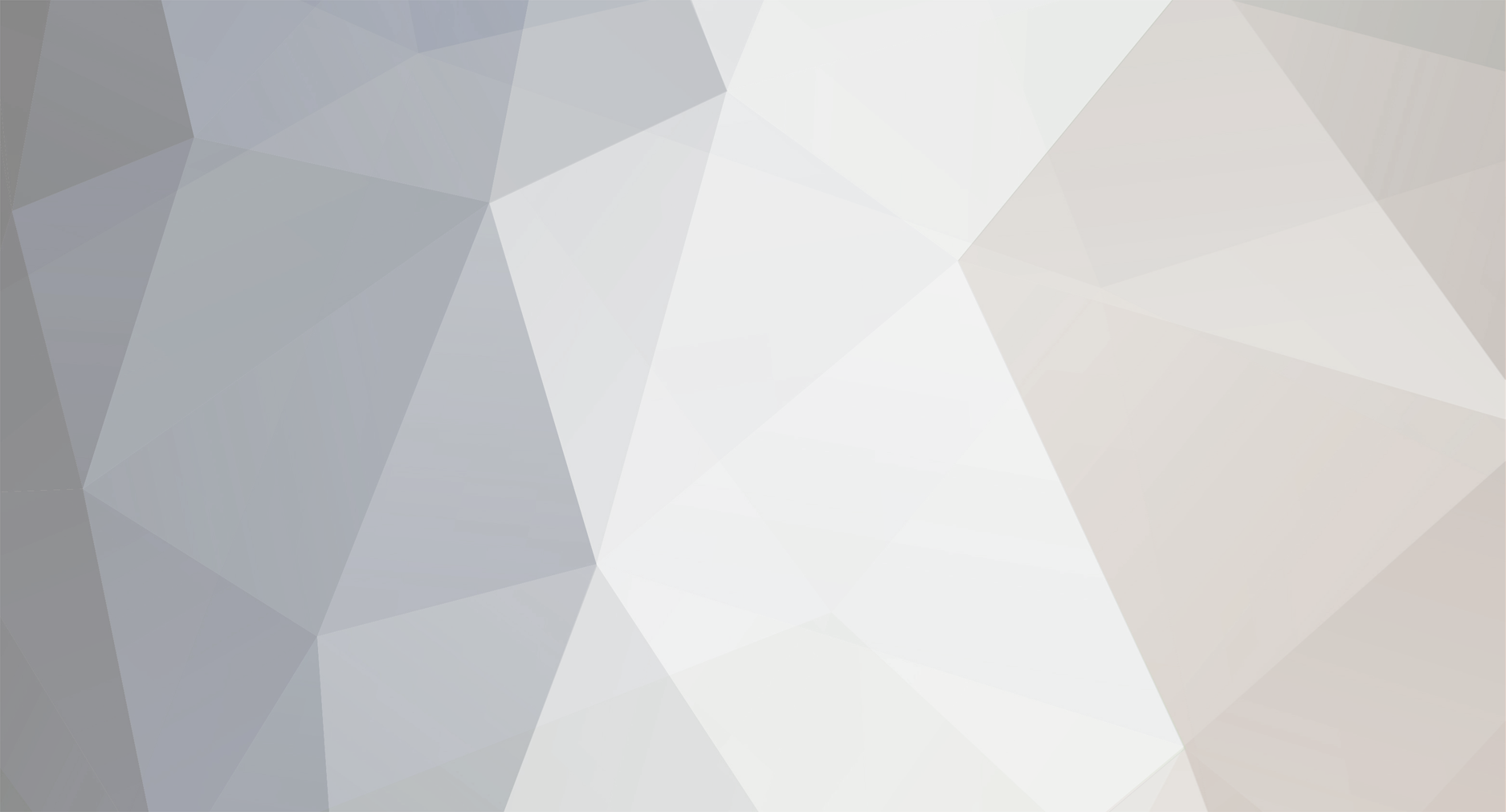
mikosiko
Members-
Posts
1,327 -
Joined
-
Last visited
-
Days Won
1
Everything posted by mikosiko
-
have you done something to debug your code?.... like echoing your query to see if it is valid or not? $query = "SELECT * FROM (table name) WHERE id='$u_id' AND firstname='$u_name' AND email='$u_email' AND password='$u_pass'"; //echo your query to validate it echo "Query : " . $query; Or use die() or trigger_error() after the query ? like $sql = mysql_query($query) or trigger_error('Query failed: ' . mysql_error(), E_USER_ERROR); also you can enable error reporting using: error_reporting(E_ALL); ini_set("display_errors", 1);
-
other than a syntax error, because you are using SECONDS instead of SECOND do you have any other problem?... which one?
-
I will say MyIsam or Innodb (in case you want transactions, referential integrity and row level locking choose Innodb) you can use triggers or stored procedures/functions in both... Federated SE is used to access data (tables) in remote servers.... more information regarding to storage engines and their characteristics here http://dev.mysql.com/doc/refman/5.0/en/storage-engines.html.
-
DavidM ...you'r right... that is what happens when I answer post before have my daily cup of coffee... so Taylor scratch the player_id PK, Unique key from my comments and stick with the id as the PK with auto-increment... thanks DavidM for that catch.
-
yes... you must read a little more... just a small example to help you start: now to calculate the score average for one player (or for all of them) you simply can do: SELECT players.id, AVG(scores.score) FROM players LEFT JOIN scores ON players.id = scores.player_id // or scores.id if you choose to have only one id in the table scores WHERE players.id = $playerid // being $playerid a parameter identifying an specific player... if you want all of them do not use the // WHERE clause GROUP BY players.id
-
Your biggest problem here is the design of your table,,, a better solution is to have 2 different tables: Players and Scores In that way you will have a better design and your queries are going to be much more easy and you will not limited to just 6 scores by player. The other thing that you should consider is to not store in the db fields that are calculated (dependent fields),,, you can always calculate whatever you want upon retrieval.
-
great... glad to help... read the document that I posted for you... it will help you to understand better Functions/Procedures and Cursors usage if you use the IF then you can't assign the city_out and state_out in that select that is why a much better solution is to use the SELECT INTO syntax that I posted before... with the IF you are able to only check for existence of the zip code... to return the city_out, state_out in that schema you must to make another select which will be totally unneficient
-
To validate the existence of the zip_code ...yes... however will be more efficient to write that in this way IF EXISTS (SELECT 1 FROM zipcodes WHERE zip_code = zip_in) THEN /// whatever ELSE // whatever END IF No clear why you will like to do that... answer depend on your objectives... why you don't tell us what exactly are you trying to do and we will try to answer accordingly. Cursors are RecordSet and you can process them almost exactly the same as you process them in PHP, therefore if your objective is return in some way the city and state to your PHP code my original example could do exactly that without any further complication. But as I said... no clear which are your objectives to give you a better answer
-
try to invest some time studying them... you will be happy with the outcome for sure... here is a small/sloppy example of one function (as I said very sloppy it could be optimized in many ways.. but valid to show you how it works) DELIMITER $$ DROP FUNCTION IF EXISTS `miltostandard` $$ CREATE DEFINER=`root`@`localhost` FUNCTION `miltostandard`(mtime VARCHAR(4)) RETURNS varchar( CHARSET latin1 BEGIN DECLARE milTime VARCHAR(4); DECLARE ampm VARCHAR(2); DECLARE milTimeHours VARCHAR(2); DECLARE milTimeMinutes VARCHAR(2); DECLARE standardTimeHours VARCHAR(4); DECLARE standardTime VARCHAR(; SET milTime = mtime; SET amPm = 'am'; SET milTimeHours = substr(milTime, 1, 2); SET milTimeMinutes = substr(milTime, 3, 2); if milTimeHours >=12 then SET amPm = 'pm'; if milTimeHours > 12 then SET standardTimeHours = milTimeHours - 12; else SET standardTimeHours = milTimeHours; end if; elseif milTimeHours = 0 then SET standardTimeHours = 12; else SET standardTimeHours = milTimeHours; end if; SET standardTime = CONCAT_WS( ":", standardTimeHours,milTimeMinutes); SET standardTime = CONCAT_WS( " ",standardTime,amPm); SELECT 'KAKA' INTO standardTime; return standardTime; END $$ DELIMITER ; and as a simple example too you can call it in this way: SELECT miltsostandard('2400'); and the results should be : 12:00 pm Stored Procedures are a little more tricky but a very powerful tool
-
function doesn't seems to have mayor complexity, at first glance I don't see why it couldn't be done in and stored function or even in a procedure
-
try using backtics here: WHERE 'date' BETWEEN should read WHERE `date` BETWEEN
-
other possible approach: Even when is true that you can't run in a SQL query a PHP function, that doesn't mean that you can't run a SQL STORED FUNCTION, therefore the alternative is write/store your FUNCTION in MYSQL and use it directly in your select... that would be simpler than reading all the rows every time and filter results in PHP... your query then should looks like the one you posted originally using the function
-
You should if you want to have a good DB design, remember that the relational model is the foundation of SQL, therefore the better your model the better and easy your queries will be (Did I sound like Yoda here ?)... and no.. is not for quick referencing (but could result on).. is for proper db modeling. that is easily covered for any aggregation function (COUNT, SUM, etc) on retrieval... in a good DB model you should avoid store dependent fields in your table. Maybe a search and read upon "Database Normalization" will help you further
-
this is better SELECT users.* FROM users JOIN teams ON users.teamname = teams.teamname AND teams.division='$division' AND teams.league='$league' ORDER BY users.won DESC, users.total ASC however, I will suggest you to review your tables design; the data modeling could be improved... per. example (just a couple of small ones): - in your table user you should be referencing/storing the team.id and not the team.teamname (same concept could be applied to the fields division and league in your table teams). - in your table user you should no be storing a dependent field (total field is a dependent one)... calculated values should be calculated upon retrieval.
-
Or do you just not understand it? In my eyes it does exactly what he wants, I just didn't set the default to San Francisco, CA mine takes the city (if found) and and state and adds it to a temp table. it counts the rows in the temp table if there is 1 row it was found, if there wasn't a row nothing was found. Oh boy.... Oh boy... Your "code" delimiter // CREATE PROCEDURE zip_code_search (IN zip_in CHAR, OUT city_out VARCHAR, OUT state_out CHAR) BEGIN create temporary table if not exists city(city char(15), state char(2)); insert into city select city, state from zipcodes where zip_code = zip_in; set @rows = (select count(*) from city); IF @rows = 1 THEN -- The value was found select * from city; ELSE -- The value wasn't found END IF; END// how it should be (in its simple form no adding error handlers) delimiter // CREATE PROCEDURE zip_code_search (IN zip_in CHAR, OUT city_out VARCHAR, OUT state_out CHAR) BEGIN SET city_out = ''; SELECT city, state INTO city_out, state_out FROM zipcodes WHERE zip_code = zip_in; IF (city_out = '') THEN SET city_out = 'San Francisco'; SET state_out = 'CA'; END IF; END// and yes... you are right... I don't understand your "fantastic code" ... it is FUBAR!! do yourself a favor a read a little the document that I did post for the OP you really need it.
-
again... that is the function of SELECT INTO... your code doesn't make any sense at all... now you included a temporary table and one insert which IS NOt what the OP is asking for... go ahead and read again his request.
-
and how do you get the values for city_out and state_out that the OP needs?... doing other SELECT?.... that is the function of SELECT INTO
-
Basically you have 2 options: a) Do the checking in the same SP; for that you have to modify your SELECT and use it with the SELECT... INTO syntax like: SELECT city, state INTO city_out, state_out FROM zipcodes WHERE zip_code = zip_in; // and validate the values in city_out or state_out to determine if they have value after the select b) or do the checking in your PHP code after you call the SP using any of the mysql API functions (mysql_num_rows($resultset), mysql_fetch.. etc). As an additional suggestion: you should include in you SP the necessary coding to control possibles errors using the DECLARE HANDLER syntax. a good source to learn how to use Stored Procedure is this PDF document... Error Handling is covered starting at the end of page 29
-
and in that case the UPDATE is simply: $sqltwo = "UPDATE events SET publish = !publish WHERE ID='$id'";
-
yes.... ALTER TABLE using the proper "table_option".... but why to do that in a sql query if you can do it with any administration tool (as phpmyadmin p.e) ?
-
JOINs on three tables - what structure is implied
mikosiko replied to DaiLaughing's topic in MySQL Help
let me answer you with a hypothetical situation using a variation of the Country-City-Language example: SELECT Country.name, City.name, CityLanguage.languagename FROM Country LEFT OUTER JOIN City ON City.CountryCode = Country.CountryCode LEFT OUTER JOIN CityLanguage ON CityLanguage.CityCode = City.CityCode; that could represent a master-child-child relation... apply the same for the surgery-doctor-appointment example -
JOINs on three tables - what structure is implied
mikosiko replied to DaiLaughing's topic in MySQL Help
then your better read your question again and prepare your palm: this select SELECT * FROM Country LEFT OUTER JOIN CountryLanguage ON Country.Code = CountryLanguage.CountryCode LEFT OUTER JOIN City ON Country.Code = City.CountryCode; doesn't imply that in any possible way... the relations are clearly established in the JOINS. -
after this line: $final = mysql_query("SELECT tickets.item, tickets.amount, tickets.paypal FROM tickets WHERE tickets.item = $id"); and assuming that your SQL is correct, check for the number of affected rows with mysql_num_rows() and proceed accordingly
-
as fenway told you... your questions are too general, hard to tell you exactly what to do or how to proceed. You said that you are just learning, therefore for you probably is no going to be easy to develop something in a short time... sure you can lean and work you way to finish with something usable, but the learning curve could be no good for your and/or your users objectives.... if you have plenty of time start reading some good books or tutorial on line (hundred if your google for it), write some code and come back whit what you have done and your doubts, more likely somebody will help you. Now, if you don't have the time and need to produce something workable soon your best bet is hire some programmer and work with him/her in your project, whichever the route that you choose... good luck . BTW.: if you want you can post your tables, a description of your project and ask for guidance (specific doubts) that help you to better deal and understand your options.