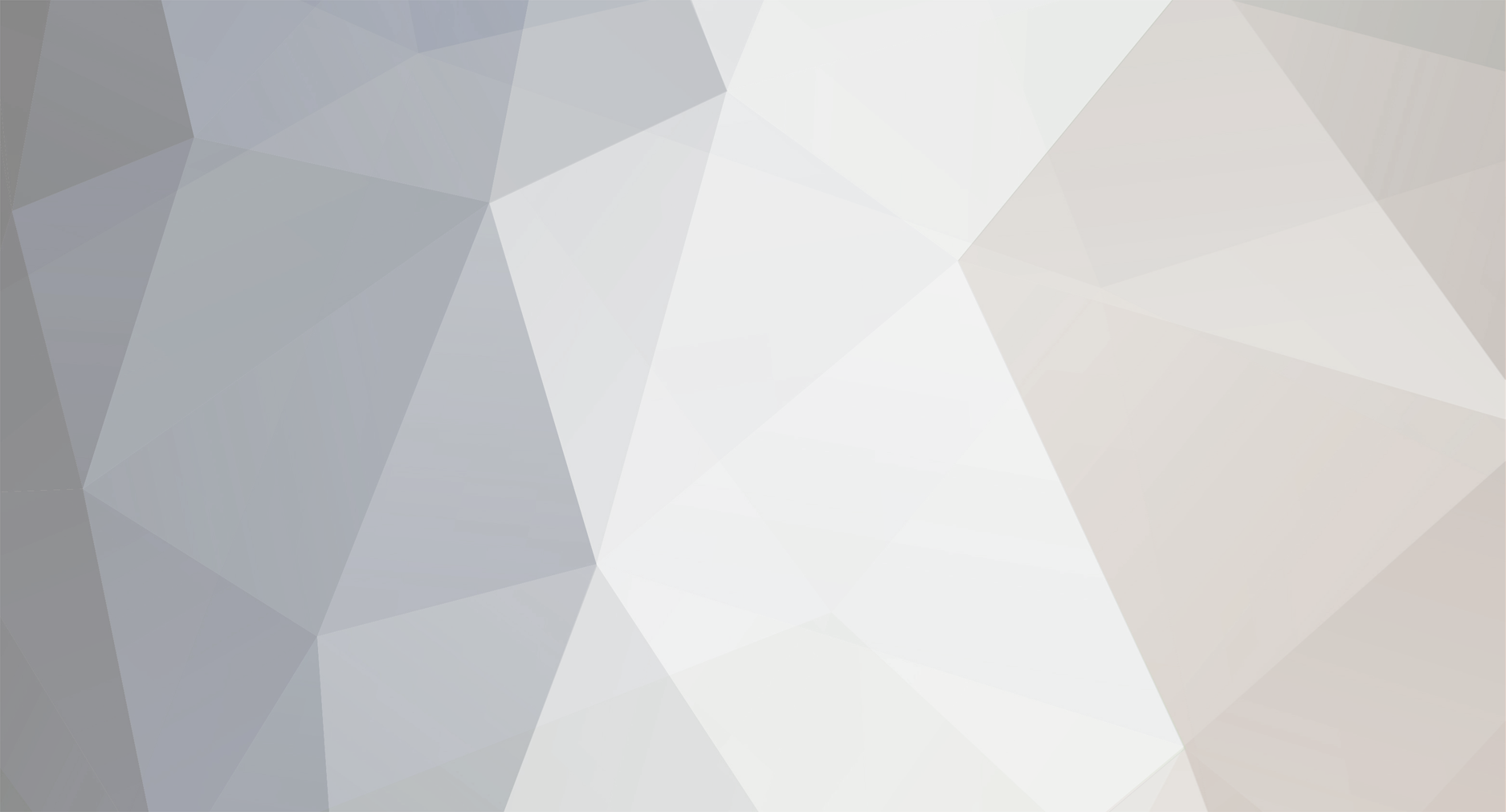
johnmerlino
Members-
Posts
71 -
Joined
-
Last visited
Never
Everything posted by johnmerlino
-
php_ini_loaded_file() says php.ini not loaded
johnmerlino replied to johnmerlino's topic in PHP Coding Help
thanks for response, display_error was set to Off, so I turned it on. -
Hey all, In this basic example: $db = new mysqli('localhost','root','pass','schools'); $query = "select * from schools where ".$type." like '%" . $term . "%'"; $result = $db->query($query); Let's say the $query local variable holds a string constructed of a mysql query that gets passed as argument to query method of mysqli instance. What exactly is the return value of query()? What is the type that is stored in the variable $result? I heard it called a "result object". Is a result object just a pointer to a mysql resource or something? Here: $num_results = $result->num_rows; for($i=0; $i < $num_results; $i++){ $row = $result->fetch_assoc(); } $result->fetch_assoc(); returns a record resource as an array $row = $result->fetch_object(); returns a record resource as an object; which is preferred? Can I work with array functions on the latter? thanks for response
-
name variable not being sent to server from form
johnmerlino replied to johnmerlino's topic in PHP Coding Help
Yeah this was silly error. I didnt realize I was using $_POST rather than $_FILE. -
php_ini_loaded_file() says php.ini not loaded
johnmerlino replied to johnmerlino's topic in PHP Coding Help
thanks for response, now that I copied the file into the new php.ini. Suddenly php stops raising exception errors and rather I just get a "server error" from the browser. Was some configuration setting reset? -
Hey all, I have a situation where there's multiple forms on a single page and when the user clicks the button, I don't want them to be navigated away from page but rather the transaction occurs on same page. Hence, everything occurs in a file called file_generator.php. I have these two forms: <form action="" method="post" enctype="multipart/form-data"> <tr> <td>Upload the data</td> <td><input type="file" name="file" /> </td> </tr> <tr> <td><input type="submit" name="upload" value="Generate List" /></td> </tr> </form> <form action="" method="post"> <tr> <td>Add Content:</td> <td><textarea rows="2" cols="20"></textarea></td> </tr> <tr> <td>Get the output:</td> <td><input type="submit" name="download" value="Get List" /></td> </tr> </form> Then when the form gets submitted it checks which form was submitted: foreach($_POST as $name => $value){ switch($name){ case 'upload': upload_data(); break; case 'download': download_data(); break; } } So if the user wants to upload a file, this function gets called: function upload_data(){ $file = $_POST['file']; if(isset($file)){ if(file_is_valid($file)){ echo "true"; } } else { echo "No file chosen"; } } For some reason, it triggers the else above, even though I selected a file, and that $file variable therefore should be set. Thanks for response
-
php_ini_loaded_file() says php.ini not loaded
johnmerlino replied to johnmerlino's topic in PHP Coding Help
Thanks for response, but from the unix shell, I did the following: cd ~ cd /etc/ ls php.ini.default hp.ini.default-5.2-previous So there was no php.ini per se. Hence, I did the following: mate php.ini.default sudo touch php.ini (copied content from php.ini.default into php.ini and then saved file) When I listed the /etc contents, the php.ini was now in there: php.ini php.ini.default php.ini.default-5.2-previous Yet, under Loaded Configuration File, it still says none. Platform is Mac OSX Thanks for response -
Hey all, this function returns 0, rather than a string consisting of html tags: function cost_per_distance_table($distance,$divisor){ $str = ""; $str += "<table>"; $str += "<tr>"; $str += "<td>Distance</td>"; $str += "<td>Cost</td>"; $str += "</tr>"; while($distance <= 250){ $str += "<tr>"; $str += "<td>" . (string)$distance . "</td>\n"; $str += "<td>" . (string)($distance/$divisor) . "</td>\n"; $str += "</tr>"; $distance += 50; } $str += "</table>"; return $str; } echo cost_per_distance_table(50,10); Not sure why. Thanks for response.
-
php_ini_loaded_file() says php.ini not loaded
johnmerlino replied to johnmerlino's topic in PHP Coding Help
In terms of configuration it says this: Configuration File (php.ini) Path /etc Loaded Configuration File (none) It says none for loaded configuration file. If this is issue, how do I correct it? Thanks for response -
Hey all, So I was have common problem with date() function in php5.3. Someone said the error message suggests that your php.ini settings have 'EDT/-4.0/DST' set as your timezone setting and should rather be 'America/New_York' instead. Now i have two php.ini files: php.ini.default php.ini.default-5.2-previous. running phpinfo() just tells me that the php.ini is located in etc but doesn't mention which php.ini is in use. So to figure out which one, I run this: $inipath = php_ini_loaded_file(); if ($inipath) { echo 'Loaded php.ini: ' . $inipath; } else { echo 'A php.ini file is not loaded'; } And this outputs the else statement "not loaded". How could the php.ini not be loaded? thanks for response
-
Hey all, I deselect a checkbox. Then click update. Yet the only thing that gets passed in the post array are the items that are already checked previously. How can I pass the item that was unchecked into the array? Thanks for response
-
iterating through array while passing an index
johnmerlino replied to johnmerlino's topic in PHP Coding Help
thanks for response -
Hey all, the foreach loop allows you to iterate through an array and pass an index: foreach($array as $key=>$value) { // $value is the current item in array and $key is index } I want to do the same thing but add the functionality as part of my Enumerator class: class Enumerator { public $arr; public function __construct($array=array()) { $this->arr = $array; } public function each($lambda) { array_walk($this->arr, $lambda); } } So for example now I can do this: $elements = new Enumerator(array('email','fax','phone','postal mail')); $elements->each(function($l){ echo form_checkbox("checked[]",$l); }); But I may want to do this: $elements = new Enumerator(array('email','fax','phone','postal mail')); $elements->each_with_index(function($l,$i){ if($i % 2 == 0){ echo form_checkbox("checked[]",$l); echo "</br>; } else{ echo form_checkbox("checked[]",$l); } }); thanks for response
-
Hey all, I have method like this: echo tinymce_tag($post->body); Basically the body property contains a string from database containing one or many paragraphs delimited by p tags: <p>Hello World.</p> <p>Goodbye World.</p> I want to give all the paragraphs a class. This function will give the first p tag a class: if ( ! function_exists('tinymce_tag')){ function tinymce_tag($content = ''){ $pos = strpos($content, '<p>'); if($pos !== false){ $content = substr_replace($content,' class="paragraph_styler"', 2, 0); } return $content; } } But I am not sure how to give all the p tags in the content variable a class. Thanks for response.
-
Thanks for response. Why did PHP not thrown an exception error "undefined variable $user on line such and such". The backtrace should have caught that one. Or can you create properties on the fly in PHP? If so, that sucks because that will happen a lot.
-
user is only a local variable in the foreach loop that refers to users, because $this->users is an array of users, so we iterate through them one at a time. Sure that's the issue?
-
Why does this return null rather than 'john must write the book'? <? abstract class User { protected $name; protected $role; function __construct($name,$role){ $this->name = $name; $this->role = $role; } abstract function run_errand(); } class Author extends User { public function run_errand(){ echo $this->name . "must write the book"; } } class Editor extends User { public function run_errand(){ echo $this->name . "must edit the book"; } } class Boss { private $users = array(); public function review(User $user){ $this->user[] = $user; } public function delegate(){ if(count($this->users)){ foreach($this->users as $user){ if($user->role == "author"){ echo $user->run_errand(); } } } } } //client code $boss = new Boss(); $boss->review(new Author('John','author')); var_dump($boss->delegate()); //returns null ?>
-
Hey all, I have two objects that contain object instances. I want to compare the properties of the instances and if the old_parent's property doesn't exist as one of the $new_parent's property, then I place the old_parent's property in an array called unneeded_ids. Problem is when I use nested foreach to loop through the instances of the two objects, despite old_parent having only one instance with a property id of 1, because it loops twice, the array gets two "1" values rather than just one: public function update(){ $vanity_url = new VanityUrl(); $vanity_url->where('user_id',$this->current_user()->id)->get(); $zones = $this->input->post('zones'); $zone = new Zone(); $zone->where_in('name', $zones)->get(); $subcategory = new Category(); $subcategory->where('id',$this->uri->segment(4))->get(); $old_parents = new Category(); $old_parents = $subcategory->related_category->get(); $unneeded_ids = array(); if(!$this->input->post('catupload')){ if($subcategory->save($zone->all)){ redirect("blogs/$vanity_url->url/categories"); } else { echo "Error occurred. Please try again."; } } else { $new_parents = new Category(); $controller = $this->input->post('catupload'); $new_parents->where_in('controller',$controller)->get(); foreach($new_parents as $new){ foreach($old_parents as $old){ if($new->id != $old->id){ array_push($unneeded_ids, $old->id); } } } var_dump($unneeded_ids); } } The var_dump outputs: array(4) { [0]=> int(126) [1]=> int(127) [2]=> int(126) [3]=> int(127) } but it should only output: array(2) {[0]=> int(126) [1]=> int(127)} That's because there's only one object instance of old_parent that has a property id value of 126 and the same for 127. The nested for each loop is causing it to iterate twice against the same object and sticking it into the array. How do I get desired result? Thanks for response.
-
Hey all, I would like to know the best way to determine whether a value has been changed in a multi select transfer. For example, I have two select fields. User clicks on button to add an item to the right select element with id of select2 from the left select element with an id of select1. When they click update, it posts to a php method. This is where I am uncertain what to do. How can I check that they indeed added or removed items from the right select element and if they did, determine which ones were removed or added to make the reflected changes in the database? This is what I have, but it's bad solution because it deletes all related records before it adds the ones it finds to the right select element: public function update(){ $subcategory = new Category(); $subcategory->where('id',$this->uri->segment(4))->get(); $subcategory->delete($subcategory->related_category->get()->all); $new_parents = $this->input->post('catupload'); $parent_category = new Category(); $parent_category->where_in('controller',$new_parents)->get(); $subcategory->save($parent_category->all); } This is what the form elements look like: echo "You can add or remove one or many parents associated with this category:"; echo "<div class='multiselect-transfer'>"; echo form_multiselect('catadd[]',$other_cats,'','id="select1"'); echo anchor('#','add >>','id="add"'); echo "</div>"; echo "<div class='multiselect-transfer'>"; echo form_multiselect('catupload[]',$default_cats,'','id="select2"'); echo anchor('#','<< remove','id="remove"'); echo "</div>"; echo form_submit('submit','Update'); echo form_close(); $other_cats variable holds array of categories that have not been asssociated with the current category being updated when this category was first created. The $default_cats variable holds an array of categories that have been associated with the current category being updated when this category was first created. And the javascript: $().ready(function() { $('#add').click(function() { return !$('#select1 option:selected').remove().appendTo('#select2'); }); $('#remove').click(function() { return !$('#select2 option:selected').remove().appendTo('#select1'); }); }); Thanks for response.
-
all my posts are being generated for all the categories
johnmerlino replied to johnmerlino's topic in PHP Coding Help
I decided to create the category_id in posts. Makes things a whole a lot easier. -
Hey all, I have a relationship where a zone has many posts and a category has many zones but a zone can also have many categories. The zones are basically parts of a page, such as the main area and sidepanel stored in database as records with name fields such as 'panel' and 'main'. There's a serious flaw right now and I would like to know the best solution to address it. Right now I access the posts of a zone of a category like this: $category->zone->get()->post->get(); The above ends up displaying all the posts in the database for any given category because when category is selected, it searches for the associating zones, and then selects all posts related to those zones, which is wrong. i want to select all posts of the zones associated with that particular category. Current database: posts id zone_id zones id name categories_zones id zone_id category_id categories id What should I do? Add a category_id column to the posts table and relate the post directly to the category as well as directly to the zones table so the post table would have two foreign keys: category_id and zone_id Would this be the most effective thing to do given my requirements? Thanks for response.
-
Hey all, If I want to have a category which can have subcategories, which in turn can have its own subcategories or belong to the parent category, and the subcategories can have more than one parent categories, is the most effective way to design this is to create a categories table and categories_join table where the categories table has a foreign key categories_join_id linking it to the categories_join table and the categories_join table has a field called parent_id which associates with the primary key of the categories table, therefore allowing me to have multiple subcategories to one category: categories_join table id parent_id 1 2 1 3 categories table id categories_join_id 1 1 2 2 3 3 So from the example above, the first category has two parents, category 2 and category 3. As an alternative option, in this post: http://stackoverflow.com/questions/5384183/database-design-question-categories-subcategories The second answer down mentions to use recursive programming? But he doesn't give an example of what he means. Is he saying you have a function call itself passing it parameters as to what the parent should be: function getParent($category,$parents = array()){ } Thanks for response.
-
dynamically generated data crawlable by google?
johnmerlino replied to johnmerlino's topic in PHP Coding Help
So if there is no home.php file, but rather when a user clicks home.php link on the browser, a method gets called in let's say a categories class which populates a generic file called show.php with data, how does the crawler know to what the content is before the method even gets called? Thanks for response.