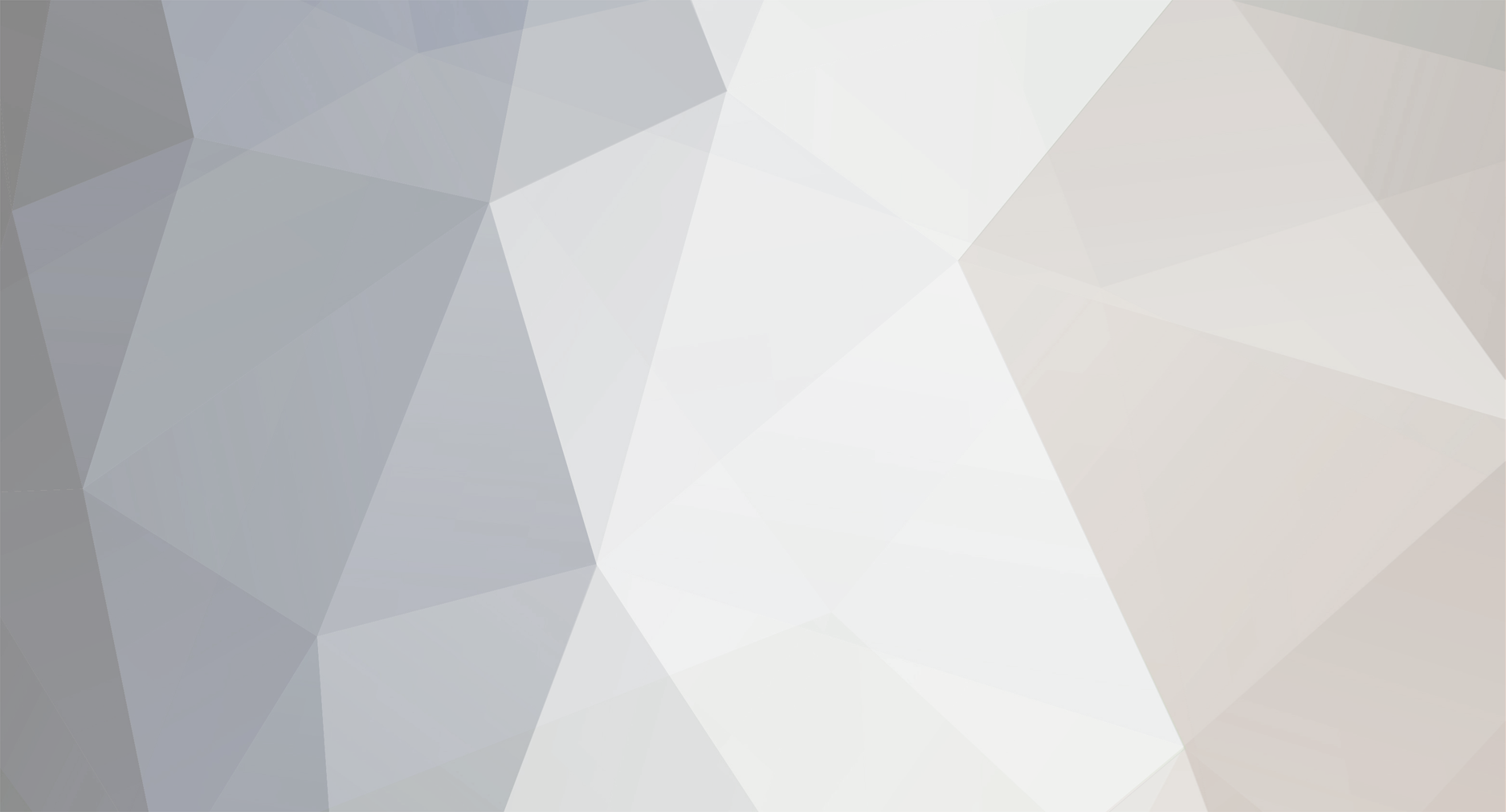
Oziam
Members-
Posts
44 -
Joined
-
Last visited
Never
Everything posted by Oziam
-
the session should end when the browser is closed, or you could try to delete your cookies!
-
try ob_start(); session_regenerate_id(); ob_end_flush(); EDIT: put ob_start(); just before the line require_once "scripts/mysqlconnect.php"; usually this error is caused when you try to send headers after they are already sent, so you need to set ob_start() function to send them to the buffer.
-
Query your database accordingly; e.g $query = "SELECT phone,email FROM users WHERE username = '$username' AND userpass = '$userpass' "; $res = mysql_query($query) or die(mysql_error()); if(mysql_num_rows($res) > 0){ $data = mysql_fetch_array($res, MYSQL_ASSOC); $phone = $data['phone']; $email = $data['email']; }
-
put GROUP BY cam_systems.id DESC LIMIT 0,10";
-
$string = 'four'; $variable1 = array("one","two","three","four","five"); echo in_array($string, $variable1) ? 'TRUE' : 'FALSE'; or if(in_array($string, $variable1)){ echo 'TRUE'; // do something } else{ echo 'FALSE'; // do something else }
-
Yes this is a very good point, I have an upload script for images which users can upload their image files, the problem is that the dir is CHMOD777 to get it to work other wise the file is rejected and php throws an error. This is because my hosts server has it set to SAFE MODE ON. This is a major security problem so I disable the php engine in a .htaccess file just for this directory. I know this is still not a fail safe method. If you have any other ideas which might help please share them! Thanks!!!
-
Or you could make sure the filetype is an actual image or pdf file! $file = $_FILES["file"]["type"]; if(($file == "image/gif") || ($file == "image/jpeg") || ($file == "image/pjpeg") || ($file == "image/x-png") || ($file == "application/pdf") || ($file == "application/x-pdf")){ // do upload } else{ // display error } you could also use a switch statement if you prefer.
-
Find your php.ini file and make sure you have error reporting on and set to error_reporting = E_ALL | E_STRICT this is about line 515 in my ini file. This will report all errors and help you trouble shoot your code!
-
Thanks for your input, I only use it for simple if else statements, and I am also aware of the pitfalls of stacking them. Cheers!
-
or you could use a common db inc file and have POST escaped automatically like; foreach ($_POST as $key => $value){ $_POST[$key] = mysql_real_escape_string($value); } this can save alot of time re-writing mysql_real_escape_string all the time! You could also use this for GET and REQUEST. Personally I use this and a similar code for htmlentities and strip_tags foreach ($_POST as $key => $value){ $_POST[$key] = htmlentities(strip_tags($value, ENT_QUOTES)); }
-
I have just discovered ternary conditionals and really like the time and space saving, they seem pretty simple and look neater than a bunch of if else statements crammed together. The thing I would like to know is what is the best or correct method of usng the following; $page = isset($_GET['page']) ? $_GET['page'] : '1'; or do I need the extra parentheses like below; $page = (isset($_GET['page'])) ? $_GET['page'] : '1'; I understand that if I used a second conditon I would need the extra parentheses e.g $page = (isset($_GET['page']) && is_numeric($_GET[page'])) ? $_GET['page'] : '1'; Thanks!
-
You have to use checked! e.g <input type=radio name=radio1 checked> and <input type=checkbox name=check1 checked> and if you want to show a select option as selected you use "selected" hope this helps!
-
There is also a cool class at PHP Classes called CSS Pagination, it can be easily included with multiple queries and styled to suit any site!
-
I assume the header.inc.php file contains the database connection and data for $openHTML and $closeHTML? Its hard to tell whats happening without knowing the openHTML and closeHTML output but try something like; <?php $rank_check = 1; $page_title = "Under Construction"; include "header.inc.php"; $query = "SELECT * FROM user_pets2 WHERE inrelationship = '0' AND gender = 'male' AND owner = '$userid'"; $result = mysql_query($query) or die(mysql_error()); $rows= null; while($a = mysql_fetch_array($result)){ $rows .= "Pet Name: $a[name] - Level: $a[level]<br />\n"; } echo $openHTML; echo $rows."<br />\n"; echo $closeHTML; exit; ?>
-
Yeah I agree, I just found more info to back this up; The method below seems to be the fastest way! $q1 = "SELECT COUNT(id) FROM table WHERE usrname='$usrname' "; $r1 = mysql_query($q1) or die(mysql_error()); $count= mysql_result($r1,0); mysql_free_result($r1); // only really needed if table has ALOT of entries // Thanks for your reply.
-
I want the quickest(least resource hungry) way of getting the number of specific rows from a large table. E.g I want to retrieve the number of entries by a specific username. What would be the best method? To use COUNT or just SELECT num_rows? Method 1: ------------- $query = "SELECT COUNT(usrname) AS usrname FROM table WHERE usrname='$usrname' "; $res = mysql_query($query) or die(mysql_error()); $array = mysql_fetch_array($res, MYSQL_ASSOC); $count = $array['usrname']; Method 2: ------------- $query = "SELECT usrname FROM table WHERE usrname='$usrname' "; $res = mysql_query($query) or die(mysql_error()); $count = mysql_num_rows($res); Personally I think method 2 would be quicker but I read alot about using count() to speed things up. Thanks!
-
Yeah I figured it out. It was the textarea wrap attribute, I had it set to virtual which reads data as a string but I changed it to physical and now it saves the data correctly as intended. Sorry a HTML gotcha nothing to do with PHP.
-
I am no expert but have you tried to free and close after setting the sessions! // If user exists log them in if($userExists){ // Set the SESSION variables $_SESSION['first_name'] = $userExists['first_name']; $_SESSION['id'] = $userExists['id']; mysql_free_result($doQuery); mysql_close();
-
Hi guys, I have come across a problem when working with session data, I have been storing data from a textarea in a session, but the problem is when I retrieve the data and display it back in a textarea or to mysql it saves the carriage returns and line breaks as \r\n not converting it to actual line breaks. e.g saving the following from a text area; Line 1 Line 2 Line 3 will actually show as: Line 1 \r\nLine 2 \r\nLine3 How do I get it to show properly as intended? I have tried str_replace('\r\n', '\n'); with double and single quotes any helpful suggestions would be much appreciated. Thanks..