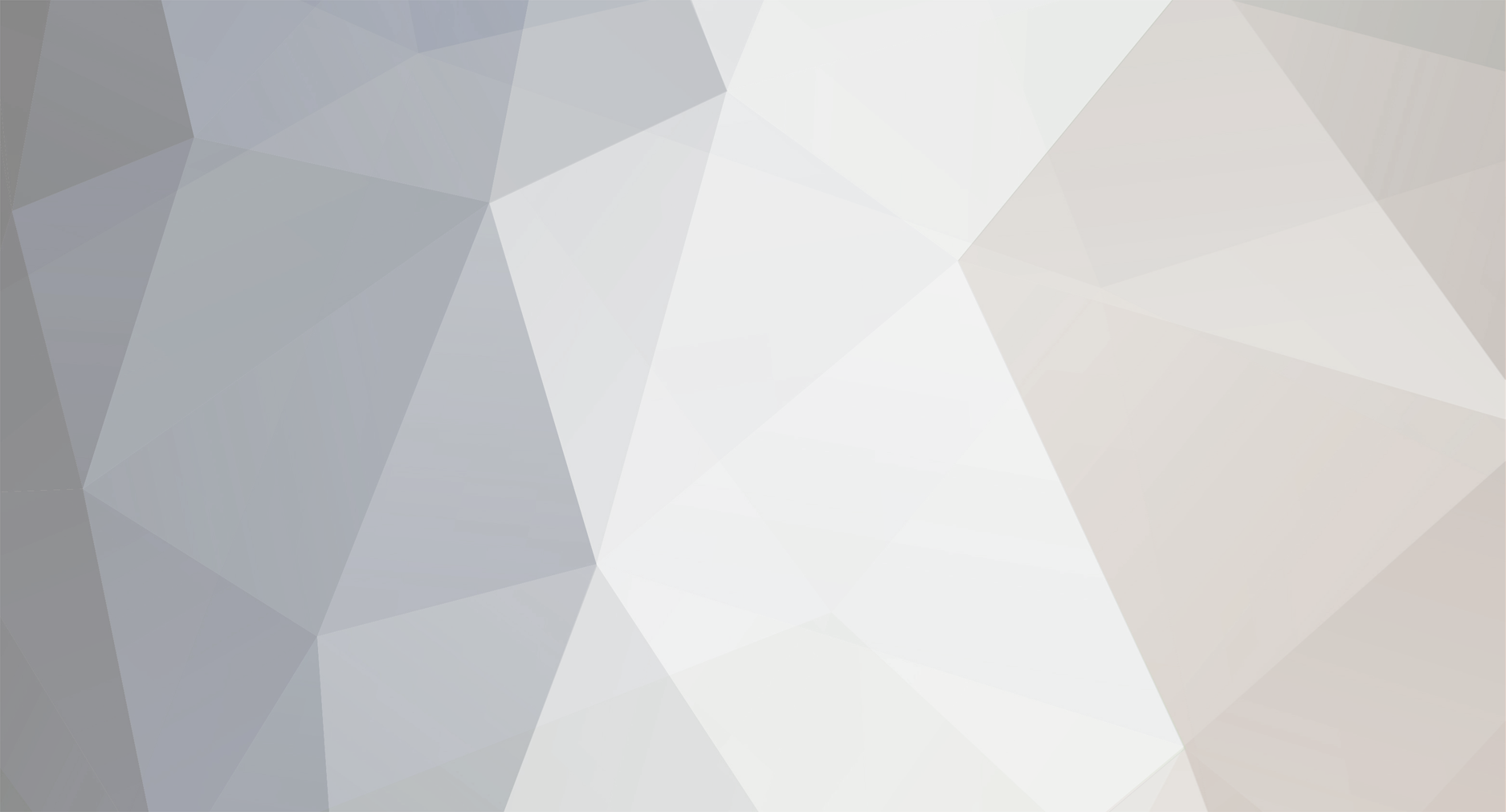
mctrivia
Members-
Posts
29 -
Joined
-
Last visited
Never
Everything posted by mctrivia
-
as promised here is some working code. I am sure there are ways to optimize. especially when to end(I am using iteration count only). But it works <?php define("POINT_COUNT",6); function lockToSphere($points) { $temp=array(); foreach ($points as $point) { //get length $length=sqrt($point[0]*$point[0]+$point[1]*$point[1]+$point[2]*$point[2]); //normalize to 1 $temp[]=array($point[0]/$length,$point[1]/$length,$point[2]/$length); } return $temp; } //generate random points in a 1x1x1 cube $points=array(); for ($i=0;$i<POINT_COUNT;$i++) { $points[]=array(rand(1,1000)/1000,rand(1,1000)/1000,rand(1,1000)/1000); } //lock points to sphere $points=lockToSphere($points); $m=10; for ($i=0;$i<10000;$i++) { if ($i%10==0) { $m/=1.01; } foreach ($points as $ka=>$pa) { $forces=array(0,0,0); foreach ($points as $kb=>$pb) { if ($ka!=$kb) { //compute difference between 2 points $d=array($pb[0]-$pa[0],$pb[1]-$pa[1],$pb[2]-$pa[2]); //compute forces $distance=sqrt($d[0]*$d[0]+$d[1]*$d[1]+$d[2]*$d[2]); $multiplier=1/($distance*$distance*$distance); //force/distance = 1/distance^3 $forces[0]-=$d[0]*$multiplier; $forces[1]-=$d[1]*$multiplier; $forces[2]-=$d[2]*$multiplier; } } //move point by scaled amount $temp[$ka]=array($pa[0]+$m*$forces[0],$pa[1]+$m*$forces[1],$pa[2]+$m*$forces[2]); } $points=lockToSphere($temp); } //print points foreach ($points as $point) { echo $point[0] . ',' . $point[1] . ',' . $point[2] . '<br>'; } ?>
-
I ditched this whole version and instead went 3d points. the pressure pushes the points off the sphere so after each iteration I normalize the distance to the center to 1. Works perfectly. will post code later when I find my thumb drive so I don't get typos from retyping.
-
there is no parsing error. there is just no edit option to allow me to put the ; back in. My test system has no internet connection so I just retyped the code out. I missed 1 ;
-
the ; got lost from the end of the one line when I copied over. Not the problem as it is present on my machine.
-
There is a cool flash app here http://members.ozemail.com.au/~llan/mpol.html that computes a shape with as far apart as possible points(keeping them on the outer plane of a sphere) using the inverse square law and multiple iterations. Problem is the flash dies if you try to run really high point counts and I wanted to be able to mess with the physics by adding fixed points. All i need is the x,y,z cowardinents of each point so I can tell my 3d printer how to print the shape. I tried to convert the script to PHP. Problem is no matter what I try I get meaningless results out. My theory was if I map my points on a longitude and latitude grid of a circle of radius 1 I could simplify things a fair bit. My complete source code is at the bottom but let me break down what I believe each section should do. In forces function it computes the distance between 2 points using the formula: acos(sin($lat1)*sin($lat2) + cos($lat1)*cos($lat2) * cos($lon2-$lon1)); since I went with a gravitational constant of 1 a radius of 1 and a mass for each point of 1 the repulsion force between the points should be: 1/($distance*$distance) direction of this force clockwise from North should be $y=sin($lon2-$lon1) * cos($lat2); $x=cos($lat1)*sin($lat2)-sin($lat1)*cos($lat2)*cos($lon2-$lon1); //invert direction $direction=atan2($y,$x)+pi(); function addResults splits the force and bearing up into rectangular format so I can add all the vectors easier then function move takes the sum off all the vectors multiplies it by a scaler to limit the points movement and computes the new point. Problem is it does not work. I have definitely screwed up. Any help would greatly be appreciated. <?php define("POINT_COUNT",6); function forces($a,$b,&$force,&$direction) { //break up arrays $lat1=$a[0]; $lat2=$b[0]; $lon1=$a[1]; $lon2=$b[1]; //compute distance between points $distance=acos(sin($lat1)*sin($lat2) + cos($lat1)*cos($lat2) * cos($lon2-$lon1)); //compute force direction if ($distance==0) { $direction=rand(0,2*pi()); //pick random direction $distance=0.1; } else { $y=sin($lon2-$lon1) * cos($lat2); $x=cos($lat1)*sin($lat2)-sin($lat1)*cos($lat2)*cos($lon2-$lon1); //invert direction $direction=atan2($y,$x)+pi(); } $force=1/($distance*$distance) } function move($point,$forcex,$forcey,$m) { $lat1=$point[0]; $lon1=$point[1]; $d=sqrt(pow($forcex*$m,2)+pow($forcey*$m,2)); if ($forcex==0) { $forcex=0.000001; } $bearing=atan(((0-$forcey)*$m)/((0-$forcex)*$m)); $lat2=asin(sin($lat1)*cos($d)+cos($lat1)*sin($d)*cos($bearing)); $lon2=$lon1+atan2(sin($bearing)*sin($d)*cos($lat1),cos($d)-sin($lat1)*sin($lat2)); return array($lat2,$lon2); } function sphereTo3D($point) { $lat=$point[0]; $lon=$point[1]; //get radius of x/y circle $r=cos($lat); //get z position $z=sin($lat); //calculate xy if ($r==0) { $x=0; $y=0; } else { $x=cos($lon)*$r; $y=sin($lon)*$r; } return array($x,$y,$z); } function addResult(&$x,&$y,$force,$theta) { $x+=cos($theta)*$force; $y+=sin($theta)*$force; } //latitude and longitue $points=array(); //add the number of points needed for ($i=0; $i<POINT_COUNT; $i++) { $points[]=array(deg2rad(rand(0,36000)/100),deg2rad(rand(0,36000)/100)); } for($i=0;$i<1000;$i++) { $m=0.01; //compute forces between each point $temp=array(); foreach ($points as $key=>$point) { //compute force on point $forcex=0; $forcey=0; foreach ($points as $a=>$b) { if ($key!=$a) { forces($point,$b,$force,$direction); addResult($forcex,$forcey,$force,$direction); } } //determine what should do to try and nutralize force $temp[]=move($point,$forcex,$forcey,$m); } $points=$temp; } //convert to 3d foreach ($points as &$point) { $point=sphereTo3D($point); echo $point[0] . ',' . $point[1] . ',' . $point[2] .'<br>'; } ?>
-
thanks I will initialize the garbage collector and see what else I can do to save memory.
-
those are all temporary. i thought if they were within a function they were automatically given up when function completed.
-
because i can only set cron jobs to run every minute. not every second.
-
class Point { protected $v=array(); public function __construct() { $this->id=++$_GET['zzz_library_model_lastpoint']; if (func_num_args()==0) { $this->v=array(0,0,0); } elseif (func_num_args()==1) { $this->v=func_get_arg(0); } elseif (func_num_args()==3) { $this->v=array(func_get_arg(0),func_get_arg(1),func_get_arg(2)); } } public function __get($name) { switch (strtolower($name)) { case 'x': return $this->v[0]; break; case 'y': return $this->v[1]; break; case 'z': return $this->v[2]; break; case 'v': return $this->v; break; case 'id': return $this->id; break; } } public function __set($name,$value) { switch (strtolower($name)) { case 'x': $this->v[0]=$value; break; case 'y': $this->v[1]=$value; break; case 'z': $this->v[2]=$value; break; case 'v': $this->v=$value; break; } } public function __toString() { return pack("f",$this->v[0]) . pack("f",$this->v[1]) . pack("f",$this->v[2]); } public function rotate($theta,$axis) { //theta in rads use deg2rad() if you want deg. switch (strtolower($axis)) { case 'x': $x=cos($theta)*$this->v[1]-sin($theta)*$this->v[2]; $y=sin($theta)*$this->v[1]+cos($theta)*$this->v[2]; $this->v[1]=$x; $this->v[2]=$y; break; case 'y': $x=cos($theta)*$this->v[2]-sin($theta)*$this->v[0]; $y=sin($theta)*$this->v[2]+cos($theta)*$this->v[0]; $this->v[2]=$x; $this->v[0]=$y; break; case 'z': $x=cos($theta)*$this->v[0]-sin($theta)*$this->v[1]; $y=sin($theta)*$this->v[0]+cos($theta)*$this->v[1]; $this->v[0]=$x; $this->v[1]=$y; break; } } public function translate($x,$y,$z) { $this->v[0]+=$x; $this->v[1]+=$y; $this->v[2]+=$z; } public function scale($x,$y=null,$z=1) { if ($y==null) { $y=$x; $z=$x; } $this->v[0]*=$x; $this->v[1]*=$y; $this->v[2]*=$z; } } // ************************************************************************************************************ class Triangle { protected $v=array(); public function __construct() { if (func_num_args()==0) { $this->v=array(new Point(),new Point(),new Point()); } elseif (func_num_args()==3) { $this->v=array(func_get_arg(0),func_get_arg(1),func_get_arg(2)); } elseif (func_num_args()==9) { $this->v=array( new Point(func_get_arg(0),func_get_arg(1),func_get_arg(2)), new Point(func_get_arg(3),func_get_arg(4),func_get_arg(5)), new Point(func_get_arg(6),func_get_arg(7),func_get_arg() ); } return !(($v[0]==$v[1]) || ($v[0]==$v[2]) || ($v[1]==$v[2])); } public function __set($name,$value) { $t=substr($value,1,1); switch (substr(strtolower($name),0,1)) { case 'x': $this->v[$t]->x=$value; break; case 'y': $this->v[$t]->y=$value; break; case 'z': $this->v[$t]->z=$value; break; case 'v': $this->v[$t]=$value; break; } } public function __get($name) { if (strlen($name>1)) { $t=substr($value,1,1); } else { $t=0; } switch (substr(strtolower($name),0,1)) { case 'x': return $this->v[$t]->x; break; case 'y': return $this->v[$t]->y; break; case 'z': return $this->v[$t]->z; break; case 'v': return $this->v[$t]; break; case 'p': return array_merge($this->v[0]->v,$this->v[1]->v,$this->v[2]->v); break; case 'n': return $this->getNormal(); break; } } public function __toString() { $n=$this->getNormal(); return pack("f",$n[0]).pack("f",$n[1]).pack("f",$n[2]).$this->v[0].$this->v[1].$this->v[2].pack("S",0); } protected function getNormal() { $a0=$this->v[2]->x-$this->v[0]->x; $a1=$this->v[2]->y-$this->v[0]->y; $a2=$this->v[2]->z-$this->v[0]->z; $b0=$this->v[1]->x-$this->v[0]->x; $b1=$this->v[1]->y-$this->v[0]->y; $b2=$this->v[1]->z-$this->v[0]->z; $x=$a1*$b2-$a2*$b1; $y=$a2*$b0-$a0*$b2; $z=$a0*$b1-$a1*$b0; $l=sqrt($x*$x+$y*$y+$z*$z); $x/=$l; $y/=$l; $z/=$l; return array($x,$y,$z); } }
-
is it possible to get an ajax call to execute a php script that will continue running even if the user leaves my site? i have used cron jobs and tokens to do in past but this can result in 1 min delay.
-
i guess my question is what makes an object require ram? i would think point would need only enough space to store the 3 floats and triangle need only enough space to hold the 3 pointers. my math says 80 bit*3 for floats+64bit*3 for pointers=54 bytes but 1000 are being used.
-
i have made 2 classes. the first "point" has an array of 3 floats and some functions to help manipulate the values the second "triangle" has an array of 3 points. in this way if i want to make a tetrahedron i can make 4 points and assign them to 4 triangles. problem is for some reason each triangle point group seems to use 1k. any idea why?
-
i am trying to find a way to render stl files into a jpg from a specific angle. any ideas? i have no clue.
-
I found a bug in the code where 1==1 would fail the test but shouldn't. Fixed now. Please let e know if you have tried to hack but failed. I think I have made this bulit proof but there are alot smarter people on here then me. <?php $whitefunc=array( 'abs()','acos()','acosh()','asin()','asinh()','atan()','atan2()','atanh()', 'base_convert()','bindec()', 'ceil()','cos()','cosh()', 'decbin()','dechex()','decoct()','deg2rad()', 'exp()','expm1()', 'floor()','fmod()', 'getrandmax()', 'hexdec()','hypot()', 'is_finite()','is_infinite()','is_nan()', 'lcg_value()','log()','log10()','log1p()', 'max()','min()','mt_getrandmax()','mt_rand()','mt_srand()', 'octdec()', 'pi()','pow()', 'rad2deg()','rand()','round()', 'sin()','sinh()','sqrt()','srand()', 'tan()','tanh()' ); function breakBracket($code,$a="(",$b=")") { $parts=array(); $end=strpos($code,$b); while (!($end===false)) { //find first start bracket before point $start=strrpos(substr($code,0,$end),$a); //find first end bracket after point $end=strpos($code,$b,$start); //break inner brackets out $parts[]=substr($code,$start+1,$end-$start-1); $code=substr($code,0,$start) . '|~-~|' . substr($code,$end+1); //find next search point $end=strrpos($code,$b); } $parts[]=$code; //replace '|~-~|' with brackets foreach ($parts as &$part) { $part=preg_replace("/\|~-~\|/", "()", $part); } return $parts; } function check_syntax($code) { return @eval('return true;' . $code); } function testRule($rule) { //replace $1,$2,$3... in rules with tag code parts $rule=preg_replace_callback( '/\$[0-9]+/', create_function( // single quotes are essential here, // or alternative escape all $ as \$ '$matches', 'return "53";' ), $rule ); //check for ilegal characters ;'" $good=(!(preg_match("/([;'\"])/",$rule)>0)); //check for assingments if ($good) { $temp=preg_replace('/([=!]==)/','',$rule); $temp=preg_replace('/([=<>!]=)/','',$temp); $good=(!(preg_match("/(=)/",temp)>0)); } //see if rule is valid if ($good) { $good=check_syntax('(' . $rule . ');'); } //check if rule only contains good functions, numbers, brackets, and math operators if ($good) { //break inside of () out of outsides $ruleparts=breakBracket($rule); //combine all parts together into 1 string $str=''; foreach ($ruleparts as $part) { $str.=$part . ' '; } //remove all valid functions global $whitefunc; //array of lower case functions user may use with () after name $str=str_replace($whitefunc,' ',$str); //remove all valid characters $str=preg_replace('|([0-9\(\)\+\-\*\/\%=])|',' ',$str); //if anything left call error if (preg_match_all("/([^ ])/",$str,$out)) { $good=false; } } return $good; } $rules=array( 'acos(56)+(ceil(56)-3+5)', 'sin(45)-35', 'a+$1*4', '1==1', 'hacked=true', ); echo '<table border="1">'; foreach($rules as $rule) { echo '<tr><td>' . $rule . '</td><td>'; if (testRule($rule)) { echo ' </td><td>Pass'; } else { echo ' </td><td>Fail'; } echo '</td></tr>'; } echo '</table>'; ?>
-
The following is my test preprocessing script so far. Any code before it can be run through the dangerous if (eval('return (' . $rule . ');')) { will pass. at the bottom $rules is an array of rules to test. It will print out the rule and if they pass or fail the test. Can anyone find a way to get a bad rule to pass this test or use this script itself to hack my server. If you have an idea how to improve is greatly appreciated also. <?php $whitefunc=array( 'abs()','acos()','acosh()','asin()','asinh()','atan()','atan2()','atanh()', 'base_convert()','bindec()', 'ceil()','cos()','cosh()', 'decbin()','dechex()','decoct()','deg2rad()', 'exp()','expm1()', 'floor()','fmod()', 'getrandmax()', 'hexdec()','hypot()', 'is_finite()','is_infinite()','is_nan()', 'lcg_value()','log()','log10()','log1p()', 'max()','min()','mt_getrandmax()','mt_rand()','mt_srand()', 'octdec()', 'pi()','pow()', 'rad2deg()','rand()','round()', 'sin()','sinh()','sqrt()','srand()', 'tan()','tanh()' ); function breakBracket($code,$a="(",$b=")") { $parts=array(); $end=strpos($code,$b); while (!($end===false)) { //find first start bracket before point $start=strrpos(substr($code,0,$end),$a); //find first end bracket after point $end=strpos($code,$b,$start); //break inner brackets out $parts[]=substr($code,$start+1,$end-$start-1); $code=substr($code,0,$start) . '|~-~|' . substr($code,$end+1); //find next search point $end=strrpos($code,$b); } $parts[]=$code; //replace '|~-~|' with brackets foreach ($parts as &$part) { $part=preg_replace("/\|~-~\|/", "()", $part); } return $parts; } function check_syntax($code) { return @eval('return true;' . $code); } function testRule($rule) { //replace $1,$2,$3... in rules with tag code parts $rule=preg_replace_callback( '/\$[0-9]+/', create_function( // single quotes are essential here, // or alternative escape all $ as \$ '$matches', 'return "53";' ), $rule ); //check for ilegal characters ;'" $good=(!(preg_match("/([;'\"])/",$rule)>0)); //check for assingments if ($good) { $temp=preg_replace('/([=!]==)/','',$rule); $temp=preg_replace('/([=<>!]=)/','',$temp); $good=(!(preg_match("/(=)/",temp)>0)); } //see if rule is valid if ($good) { $good=check_syntax('(' . $rule . ');'); } //check if rule only contains good functions, numbers, brackets, and math operators if ($good) { //break inside of () out of outsides $ruleparts=breakBracket($rule); //combine all parts together into 1 string $str=''; foreach ($ruleparts as $part) { $str.=$part . ' '; } //remove all valid functions global $whitefunc; //array of lower case functions user may use with () after name $str=str_replace($whitefunc,' ',$str); //remove all valid characters $str=preg_replace('|([0-9\(\)\+\-\*\/\%])|',' ',$str); //if anything left call error if (preg_match_all("/([^ ])/",$str,$out)) { $good=false; } } return $good; } $rules=array( 'acos(56)+(ceil(56)-3+5)', 'sin(45)-35', 'a+$1*4', ); echo '<table border="1">'; foreach($rules as $rule) { echo '<tr><td>' . $rule . '</td><td>'; if (testRule($rule)) { echo ' </td><td>Pass'; } else { echo ' </td><td>Fail'; } echo '</td></tr>'; } echo '</table>'; ?>
-
problem solved. use encodeURIComponent instead of escape.
-
function getXMLHttp() { var xmlHttp try { //Firefox, Opera 8.0+, Safari xmlHttp = new XMLHttpRequest(); } catch(e) { //Internet Explorer try { xmlHttp = new ActiveXObject("Msxml2.XMLHTTP"); } catch(e) { try { xmlHttp = new ActiveXObject("Microsoft.XMLHTTP"); } catch(e) { alert("Your browser does not support AJAX!") return false; } } } return xmlHttp; } function MakeRequest() //$request1,$request2,...,$callback=null { $request="av0=" + escape(arguments[0]); var xmlHttp = getXMLHttp(); if (arguments.length > 1) { for ($i=1;$i<arguments.length-1;$i++) { $request=$request + "&av" + $i + "=" + escape(arguments[$i]); } if (arguments[arguments.length-1]==null) { xmlHttp.onreadystatechange = function () {}; } else { eval("xmlHttp.onreadystatechange = " + "function () { if (xmlHttp.readyState == 4) {" + arguments[arguments.length-1] + "(xmlHttp.responseText);}};"); } } else { xmlHttp.onreadystatechange = function () {}; } xmlHttp.open("POST", "ajax", true); xmlHttp.setRequestHeader("Content-Type", "application/x-www-form-urlencoded"); xmlHttp.send($request); } The previous code is my AJAX script. I wrote it myself as a way to understand how it works and to deal with my sites strange structure. It works except when I pass a value to it with a + symbol the php sees this as a space probably because i use escape(arguments[$i]) to deal with the issue of some other special characters being in the input. Any ideas how to fix the script or process results in php so all characters can be passe?
-
Definitely eval will not be on the list of allowed commands.
-
Thanks for the input. I am going to go with a white list String Comparisons: http://www.w3schools.com/PHP/php_ref_string.asp Math Functions: http://www.w3schools.com/php/php_ref_math.asp Math Operators: -,+,/,*,%,&,| Comparators: <,>,=,! Symbols: $,0..9,(,),comma Any symbol as long as it is within single quotes would remove echo, and print functions from string list but otherwise this list should give a lot of versatility and I do not believe there is anything on there that is unsafe. Anyone see anything I have included that would be bad?
-
I know the following line of code is dangerous since the user has control of $query but I do not know any other way to realistically do it. if (eval('return (' . $query . ');')) { The perpose is to allow the user to type in any valid php statement and see if it evaluates true or false. I.E. 1==1 is true substr('cat',1,2)=='at' is true 12<6 is false the problem is I am sure there are ways a hacker could use this code to upload code to my site and take over. I have 2 options. 1 filter out any possible way a hacker could hack it or 2 only allow the user to enter functions that can't be used to hack it. I will probably go the later route but either way I need to know what to look for. Please let me know if you can think of any values for $query that would let you hack my site (p.s. this code is not up yet and will not go up until any possible security flaws are fixed.)
-
how to search for patterns with special characters?
mctrivia replied to mctrivia's topic in Regex Help
this may not be the best way but it works: $from=array('/\./','/\+/','/\*/','/\?/','/\^/','/\$/','/\[/','/\]/','/\(/','/\)/','/\|/','/\{/','/\}/','/\//',"/\'/",'/\#/'); $to=array('\\\.','\\\+','\\\*','\\\?','\\\^','\\\$','\\\[','\\\]','\\\(','\\\)','\\\|','\\\{','\\\}','\\\/',"\\\'",'\\\#'); $tag = preg_replace($from,$to,$tag); $this->code = preg_replace('/<TEMPLATE_' . $tag . '>/',$value . '',$this->code); goes through and adds a \ in front of all(I think) the special characters. -
The following code works as long as the $tag variable does not contain a $ symbole(and presumably other special symbols as well) how can I easily replace all special symbols with there regexp equivalents? Is there a useful function that I can't find like mysql_real_escape_string works for mySQL? $this->code = preg_replace('/<TEMPLATE_' . $tag . '>/',$value . '',$this->code);
-
can't figure out why recursive replace is not working
mctrivia replied to mctrivia's topic in PHP Coding Help
found the line that is not working. $this->code = ereg_replace('<TEMPLATE_' . $tag . '>',$value . '',$this->code); echo before and after show the values are there to be replaced but aren't getting replaced. -
if ($template->load($maintemplatefile,$values)) { //load every php file in the plugin folder //Handle invalid page Requests } else { echo '404 File Not Found'; //exit; } //print page code echo $template->html(); } I call the above code it prints out the template file but it does not recursively replace the <TEMPLATE_LOADTEMPLATE_$1> that is in the file even though it properly parses both the $tag and $value variables going to the $this->replace($tag,$value); line. Any idea why this does not work? <?php class Template { var $code=''; function load($file,$values) { if (file_exists($file)) { //get template directory $dir=substr($file,0,strrpos($file,'/')) . '/'; //get template $this->code=file_get_contents($file); //check if any recurseve templates to add $tags=$this->tags(); //process all internal template tags $newValues=array_slice($values,1); foreach ($tags as $tag) { //handle inserting new template file if (substr($tag,0,13)=='LOADTEMPLATE_') { $file=substr($tag,13); if ($file[0]=='$') { $file=$values[(substr($file,1)-1)]; } $temp=new Template; $filename=$dir . $file . '.xhtml'; if ($temp->load($filename,$newValues)) { $value=$temp->html(); } else { $value='File Not Found'; } $this->replace($tag,$value); } } return true; } else { return false; } } function tags() { preg_match_all('/<TEMPLATE_[^<]+?>/', $this->code, $tags); $tags=$tags[0]; foreach ($tags as $key=>$value) { $tags[$key]=substr($value,10,-1); } return array_unique($tags); } function replace($tag,$value) { $this->code = ereg_replace('<TEMPLATE_' . $tag . '>',$value . '',$this->code); } function replaceSection($tag,$value) { $replace='~<TEMPLATE_' . $tag . '>[\s\S]*?</TEMPLATE_' . $tag . '>~'; $this->code = preg_replace($replace,$value . '',$this->code); } function compress() { $this->code = ereg_replace("\n",'',$this->code); $this->code = ereg_replace(" ",' ',$this->code); $this->code = ereg_replace("\r",'',$this->code); } function html() { //remove <TEMPLATE_*> & </TEMPLATE_*> tags then return html. return preg_replace('/(<\/?)TEMPLATE_[^<]+?>/','',$this->code); } } ?> All code listed is available for use under GNU public license.
-
I have seen many web sites that will have there page set up with a directory structure instead of the ?x=f&a=s... for example shapeways has for each product a page like this: http://www.shapeways.com/model/194992/ They are not generating a folder and index.php for each product because you can type any file name you want in after the / and you will still get the same page. Was wondering how it is done as it makes much more human remember able addresses.