to create web apps for entertainment sake, browser game type stuff. I heard a recommendation for using Code Igniter. My question is this: As I'm teaching myself PHP, can/should I attempt to utilize CI? Or am I going to be better off learning PHP, and *then* tackling CI or something like it?