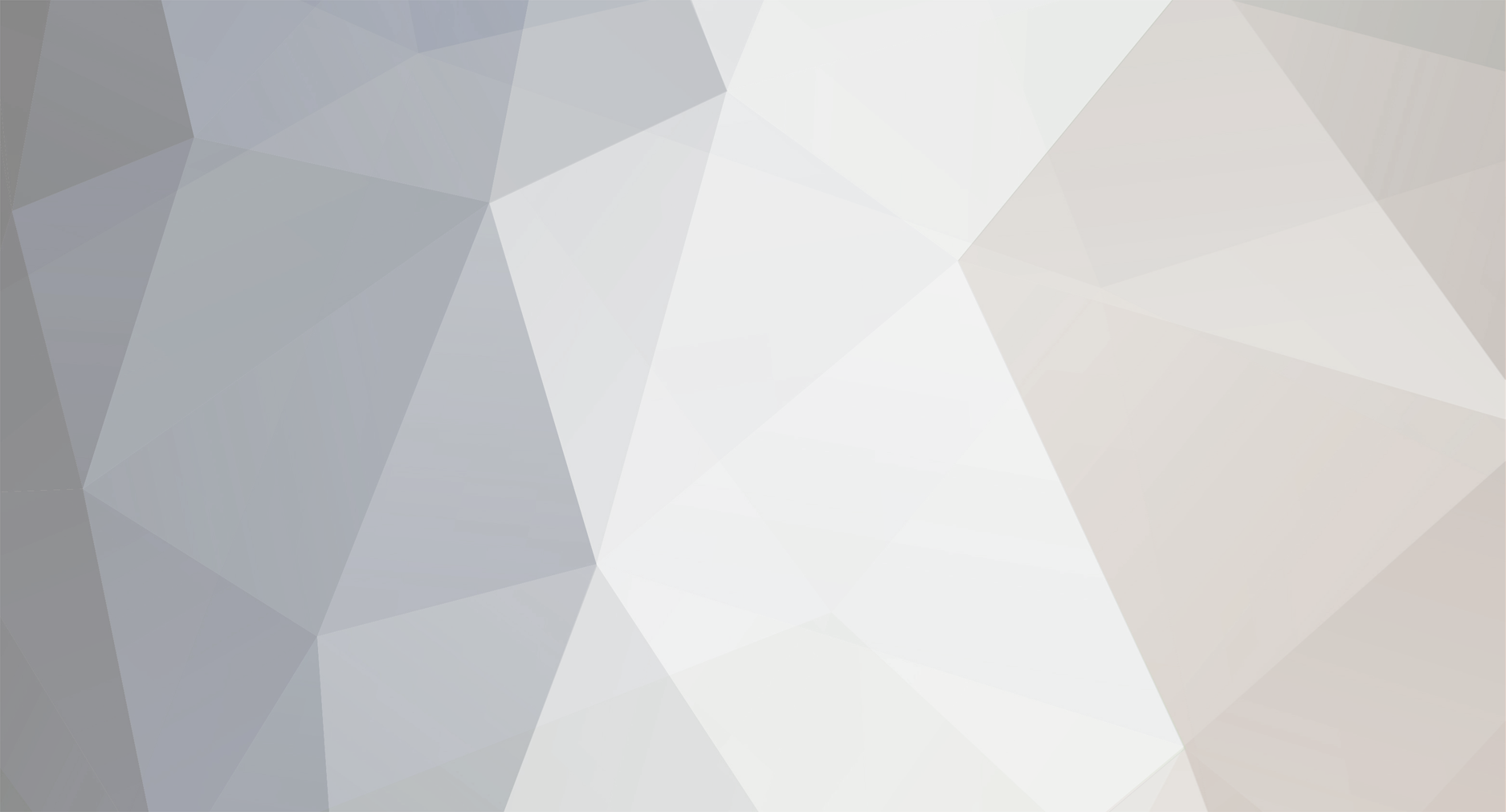
linus72982
Members-
Posts
96 -
Joined
-
Last visited
Everything posted by linus72982
-
I believe you have to use "NOT" instead of != within an sql query.
-
It might be a problem with the quotes, though I didn't look too deeply, try putting quotes around every instance of EMAIL_REGISTERED and USERNAME_REGISTERED. Right now it appears you are returning constants instead of strings. If that isn't the problem, you have to realize that what the script is doing is telling you exactly where the problem is. It is telling you that: $this->isAccountRegistered($AccountUsername, $AccountEmail) == USERNAME_REGISTERED and: $this->isAccountRegistered($AccountUsername, $AccountEmail) == EMAIL_REGISTERED are both false so it is running the else portion every time. To test this theory, put this as your else: else {echo 'This is where the problem is'; die(); } If you see that text when you run it, it means what i said above is true. This tells us the problem with your code is either in the syntax of your if and elseif or, more likely, your code in the isAccountRegistered function is bad in some way.
-
Well, I'd think you'll have to change up something a bit to get there. Instead of echoing out the results in the search.php page, let's put them into an array and then store it in session. There might be a better way, but I'm tired. Alright, so: Change the bottom of search.php (the while section) to something like this: session_start(); $i=1; while ($row = mysql_fetch_array ($result)) { $_SESSION['names'][$i] = "Name: {$row['name']} " . "{$row['lname']} <br>" . "Email: {$row['email]} <br>"; $i++; } Now, on your display page your textarea tag is going to look something like: <textarea value="<?php for ($i=1; $i<=count($_SESSION['names']); $i++){echo $_SESSION['names'][$i]\n;} ?>"></textarea> Somewhere around there, this is untested code, but it's either good or in the ballpark. *EDIT: remember to add <?php session_start; ?> to the first line of your "page.html" if it isn't already there or you won't be able to pull the session variables.
-
multiple submit buttons - how to show usernames instead of userid numbers
linus72982 replied to jasonc's topic in HTML Help
I see one error I wrote, the first piece of code should actually be: echo '<input type="submit" name="submitter" value="'.$userid.'->'.$username.'" />';} ?> Notice I added '; to the very end of the line. Small error, but there nonetheless. -
multiple submit buttons - how to show usernames instead of userid numbers
linus72982 replied to jasonc's topic in HTML Help
You don't have to use buttons. You can have multiple submits in a form and differentiate which was clicked. Try something like: <?php for ($i=1, $i<=$numberOfUsers, $i++){ echo '<input type="submit" name="submitter" value="'.$userid.'->'.$username.'" />} ?> and then have something like this in the processing script: $temp = stripos($_POST['submitter'], '->'); $userID = substr($_POST['submitter'], 0, $temp); $temp = $temp + 2; $userName = substr($_POST['submitter'], $temp); At least that's how I'd do it, now you have the userID and the userName in the processing script of the button they pressed. I didn't test the code, there might be little errors, but it should work overall (*should*). -
Just have it store offline information in a file and then when it discovers a connection online it can update the online database.
-
Actually, thinking on it more, you could even have it display correctly and print differently with CSS style switching based on print action.
-
I bet you could work up a script that could determine the length of the table, figure out how many full rows you can fit on a page even if the height of the rows are variable and then break the table after that to continue on the next page. Do you know enough of PHP to do this yourself or do you need a push in the right direction? Of course, this method would display the table in an odd way, with a few empty rows or whitespace every page, but it should solve your problem.
-
how to get details of an unregistred user ???
linus72982 replied to sheraz's topic in PHP Coding Help
What kind of information are you wanting? You can grab the IP address and a few browser specifics, etc, but you can't grab their name or anything. You could always try to grab their information through an API of one of the many many many systems people are logged in to at once (Facebook, gmail, twitter, etc), but that requires them, of course, to be logged into one and they would have to approve your access to their information. -
From php.net: mysql_real_escape_string — Escapes special characters in a string for use in an SQL statement Yep, it escapes the quotes. PHP.net recommends you use this in place of addslashes() if possible.
-
mysql_real_escape_string($CONTENT, $CONNECTION); Actually, I'm not positive that will escape ANDs, but you should use that on all input anyway.
-
How can i Include an array from another php file?
linus72982 replied to Jarid's topic in PHP Coding Help
I think you'll need to include: global $Ratethese; in your first file before you assign. Or maybe not, who knows, I've never been good at encapsulation and scope. -
Try this instead: if (isset($_GET['button'])){ To prevent the auto-redirect.
-
Oh, and I overlooked this at first, you'll want the top to look like this to prevent the code from triggering the first time the person visits the page: <?php if (isset($_GET)){ $newurl = "Location: http://chaos67731.com/demo/".$_GET['button']; header ($newurl);} ?> Hope that didn't cause too much headache, I was lost in a fog yesterday
-
Try changing the "height: 500px;" to "min-height: 500px;" in the contentmiddle section. You can't define a definite height and still have it stretch. Min-height should do what you want.
-
Is there a good, simple program out there to visually design your OOP? I've found a few but they are way too complex and seem to be made for enterprises and huge business projects, etc. I just want a simple visual bubble system where I can lay out attributes and methods and maybe arrows between objects, etc. Thanks for any leads.
-
If I understand you correctly, you'll want something like this: <?php $newurl = "Location: http://chaos67731.com/demo/".$_GET['button']; header ($newurl); ?> <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <meta http-equiv="Content-Type" content="text/html; charset=utf-8" /> <title>Demo Page</title> <style type="text/css"> html { background:#799ABC url(images/site-bg.png) repeat-x top left; } #main { width:700px; height:640px; margin:50px auto; border:0px solid #111; background:url(images/logo-bk.png) no-repeat; } #main .opening { padding-top:250px; font-weight:bolder; text-align:center; text-transform:uppercase; } </style> </head> <body> <div id="main"> <p class="opening">To get to your demo splash page enter you client id in the search field below.</p> <p> <center> <form method="get" action="http://chaos67731.com/"> <input id="button" name="button" type="text" /> <input type="submit" value="SEARCH" /> </form> <strong> <?php echo "$newurl"; ?> </strong> </center> </p> </div> </body> </html> I was just assuming the page URL for where this page is located was http://chaos67731.com/ If it is something different, you'll want to change the action= portion of the form tag to equal whatever the URL is. The thing is, you want the submit to process at the top of this very page and then header to the website you want. That is accomplished at the top with the parsing of the GET variable into the header, but first the submit has to point right back to the page so it will essentially "refresh" and start from the beginning.
-
You confused me, wouldn't $_POST['titel'][$n] be a multidimensional array? It seems like he's actually setting the name to something explicit, the variable being added to the text of the name. If $n were 1, the name of the element would be titel1, for 2 it would be titel2, from there, $_POST['titel2'] should grab it. It seems like $_POST['titel'][2] would never be set. I'm still fairly new at all this, could you explain how you got here?
-
Well, as I'm still not a complete expert, I may just not be seeing the problem, but now that I read the code a bit more I think the problem is that you've made all the <a> elements blocks with display:block which makes them 100% width and therefore trying to text-align them won't do anything. Here's what I would do: move the class attributes I gave you earlier from the <li> elements to the <a> elements that you'd like to align and then change the css to a.alignedText instead of li.alignedText. See if that works. Outside of that, we might need to wait for someone else to help out :S
-
Post the edited CSS if you could please.
-
Ideally you would want to make the "curtains" a background for the wrapper div and then it will extend down to fit the content. A background image on repeat will only go as far as the height, if you don't define a height and have nothing in the div (a background image doesn't count as an item in a div) then it won't have any height. As you add things to the div, the height shrink wraps to fit and then applies whatever background it can. I would suggest making an image the width of your wrapper and maybe a height of 30px or something, then paint the edges of that image so that when you repeat the image down it appears to be curtains, then just add this as the background image of your wrapper and put in background-repeat: repeat-y; Any reason that wouldn't work? I can't think of any other way to dynamically change the height of an element based on the height of another element, I think you'll have to nest it.
-
Gotcha, yeah, the class attribute should work for what you need, it's a way to demarcate items that otherwise would be captured in the same style. Another way you could do it, though frowned upon, is to add the alignment to the inline style of each element, but that's far more typing than you need and best practice is to separate content and layout to the maximum extent possible.
-
If I read correctly what you were trying to do, just add a class to the LI portions you want to text-align and don't put the class on the portions you don't want to text align. Add: class="alignedText" to each of the elements to be aligned and then add: li.alignedText {text-align: center;} (or whatever alignment you want) section to your CSS. Is this what you meant?
-
I didn't delve too far into the script, but I think your problem is with this line: $titel = $_POST['titel[$n]']; From my quick once-over, I think it should be: $titel = $_POST['titel['.$n.']']; The difference is the '. and .' added before and after the $n. As it is part of a string, I don't think you can just insert a variable like that without adding it to the string.
-
I got to thinking about it more and decided I'd show you how I'd design the page you showed with CSS. I'm doing this according to your first image there. It appears you have three main divs you can work with. First, you have a wrapper, this will encompass everything else and it can limit the width of your displayable content. Place everything in these div tags. Then you have two columns, left and right. You can then place your divs inside of these "columns". Something like this: <div id="wrapper"> <div id="leftColumn"> <img src="buyNowPicture" /> <div id="topBlock"></div> <div id="middleBlock"></div> <div id="bottomBlock"></div> </div> <div id="rightColumn"> <img src="mainHeader" /> <div id="mainViewableArea"></div> <span id="viewableBottomText"></span> </div> </div> Now for the CSS: #wrapper: {width: 900px; max-width: 900px; min-width: 900px; margin: 0 auto;} #leftColumn {width: 300px; float: left;} #topBlock {width: 280px; height: 200px; margin: 0 auto;} #middleBlock {width: 280px; height: 230px; margin: 0 auto;} #bottomBlock {width: 280px; height: 150px; margin: 0 auto;} #rightColumn {width: 600px; float: left;} #mainViewableArea {width: 580px; height: 500px; margin: 0 auto;} #viewableBottomText {text-align: center; font-size: 16px; font-weight: bold;} You get the picture. Basically, what you need to know is you have two columns, they are floated left, and then a wrapper that encompasses both and centers everything on the page. You can add background images as necessary to show the borders of the blocks and such.