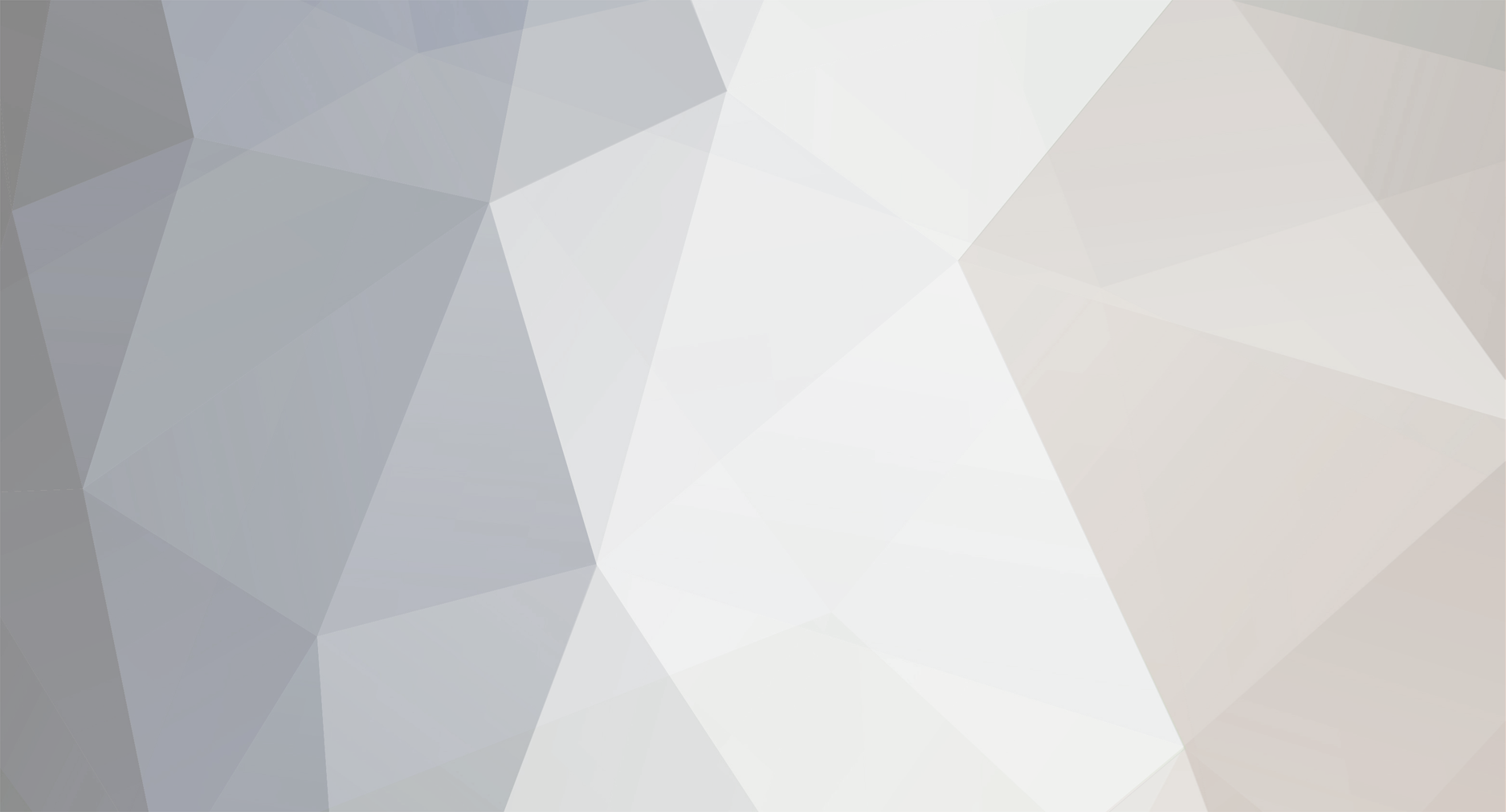
smerny
Members-
Posts
855 -
Joined
-
Last visited
Profile Information
-
Gender
Not Telling
smerny's Achievements

Advanced Member (4/5)
0
Reputation
-
I have an Item model which has the following associations: public $hasOne = array( 'Project' => array( 'className' => 'Project', 'foreignKey' => 'item_id' ) ); public $hasMany = array( 'ItemPic' => array( 'className' => 'ItemPic', 'foreignKey' => 'item_id', 'dependent' => false ) ); I am wanting custom data for different views of Item. It seems like CakePHP automatically includes Project data (maybe because it is hasOne?) and does not include the ItemPic data. In the index I really don't even want the Project data... however, I do want the ItemPic data. For each Item record pulled, I want a single ItemPic record joined to it. This ItemPic should be basically ItemPic.item_id = Item.id and then only the one with the smallest rank (if there are multiple with the same rank it doesn't really matter which one is pulled). The purpose of this is basically so that in the index I can show a list of Items and a picture associated with each item. I would like all of the images along with the Project data in the view for a single Item, but not in the list/index. I've learned I can use containable like this: // In the model public $actsAs = array('Containable'); // In the controller $this->paginate = array( 'conditions' => $conditions, 'contain' => array( 'ItemPic' => array( 'fields' => array('file_name'), 'order' => 'rank', 'limit' => 1 ) ) ); The above actually works how I want... however, I was also told that doing this would cause an extra query to be ran for every single Item... which I feel I should avoid... but perhaps I am wrong on feeling that I should avoid this? I tried doing this, but I get duplicate data... I'm assuming the order and limit don't work here (or atleast not how I assumed it would). It is also still joining Project which I would like to avoid if possible as that data is not necessary in the Items index: $this->paginate = array( 'conditions' => $conditions, 'joins' => array( array( 'table' => 'item_pics', 'alias' => 'ItemPic', 'type' => 'LEFT', 'conditions' => array( 'ItemPic.item_id = Item.id' ), 'order' => 'rank ASC', 'limit' => 1 ) ), 'fields' => array('Item.*','ItemPic.*') ); $paginated = $this->Paginator->paginate(); Resulting SQL: (still joining Project, not restricting ItemPic join) SELECT `Item`.*, `ItemPic`.*, `Item`.`id` FROM `abc`.`items` AS `Item` LEFT JOIN `abc`.`item_pics` AS `ItemPic` ON(`ItemPic`.`item_id` = `Item`.`id`) LEFT JOIN `abc`.`projects` AS `Project` ON (`Project`.`item_id` = `Item`.`id`) WHERE `Item`.`type` IN (0, 2) LIMIT 20 Resulting Data: (getting the same Item with all its associated ItemPics) array( (int) 0 => array( 'Item' => array( 'id' => '3', ... ), 'ItemPic' => array( 'id' => '1', 'item_id' => '3', ... ) ), (int) 1 => array( 'Item' => array( 'id' => '3', ... ), 'ItemPic' => array( 'id' => '3', 'item_id' => '3', ... ) ), (int) 2 => array( 'Item' => array( 'id' => '3', ... ), 'ItemPic' => array( 'id' => '4', 'item_id' => '3', ... ) ), ... )
-
Thanks, that gives me something to work with anyway. I'm also going to look into the "long poll" or "Comet" approach based on what I'm seeing here: http://stackoverflow.com/a/1086448/684368
- 6 replies
-
- php
- javascript
-
(and 1 more)
Tagged with:
-
Can you give an example value of $name and what the resulting record's name field is?
-
Okay, I'm not exactly sure how this "cache file" thing would work. I need to update the page for all of the users viewing it as soon as possible. If I have a high refresh rate it will mean too many database pings (there are often over 100 people at one time, which would mean 5 database pings per second even with 20 second intervals). If I don't ping the database each time, how will it update? My thoughts/questions after seeing your suggestions... Does this cache file mean that I create an xml or json file or something like that with the current data? Then instead of checking the database, each refresh (lets say I bring it to a couple seconds instead of 20) will check for the date this cache file was created. If the cache file was created more than (lets say) 2 seconds ago, it will read that. If the cache file is more than 2 seconds ago, it will ping the database and create a new cache file. This should then only ping the database every 2 seconds even with 100 people, instead of 0.2 seconds. Do I have the basic idea right? This leads me to a couple more questions: will this create any race conditions where multiple clients hit when the cache file is 2 seconds old or more before the first client creates the cache file? will checking the creation time of this file and loading this file really be much quicker than just reading the database?
- 6 replies
-
- php
- javascript
-
(and 1 more)
Tagged with:
-
Thanks for your response. I have to leave soon now so I will read it more thoroughly later, meanwhile I don't know if it will change your answer at all but I will answer your questions. I have a table with 100+ items, but only 10-15 of these items are showing at any time (there is a field determining whether or not they will belong in this list, and that field can change if a moderator determines it should show up or not). I allow the public to modify a certain field relating to these items, but because I want to save History of these edits, I have this "field" in its own table which has a foreign key to match up these "edits" with the item they belong to. On the webpage with the list, I show the items (various fields that don't generally change) that have the status field set to 1 along with a couple fields from the most latest record of the "edits" table associated with each item. The "edits" table has many updates (actually inserts) throughout the day. These inserts are done via public users doing a form submission. The "status" field of the main list items table is generally only updated a few times during the same half hour period every week. There will not be deleted records, but if an "edit" is flagged as bad, I will actually show the previous edit instead. This is currently done by showing (associated with each list item) the most recent "edit" that does not have the flag status. If you believe I should change any of this to get a better result, please feel free to offer any suggestions.
- 6 replies
-
- php
- javascript
-
(and 1 more)
Tagged with:
-
So I have a webpage where I pull several rows from the database and create a list on the webpage. Any one of these rows can get updated independently at any time. For the past several years I've used something like this: function execRefresh() { $("#refreshIcon").fadeIn("fast"); $("#'. $p .'").load("/data.php?inid='. $p .'&rand=" + Math.random(), "", function () { $("#refreshIcon").fadeOut("slow"); } ); setTimeout(execRefresh, 20000) } $(document).ready(function () { execRefresh(); }); This will reload the entire content from the database to repopulate the list on the webpage. I'm wondering if there is a better way to do this. The downfalls I'd like to overcome if possible would be (and I realize these two overlap a bit): 1. I am loading the entire table for everyone on the webpage even if there are no changes (unnecessary loads/database pings). - Is there a way to only pull new data if there is a change? - Is there a way to only pull the rows that got changed? - Is there a way to make it so that I don't have to make a call to the database for every single user? 2. I have to create an interval to determine how often I reload the data. Currently I have 20 seconds, this means if an update occurs right after the user loaded data, it could be up to 20 seconds before they see that change (not loading when necessary). - Is there a way to tell the client there has been a change and it needs to update so that it doesn't have extended periods of time where the data isn't updated without just making the interval shorter (and thus having more unnecessary loads)? I know that, for example, Google chat is nearly instantaneous in telling you "Someone is typing" and then showing what they sent as a chat. I imagine that they don't have millions of users constantly pinging a database that contains the chats and whether or not a user is currently typing every second. What would the best way to do this be? I assume it's relatively common and there are possibly some best practices for things such as this. Thanks
- 6 replies
-
- php
- javascript
-
(and 1 more)
Tagged with:
-
i guess i'm just not sure why you are repeating one of the issues i said that i am unable to solve
-
i'm assuming you are getting only the "open" status rows of Team 1, but "open" and "closed" for the other teams? put parens around all the helptopic conditionals
-
that is fine, "blah" was one of the "Examples of what I want to pass would be" list. the problems i'm having is: in the second line, blah has a dot at the end... i want to prevent that.. as well as allow for just ".."
-
i guess the "has an a-z char at the end" part isn't working either... i can see why i think (getting caught in the part before it) but not sure how to fix it so right now, the best i have is "^([a-z]+[a-z.\\-]*)([\\*]*)[\\[]*([^\\]]*)[\\]]*" but it doesn't find just ".." and it includes like "blah." for example, when it shouldn't
-
btw, at this point i'm not really concerned with the exact format of the attribute list (just capture anything within square brackets) I redid the main part like this: "^([a-z]+[a-z.\\-]*[a-z])([\\*]*)[\\[]*([^\\]]*)[\\]]*" and it seems to work (besides the ".." case)... this is what I want to do to add the ".." case: "^([\\.]{2})$ | ^([a-z]+[a-z.\\-]*[a-z])([\\*]*)[\\[]*([^\\]]*)[\\]]*" but that doesn't work ^([\\.]{2})$ - starts and ends with ".." | - or ^([a-z]+[a-z.\\-]*[a-z]) - starts with atleast one a-z char... can contain 0 or more a-z, dots, or dash... has an a-z char at the end ([\\*]*) - can have optional * [\\[]*([^\\]]*)[\\]]* - can have optional [ ] with content between
-
Currently have this regex pattern thats probably pretty bad as I am not a regex pro and have been trying to tweak on it to make it work for what I need: "^(^[a-z.{2}][^\\s][a-z|.{2}]*[.]*[a-z]*)([\\*]*)[\\[]*([^\\]]*)[\\]]*" and it is working for most cases... but not all, and I'm having difficulties Examples of what I want to pass would be: [*]".." [*]"blah" [*]"blah*" [*]"blah.blah" [*]"blah.blah*" [*]any of 2-5 with [@attrib=value] or [@attrib="value" and @attrib2="value2" and ...] appended to the end examples of what I don't want to pass would be: [*]"" [*]"." [*]" " [*]"..." [*]".blah" [*]"-blah" basically want ".." or a string of a-z that could include but not start with dots or dashes -that is optionally followed by a * -that is optionally followed by a list of attributes/values with groups being: 1) ".." or a string a-z including but not starting with dots/dashes 2) "*" or StringUtils.EMPTY if no match 3) the group of attributes or StringUtils.EMPTY if no match
-
I have a database with many records... does anyone know a good script that if I start typing, and as I type it filters down the results? or some helpful pointers on going about it? For instance, if I type "East" in a text field, it will filter down the results to anything that has East (case insensitive) in it? Like: - blah Northeast blah - blah East blah - blah Eastern blah Thanks
-
AJAX is if you need to get show the data without loading an entire page, your current code appears to load the psearch.php page upon submit. After your: $result = mysql_query($query) or die(mysql_error()); Put: $row = mysql_fetch_assoc($result); echo $row['FName']; This should give you an idea of how to do whatever you need to do.
-
nothing is changing in the WHERE you are setting whatever rows match your WHERE to 1, then to 2, then to 3, etc...