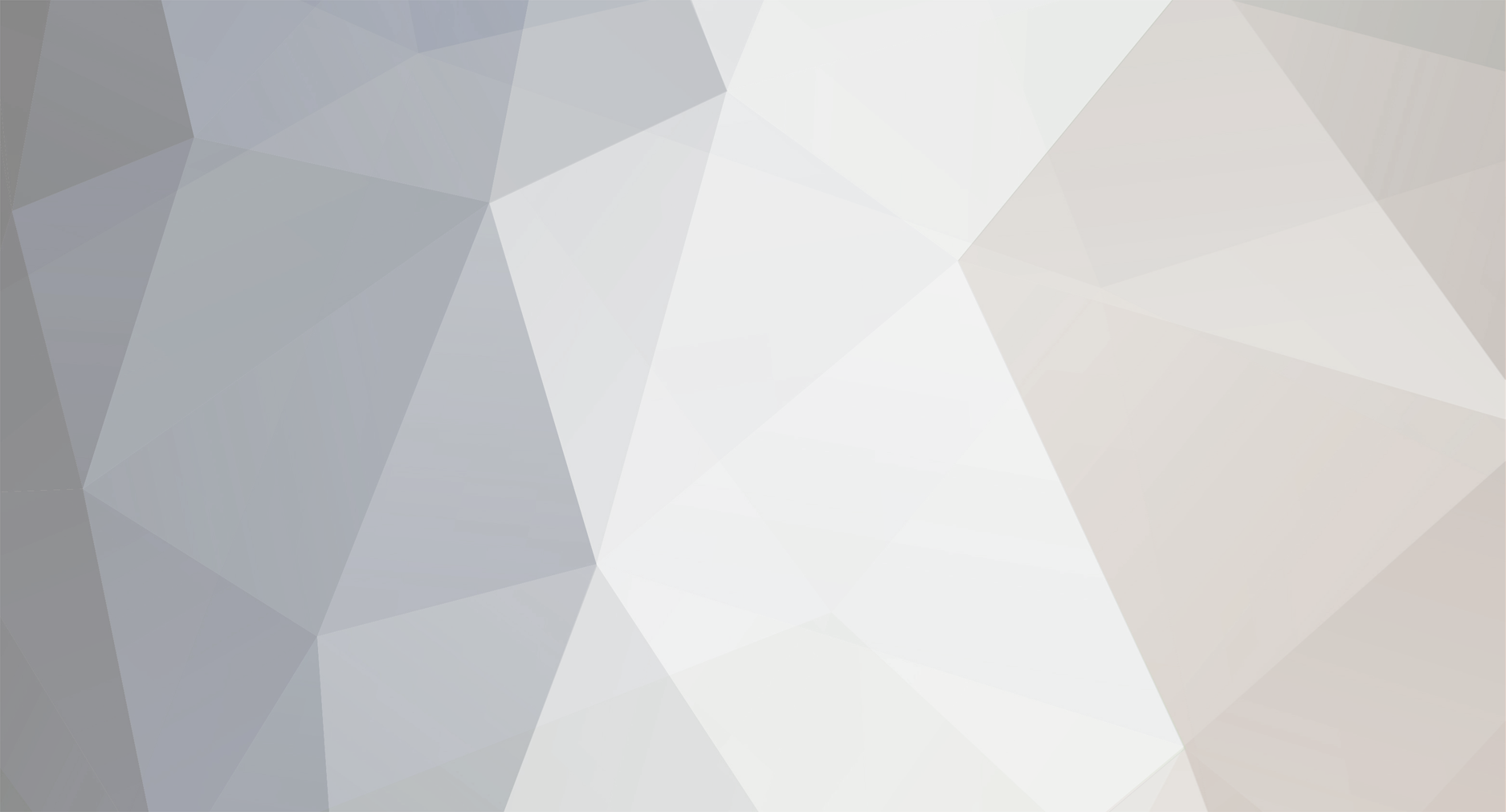
spacepoet
Members-
Posts
320 -
Joined
-
Last visited
Everything posted by spacepoet
-
Hi: Can someone help me understand how to fix my code below so that I can update it properly? The SELECT dropdown does not update, and I am at a loss about how to make this work. I basically trying to make a very simple product catalog to create Categories, and then add items to it: <?php include('../include/myConn.php'); $photo_id = $_REQUEST['photo_id']; if ($_SERVER['REQUEST_METHOD'] == 'POST') { $photo_id = mysql_real_escape_string($_POST['photo_id']); $photo_title = mysql_real_escape_string($_POST['photo_title']); $photo_price = mysql_real_escape_string($_POST['photo_price']); $photo_caption = mysql_real_escape_string($_POST['photo_caption']); $photo_category = mysql_real_escape_string($_POST['photo_category']); $sql = " UPDATE gallery_photos SET photo_id = '$photo_id', photo_title = '$photo_title', photo_price = '$photo_price', photo_caption = '$photo_caption', photo_category = '$photo_category' WHERE photo_id = $photo_id "; mysql_query($sql) && mysql_affected_rows() ?> <?php } $query=mysql_query("SELECT photo_id,photo_title,photo_price,photo_caption,photo_category FROM gallery_photos WHERE photo_id=$photo_id") or die("Could not get data from db: ".mysql_error()); while($result=mysql_fetch_array($query)) { $photo_id=$result['photo_id']; $photo_title=$result['photo_title']; $photo_price=$result['photo_price']; $photo_caption=$result['photo_caption']; $photo_category=$result['photo_category']; } ?> .... Product Category: $result = mysql_query( "SELECT category_id,category_name FROM gallery_category" ); echo "<select name='photo_category' size='1'>"; while( $row = mysql_fetch_array( $result ) ) { echo "<option value=\"".$row['category_id']."\">".$row['category_name']."</option>"; } echo "</select>"; Title: <input type="text" name="photo_title" size="45" maxlength="200" value="<?php echo $photo_title; ?>" /> Price: <input type="text" name="photo_price" size="45" maxlength="200" value="<?php echo $photo_price; ?>" /> Description: <textarea cols="107" rows="1" name="photo_caption"><?php echo $photo_caption; ?></textarea> These are the database tables: -- -- Table structure for table `gallery_category` -- CREATE TABLE `gallery_category` ( `category_id` bigint(20) unsigned NOT NULL auto_increment, `category_name` varchar(50) NOT NULL default '0', PRIMARY KEY (`category_id`), KEY `category_id` (`category_id`) ) ENGINE=MyISAM AUTO_INCREMENT=2 DEFAULT CHARSET=latin1 AUTO_INCREMENT=2 ; -- -- Dumping data for table `gallery_category` -- INSERT INTO `gallery_category` VALUES (1, 'My First Gallery'); -- -------------------------------------------------------- -- -- Table structure for table `gallery_photos` -- CREATE TABLE `gallery_photos` ( `photo_id` bigint(20) unsigned NOT NULL auto_increment, `listorder` int(11) default NULL, `photo_filename` varchar(25) default NULL, `photo_title` varchar(255) default NULL, `photo_price` varchar(255) default NULL, `photo_caption` text, `photo_category` bigint(20) unsigned NOT NULL default '0', PRIMARY KEY (`photo_id`), KEY `photo_id` (`photo_id`) ) ENGINE=MyISAM AUTO_INCREMENT=105 DEFAULT CHARSET=latin1 AUTO_INCREMENT=105 ; -- -- Dumping data for table `gallery_photos` -- INSERT INTO `gallery_photos` VALUES (97, NULL, '97.jpg', '', 1); INSERT INTO `gallery_photos` VALUES (99, NULL, '99.jpg', '', 1); INSERT INTO `gallery_photos` VALUES (100, NULL, '100.jpg', '', 1); INSERT INTO `gallery_photos` VALUES (101, NULL, '101.jpg', '', 1); INSERT INTO `gallery_photos` VALUES (102, NULL, '102.jpg', '', 1); INSERT INTO `gallery_photos` VALUES (103, NULL, '103.jpg', '', 1); INSERT INTO `gallery_photos` VALUES (104, NULL, '104.jpg', '', 1); Or, if this is not "fixable" the way I am doing it, is there a tutorial or a example someone can post that will show me how to do this? I just want to be able to make Categories, and then add a Photo with title, price, and decsription to it. And of course be able to edit or delete the Categories and/or Items. The examples I'm finding via GOOGLE are too much - I just want something simple. Anyone?
-
Pulling and updating data from a seperate DB Table
spacepoet replied to spacepoet's topic in PHP Coding Help
Hi: No, I know that isn't it. These are the 2 tables: gallery_category -- -- Table structure for table `gallery_category` -- CREATE TABLE `gallery_category` ( `category_id` bigint(20) unsigned NOT NULL auto_increment, `category_name` varchar(50) NOT NULL default '0', PRIMARY KEY (`category_id`), KEY `category_id` (`category_id`) ) ENGINE=MyISAM AUTO_INCREMENT=2 DEFAULT CHARSET=latin1 AUTO_INCREMENT=2 ; -- -- Dumping data for table `gallery_category` -- INSERT INTO `gallery_category` VALUES (1, 'My First Gallery'); gallery_photos -- -- Table structure for table `gallery_photos` -- CREATE TABLE `gallery_photos` ( `photo_id` bigint(20) unsigned NOT NULL auto_increment, `listorder` int(11) default NULL, `photo_filename` varchar(25) default NULL, `photo_title` varchar(255) default NULL, `photo_price` varchar(255) default NULL, `photo_caption` text, `photo_category` bigint(20) unsigned NOT NULL default '0', PRIMARY KEY (`photo_id`), KEY `photo_id` (`photo_id`) ) ENGINE=MyISAM AUTO_INCREMENT=105 DEFAULT CHARSET=latin1 AUTO_INCREMENT=105 ; -- -- Dumping data for table `gallery_photos` -- INSERT INTO `gallery_photos` VALUES (97, NULL, '97.jpg', '', 1); Where "category_id" is the same number as "photo_category" The "photo_category" number is created/selected from the "category_id" number when first creating the new record (a different page). I'm now trying to get the EDIT page to work properly - just stumped on this last part. Does that help? -
Pulling and updating data from a seperate DB Table
spacepoet replied to spacepoet's topic in PHP Coding Help
No, tried that but it's still empty (value="") .. Can I do it where I use the "category_id" (like I just posted) since it works properly, but somehow change the UPDATE code to make the "category_id" equal the "photo_category": <?php $photo_id = $_REQUEST['photo_id']; if ($_SERVER['REQUEST_METHOD'] == 'POST') { $photo_id = mysql_real_escape_string($_POST['photo_id']); $photo_title = mysql_real_escape_string($_POST['photo_title']); $photo_price = mysql_real_escape_string($_POST['photo_price']); $photo_caption = mysql_real_escape_string($_POST['photo_caption']); $photo_category = mysql_real_escape_string($_POST['photo_category']); $sql = " UPDATE gallery_photos SET photo_id = '$photo_id', photo_title = '$photo_title', photo_price = '$photo_price', photo_caption = '$photo_caption', photo_category = '$photo_category' WHERE photo_id = $photo_id "; mysql_query($sql) && mysql_affected_rows() ?> <?php } $query=mysql_query("SELECT photo_id,photo_title,photo_price,photo_caption,photo_category FROM gallery_photos WHERE photo_id=$photo_id") or die("Could not get data from db: ".mysql_error()); while($result=mysql_fetch_array($query)) { $photo_id=$result['photo_id']; $photo_title=$result['photo_title']; $photo_price=$result['photo_price']; $photo_caption=$result['photo_caption']; $photo_category=$result['photo_category']; } ?> ... <form method="post" action="<?php echo $PHP_SELF;?>"> <? $result = mysql_query( "SELECT category_id,category_name FROM gallery_category" ); echo "<select name='photo_category' size='1'>"; while( $row = mysql_fetch_array( $result ) ) { echo "<option value=\"".$row['category_id']."\">".$row['category_name']."</option>"; } echo "</select>"; ?> </form> Somehow change the UPDATE SQL to do that? Would that work? Sorry, I know I'm being a pain but this is the last part and it's driving me nuts! -
Pulling and updating data from a seperate DB Table
spacepoet replied to spacepoet's topic in PHP Coding Help
Hi: This works to display the dropdown properly. Thank you. But, I still can not get the dropdown to update properly. "photo_category" is a number that is used to determine what CATEGORY an item should be assigned to. Looking at the current code, I think that "category_id" should be "photo_category": <? $result = mysql_query( "SELECT category_id,category_name FROM gallery_category" ); echo "<select name='photo_category' size='1'>"; while( $row = mysql_fetch_array( $result ) ){ //echo "<option value=\"".$row['photo_category']."\">".$row['category_name']."</option>"; echo "<option value=\"$photo_category\">".$row['category_name']."</option>"; } echo "</select>"; ?> When I do that, here is no number displayed in the source code: <select name='photo_category' size='1'> <option value="0">CATEGORY 2</option> <option value="0">CATEGORY 1</option> </select> However, when I am inserting the item, the dropdown populates fine: <select name='category'> <option value="39">CATEGORY 2</option> <option value="38">CATEGORY 1</option> </select> So, I seem to be missing one last thing to get this to work. Am I missing some type of syntax to get the values to display properly? Thanks for your help on this! -
Pulling and updating data from a seperate DB Table
spacepoet replied to spacepoet's topic in PHP Coding Help
I'm still not getting it ... I'm trying to do it this way: <? $result = mysql_query( "SELECT category_id,category_name FROM gallery_category" ); while( $row = mysql_fetch_array( $result ) ) { echo "<select name='photo_category' size='1'>"; while( $row = mysql_fetch_array( $result ) ) { echo "<option value=\"".$row['category_id']."\">".$row['category_name']."</option>"; } echo "</select>"; } ?> to pull the category names from the gallery_category TABLE and be able to update the category name an item is assigned to. Can it be done this way? I thought it could, but I somehow need to JOIN the two TABLES, which is where I am rather lost. Everything works fine with the original code I posted, but I can't figure out how to get this last part to work correctly. Ideas .. ?? -
Pulling and updating data from a seperate DB Table
spacepoet replied to spacepoet's topic in PHP Coding Help
Hi: Thanks for pointing me in the right direction .. still not getting it to work .. Everything is OK, except for populating the VALUES in the OPTION. ... <?php } $query=mysql_query("SELECT photo_title,photo_price,photo_caption,photo_category FROM gallery_photos") or die("Could not get data from db: ".mysql_error()); while($result=mysql_fetch_array($query)) { $photo_title=$result['photo_title']; $photo_price=$result['photo_price']; $photo_caption=$result['photo_caption']; $photo_category=$result['photo_category']; } ?> ... ... <? echo "<select name='photo_category' size='1'>"; while( $row = mysql_fetch_array( $result ) ) { echo "<option value=\"$row\">$row</option>"; } echo "</select>"; ?> ... I'm getting this error: <b>Warning</b>: mysql_fetch_array(): supplied argument is not a valid MySQL result resource in <b>...admin/a_Photo_Edit2.php</b> on line <b>112</b><br /> What am I missing ?? -
Hello: I have a DB table with this structure: CREATE TABLE `gallery_category` ( `category_id` bigint(20) unsigned NOT NULL auto_increment, `category_name` varchar(50) NOT NULL default '0', PRIMARY KEY (`category_id`), KEY `category_id` (`category_id`) ) ENGINE=MyISAM AUTO_INCREMENT=2 DEFAULT CHARSET=latin1 AUTO_INCREMENT=2 ; What I am trying to do id pull this data into my "Edit Product" page and populate a SELECT menu with it. I want the user to be able to re-assign a product to a new category if they chose to do so. I want to add the data (and update the DB) to this area: <div style="float: left; width: 550px;"> <select name='category_name'> <option></option> </select> </div> This is the full page code that allows users to update product info: <?php include('../include/myConn.php'); include('../include/myCodeLib.php'); include('include/myCheckLogin.php'); include('include/myAdminNav.php'); include('ckfinder/ckfinder.php'); include('ckeditor/ckeditor.php'); $photo_id = $_REQUEST['photo_id']; if ($_SERVER['REQUEST_METHOD'] == 'POST') { $photo_title = mysql_real_escape_string($_POST['photo_title']); $photo_price = mysql_real_escape_string($_POST['photo_price']); $photo_caption = mysql_real_escape_string($_POST['photo_caption']); $sql = " UPDATE gallery_photos SET photo_title = '$photo_title', photo_price = '$photo_price', photo_caption = '$photo_caption' WHERE photo_id = $photo_id "; mysql_query($sql) && mysql_affected_rows() ?> <?php } $query=mysql_query("SELECT photo_title,photo_price,photo_caption FROM gallery_photos") or die("Could not get data from db: ".mysql_error()); while($result=mysql_fetch_array($query)) { $photo_title=$result['photo_title']; $photo_price=$result['photo_price']; $photo_caption=$result['photo_caption']; } ?> <!DOCTYPE HTML> <html> <head> </head> <body> <div id="siteContainer"> <p> <?php if ($_SERVER['REQUEST_METHOD'] == 'POST') echo "<span class=\"textError\">". $photo_title ." successfully updated!</span>" ?> </p> <p> <form method="post" action="<?php echo $PHP_SELF;?>"> <input type="hidden" name="POSTBACK" value="EDIT"> <input type='hidden' name='photo_id' value='<?php echo $photo_id; ?>' /> <div style="float: left; width: 120px; margin-right: 30px;"> Category: </div> <div style="float: left; width: 550px;"> <select name='category_name'> <option></option> </select> </div> <div style="float: left; width: 120px; margin-right: 30px;"> Title: </div> <div style="float: left; width: 550px;"> <input type="text" name="photo_title" size="45" maxlength="200" value="<?php echo $photo_title; ?>" /> </div> <div style="clear: both;"><br /></div> <div style="float: left; width: 120px; margin-right: 30px;"> Price: </div> <div style="float: left; width: 550px;"> <input type="text" name="photo_price" size="45" maxlength="200" value="<?php echo $photo_price; ?>" /> </div> <div style="clear: both;"><br /></div> Description:<br /> <textarea cols="107" rows="1" name="photo_caption"><?php echo $photo_caption; ?></textarea> <div style="clear: both;"><br /></div> <br /> <input type="submit" value="Submit" /> </form> </p> </div> </body> </html> How can I do this? I'm stumped ... Thanks!
-
Hi: Yes, I want them to be 690px wide, but to keep the height in proportion. Example: if the raw image is 1380px wide X 900px high, I want it to scale to 690px X 450px high, based on setting the width only. Or, if the next image is 1500px wide X 1300px high, I want it to scale to 690px X 650px high, based on setting the width only. I think it needs to happen here: $size = GetImageSize( $images_dir."/".$filename ); if($size[0] > $size[1]) //{ //$thumbnail_width = 100; //$thumbnail_height = (int)(100 * $size[1] / $size[0]); //} //else //{ //$thumbnail_width = (int)(100 * $size[0] / $size[1]); //$thumbnail_height = 100; //} { //$thumbnail_width = 690; //$thumbnail_height = (int)(500 * $size[1] / $size[0]); $old_width = $size[0]; $old_height = $size[1]; $thumbnail_width = 690; $thumbnail_height = ($old_height * $thumbnail_width / $old_width); } else { $thumbnail_width = (int)(690 * $size[0] / $size[1]); $thumbnail_height = 500; } Any ideas on how to revise the above code and get this to work? Thanks much.
-
Hello: Hoping someone can help me figure this out... I am resizing a photo/thumbnails with GD (I think that's the correct term) upon uploading. Works fine but the one thing I can't figure out is how to scale the height proportionate with the width. Meaning, I have the width set to 690px, and the height set to 500px. It is making the images look squished. What I would like to do is have a set width (like 690px), but have the height scale down proportionalety. Or, can I just remove the height (although I tried that and it did not work). This is the code: // Let's get the Thumbnail size $size = GetImageSize( $images_dir."/".$filename ); if($size[0] > $size[1]) //{ //$thumbnail_width = 100; //$thumbnail_height = (int)(100 * $size[1] / $size[0]); //} //else //{ //$thumbnail_width = (int)(100 * $size[0] / $size[1]); //$thumbnail_height = 100; //} { //$thumbnail_width = 690; //$thumbnail_height = (int)(500 * $size[1] / $size[0]); $old_width = $size[0]; $old_height = $size[1]; $thumbnail_width = 690; $thumbnail_height = ($old_height * $thumbnail_width / $old_width); } else { $thumbnail_width = (int)(690 * $size[0] / $size[1]); $thumbnail_height = 500; } // Build Thumbnail with GD 1.x.x, you can use the other described methods too $function_suffix = $gd_function_suffix[$filetype]; $function_to_read = "ImageCreateFrom".$function_suffix; $function_to_write = "Image".$function_suffix; // Read the source file $source_handle = $function_to_read ( $images_dir."/".$filename ); if($source_handle) { // Let's create an blank image for the thumbnail //$destination_handle = ImageCreate ( $thumbnail_width, $thumbnail_height ); $destination_handle = imagecreatetruecolor( $thumbnail_width, $thumbnail_height ); // Now we resize it ImageCopyResized( $destination_handle, $source_handle, 0, 0, 0, 0, $thumbnail_width, $thumbnail_height, $size[0], $size[1] ); } // Let's save the thumbnail $function_to_write( $destination_handle, $images_dir."/tb_".$filename ); ImageDestroy($destination_handle ); // $result_final .= "<img src='".$images_dir. "/tb_".$filename."' style='margin-right: 20px; width: 100px;' />"; Anyone have any ideas on this? Thanks much.
-
Hello: Can someone give me some insight on how to be able to not just add (INSERT) a photo and photo description with the following code, but also how to add the ability to UPDATE (if the user wants to replace a photo or adjust the photo description): <?php include("config.inc.php"); // initialization $result_final = ""; $counter = 0; // List of our known photo types $known_photo_types = array( 'image/pjpeg' => 'jpg', 'image/jpeg' => 'jpg', 'image/gif' => 'gif', 'image/bmp' => 'bmp', 'image/x-png' => 'png' ); // GD Function List $gd_function_suffix = array( 'image/pjpeg' => 'JPEG', 'image/jpeg' => 'JPEG', 'image/gif' => 'GIF', 'image/bmp' => 'WBMP', 'image/x-png' => 'PNG' ); // Fetch the photo array sent by preupload.php $photos_uploaded = $_FILES['photo_filename']; // Fetch the photo caption array $photo_caption = $_POST['photo_caption']; while( $counter <= count($photos_uploaded) ) { if($photos_uploaded['size'][$counter] > 0) { if(!array_key_exists($photos_uploaded['type'][$counter], $known_photo_types)) { $result_final .= "File ".($counter+1)." is not a photo<br />"; } else { mysql_query( "INSERT INTO gallery_photos(`photo_filename`, `photo_caption`, `photo_category`) VALUES('0', '".addslashes($photo_caption[$counter])."', '".addslashes($_POST['category'])."')" ); $new_id = mysql_insert_id(); $filetype = $photos_uploaded['type'][$counter]; $extention = $known_photo_types[$filetype]; $filename = $new_id.".".$extention; mysql_query( "UPDATE gallery_photos SET photo_filename='".addslashes($filename)."' WHERE photo_id='".addslashes($new_id)."'" ); // Store the orignal file copy($photos_uploaded['tmp_name'][$counter], $images_dir."/".$filename); // Let's get the Thumbnail size $size = GetImageSize( $images_dir."/".$filename ); if($size[0] > $size[1]) { $thumbnail_width = 100; $thumbnail_height = (int)(100 * $size[1] / $size[0]); } else { $thumbnail_width = (int)(100 * $size[0] / $size[1]); $thumbnail_height = 100; } // Build Thumbnail with GD 1.x.x, you can use the other described methods too $function_suffix = $gd_function_suffix[$filetype]; $function_to_read = "ImageCreateFrom".$function_suffix; $function_to_write = "Image".$function_suffix; // Read the source file $source_handle = $function_to_read ( $images_dir."/".$filename ); if($source_handle) { // Let's create an blank image for the thumbnail $destination_handle = ImageCreate ( $thumbnail_width, $thumbnail_height ); // Now we resize it ImageCopyResized( $destination_handle, $source_handle, 0, 0, 0, 0, $thumbnail_width, $thumbnail_height, $size[0], $size[1] ); } // Let's save the thumbnail $function_to_write( $destination_handle, $images_dir."/tb_".$filename ); ImageDestroy($destination_handle ); // $result_final .= "<img src='".$images_dir. "/tb_".$filename."' /> File ".($counter+1)." Added<br />"; } } $counter++; } // Print Result echo <<<__HTML_END <html> <head> <title>Photos uploaded</title> </head> <body> $result_final </body> </html> __HTML_END; ?> The tutorial says to edit a photo, simply add: function edit_photo( $photo_id, $new_caption, $new_category ) <br> { <br> mysql_query( "UPDATE gallery_photo SET photo_caption='".addslashes( $new_caption )."', photo_category='".addslashes( $new_category )."' WHERE photo_id='".addslashes( $photo_id )."'" ); <br> } But I can not figure out how to call that function. Anyone help me out? Thanks.
-
Hello: I am trying to figure out how to do an UPDATE SET for a photo gallery based on the "photo_id" ... not working to well ... Currently, it just uploads a photo but updates everything. This is the code: Photo_Edit.php <?php $photo_id = $_REQUEST['photo_id']; ?> ... <?php $query=mysql_query("SELECT * FROM gallery_photos WHERE photo_id = $photo_id") or die("Could not get data from db: ".mysql_error()); while($result=mysql_fetch_array($query)) { $photo_id=$result['photo_id']; $photo_filename=$result['photo_filename']; $photo_caption=$result['photo_caption']; } ?> <form enctype="multipart/form-data" action="a_Photo_Upload2.php" method="post" name="upload_form"> <input type='hidden' name='photo_id' value='<?php echo $photo_id; ?>' /> <table width='600' border='0' id='tablepadding' align='center'> <tr> <td width='50'> Photo: <td width='550'> <input name='photo_filename[]' id='my_photo' type='file' /> <div style='clear: both;'></div> Current photo: <div style='clear: both;'></div> <img src="../gallery/tb_<?php echo $photo_filename; ?>" width="100px" class='imgCenter' /> <div style='clear: both;'></div> <a href="javascript:void(0);" onmouseover="tooltip.show('<img src=../gallery/tb_<?php echo $photo_filename; ?> width=500 />');" onmouseout="tooltip.hide();">Larger view</a> </td> </tr> <tr> <td> Description: </td> <td> <textarea name='photo_caption[]' cols='100' rows='10'><?php echo $photo_caption; ?></textarea> </td> </tr> <tr> <td> <input type='submit' name='submit' value='Edit Product' /> </td> </tr> </table> </form> Goes to this page, where I am having the problems: a_Photo_Upload2.php <?php echo $photo_id; ?> <?php // initialization $result_final = ""; $counter = 0; // List of our known photo types $known_photo_types = array( 'image/pjpeg' => 'jpg', 'image/jpeg' => 'jpg', 'image/gif' => 'gif', 'image/bmp' => 'bmp', 'image/x-png' => 'png' ); // GD Function List $gd_function_suffix = array( 'image/pjpeg' => 'JPEG', 'image/jpeg' => 'JPEG', 'image/gif' => 'GIF', 'image/bmp' => 'WBMP', 'image/x-png' => 'PNG' ); // Fetch the photo array sent by preupload.php $photos_uploaded = $_FILES['photo_filename']; // Fetch the photo caption array $photo_caption = $_POST['photo_caption']; while( $counter <= count($photos_uploaded) ) { if($photos_uploaded['size'][$counter] > 0) { if(!array_key_exists($photos_uploaded['type'][$counter], $known_photo_types)) { $result_final .= "File ".($counter+1)." is not a photo<br />"; } else { $new_id = mysql_insert_id(); $filetype = $photos_uploaded['type'][$counter]; $extention = $known_photo_types[$filetype]; $filename = $new_id.".".$extention; mysql_query( "UPDATE gallery_photos SET photo_filename='".addslashes($filename)."' WHERE photo_id='".addslashes($new_id)."'" ); // Store the orignal file copy($photos_uploaded['tmp_name'][$counter], $images_dir."/".$filename); // Let's get the Thumbnail size $size = GetImageSize( $images_dir."/".$filename ); if($size[0] > $size[1]) //{ //$thumbnail_width = 100; //$thumbnail_height = (int)(100 * $size[1] / $size[0]); //} //else //{ //$thumbnail_width = (int)(100 * $size[0] / $size[1]); //$thumbnail_height = 100; //} { //$thumbnail_width = 690; //$thumbnail_height = (int)(500 * $size[1] / $size[0]); $old_width = $size[0]; $old_height = $size[1]; $thumbnail_width = 690; $thumbnail_height = ($old_height * $thumbnail_width / $old_width); } else { $thumbnail_width = (int)(690 * $size[0] / $size[1]); $thumbnail_height = 500; } // Build Thumbnail with GD 1.x.x, you can use the other described methods too $function_suffix = $gd_function_suffix[$filetype]; $function_to_read = "ImageCreateFrom".$function_suffix; $function_to_write = "Image".$function_suffix; // Read the source file $source_handle = $function_to_read ( $images_dir."/".$filename ); if($source_handle) { // Let's create an blank image for the thumbnail //$destination_handle = ImageCreate ( $thumbnail_width, $thumbnail_height ); $destination_handle = imagecreatetruecolor( $thumbnail_width, $thumbnail_height ); // Now we resize it ImageCopyResized( $destination_handle, $source_handle, 0, 0, 0, 0, $thumbnail_width, $thumbnail_height, $size[0], $size[1] ); } // Let's save the thumbnail $function_to_write( $destination_handle, $images_dir."/tb_".$filename ); ImageDestroy($destination_handle ); // $result_final .= "<img src='".$images_dir. "/tb_".$filename."' style='margin-right: 20px; width: 100px;' />"; } } $counter++; } // Print Result echo <<<__HTML_END $result_final __HTML_END; ?> Can anyone show me how to make this work, OR show me a way to do this ... Thank you.
-
Hello: I Need help to see if I can do an inline JavaScript code that will disable the Submit button and write a little message *AND* that works in all browsers. For this project, it has to be inline JavaScript. I have the below code working very well, except it does not work in FireFox. <input type='submit' value='Submit' onclick="this.disabled=true; this.value='Results Submitted!';" /> Anyone know if I can get it to work in all browers, and still keep it inline?? Thanks!
-
How can I display this from an included file ??
spacepoet replied to spacepoet's topic in PHP Coding Help
Well, tried adding that to the php.ini file: ... display_errors = ON error_reporting = E_ALL ... But, it's doing the same thing ... Thanks for looking at it for me .. I guess I need to just add it to the code on each page .. need to finish this up. -
How can I display this from an included file ??
spacepoet replied to spacepoet's topic in PHP Coding Help
Hi: Let me try the error reporting .. I assume in the php.ini file? Sorry, I'm making the switch from old ASP to PHP and still trying to learn a lot of the tricks of the trade. A bit frustrated cause I didn't think it would be so hard to get this to show up! -
How can I display this from an included file ??
spacepoet replied to spacepoet's topic in PHP Coding Help
Here's where I'm confused: if I put it in the page like this: <?php echo $mySideBarPageData; ?> It works fine. When I try to pull it in with an included file, it doesn't work. And I'm stuck trying to understand why it does not work. -
How can I display this from an included file ??
spacepoet replied to spacepoet's topic in PHP Coding Help
Not sure I understand ... This: <p> <div id=\"myLeftNavPaper\"> <img src=\"images/sidePaperTop.png\" alt=\"\" /> <div id=\"myLeftNavPaper2\"> echo ". ('$mySideBarPageData;') ." </div> <img src=\"images/sidePaperBottom.png\" alt=\"\" /> </div> </p> is not working, either ... -
Hello: I have this code in an included file: myNav.php function spLeftMenu() { $spLeftMenu = " <p> <div id=\"myLeftNavPaper\"> <img src=\"images/sidePaperTop.png\" alt=\"\" /> <div id=\"myLeftNavPaper2\"> echo \". $mySideBarPageData .\" </div> <img src=\"images/sidePaperBottom.png\" alt=\"\" /> </div> </p> "; return $spLeftMenu; } I can not get: echo \". $mySideBarPageData .\" To display the results on this page: Page.php <html> ... <?php echo spLeftMenu(); ?> ... </html> What am I missing ??
-
Exporting mySQL data as a .TXT or .SQL file for backup
spacepoet replied to spacepoet's topic in MySQL Help
Hi: No, I tried it that was as well .. Don't know what to do .. -
Exporting mySQL data as a .TXT or .SQL file for backup
spacepoet replied to spacepoet's topic in MySQL Help
Hi: Maybe I am not understanding it properly .. Do I need to do soemthing like this: <?php include('../include/myConn.php'); $table_dump = mysql_query("SELECT * INTO OUTFILE 'DataBaseBackUp.txt'") or die("unable to move data"); while($result=mysql_fetch_array($query)) echo ". $result()."; ?> Just using the one line produced "unable to move data" .. As does the above code ... Do I somehow need to write it out into the file? -
Exporting mySQL data as a .TXT or .SQL file for backup
spacepoet replied to spacepoet's topic in MySQL Help
OK, I see what you mean .. but what if I want to do all the tables, not just one. Meaning, I want to export the whole database as one file. Also, is there a trick to add the date and time to each file, so it can be easy to find by date and time. Thanks very much! -
Exporting mySQL data as a .TXT or .SQL file for backup
spacepoet replied to spacepoet's topic in MySQL Help
Hi: Thanks for the reply, but I'm not sure what you mean. Something like: Export.php <?php SELECT myDataBase INTO OUTFILE 'file_name' ?> Admin.php <a href="Export.php">Export Database</a> ?? Sorry, a little confused .. -
Hello: I have a question and I think this is where to ask it. I want to add a feature in my admin panel that is a link that - when click - will export all the data from a mySQL database as a .TXT or .SQL (preferred) and open it in a "Save As" prompt box so the admin can save a copy to his or her computer. Basically, I want the admin to download a copy of the database each time they make an update so he or she has a current copy of the database stored as a backup on his or her local machine. Can this be done? If so, how? My GOOGLE searches have not produced any results for this. Thanks.
-
Excellent! Got it: <?php $query = mysql_query("SELECT menuID,menuTitle FROM theOfficeMenus"); if(mysql_num_rows($query) == 0) { echo "<span class=\"myBold\">Currently there are no Menus</span>"; } else { echo "<span class=\"myBold\">Current Menus</span>"; } while($menuData = mysql_fetch_array($query)) { echo "<li style=\"margin: 0 0 20px 0;\"> <a href=\"a_MenusDelete.php?menuID=".$menuData['menuID']."\" onclick=\"return confirm('Are you sure you want to delete this Menu? There is NO undo! Click OK to continue deleting, or CANCEL to stop deleting.');\">Delete Menu</a> || <a href=\"a_MenusEdit.php?menuID=".$menuData['menuID']."\"><span class=\"myBold\">". $menuData['menuTitle'] ."</span></a></li>"; } ?> Thanks for the tip!
-
Hi: Thanks for all the tips. For doing a "setcookie("TestCookie", $value, time()+1200);" Is that something I add to the myCheckLogin.php page? Just like that? Not to much experience with cookies but want to learn more. Thanks!
-
Hello: I am using this code to try and display one of two statements: <?php $query = mysql_query("SELECT menuID,menuTitle FROM theOfficeMenus"); if(trim($menuData["menuTitle"]) == "") { echo "<span class=\"myBold\">Currently there are no Menus</span>"; } else { echo "<span class=\"myBold\">Current Menus</span>"; } while($menuData = mysql_fetch_array($query)) { echo "<li style=\"margin: 0 0 20px 0;\"> <a href=\"a_MenusDelete.php?menuID=".$menuData['menuID']."\" onclick=\"return confirm('Are you sure you want to delete this Menu? There is NO undo! Click OK to continue deleting, or CANCEL to stop deleting.');\">Delete Menu</a> || <a href=\"a_MenusEdit.php?menuID=".$menuData['menuID']."\"><span class=\"myBold\">". $menuData['menuTitle'] ."</span></a></li>"; } ?> If "menuTitle" is empty display "Currently there are no Menus" , if it is not empty display "Current Menus" However ever, it keeps displaying only the first statement. Why ??