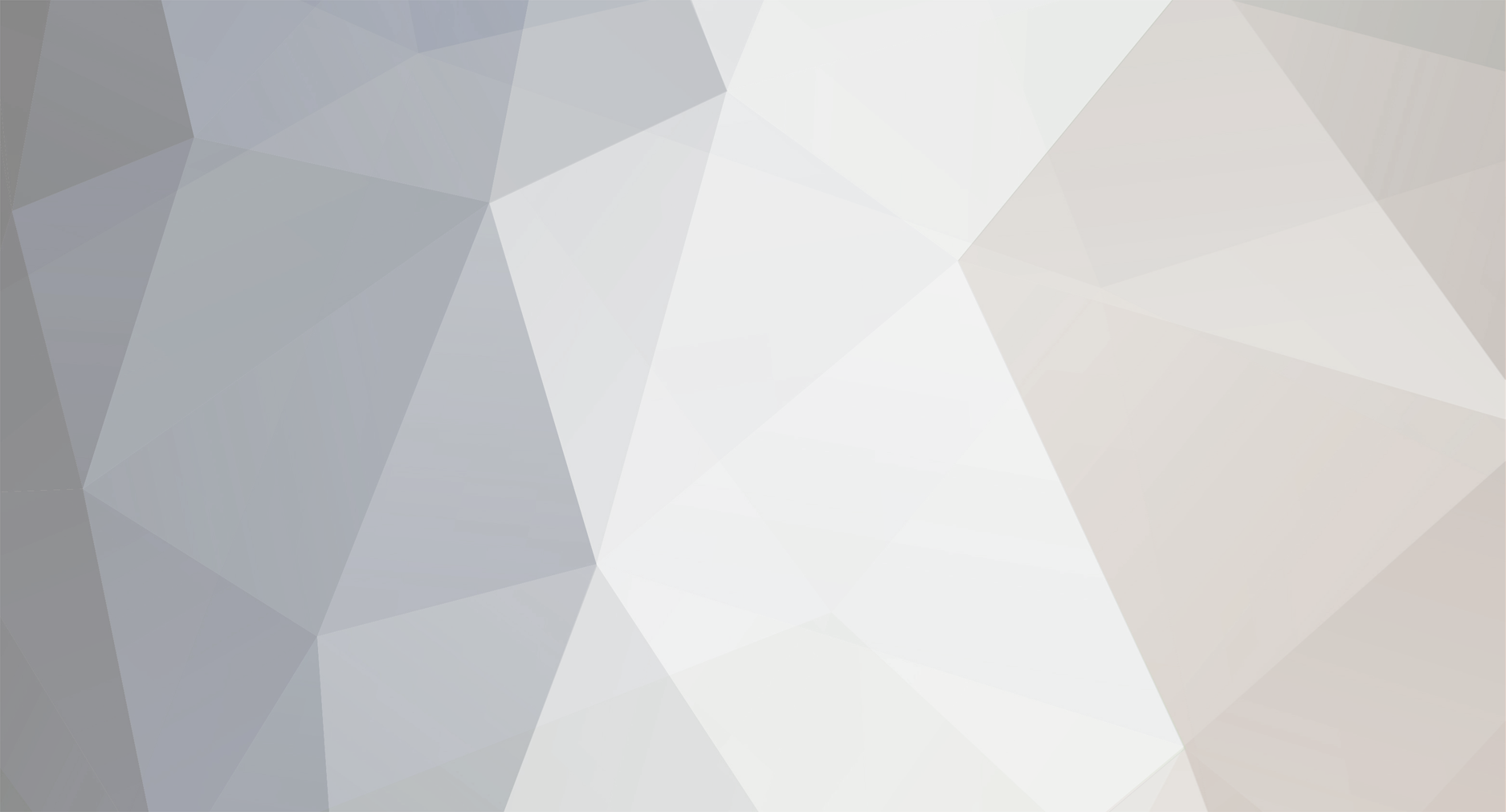
fishbaitfood
Members-
Posts
97 -
Joined
-
Last visited
Never
Everything posted by fishbaitfood
-
Hello all, I'm currently finding myself in the situation where my code on localhost works perfectly, but not on my domain. What my code is about: - enter a customer name in a textfield - (for selecting a customer name: autocomplete script which gathers the customer names from a database through a file called "customers.php") - jquery $.ajax customer name submit, to file "get-customer-data.php" which json_encode outputs additional data of the entered customer - success in $.ajax to fill other textfields with the json data And this WORKS on my localhost perfectly! But on my domain I get the error in this topic title. So far, I've figured out that the json_encode function won't output anything on my domain. So I guess $.ajax is receiving that "null" value, right? This is the php file that outputs json_encode additional data, to be received by $.ajax: <?php if (strlen($_POST['customer']) > 0) { $customer = $_POST['customer']; $select_customer_data = "SELECT address, city, country FROM customers WHERE customer = '$customer';"; include_once('connection.inc.php'); $con = mysql_connect(MYSQL_SERVER, MYSQL_USERNAME, MYSQL_PASSWORD) or die ("Connection failed: " . mysql_error()); $dbname = "mydatabase"; $select_db = mysql_select_db($dbname, $con) or die ("Can't open database: " . mysql_error()); $get_customer_data = mysql_query($select_customer_data) or die ("Query failed: " . mysql_error()); $customer_data = mysql_fetch_array($get_customer_data); $customer_address = $customer_data['address']; $customer_city = $customer_data['city']; $customer_country = $customer_data['country']; $output = array('address' => $customer_address, 'city' => $customer_city, 'country' => $customer_country); echo json_encode($output); } ?> And the webpage: <!DOCTYPE html> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=utf-8"> <!--[if lt IE 9] <script src="http://html5shiv.googlecode.com/svn/divunk/html5.js"></script> <![endif]--> <script type="text/javascript" src="http://ajax.googleapis.com/ajax/libs/jquery/1.6.2/jquery.min.js"></script> <script type="text/javascript" src="http://ajax.googleapis.com/ajax/libs/jqueryui/1.8/jquery-ui.min.js"></script> <script type="text/javascript" src="js/autocomplete.js"></script> <title></title> <script type="text/javascript"> $(document).ready(function(){ $("#input_customer").autocomplete("customers.php", { minChars:1, width:186 }); $(".btn-submit").click(function() { var customer = $("#input_customer").val(); var dataString = "customer="+customer; $.ajax({ type: "POST", url: "get-customer-data.php", data: dataString, dataType: "json", success: function(data) { $(".input-address").val(data.address); $(".input-city").val(data.city); $(".input-country").val(data.country); } }); }); }); </script> </head> <body> <div class="fact-to"> <h3 class="fact-to-heading">Customer data</h3> <div class="fact-to-contact-box"> <input type="text" id="input_customer" name="customer" /> <input type="image" src="images/btn-submit.png" class="btn-submit" title="Get customer data" /> <input type="text" class="input-address" /><br /> <input type="text" class="input-city" /><br /> <input type="text" class="input-country" /> </div> </div> </body> </html> ps: when using $.ajax, it isn't necessary to use form tags, right? (besides validation) Don't think that's causing the javascript error. Why won't the exact same thing work on my domain, when it does on localhost?
-
If I use classes, that's about the same principle, but doesn't work at all. $("#button").toggle( function() { $(this).mousedown(function() { $(this).addClass("unchecked-highlight"); }); $(this).mouseup(function() { $(this).addClass("checked"); }); // other actions (won't affect issue) }, function() { $(this).mousedown(function() { $(this).addClass("checked-highlight") }); $(this).mouseup(function() { $(this).addClass("unchecked"); }); // other actions (won't affect issue) } ); #button { width: 120px; height: 35px; display: block; background: url('../images/check-sprite.png') no-repeat; background-position: 0 0; /* doesn't matter if I remove this */ } .unchecked { background-position: 0 0; } .unchecked-highlight { background-position: 0 -25px; } .checked { background-position: 0 -50px; } .checked-highlight { background-position: 0 -50px; } Now the button background doesn't change at all.
-
Doesn't toggle "count" two different clicks? Because I need those two different clicks, because my button background-image has 4 different states, so I'm using a sprite image. And if I put the mousedown and mouseup outside my toggle, I can only have 2 different states. Isn't this possible some way? Do I need to make a function which checks the toggle function state, or something like that?
-
Hello there, I have this code: $("#button").toggle( function() { $(this).mousedown(function() { $(this).css({ backgroundPosition: "0 -25px" }); }); $(this).mouseup(function() { $(this).css({ backgroundPosition: "0 -50px" }); }); // other actions (won't affect issue) }, function() { $(this).mousedown(function() { $(this).css({ backgroundPosition: "0 -75px" }); }); $(this).mouseup(function() { $(this).css({ backgroundPosition: "0 0px" }); }); // other actions (won't affect issue) } ); And the mouseDown and mouseUp functions ONLY work AFTER the first time I clicked my button. How do I solve this? Thank you.
-
Hi all, So I want to fill a table with rows (obviously), with jQuery, when clicking on a list-item label. But clicking on the same button also needs to remove the same row. Therefor I generated a classname for each row, based on the list-item label's html text. But with the code I have now, it won't remove the row. $("ul.checklist label").toggle( function() { var product = $(this).html(); $("table tr:last").after("<tr class=\"tr-"+product+"\"><td>"+product+"</td></tr>"); }, function() { var product= $(this).html(); // Guess this won't work, right? $("table tr.tr"+product).remove(); } ); Thanks.
-
How to display styled xml generated by a .asp link?
fishbaitfood replied to fishbaitfood's topic in PHP Coding Help
Thanks thorpe! Just what I needed! So many useful PHP functions I haven't discovered yet... -
How to display styled xml generated by a .asp link?
fishbaitfood replied to fishbaitfood's topic in PHP Coding Help
Bump. :-\ Is there any other way to retrieve the XML output? Which lets me style it the way I want? -
Hello guys, I want to make an xml feed into my webpage, but the xml is generated from an .asp file on another website, so it's not actually an xml file, but xml output. Now, I want to retrieve this xml output on my webpage, but I'm having difficulties while doing this. What I've tried so far: - With PHP: <?php echo file_get_contents("http://link-on-other-website.asp?XML=1"); ?> - With javascript: <script type="text/javascript"> if (window.XMLHttpRequest) {// code for IE7+, Firefox, Chrome, Opera, Safari xmlhttp=new XMLHttpRequest(); } else {// code for IE6, IE5 xmlhttp=new ActiveXObject("Microsoft.XMLHTTP"); } xmlhttp.open("GET","http://link-on-other-website.asp?XML=1", false); xmlhttp.send(); xmlDoc=xmlhttp.responseXML; document.write("<table border='1'>"); var x=xmlDoc.getElementsByTagName("RECORD"); for (i=0;i<x.length;i++) { document.write("<tr><td>"); document.write(x[i].getElementsByTagName("NAME")[0].childNodes[0].nodeValue); document.write("</td><td>"); document.write(x[i].getElementsByTagName("DATE")[0].childNodes[0].nodeValue); document.write("</td></tr>"); } document.write("</table>"); </script> When using the PHP code, I can retrieve it obviously, but I get an <?xml ... > declaration within my body, which is not allowed. When using the Javascript code, I can't even retrieve anything. And the goal is to style this xml output to match my website. That's the whole purpose of this xml link, because I couldn't style the iframe I had earlier. Can someone help me on this, on how to properly embed this asp generated xml output, within my HTML5 document? ps: I've asked for the xml-feed link from that other website, so using the above PHP code is legit in my case.
-
Dang, it's really that simple huh?! It worked. I just needed to remove that header('Location: index.php') in my process page. Weird that I haven't tested this earlier... :-\ Thanks!
-
So this line should do it? $.post('process.php', $("#myform").serialize()); But the header('Location: index.php') when the process is complete, will cause a refresh I guess, right? :-\
-
Thanks for your reply! But in all scripts I've seen so far, to send a form with ajax, it needs an "output" back, right? In my case, I just want to send the form, only send. My process page has a header('Location: index.php'), to get back. But I guess that won't work, right?
-
Anybody can help me with this?
-
Hey all, I have this form I'd like to submit without a page refresh. Someone said it was as simple as using the Ajax .request() function.. Seems unlikely to me? So basically, I have this form with data, which will be inserted into a database after submit, and instantly goes back to the form page using header('Location: index.php'). Besides the refresh, this works perfectly. Is it possible, to post my form without a refresh, but still submitting data to the process page where the data is inserted into tables and brings me back with header? So Ajax or jQuery doesn't need to fetch the output from the process page, but just post it. Sure this is possible, right? I've tried to find examples on my situation, but I haven't found one. Thank you.
-
Explanation inside code, I did my best. I think there are some ways to make it more efficient, but this is the most logical to me for now. (Best viewed when copied to your code editor) <?php $check_customers = "SELECT * FROM customers;"; // This is for incrementing Customer-ID and Project-ID when Customer(name) is new $check_customer = "SELECT * FROM customers WHERE customer = '$customer';"; // To check if the entered Customer already exists $check_checklist = "SELECT * FROM checklist WHERE customer = '$customer';"; // This is for incrementing Project-id when Customer exists $check_last = "SELECT added FROM customers ORDER BY added DESC LIMIT 1;"; // To check if current year is "bigger" than latest Customer's added year $result_customers = mysql_query($check_customers) or die ("Query failed: " . mysql_error()); // See above $result_customer = mysql_query($check_customer) or die ("Query failed: " . mysql_error()); // See above $result_checklist = mysql_query($check_checklist) or die ("Query failed: " . mysql_error()); // See above $result_last = mysql_query($check_last) or die ("Query failed: " . mysql_error()); // See above $num_customers = mysql_numrows($result_customers); // See above $row_customer = mysql_fetch_array($result_customer); // See above $num_checklist = mysql_numrows($result_checklist); // See above $row_last = mysql_fetch_array($result_last); // See above $date = date('Y-m-d'); // Get current date $year = date('Y'); // Current year // Starting variables $project = (int) ((string)$year . '00011'); // Project-ID, consists of current year + 5 digits ,, last digit is for incrementing when Customer is already in table $id = (int) substr($project, 0, ; // Customer-ID = Project-ID without last digit ,, last digit is for incrementing when Customer is new if (($num_customers < 1) || ($year > (int) substr($row_last['added'], 0, 4))){ // Check IF Customers table IS EMPTY ,, OR ,, Current year is newer than last Customer's year (If last Customer was added in 2011, and new Customer is added in 2012, the new Customer-ID will be 20120001) $add_checklist = "INSERT INTO checklist VALUES ('$project', '$id', '$customer');"; // Starting variables (see above) $add_project = "INSERT INTO customers VALUES ('$id', '$customer', '$date');"; // Starting variables (see above) mysql_query($add_checklist) or die ("Query failed: " . mysql_error()); mysql_query($add_project) or die ("Query failed: " . mysql_error()); } elseif ($customer == $row_customer['customer']) { // Check IF entered Customer-Name already exists in table Customers $id = $row_customer['id']; // Obviously, get that same Customer-ID $project = (int) ((string) $id) . ((string) ($num_checklist+1)); // Project-ID will also contain that Customer-ID, with an extra digit (as stated above), which will be incremented (starting variable + numrows + 1) ($check_checklist Query) $add_checklist = "INSERT INTO checklist VALUES ('$project', '$id', '$customer');"; // Only the new Project will be added to the table Checklist, since the Customer already exists in table Customers mysql_query($add_checklist) or die ("Query failed: " . mysql_error()); } else { // When Customer(name) is new $id = $id + $num_customers; // Customer-ID is incremented (starting variable + numrows in table customers) $project = (int) substr((string)$project, 0, ; // Project-ID, 2nd last digit needs to be incremented $project = (string) ($project + $num_customers); // Project-ID, 2nd last digit needs to be incremented $project = (int) ($project . '1'); // Because new customer, Project-ID last digit will start with 1 again $add_checklist = "INSERT INTO checklist VALUES ('$project', '$id', '$customer');"; // New Project $add_project = "INSERT INTO customers VALUES ('$id', '$customer', '$date');"; // New Customer mysql_query($add_checklist) or die ("Query failed: " . mysql_error()); mysql_query($add_project) or die ("Query failed: " . mysql_error()); } ?>
-
Solved it myself! Now I got a headache, damn... I'd like to post my solution, but it's quite different now, and not easy to understand without my whole code. And the whole code uses weird variable names (dutch), so it's kinda hard to place, I think. If someone would really like to have the solution posted, I'll do so and rename my variables. Thanks anyway guys, for taking the time.
-
Those "no-values" are the project and id fields, and will be auto-incremented if they are empty. There ARE other values in those queries, but they aren't important to explain here... Well, now they are actually. Hehe. Sorry that I haven't made this clear in a better way. Hope you get my point now? But ofcourse, if you know a better way, please do tell me.
-
So after 1 record, I need to specify the uniqueness of $project and $id in my queries, even when it's auto-increment? Could you guide me a little with the code? What do you mean, empty row? There are other fields ofcourse, but I didn't include them here, to make it easier on you guys. When the first project is added, it uses the first two queries. From then on, the 3th and 4th, for auto-incrementing. Only the primary keys are blank for auto-incrementing. But like I said, this auto-incrementing is causing the foreign key failure. What exactly do you mean with NULL out those FK?
-
Yeh, sorry for that. I started the topic a bit too early... My previous post is my last situation. When both tables are empty, it WORKS. Yay! Because I specify the $project (checklist) and $id (customers). This is only when the table is empty, or a new year has started (201100011, 201200011, ...) Customer id uses 8/9 digits of project. In my previous post, you can see how my table needs to work. BUT when I want to add another project, I want to use auto-increment, obviously. But then I get that Error about my foreign key failing. Also, when auto-incrementing this way, I cannot achieve the functionality of my tables (no duplicate customer id/names).
-
UPDATE: I now first create a project, and then get the customer id value of that project (see code) So now I can add projects, instead of customers. So it would go like this: projects: - 201100011 - 20110001 - John Doe - 201100012 - 20110001 - John Doe - 201100021 - 20110002 - Foo Bar customers: - 20110001 - John Doe - 20110002 - Foo Bar So now the problem is still, when auto-incrementing, but also ignoring duplicate customers (when entering name, not id). Hope this is clear enough? The last updated code: <?php $year = (string) date('Y'); $project = $year . '00011'; // Will be like 201100011 $id = substr($project, 0, ; // Will be like 20110001 $project = (int) $project; $id = (int) $id; $add_customer1 = "INSERT INTO customers VALUES ('$id');"; // for adding when new year starts or table empty -- WORKING -- $add_checklist1 = "INSERT INTO checklist VALUES ('$project', '$id');"; // for adding when new year starts or table empty -- WORKING -- $add_customer = "INSERT INTO customers VALUES ('');"; // for adding with autoincrement -- WORKING -- $add_checklist = "INSERT INTO checklist VALUES ('', '');"; // for adding with autoincrement -- DOES NOT WORK -- if(($num < 1) || ((int)date('Y') > (int)substr($row['added'], 0, 4))){ // check if table empty OR current year is "bigger" than last record's year mysql_query($add_customer1) or die ("Query mislukt: " . mysql_error()); mysql_query($add_checklist1) or die ("Query mislukt: " . mysql_error()); } else { // else autoincrement mysql_query($add_customer) or die ("Query mislukt: " . mysql_error()); mysql_query($add_checklist) or die ("Query mislukt: " . mysql_error()); } ?> Error I get: Query failed: Cannot add or update a child row: a foreign key constraint fails (`cre8`.`checklist`, CONSTRAINT `checklist_ibfk_1` FOREIGN KEY (`id`) REFERENCES `customers` (`id`) ON DELETE CASCADE ON UPDATE CASCADE)
-
Okay, so at first, I needed to cast my id's to integers, since my table uses integers. The ONLY problem so far is with adding rows while auto-incrementing. This is my code (explanation inside): <?php $year = (string) date('Y'); $id = $year . '0001'; // will be like 20110001 (for when a new year starts) $project = $id . '1'; // will be like 201100011 $id = (int) $id; $project = (int) $project; $add_customer = "INSERT INTO customers VALUES ('$id');"; // for adding when new year starts or table empty -- WORKING -- $add_checklist1 = "INSERT INTO checklist VALUES ('$project', '$id');"; // for adding when new year starts or table empty -- WORKING -- $add_customer = "INSERT INTO customers VALUES ('');"; // for adding with autoincrement -- WORKING -- $add_checklist = "INSERT INTO checklist VALUES ('', '');"; // for adding with autoincrement -- DOES NOT WORK -- if(($num < 1) || ((int)date('Y') > (int)substr($row['toegevoegd'], 0, 4))){ // check if table empty OR current year is "bigger" than last record's year mysql_query($add_dossier1) or die ("Query mislukt: " . mysql_error()); mysql_query($add_checklist1) or die ("Query mislukt: " . mysql_error()); } else { // else autoincrement mysql_query($add_dossier) or die ("Query mislukt: " . mysql_error()); mysql_query($add_checklist) or die ("Query mislukt: " . mysql_error()); } ?> So basically, when I've added a customer (id = 20110001), it needs to add a project (id = 201100011) to another table. When the table is NOT empty, I want to use increment, for both id's. BUT, the project id MUST contain the customer id. My initial plan was to have more projects (201100011, 201100012, ...) for EACH customer. But I think that's not possible anymore, when using auto-increment, right? As of now, the only problem occurs when the code needs to execute the 4th query. The 3th query is still executed succesfully. How do I solve this, preferrably with the functionality of my initial plan? Thank you.