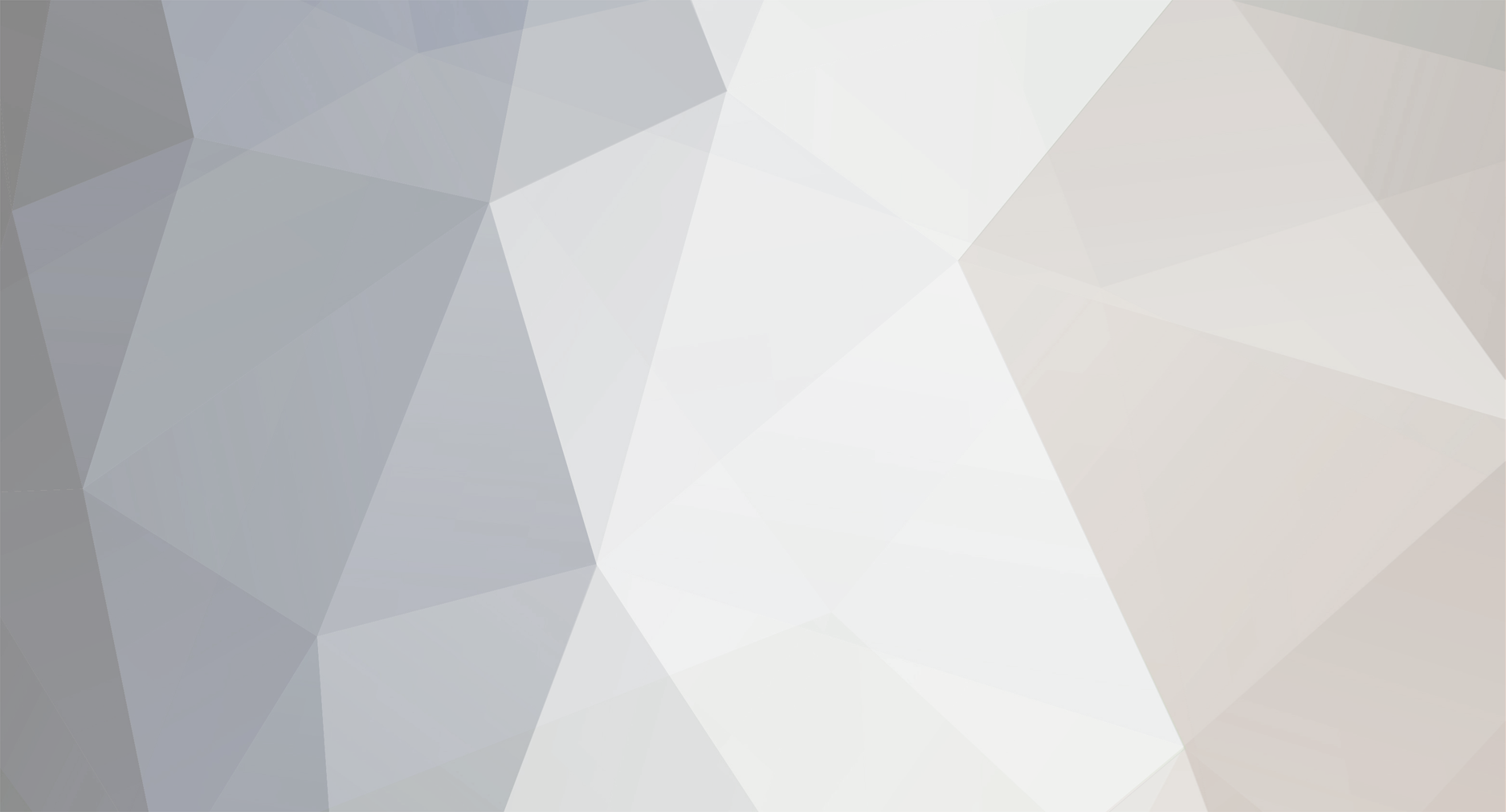
spacepoet
Members-
Posts
320 -
Joined
-
Last visited
Everything posted by spacepoet
-
Well, I do allow the user to be able to update their username / password, so I do need it in a database for that reason ... (I don't want to use a flat file - if it can be done that way). Any ideas where to find a script like this? This is what I am currently using: Login.php <?php include('../include/myConn.php'); include('include/myAdminNav.php'); session_start(); session_destroy(); $message=""; $Login=$_POST['Login']; if($Login){ $myUserName=$_POST['myUserName']; $myPassword=$_POST['myPassword']; $result=mysql_query("select * from myAdmins where myUserName='$myUserName' and myPassword='$myPassword'"); if(mysql_num_rows($result)!='0'){ session_register("myUserName"); header("location:a_Home.php"); exit; }else{ $message="<div class=\"myAdminLoginError\">Incorrect Username or Password</div>"; } } ?> ... <html> <form id="form1" name="form1" method="post" action="<? echo $PHP_SELF; ?>"> <? echo $message; ?> User Name: Password: <input name="myUserName" type="text" id="myUserName" size="40" /> <input name="myPassword" type="password" id="myPassword" size="40" /> <input name="Login" type="submit" id="Login" value="Login" /> </form> </html> a_Home.php <? session_start(); if(!session_is_registered(myUserName)){ //setcookie("TestCookie", $value, time()+1200); header("location:Login.php"); } ?> <html> ... </html> a_Admins.php (to update the username / password, if desired): <?php include('../include/myConn.php'); if ($_SERVER['REQUEST_METHOD'] == 'POST') { $myUserName = mysql_real_escape_string($_POST['myUserName']); $myPassword = mysql_real_escape_string($_POST['myPassword']); $sql = " UPDATE myAdmins SET myUserName = '$myUserName', myPassword = '$myPassword' "; mysql_query($sql) && mysql_affected_rows() ?> <?php } $query=mysql_query("SELECT * FROM myAdmins") or die("Could not get data from db: ".mysql_error()); while($result=mysql_fetch_array($query)) { $myUserName=$result['myUserName']; $myPassword=$result['myPassword']; } ?> <html> ... <?php if ($_SERVER['REQUEST_METHOD'] == 'POST') echo "<span class=\"textError\">Section successfully updated!</span>" ?> <form method="post" action="<?php echo $PHP_SELF;?>"> <input type="hidden" name="POSTBACK" value="EDIT"> User Name: <input type="text" size="60" maxlength="60" name="myUserName" value="<?php echo $myUserName; ?>"> Password: <input type="password" size="60" maxlength="60" name="myPassword" value="<?php echo $myPassword; ?>"> <input type="submit" value="Submit" /> </form> ... </html> Maybe my original question should have been - "how do I make this login method more secure / better ..." ???
-
Data will not display for Staff listings .. ??
spacepoet replied to spacepoet's topic in PHP Coding Help
Hi: I have found another solution. I abandoned the above and have turned my photo gallery into a staff list! Working so far, but I am sure I will have some questions. Thanks for the help! -
Hi: OK, thanks for pointing this out .. lol .. I was beginning to wonder how "good" the script is, since I'm having great difficulty getting it to work. So, may I ask you: Are there any scripts you can show me or point me to that would be considered "solid" and "modern" in terms off secure login? I am not sure what to look for. What I really need is a password protected page system - no need to register or any of that. Just a way to let 1 admin login securely and view password protected pages. The way I current do it when setting up a site is to create a "myAdmins" SQL table via phpMyAdmin that contains a pre-defined username and password (I use "test" and "test"), and I have a single-page login form. And of course, a small bit of code on top of each password-protected page to make sure the user has logged-in properly. That's really all I need. I didn't think finding a more modern, encrypted solution was going to be so frustrating. Can you help me with that?
-
Hi: Not sure I understand what you mean... The database has a "test" username and password. Is that what you mean?
-
Hello: I am using this tutorial to make a secure login system (if there is a "better" way, please let me know): http://tinsology.net/2009/06/creating-a-secure-login-system-the-right-way/ I am having a problem with the login form - it keeps moving to the "a_Home.php" page (the one that is suppose to be password protected) without any login information being entered. This is the mmLogin.php page: <?php include('../include/myConn.php'); include('include/myAdminCodeLib.php'); session_start(); $username = $_POST['username']; $password = $_POST['password']; $username = mysql_real_escape_string($username); $query = "SELECT password, salt FROM users WHERE username = '$username';"; $result = mysql_query($query); if(mysql_num_rows($result) < 1) { header('Location: mmLogin.php'); die(); } $userData = mysql_fetch_array($result, MYSQL_ASSOC); $hash = hash('sha256', $userData['salt'] . hash('sha256', $password) ); if($hash != $userData['password']) { header('Location: mmLogin.php'); die(); } else { validateUser(); header('Location: a_Home.php'); } ?> <html> <head></head> <body> <form name="login" action="mmLogin.php" method="post"> Username: <input type="text" name="username" /> Password: <input type="password" name="password" /> <input type="submit" value="Login" /> </form> </body> </html> This is the a_Home.php page: <?php include('include/myAdminCodeLib.php'); include('include/myCheckLogin.php'); ?> <html> <head></head> <body> <a href="mmLogin.php">Log Off</a> </body> </html> This is the myCheckLogin.php page: <?php session_start(); if(!isLoggedIn()) { header('Location: mmLogin.php'); die(); } ?> This is the myAdminCodeLib.php page: <?php function validateUser() { session_regenerate_id (); $_SESSION['valid'] = 1; $_SESSION['userid'] = $userid; } function isLoggedIn() { if(isset($_SESSION['valid']) && $_SESSION['valid']) return true; return false; } function logout() { $_SESSION = array(); session_destroy(); } ?> Can anyone tell me why this is not working? And, am I calling the functions properly? Thanks.
-
Data will not display for Staff listings .. ??
spacepoet replied to spacepoet's topic in PHP Coding Help
Hi: Thanks, but I had tried that earlier - no luck .. Any other suggestions? Thanks! -
Hello: I am building a Staff list, that I want to use to allow the owner of a company to upload a photo, name, phone, and email of each person on his staff. I am using this approach from a tutorial I found online: Database: CREATE TABLE employees (id int(5), name VARCHAR(30), email VARCHAR(30), phone VARCHAR(30), photo VARCHAR(30)) Form: <form enctype="multipart/form-data" action="Add.php" method="POST"> Name: <input type="text" name="name"><br> E-mail: <input type="text" name = "email"><br> Phone: <input type="text" name = "phone"><br> Photo: <input type="file" name="photo"><br> <input type="submit" value="Add"> </form> Add.php <?php include('include/myConn.php'); ?> ... <?php $target = "images/"; $target = $target . basename( $_FILES['photo']['name']); $name=$_POST['name']; $email=$_POST['email']; $phone=$_POST['phone']; $pic=($_FILES['photo']['name']); mysql_query("INSERT INTO `employees` VALUES ('$name', '$email', '$phone', '$pic')") ; if(move_uploaded_file($_FILES['photo']['tmp_name'], $target)) { echo "The file ". basename( $_FILES['uploadedfile']['name']). " has been uploaded, and your information has been added to the directory"; } else { echo "Sorry, there was a problem uploading your file."; } ?> Display.php <?php include('include/myConn.php'); ?> ... <?php $data = mysql_query("SELECT * FROM employees") or die(mysql_error()); while($info = mysql_fetch_array($data)); { echo "<img src=images/".$info['photo'] ."> <br>"; echo "<b>Name:</b> ".$info['name'] . "<br> "; echo "<b>Email:</b> ".$info['email'] . " <br>"; echo "<b>Phone:</b> ".$info['phone'] . " <hr>"; } ?> I am not getting any errors and the photo is uploaded to the "images" folder, but none of the data displays ... I do not see why .. Any ideas? Also, if any one has done something like this before and has a better approach to doing this, I am all ears. I do want to allow the owner to edit and delete the profiles as well. Thanks.
-
Hi again - OK, thanks for the tip. Let me try to add this code and see what happens. Much appreciated!
-
Hi: Thank you. So, I would add it under the code I posted? Something like: mail( "me@mywebsite.com", "Sign-up Sheet Request", "Date Sent: $myDate\n Parent's Name: $ParentsName Best Phone: $BestPhone Email: $Email Student's Name: $StudentsName Student's School: $StudentsSchool\n ", "From: $Email" mail( $_POST['email'], "Thank you", "$ParentsName, Thank you. etc etc ", 'From: admin@domain.com' . "\r\n" . 'Reply-To: admin@domain.com' . "\r\n" . 'X-Mailer: PHP/' . phpversion() ); Something like that? Please let me know and thanks.
-
Hello: I have this form below that stores the data in a database, and sends an email to my client so he gets the information (And if there is a more proper way to code this, I am all ears): <?php include('include/myConn.php'); ?> <html> ... <?php $error = NULL; $myDate = NULL; $ParentsName = NULL; $BestPhone = NULL; $Email = NULL; $StudentsName = NULL; $StudentsSchool = NULL; if(isset($_POST['submit'])) { $myDate = $_POST['myDate']; $ParentsName = $_POST['ParentsName']; $BestPhone = $_POST['BestPhone']; $Email = $_POST['Email']; $StudentsName = $_POST['StudentsName']; $StudentsSchool = $_POST['StudentsSchool']; if(empty($ParentsName)) { $error .= '<div style=\'margin-bottom: 6px;\'>- Enter the parent\'s name.</div>'; } if(empty($BestPhone)) { $error .= '<div style=\'margin-bottom: 6px;\'>- Enter the best phone number to reach you.</div>'; } if(empty($Email) || !preg_match('~^([0-9a-zA-Z]([-.\w]*[0-9a-zA-Z])*@([0-9a-zA-Z][-\w]*[0-9a-zA-Z]\.)+[a-zA-Z]{2,9})$~',$Email)) { $error .= '<div style=\'margin-bottom: 6px;\'>- Enter a valid email.</div>'; } if(empty($StudentsName)) { $error .= '<div style=\'margin-bottom: 6px;\'>- Enter the student\'s name.</div>'; } if(empty($StudentsSchool)) { $error .= '<div style=\'margin-bottom: 6px;\'>- Enter the student\'s school.</div>'; } if($error == NULL) { $sql = sprintf("INSERT INTO mySignUpData(myDate,ParentsName,BestPhone,Email,StudentsName,StudentsSchool) VALUES ('%s','%s','%s','%s','%s','%s')", mysql_real_escape_string($myDate), mysql_real_escape_string($ParentsName), mysql_real_escape_string($BestPhone), mysql_real_escape_string($Email), mysql_real_escape_string($StudentsName), mysql_real_escape_string($StudentsSchool); if(mysql_query($sql)) { $error .= '<div style=\'margin-bottom: 6px;\'>Thank you for using the sign-up sheet.</div>'; mail( "me@mywebsite.com", "Sign-up Sheet Request", "Date Sent: $myDate\n Parent's Name: $ParentsName Best Phone: $BestPhone Email: $Email Student's Name: $StudentsName Student's School: $StudentsSchool\n ", "From: $Email" ); unset($ParentsName); unset($BestPhone); unset($Email); unset($StudentsName); unset($StudentsSchool); } else { $error .= 'There was an error in our Database, please Try again!'; } } } ?> <form name="myform" action="" method="post"> <input type="hidden" name="myDate" size="45" maxlength="50" value="<?php echo date("F j, Y"); ?>" /> <div id="tableFormDiv2"> <fieldset> <hr /> </fieldset> <fieldset><span class="floatLeftFormWidth2"><span class="textErrorItalic">* - Required</span></span> <span class="floatFormLeft"> </span></fieldset> <?php echo '<span class="textError">' . $error . '</span>';?> <fieldset><span class="floatLeftFormWidth2"><span class="textErrorItalic">*</span> Parent's Name:</span> <span class="floatFormLeft"><input type="text" name="ParentsName" size="45" maxlength="50" value="<?php echo $ParentsName; ?>" /></span></fieldset> <fieldset><span class="floatLeftFormWidth2"><span class="textErrorItalic">*</span> Best Phone # To Reach You:</span> <span class="floatFormLeft"><input type="text" name="BestPhone" size="45" maxlength="50" value="<?php echo $BestPhone; ?>" /></span></fieldset> <fieldset><span class="floatLeftFormWidth2"><span class="textErrorItalic">*</span> Email:</span> <span class="floatFormLeft"><input type="text" name="Email" size="45" maxlength="50" value="<?php echo $Email; ?>" /></span></fieldset> <fieldset><span class="floatLeftFormWidth2"><span class="textErrorItalic">*</span> Student's Name:</span> <span class="floatFormLeft"><input type="text" name="StudentsName" size="45" maxlength="50" value="<?php echo $StudentsName; ?>" /></span></fieldset> <fieldset><span class="floatLeftFormWidth2"><span class="textErrorItalic">*</span> Student's School:</span> <span class="floatFormLeft"><input type="text" name="StudentsSchool" size="45" maxlength="50" value="<?php echo $StudentsSchool; ?>" /></span></fieldset> </div> <input type="submit" name="submit" value="Click here to submit your registration form" class="submitButton2" /><br /> </form> </html> I want to add a feature that will also send an autoresponder email with a message to the user who submitted the form. How can that be done? Do I need to somehow revise this section: mail( "me@mywebsite.com", "Sign-up Sheet Request", "Date Sent: $myDate\n Parent's Name: $ParentsName Best Phone: $BestPhone Email: $Email Student's Name: $StudentsName Student's School: $StudentsSchool\n ", "From: $Email" Please let me know and thanks in advance.
-
Excellent! Thank you very much. Just what I was looking for. Thanks!
-
Dynamically Make ALL UPPERCASE to Capitalize ...?
spacepoet replied to spacepoet's topic in PHP Coding Help
Hi ... Could you possibly give me an example of how to add that? I really do not know where to start with it... Thanks. -
OK, thank you. I think I see where you are going with it. Something like: <?php echo . $city . " ," . $full_state . $zip . ?> ??? I basically want it to read "City, State Zipcode" Example: Downingtown, Pennsylvania 19335 Will that work that way?
-
Hello: I am writing this code: <?php include('include/myConn.php'); include('include/myCodeLib.php'); $zip_id = $_REQUEST['zip_id']; $query=mysql_query("SELECT zip_id,city,full_state,zip FROM zip_codes WHERE zip_id = $zip_id") or die("Could not get data from db: ".mysql_error()); while($result=mysql_fetch_array($query)) { $zip_id=$result['zip_id']; $city=$result['city']; $full_state=$result['full_state']; $zip=$result['zip']; } ?> <!DOCTYPE HTML> <head> <title></title> </head> <body> <?php echo $city; ?>, <?php echo $full_state; ?> <?php echo $zip; ?> </body> </html> It works fine as far as writing out the "City, State Zipcode", but I wanted to see if there is a way to "join" the 3 statements together so it can be written as just 1 statement. Like: <?php echo $city, $full_state $zip; ?> What is the trick to doing this? Thanks in advance.
-
Hello: I have this little bit of code to pull all the towns and zip codes from a mySQL database: <?php $result = mysql_query("SELECT zip_id,city,abbr_state,full_state,zip FROM zip_codes WHERE abbr_state = '" . mysql_real_escape_string ( $_GET['abbr_state'] ) . "' ORDER BY `city` ASC"); while($row = mysql_fetch_array($result)) { echo "<a href=\"Stores.php?zip_id=".$row['zip_id']."\" title=\"My Products ". $row['city']. ", ". $row['abbr_state']." ". $row['zip']." | My Items ". $row['city']. ", ". $row['abbr_state']." ". $row['zip']."\">". $row['city']. ", ". $row['abbr_state']." ". $row['zip']."</a>"; echo "<div style=\"clear: both; margin-bottom: 15px;\"></div>"; } ?> Works fine (unless some can offer a way to "improve" the code). However, all of the City Names ($row['city']) were put into the database as ALL CAPITALS, which is a bit harder on the eyes to read. Is there a "PHP way" - working with the code I posted - that will transform the ALL CAPITALS to a more readable Capitalize (in other words, Capitalize just the first letter of each town). I know that CSS offers "text-transform" and if that is a solution I'm fine with that. I just need it to display that way, not re-write it in the database. Ideas? Solution? Thanks!
-
Better Password Protected Pages / Login Form .. ??
spacepoet replied to spacepoet's topic in PHP Coding Help
Hi: Thanks for the input. Is there an example / tutorial out there that you know of that will show this? I'm not sure I understand what you mean .. Haven't been having much luck with this .. Thanks for the help. -
Do not show emailed results if checkbox is empty .. ??
spacepoet replied to spacepoet's topic in PHP Coding Help
Hi: Do you have an example of what you mean? I'm not sure how to do this ... -
Hello: I wanted to see if I can make my password protected pages in my admin area, and the login form "more secure." I was told I should use MD5 / SALTING / HASHING to do this. I have tried some online tutorials, but am not understanding it, so I wanted to start from what I have and build upon it> This is my database table storing the myAdmins data (when I initially insert it into the database): CREATE TABLE `myAdmins` ( `id` int(4) NOT NULL auto_increment, `myUserName` varchar(65) NOT NULL default '', `myPassword` varchar(65) NOT NULL default '', PRIMARY KEY (`id`) ) ENGINE=MyISAM AUTO_INCREMENT=2 DEFAULT CHARSET=utf8; INSERT INTO myAdmins VALUES("1","abc","123"); This is the login form I use: <?php include('../include/myConn.php'); include('include/myAdminNav.php'); session_start(); session_destroy(); $message=""; $Login=$_POST['Login']; if($Login){ $myUserName=$_POST['myUserName']; $myPassword=$_POST['myPassword']; $result=mysql_query("select * from myAdmins where myUserName='$myUserName' and myPassword='$myPassword'"); if(mysql_num_rows($result)!='0'){ session_register("myUserName"); header("location:a_Home.php"); exit; }else{ $message="<div class=\"myAdminLoginError\">Incorrect Username or Password</div>"; } } ?> <form id="form1" name="form1" method="post" action="<? echo $PHP_SELF; ?>"> <? echo $message; ?> Username: <input name="myUserName" type="text" id="myUserName" size="40" /> Password: <input name="myPassword" type="password" id="myPassword" size="40" /> <input name="Login" type="submit" id="Login" value="Login" /> </form> This is the code on top of each page I password protect: <? session_start(); if(!session_is_registered(myUserName)){ header("location:Login.php"); } ?> Works well, but can it be "better"?? And, if I am allowing the admin to update his/her username or password, I do it this way: <?php include('../include/myConn.php'); include('include/myCheckLogin.php'); if ($_SERVER['REQUEST_METHOD'] == 'POST') { $myUserName = mysql_real_escape_string($_POST['myUserName']); $myPassword = mysql_real_escape_string($_POST['myPassword']); $sql = " UPDATE myAdmins SET myUserName = '$myUserName', myPassword = '$myPassword' "; mysql_query($sql) && mysql_affected_rows() ?> <?php } $query=mysql_query("SELECT * FROM myAdmins") or die("Could not get data from db: ".mysql_error()); while($result=mysql_fetch_array($query)) { $myUserName=$result['myUserName']; $myPassword=$result['myPassword']; } ?> <form method="post" action="<?php echo $PHP_SELF;?>"> <input type="hidden" name="POSTBACK" value="EDIT"> Username: <input type="text" size="60" maxlength="60" name="myUserName" value="<?php echo $myUserName; ?>"> Password: <input type="password" size="60" maxlength="60" name="myPassword" value="<?php echo $myPassword; ?>"> <input type="submit" value="Submit" /> </form> Should it be "better" .. ?? I don't seem to understand how to "encrypt" all of this to make it "stronger" .. Ideas? Improvements?
-
Hello: I'm trying to figure out how to NOT show emailed results if the checkbox is empty (not checked). I only want it included in the message of the email if the checkbox was checked. It is writing the data to the email properly, but it is including some of the code as well. This is my email code: mail( "me@digital.com", "Sign-up Sheet Request", "Date Sent: $myDate\n Parent's Name: $ParentsName Best Phone: $BestPhone Email: $Email Student's Name: $StudentsName Student's School: $StudentsSchool\n\n if(trim($SATTest1) != \"\" ) { echo \"$SATTest1\"; } ", "From: $Email" ); The "$SATTest1" data is displaying properly in the emails, but so is some of the code. Like this: if(trim(Reading and Math Classes for March 10) != "" ) { echo "Reading and Math Classes for March 10"; } Any idea how to correct this? Thanks!
-
Hi: Thanks! That looks much easier than I was making it out to be. I will play around with your code and see how I do. Thanks very much for the help!
-
Hi: Ah! Yes, this makes sense - I think I was overlooking the obvious a bit. OK, but what if I want to have the user be able to select items and attributes of items. I want to add a few SELECT dropdown menus to the products as well and send them in the email. Meaning if they are ordering T-Shirts - I want them to enter the amount, the color, and the size. And if they want to add pants to the order, let them chose the color, size, amount, and have all the data emailed as one email when they hit submit. Any ideas? This is why I thought they would need a SESSION or something like that. Thanks!
-
Hello: I want to create a feedback form that will allow a user to select from 30 or so items on a page, and send the data to an email address. I think it's something like a shopping cart but without a need to process a payment. I want to list items (like a soccer ball, a basket ball, etc.) and allow the user to select the size of the ball, the color, the number of balls, etc. I want the user to be able to select 1 item or many items. Once they are done, they would hit submit, and the data would get sent to an email address. Do I need to use a session to do this? I was trying to work with this code: <?php session_start(); session_register('product'); session_register('amount'); $_SESSION['product'] = $_POST['product']; $_SESSION['amount'] = $_POST['amount']; //SEND THE EMAIL CODE HERE ???? ?> <form method="post" action=""> <input type="text" size="10" name="product"><br /> <input type="text" size="10" name="amount"><br /> <input type="submit" value="Finish"> </form> But I'm pretty sure I'm not in the ballpark on how to do this. Anyone have any ideas? Thanks in advance.
-
OK, thanks for the help and for clarifying! Much appreciated.
-
Hi: Thanks for the reply, but it did not work. Speaking of variables, see something missing? Not sure what you mean ... what did I miss? The: <?php echo spLeftMenu(); ?> Works fine as far as displaying the DIVs/layout/Design on the "Index.php" page: <div id=\"sideEvents\"> echo \" $mySideBarData; \"; </div> I just can't get the data from the database (mySideBarData) to display. Any other ideas?
-
Hello: I wanted to see if someone can help me figure out how to include data from my included navigation file. Meaning: I have this file called myNav.php (the database table storing this is "myUpcomingEvents"): function spLeftMenu() { $spLeftMenu = " <div id=\"sideEvents\"> echo \" $mySideBarData; \"; </div> "; return $spLeftMenu; } I am trying to pull it into the Index.php page like this (the database table storing this is "myHome"): <?php include('include/myConn.php'); include('include/myNav.php'); $query=mysql_query("SELECT * FROM myHome,myUpcomingEvents") or die("Could not get data from db: ".mysql_error()); while($result=mysql_fetch_array($query)) { $myPageData=$result['myPageData']; $mySideBarPageData=$result['mySideBarPageData']; } ?> <!DOCTYPE HTML> <html> <head></head> <body> <div id="siteContainer"> <div id="leftColumn"> <?php echo spLeftMenu(); ?> </div> <div id="mainContent"> <?php echo $myPageData; ?> </div> </div> </body> </html> Can't get it to work. It just writes out: echo " ; "; Anyone know how I can get this to work? Thanks.