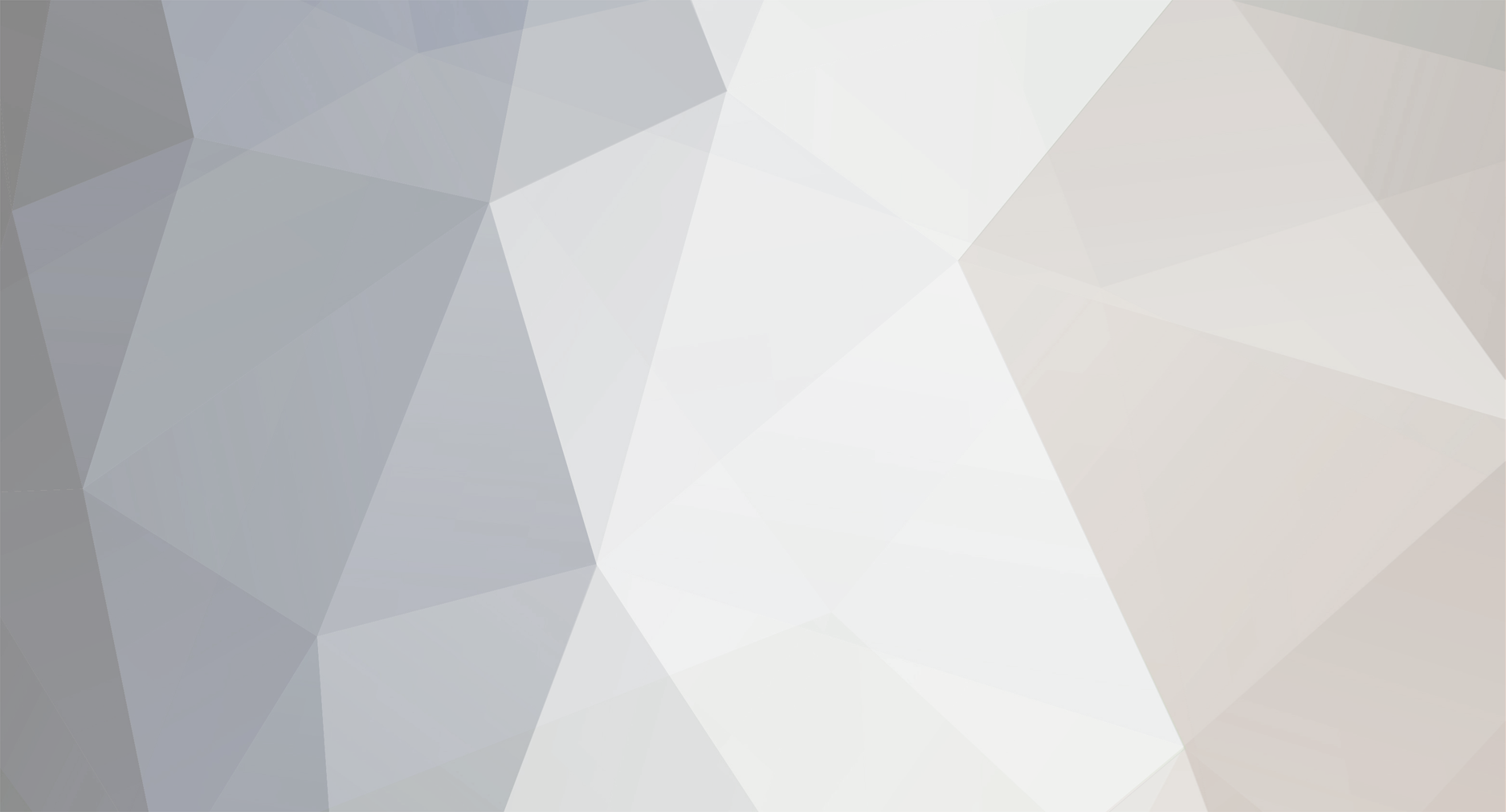
zachatk1
Members-
Posts
38 -
Joined
-
Last visited
Never
Everything posted by zachatk1
-
I hate to be the guy to bump this... perhaps I worded the question a little bit too long!
-
My site is nearly done. This is the last bug I have to work out. I have a form and a user can fill out all the fields. I have the data $_POST over onto the verify page into $_SESSION variables (no reason really... it can just be a variable I suppose and no need for a session). Each variable is checked to see if it is filled out. If it is not, it re-displays that input field that wasn't filled in. The input fields that are filled in are put into a hidden input so when the user re-submits the form after filling out the forgotten field, the already filled in data is there. Now here's the problem. There is a large textarea form. The problem is with quotes. I'll give an example with some code: //Bunch of variables... $_SESSION['type'] = $_POST["type"]; //radio buttons //ect... //Large text form $_SESSION['details'] = $_POST["details"]; //not escaping strings, explanation and example later //TYPE if ( empty($_SESSION['type']) ) { $flag[] = 1; ?> <input type="radio" name="type" value="Modification" /> Modification <input type="radio" name="type" value="Maintenance" /> Maintenance <?php } else { echo $_SESSION['type']; ?> <input type="hidden" name="type" value="<?php print $_SESSION['type'] ?>" /> //put back in form <?php } //DETAILS if ( empty($_SESSION['details']) ) { $flag[] = 1; ?> //this is a tinymce form, not sure if that makes a difference, but it could output something different. <textarea name="details" style="width:100%"> </textarea> <?php } else { echo $_SESSION['details']; ?> <input type="hidden" name="details" value="<?php print $_SESSION['details'] ?>" /> <?php } ?> //theres a bunch of other error checking with the other variables... <input type="submit" value="Submit" name="different" class="btn" /> //submit button //now check if flag = 1 which means theres an error <?php if ( in_array ( 1, $flag ) ) { } else { //this is where the data will be sanitized, escaped and submitted to the database... } So for example if I were to type this into the details box WITHOUT mysql_real_escape_string: This is a "quote". It would output this the first time: This is a "quote". This is perfect and how it should be. But lets say the user forgot another text box. This is now sending the data again through the whole $_SESSION POST deal. This is how it looks sending it through again: This is a That's it! No quotes and everything past the first quote is gone. What's the deal with that!? Now if I were to do mysql_real_escape_string on the $_SESSION variable $_POST, it'd work the first time like this: This is a \"quote\". But the the second time it's this: This is a \\ Now there's multiple ways to fix this I believe... except I don't really know how to use these methods. I believe I could check if the characters are entities, then convert them to entities if they aren't. So a quote would be " instead of ". My original plan was to have the data that was submitted go onto a session that would store it until everything was correct. Then pull it off the session and sanitize and submit. For some reason I couldn't get that to work because if I have those $_SESSION['var'] = $_POST["var"]; at the top of the page it will overwrite the existing data on the session. To fix this I'd have to check if that specific variable was $_POST'ed, but I don't exactly know how to do that (if you even can). Wow, that was long... hopefully you understand, thanks!
-
WOW... duh. Can't believe I never saw that... thanks!
-
Here's the code: $year_values = array('Any', 'N/A', '2000', '2001', '2002', '2003', '2004', '2005', '2006', '2007', '2008', '2009', '2010', '2011', '2012'); $db_year = explode(', ', $row['YEAR']); foreach($year_values as $year_value) { $selected_year = null; $year_label = $year_value; if(in_array($year_value, $db_year)) { $selected_year = ' checked="checked"'; $year_label = "<b>$year_value</b>"; } echo '<input type="checkbox" name="year" value="' . $year_value . '"' . $selected_year . ' />' . $year_label . ' '; } ?> [/code That pulls the data of the database and puts it in check boxes. The user can check/uncheck certain boxes but the problem is when it goes to the verify page it has only one value from the array that was selected. On the verify page it just checks if a box was checked and then implodes the array before submitting it into the database. [code] $year_s=implode($year,", "); So like I said there is only one value from the array when there should be as many as the user checks. So basically I believe the value isn't added to the array.
-
Hey, I don't know javascript and I have this code that is a dynamic drop down list. The 2 PHP variables are these: $sel1 = $_POST["sel1"] $sel2 = $_POST["sel2"] Here's the javascript, I took out the huge amounts of selections: First_selection = new Array( "1", "2", "3", .... Lots of data ); First selection second array = new Array( ...more data ); function changeval() { var val1 = document.change.sel1.value; var optionArray = eval(val1); for(var df=0; df<optionArray.length; df++) { var ss = document.change.sel2; ss.options.length = 0; for(var ff=0; ff<optionArray.length; ff++) { var val = optionArray[ff]; ss.options[ff] = new Option(val,val); } } } </script> <form name="change" action="verify.php" method="post"> <select name=sel1 onchange=changeval()> <script type="text/javascript"> for(var model=0; model<make.length; model++) { document.write("<option value=\""+make[model]+"\">"+make[model]+"</option>"); } </script> </select> <select name=sel2> </select> When the page loads, the drop down menu needs to have sel1 and sel2 selected in the drop down list. Any ideas?
-
Is there a way to check if a value needs to be imploded and if it does, it implodes the variable? And vice versa with explode? How would this be done? Thanks!
-
WHERE clause messing up query for pagination!
zachatk1 replied to zachatk1's topic in PHP Coding Help
Thanks everyone! I got it figured out!!! -
WHERE clause messing up query for pagination!
zachatk1 replied to zachatk1's topic in PHP Coding Help
Wow, sorry! I can't believe I didn't see that missing ). Except the code is triggering the error handling part. -
WHERE clause messing up query for pagination!
zachatk1 replied to zachatk1's topic in PHP Coding Help
Oh I should have probably said that sel2 is the model! That's why $_SESSION['model'] = $_POST['sel2']. I tried your code, but I get this: Parse error: syntax error, unexpected '{' -
WHERE clause messing up query for pagination!
zachatk1 replied to zachatk1's topic in PHP Coding Help
That makes sense! So I tried doing a session but I get the same thing. I'm not exactly sure how to have it post to the same page, so I don't know if this is right. session_start(); $_SESSION['make'] = $_POST["sel1"]; $_SESSION['model'] = $_POST["sel2"]; //then i just stuck $_SESSION['make and model'] in the search query like this: $sql = "SELECT COUNT(*) FROM ACTIVE WHERE MODEL='$_SESSION[model]'"; //and $sql = "SELECT * FROM ACTIVE WHERE MODEL='$_SESSION[model]' ORDER BY INDEX_ID DESC LIMIT $offset, $rowsperpage"; I think I need to retrieve the session, but I'm not exactly sure how... -
I had originally created a topic on my pagination not working, but I found out what the problem was. The topic I had created was irrelevant to what the problem was... but anyway, here's my situation: I've got a query that searches in a MySQL table for a certain value. Then it list's all the findings in a table (pretty simple). Anyway, I obviously want to have a pagination system to make it easier to manage the amount of data per page. The pagination works fine, it's used for another query that just pulls data (so NO WHERE clause is used!). That is what is causing the problem. I spent hours trying to figure out why this is happening, then I figured out that the only difference between the pagination that works and the one that doesn't is the query have the where clause. What happens when the where clause is used is that the pagination works fine on the first page, but if you click the link to go to a different page, the page is empty and turns up no results so the mysql data that is pulled is lost. So here is my code. I don't know exactly why the where clause causes the pagination to not work. $conn = mysql_connect("$mysql_host","$mysql_user","$mysql_password") or trigger_error("SQL", E_USER_ERROR); $db = mysql_select_db("$mysql_database",$conn) or trigger_error("SQL", E_USER_ERROR); // find out how many rows are in the table $sql = "SELECT COUNT(*) FROM ACTIVE WHERE MODEL='$model'"; $result = mysql_query($sql, $conn) or trigger_error("SQL", E_USER_ERROR); $r = mysql_fetch_row($result); $numrows = $r[0]; // number of rows to show per page $rowsperpage = 5; // find out total pages $totalpages = ceil($numrows / $rowsperpage); // get the current page or set a default if (isset($_GET['currentpage']) && is_numeric($_GET['currentpage'])) { // cast var as int $currentpage = (int) $_GET['currentpage']; } else { // default page num $currentpage = 1; } // end if // if current page is greater than total pages... if ($currentpage > $totalpages) { // set current page to last page $currentpage = $totalpages; } // end if // if current page is less than first page... if ($currentpage < 1) { // set current page to first page $currentpage = 1; } // end if // the offset of the list, based on current page $offset = ($currentpage - 1) * $rowsperpage; $sql = "SELECT * FROM ACTIVE WHERE MODEL='$model' ORDER BY INDEX_ID DESC LIMIT $offset, $rowsperpage"; $result = mysql_query($sql, $conn) or die('Error: ' . mysql_error()); echo " <table width=100% align=center border=1 class=\"mod\"><tr> <td align=center bgcolor=#00FFFF><b>Rating</b></td> <td align=center bgcolor=#00FFFF><b>Title</b></td> <td align=center bgcolor=#00FFFF><b>Make/Model</b></td> <td align=center bgcolor=#00FFFF><b>Type</b></td> <td align=center bgcolor=#00FFFF><b>Difficulty</b></td> <td align=center bgcolor=#00FFFF><b>Comments</b></td> <td align=center bgcolor=#00FFFF><b>Views</b></td> </tr>"; while ($row = mysql_fetch_assoc($result)) { { // Begin while $title = $row["TITLE"]; $type = $row["TYPE"]; $difficulty = $row["DIFFICULTY"]; $comments = $row["COMMENT_TOTAL"]; $id = $row['INDEX_ID']; $rating = $row["RATING_AVERAGE"]; $make = $row["MAKE"]; $model = $row["MODEL"]; $views = $row["HITS"]; echo " <tr> <td>$rating/10</td> <td><a href=\"mod.php?id=$id\">$title</a></td> <td>$make - $model</td> <td>$type</td> <td>$difficulty</td> <td>$comments</td> <td>$views</td> </tr>"; } } echo "</table><br />"; /****** build the pagination links ******/ echo "<center>"; // range of num links to show $range = 3; echo "<i>Page: </i>"; // if not on page 1, don't show back links if ($currentpage > 1) { // get previous page num $prevpage = $currentpage - 1; // show < link to go back to 1 page echo " <a stylehref='{$_SERVER['PHP_SELF']}?currentpage=$prevpage'>Previous</a> - "; } // end if // loop to show links to range of pages around current page for ($x = ($currentpage - $range); $x < (($currentpage + $range) + 1); $x++) { // if it's a valid page number... if (($x > 0) && ($x <= $totalpages)) { // if we're on current page... if ($x == $currentpage) { // 'highlight' it but don't make a link echo " <b>$x</b> "; // if not current page... } else { // make it a link echo " <a href='{$_SERVER['PHP_SELF']}?currentpage=$x'>$x</a> "; } // end else } // end if } // end for // if not on last page, show forward and last page links if ($currentpage != $totalpages) { // get next page $nextpage = $currentpage + 1; // echo forward link for next page echo " - <a href='{$_SERVER['PHP_SELF']}?currentpage=$nextpage'>Next</a> "; } // end if /****** end build pagination links ******/ mysql_close($conn); } echo "$pagination</center>"; ?> I really appreciate the help! Thanks.
-
I don't understand why this is happening. I use the same exact code (but different mysql table) and the pagination works fine. Here's the code: ..... <body id="set-database"> <div class="wrapper"> <?php include("navigation.php"); ?> <div class="full"> <?php $make = $_POST["sel1"]; $model = $_POST["sel2"]; echo "$make - $model"; if ( $make == "--MAKES--" ) { echo "<div class=\"red\">Please select a Make</div>"; } else { echo "<center><font color=\"#3333FF\">$make</font> - <font color=\"#3333FF\">$model</font></center><br />"; $mysql_host = -- $mysql_database= -- $mysql_user = -- $mysql_password = -- $conn = mysql_connect("$mysql_host","$mysql_user","$mysql_password") or trigger_error("SQL", E_USER_ERROR); $db = mysql_select_db("$mysql_database",$conn) or trigger_error("SQL", E_USER_ERROR); // find out how many rows are in the table $sql = "SELECT COUNT(*) FROM ACTIVE WHERE MODEL='$model'"; $result = mysql_query($sql, $conn) or trigger_error("SQL", E_USER_ERROR); $r = mysql_fetch_row($result); $numrows = $r[0]; // number of rows to show per page $rowsperpage = 5; // find out total pages $totalpages = ceil($numrows / $rowsperpage); // get the current page or set a default if (isset($_GET['currentpage']) && is_numeric($_GET['currentpage'])) { // cast var as int $currentpage = (int) $_GET['currentpage']; } else { // default page num $currentpage = 1; } // end if // if current page is greater than total pages... if ($currentpage > $totalpages) { // set current page to last page $currentpage = $totalpages; } // end if // if current page is less than first page... if ($currentpage < 1) { // set current page to first page $currentpage = 1; } // end if // the offset of the list, based on current page $offset = ($currentpage - 1) * $rowsperpage; $sql = "SELECT * FROM ACTIVE WHERE MODEL='$model' ORDER BY INDEX_ID DESC LIMIT $offset, $rowsperpage"; $result = mysql_query($sql, $conn) or die('Error: ' . mysql_error()); echo " <table width=100% align=center border=1 class=\"mod\"><tr> <td align=center bgcolor=#00FFFF><b>Rating</b></td> <td align=center bgcolor=#00FFFF><b>Title</b></td> <td align=center bgcolor=#00FFFF><b>Make/Model</b></td> <td align=center bgcolor=#00FFFF><b>Type</b></td> <td align=center bgcolor=#00FFFF><b>Difficulty</b></td> <td align=center bgcolor=#00FFFF><b>Comments</b></td> <td align=center bgcolor=#00FFFF><b>Views</b></td> </tr>"; while ($row = mysql_fetch_assoc($result)) { { // Begin while $title = $row["TITLE"]; $type = $row["TYPE"]; $difficulty = $row["DIFFICULTY"]; $comments = $row["COMMENT_TOTAL"]; $id = $row['INDEX_ID']; $rating = $row["RATING_AVERAGE"]; $make = $row["MAKE"]; $model = $row["MODEL"]; $views = $row["HITS"]; echo " <tr> <td><div class=\"\">$rating/10</div></td> <td><div class=\"\"><a href=\"mod.php?id=$id\">$title</a></div></td> <td><div class=\"\">$make - $model</div></td> <td><div class=\"\">$type</div></td> <td><div class=\"\">$difficulty</div></td> <td><div class=\"\">$comments</div></td> <td><div class=\"\">$views</div></td> </tr>"; } } echo "</table><br />"; /****** build the pagination links ******/ echo "<center>"; // range of num links to show $range = 3; echo "<i>Page: </i>"; // if not on page 1, don't show back links if ($currentpage > 1) { // get previous page num $prevpage = $currentpage - 1; // show < link to go back to 1 page echo " <a stylehref='{$_SERVER['PHP_SELF']}?currentpage=$prevpage'>Previous</a> - "; } // end if // loop to show links to range of pages around current page for ($x = ($currentpage - $range); $x < (($currentpage + $range) + 1); $x++) { // if it's a valid page number... if (($x > 0) && ($x <= $totalpages)) { // if we're on current page... if ($x == $currentpage) { // 'highlight' it but don't make a link echo " <b>$x</b> "; // if not current page... } else { // make it a link echo " <a href='{$_SERVER['PHP_SELF']}?currentpage=$x'>$x</a> "; } // end else } // end if } // end for // if not on last page, show forward and last page links if ($currentpage != $totalpages) { // get next page $nextpage = $currentpage + 1; // echo forward link for next page echo " - <a href='{$_SERVER['PHP_SELF']}?currentpage=$nextpage'>Next</a> "; } // end if /****** end build pagination links ******/ mysql_close($conn); } echo "$pagination</center>"; ?> <br /> <FORM><INPUT TYPE="button" class="btn" VALUE="Back" onClick="history.go(-1);return true;"> </FORM> </div> <?php include("footer.html"); ?> When I click the link for the next page and goes to it, there is nothing in the table (so the information isn't there). So the links and everything bring you the correct page, but that page doesn't have any of the information. Like I said, I use the same exact code except it pulls different information and that works no problem. Thanks!
-
Pulling MySQL data for check boxes and radio buttons.
zachatk1 replied to zachatk1's topic in PHP Coding Help
Ok so for 2 things in the years array, this is what I got. Array ( [0] => N/A, 2004 ) Here's what the form looks like: <input type="checkbox" name="year[0]" value="Any" /> Any <input type="checkbox" name="year[1]" value="N/A" /> N/A <br /><br /> <input type="checkbox" name="year[2]" value="2000" /> 2000 <input type="checkbox" name="year[3]" value="2001" /> 2001 <input type="checkbox" name="year[4]" value="2002" /> 2002 <input type="checkbox" name="year[5]" value="2003" /> 2003 <input type="checkbox" name="year[6]" value="2004" /> 2004 <input type="checkbox" name="year[7]" value="2005" /> 2005 <input type="checkbox" name="year[8]" value="2006" /> 2006 <input type="checkbox" name="year[9]" value="2007" /> 2007 <input type="checkbox" name="year[10]" value="2008" /> 2008 <input type="checkbox" name="year[11]" value="2009" /> 2009 <input type="checkbox" name="year[12]" value="2010" /> 2010 <input type="checkbox" name="year[13]" value="2011" /> 2011 <input type="checkbox" name="year[14]" value="2012" /> 2012 Now here is where it is processed. $year = $_POST["year"]; //now checks if it was filled out //YEAR if(count($_POST['year'])==0) { echo "<div class=\"red\"><font color=\"#ff0000\">Please go back and check a Year Box</font></div>"; echo "<div class=\"space\"></div>"; $flag[] = 1; } else { $year_s=implode($year,", "); echo "Year(s): <font color=\"#3333FF\">$year_s </font><br /><br />"; } If $flag is 1, it won't submit the data to the database. If it's ok, it will submit the info, and redirect the user to the topic they just created. $year_s is the variable submitted to the base (the one that is imploded). I have a feeling that the implode has something to do with it not working. -
Pulling MySQL data for check boxes and radio buttons.
zachatk1 replied to zachatk1's topic in PHP Coding Help
Ok so here is everything used for the check boxes: $connect = mysql_connect("$mysql_host", "$mysql_user", "$mysql_password") or die(mysql_error()); mysql_select_db("$mysql_database") or die(mysql_error()); $res=mysql_query("SELECT * FROM ACTIVE WHERE INDEX_ID=$id"); if(mysql_num_rows($res)==0) echo "There is no data in the table <br /> <br />"; else { for($i=0;$i<mysql_num_rows($res);$i++) { $row=mysql_fetch_assoc($res); $db_year[] = $row[YEAR]; } } $year_values = array('Any', 'N/A', '2000', '2001', '2002', '2003', '2004', '2005', '2006', '2007', '2008', '2009', '2010', '2011', '2012'); <?php foreach($year_values as $year_value) { $selected_year = null; $year_label = $year_value; if(in_array($year_value, $db_year)) { $selected_year = ' checked="checked"'; $year_label = "<b>$year_value</b>"; } echo '<input type="checkbox" name="year" value="' . $year_value . '"' . $selected_year . ' />' . $year_label . ' '; } ?> Yea like I said, it doesn't work when more than one box is selected (but does work with only one selected). -
Pulling MySQL data for check boxes and radio buttons.
zachatk1 replied to zachatk1's topic in PHP Coding Help
Thanks!! It works great... except I think I have one problem. There is one input that has like 12 checkboxes... so more than one checkbox can be selected. When one is selected, it works fine. But when there is more than one, nothing is selected or bolded. -
Pulling MySQL data for check boxes and radio buttons.
zachatk1 replied to zachatk1's topic in PHP Coding Help
Great it works!! Now only thing, how would you have the check box be bolded (so if the user clicked a different radio box but wasn't sure what they had)? -
Pulling MySQL data for check boxes and radio buttons.
zachatk1 replied to zachatk1's topic in PHP Coding Help
Yea, it's like if you went to go edit your profile on this forum on some radio buttons. Your previous selection would be the checked bullet when you first filled it out so you know what you previously put. Anyway, I tried wildteens script and am getting an error on the 3rd line in the 3rd script (if statement). Parse error: syntax error, unexpected T_IF -
Hey! I have it so users can submit info to my site. They have to check some check boxes and radio buttons. I want them to be able to edit this information, so I want to have the data pulled from the MySQL database and have the correct radio button selected (and the text bolded). So I'm thinking some sort of array to check for each value in the database... but I don't really know. There are a few groups of radio boxes and check boxes but here is an example of one. <input type="radio" name="stage" value="Stage 1" /> Stage 1 <input type="radio" name="stage" value="Stage 2" /> Stage 2 <input type="radio" name="stage" value="Stage 3" /> Stage 3 The information is pulled from the database like this: $res=mysql_query("SELECT * FROM ACTIVE WHERE INDEX_ID=$id"); if(mysql_num_rows($res)==0) echo "There is no data in the table <br /> <br />"; else { for($i=0;$i<mysql_num_rows($res);$i++) { $row=mysql_fetch_assoc($res); } } So when I pull information (lets say I want to echo it) it'd look like this: $row[sTAGE] The value for the stage selection can only be Stage 1, Stage 2 or Stage 3 so I need it to be selected when the page loads. Thanks!
-
Hi. I'm trying to make a horizontal navigation bar on my site. I want it to be centered on the page and all the buttons to be next to each other. So here's my CSS: .home { display: block; width: 86px; height: 34px; background: url('/img/nav/home.gif') bottom; } .home:hover { background: url('/img/nav/home-lit.gif') bottom; text-align: right; } I have a total of 5 of these that I want lined up next to each other horizontally and centered. My HTML is this: <body> <div class="main"> <div class="navigation"> <center> <a class="home" href="/index.php"></a> <a class="database" href="/database.php"></a> <a class="rankings" href="/rankings.php"></a> <a class="contact" href="/contact.php"></a> <a class="submit" href="/submit.php"></a> </center> </div> </div> </body> For some reason the images are vertically stacked and to the left. Any remedy to this?
-
Sorry for the double post. I don't know how to edit my previous one. I uploaded the pages to a web host and it gives me the standard error messages. When I have errors and I preview the page on the local server it has this orange tables that have a call stack title. Is there a way to view errors like I'm used to with a web host?
-
Whoah! I put the php after the ? and now have this huge thing of code being displayed. There are some errors also. Maybe all the file paths aren't correct but I never had this problem when I had this same code on a web host. Is it my PHP configuration or something along those lines? Here's what it says: time = time(); $this->startSession(); } /** * startSession - Performs all the actions necessary to * initialize this session object. Tries to determine if the * the user has logged in already, and sets the variables * accordingly. Also takes advantage of this page load to * update the active visitors tables. */ function startSession(){ global $database; //The database connection session_start(); //Tell PHP to start the session /* Determine if user is logged in */ $this->logged_in = $this->checkLogin(); /** * Set guest value to users not logged in, and update * active guests table accordingly. */ if(!$this->logged_in){ $this->username = $_SESSION['username'] = GUEST_NAME; $this->userlevel = GUEST_LEVEL; $database->addActiveGuest($_SERVER['REMOTE_ADDR'], $this->time); } /* Update users last active timestamp */ else{ $database->addActiveUser($this->username, $this->time); } /* Remove inactive visitors from database */ $database->removeInactiveUsers(); $database->removeInactiveGuests(); /* Set referrer page */ if(isset($_SESSION['url'])){ $this->referrer = $_SESSION['url']; }else{ $this->referrer = "/"; } /* Set current url */ $this->url = $_SESSION['url'] = $_SERVER['PHP_SELF']; } /** * checkLogin - Checks if the user has already previously * logged in, and a session with the user has already been * established. Also checks to see if user has been remembered. * If so, the database is queried to make sure of the user's * authenticity. Returns true if the user has logged in. */ function checkLogin(){ global $database; //The database connection /* Check if user has been remembered */ if(isset($_COOKIE['cookname']) && isset($_COOKIE['cookid'])){ $this->username = $_SESSION['username'] = $_COOKIE['cookname']; $this->userid = $_SESSION['userid'] = $_COOKIE['cookid']; } /* Username and userid have been set and not guest */ if(isset($_SESSION['username']) && isset($_SESSION['userid']) && $_SESSION['username'] != GUEST_NAME){ /* Confirm that username and userid are valid */ if($database->confirmUserID($_SESSION['username'], $_SESSION['userid']) != 0){ /* Variables are incorrect, user not logged in */ unset($_SESSION['username']); unset($_SESSION['userid']); return false; } /* User is logged in, set class variables */ $this->userinfo = $database->getUserInfo($_SESSION['username']); $this->username = $this->userinfo['username']; $this->userid = $this->userinfo['userid']; $this->userlevel = $this->userinfo['userlevel']; return true; } /* User not logged in */ else{ return false; } } /** * login - The user has submitted his username and password * through the login form, this function checks the authenticity * of that information in the database and creates the session. * Effectively logging in the user if all goes well. */ function login($subuser, $subpass, $subremember){ global $database, $form; //The database and form object /* Username error checking */ $field = "user"; //Use field name for username if(!$subuser || strlen($subuser = trim($subuser)) == 0){ $form->setError($field, "* Username not entered"); } else{ /* Check if username is not alphanumeric */ if(!eregi("^([0-9a-z])*$", $subuser)){ $form->setError($field, "* Username not alphanumeric"); } } /* Password error checking */ $field = "pass"; //Use field name for password if(!$subpass){ $form->setError($field, "* Password not entered"); } /* Return if form errors exist */ if($form->num_errors > 0){ return false; } /* Checks that username is in database and password is correct */ $subuser = stripslashes($subuser); $result = $database->confirmUserPass($subuser, md5($subpass)); /* Check error codes */ if($result == 1){ $field = "user"; $form->setError($field, "* Username not found"); } else if($result == 2){ $field = "pass"; $form->setError($field, "* Invalid password"); } /* Return if form errors exist */ if($form->num_errors > 0){ return false; } /* Username and password correct, register session variables */ $this->userinfo = $database->getUserInfo($subuser); $this->username = $_SESSION['username'] = $this->userinfo['username']; $this->userid = $_SESSION['userid'] = $this->generateRandID(); $this->userlevel = $this->userinfo['userlevel']; /* Insert userid into database and update active users table */ $database->updateUserField($this->username, "userid", $this->userid); $database->addActiveUser($this->username, $this->time); $database->removeActiveGuest($_SERVER['REMOTE_ADDR']); /** * This is the cool part: the user has requested that we remember that * he's logged in, so we set two cookies. One to hold his username, * and one to hold his random value userid. It expires by the time * specified in constants.php. Now, next time he comes to our site, we will * log him in automatically, but only if he didn't log out before he left. */ if($subremember){ setcookie("cookname", $this->username, time()+COOKIE_EXPIRE, COOKIE_PATH); setcookie("cookid", $this->userid, time()+COOKIE_EXPIRE, COOKIE_PATH); } /* Login completed successfully */ return true; } /** * logout - Gets called when the user wants to be logged out of the * website. It deletes any cookies that were stored on the users * computer as a result of him wanting to be remembered, and also * unsets session variables and demotes his user level to guest. */ function logout(){ global $database; //The database connection /** * Delete cookies - the time must be in the past, * so just negate what you added when creating the * cookie. */ if(isset($_COOKIE['cookname']) && isset($_COOKIE['cookid'])){ setcookie("cookname", "", time()-COOKIE_EXPIRE, COOKIE_PATH); setcookie("cookid", "", time()-COOKIE_EXPIRE, COOKIE_PATH); } /* Unset PHP session variables */ unset($_SESSION['username']); unset($_SESSION['userid']); /* Reflect fact that user has logged out */ $this->logged_in = false; /** * Remove from active users table and add to * active guests tables. */ $database->removeActiveUser($this->username); $database->addActiveGuest($_SERVER['REMOTE_ADDR'], $this->time); /* Set user level to guest */ $this->username = GUEST_NAME; $this->userlevel = GUEST_LEVEL; } /** * register - Gets called when the user has just submitted the * registration form. Determines if there were any errors with * the entry fields, if so, it records the errors and returns * 1. If no errors were found, it registers the new user and * returns 0. Returns 2 if registration failed. */ function register($subuser, $subpass, $subemail){ global $database, $form, $mailer; //The database, form and mailer object /* Username error checking */ $field = "user"; //Use field name for username if(!$subuser || strlen($subuser = trim($subuser)) == 0){ $form->setError($field, "* Username not entered"); } else{ /* Spruce up username, check length */ $subuser = stripslashes($subuser); if(strlen($subuser) < 5){ $form->setError($field, "* Username below 5 characters"); } else if(strlen($subuser) > 30){ $form->setError($field, "* Username above 30 characters"); } /* Check if username is not alphanumeric */ else if(!eregi("^([0-9a-z])+$", $subuser)){ $form->setError($field, "* Username not alphanumeric"); } /* Check if username is reserved */ else if(strcasecmp($subuser, GUEST_NAME) == 0){ $form->setError($field, "* Username reserved word"); } /* Check if username is already in use */ else if($database->usernameTaken($subuser)){ $form->setError($field, "* Username already in use"); } /* Check if username is banned */ else if($database->usernameBanned($subuser)){ $form->setError($field, "* Username banned"); } } /* Password error checking */ $field = "pass"; //Use field name for password if(!$subpass){ $form->setError($field, "* Password not entered"); } else{ /* Spruce up password and check length*/ $subpass = stripslashes($subpass); if(strlen($subpass) < 4){ $form->setError($field, "* Password too short"); } /* Check if password is not alphanumeric */ else if(!eregi("^([0-9a-z])+$", ($subpass = trim($subpass)))){ $form->setError($field, "* Password not alphanumeric"); } /** * Note: I trimmed the password only after I checked the length * because if you fill the password field up with spaces * it looks like a lot more characters than 4, so it looks * kind of stupid to report "password too short". */ } /* Email error checking */ $field = "email"; //Use field name for email if(!$subemail || strlen($subemail = trim($subemail)) == 0){ $form->setError($field, "* Email not entered"); } else{ /* Check if valid email address */ $regex = "^[_+a-z0-9-]+(\.[_+a-z0-9-]+)*" ."@[a-z0-9-]+(\.[a-z0-9-]{1,})*" ."\.([a-z]{2,}){1}$"; if(!eregi($regex,$subemail)){ $form->setError($field, "* Email invalid"); } $subemail = stripslashes($subemail); } /* Errors exist, have user correct them */ if($form->num_errors > 0){ return 1; //Errors with form } /* No errors, add the new account to the */ else{ if($database->addNewUser($subuser, md5($subpass), $subemail)){ if(EMAIL_WELCOME){ $mailer->sendWelcome($subuser,$subemail,$subpass); } return 0; //New user added succesfully }else{ return 2; //Registration attempt failed } } } /** * editAccount - Attempts to edit the user's account information * including the password, which it first makes sure is correct * if entered, if so and the new password is in the right * format, the change is made. All other fields are changed * automatically. */ function editAccount($subcurpass, $subnewpass, $subemail){ global $database, $form; //The database and form object /* New password entered */ if($subnewpass){ /* Current Password error checking */ $field = "curpass"; //Use field name for current password if(!$subcurpass){ $form->setError($field, "* Current Password not entered"); } else{ /* Check if password too short or is not alphanumeric */ $subcurpass = stripslashes($subcurpass); if(strlen($subcurpass) < 4 || !eregi("^([0-9a-z])+$", ($subcurpass = trim($subcurpass)))){ $form->setError($field, "* Current Password incorrect"); } /* Password entered is incorrect */ if($database->confirmUserPass($this->username,md5($subcurpass)) != 0){ $form->setError($field, "* Current Password incorrect"); } } /* New Password error checking */ $field = "newpass"; //Use field name for new password /* Spruce up password and check length*/ $subpass = stripslashes($subnewpass); if(strlen($subnewpass) < 4){ $form->setError($field, "* New Password too short"); } /* Check if password is not alphanumeric */ else if(!eregi("^([0-9a-z])+$", ($subnewpass = trim($subnewpass)))){ $form->setError($field, "* New Password not alphanumeric"); } } /* Change password attempted */ else if($subcurpass){ /* New Password error reporting */ $field = "newpass"; //Use field name for new password $form->setError($field, "* New Password not entered"); } /* Email error checking */ $field = "email"; //Use field name for email if($subemail && strlen($subemail = trim($subemail)) > 0){ /* Check if valid email address */ $regex = "^[_+a-z0-9-]+(\.[_+a-z0-9-]+)*" ."@[a-z0-9-]+(\.[a-z0-9-]{1,})*" ."\.([a-z]{2,}){1}$"; if(!eregi($regex,$subemail)){ $form->setError($field, "* Email invalid"); } $subemail = stripslashes($subemail); } /* Errors exist, have user correct them */ if($form->num_errors > 0){ return false; //Errors with form } /* Update password since there were no errors */ if($subcurpass && $subnewpass){ $database->updateUserField($this->username,"password",md5($subnewpass)); } /* Change Email */ if($subemail){ $database->updateUserField($this->username,"email",$subemail); } /* Success! */ return true; } /** * isAdmin - Returns true if currently logged in user is * an administrator, false otherwise. */ function isAdmin(){ return ($this->userlevel == ADMIN_LEVEL || $this->username == ADMIN_NAME); } /** * generateRandID - Generates a string made up of randomized * letters (lower and upper case) and digits and returns * the md5 hash of it to be used as a userid. */ function generateRandID(){ return md5($this->generateRandStr(16)); } /** * generateRandStr - Generates a string made up of randomized * letters (lower and upper case) and digits, the length * is a specified parameter. */ function generateRandStr($length){ $randstr = ""; for($i=0; $i<$length; $i++){ $randnum = mt_rand(0,61); if($randnum < 10){ $randstr .= chr($randnum+48); }else if($randnum < 36){ $randstr .= chr($randnum+55); }else{ $randstr .= chr($randnum+61); } } return $randstr; } }; /** * Initialize session object - This must be initialized before * the form object because the form uses session variables, * which cannot be accessed unless the session has started. */ $session = new Session; /* Initialize form object */ $form = new Form; ?>
-
Hey! I'm trying to use a local server to test my site out. I'm using dreamweaver for all the coding. I installed WAMP server and have the local host working. When I preview the site, it really is messed up. For example, if I have this code: <? /** * Main.php * * This is an example of the main page of a website. Here * users will be able to login. However, like on most sites * the login form doesn't just have to be on the main page, * but re-appear on subsequent pages, depending on whether * the user has logged in or not. * * Written by: Jpmaster77 a.k.a. The Grandmaster of C++ (GMC) * Last Updated: August 26, 2004 */ include("include/session.php"); ?> <table> <tr><td> <? /** * User has already logged in, so display relavent links, including * a link to the admin center if the user is an administrator. */ if($session->logged_in){ echo "<h1>Logged In</h1>"; echo "Welcome <b>$session->username</b>, you are logged in. <br><br>" ."[<a href=\"userinfo.php?user=$session->username\">My Account</a>] " ."[<a href=\"useredit.php\">Edit Account</a>] "; if($session->isAdmin()){ echo "[<a href=\"admin/admin.php\">Admin Center</a>] "; } echo "[<a href=\"process.php\">Logout</a>]"; } else{ ?> <h3>Login</h3> <? /** * User not logged in, display the login form. * If user has already tried to login, but errors were * found, display the total number of errors. * If errors occurred, they will be displayed. */ if($form->num_errors > 0){ echo "<font size=\"2\" color=\"#ff0000\">".$form->num_errors." error(s) found</font>"; } ?> <form action="process.php" method="POST"> <table align="left" border="0" cellspacing="0" cellpadding="3"> <tr><td>Username:</td><td><input type="text" name="user" maxlength="30" value="<? echo $form->value("user"); ?>"></td><td><? echo $form->error("user"); ?></td></tr> <tr><td>Password:</td><td><input type="password" name="pass" maxlength="30" value="<? echo $form->value("pass"); ?>"></td><td><? echo $form->error("pass"); ?></td></tr> <tr><td colspan="2" align="left"><input type="checkbox" name="remember" <? if($form->value("remember") != ""){ echo "checked"; } ?>> <font size="2">Remember me next time <input type="hidden" name="sublogin" value="1"> <input type="submit" value="Login"></td></tr> <tr><td colspan="2" align="left"><br><font size="2">[<a href="forgotpass.php">Forgot Password?</a>]</font></td><td align="right"></td></tr> <tr><td colspan="2" align="left"><br>Not registered? <a href="register.php">Sign-Up!</a></td></tr> </table> </form> <? } /** * Just a little page footer, tells how many registered members * there are, how many users currently logged in and viewing site, * and how many guests viewing site. Active users are displayed, * with link to their user information. */ echo "</td></tr><tr><td align=\"center\"><br><br>"; echo "<b>Member Total:</b> ".$database->getNumMembers()."<br>"; echo "There are $database->num_active_users registered members and "; echo "$database->num_active_guests guests viewing the site.<br><br>"; include("include/view_active.php"); ?> </td></tr> </table> I get this: logged_in){ echo " Logged In "; echo "Welcome $session->username, you are logged in. " ."[username\">My Account] " ."[Edit Account] "; if($session->isAdmin()){ echo "[Admin Center] "; } echo "[Logout]"; } else{ ?> Login num_errors > 0){ echo "".$form->num_errors." error(s) found"; } ?> Username: "> error("user"); ?> Password: "> error("pass"); ?> value("remember") != ""){ echo "checked"; } ?>> Remember me next time [Forgot Password?] Not registered? Sign-Up! "; echo "Member Total: ".$database->getNumMembers()." "; echo "There are $database->num_active_users registered members and "; echo "$database->num_active_guests guests viewing the site. "; include("include/view_active.php"); ?> To me, it seems like a " problem but I don't see why it would be happening.
-
Still having issues. I viewed the page source and discovered something that wasn't displaying on the webpage. It says: PHP Error Message Warning: mysql_fetch_assoc(): supplied argument is not a valid MySQL result resource in /home/a2986016/public_html/modform.php on line 22 What does it mean?
-
I'm wondering what makes a pop-up login window run differently than inside a webpage. I can't seem to get my login system to bring the user back to the page (brings them to a page can't be found) when clicking a form submit button when it is included in the webpage. But if your in the popup page that I'm trying to include, it works no problem so I was wondering if it changes anything. Or if there is some sort of work around (such as embedding a popup window into the webpage). Thanks a lot!
-
Here is process.php. It process's the login form. <? /** * Process.php * * The Process class is meant to simplify the task of processing * user submitted forms, redirecting the user to the correct * pages if errors are found, or if form is successful, either * way. Also handles the logout procedure. * * Written by: Jpmaster77 a.k.a. The Grandmaster of C++ (GMC) * Last Updated: August 19, 2004 */ include("session.php"); class Process { /* Class constructor */ function Process(){ global $session; /* User submitted login form */ if(isset($_POST['sublogin'])){ $this->procLogin(); } /* User submitted registration form */ else if(isset($_POST['subjoin'])){ $this->procRegister(); } /* User submitted forgot password form */ else if(isset($_POST['subforgot'])){ $this->procForgotPass(); } /* User submitted edit account form */ else if(isset($_POST['subedit'])){ $this->procEditAccount(); } /** * The only other reason user should be directed here * is if he wants to logout, which means user is * logged in currently. */ else if($session->logged_in){ $this->procLogout(); } /** * Should not get here, which means user is viewing this page * by mistake and therefore is redirected. */ else{ header("Location: index.php"); } } /** * procLogin - Processes the user submitted login form, if errors * are found, the user is redirected to correct the information, * if not, the user is effectively logged in to the system. */ function procLogin(){ global $session, $form; /* Login attempt */ $retval = $session->login($_POST['user'], $_POST['pass'], isset($_POST['remember'])); /* Login successful */ if($retval){ header("Location: ".$session->referrer); } /* Login failed */ else{ $_SESSION['value_array'] = $_POST; $_SESSION['error_array'] = $form->getErrorArray(); header("Location: ".$session->referrer); } } /** * procLogout - Simply attempts to log the user out of the system * given that there is no logout form to process. */ function procLogout(){ global $session; $retval = $session->logout(); header("Location: logout.php"); } /** * procRegister - Processes the user submitted registration form, * if errors are found, the user is redirected to correct the * information, if not, the user is effectively registered with * the system and an email is (optionally) sent to the newly * created user. */ function procRegister(){ global $session, $form; /* Convert username to all lowercase (by option) */ if(ALL_LOWERCASE){ $_POST['user'] = strtolower($_POST['user']); } /* Registration attempt */ $retval = $session->register($_POST['user'], $_POST['pass'], $_POST['email']); /* Registration Successful */ if($retval == 0){ $_SESSION['reguname'] = $_POST['user']; $_SESSION['regsuccess'] = true; header("Location: ".$session->referrer); } /* Error found with form */ else if($retval == 1){ $_SESSION['value_array'] = $_POST; $_SESSION['error_array'] = $form->getErrorArray(); header("Location: ".$session->referrer); } /* Registration attempt failed */ else if($retval == 2){ $_SESSION['reguname'] = $_POST['user']; $_SESSION['regsuccess'] = false; header("Location: ".$session->referrer); } } /** * procForgotPass - Validates the given username then if * everything is fine, a new password is generated and * emailed to the address the user gave on sign up. */ function procForgotPass(){ global $database, $session, $mailer, $form; /* Username error checking */ $subuser = $_POST['user']; $field = "user"; //Use field name for username if(!$subuser || strlen($subuser = trim($subuser)) == 0){ $form->setError($field, "* Username not entered<br>"); } else{ /* Make sure username is in database */ $subuser = stripslashes($subuser); if(strlen($subuser) < 5 || strlen($subuser) > 30 || !eregi("^([0-9a-z])+$", $subuser) || (!$database->usernameTaken($subuser))){ $form->setError($field, "* Username does not exist<br>"); } } /* Errors exist, have user correct them */ if($form->num_errors > 0){ $_SESSION['value_array'] = $_POST; $_SESSION['error_array'] = $form->getErrorArray(); } /* Generate new password and email it to user */ else{ /* Generate new password */ $newpass = $session->generateRandStr(; /* Get email of user */ $usrinf = $database->getUserInfo($subuser); $email = $usrinf['email']; /* Attempt to send the email with new password */ if($mailer->sendNewPass($subuser,$email,$newpass)){ /* Email sent, update database */ $database->updateUserField($subuser, "password", md5($newpass)); $_SESSION['forgotpass'] = true; } /* Email failure, do not change password */ else{ $_SESSION['forgotpass'] = false; } } header("Location: ".$session->referrer); } /** * procEditAccount - Attempts to edit the user's account * information, including the password, which must be verified * before a change is made. */ function procEditAccount(){ global $session, $form; /* Account edit attempt */ $retval = $session->editAccount($_POST['curpass'], $_POST['newpass'], $_POST['email']); /* Account edit successful */ if($retval){ $_SESSION['useredit'] = true; header("Location: ".$session->referrer); } /* Error found with form */ else{ $_SESSION['value_array'] = $_POST; $_SESSION['error_array'] = $form->getErrorArray(); header("Location: ".$session->referrer); } } }; /* Initialize process */ $process = new Process; ?> Here's session.php. <? /** * Session.php * * The Session class is meant to simplify the task of keeping * track of logged in users and also guests. * * Written by: Jpmaster77 a.k.a. The Grandmaster of C++ (GMC) * Last Updated: August 19, 2004 */ include("database.php"); include("mailer.php"); include("form.php"); class Session { var $username; //Username given on sign-up var $userid; //Random value generated on current login var $userlevel; //The level to which the user pertains var $time; //Time user was last active (page loaded) var $logged_in; //True if user is logged in, false otherwise var $userinfo = array(); //The array holding all user info var $url; //The page url current being viewed var $referrer; //Last recorded site page viewed /** * Note: referrer should really only be considered the actual * page referrer in process.php, any other time it may be * inaccurate. */ /* Class constructor */ function Session(){ $this->time = time(); $this->startSession(); } /** * startSession - Performs all the actions necessary to * initialize this session object. Tries to determine if the * the user has logged in already, and sets the variables * accordingly. Also takes advantage of this page load to * update the active visitors tables. */ function startSession(){ global $database; //The database connection session_start(); //Tell PHP to start the session /* Determine if user is logged in */ $this->logged_in = $this->checkLogin(); /** * Set guest value to users not logged in, and update * active guests table accordingly. */ if(!$this->logged_in){ $this->username = $_SESSION['username'] = GUEST_NAME; $this->userlevel = GUEST_LEVEL; $database->addActiveGuest($_SERVER['REMOTE_ADDR'], $this->time); } /* Update users last active timestamp */ else{ $database->addActiveUser($this->username, $this->time); } /* Remove inactive visitors from database */ $database->removeInactiveUsers(); $database->removeInactiveGuests(); /* Set referrer page */ if(isset($_SESSION['url'])){ $this->referrer = $_SESSION['url']; }else{ $this->referrer = "/"; } /* Set current url */ $this->url = $_SESSION['url'] = $_SERVER['PHP_SELF']; } /** * checkLogin - Checks if the user has already previously * logged in, and a session with the user has already been * established. Also checks to see if user has been remembered. * If so, the database is queried to make sure of the user's * authenticity. Returns true if the user has logged in. */ function checkLogin(){ global $database; //The database connection /* Check if user has been remembered */ if(isset($_COOKIE['cookname']) && isset($_COOKIE['cookid'])){ $this->username = $_SESSION['username'] = $_COOKIE['cookname']; $this->userid = $_SESSION['userid'] = $_COOKIE['cookid']; } /* Username and userid have been set and not guest */ if(isset($_SESSION['username']) && isset($_SESSION['userid']) && $_SESSION['username'] != GUEST_NAME){ /* Confirm that username and userid are valid */ if($database->confirmUserID($_SESSION['username'], $_SESSION['userid']) != 0){ /* Variables are incorrect, user not logged in */ unset($_SESSION['username']); unset($_SESSION['userid']); return false; } /* User is logged in, set class variables */ $this->userinfo = $database->getUserInfo($_SESSION['username']); $this->username = $this->userinfo['username']; $this->userid = $this->userinfo['userid']; $this->userlevel = $this->userinfo['userlevel']; return true; } /* User not logged in */ else{ return false; } } /** * login - The user has submitted his username and password * through the login form, this function checks the authenticity * of that information in the database and creates the session. * Effectively logging in the user if all goes well. */ function login($subuser, $subpass, $subremember){ global $database, $form; //The database and form object /* Username error checking */ $field = "user"; //Use field name for username if(!$subuser || strlen($subuser = trim($subuser)) == 0){ $form->setError($field, "* Username not entered"); } else{ /* Check if username is not alphanumeric */ if(!eregi("^([0-9a-z])*$", $subuser)){ $form->setError($field, "* Username not alphanumeric"); } } /* Password error checking */ $field = "pass"; //Use field name for password if(!$subpass){ $form->setError($field, "* Password not entered"); } /* Return if form errors exist */ if($form->num_errors > 0){ return false; } /* Checks that username is in database and password is correct */ $subuser = stripslashes($subuser); $result = $database->confirmUserPass($subuser, md5($subpass)); /* Check error codes */ if($result == 1){ $field = "user"; $form->setError($field, "* Username not found"); } else if($result == 2){ $field = "pass"; $form->setError($field, "* Invalid password"); } /* Return if form errors exist */ if($form->num_errors > 0){ return false; } /* Username and password correct, register session variables */ $this->userinfo = $database->getUserInfo($subuser); $this->username = $_SESSION['username'] = $this->userinfo['username']; $this->userid = $_SESSION['userid'] = $this->generateRandID(); $this->userlevel = $this->userinfo['userlevel']; /* Insert userid into database and update active users table */ $database->updateUserField($this->username, "userid", $this->userid); $database->addActiveUser($this->username, $this->time); $database->removeActiveGuest($_SERVER['REMOTE_ADDR']); /** * This is the cool part: the user has requested that we remember that * he's logged in, so we set two cookies. One to hold his username, * and one to hold his random value userid. It expires by the time * specified in constants.php. Now, next time he comes to our site, we will * log him in automatically, but only if he didn't log out before he left. */ if($subremember){ setcookie("cookname", $this->username, time()+COOKIE_EXPIRE, COOKIE_PATH); setcookie("cookid", $this->userid, time()+COOKIE_EXPIRE, COOKIE_PATH); } /* Login completed successfully */ return true; } /** * logout - Gets called when the user wants to be logged out of the * website. It deletes any cookies that were stored on the users * computer as a result of him wanting to be remembered, and also * unsets session variables and demotes his user level to guest. */ function logout(){ global $database; //The database connection /** * Delete cookies - the time must be in the past, * so just negate what you added when creating the * cookie. */ if(isset($_COOKIE['cookname']) && isset($_COOKIE['cookid'])){ setcookie("cookname", "", time()-COOKIE_EXPIRE, COOKIE_PATH); setcookie("cookid", "", time()-COOKIE_EXPIRE, COOKIE_PATH); } /* Unset PHP session variables */ unset($_SESSION['username']); unset($_SESSION['userid']); /* Reflect fact that user has logged out */ $this->logged_in = false; /** * Remove from active users table and add to * active guests tables. */ $database->removeActiveUser($this->username); $database->addActiveGuest($_SERVER['REMOTE_ADDR'], $this->time); /* Set user level to guest */ $this->username = GUEST_NAME; $this->userlevel = GUEST_LEVEL; } /** * register - Gets called when the user has just submitted the * registration form. Determines if there were any errors with * the entry fields, if so, it records the errors and returns * 1. If no errors were found, it registers the new user and * returns 0. Returns 2 if registration failed. */ function register($subuser, $subpass, $subemail){ global $database, $form, $mailer; //The database, form and mailer object /* Username error checking */ $field = "user"; //Use field name for username if(!$subuser || strlen($subuser = trim($subuser)) == 0){ $form->setError($field, "* Username not entered"); } else{ /* Spruce up username, check length */ $subuser = stripslashes($subuser); if(strlen($subuser) < 5){ $form->setError($field, "* Username below 5 characters"); } else if(strlen($subuser) > 30){ $form->setError($field, "* Username above 30 characters"); } /* Check if username is not alphanumeric */ else if(!eregi("^([0-9a-z])+$", $subuser)){ $form->setError($field, "* Username not alphanumeric"); } /* Check if username is reserved */ else if(strcasecmp($subuser, GUEST_NAME) == 0){ $form->setError($field, "* Username reserved word"); } /* Check if username is already in use */ else if($database->usernameTaken($subuser)){ $form->setError($field, "* Username already in use"); } /* Check if username is banned */ else if($database->usernameBanned($subuser)){ $form->setError($field, "* Username banned"); } } /* Password error checking */ $field = "pass"; //Use field name for password if(!$subpass){ $form->setError($field, "* Password not entered"); } else{ /* Spruce up password and check length*/ $subpass = stripslashes($subpass); if(strlen($subpass) < 4){ $form->setError($field, "* Password too short"); } /* Check if password is not alphanumeric */ else if(!eregi("^([0-9a-z])+$", ($subpass = trim($subpass)))){ $form->setError($field, "* Password not alphanumeric"); } /** * Note: I trimmed the password only after I checked the length * because if you fill the password field up with spaces * it looks like a lot more characters than 4, so it looks * kind of stupid to report "password too short". */ } /* Email error checking */ $field = "email"; //Use field name for email if(!$subemail || strlen($subemail = trim($subemail)) == 0){ $form->setError($field, "* Email not entered"); } else{ /* Check if valid email address */ $regex = "^[_+a-z0-9-]+(\.[_+a-z0-9-]+)*" ."@[a-z0-9-]+(\.[a-z0-9-]{1,})*" ."\.([a-z]{2,}){1}$"; if(!eregi($regex,$subemail)){ $form->setError($field, "* Email invalid"); } $subemail = stripslashes($subemail); } /* Errors exist, have user correct them */ if($form->num_errors > 0){ return 1; //Errors with form } /* No errors, add the new account to the */ else{ if($database->addNewUser($subuser, md5($subpass), $subemail)){ if(EMAIL_WELCOME){ $mailer->sendWelcome($subuser,$subemail,$subpass); } return 0; //New user added succesfully }else{ return 2; //Registration attempt failed } } } /** * editAccount - Attempts to edit the user's account information * including the password, which it first makes sure is correct * if entered, if so and the new password is in the right * format, the change is made. All other fields are changed * automatically. */ function editAccount($subcurpass, $subnewpass, $subemail){ global $database, $form; //The database and form object /* New password entered */ if($subnewpass){ /* Current Password error checking */ $field = "curpass"; //Use field name for current password if(!$subcurpass){ $form->setError($field, "* Current Password not entered"); } else{ /* Check if password too short or is not alphanumeric */ $subcurpass = stripslashes($subcurpass); if(strlen($subcurpass) < 4 || !eregi("^([0-9a-z])+$", ($subcurpass = trim($subcurpass)))){ $form->setError($field, "* Current Password incorrect"); } /* Password entered is incorrect */ if($database->confirmUserPass($this->username,md5($subcurpass)) != 0){ $form->setError($field, "* Current Password incorrect"); } } /* New Password error checking */ $field = "newpass"; //Use field name for new password /* Spruce up password and check length*/ $subpass = stripslashes($subnewpass); if(strlen($subnewpass) < 4){ $form->setError($field, "* New Password too short"); } /* Check if password is not alphanumeric */ else if(!eregi("^([0-9a-z])+$", ($subnewpass = trim($subnewpass)))){ $form->setError($field, "* New Password not alphanumeric"); } } /* Change password attempted */ else if($subcurpass){ /* New Password error reporting */ $field = "newpass"; //Use field name for new password $form->setError($field, "* New Password not entered"); } /* Email error checking */ $field = "email"; //Use field name for email if($subemail && strlen($subemail = trim($subemail)) > 0){ /* Check if valid email address */ $regex = "^[_+a-z0-9-]+(\.[_+a-z0-9-]+)*" ."@[a-z0-9-]+(\.[a-z0-9-]{1,})*" ."\.([a-z]{2,}){1}$"; if(!eregi($regex,$subemail)){ $form->setError($field, "* Email invalid"); } $subemail = stripslashes($subemail); } /* Errors exist, have user correct them */ if($form->num_errors > 0){ return false; //Errors with form } /* Update password since there were no errors */ if($subcurpass && $subnewpass){ $database->updateUserField($this->username,"password",md5($subnewpass)); } /* Change Email */ if($subemail){ $database->updateUserField($this->username,"email",$subemail); } /* Success! */ return true; } /** * isAdmin - Returns true if currently logged in user is * an administrator, false otherwise. */ function isAdmin(){ return ($this->userlevel == ADMIN_LEVEL || $this->username == ADMIN_NAME); } /** * generateRandID - Generates a string made up of randomized * letters (lower and upper case) and digits and returns * the md5 hash of it to be used as a userid. */ function generateRandID(){ return md5($this->generateRandStr(16)); } /** * generateRandStr - Generates a string made up of randomized * letters (lower and upper case) and digits, the length * is a specified parameter. */ function generateRandStr($length){ $randstr = ""; for($i=0; $i<$length; $i++){ $randnum = mt_rand(0,61); if($randnum < 10){ $randstr .= chr($randnum+48); }else if($randnum < 36){ $randstr .= chr($randnum+55); }else{ $randstr .= chr($randnum+61); } } return $randstr; } }; /** * Initialize session object - This must be initialized before * the form object because the form uses session variables, * which cannot be accessed unless the session has started. */ $session = new Session; /* Initialize form object */ $form = new Form; ?> If you want to see all the code, I got it from here: http://evolt.org/PHP-Login-System-with-Admin-Features?from=2450&comments_per_page=50 Thanks