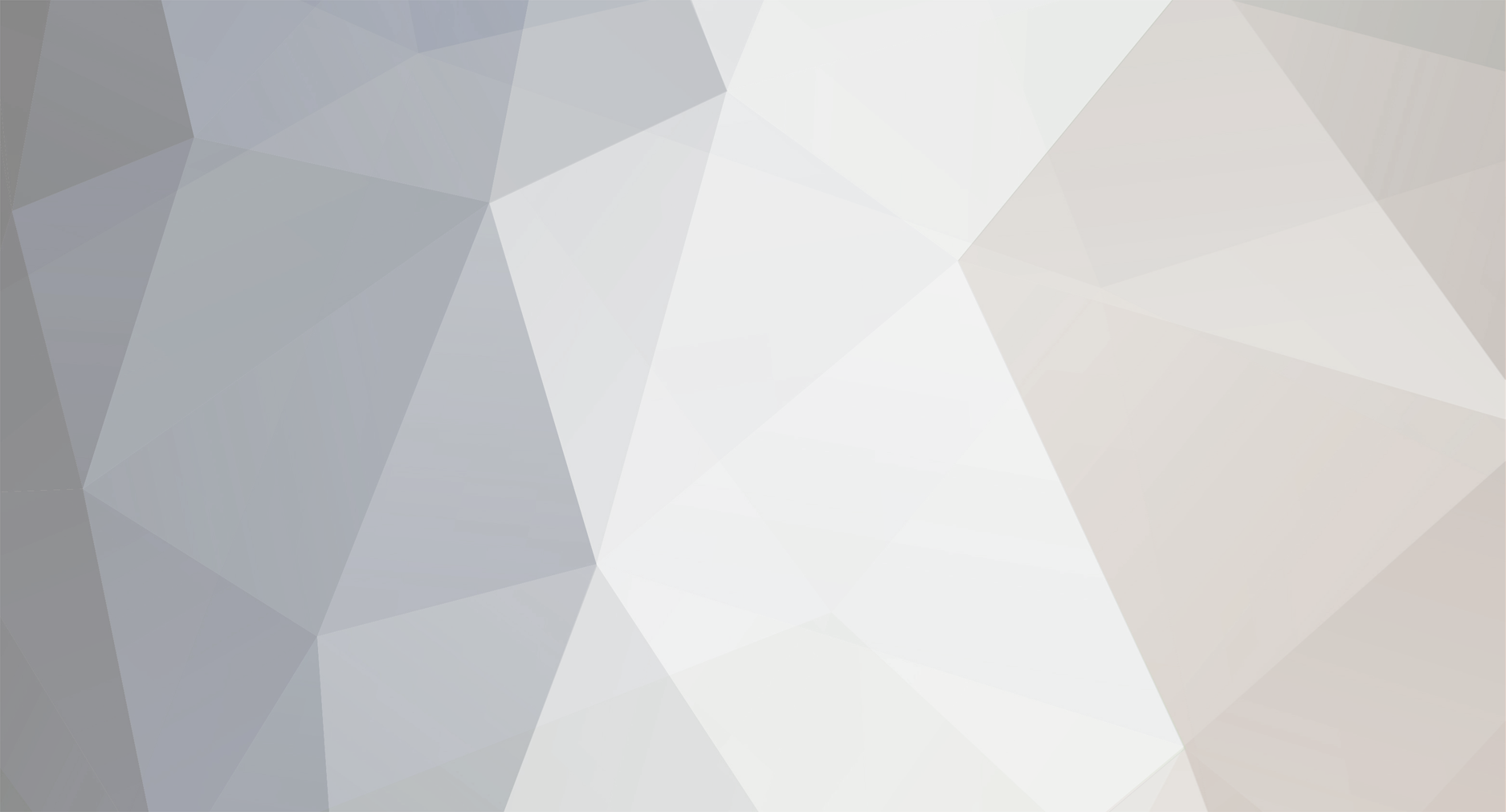
QuickOldCar
Staff Alumni-
Posts
2,972 -
Joined
-
Last visited
-
Days Won
28
Everything posted by QuickOldCar
-
Code from PHP/mySQL tutorial doesn't work.
QuickOldCar replied to macfall's topic in PHP Coding Help
Alright, I fixed it. This does need more error checking for a few items. Use everything I have here and it should work. create table CREATE TABLE dbUsers ( id int NOT NULL AUTO_INCREMENT, username varchar(32) Unique, password char(32), email varchar(32), PRIMARY KEY (id) ) dbConfig.php <?php //use your database connection information $host = "localhost";//usually localhost,change if need different $db_username = "user";//change database user name $db_password = "password";//change database password $db_name = "database";//change database name $db = mysql_pconnect($host, $db_username, $db_password); if ( !$db ) { echo "Error connecting to database.\n"; } mysql_select_db($db_name,$db); ?> index.php <?php session_start(); include('nav.php'); echo "This is the index page"; ?> login.php <?php session_start(); include('dbConfig.php'); if(isset($_POST)){ $username = trim($_POST['username']); $password = trim($_POST['password']); $md5pass = md5($password); if (!empty($_POST["username"]) || !empty($_POST["password"])) { $sql_query = mysql_query("SELECT * FROM dbUsers WHERE username='$username'"); $row = mysql_fetch_array($sql_query) or die(mysql_error()); $user_id = $row['id']; $user_name = $row['username']; $user_password = $row['password']; if($username == $user_name && $md5pass == $user_password) { // Login good, create session variables $_SESSION["valid_id"] = $user_id; $_SESSION["valid_user"] = $user_name; $_SESSION["valid_time"] = time(); //change where to redirect after login //header("Location: index.php"); header("Location: members.php"); } else { $message = "Invalid Login."; } } else { $message = "Insert user name or password."; } include('nav.php'); echo "<form action='' method='POST'>"; echo "Username: (32 Characters Max) <input name='username' size='32'><br />"; echo "Password: (32 Characters Max) <input type='password' name='password' size='32'><br />"; echo "<input type='submit' value='Login'>"; echo "</form>"; echo $message; } ?> logout.php <?php session_start(); session_unset(); session_destroy(); // Logged out, return home. header("Location: index.php"); ?> members.php <?php session_start(); if (!$_SESSION["valid_user"]) { // User not logged in, redirect to login page header("Location: login.php"); } include('nav.php'); // Member only content // ... // ... // ... // Display Member information echo "<p>User ID: " . $_SESSION["valid_id"]; echo "<p>Username: " . $_SESSION["valid_user"]; echo "<p>Logged in: " . date("m/d/Y", $_SESSION["valid_time"]); // Display logout link echo "<p><a href=\"logout.php\">Click here to logout!</a></p>"; ?> nav.php <a href="index.php"> HOME </a> <a href="members.php"> Members </a> <a href="login.php"> Login </a> <a href="logout.php"> Logout </a> <a href="register.php"> Register </a> <br /> register.php <?php // dbConfig.php is a file that contains your // database connection information. This // tutorial assumes a connection is made from // this existing file. include ("dbConfig.php"); //Input vaildation and the dbase code if ( $_GET["op"] == "reg" ) { $bInputFlag = false; foreach ( $_POST as $field ) { if ($field == "") { $bInputFlag = false; } else { $bInputFlag = true; } } // If we had problems with the input, exit with error if ($bInputFlag == false) { die( "Problem with your registration info. " ."Please go back and try again."); } $user = mysql_real_escape_string(trim($_POST['username'])); $pass = md5(mysql_real_escape_string(trim($_POST['password']))); $mail = mysql_real_escape_string(trim($_POST['email'])); // Fields are clear, add user to database // Setup query $r = mysql_query("INSERT INTO dbUsers (username, password, email) VALUES('$user', '$pass', '$mail' ) ") or die(mysql_error()); // Make sure query inserted user successfully if ( !$r ) { die("Error: User not added to database."); } else { // Redirect to thank you page. header("Location: register.php?op=thanks"); } } // end if //The thank you page elseif ( $_GET["op"] == "thanks" ) { echo "<h2>Thanks for registering!</h2>"; echo "Redirecting you to log in<br />"; echo "<meta http-equiv='refresh' content='5;url=login.php'>"; } //The web form for input ability else { include('nav.php'); echo "<form action='?op=reg' method='POST'>\n"; echo "Username: <input name='username' MAXLENGTH='32'><br />\n"; echo "Password: <input name='password' MAXLENGTH='32'<br />\n"; echo "Email Address: <input name='email' MAXLENGTH='32'><br />\n"; echo "<input type='submit'>\n"; echo "</form>\n"; } // EOF ?> -
Code from PHP/mySQL tutorial doesn't work.
QuickOldCar replied to macfall's topic in PHP Coding Help
oops, is still an issue inserting the md5 password on register form, looking into it, is adding an extra * to beginning of md5 -
Code from PHP/mySQL tutorial doesn't work.
QuickOldCar replied to macfall's topic in PHP Coding Help
I rewrote some of this, it works ok. to create the database CREATE TABLE dbUsers ( id int NOT NULL AUTO_INCREMENT, username varchar(16) Unique, password char(32), email varchar(25), PRIMARY KEY (id) ) dbConfig.php <?php //use your database connection information $host = "localhost";//usually localhost,change if need different $db_username = "dbuser";//change database user name $db_password = "dbpass";//change database password $db_name = "dbname";//change database name $db = mysql_pconnect($host, $db_username, $db_password); if ( !$db ) { echo "Error connecting to database.\n"; } mysql_select_db($db_name,$db); ?> index.php <?php session_start(); include('nav.php'); echo "This is the index page"; ?> login.php <?php session_start(); include('dbConfig.php'); if(isset($_POST)){ $username = trim($_POST['username']); $password = trim($_POST['password']); $md5pass = md5($password); if (!empty($_POST["username"]) || !empty($_POST["password"])) { $sql_query = mysql_query("SELECT * FROM dbUsers WHERE username='$username'"); $row = mysql_fetch_array($sql_query) or die(mysql_error()); $user_id = $row['id']; $user_name = $row['username']; $user_password = $row['password']; if($username == $user_name && $md5pass == $user_password) { // Login good, create session variables $_SESSION["valid_id"] = $user_id; $_SESSION["valid_user"] = $user_name; $_SESSION["valid_time"] = time(); //change where to redirect after login //header("Location: index.php"); header("Location: members.php"); } else { $message = "Invalid Login."; } } else { $message = "Insert user name or password."; } include('nav.php'); echo "<form action='' method='POST'>"; echo "Username: (16 Characters Max) <input name='username' size='16'><br />"; echo "Password: (16 Characters Max) <input type='password' name='password' size='16'><br />"; echo "<input type='submit' value='Login'>"; echo "</form>"; echo $message; } ?> logout.php <?php session_start(); session_unset(); session_destroy(); // Logged out, return home. header("Location: index.php"); ?> members.php <?php session_start(); if (!$_SESSION["valid_user"]) { // User not logged in, redirect to login page header("Location: login.php"); } include('nav.php'); // Member only content // ... // ... // ... // Display Member information echo "<p>User ID: " . $_SESSION["valid_id"]; echo "<p>Username: " . $_SESSION["valid_user"]; echo "<p>Logged in: " . date("m/d/Y", $_SESSION["valid_time"]); // Display logout link echo "<p><a href=\"logout.php\">Click here to logout!</a></p>"; ?> nav.php <a href="index.php"> HOME </a> <a href="members.php"> Members </a> <a href="login.php"> Login </a> <a href="logout.php"> Logout </a> <a href="register.php"> Register </a> <br /> register.php <?php // dbConfig.php is a file that contains your // database connection information. This // tutorial assumes a connection is made from // this existing file. include ("dbConfig.php"); //Input vaildation and the dbase code if ( $_GET["op"] == "reg" ) { $bInputFlag = false; foreach ( $_POST as $field ) { if ($field == "") { $bInputFlag = false; } else { $bInputFlag = true; } } // If we had problems with the input, exit with error if ($bInputFlag == false) { die( "Problem with your registration info. " ."Please go back and try again."); } // Fields are clear, add user to database // Setup query $q = "INSERT INTO `dbUsers` (`username`,`password`,`email`) " ."VALUES ('".$_POST["username"]."', " ."PASSWORD('".md5($_POST["password"])."'), " ."'".$_POST["email"]."')"; // Run query $r = mysql_query($q); // Make sure query inserted user successfully if ( !r ) { die("Error: User not added to database."); } else { // Redirect to thank you page. header("Location: register.php?op=thanks"); } } // end if //The thank you page elseif ( $_GET["op"] == "thanks" ) { echo "<h2>Thanks for registering!</h2>"; echo "Redirecting you to log in<br />"; echo "<meta http-equiv='refresh' content='5;url=login.php'>"; } //The web form for input ability else { include('nav.php'); echo "<form action=\"?op=reg\" method=\"POST\">\n"; echo "Username: <input name=\"username\" MAXLENGTH=\"16\"><br />\n"; echo "Password: <input type=\"password\" name=\"password\" MAXLENGTH=\"16\"><br />\n"; echo "Email Address: <input name=\"email\" MAXLENGTH=\"25\"><br />\n"; echo "<input type=\"submit\">\n"; echo "</form>\n"; } // EOF ?> Hope this helps some people, and I'm sure can use some improvements as well. -
Code from PHP/mySQL tutorial doesn't work.
QuickOldCar replied to macfall's topic in PHP Coding Help
if ( !$r) Ha! I sure did, must be tired Let me play with this a little more, I did get it working and will post it when made it a little better. -
Code from PHP/mySQL tutorial doesn't work.
QuickOldCar replied to macfall's topic in PHP Coding Help
try changing this line if ( !mysql_insert_id() ) to this if ( !r) I'll try the tutorial and let you know if works or changes need to be made, it should be more secure. -
I always use godaddy to register domains.
-
php mysql time funtion??? datetime??
QuickOldCar replied to YoungNate_Black_coder's topic in PHP Coding Help
hilarious, -
oops, forgot to comment example 2 //example 1 $data = "|2011-07-04_This is a sample text|2011-07-14_this is a second sample text "; $data = ltrim($data,"|"); $explodedata = explode('|', $data); foreach($explodedata as $exdata) { $exdata = explode('_', $exdata); $date_entered = date("Y-m-d", strtotime($exdata[0])); echo $date_entered ." ". $exdata[1]."<br />"; } //example 2 $data = "|2011-07-04_This is a sample text|2011-07-14_this is a second sample text "; $data = ltrim($data,"|"); $explodedata = explode('|', $data); foreach($explodedata as $exdata) { $exdata = explode('_', $exdata); echo $exdata[0] ."<br />". $exdata[1]."<br /><br />"; }
-
I did 2 different examples, removed extra pipe beginning, then exploded the data, then echo by each exploded position is your date saved as that actual date value? if it is you don't need to use the strtotime and date function look at the difference for both examples //example 1 $data = "|2011-07-04_This is a sample text|2011-07-14_this is a second sample text "; $data = ltrim($data,"|"); $explodedata = explode('|', $data); foreach($explodedata as $exdata) { $exdata = explode('_', $exdata); $date_entered = date("Y-m-d", strtotime($exdata[0])); echo $date_entered ." ". $exdata[1]."<br />"; } example 2 $data = "|2011-07-04_This is a sample text|2011-07-14_this is a second sample text "; $data = ltrim($data,"|"); $explodedata = explode('|', $data); foreach($explodedata as $exdata) { $exdata = explode('_', $exdata); echo $exdata[0] ."<br />". $exdata[1]."<br /><br />"; } output for each would be: 2011-07-04 This is a sample text 2011-07-14 this is a second sample text and 2011-07-04 This is a sample text 2011-07-14 this is a second sample text
-
It's always best to show your current code. Basically you can just echo the results on the same echo line. echo "$date $string <br />"; or echo $date." ". $string."<br />"; or even style it in a divider with css echo "<div class='mystyle'>$date $string</div>"; Is other ways as well.
-
Here's an example of how I made different images for the site names link in the default twentyeleven theme. I placed 2 images in the themes images folder, gave full url of the image. The new php area with check for is_home and is_front_page just after </head> For the sites link had to add <?php echo $custom_image;?> </head> <?php //php block added if (is_home() || is_front_page() ){ $custom_image ="<img src='http://localhost/wp-content/themes/twentyeleven/images/headers/logo.png'/>"; } else { $custom_image ="<img src='http://localhost/wp-content/themes/twentyeleven/images/headers/banner.jpg'/>"; } ?> <body <?php body_class(); ?>> <div id="page" class="hfeed"> <header id="branding" role="banner"> <hgroup> <h1 id="site-title"><span><a href="<?php echo esc_url( home_url( '/' ) ); ?>" title="<?php echo esc_attr( get_bloginfo( 'name', 'display' ) ); ?>" rel="home"><?php echo $custom_image;//this instead of sites name ?></a></span></h1> <h2 id="site-description"><?php bloginfo( 'description' ); ?></h2> </hgroup>
-
going by how wordpress displays the site description in header.php of a theme.... if (is_home() || is_front_page() ){ echo "HOME"; } else { echo "NOT HOME"; } It works, I tried it in a new wordpress install
-
Which is better to use echo 'Hello world' or echo "Hello world" ?
QuickOldCar replied to colap's topic in PHP Coding Help
It's interesting to see in those tests that the first test with single quotes took longer than the double quotes. (knowing that double quotes is supposedly using the parser) My test results were this: first run 0.000709 0.000308 0.000279 0.000278 multiple runs afterwards, all very similar and average the 500 range for all, so not much a difference at all. 0.000486 0.000401 0.000505 0.000569 -
There is a wordpress home_url() and site_url() function http://codex.wordpress.org/Function_Reference/home_url Maybe can use that in some way, I don't know personally as I never tried to determine if was home or not in wordpress.
-
Odd error not letting me search more than 50 results.
QuickOldCar replied to Jragon's topic in PHP Coding Help
with the standard agreement is 50 results -
I don't know what system you use, possible your own just saving pages into a folder related to urls. Depending on what your cached server pages expire times are set to, they should really overwrite in a short time anyway. If just want them gone now, Delete the cached pages.
-
Yes i can see how that is possible. This will find home within each folder, meaning per script path or could very well be a subdomain path <?php //get the url from the address bar $current_url = filter_var("http://".$_SERVER['HTTP_HOST'].$_SERVER['SCRIPT_NAME'], FILTER_SANITIZE_STRING); if (!empty($_SERVER["QUERY_STRING"])){ $query_string = filter_var($_SERVER['QUERY_STRING'], FILTER_SANITIZE_STRING); $current_url .= "?".$query_string; } //explode url into parts by /,get the last part $current_file_path = end(explode("/", $current_url)); echo $current_url."<br />"; echo $current_file_path."<br />"; if(empty($_SERVER["QUERY_STRING"]) && $current_file_path == "index.php"){ echo "HOME"; } else { echo "NOT HOME"; } ?> Otherwise, this may just work for you <?php //get the url from the address bar $current_url = filter_var("http://".$_SERVER['HTTP_HOST'].$_SERVER['SCRIPT_NAME'], FILTER_SANITIZE_STRING); if (!empty($_SERVER["QUERY_STRING"])){ $query_string = filter_var($_SERVER['QUERY_STRING'], FILTER_SANITIZE_STRING); $current_url .= "?".$query_string; } $home_url = filter_var("http://".$_SERVER['HTTP_HOST']."/", FILTER_SANITIZE_STRING); $index_url = filter_var("http://".$_SERVER['HTTP_HOST']."/index.php", FILTER_SANITIZE_STRING); if($current_url == $home_url || $current_url == $index_url){ $is_home = true; } if(empty($_SERVER["QUERY_STRING"]) && $is_home == true){ $logo = "./images/logo1.png";//use your logo location } else { $logo = "./images/logo2.png"; } ?>
-
Do you use page caching?
-
reducing queries - mysql insert & foreach
QuickOldCar replied to perky416's topic in PHP Coding Help
If saved the urls information as text or cvs you can cycle the lists with LOAD DATA INFILE http://dev.mysql.com/doc/refman/5.6/en/load-data.html LOAD DATA INFILE 'data.txt' INTO TABLE tbl_name FIELDS TERMINATED BY ',' ENCLOSED BY '"' LINES TERMINATED BY '\r\n' IGNORE 1 LINES; -
<?php $server_page = filter_var("http://".$_SERVER['HTTP_HOST'].$_SERVER['SCRIPT_NAME'], FILTER_SANITIZE_STRING); //echo $server_page."<br />"; $current_page = end(explode("/", $server_page)); if($current_page == "index.php") { $logo = "./images/logo1.png";//use your logo location } else { $logo = "./images/logo2.png"; } ?>
-
A black image is created instead of thumbnails
QuickOldCar replied to upendra470's topic in PHP Coding Help
This most likely works if all your images are .jpg, but not others. GD manual http://php.net/manual/en/book.image.php look at the imagecreatefrom functions imagecreatefromgif — Create a new image from gif imagecreatefromjpeg — Create a new image from jpg,jpeg imagecreatefrompng — Create a new image from png bmp would need to be converted first if need something that for sure resizes a jpg image <?php $file = 'original.jpg'; $save = 'new.jpg'; echo "Created new image $save"; $size = 0.50;//resize % list($width, $height) = getimagesize($file) ; $modwidth = $width * $size; $modheight = $height * $size; $tn = imagecreatetruecolor($modwidth, $modheight) ; $image = imagecreatefromjpeg($file) ; imagecopyresampled($tn, $image, 0, 0, 0, 0, $modwidth, $modheight, $width, $height) ; imagejpeg($tn, $save, 100) ; ?> If want an already made and good script for image resizing all types http://phpthumb.sourceforge.net/ -
Yeah sorry again, that appends but overwrites. To really append at the beginning with no mess ups, you have to first get all the contents of the file (big files would consume lots of memory), save it something like temp.txt, clear the original file, put the new contents and append the saved contents at the end. You can't just reverse the data when call upon the file?
-
Sorry, missed that part. http://php.net/manual/en/function.rewind.php
-
Here's a small script to read and write to a text file. a+ is append and w is write new <?php //read a file $my_file = "filename.txt"; if (file_exists($my_file)) { $data = file($my_file); $total = count($data); echo "<br />Total lines: $total<br />"; foreach ($data as $line) { $line = trim($line); echo "$line<br />"; } //add a new line to end $write = fopen($my_file, 'a+'); $message = "got a new line here\r\n"; fputs($write, $message); fclose($write); /*note the w, this will overwrite the entire contents $write = fopen($my_file, 'w'); $message = "I just added this line and overwrote the file\r\n"; fputs($write, $message); fclose($write); */ } else { echo "No $my_file to display"; } ?>