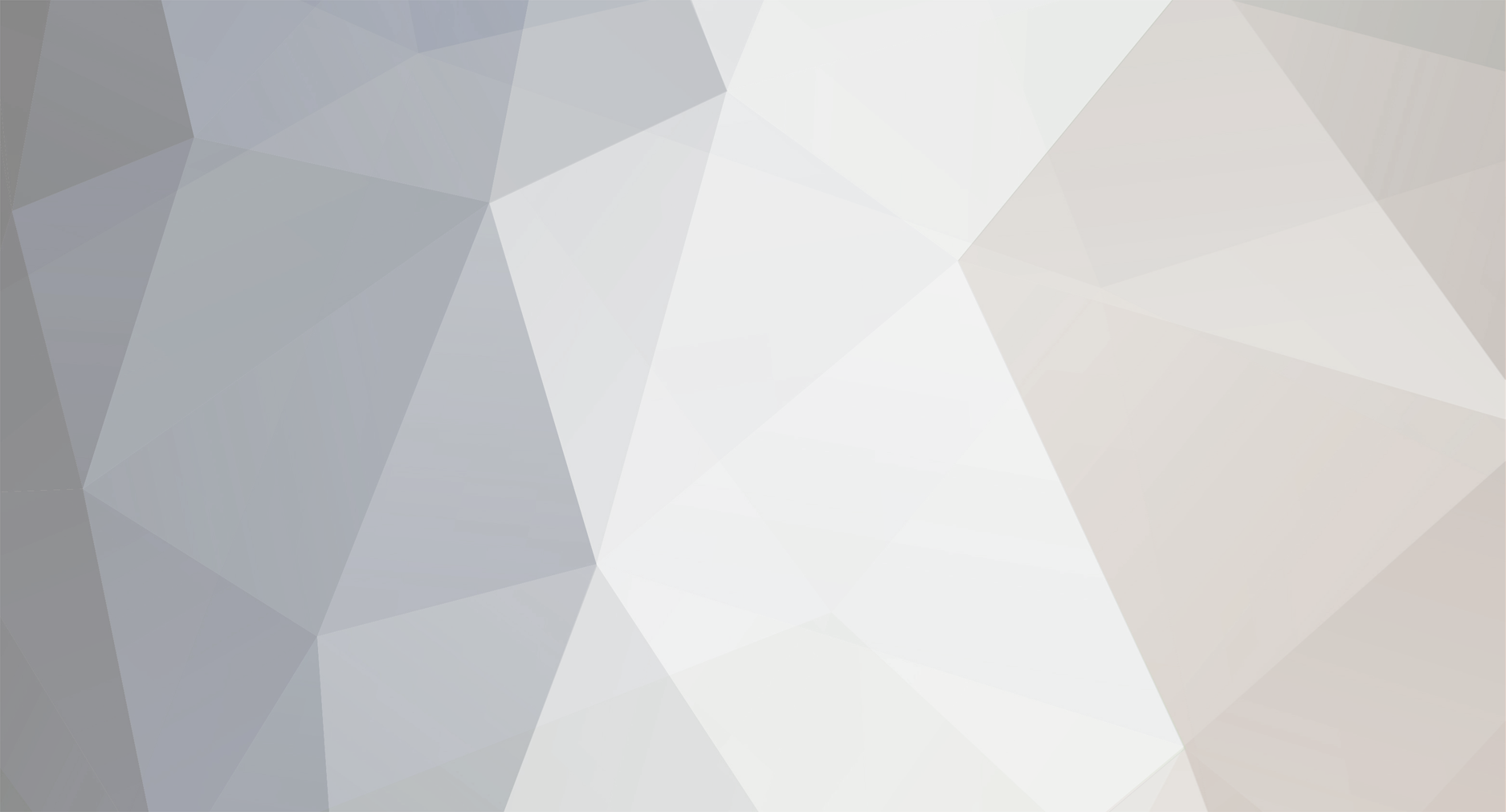
ManiacDan
Staff Alumni-
Posts
2,604 -
Joined
-
Last visited
-
Days Won
10
Everything posted by ManiacDan
-
Help with preg_match_all('!/search/(.*)/([0-9])!i', $page, $pages);
ManiacDan replied to jamesmiller's topic in Regex Help
This isn't valid output from a print_r statement, there appears to be a great amount of this array missing. Are you using preg_match or preg_match_all? If you're using preg_match_all then your result will be an array of arrays of matches like I described. Show some more code so we can tell exactly what's up here. Maybe a little sample app -
If you want only unique items, array_unique() is the solution. If you want the items grouped by count, array_count_values() is the solution. Since you said you wanted it similar to GROUP BY (which implies a count) rather than UNIQUE (which implies uniqueness), I gave you the GROUP BY solution. -Dan
-
Help with preg_match_all('!/search/(.*)/([0-9])!i', $page, $pages);
ManiacDan replied to jamesmiller's topic in Regex Help
The parentheses determine the "capture group." $matches[0] will be the whole pattern. $matches[1] will be the first set of parens, $matches[2] will be the second set, etc. What you've posted is too poorly formatted for me to tell what exactly is going on. Use [ code ] tags around your output so we can see it better. -Dan -
So you're requiring a login on every single click? Why? What possible security can that afford? Aren't people going to be pissed off at having to type a password every time they do anything on your site?
-
As much as I hate sounding like a jerk for saying so: if your database was designed properly you wouldn't have run into this problem. You should have made this into a cross-reference table so you can do your many-to-many relationships without resorting to storing a delimited string (which as you've discovered is difficult to maintain and display). To solve your immediate problem: put all these items into a temporary array then call array_count_values -Dan
-
Nothing at all? If one field is emtpy, don't perform any actions or display any errors, just stop where you are? If you want the field to not be updated unless there's content submitted, then you'll have to build your field list by checking to see which submitted fields are empty. -Dan
-
Help with preg_match_all('!/search/(.*)/([0-9])!i', $page, $pages);
ManiacDan replied to jamesmiller's topic in Regex Help
Regular expressions have "modifiers" that alter the number of items a specific block can match. Putting a plus after something means "one or more times." So if you did [0-9]+ that would match any number of characters. Regular expressions also have built in character classes. \d means "any digit," so you can replace [0-9]+ with \d+ and get the same results, plus many people think it's more readable. -Dan -
There is absolutely no reason for your inner query and loop at all. Your outer query already fetches all the data from the user table, don't make another round trip to the database for every status.
-
I disagree with portions of that article posted. He claims there's no reason to use sessions and talks about using cookies instead. The problem with that is cookies are limited to 2kb in some browsers and are not at all serial in nature. You cannot have a shared read-write of cookies between scripts. Only the most recent request counts, and cookies can easily be overwritten if you rely on them for data storage. Cookies are sent along with every web request, so if you store as much data as you can in the cookies you're increasing the size of EVERY request, in both directions. Cookies can be read by the user and anyone on the same network or who can sniff traffic in the middle (unless encrypted, like it says in the article) Having been a developer on a number of very large websites ("very large" as in 100,000+ hits per second), the concept of server-stored sessions is very powerful, but once you scale beyond a single server you cannot rely on the default PHP session handler, you have to write your own. I'd never rely on cookies for this, I've always uses memcache or a custom listener that accepted read/write requests in parallel from multiple running scripts. -Dan
-
Not at all, no. The session is stored on your server and can only be accessed by a user with the proper cookie. Sessions are vulnerable to hijacking, but it's generally not a huge concern. Your site itself will be vulnerable to this sort of attack, the location of the personal data doesn't really matter. Once your site is big enough to where session hijacking (through something like firesheep) is a problem, you'll know enough to roll your own session handler with built-in security and verification. -Dan
-
Isn't that...precisely what you want? Why is that solution not correct for you? You could try floor($var2/10)+1; -Dan
-
I worked at a social network for the last couple years. It's possible to exist alongside facebook, but you need tens of millions of dollars and at least a dozen developers, hopefully more. Plus, advertising, compelling content, and lots and lots of luck. -Dan
-
Make one table with all the "other" fields from this one, and another table that stores the Key and the ID of the first table. When the page is submitted: - take all the "other" data and make an entry in the metadata table - retrieve the ID you just inserted - insert all the keys in a loop (or in one multi-line insert statement) specifying the ID of the table to link to.
-
You absolutely should never do SQL inside of a loop, a JOIN is almost always the correct replacement. In fact, you've DONE your join, yet you still do unnecessary queries inside your loop. print_r($row) to see what the contents are. Maybe you're doing it wrong. There's nothing here to indicate that your array indicies are correct. If you're making a social network for the fun of it and to learn, it's a good place to start. Lots of complex relationships in a social network. If you're doing it to actually get traffic or make a business, stop right now. -Dan
-
If you have all the other variables from the form, then include them in your statement. Get $keystatus and all the others into their variables, then include them in your loop. Also, repeating the data like this is a violation of normal forms, take a look at that link for database design tips. -Dan
-
It would probably be best if you hired someone to do this. I doubt someone will dive into your CMS and make a custom VIP membership module for free. -Dan
-
That won't speed anything up. In fact, it will slow it down. If your goal is to display all the records in this table on the same page, you're doing it the fastest way possible. It would be slightly faster to replace your table data with <br /> so that you're sending fewer characters, but not much faster. You may also want to look into pagination so you only print 100 at a time instead of the whole table (however big it is). There is also the option of flushing the output buffer. When you output data in PHP, the web server "remembers" what you're trying to send and will send it in large chunks. If you have 5,000 lines in your output, the webserver may send 1,000 at a time, 5 times. The delay between these "chunks" appears like slowness to the user. You can put this line after your echo to make the records show up faster: ob_flush();flush(); However, note that this actually slows down the site because there's a lot of extra overhead in sending a small amount of output through a system designed for an entire HTML document. -Dan
-
Facebook uses a graph database, not a traditional relational database. You have to think about your data differently. -Dan
-
A very large percentage of people use IE still, sadly. IE should be what you target first, the others should be the cleanup. I'd go back and fix your site in IE right now, and work on making it cross-browser compatible from now on. -Dan
-
You* Seriously, this is a technical forum for professionals and amateurs in a highly skilled and educated field. It's not a text message to your friends. That kind of typing will get some people to actively antagonize you.
-
The EXACT same error? Or now it's saying "access denied for user 'root'@'localhost' (using password: NO)"? If it's exactly the same, your code isn't what you say it is, paste the whole thing again. If it's changed the one I posted above, then your password for root is not "null," you probably just want an empty string "" -Dan
-
Trying to make my form send info to two different email addresses.
ManiacDan replied to KDM's topic in PHP Coding Help
None of that will work. You can't single-quote PHP variables. $to = array('********@yahoo.com', '********@************.com'); foreach ( $to as $address ) { $mailSent = mail($address, $subject, $message, $headers); } -Dan -
You're still not doing it right. Take the $this-> out of the mysql_connect call, just use $host, $user, and $pass that you're passing in. Research variable scope and OOP -Dan