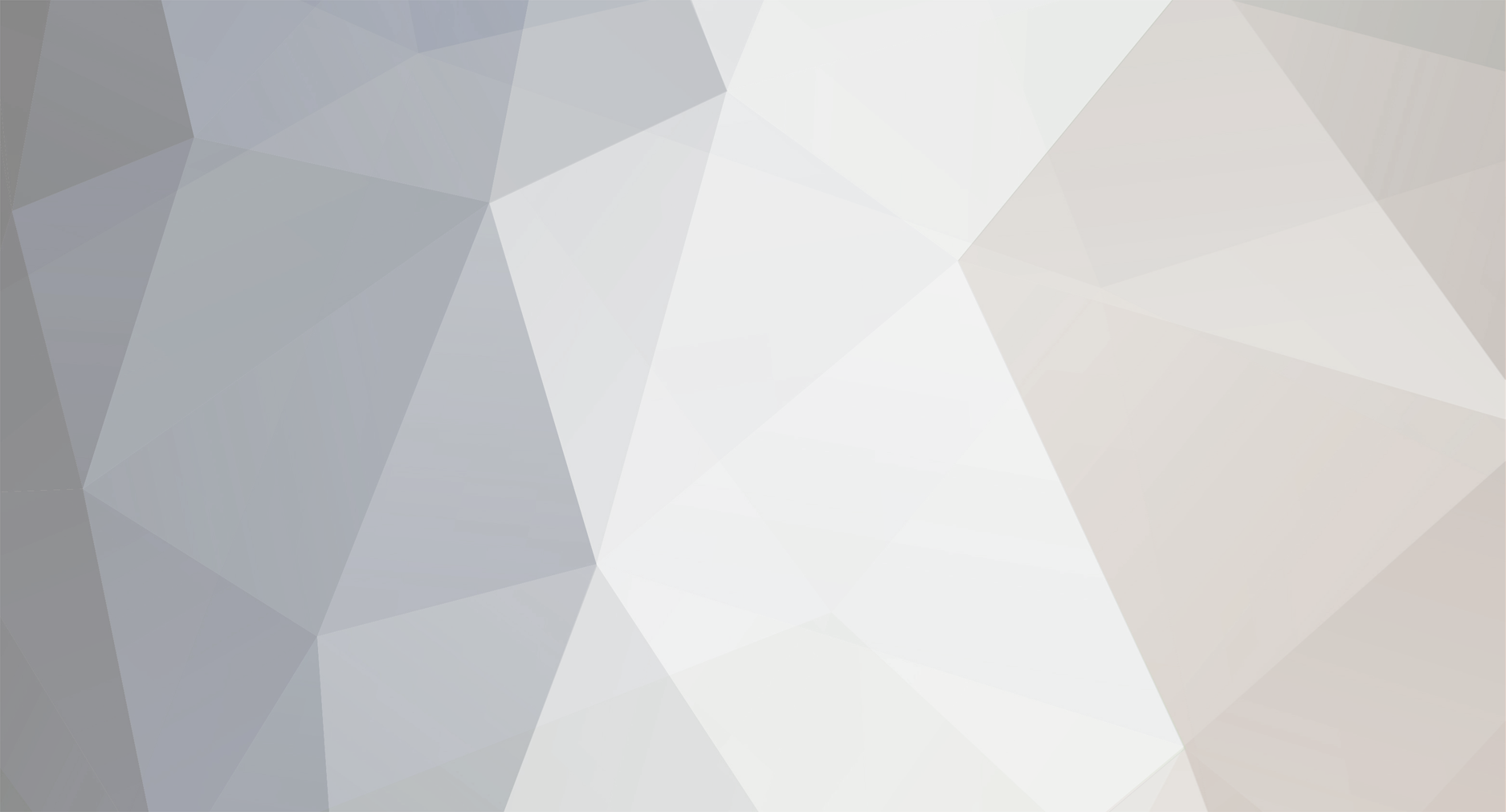
Ryflex
-
Posts
115 -
Joined
-
Last visited
Posts posted by Ryflex
-
-
Hi all,
I have a live search which gives as return value the name your looking for minus the letters you already typed in.
Is it possible to get the return value in the textfield behind what you already typed.
Underneath is my current code.
<html> <head> <script type="text/javascript"> function showHint(str) { var xmlhttp; if (str.length==0) { document.getElementById("txtHint").innerHTML=""; return; } if (window.XMLHttpRequest) {// code for IE7+, Firefox, Chrome, Opera, Safari xmlhttp=new XMLHttpRequest(); } else {// code for IE6, IE5 xmlhttp=new ActiveXObject("Microsoft.XMLHTTP"); } xmlhttp.onreadystatechange=function() { if (xmlhttp.readyState==4 && xmlhttp.status==200) { document.getElementById("txtHint").innerHTML=xmlhttp.responseText; } } xmlhttp.open("GET","ajax_test.php?q="+str,true); xmlhttp.send(); } </script> </head> <body> <h3>Start typing a name in the input field below:</h3> <form action=""> First name: <input type="text" id="txt1" onkeyup="showHint(this.value)"/> </form> <p>Suggestions: <span id="txtHint"></span></p> </body> </html>
-
Thanks for the help everyone,
The last comment of Zanus did the trick *mumbles about not using help in the full extend*
mikosiko I did change to voornaam but after I added the reply but the change didn't change it because my query was wrong.
THANKS ALL
-
Like Homer: DOH
I forgot to mention it didn't work.
It keeps telling no suggestion also when filled in the complete name.
-
Wow!!!
Thanks for al the quick replies!!!
Here is my AJAX code:
<html> <head> <script type="text/javascript"> function showHint(str) { var xmlhttp; if (str.length==0) { document.getElementById("txtHint").innerHTML=""; return; } if (window.XMLHttpRequest) {// code for IE7+, Firefox, Chrome, Opera, Safari xmlhttp=new XMLHttpRequest(); } else {// code for IE6, IE5 xmlhttp=new ActiveXObject("Microsoft.XMLHTTP"); } xmlhttp.onreadystatechange=function() { if (xmlhttp.readyState==4 && xmlhttp.status==200) { document.getElementById("txtHint").innerHTML=xmlhttp.responseText; } } xmlhttp.open("GET","ajax_test.php?q="+str,true); xmlhttp.send(); } </script> </head> <body> <h3>Start typing a name in the input field below:</h3> <form action=""> First name: <input type="text" id="txt1" onkeyup="showHint(this.value)" /> </form> <p>Suggestions: <span id="txtHint"></span></p> </body> </html>
Btw to make it easier to understand for you all Voornaam means firstname in dutch.
I'm going to use this query so people can fill in the first part of a name of a user and it gives some options.
EDIT:
I used Zanus part with the wildcard to do the following in combination with mikosiko's:
$query = "SELECT * FROM users WHERE voornaam ='$q%'"; $result = mysql_query($query) or die('Query1 failed: ' . mysql_error()); $totalRows = mysql_num_rows($result); for ($j=0; $j<$totalRows; $j++) { $a[$j] = mysql_fetch_object($result)->predicted; // assuming that "predicted" is a field name in your table users.. otherwise replace it for a valid fieldname.. I would say that you should want to replace it for "voornaam". }
-
Hi all,
I have a PHP script ment for an AJAX livesearch.
It is supposed to check a hint (a few letters from a textfield) against 1 column in the database.
Somehow whatever I try it just won't give a suggestion from the database.
I used mysql_fetch_row which kept saying no suggestion but when I entered the exact name as in the database it gave the following error:
Warning: mysql_fetch_row() expects parameter 1 to be resource, string given in /customers/ryflex.nl/ryflex.nl/httpd.www/ajax_test.php on line 31 no suggestion
After asking on the AJAX part of the forum someone told me to use:
$a[] = mysql_fetch_object($result)->predicted;
So I made the following of it:
$query = "SELECT * FROM users WHERE voornaam ='".$q."'"; $result = mysql_query($query) or die('Query1 failed: ' . mysql_error()); $totalRows = mysql_num_rows($result); $a[] = mysql_fetch_object($result)->predicted;
Since that didn't do anything I set the code back to:
<?php // Fill up array with names //Include database connection details require_once('config.php'); //get the q parameter from URL $q=$_GET["q"]; //Connectie naar mysql server $link = mysql_connect(DB_HOST, DB_USER, DB_PASSWORD); if(!$link) { die('Failed to connect to server: ' . mysql_error()); } //Selecteer database $db = mysql_select_db(DB_DATABASE); if(!$db) { die("Unable to select database"); } $query = "SELECT * FROM users WHERE voornaam ='".$q."'"; $result = mysql_query($query) or die('Query1 failed: ' . mysql_error()); $totalRows = mysql_num_rows($result); $a = array(); for ($j=0; $j<$totalRows; $j++) { $a[$j] = mysql_fetch_row($result); } //lookup all hints from array if length of q>0 if (strlen($q) > 0) { $hint=""; for($i=0; $i<count($a); $i++) { if (strtolower($q)==strtolower(substr($a[$i],0,strlen($q)))) { if ($hint=="") { $hint=$a[$i]; } else { $hint=$hint." , ".$a[$i]; } } } } // Set output to "no suggestion" if no hint were found // or to the correct values if ($hint == "") { $response="no suggestion"; } else { $response=$hint; } //output the response echo $response; ?>
The AJAX part of the code is:
<?php // Fill up array with names //Include database connection details require_once('config.php'); //get the q parameter from URL $q=$_GET["q"]; //Connectie naar mysql server $link = mysql_connect(DB_HOST, DB_USER, DB_PASSWORD); if(!$link) { die('Failed to connect to server: ' . mysql_error()); } //Selecteer database $db = mysql_select_db(DB_DATABASE); if(!$db) { die("Unable to select database"); } $query = "SELECT * FROM users WHERE voornaam ='".$q."'"; $result = mysql_query($query) or die('Query1 failed: ' . mysql_error()); $totalRows = mysql_num_rows($result); $a = array(); for ($j=0; $j<$totalRows; $j++) { $a[$j] = mysql_fetch_row($result); } //lookup all hints from array if length of q>0 if (strlen($q) > 0) { $hint=""; for($i=0; $i<count($a); $i++) { if (strtolower($q)==strtolower(substr($a[$i],0,strlen($q)))) { if ($hint=="") { $hint=$a[$i]; } else { $hint=$hint." , ".$a[$i]; } } } } // Set output to "no suggestion" if no hint were found // or to the correct values if ($hint == "") { $response="no suggestion"; } else { $response=$hint; } //output the response echo $response; ?>
I hope someone will be able to help me out here since I'm getting nuts from this problem.
Greetz Ryflex
-
ok after modyfing the php file to what you told me th query i run is now th following and I still don't get a result.
the error when I fill out an entire name is gone as well.
$query = "SELECT * FROM users WHERE voornaam ='".$q."'"; $result = mysql_query($query) or die('Query1 failed: ' . mysql_error()); $totalRows = mysql_num_rows($result); $a[] = mysql_fetch_object($result)->predicted;
-
Maybe it helps to know that when I type in one of the names in de database which should be "predicted" it gives the following error:
Warning: mysql_fetch_row() expects parameter 1 to be resource, string given in /customers/ryflex.nl/ryflex.nl/httpd.www/ajax_test.php on line 31 no suggestion
When I put in 1 letter more or less it jumps back to no suggestion.
-
Can anyone please help me out here
-
Hi,
This is the site which i'm using the base code from and modifying it to my use.
http://w3schools.com/ajax/ajax_aspphp.asp
There they manually fill the array and do the same.
So I still can't make it work.
-
We'll it kind of should be an array so it can itterate through the array to give the suggestions.
-
Hi,
Sorry actually forgot ta ask the question.
When I type something in the textfield it should give me some suggestions from my db.
Somehow it keeps telling me there are no suggestions.
I think I'm doing something wrong in the call to the db but I tried different approaches and it still won't work.
Anyone have a clue?
-
Hi all,
I'm experimenting with Ajax and I'm kinda stuck. Underneath is my code please help me out.
<html> <head> <script type="text/javascript"> function showHint(str) { var xmlhttp; if (str.length==0) { document.getElementById("txtHint").innerHTML=""; return; } if (window.XMLHttpRequest) {// code for IE7+, Firefox, Chrome, Opera, Safari xmlhttp=new XMLHttpRequest(); } else {// code for IE6, IE5 xmlhttp=new ActiveXObject("Microsoft.XMLHTTP"); } xmlhttp.onreadystatechange=function() { if (xmlhttp.readyState==4 && xmlhttp.status==200) { document.getElementById("txtHint").innerHTML=xmlhttp.responseText; } } xmlhttp.open("GET","ajax_test.php?q="+str,true); xmlhttp.send(); } </script> </head> <body> <h3>Start typing a name in the input field below:</h3> <form action=""> First name: <input type="text" id="txt1" onkeyup="showHint(this.value)" /> </form> <p>Suggestions: <span id="txtHint"></span></p> </body> </html>
<?php // Fill up array with names //Include database connection details require_once('config.php'); //get the q parameter from URL $q=$_GET["q"]; //Connectie naar mysql server $link = mysql_connect(DB_HOST, DB_USER, DB_PASSWORD); if(!$link) { die('Failed to connect to server: ' . mysql_error()); } //Selecteer database $db = mysql_select_db(DB_DATABASE); if(!$db) { die("Unable to select database"); } $query = "SELECT * FROM users WHERE voornaam ='".$q."'"; $result = mysql_query($query) or die('Query1 failed: ' . mysql_error()); $totalRows = mysql_num_rows($result); $a = array(); for ($j=0; $j<$totalRows; $j++) { $a[$j] = mysql_fetch_row($result); } //lookup all hints from array if length of q>0 if (strlen($q) > 0) { $hint=""; for($i=0; $i<count($a); $i++) { if (strtolower($q)==strtolower(substr($a[$i],0,strlen($q)))) { if ($hint=="") { $hint=$a[$i]; } else { $hint=$hint." , ".$a[$i]; } } } } // Set output to "no suggestion" if no hint were found // or to the correct values if ($hint == "") { $response="no suggestion"; } else { $response=$hint; } //output the response echo $response; ?>
-
Hi vengod,
You should redirect the submit to a page where the credentials are checked and then let it redirect to th hidden page if the credentials are ok.
Ryflex
-
Thanks!!!!
-
Hi MrAdam,
I wil make another script which will run everyday and delete blank records.
My big question with the solution I have won't 2 users end up with the same number because the clicked create at the same time.
-
Hi MrAdam,
Thanks for the quick reaction!
Well in my idea when you open the new incident page you create the new row and query it. That is because when I open the page I want the Incident number to automaticaly show.
Ryflex
-
Hi all,
I'm starting an new project where I'm making an Incident Management System and right from the start I don't know what to do.
Could anyone get me on my way. I need to make a script where if I open a new incident the new page opens and automatically gets a new Incident number. This number is offcourse 1 number higher every time you make a incident.
I thought about make a column with autoincremetn and then query the highest number. Problem with that is when you open a page at multiple computers at the same time they get the same number.
Does anyone have an idea?
Ryflex
-
Hi all,
Already found the problem, the value statement of the first line was value- instead of value=
Thnx Ryflex
-
Hi all,
I changed the form with radio buttons but when I get the $_POST[train] it only says: on.
<form id="create" name="create" method="POST" action="infantry_exec.php"> <table> <tr> <td><b><?php echo $n[0]; ?></b></td> <td><b><input name="train" type="radio" value-"<?php echo $n[0]; ?>" /></b></td> <td rowspan="4"> How many troops do you want to train?<BR><input name="amount" maxlength="10" <?php echo $ro; ?> id="amount"></td> </tr> <tr> <td><b><?php echo $n[1]; ?></b></td> <td><b><input name="train" type="radio" value="<?php echo $n[1]; ?>" /></b></td> </tr> <tr> <td><b><?php echo $n[2]; ?></b></td> <td><b><input name="train" type="radio" value="<?php echo $n[2]; ?>" /></b></td> </tr> <tr> <td><b><?php echo $n[3]; ?></b></td> <td><b><input name="train" type="radio" value="<?php echo $n[3]; ?>" /></b></td> </tr> <tr> <td colspan="2"><center><input type="submit" name="Submit" value="Train!!!" /></center></td> </tr> </table> </form>
//convert POST to normal variable $amount = $_POST[amount]; $type = $_POST[train]; echo "$amount <BR> $type";
Thnx Ryflex
-
Hi sloth456,
This is what the Form page shows
You can only train 1 type of infantry at a time.
gunner [textbox]
marine [textbox]
sniper [textbox]
special ops [textbox]
Train!!!
The EXEC page only puts the amounts out of the textbox into the database.
In the FORM page I need something that functions like a radio button idea so only one thing can be "trained" at a time.
Thnx Ryflex
-
Hi hodgey87,
You're on the right way but when using a FORM with the METHOD POST you do need to give the $detail a value.
You could do that by using
$detail = $_POST['detail'];
And then you simply insert $detail in the database
mysql_query("UPDATE aboutenglish SET body = '$detail' where ID = '1'");
since you're not using the mysql_affected_rows you don't have to use $result you can simpy let the query run.
Ryflex
-
Hi all,
I have a page with 4 textboxes which can be filled out. The thing is I want people to be able to only train 1 type at a time.
Underneath I posted the Form page and the exec page does anyone have and idea??
Thnx Ryflex
FORM:
<?php //authentication & database configuration require_once('auth.php'); require_once('config.php'); //member_ID and city_ID and greyed out empty declaration $member_ID = $_SESSION['SESS_MEMBER_ID']; $city_ID = $_SESSION['SESS_CITY_ID']; $ro = ""; //Database connection $global_dbh = mysql_connect(DB_HOST, DB_USER, DB_PASSWORD) or die("Could not connect to database"); mysql_select_db(DB_DATABASE, $global_dbh) or die("Could not select database"); //get infantry units type = 1 $sql = "SELECT name, resource_1, resource_2, resource_3, timestamp FROM unittype WHERE type = 1"; $result = mysql_query($sql) or die("Could not find units"); //get the 4 unit types in the $n[] array while($data = mysql_fetch_assoc($result)) { $one[] = $data[resource_1]; $two[] = $data[resource_2]; $three[] = $data[resource_3]; $time[] = $data[timestamp]; } //query amount of infantry troops $sql = "SELECT * FROM infantry WHERE member_id = '$member_ID'"; $result = mysql_query($sql) or die("Could not find amount of troops"); //get amount of infantry troops while($data = mysql_fetch_assoc($result)) { $n[] = $data[name]; $training[] = $data[training]; $timestamp[] = $data[timestamp]; $amount[] = $data[amount]; } //check if a training is underway $sql = "SELECT * FROM infantry WHERE member_id = '$member_ID' AND training = 1"; $trcurr = mysql_fetch_array( mysql_query($sql)); if($trcurr['training'] == 1) { $ro = "READONLY STYLE='color:#999999;background:#DEDEDE'"; } ?> <html> <head> <title>Infantry Training</title> <link href="loginmodule.css" rel="stylesheet" type="text/css" /> </head> <body> <p>You can only train 1 type of infantry at a time.</p> <form id="create" name="create" method="POST" action="infantry_exec.php"> <table> <tr> <td><b><?php echo $n[0]; ?></b></td> <td><b><input name="<?php echo $n[0]; ?>" type="text" <?php echo $ro; ?> maxlength="10" id="<?php echo $n[0]; ?>" /></b></td> </tr> <tr> <td><b><?php echo $n[1]; ?></b></td> <td><b><input name="<?php echo $n[1]; ?>" type="text" <?php echo $ro; ?> maxlength="10" id="<?php echo $n[1]; ?>" /></b></td> </tr> <tr> <td><b><?php echo $n[2]; ?></b></td> <td><b><input name="<?php echo $n[2]; ?>" type="text" <?php echo $ro; ?> maxlength="10" id="<?php echo $n[2]; ?>" /></b></td> </tr> <tr> <td><b><?php echo $n[3]; ?></b></td> <td><b><input name="<?php echo $n[3]; ?>" type="text" <?php echo $ro; ?> maxlength="10" id="<?php echo $n[3]; ?>" /></b></td> </tr> <tr> <td colspan="2"><center><input type="submit" name="Submit" value="Train!!!" /></center></td> </tr> </table> </form> </body> </html>
EXEC:
<?php //authentication & database configuration require_once('auth.php'); require_once('config.php'); //member_ID and city_ID $member_ID = $_SESSION['SESS_MEMBER_ID']; $city_ID = $_SESSION['SESS_CITY_ID']; //Database connection $global_dbh = mysql_connect(DB_HOST, DB_USER, DB_PASSWORD) or die("Could not connect to database"); mysql_select_db(DB_DATABASE, $global_dbh) or die("Could not select database"); //query names of infantry troops $sql = "SELECT * FROM infantry WHERE member_id = '$member_ID'"; $result = mysql_query($sql) or die("Could not find amount of troops"); //get names of infantry troops while($data = mysql_fetch_assoc($result)) { $n[] = $data[name]; } //convert POST to normal variable $amount = array(0 => $_POST[$n[0]], 1 => $_POST[$n[1]], 2 => $_POST[$n[2]], 3 => $_POST[$n[3]]); ?>
-
Thnx that worked
Ryflex
-
Hi all,
Can anyone tell me why the declaration below won't work???
Thnx Ryflex
$amount = array(0 => $_POST['$n[0]'], 1 => $_POST['$n[1]'], 2 => $_POST['$n[2]'], 3 => $_POST['$n[3]']);
Making a livesearch
in Javascript Help
Posted
nvm solved in php part of the forum