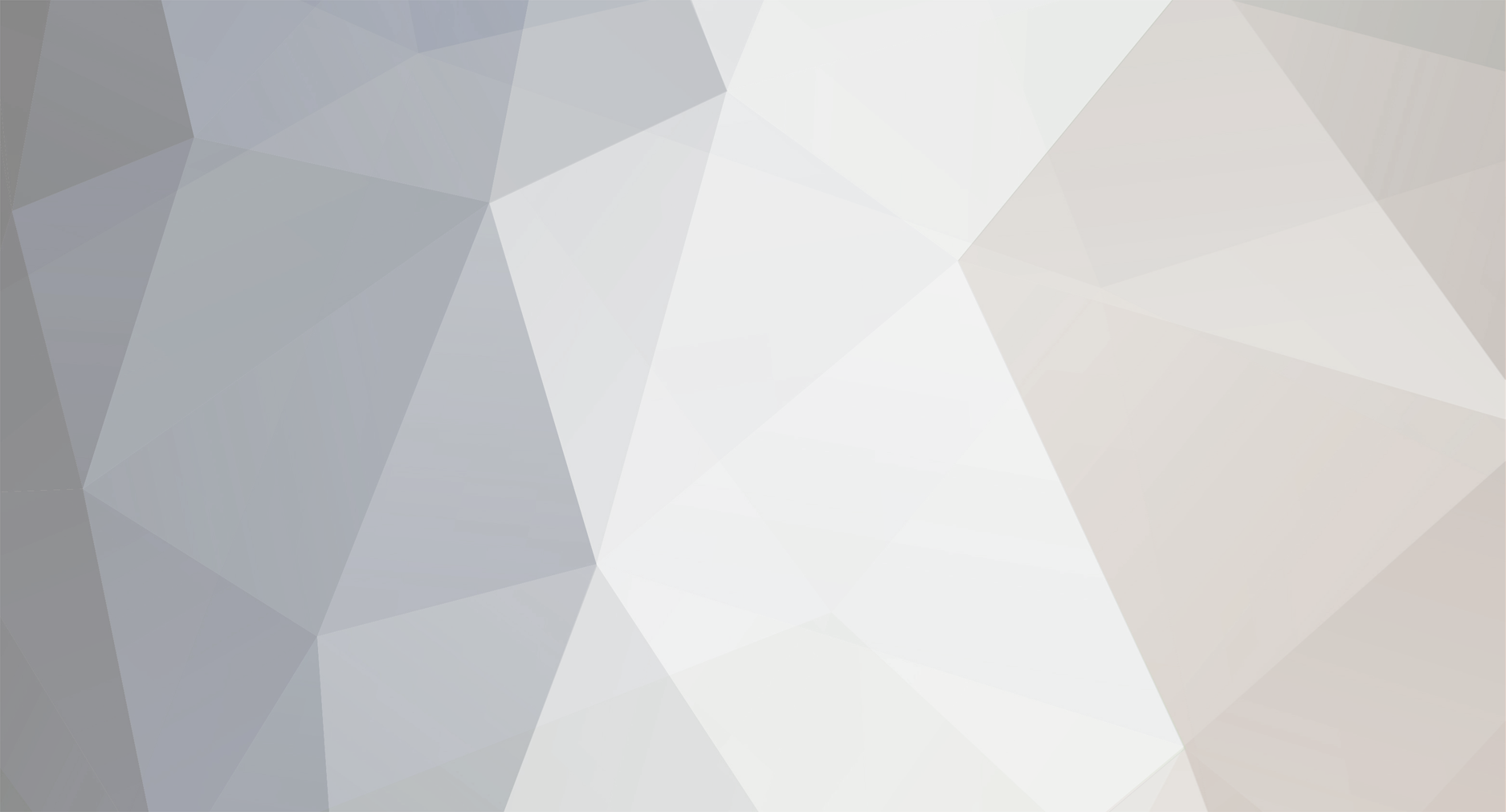
QuickOldCar
Staff Alumni-
Posts
2,972 -
Joined
-
Last visited
-
Days Won
28
Everything posted by QuickOldCar
-
There is a few ways to go about getting the data from your own database, have to look around the one for your csv you are using. It's claimed some queries aren't that efficient and some others are better. If you use it locally is no need to pay for their service. With the external api's to the maxmind site you do, that's because they are always getting updated or improved upon using their database.
-
I agree, and along the way each category will have to be added to an array and used, unless will always be the same category all uploaded images. Should also only insert if the array is not empty if(!empty($insertValues)){ $sql="INSERT INTO galerija_slike (kategorija_id, url_slike) VALUES " . implode(',', $insertValues); }
-
You can move your mysql query within the foreach loop <?PHP include "config.php"; $uploads_dir = 'slike'; foreach ($_FILES["uploaded_file"]["error"] as $key => $error) { if ($error == UPLOAD_ERR_OK) { $tmp_name = $_FILES["uploaded_file"]["tmp_name"][$key]; $name = $_FILES["uploaded_file"]["name"][$key]; move_uploaded_file($tmp_name, "$uploads_dir/$name"); $sql="INSERT INTO galerija_slike (kategorija_id, url_slike) VALUES ('".$_POST['kategorija']."', '".$_FILES['uploaded_file']['name'][$key]."')"; if (mysql_query($sql)) { echo "success"; } else { echo "error" . mysql_error(); } } } ?>
-
Change output of a PHP CLI file add commas?
QuickOldCar replied to chadrt's topic in PHP Coding Help
http://php.net/manual/en/function.number-format.php -
This should be able to get you their ip $ip = getenv('HTTP_CLIENT_IP')?: getenv('HTTP_X_FORWARDED_FOR')?: getenv('HTTP_X_FORWARDED')?: getenv('HTTP_FORWARDED_FOR')?: getenv('HTTP_FORWARDED')?: getenv('REMOTE_ADDR'); if (strstr($ip, ', ')) { $ips = explode(', ', $ip); $ip = $ips[0]; } They have documentation on the maxmind site. http://dev.maxmind.com/geoip/legacy/csv/ The api from github may be of use to you https://github.com/maxmind/geoip-api-php I may as well mention this http://php.net/manual/en/ref.geoip.php
-
It's a csv file not cvs Use load data infile and import to a mysql database or follow this tutorial how to import those exact 2 files
-
One thing I did notice is you are using both GET and POST for this All your variables could be held all in a GET request and are an array which http_build_query() could use A trick here is to unset $_GET['page'] from the array and make it what you desire. Besides the approach here building arrays, you could also unset get and manually append either page=* or &page=* to the ends, but I feel letting http_build_query do it is a cleaner approach. A demo for you to try minus your actual queries. <?php $total_pages = 40; //dummy data if (empty($_GET) || !isset($_GET['page']) || !ctype_digit(trim($_GET['page'])) || trim($_GET['page']) <= 0) { $_GET['page'] = 1; $current_page = 1; } else { $current_page = trim($_GET['page']); } $prev_page = $current_page - 1; $next_page = $current_page + 1; //showing your build query echo http_build_query($_GET, '', '&'); //set array $nav_array = array(); //back to first page if ($prev_page >= 2) { $_GET['page'] = 1; $nav_array[] = "<a style='text-decoration:none;' href='?" . http_build_query($_GET, '', '&') . "'>1...</a> "; } //previous page $_GET['page'] = $prev_page; if ($prev_page >= 1) { $nav_array[] = "<a style='text-decoration:none;' href='?" . http_build_query($_GET, '', '&') . "'>$prev_page</a> "; } //current page $_GET['page'] = $current_page; $nav_array[] = "<a style='text-decoration:none;font-weight: bold;' href='?" . http_build_query($_GET, '', '&') . "'>$current_page</a> "; //next page $_GET['page'] = $next_page; if ($next_page <= $total_pages) { $nav_array[] = "<a style='text-decoration:none;' href='?" . http_build_query($_GET, '', '&') . "'>$next_page</a>"; } //last page if ($next_page < $total_pages) { $_GET['page'] = $total_pages; $nav_array[] = "<a style='text-decoration:none;' href='?" . http_build_query($_GET, '', '&') . "'>...$total_pages</a> "; } ?> <form action="" method="GET"> <div class="formSep"><input type="text" name="stud_id" id="stud_id" value="" placeholder="Enroll No" /> <input type="text" name="stud_name" id="stud_name" value="" placeholder="Student Name"/> <select name="class" id="class"> <option value=""> CLASS </option> <option value="Nursery">Nursery</option> <option value="LKG">LKG</option> <option value="UKG">UKG</option> <option value="I">I</option> <option value="II">II</option> <option value="III">III</option> <option value="IV">IV</option> <option value="V">V</option> <option value="VI">VI</option> <option value="VII">VII</option> <option value="VIII">VIII</option> <option value="IX">IX</option> <option value="X">X</option> <option value="XI">XI</option> <option value="XII">XII</option> </select> <select name="section" id="section"><option value=""> SECTION </option> <option value="A">A</option> <option value="B">B</option> <option value="C">C</option> <option value="D">D</option> </select> <input type="submit" name="search" id="search" value="Search" class="btn btn-info" /> </div> </form> <?php foreach ($nav_array as $nav) { echo "$nav "; } ?>
-
I always trim and lower user names as well as doing a unique on them in the database. If are saving then as a *_ci collation in mysql is case insensitive If retrieve them from a database or doing any sort of comparisons should lower them anyway. Maybe is a force of habit or just my way of coding, trying to make it always work than the chance of failing somewhere.
-
Try using full php tags <?php versus short tags <? BTW, no password hashing?
-
I would use header versus all the javascript
-
can anyone help me create search script?
QuickOldCar replied to sopranolv's topic in PHP Coding Help
I had a little time to spare Edit to your database credentials and should see it working <?php //POST minimum age if (isset($_POST['minage']) && ctype_digit(trim($_POST['minage']))) { $minage = trim($_POST['minage']); } else { $minage = "1"; //default } //POST maximum age if (isset($_POST['maxage']) && ctype_digit(trim($_POST['maxage']))) { $maxage = trim($_POST['maxage']); } else { $maxage = "120"; //default } //POST city if set if (isset($_POST['city']) && trim($_POST['city']) != '') { $city = trim($_POST['city']); } else { $city = ''; } ?> <form action="" method="POST"> Age from <input type="text" name="minage" value="<?php echo $minage;?>" size="3"> to <input type="text" name="maxage" value="<?php echo $maxage;?>" size="3"><br /> City <input type="text" name="city" value="<?php echo $city;?>"> <input type="submit" name="submit" value="Find User"> </form> <?php if (isset($_POST['submit'])) { //mysql connection $link = mysqli_connect("localhost", "database_user", "database_password", "database_name");//edit to your information //check connection if (mysqli_connect_errno()) { printf("Connect failed: %s\n", mysqli_connect_error()); exit(); } //build query $query = "SELECT username FROM users WHERE (age BETWEEN $minage AND $maxage)"; if ($city != '') { $query .= " AND city='" . mysqli_real_escape_string($link, $city) . "'"; } //see query //echo $query; //fetch results if ($result = mysqli_query($link, $query)) { //check if is no results if (mysqli_num_rows($result) <= 0) { echo "No Results"; } else { //fetch associative array while ($row = mysqli_fetch_assoc($result)) { echo $row["username"] . "<br />"; } } //free result set mysqli_free_result($result); } //close connection mysqli_close($link); } ?> -
can anyone help me create search script?
QuickOldCar replied to sopranolv's topic in PHP Coding Help
The purpose of this forum is to help people with their existing code, not create it. Do you have any code right now? You need retrieve the POST or GET data from that form connect to the database do an sql query using WHERE and BETWEEN using the POST dynamic values return the results in a while loop http://php.net/manual/en/book.pdo.php http://php.net/manual/en/book.mysqli.php http://php.net/manual/en/mysqli-result.fetch-assoc.php http://dev.mysql.com/doc/refman/5.0/en/comparison-operators.html#operator_between -
Take the steps to first learn php, then the wordpress codex would make more sense to you. Wordpress is an ever changing complicated mess of their own renamed functions.
-
PLEASE HELP $IP = $_SERVER['REMOTE_ADDR'];
QuickOldCar replied to otuatail's topic in PHP Coding Help
Maybe the site is dead. -
It was refreshing to read this, nice to see appreciative people.
-
Is this a plugin, the posts loop or some random code you are sticking there? If it's in the loop you can use get_the_ID() <?php get_the_ID(); ?> Can also make it a variable like this <?php $postid = get_the_ID(); ?> If the ID is not called within the_loop, then we can use the_ID rather than echo get_the_ID(); <?php the_ID(); ?>
-
I would say some sort of notify script or a message system (including email optional) in a content management system (cms) that can send to each user. A cron job to handle when they get notified. Some of the other things mentioned can be added additional and using 0/1 values a database depending the actions. There could possibly be an exact script out there what you are looking for, most likely will have to make one custom.
-
Developed on 5.5.16 deployed to 5.4.34 - issues
QuickOldCar replied to enveetee's topic in PHP Coding Help
You should add to check if the function exists and take different actions if (function_exists('password_hash')) { //use password_hash } else { //use crypt blowfish with a salt http://php.net/manual/en/function.crypt.php } blowfish is only available 5.3+ A function I found on the net function generateHash($password) { if (defined("CRYPT_BLOWFISH") && CRYPT_BLOWFISH) { $salt = '$2y$11$' . substr(md5(uniqid(rand(), true)), 0, 22); return crypt($password, $salt); } } can also do something like this function salted_pass($value) { if (!$value) { $value = "some-default-value"; } $salt = mcrypt_create_iv(22, MCRYPT_RAND); $salt = base64_encode($salt); $salt = str_replace('+', '.', $salt); return crypt($value, '$2y$10$' . $salt . '$'); } mcrypt_create_iv -
Developed on 5.5.16 deployed to 5.4.34 - issues
QuickOldCar replied to enveetee's topic in PHP Coding Help
http://php.net/manual/en/migration55.incompatible.php http://php.net/manual/en/migration55.new-features.php -
You should make a dynamic query depending what is set. Btw, I saw all you past queries you had Category as uppercased if (isset($_GET['category'] && trim($_GET['category']) != ''){ $category = trim($_GET['category']); } if (isset($_GET['subcategory'] && trim($_GET['subcategory']) != ''){ $subcategory = trim($_GET['subcategory']); } $sql_array = array(); if ($category) { $sql_array[] = " category = '$category' "; } if ($subcategory) { $sql_array[] = " subcategory = '$subcategory' "; } $sql = "SELECT * FROM categories"; if (!empty($sql_array)) { $sql .= ' WHERE ' . implode(' AND ', $sql_array); }
-
Don't you have a category and also subcategory column in database? Seems the below code is trying to return a subcategory name within the category column $sql = "SELECT * FROM categories WHERE Category = '$subcategory' ORDER BY subcategory ASC";
-
It's up to you how you want to set this all up. You could make an included menu just hrefs Optionally can just do a form and a select dropdown in a loop, when selected would set the GET value instead of all those href links could do if/else or build dynamic sql queries based upon what is set an example would be if no subcategories is set to just display all, if a subcategory is set only display that $sql2 = "SELECT * FROM categories"; if(isset($subcategory)){ $sql2 .= " WHERE subcategory='".mysql_real_escape_string($subcategory)."'"; }
-
As strider mentioned the other post you have to pull the GET value if it exists. if(isset($_GET['subcategory']) && trim($_GET['subcategory']) !=''){ $subcategory = trim($_GET['subcategory']) !=''); } if(isset($subcategory)){ $sql2 = "SELECT * FROM categories WHERE subcategory='".mysql_real_escape_string($subcategory)."'"; $result2 = mysql_query($sql2) or die(mysql_error()."<br>".$sql2); while($row2 = mysql_fetch_assoc($result2)){ //whatever your values are echo $row2['id']."<br />"; echo $row2['name']."<br />"; } } If you want to retain your href loop to navigate, need to do an additional query and display the results mysql_* functions are deprecated and should be using mysqli or pdo should escape anything being inserted to a database mysql_real_escape_string
-
Show the entire code for computing.php
-
If are looking for the first 3 characters for liz this could work. if(substr($_SERVER['HTTP_HOST'], 0, 3) == "liz"){ echo "yes"; } else { echo "no"; } (this would be better if you did a check if was not your servers actual name) example: if($_SERVER['HTTP_HOST'] != "website.com"){ $is_subdomain = true; } if(isset($is_subdomain) && substr($_SERVER['HTTP_HOST'], 0, 3) == "liz"){ echo "yes"; } else { echo "no"; } below will look only within the subdomain an exact subdomain $explode_host = explode(".",$_SERVER['HTTP_HOST']); $subdomain = $explode_host[0]; if($subdomain == "liz-4"){ echo "yes"; } else { echo "no"; } anywhere it exists within subdomain as requinix mentioned $explode_host = explode(".",$_SERVER['HTTP_HOST']); $subdomain = $explode_host[0]; $pos = strpos($subdomain, "liz"); if ($pos !== false){ echo "yes"; } else { echo "no"; }