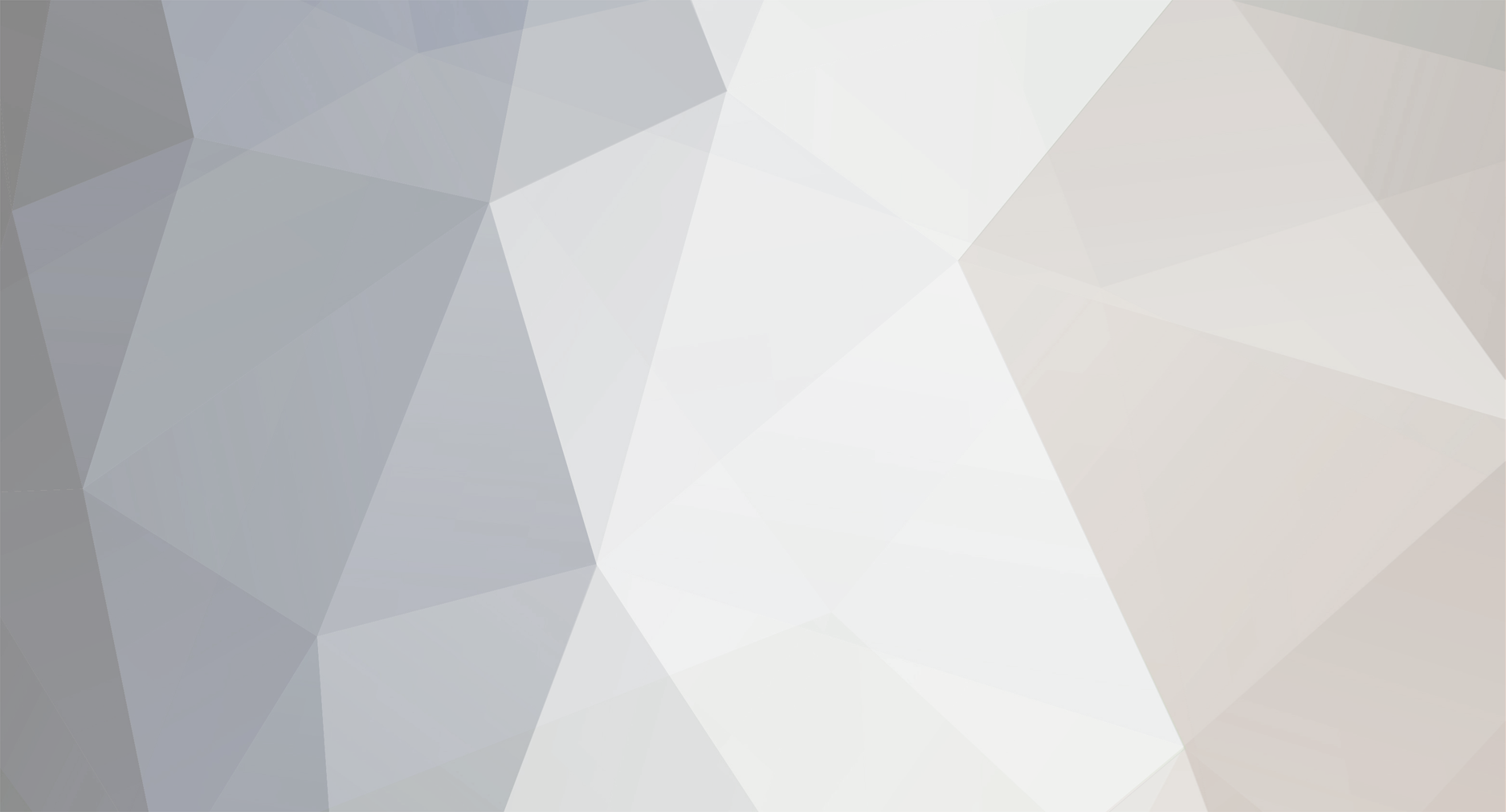
beegro
Members-
Posts
69 -
Joined
-
Last visited
Never
Everything posted by beegro
-
What you have shown as your $query has no quotes around the fields you are trying to assign. Assuming that you're not trying to assign all numeric values then you'll need to wrap your VALUE fields in quotes. (look at the single quotes around $charactername and $shortname. $query = "INSERT INTO `characters` (charactername, shortname, status_id, style_id, division_id, alignment_id, sortorder, creator_id, datecreated, enabled) VALUES ('".$charactername."','".$shortname."',".$status.",".$style.",".$division.",".$alignment.",".$sortorder.", 1, NOW(), 0)";
-
Ah yes, the culprit is that you are trying to set cookies after you've already sent some HTML out to the browser. Setting cookies, headers and session information all needs to be done before anything is outputted to the browser. So, you'd have to move your PHP code to the top of your script for it to work.
-
Do you have the code for the page that processes the information and inserts it into the database? That would be the first place to start.
-
Are the "login.php" and "index.php" scripts in the same directory?
-
Yes, generally it is not a good idea to store password information. Best practice is to verify it when the user enters it and then have your program forget it.
-
Those functions haven't been replaced outright by other functions. Instead PHP now wants you to explicitly start sessions and assign values to the global _SESSION array. i.e. session_start(); $_SESSION['username'] = $username; $_SESSION['password'] = $password; you can then check on other pages for the existence of values i.e. session_start(); if (isset($_SESSION['username'])) { // do something }
-
You're using single quotes around a PHP variable $image->save('$picturename'); That treats the string literally instead of as the $picturename value. Try $image->save($picturename);
-
You shouldn't use the function session_register() anymore. It's been deprecated as of PHP 5.3. Same thing with session_is_registered(). Now, what seems to be the issue? Are you not getting session values back on the members page?
-
Fatal error: Call to a member function call() on a non-object
beegro replied to rowiebiz's topic in Other Libraries
You're passing the constructor argument by reference, which is unnecessary because you're then storing it as an attribute in the class. This may also be causing the element to fall out of scope though I don't know for sure. Try passing the constructor argument by value instead. -
You'll have to hold off on returning a result from the function until all processes are complete. Example: function validate_form(thisform) { var result = true; with (thisform) { if (validate_emailadd(emailadd,"Not a valid e-mail address!")==false) {emailadd.focus;result = false;} if (validate_fname(fname,"Please enter your forename.")==false) {fname.focus();result = false;} if (validate_sname(sname,"Please enter your surname.")==false) {sname.focus();result = false;} return result; } }
-
Is error reporting turned on? If it's off, you may want to turn it on to see if you get any errors. If no errors, you can always write print_r($form); in the area you're writing those echo statements to see if the variable is set properly and has values;
-
This area in your code is assigning and echoing back a file system location. This needs to be transformed into a web URL for a browser to understand where to locate something via and HTTP request. eg. $image_address = $row['ImageAddress']; $image_name = $row['ImageName']; // assuming you have this echo "<td><a href=\"$image_address\">$image_name</a></td>"; Let me know if I'm missing something
-
Not finding entry in SQL database - help please
beegro replied to jancooper's topic in PHP Coding Help
Conversations about user authentication systems and how to hash credentials can go on forever. Here's a brief summary as I understand it. PFMaBiSmAd is saying to simply use the MD5() function in MySQL to hash the password you create. However you will also need to use the MD5() function when you SELECT from the table as well. Your INSERT $newpass = substr(md5(time()),0,6); $sql = "INSERT INTO user SET userid = '$_POST[newid]', password = MD5($newpass), fullname = '$_POST[newname]', email = '$_POST[newemail]', notes = '$_POST[newnotes]'"; if (!mysql_query($sql)) error('A database error occurred in processing your '. 'submission.\\nIf this error persists, please '. 'contact jan-c@o2.co.uk.\\n' . mysql_error()); Your SELECT (for retrieving user information) assuming the information comes from a POSTed form $entered_password = $_POST['password_field']; $user_id = $_POST['user_id_field']; $sql = "SELECT * FROM user WHERE userid = '$user_id' AND password = MD5($entered_password)"; $res = mysql_query($sql); $row = mysql_fetch_row($res); if ($row != FALSE) { // $row should have the user info } else { // no user info was found for that user+password combination } One other thing to note is that you should probably clean any GET, POST, COOKIE information before inserting it into a query with mysql_real_escape_string() to help prevent SQL injection. See http://us2.php.net/manual/en/function.mysql-real-escape-string.php -
The variable $sXML is now an object with all the XML tags being attributes. So, to retrieve the tag [statuses_count] from the XML simply access it in PHP's object form $sXML->statuses_count That will return the value of [statuses_count], this case being 3.
-
You can use SimpleXML to process XML data. $sXML = new SimpleXMLElement('http://api.twitter.com/1/users/show/seobpo.xml', NULL, TRUE); print_r($sXML); You should be able to see the Twitter XML you referenced as a PHP object now and you can perform all the counting and/or transforming you want.
-
I think this may be an issue with the first implementation of MAX in MySQL 5. See: http://bugs.mysql.com/bug.php?id=36300 Of course, you could always rewrite the query like this: $sql = "SELECT position FROM ".self::$table_name." ORDER BY position ASC LIMIT 1";
-
What version of MySQL are you using?
-
I have this argument with one of my coworkers quite often. I'm of the persuasion that object-oriented development is vastly better for the development of applications both big and small. It is undeniable that almost any application with even a marginal amount of success will grow in both features and functionality. As those features and functions increase so does the code base. The benefits of OO development and design are that the data and the methods that operate on those data elements are largely contained in classes. Procedural code doesn't offer that. Now, while a single developer writing procedural code may know exactly where he/she placed the functions he/she wants to use on particular data elements, future developers will often grit their teeth and curse their predecessors as they search through scripts for that function they were told "validates an md5 hash against a particular database field." In addition to containment, additional methods and operation can be extended or overwritten from a parent class to new classes when necessary. This allows a load of benefits from a greater control on backwards compatibility to the security of functionally sensitive operations. To do the same thing in procedural code one must either call a series of previous functions and manipulate/store their results in a new function or write new code that performs very similar tasks as older code that adds to the confusion. It's also worth noting that many of PHPs new functionality is also written in OO style. Take a look at PDO, SimpleXML, DOM, etc. This is not an exhaustive list of the benefits of OO development, merely the tip of the iceberg in my opinion. P.S. - I know emoticons are ridiculous but I can't stop using them.