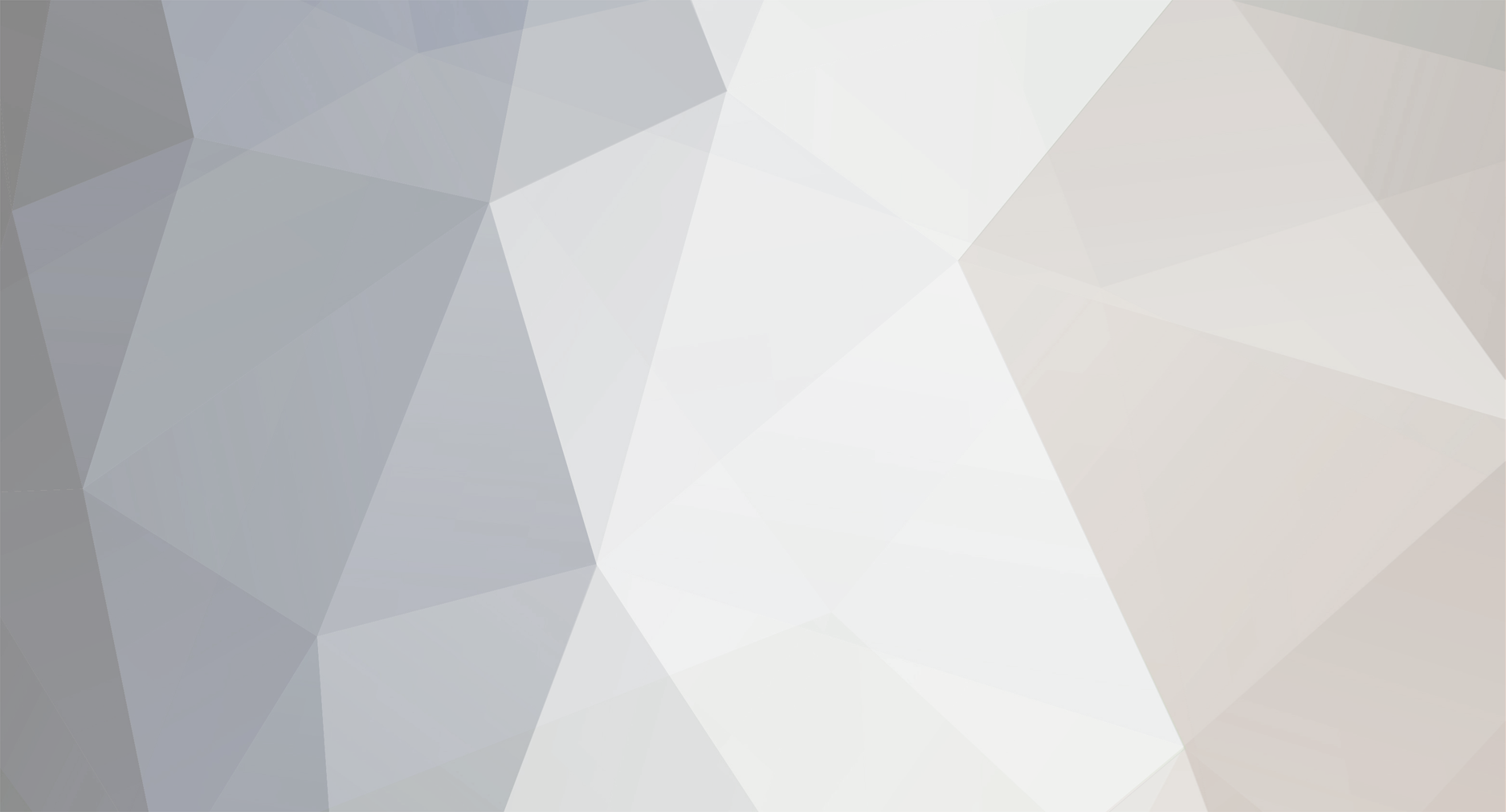
Namtip
Members-
Posts
110 -
Joined
-
Last visited
Never
Everything posted by Namtip
-
I've had a look and I'm sorry but I haven't got any knowledge to fall back on with some of the functions you use e.g error_reporting . I'm a bit of a newbie too. If you need any help with my method, I'll respond. Also if there are any php pros on here, could you help with my image upload post.
-
This is a registration script from a book I'm using. You send the information from the form into the same file below. it checks if the field is empty. if its empty it sends you back to the form with an error message. if empty example: <?php session_start(); include 'db.inc.php'; $db = mysql_connect(MYSQL_HOST, MYSQL_USER, MYSQL_PASSWORD) or die ('Unable to connect. Check your connection parameters.'); mysql_select_db(MYSQL_DB, $db) or die(mysql_error($db)); $hobbies_list = array('Computers', 'Dancing', 'Exercise', 'Flying', 'Golfing', 'Hunting', 'Internet', 'Reading', 'Traveling', 'Other than listed'); // filter incoming values $username = (isset($_POST['username'])) ? trim($_POST['username']) : ''; $password = (isset($_POST['password'])) ? $_POST['password'] : ''; $first_name = (isset($_POST['first_name'])) ? trim($_POST['first_name']) : ''; $last_name = (isset($_POST['last_name'])) ? trim($_POST['last_name']) : ''; $email = (isset($_POST['email'])) ? trim($_POST['email']) : ''; $city = (isset($_POST['city'])) ? trim($_POST['city']) : ''; $state = (isset($_POST['state'])) ? trim($_POST['state']) : ''; $hobbies = (isset($_POST['hobbies']) && is_array($_POST['hobbies'])) ? $_POST['hobbies'] : array(); if (isset($_POST['submit']) && $_POST['submit'] == 'Register') { $errors = array(); // make sure manditory fields have been entered if (empty($username)) { $errors[] = 'Username cannot be blank.'; } // check if username already is registered $query = 'SELECT username FROM site_user WHERE username = "' . $username . '"'; $result = mysql_query($query, $db) or die(mysql_error()); if (mysql_num_rows($result) > 0) { $errors[] = 'Username ' . $username . ' is already registered.'; $username = ''; } mysql_free_result($result); if (empty($password)) { $errors[] = 'Password cannot be blank.'; } if (empty($first_name)) { $errors[] = 'First name cannot be blank.'; } if (empty($last_name)) { $errors[] = 'Last name cannot be blank.'; } if (empty($email)) { $errors[] = 'Email address cannot be blank.'; } if (count($errors) > 0) { echo '<p><strong style="color:#FF000;">Unable to process your ' . 'registration.</strong></p>'; echo '<p>Please fix the following:</p>'; echo '<ul>'; foreach ($errors as $error) { echo '<li>' . $error . '</li>'; } echo '</ul>'; } else { // No errors so enter the information into the database. $query = 'INSERT INTO site_user (user_id, username, password) VALUES (NULL, "' . mysql_real_escape_string($username, $db) . '", ' . 'PASSWORD("' . mysql_real_escape_string($password, $db) . '"))'; $result = mysql_query($query, $db) or die(mysql_error()); $user_id = mysql_insert_id($db); $query = 'INSERT INTO site_user_info (user_id, first_name, last_name, email, city, state, hobbies) VALUES (' . $user_id . ', ' . '"' . mysql_real_escape_string($first_name, $db) . '", ' . '"' . mysql_real_escape_string($last_name, $db) . '", ' . '"' . mysql_real_escape_string($email, $db) . '", ' . '"' . mysql_real_escape_string($city, $db) . '", ' . '"' . mysql_real_escape_string($state, $db) . '", ' . '"' . mysql_real_escape_string(join(', ', $hobbies), $db) . '")'; $result = mysql_query($query, $db) or die(mysql_error()); $_SESSION['logged'] = 1; $_SESSION['username'] = $username; header('Refresh: 5; URL=main.php'); ?> <html> <head> <title>Register</title> </head> <body> <p><strong>Thank you <?php echo $username; ?> for registering!</strong></p> <p>Your registration is complete! You are being sent to the page you requested. If your browser doesn't redirect properly after 5 seconds, <a href="main.php">click here</a>.</p> </body> </html> <?php die(); } } ?> <html> <head> <title>Register</title> <style type="text/css"> td { vertical-align: top; } </style> </head> <body> <form action="register.php" method="post"> <table> <tr> <td><label for="username">Username:</label></td> <td><input type="text" name="username" id="username" size="20" maxlength="20" value="<?php echo $username; ?>"/></td> </tr><tr> <td><label for="password">Password:</label></td> <td><input type="password" name="password" id="password" size="20" maxlength="20" value="<?php echo $password; ?>"/></td> </tr><tr> <td><label for="email">Email:</label></td> <td><input type="text" name="email" id="email" size="20" maxlength="50" value="<?php echo $email; ?>"/></td> </tr><tr> <td><label for="first_name">First name:</label></td> <td><input type="text" name="first_name" id="first_name" size="20" maxlength="20" value="<?php echo $first_name; ?>"/></td> </tr><tr> <td><label for="last_name">Last name:</label></td> <td><input type="text" name="last_name" id="last_name" size="20" maxlength="20" value="<?php echo $last_name; ?>"/></td> </tr><tr> <td><label for="city">City:</label></td> <td><input type="text" name="city" id="city" size="20" maxlength="20" value="<?php echo $city; ?>"/></td> </tr><tr> <td><label for="state">State:</label></td> <td><input type="text" name="state" id="state" size="2" maxlength="2" value="<?php echo $state; ?>"/></td> </tr><tr> <td><label for="hobbies">Hobbies/Interests:</label></td> <td><select name="hobbies[]" id="hobbies" multiple="multiple"> <?php foreach ($hobbies_list as $hobby) { if (in_array($hobby, $hobbies)) { echo '<option value="' . $hobby . '" selected="selected">' . $hobby . '</option>'; } else { echo '<option value="' . $hobby . '">' . $hobby . '</option>'; } } ?> </select></td> </tr><tr> <td> </td> <td><input type="submit" name="submit" value="Register"/></td> </tr> </table> </form> </body> </html> obviously that's alright but what if you need something abit more detailed. For example you don't want user(chump) to use a specific character (e.g "*") Then you have to use preg_match and regular expressions(it's hard) . Here is an example. preg_match <?php //let's start the session session_start(); //connect to database include 'db.inc.php'; $db = mysql_connect('localhost', 'root', '') or die ('Unable to connect. Check your connection parameters.'); mysql_select_db('ourgallery', $db) or die(mysql_error($db)); //finally, let's store our posted values in the session variables if (isset($_POST['submiti'])) { // do something $_SESSION['values'] = $_POST; $error = array(); $query = 'SELECT name FROM user WHERE ' . 'name = "' . mysql_real_escape_string($_SESSION['values']['name'], $db) . '"' ; $result = mysql_query($query, $db) or die(mysql_error($db)); if(mysql_num_rows($result) > 0) { $error['uname'] = '<p class="errText">Username is taken.</p>'; } if( preg_match('/[^a-zA-Z]/',$_SESSION['values']['name']) ) { $error['name'] = '<p class="errText">Your Username must be made from letters, dashes, spaces and must not start with dash</p>'; } if( preg_match('/[^a-zA-Z -]{3,30}/',$_SESSION['values']['first_name']) ) { $error['first_name'] = '<p class="errText">Your first name must be typed with letters, dashes, spaces and must not start with dash</p>'; } if( preg_match('/[^a-zA-Z -]{3,30}/',$_SESSION['values']['last_name']) ) { $error['last_name'] = '<p class="errText">Your last name must be typed with letters, dashes, spaces and must not start with dash</p>'; } if( preg_match('/[^a-zA-Z]\w+(\.\w+)*\@\w+(\.[^0-9a-zA-Z]+)*\.[^a-zA-Z]{2,4}$/',$_SESSION['values']['email']) ) { $error['email'] = '<p class="errText">This is not a valid email address.'; } if( preg_match('/[^a-zA-Z0-9 .,]+$/',$_SESSION['values']['address']) ) { $error['address'] = '<p class="errText">Address must be only letters, numbers or one of the following ". , : /"</p>'; } if( preg_match('/[^a-zA-Z]+/',$_SESSION['values']['city']) ) { $error['city'] = '<p class="errText">Your city must contain alphabetical characters only.'; } if( preg_match('/[^a-zA-Z]+/',$_SESSION['values']['county']) ) { $error['county'] = '<p class="errText">Your county must contain alphabetical characters only.'; } if( preg_match('/(^GIR 0AA)|((([^A-Z-[QVX]][^0-9][^0-9]?)|(([^A-Z-[QVX]][^A-Z-[^IJZ]][^0-9][^0-9]?)|(([^A-Z-[^QVX]][^0-9][^A-HJKSTUW])|([^A-Z-[^QVX]][^A-Z-[^IJZ]][^0-9][^ABEHMNPRVWXY])))) [^0-9][^A-Z-[^CIKMOV]]{2})/',$_SESSION['values']['post']) ) { $error['post'] = '<p class="errText">This is not a valid UK Postcode.'; } if( preg_match('/[^0-9]{1,13}/',$_SESSION['values']['home']) ) { $error['home'] = '<p class="errText">Your UK local phone number must contain number characters only.'; } if( preg_match('/[^0-9]{1,13}/',$_SESSION['values']['mobile']) ) { $error['mob'] = '<p class="errText">Your UK mobile phone number must contain number characters only.'; } //if we have errors let's assign them to the session and redirect back, you could just assign to $_SESSION['error']['name'] etc.. above if(!empty($error)) { $_SESSION['error'] = $error; header("Location: form1.php"); exit; That is some of my actual multi page registration script . Have a look at it and tell me what you don't understand. My response may be sluggish I'm playing counterstrike, so google preg_match and regular expressions if I don't respond immediately.
-
I use preg_match and regular expressions to validate forms. I've also used "if empty" I can post you some example code if you like.
-
So, I'm learning how to upload pictures into a system from my awesome PHP book. I've looked and looked through the script but I can't figure out whats wrong with it. Goal: The script is meant to save a full version of the image in the images folder and a thumbnail in the thumbnail folder. Bug: The full image does not appear in any folder, and the thumbnail is created but its put in the images folder. I've checked the GD library, and everything is supported. image_effect.php <?php //change this path to match your images directory $dir ='C:/x/xampp/htdocs/images'; //change this path to match your fonts directory and the desired font putenv('GDFONTPATH=' . 'C:/Windows/Fonts'); $font = 'arial'; // make sure the requested image is valid if (isset($_GET['id']) && ctype_digit($_GET['id']) && file_exists($dir . '/' . $_GET['id'] . '.jpg')) { $image = imagecreatefromjpeg($dir . '/' . $_GET['id'] . '.jpg'); } else { die('invalid image specified'); } // apply the filter $effect = (isset($_GET['e'])) ? $_GET['e'] : -1; switch ($effect) { case IMG_FILTER_NEGATE: imagefilter($image, IMG_FILTER_NEGATE); break; case IMG_FILTER_GRAYSCALE: imagefilter($image, IMG_FILTER_GRAYSCALE); break; case IMG_FILTER_EMBOSS: imagefilter($image, IMG_FILTER_EMBOSS); break; case IMG_FILTER_GAUSSIAN_BLUR: imagefilter($image, IMG_FILTER_GAUSSIAN_BLUR); break; } // add the caption if requested if (isset($_GET['capt'])) { imagettftext($image, 12, 0, 20, 20, 0, $font, $_GET['capt']); } //add the logo watermark if requested if (isset($_GET['logo'])) { // determine x and y position to center watermark list($width, $height) = getimagesize($dir . '/' . $_GET['id'] . '.jpg'); list($wmk_width, $wmk_height) = getimagesize('images/logo.png'); $x = ($width - $wmk_width) / 2; $y = ($height - $wmk_height) / 2; $wmk = imagecreatefrompng('images/logo.png'); imagecopymerge($image, $wmk, $x, $y, 0, 0, $wmk_width, $wmk_height, 20); imagedestroy($wmk); } // show the image header('Content-Type: image/jpeg'); imagejpeg($image, '', 100); ?> check_image.php <?php include 'db.inc.php'; //connect to MySQL $db = mysql_connect(MYSQL_HOST, MYSQL_USER, MYSQL_PASSWORD) or die ('Unable to connect. Check your connection parameters.'); mysql_select_db(MYSQL_DB, $db) or die(mysql_error($db)); //change this path to match your images directory $dir ='C:/x/xampp/htdocs/images'; //change this path to match your thumbnail directory $thumbdir = $dir . '/thumbs'; //change this path to match your fonts directory and the desired font putenv('GDFONTPATH=' . 'C:/Windows/Fonts'); $font = 'arial'; // handle the uploaded image if ($_POST['submit'] == 'Upload') { //make sure the uploaded file transfer was successful if ($_FILES['uploadfile']['error'] != UPLOAD_ERR_OK) { switch ($_FILES['uploadfile']['error']) { case UPLOAD_ERR_INI_SIZE: die('The uploaded file exceeds the upload_max_filesize directive ' . 'in php.ini.'); break; case UPLOAD_ERR_FORM_SIZE: die('The uploaded file exceeds the MAX_FILE_SIZE directive that ' . 'was specified in the HTML form.'); break; case UPLOAD_ERR_PARTIAL: die('The uploaded file was only partially uploaded.'); break; case UPLOAD_ERR_NO_FILE: die('No file was uploaded.'); break; case UPLOAD_ERR_NO_TMP_DIR: die('The server is missing a temporary folder.'); break; case UPLOAD_ERR_CANT_WRITE: die('The server failed to write the uploaded file to disk.'); break; case UPLOAD_ERR_EXTENSION: die('File upload stopped by extension.'); break; } } //get info about the image being uploaded $image_caption = $_POST['caption']; $image_username = $_POST['username']; $image_date = @date('Y-m-d'); list($width, $height, $type, $attr) = getimagesize($_FILES['uploadfile']['tmp_name']); // make sure the uploaded file is really a supported image $error = 'The file you uploaded was not a supported filetype.'; switch ($type) { case IMAGETYPE_GIF: $image = imagecreatefromgif($_FILES['uploadfile']['tmp_name']) or die($error); break; case IMAGETYPE_JPEG: $image = imagecreatefromjpeg($_FILES['uploadfile']['tmp_name']) or die($error); break; case IMAGETYPE_PNG: $image = imagecreatefrompng($_FILES['uploadfile']['tmp_name']) or die($error); break; default: die($error); } //insert information into image table $query = 'INSERT INTO images (image_caption, image_username, image_date) VALUES ("' . $image_caption . '", "' . $image_username . '", "' . $image_date . '")'; $result = mysql_query($query, $db) or die (mysql_error($db)); //retrieve the image_id that MySQL generated automatically when we inserted //the new record $last_id = mysql_insert_id(); // save the image to its final destination $image_id = $last_id; imagejpeg($image, $dir . '/' . $image_id . '.jpg'); imagedestroy($image); } else { // retrieve image information $query = 'SELECT image_id, image_caption, image_username, image_date FROM images WHERE image_id = ' . $_POST['id']; $result = mysql_query($query, $db) or die (mysql_error($db)); extract(mysql_fetch_assoc($result)); list($width, $height, $type, $attr) = getimagesize($dir . '/' . $image_id . '.jpg'); } if ($_POST['submit'] == 'Save') { // make sure the requested image is valid if (isset($_POST['id']) && ctype_digit($_POST['id']) && file_exists($dir . '/' . $_POST['id'] . '.jpg')) { $image = imagecreatefromjpeg($dir . '/' . $_POST['id'] . '.jpg'); } else { die('invalid image specified'); } // apply the filter $effect = (isset($_POST['effect'])) ? $_POST['effect'] : -1; switch ($effect) { case IMG_FILTER_NEGATE: imagefilter($image, IMG_FILTER_NEGATE); break; case IMG_FILTER_GRAYSCALE: imagefilter($image, IMG_FILTER_GRAYSCALE); break; case IMG_FILTER_EMBOSS: imagefilter($image, IMG_FILTER_EMBOSS); break; case IMG_FILTER_GAUSSIAN_BLUR: imagefilter($image, IMG_FILTER_GAUSSIAN_BLUR); break; } // add the caption if requested if (isset($_POST['emb_caption'])) { imagettftext($image, 12, 0, 20, 20, 0, $font, $image_caption); } //add the logo watermark if requested if (isset($_POST['emb_logo'])) { // determine x and y position to center watermark list($wmk_width, $wmk_height) = getimagesize('images/logo.png'); $x = ($width - $wmk_width) / 2; $y = ($height - $wmk_height) / 2; $wmk = imagecreatefrompng('images/logo.png'); imagecopymerge($image, $wmk, $x, $y, 0, 0, $wmk_width, $wmk_height, 20); imagedestroy($wmk); } // save the image with the filter applied imagejpeg($image, $dir . '/' . $_POST['id'] . '.jpg', 100); //set the dimensions for the thumbnail $thumb_width = $width * 0.10; $thumb_height = $height * 0.10; //create the thumbnail $thumb = imagecreatetruecolor($thumb_width, $thumb_height); imagecopyresampled($thumb, $image, 0, 0, 0, 0, $thumb_width, $thumb_height, $width, $height); imagejpeg($thumb, $dir . '/' . $_POST['id'] . '.jpg', 100); imagedestroy($thumb); ?> <html> <head> <title>Here is your pic!</title> </head> <body> <h1>Your image has been saved!</h1> <img src="images/<?php echo $_POST['id']; ?>.jpg" /> </body> </html> <?php } else { ?> <html> <head> <title>Here is your pic!</title> </head> <body> <h1>So how does it feel to be famous?</h1> <p>Here is the picture you just uploaded to our servers:</p> <?php if ($_POST['submit'] == 'Upload') { $imagename = 'images/' . $image_id . '.jpg'; } else { $imagename = 'image_effect.php?id=' . $image_id . '&e=' . $_POST['effect']; if (isset($_POST['emb_caption'])) { $imagename .= '&capt=' . urlencode($image_caption); } if (isset($_POST['emb_logo'])) { $imagename .= '&logo=1'; } } ?> <img src="<?php echo $imagename; ?>" style="float:left;"> <table> <tr><td>Image Saved as: </td><td><?php echo $image_id . '.jpg'; ?></td></tr> <tr><td>Height: </td><td><?php echo $height; ?></td></tr> <tr><td>Width: </td><td><?php echo $width; ?></td></tr> <tr><td>Upload Date: </td><td><?php echo $image_date; ?></td></tr> </table> <p>You may apply special options to your image below. Note: saving an image with any of the options applied <em>cannot be undone</em>.</p> <form action="<?php echo $_SERVER['PHP_SELF']; ?>" method="post"> <div> <input type="hidden" name="id" value="<?php echo $image_id;?>"/> Filter: <select name="effect"> <option value="-1">None</option> <?php echo '<option value="' . IMG_FILTER_GRAYSCALE . '"'; if (isset($_POST['effect']) && $_POST['effect'] == IMG_FILTER_GRAYSCALE) { echo ' selected="selected"'; } echo '>Black and White</option>'; echo '<option value="' . IMG_FILTER_GAUSSIAN_BLUR . '"'; if (isset($_POST['effect']) && $_POST['effect'] == IMG_FILTER_GAUSSIAN_BLUR) { echo ' selected="selected"'; } echo '>Blur</option>'; echo '<option value="' . IMG_FILTER_EMBOSS . '"'; if (isset($_POST['effect']) && $_POST['effect'] == IMG_FILTER_EMBOSS) { echo ' selected="selected"'; } echo '>Emboss</option>'; echo '<option value="' . IMG_FILTER_NEGATE . '"'; if (isset($_POST['effect']) && $_POST['effect'] == IMG_FILTER_NEGATE) { echo ' selected="selected"'; } echo '>Negative</option>'; ?> </select> <br/><br/> <?php echo '<input type="checkbox" name="emb_caption"'; if (isset($_POST['emb_caption'])) { echo ' checked="checked"'; } echo '>Embed caption in image?'; echo '<br/><br/><input type="checkbox" name="emb_logo"'; if (isset($_POST['emb_logo'])) { echo ' checked="checked"'; } echo '>Embed watermarked logo in image?'; ?> <br/><br/> <input type="submit" value="Preview" name="submit" /> <input type="submit" value="Save" name="submit" /> </div> </form> </body> </html> <?php } ?> Any help appreciated.
-
Session variables do not get returned into previous page's form.
Namtip replied to Namtip's topic in PHP Coding Help
Hmmm, Yup, that works, except its giving me error messages for the correctly entered parts of the form. I should be able to sort that out though. Hopefully. Thanks for the help! -
Session variables do not get returned into previous page's form.
Namtip replied to Namtip's topic in PHP Coding Help
Ah! good spot! I could kiss you! That's go the sessions to pass through to form1 but my error messages still aren't coming up . Any clues? -
This is a multi page registration form. I want to check the user input from form1.php with preg_match in form2.php then return the users details back into form1 with error messages(e.g $errname) so the user doesn't need to re enter the information. Neither the error messages or the session variables are shown in form1.php's form. I've echoed out the session variables found that the value makes it to form 2. But the variables are empty when it goes back to form 1. form1.php <html> <head> <title>Register</title> <style type="text/css"> td { vertical-align: top; } .errText { font-family: Arial; font-size: 10px; color: #CC0000; text-decoration: none; font-weight: normal; } </style> </head> <body> <form action="form2.php" method="post"> <table> <tr> <td><label for="name">Username:</label></td> <td><input type="text" name="name" id="name" size="20" maxlength="20" value="<?php echo $_SESSION['name'];?>"/> <?php if(isset($_POST[$errname])) echo $errname; ?></td> </tr><tr> <td><label for="password">Password:</label></td> <td><input type="password" name="password" id="password" size="20" maxlength="20" value=""/></td> </tr><tr> <td><label for="first_name">First name:</label></td> <td><input type="text" name="first_name" id="first_name" size="20" maxlength="20" value="<?php echo $_SESSION['first_name'];?>"/> <?php if(isset($_POST[$errfirst])) echo $errfirst; ?></td> </tr><tr> <td><label for="last_name">Last name:</label></td> <td><input type="text" name="last_name" id="last_name" size="20" maxlength="20" value="<?php echo $_SESSION['last_name'];?>"/> <?php if(isset($_POST[$errlast])) echo $errlast; ?> </td> </tr><tr> <td><label for="email">Email:</label></td> <td><input type="text" name="email" id="email" size="20" maxlength="50" value="<?php echo $_SESSION['email'];?>"/> <?php if(isset($_POST[$erremail])) echo $erremail; ?></td> </tr><tr> <td><label for="address">Address:</label></td> <td><input type="text" name="address" id="address" size="20" maxlength="20" value="<?php echo $_SESSION['address'];?>"/> <?php if(isset($_POST[$erraddress])) echo $erraddress; ?></td> </tr><tr> <td><label for="city">City/Town:</label></td> <td><input type="text" name="city" id="city" size="20" maxlength="20" value="<?php echo $_SESSION['city'];?>"/> <?php if(isset($_POST[$errcity])) echo $errcity; ?></td> </tr><tr> <td><label for="county">County:</label></td> <td><input type="text" name="county" id="county" size="20" maxlength="20" value="<?php echo $_SESSION['county'];?>"/> <?php if(isset($_POST[$errcounty])) echo $errcounty; ?></td> </tr><tr> <td><label for="post">Postcode:</label></td> <td><input type="text" name="post" id="post" size="20" maxlength="20" value="<?php echo $_SESSION['post'];?>"/> <?php if(isset($_POST[$errpost])) echo $errpost; ?></td> </tr><tr> <td><label for="home">Home Number:</label></td> <td><input type="text" name="home" id="home" size="20" maxlength="20" value="<?php echo $_SESSION['home'];?>"/> <?php if(isset($_POST[$errhome])) echo $errhome; ?></td> </tr><tr> <td><label for="mobile">Mobile:</label></td> <td><input type="text" name="mobile" id="mobile" size="20" maxlength="20" value="<?php echo $_SESSION['mobile'];?>"/> <?php if(isset($_POST[$errmobile])) echo $errmobile; ?></td> </tr><tr> <td> </td> <td><input type="submit" name="submit" value="Sumbit"/></td> </tr> </table> </form> </body> </html> form2.php <?php //let's start the session session_start(); //finally, let's store our posted values in the session variables $_SESSION['name'] = $_POST['name']; $_SESSION['password'] = $_POST['password']; $_SESSION['first_name'] = $_POST['first_name']; $_SESSION['last_name'] = $_POST['last_name']; $_SESSION['email'] = $_POST['email']; $_SESSION['address'] = $_POST['address']; $_SESSION['city'] = $_POST['city']; $_SESSION['county'] = $_POST['county']; $_SESSION['post'] = $_POST['post']; $_SESSION['home'] = $_POST['home']; $_SESSION['mobile'] = $_POST['mobile']; $errname = ""; $errfirst = ""; $errlast = ""; $erremail = ""; $erraddress = ""; $errcity = ""; $errcounty = ""; $errpost = ""; $errhome = ""; $errmob = ""; if( preg_match('/^[A-Z][a-zA-Z -]{3,30}+$/',$_SESSION['name']) ) { $errname = '<p class="errText">Name must be from letters, dashes, spaces and must not start with dash</p>'; } if( preg_match('/^[A-Z][a-zA-Z -]{3,30}+$/',$_SESSION['first_name']) ) { $errfirst = '<p class="errText">Name must be from letters, dashes, spaces and must not start with dash</p>'; } if( preg_match('/^[A-Z][a-zA-Z -]{3,30}+$/',$_SESSION['last_name']) ) { $errlast = '<p class="errText">Name must be from letters, dashes, spaces and must not start with dash</p>'; } if( preg_match('/^[a-zA-Z]\w+(\.\w+)*\@\w+(\.[0-9a-zA-Z]+)*\.[a-zA-Z]{2,4}$/',$_SESSION['email']) ) { $erremail = '<p class="errText">This is not a valid email address.'; } if( preg_match('/^[a-zA-Z0-9 _.,:\"\']+$/',$_SESSION['address']) ) { $erraddress = '<p class="errText">Address must be only letters, numbers or one of the following ". , : /"</p>'; } if( preg_match('/[a-zA-Z]+/',$_SESSION['city']) ) { $errcity = '<p class="errText">Your city must contain a letter.'; } if( preg_match('/[a-zA-Z]+/',$_SESSION['county']) ) { $errcounty = '<p class="errText">Your county must contain a letter.'; } if( preg_match('/(GIR 0AA)|((([A-Z-[QVX]][0-9][0-9]?)|(([A-Z-[QVX]][A-Z-[iJZ]][0-9][0-9]?)|(([A-Z-[QVX]][0-9][A-HJKSTUW])|([A-Z-[QVX]][A-Z-[iJZ]][0-9][ABEHMNPRVWXY])))) [0-9][A-Z-[CIKMOV]]{2})/',$_SESSION['post']) ) { $errpost = '<p class="errText">This is not a valid UK Postcode.'; } if( preg_match('/s*\(?0\d{4}\)?(\s*|-)\d{3}(\s*|-)(\d{3}\s*)|(\s*\(?0\d{3}\)?(\s*|-)\d{3}(\s*|-)\d{4}\s*)|(\s*)(7|(\d{7}|\d{3}(\-|\s{1})\d{4})\s*/',$_SESSION['home']) ) { $errhome = '<p class="errText">This is not a valid UK local phone number.'; } if( preg_match('/^(\+44\s?7\d{3}|\(?07\d{3}\)?)\s?\d{3}\s?\d{3}$/',$_SESSION['mobile']) ) { $errmob = '<p class="errText">This is not a valid UK mobile phone number.'; } else{ $errname = ($_POST[$errname]); $errfirst = ($_POST[$errfirst]); $errlast = ($_POST[$errlast]); $erremail = ($_POST[$erremail]); $erraddress = ($_POST[$erraddress]); $errcity = ($_POST[$errcity]); $errcounty = ($_POST[$errcounty]); $errpost = ($_POST[$errpost]); $errhome = ($_POST[$errhome]); $errmob = ($_POST[$errmob]); header("Location: form1.php"); exit; } ?> Any help appreciated. I think I should buy a professional book, instead of my beginner one.
-
Looks like nobody knows, so I'm going to help out. lucky you! These links says you can and has some information about how to do it: http://www.coderanch.com/t/111999/HTML-JavaScript/anchor-tag-passing-variable http://www.ahfb2000.com/webmaster_help_desk/showthread.php?t=7579 I'm afraid I don't know how to do it but at least 2 examples in from the links above (that I don't understand). Please see my signature warning.
-
Thanks guys! JCBones, I checked out ajax with this awesome tutorial:http://www.9lessons.info/2008/12/twitter-used-jquery-plug-in.html which I got working, but I need to get better at php before I start to use that stuff. For anyone that is interested in this post I recommend the following sites http://www.webcheatsheet.com/php/regular_expressions.php#replace http://regexlib.com/ and the parse error: unmatched parentheses means you have one more ")" in your regular expressions than you have "(". the parse error: preg_match() [function.preg-match]: No ending delimiter '^' means you need to end the regular expression with the same character that you started it with. but I'm having problems with my scripts not doing what I intended them to do. form1.php <html> <head> <title>Register</title> <style type="text/css"> td { vertical-align: top; } </style> </head> <body> <form action="form2.php" method="post"> <table> <tr> <td><label for="name">Username:</label></td> <td><input type="text" name="name" id="name" size="20" maxlength="20" value="<?php if(isset($_SESSION['name'])) {echo $_SESSION['name']; }else{ } ?>"/> <?php if(isset($_POST[$errname])) echo $errname; ?></td> </tr><tr> <td><label for="password">Password:</label></td> <td><input type="password" name="password" id="password" size="20" maxlength="20" value=""/> </td> </tr><tr> <td><label for="first_name">First name:</label></td> <td><input type="text" name="first_name" id="first_name" size="20" maxlength="20" value="<?php if(isset($_SESSION['first_name'])) {echo $_SESSION['first_name']; }else{ } ?>"/> <?php if(isset($_POST[$errfirst])) echo $errfirst; ?></td> </tr><tr> <td><label for="last_name">Last name:</label></td> <td><input type="text" name="last_name" id="last_name" size="20" maxlength="20" value="<?php if(isset($_SESSION['last_name'])) {echo $_SESSION['last_name']; }else{ } ?>"/> <?php if(isset($_POST[$errlast])) echo $errlast; ?> </td> </tr><tr> <td><label for="email">Email:</label></td> <td><input type="text" name="email" id="email" size="20" maxlength="50" value="<?php if(isset($_SESSION['email'])) {echo $_SESSION['email']; }else{ } ?>"/> <?php if(isset($_POST[$erremail])) echo $erremail; ?></td> </tr><tr> <td><label for="address">Address:</label></td> <td><input type="text" name="address" id="address" size="20" maxlength="20" value="<?php if(isset($_SESSION['address'])) {echo $_SESSION['address']; }else{ } ?>"/> <?php if(isset($_POST[$erraddress])) echo $erraddress; ?></td> </tr><tr> <td><label for="city">City/Town:</label></td> <td><input type="text" name="city" id="city" size="20" maxlength="20" value="<?php if(isset($_SESSION['city'])) {echo $_SESSION['city']; }else{ } ?>"/> <?php if(isset($_POST[$errcity])) echo $errcity; ?></td> </tr><tr> <td><label for="county">County:</label></td> <td><input type="text" name="county" id="county" size="20" maxlength="20" value="<?php if(isset($_SESSION['county'])) {echo $_SESSION['county']; }else{ } ?>"/> <?php if(isset($_POST[$errcounty])) echo $errcounty; ?></td> </tr><tr> <td><label for="post">Postcode:</label></td> <td><input type="text" name="post" id="post" size="20" maxlength="20" value="<?php if(isset($_SESSION['post'])) {echo $_SESSION['post']; }else{ } ?>"/> <?php if(isset($_POST[$errpost])) echo $errpost; ?></td> </tr><tr> <td><label for="home">Home Number:</label></td> <td><input type="text" name="home" id="home" size="20" maxlength="20" value="<?php if(isset($_SESSION['home'])) {echo $_SESSION['home']; }else{ } ?>"/> <?php if(isset($_POST[$errhome])) echo $errhome; ?></td> </tr><tr> <td><label for="mobile">Mobile:</label></td> <td><input type="text" name="mobile" id="mobile" size="20" maxlength="20" value="<?php if(isset($_SESSION['mobile'])) {echo $_SESSION['mobile']; }else{ } ?>"/> <?php if(isset($_POST[$errmobile])) echo $errmobile; ?></td> </tr><tr> <td> </td> <td><input type="submit" name="submit" value="Sumbit"/></td> </tr> </table> </form> </body> </html> form2.php <?php //let's start the session session_start(); //finally, let's store our posted values in the session variables $_SESSION['name'] = $_POST['name']; $_SESSION['password'] = $_POST['password']; $_SESSION['first_name'] = $_POST['first_name']; $_SESSION['last_name'] = $_POST['last_name']; $_SESSION['email'] = $_POST['email']; $_SESSION['address'] = $_POST['address']; $_SESSION['city'] = $_POST['city']; $_SESSION['county'] = $_POST['county']; $_SESSION['post'] = $_POST['post']; $_SESSION['home'] = $_POST['home']; $_SESSION['mobile'] = $_POST['mobile']; $errname = ""; $errfirst = ""; $errlast = ""; $erremail = ""; $erraddress = ""; $errcity = ""; $errcounty = ""; $errpost = ""; $errhome = ""; $errmob = ""; if( preg_match('/^[A-Z][a-zA-Z -]{3,30}+$/',$_SESSION['name']) ) { $errname = '<p class="errText">Name must be from letters, dashes, spaces and must not start with dash</p>'; } if( preg_match('/^[A-Z][a-zA-Z -]{3,30}+$/',$_SESSION['first_name']) ) { $errfirst = '<p class="errText">Name must be from letters, dashes, spaces and must not start with dash</p>'; } if( preg_match('/^[A-Z][a-zA-Z -]{3,30}+$/',$_SESSION['last_name']) ) { $errlast = '<p class="errText">Name must be from letters, dashes, spaces and must not start with dash</p>'; } if( preg_match('/^[a-zA-Z]\w+(\.\w+)*\@\w+(\.[0-9a-zA-Z]+)*\.[a-zA-Z]{2,4}$/',$_SESSION['email']) ) { $erremail = '<p class="errText">This is not a valid email address.'; } if( preg_match('/^[a-zA-Z0-9 _.,:\"\']+$/',$_SESSION['address']) ) { $erraddress = '<p class="errText">Address must be only letters, numbers or one of the following ". , : /"</p>'; } if( preg_match('/[a-zA-Z]+/',$_SESSION['city']) ) { $errcity = '<p class="errText">Your city must contain a letter.'; } if( preg_match('/[a-zA-Z]+/',$_SESSION['county']) ) { $errcounty = '<p class="errText">Your county must contain a letter.'; } if( preg_match('/(GIR 0AA)|((([A-Z-[QVX]][0-9][0-9]?)|(([A-Z-[QVX]][A-Z-[iJZ]][0-9][0-9]?)|(([A-Z-[QVX]][0-9][A-HJKSTUW])|([A-Z-[QVX]][A-Z-[iJZ]][0-9][ABEHMNPRVWXY])))) [0-9][A-Z-[CIKMOV]]{2})/',$_SESSION['post']) ) { $errpost = '<p class="errText">This is not a valid UK Postcode.'; } if( preg_match('/s*\(?0\d{4}\)?(\s*|-)\d{3}(\s*|-)(\d{3}\s*)|(\s*\(?0\d{3}\)?(\s*|-)\d{3}(\s*|-)\d{4}\s*)|(\s*)(7|(\d{7}|\d{3}(\-|\s{1})\d{4})\s*/',$_SESSION['home']) ) { $errhome = '<p class="errText">This is not a valid UK local phone number.'; } if( preg_match('/^(\+44\s?7\d{3}|\(?07\d{3}\)?)\s?\d{3}\s?\d{3}$/',$_SESSION['mobile']) ) { $errmob = '<p class="errText">This is not a valid UK mobile phone number.'; } else{ $errname = ($_POST[$errname]); $errfirst = ($_POST[$errfirst]); $errlast = ($_POST[$errlast]); $erremail = ($_POST[$erremail]); $erraddress = ($_POST[$erraddress]); $errcity = ($_POST[$errcity]); $errcounty = ($_POST[$errcounty]); $errpost = ($_POST[$errpost]); $errhome = ($_POST[$errhome]); $errmob = ($_POST[$errmob]); header("Location: form1.php"); exit; } ?> <html> <head> <title>Register</title> <style type="text/css"> td { vertical-align: top; } </style> </head> <body> <form action="form3.php" method="post"> <table> <tr> <td><label for="bio">Biography:</label></td> <td><input type="text" name="bio" id="bio" size="400" maxlength="500" value=""/></td> </tr><tr> <td> </td> <td><input type="submit" name="submit" value="Sumbit"/></td> </tr> </table> </form> </body> </html> I want the error messages to come up in form 1 with the session information from form 2. *edit* but it just sends me back to form one with no error messages or session info in the forms.
-
Besides "mysql_real_escape_string"ing all the user input what other security strings should you definitely include n your site?
-
Set up: * XAMPP 1.7.3 * Apache 2.2.14 (IPv6 enabled) + OpenSSL 0.9.8l * MySQL 5.1.41 + PBXT engine * PHP 5.3.1 * phpMyAdmin 3.2.4 * Perl 5.10.1 * FileZilla FTP Server 0.9.33 * Mercury Mail Transport System 4.72 I'm trying to set up a multipage registration script. It's tuff! I've set up some basic scripts to distribute variables into the correct tables from previous forms using a session. But I want the script to check the input from form one is valid before it moves on to form 2. Here are my scripts: form 1: <html> <head> <title>Register</title> <style type="text/css"> td { vertical-align: top; } </style> </head> <body> <form action="form2.php" method="post"> <table> <tr> <td><label for="name">Username:</label></td> <td><input type="text" name="name" id="name" size="20" maxlength="20" value=""/></td> </tr><tr> <td><label for="password">Password:</label></td> <td><input type="password" name="password" id="password" size="20" maxlength="20" value=""/></td> </tr><tr> <td><label for="first_name">First name:</label></td> <td><input type="text" name="first_name" id="first_name" size="20" maxlength="20" value=""/></td> </tr><tr> <td><label for="last_name">Last name:</label></td> <td><input type="text" name="last_name" id="last_name" size="20" maxlength="20" value=""/></td> </tr><tr> <td><label for="email">Email:</label></td> <td><input type="text" name="email" id="email" size="20" maxlength="50" value=""/></td> </tr><tr> <td><label for="address">Address:</label></td> <td><input type="text" name="address" id="address" size="20" maxlength="20" value=""/></td> </tr><tr> <td><label for="city">City/Town:</label></td> <td><input type="text" name="city" id="city" size="20" maxlength="20" value=""/></td> </tr><tr> <td><label for="county">County:</label></td> <td><input type="text" name="county" id="county" size="20" maxlength="20" value=""/></td> </tr><tr> <td><label for="post">Postcode:</label></td> <td><input type="text" name="post" id="post" size="20" maxlength="20" value=""/></td> </tr><tr> <td><label for="home">Home Number:</label></td> <td><input type="text" name="home" id="home" size="20" maxlength="20" value=""/></td> </tr><tr> <td><label for="mobile">Mobile:</label></td> <td><input type="text" name="mobile" id="mobile" size="20" maxlength="20" value=""/></td> </tr><tr> <td> </td> <td><input type="submit" name="submit" value="Sumbit"/></td> </tr> </table> </form> </body> </html> Form 2: <?php //let's start the session session_start(); //now, let's register our session variables session_register('name'); session_register('password'); session_register('first_name'); session_register('last_name'); session_register('email'); session_register('address'); session_register('city'); session_register('county'); session_register('post'); session_register('home'); session_register('mobile'); //finally, let's store our posted values in the session variables $_SESSION['name'] = $_POST['name']; $_SESSION['password'] = $_POST['password']; $_SESSION['first_name'] = $_POST['first_name']; $_SESSION['last_name'] = $_POST['last_name']; $_SESSION['email'] = $_POST['email']; $_SESSION['address'] = $_POST['address']; $_SESSION['city'] = $_POST['city']; $_SESSION['county'] = $_POST['county']; $_SESSION['post'] = $_POST['post']; $_SESSION['home'] = $_POST['home']; $_SESSION['mobile'] = $_POST['mobile']; ?> <html> <head> <title>Register</title> <style type="text/css"> td { vertical-align: top; } </style> </head> <body> <form action="form3.php" method="post"> <table> <tr> <td><label for="bio">Biography:</label></td> <td><input type="text" name="bio" id="bio" size="400" maxlength="500" value=""/></td> </tr><tr> <td> </td> <td><input type="submit" name="submit" value="Sumbit"/></td> </tr> </table> </form> </body> </html> I've also got form3.php and process_forms.php(that's where I mysql_real_escape_string and input the data) but that's probably not relevant. How would I get this to work? Are there any sites I should look at that you'd recommend? Any help appreciated.
-
using a database to store username/password combos.
Namtip replied to amplexus's topic in PHP Coding Help
and damn mark as solved and start up a new post -
Foreign ID doesn't increment with the other tables.
Namtip replied to Namtip's topic in PHP Coding Help
Thanks that works great -
using a database to store username/password combos.
Namtip replied to amplexus's topic in PHP Coding Help
I'm tired :-\ I've changed the code run these scripts. save the script as "db_namtipisawesome". <?php require 'db.inc.php'; $db = mysql_connect(MYSQL_HOST, MYSQL_USER, MYSQL_PASSWORD) or die ('Unable to connect. Check your connection parameters.'); mysql_select_db(MYSQL_DB, $db) or die(mysql_error($db)); // create the user table $query = 'CREATE TABLE IF NOT EXISTS site_user ( user_id INTEGER NOT NULL AUTO_INCREMENT, username VARCHAR(20) NOT NULL, password CHAR(41) NOT NULL, PRIMARY KEY (user_id) ) ENGINE=MyISAM'; mysql_query($query, $db) or die (mysql_error($db)); // populate the user table $query = 'INSERT IGNORE INTO site_user (user_id, username, password) VALUES (1, "namtip", PASSWORD("secret")), (2, "amplexus", PASSWORD("password"))'; mysql_query($query, $db) or die (mysql_error($db)); echo 'Success!'; ?> Save as login.php: <?php session_start(); include 'db.inc.php'; $db = mysql_connect(MYSQL_HOST, MYSQL_USER, MYSQL_PASSWORD) or die ('Unable to connect. Check your connection parameters.'); mysql_select_db(MYSQL_DB, $db) or die(mysql_error($db)); // filter incoming values $username = (isset($_POST['username'])) ? trim($_POST['username']) : ''; $password = (isset($_POST['password'])) ? $_POST['password'] : ''; $redirect = (isset($_REQUEST['redirect'])) ? $_REQUEST['redirect'] : 'main.php'; if (isset($_POST['submit'])) { $query = 'SELECT user_id FROM site_user WHERE ' . 'username = "' . mysql_real_escape_string($username, $db) . '" AND ' . 'password = PASSWORD("' . mysql_real_escape_string($password, $db) . '")'; $result = mysql_query($query, $db) or die(mysql_error($db)); if (mysql_num_rows($result) > 0) { $row = mysql_fetch_assoc($result); $_SESSION['username'] = $username; $_SESSION['logged'] = 1; header ('Refresh: 5; URL=' . $redirect); echo '<p>You will be redirected to your original page request.</p>'; echo '<p>If your browser doesn\'t redirect you properly automatically, ' . '<a href="' . $redirect . '">click here</a>.</p>'; mysql_free_result($result); mysql_close($db); die(); } else { // set these explicitly just to make sure $_SESSION['username'] = ''; $_SESSION['logged'] = 0; $error = '<p><strong>You have supplied an invalid username and/or ' . 'password!</strong> Please <a href="register.php">click here ' . 'to register</a> if you have not done so already.</p>'; } mysql_free_result($result); } ?> <html> <head> <title>Login</title> </head> <body> <?php if (isset($error)) { echo $error; } ?> <form action="login.php" method="post"> <table> <tr> <td>Username:</td> <td><input type="text" name="username" maxlength="20" size="20" value="<?php echo $username; ?>"/></td> </tr><tr> <td>Password:</td> <td><input type="password" name="password" maxlength="20" size="20" value="<?php echo $password; ?>"/></td> </tr><tr> <td> </td> <td> <input type="hidden" name="redirect" value="<?php echo $redirect ?>"/> <input type="submit" name="submit" value="Login"/> </tr> </table> </form> </body> </html> <?php mysql_close($db); ?> Run both scripts in order. I use the include script to hold the variables (MYSQL_HOST, MYSQL_USER, MYSQL_PASSWORD) so I can access my script. Nothing else to do with tables in there. I've changed it so the script checks if the username and password's user_id is above 0 value. Check if it works on values over 3. should ddo but I'm tired and I have to work on my script . because I newbie too. See if you can help on my port yeah? yeah! -
SET UP: Windows vista # XAMPP 1.7.3, # Apache 2.2.14 (IPv6 enabled) + OpenSSL 0.9.8l # MySQL 5.1.41 + PBXT engine # PHP 5.3.1 # phpMyAdmin Problem: The name_id column from the card_numbers table doesn't increment at all. Even if if I put in two rows of data I'm left with the name_id not increasing. Main Goal: I want that column to increment with the same values as the other tables. Code for Mysql database: <?php require 'db.inc.php'; $db = mysql_connect(MYSQL_HOST, MYSQL_USER, MYSQL_PASSWORD) or die ('Unable to connect. Check your connection parameters.'); mysql_select_db(MYSQL_DB, $db) or die(mysql_error($db)); $query = 'CREATE TABLE IF NOT EXISTS subscriptions ( name_id INTEGER UNSIGNED NOT NULL AUTO_INCREMENT, name VARCHAR(50) NOT NULL, email_address VARCHAR(50), membership_type VARCHAR(50), PRIMARY KEY (name_id) ) ENGINE=MyISAM'; mysql_query($query, $db) or die (mysql_error($db)); // create the user information table $query = 'CREATE TABLE IF NOT EXISTS site_user_info ( name_id INTEGER UNSIGNED NOT NULL, terms_and_conditions VARCHAR(50) NOT NULL, name_on_card VARCHAR(50), credit_card_number VARCHAR(50), FOREIGN KEY (name_id) REFERENCES subscriptions(name_id) ) ENGINE=MyISAM'; mysql_query($query, $db) or die (mysql_error($db)); $query = 'CREATE TABLE IF NOT EXISTS card_numbers ( name_id INTEGER UNSIGNED NOT NULL, credit_card_expiration_data VARCHAR(50), FOREIGN KEY (name_id) REFERENCES subscriptions(name_id) ) ENGINE=MyISAM'; mysql_query($query, $db) or die (mysql_error($db)); echo 'Success!'; ?> Code of the data being inserted into mysql from forms: <?php //let's start our session, so we have access to stored data session_start(); session_register('membership_type'); session_register('terms_and_conditions'); include 'db.inc.php'; $db = mysql_connect('localhost', 'root', '') or die ('Unable to connect. Check your connection parameters.'); mysql_select_db('ourgallery', $db) or die(mysql_error($db)); //let's create the query $query = sprintf("INSERT INTO subscriptions ( name_id, name, email_address, membership_type) VALUES ('%s','%s','%s','%s')", name_id, mysql_real_escape_string($_SESSION['name']), mysql_real_escape_string($_SESSION['email_address']), mysql_real_escape_string($_SESSION['membership_type'])); //let's run the query $result = mysql_query($query, $db) or die(mysql_error($db)); $name_id = mysql_insert_id(); $query = sprintf("INSERT INTO site_user_info ( name_id, terms_and_conditions, name_on_card, credit_card_number ) VALUES ('%s','%s','%s','%s')", mysql_insert_id(), mysql_real_escape_string($_SESSION['terms_and_conditions']), mysql_real_escape_string($_POST['name_on_card']), mysql_real_escape_string($_POST['credit_card_number'])); //let's run the query $result = mysql_query($query, $db) or die(mysql_error($db)); $name_id = mysql_insert_id(); $query = sprintf("INSERT INTO card_numbers ( name_id, credit_card_expiration_data) VALUES ('%s','%s')", mysql_insert_id(), mysql_real_escape_string($_POST['credit_card_expiration_data'])); $result = mysql_query($query, $db) or die(mysql_error($db)); echo '$result'; ?> Should I be JOINING these tables together? I don't want the IDs to get muddled up otherwise it will screw up my database.
-
using a database to store username/password combos.
Namtip replied to amplexus's topic in PHP Coding Help
Hello amplexus! Nice to hear from you again, I'm sorry I'm late with my reply I wasn't checking up on the post because I thought you didn't like my script. Bet you love me now eh? OK, my friend, admin_level isn't a mysql function. It is the name of a column in the data base code I was using(does that make sense?). The column would give some users special privileges in the site. So If I were you I'd just delete the relevant code. So you've loaded the script with no erros but you can't log in? I'm guessing you're getting the error message from the script you've written not a parse error( if its a parse error show us? If not, hmm.. I'm guessing the admin_level column and the login script don't match. Could you post your database stuff? Don't worry I'll be checking your post frequently tonight and tomorrow. hopefully get something sorted tonight. But yeah post your mysql database up please. -
Set up Windows Vista * XAMPP 1.7.3, including: * Apache 2.2.14 (IPv6 enabled) + OpenSSL 0.9.8l * MySQL 5.1.41 + PBXT engine * PHP 5.3.1 * phpMyAdmin Ultimate objective: I want to insert session data into multiple tables whilst ensuring that the data is in the appropriate column. Problem: I managed to insert the data into the correct tables and column, but after reading various forums I have been given the impression that if I was to have multiple site users the data's columns could get muddled up if they execute the script at the same time (hope I'm making sense, say if I'm not). The suggested method was mysql_insert_id() but I do not know how to make this work with in conjunction with sprintf(). As I said the script worked before I added the code with the star by it in an attempt to reach my ultimate objective. <?php //let's start our session, so we have access to stored data session_start(); session_register('membership_type'); session_register('terms_and_conditions'); include 'db.inc.php'; $db = mysql_connect('localhost', 'root', '') or die ('Unable to connect. Check your connection parameters.'); mysql_select_db('ourgallery', $db) or die(mysql_error($db)); //let's create the query $query = sprintf("INSERT INTO subscriptions ( name, email_address, membership_type) VALUES ('%s','%s','%s')", mysql_real_escape_string($_SESSION['name']), mysql_real_escape_string($_SESSION['email_address']), mysql_real_escape_string($_SESSION['membership_type'])); //let's run the query $result = mysql_query($query, $db) or die(mysql_error($db)); *mysql_real_escape_string($_SESSION['name']) = mysql_insert_id($db);* $query = sprintf("INSERT INTO site_user_info ( terms_and_conditions, name_on_card, credit_card_number ) VALUES ('%s','%s','%s')", *mysql_real_escape_string($_SESSION['name']),* mysql_real_escape_string($_SESSION['terms_and_conditions']), mysql_real_escape_string($_POST['name_on_card']), mysql_real_escape_string($_POST['credit_card_number']), mysql_real_escape_string($_POST['credit_card_expiration_data'])); //let's run the query $result = mysql_query($query, $db) or die(mysql_error($db)); *mysql_real_escape_string($_SESSION['credit_card_number']) = mysql_insert_id($db);* $query = sprintf("INSERT INTO card_numbers ( credit_card_expiration_data) VALUES ('%s')", *mysql_real_escape_string($_POST['credit_card_number']),* mysql_real_escape_string($_POST['credit_card_expiration_data'])); $result = mysql_query($query, $db) or die(mysql_error($db)); echo '$result'; ?> All the other facets of the script work but I get the following error message with the above script: Fatal error: Can't use function return value in write context in C:\x\xampp\htdocs\form_process.php on line 27 But it's not so much the error message its the ultimate objective. Any help appreciated.
-
SQL errors as I attempt split data from a session into two tables
Namtip replied to Namtip's topic in PHP Coding Help
Thanks buddy fixed. -
SET UP: Windows vista # XAMPP 1.7.3, # Apache 2.2.14 (IPv6 enabled) + OpenSSL 0.9.8l # MySQL 5.1.41 + PBXT engine # PHP 5.3.1 # phpMyAdmin Error message: You have an error in your SQL syntax; check the manual that corresponds to your MySQL server version for the right syntax to use near ') VALUES ('qwerty','uiop','asd')' at line 2 I'm trying to get this multi page order form to insert information into two tables via a session. But it comes up with the above error message. This script worked perfectly with one table but as soon as I coded he information to go into two tables it screwed up. Is it the sprint <?php //let's start our session, so we have access to stored data session_start(); session_register('membership_type'); session_register('terms_and_conditions'); include 'db.inc.php'; $db = mysql_connect('localhost', 'root', '') or die ('Unable to connect. Check your connection parameters.'); mysql_select_db('ourgallery', $db) or die(mysql_error($db)); //let's create the query $query = sprintf("INSERT INTO subscriptions ( name, email_address, membership_type,) VALUES ('%s','%s','%s')", mysql_real_escape_string($_SESSION['name']), mysql_real_escape_string($_SESSION['email_address']), mysql_real_escape_string($_SESSION['membership_type'])); //let's run the query $result = mysql_query($query, $db) or die(mysql_error($db)); $query = sprintf("INSERT INTO site_user_info ( terms_and_conditions, name_on_card, credit_card_number, credit_card_expiration_data) VALUES ('%s','%s','%s','%s')", mysql_real_escape_string($_SESSION['terms_and_conditions']), mysql_real_escape_string($_POST['name_on_card']), mysql_real_escape_string($_POST['credit_card_number']), mysql_real_escape_string($_POST['credit_card_expiration_data'])); //let's run the query $result = mysql_query($query, $db) or die(mysql_error($db)); echo '$result'; ?> I'm trying to insert into this database: <?php require 'db.inc.php'; $db = mysql_connect(MYSQL_HOST, MYSQL_USER, MYSQL_PASSWORD) or die ('Unable to connect. Check your connection parameters.'); mysql_select_db(MYSQL_DB, $db) or die(mysql_error($db)); $query = 'CREATE TABLE IF NOT EXISTS subscriptions ( name VARCHAR(50) NOT NULL, email_address VARCHAR(50), membership_type VARCHAR(50), PRIMARY KEY (name) ) ENGINE=MyISAM'; mysql_query($query, $db) or die (mysql_error($db)); // create the user information table $query = 'CREATE TABLE IF NOT EXISTS site_user_info ( name VARCHAR(50) NOT NULL, terms_and_conditions VARCHAR(50) NOT NULL, name_on_card VARCHAR(50), credit_card_number VARCHAR(50), credit_card_expiration_data VARCHAR(50), FOREIGN KEY (name) REFERENCES subscriptions(name) ) ENGINE=MyISAM'; mysql_query($query, $db) or die (mysql_error($db)); echo 'Success!'; ?> What am I doing wrong? is there a code spell checker ? Also should I use the mysql_real_escape_string() on the user input as they become sessions variables or is it okay to wait and clean the input as it gets inserted in the table? Thanks for your help.
-
sql error on Multi-page Order script (using session).
Namtip replied to Namtip's topic in PHP Coding Help
PFMaBiSmAd, thanks. I did that and worked out the error by myself . It turned out that it was exactly what mad techie said (I really did figure it out before I read his post)! It's fixed! I have a multi-page script that inserts information into a database. Watch out facebook! Thanks guys. -
SET UP: Windows vista # XAMPP 1.7.3, # Apache 2.2.14 (IPv6 enabled) + OpenSSL 0.9.8l # MySQL 5.1.41 + PBXT engine # PHP 5.3.1 # phpMyAdmin So I'm getting this error message: You have an error in your SQL syntax; check the manual that corresponds to your MySQL server version for the right syntax to use near '", )' at line 8. I can't see where the '", )' code is in that script. I've looked and contrl + find it but no joy. I've looked through the previous pages and mysql database to ensure the variables and the tables match up. Any help would be appreciated. Maybe some good mysql debug advice. Is there a good debug open source program out there? Any help would be appreciated. :'( <?php //let's start our session, so we have access to stored data session_start(); include 'db.inc.php'; $db = mysql_connect('localhost', 'root', '') or die ('Unable to connect. Check your connection parameters.'); mysql_select_db('ourgallery', $db) or die(mysql_error($db)); //let's create the query $query = sprintf("INSERT INTO subscriptions ( name, email_address, membership_type, terms_and_conditions, name_on_card, credit_card_number, credit_card_expiration_data) VALUES ('%s','%s','%s','%s','%s','%s','%s')", $_SESSION['email_address'], $_SESSION['membership_type'], $_SESSION['terms_and_conditions'], $_POST['name_on_card'], $_POST['credit_card_number'], $_POST['credit_card_expiration_data']); //let's run the query mysql_query($insert_query); ?>