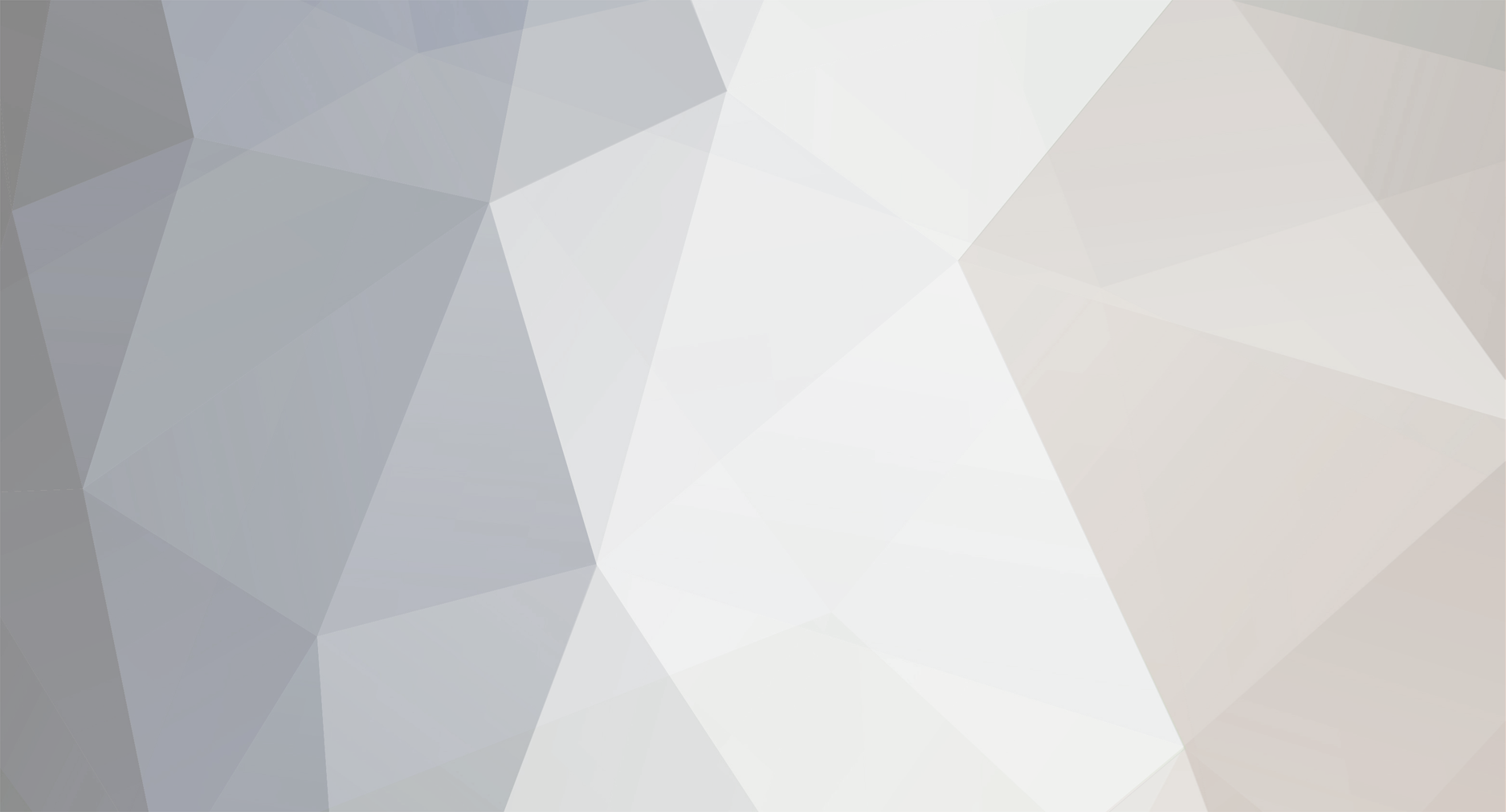
jamesjmann
Members-
Posts
247 -
Joined
-
Last visited
Everything posted by jamesjmann
-
I've looked at some tutorials, but all of them seem too complicated and there wasn't much explanation. And thanks for the link! That definitely answered my question!
-
Hey, all! I posted a thread before about a content management system for my news, and so far the php script works fine. I would like to add additional features to this system, though, and was wondering if anyone could help. Here's the code for all the files needed for the news content management system to work... 1.) First, is the mysql table, called "mynews" with the fields: "id" - primary key; auto-increment. "title" - the title of the news article. "user" - the person who posted the article (would be me in all cases) "message" - the body of the article; this would contain the actual news. "url" - the url to the full article. "time" - time and date the article was posted, or added to database. "type" - the type of news, like 'update', or 'random'. 2.) Next, is the code to connect to the database in mysql, called "dbconnect.php": <?php $username = "your username"; //your username $password = "your password"; //your password $host = "your hostname"; //your mySQL server $database = "your database name"; //The name of the database your table is in mysql_connect($host,$username,$password) or die("Error connecting to Database!<br>" . mysql_error()); //connect, but if there is a problem, display an error message telling why there is a problem mysql_select_db($database) or die("Cannot select database!<br>" . mysql_error()); //Choose the database from your mySQL server, but if there is a problem, display an error telling why ?> 3.) Third, is the forms for posting new articles, called "postnews.php": <form action="process.php" method="post" id="news"> <h1>Post New Article</h1> <p>Please fill out all of the following fields:</p> <table width="100%" border="0" cellpadding="0" cellspacing="0"> <tr> <td class="cmsNewsformText">Type*:</td> <td><font size="1"> <input name="type" type="text" class="cmsNewforms" size="50" /> </font></td> </tr> <tr> <td class="cmsNewsformText">News Topic/Title*: </td> <td><font size="1"> <input name="title" type="text" class="cmsNewforms" value="enter title of article" size="50" /> </font></td> </tr> <tr> <td class="cmsNewsformText">Username*:</td> <td><font size="1"> <input name="user" type="text" class="cmsNewforms" value="enter username" size="50" /> </font></td> </tr> <tr> <td class="cmsNewsformText">Url*:</td> <td><font size="1"> <input name="url" type="text" class="cmsNewforms" value="enter url of news article" size="50" /> </font></td> </tr> <tr> <td class="cmsNewsformText">Message*:</td> <td><font size="1"> <textarea name="message" cols="43" rows="10" class="cmsNewforms">enter body of article </textarea> </font></td> </tr> </table> <p> <font size="1"> <input name="Submit" type="submit" class="Button1" value="Submit" /> </font> </p> </form> 4.) Fourth, is process.php. This script sends the data entered by the administrator (me) on "postnews.php" to the database, and displays a message saying that the information was sent successfully. <?php $user=$_POST['user']; $title=$_POST['title']; $message=$_POST['message']; $type=$_POST['type']; $url=$_POST['url']; mysql_connect("your hostname", "your database name", "your password") or die(mysql_error()); mysql_select_db("cmsnewsacp") or die(mysql_error()); $sql = sprintf("INSERT INTO mynews (user, title, message, type, url) VALUES ('%s', '%s', '%s', '%s', '%s')", mysql_real_escape_string($user), mysql_real_escape_string($title), mysql_real_escape_string($message), mysql_real_escape_string($type), mysql_real_escape_string($url)); $result = mysql_query($sql); Print "The article has successfully been posted"; ?> 5.) And lastly, here's the script for displaying the news on the home page: <? include("dbconnect.php"); //include the file to connect to the database $getnews = mysql_query("SELECT * FROM mynews ORDER BY id DESC"); //query the database for all of the news while($r=mysql_fetch_array($getnews)){ //while there are rows in the table extract($r); //remove the $r so its just $variable echo(" <span class=NewsID>$type</span> <span class=h2>$title</span><br><br> <em>posted by <strong>$user</strong> on $time</em><br> <span class$message<br><br /> <font color=0099FF><a href=$url>Read more - $url</a></font><br><br><p>"); } ?> Now that you've got all the code, let's take a look back at the table "mynews" with its fields. See "url"? This variable would contain the url I type in the forms on "postnews.php" and would be called to form a "Read more" link at the end of each article displayed on the home page. The question I have here, is how can I modify this script to where everytime a new 'article' is added to the database, a new page is created with the article and the url I specified? Also, I want to limit the amount of characters of the article, so that it'll only display a little bit on the home page, with a "read more" link that takes them to that page. Now last but not least, how in the bloody fudge do I script pagination into this script?
-
Where would this code be inserted in the script I posted?
-
PHP & Mysql For Dummies By Janet Valade
jamesjmann replied to jamesjmann's topic in Third Party Scripts
True, it's just I'm so anxious to get my scripts up and running on my website, and I don't exactly have unlimited time. But I'm just going to abandon the book and write the codes from scratch myself. Perhaps I can understand the code better that way. -
Okay, I replaced it with the code you gave me and everything still works. Now...what exactly does this piece of code do? I did some googling but didn't find out very much. All I gleaned was that it prevents database attacks by users exploiting your forms?
-
How and where would I insert the escape string and how would I modify it accordingly?
-
Yeah, I fixed that about a second before you posted this. I guess I misnamed a variable in process.php <?php $user=$_POST['user']; //for example, "$user" was named something else which is why it didn't display your name you typed $title=$_POST['title']; $message=$_POST['message']; $type=$_POST['type']; $url=$_POST['url']; mysql_connect("hostname", "username", "password") or die(mysql_error()); mysql_select_db("cmsnewsacp") or die(mysql_error()); mysql_query("INSERT INTO mynews (user, title, message, type, url) VALUES ('$user', '$title', '$message', '$type', '$url')"); Print "The article has successfully been posted"; ?> MOD Edit: DB login credentials removed . . .
-
I got this book at the library and thought it might be useful. I'm no expert in PHP, but this book's for dummies, right? Well, not exactly...or maybe at least not for someone who has limited knowlege of PHP. I perused through it and found several very useful applications that I am interested in using on my site, but the problem is, I can't integrate any of them into my existing site. The scripts are "stand-alone", i.e., they function outside of your website template. There six in total, but only three of which I'll state here: Login system, Content Management System (CMS), and Forum. I want all of these for my site, but can't figure out how to integrate them into my existing website template. There are no instructions on such a procedure in the book or on the CD, nor in the help files located in the script folders provided by the CD. How can I integrate these scripts into my existing template? How can I combine the login system with both the forum and the CMS?
-
Hello all! I'm a beginner at PHP, and thanks to PHPFreaks.Com, I have just completed one of my first scripts! I have joined in the hopes that I can discuss and learn in order to achieve the desired results in my scripting endeavors. I give thanks to the ever-so friendly members on the site that have helped me on a rather problematic php script after a mere hour of joining the site. I can see that being a part of the community of PHPFreaks will prove to be very beneficial. GO PHPFREAKS!
-
What do you mean?
-
OMG thank you quick car that worked!!!!!! i can now post articles online!!! yay!!!
-
Or even better, since $_SERVER['PHP_SELF'] is a known XSS vulnerability as a form action, just use action="" to submit a form to itself. This didn't work either, so I decided to change the action="" to action="process.php" Here is the code for process.php <?php $name=$_POST['user']; $email=$_POST['title']; $location=$_POST['message']; $type=$_POST['type']; $url=$_POST['url']; mysql_connect("your hostname", "cmsnewsacp", "your password") or die(mysql_error()); mysql_select_db("cmsnewsacp") or die(mysql_error()); mysql_query("INSERT INTO `mynews` VALUES ('$user', '$title', '$message', '$type', '$url')"); Print "The article has successfully been posted"; ?> Now, when you send the form, it directs you to the "The article has successfully been posted" message, but it's still not getting sent to my database and IDK Y Here's the code again for postnews.php <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <meta http-equiv="Content-Type" content="text/html; charset=utf-8" /> <title>Post News</title> </head> <body> <form name="news" method="post" action="process.php"> <h1>Post New Article</h1> <p>Please fill out all of the following fields:</p> <table width="100%" border="0" cellpadding="0" cellspacing="0"> <tr> <td class="cmsNewsformText">Type*:</td> <td><font size="1"> <input name="type" type="text" class="cmsNewforms" size="50" /> </font></td> </tr> <tr> <td width="109" class="cmsNewsformText">News Topic/Title*: </td> <td width="471"> <font size="1"> <input name="title" type="text" class="cmsNewforms" value="enter title of article" size="50"> </font></td> </tr> <tr> <td width="109" class="cmsNewsformText">Username*:</td> <td width="471"> <font size="1"> <input name="user" type="text" class="cmsNewforms" value="enter username" size="50"> </font></td> </tr> <tr> <td class="cmsNewsformText">Url*:</td> <td><font size="1"> <input name="url" type="text" class="cmsNewforms" value="enter url of news article" size="50" /> </font></td> </tr> <tr> <td width="109" class="cmsNewsformText">Message*:</td> <td width="471"> <font size="1"> <textarea name="message" cols=43 rows=10 class="cmsNewforms">enter body of article </textarea> </font></td> </tr> </table> <p> <font size="1"> <input name="Submit" type="submit" class="Button1" value="Submit"> </font> </p> </form> </body> </html> And here's displaynews.php <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <meta http-equiv="Content-Type" content="text/html; charset=utf-8" /> <title>Untitled Document</title> </head> <body> <? include("dbconnect.php"); //include the file to connect to the database $getnews = mysql_query("SELECT * FROM mynews ORDER BY id DESC"); //query the database for all of the news while($r=mysql_fetch_array($getnews)){ //while there are rows in the table extract($r); //remove the $r so its just $variable echo("<hr> <font size=3>$title added on $date</font><br> <font size=1>Posted by $user</font><br> <font size=2>$message</font><p>"); } ?> </body> </html> Does anyone know why this could be? The link to the form is http://www.djsmiley.net/postnews.php To see the news displayed from the database: http://www.djsmiley.net/displaynews.php
-
I just reviewed the code from the links you provided and all of it seems like japanese to me. Do you know how and where I can insert this code into the script I have posted? Or is it a more complicated matter than just copying and inserting?
-
OMG dude i just realized in my "dbconnect.php" file i was pointing to the wrong database lol. i fixed that and now the original code i had works. u can view it at http://www.djsmiley.net/displaynews.php im still encountering issues with the form though. im still using the code with ur modifications and it's not working.
-
I tried all of the above methods but im still getting errors, and i checked my database and no new columns have been added.
-
I'm also having an issue when launching the "displaynews.php". I've never encountered this issue before now: Warning: mysql_fetch_array(): supplied argument is not a valid MySQL result resource in /home/content/d/j/s/djsmiley/html/displaynews.php on line 13 Here's the code for "displaynews.php". The error in action: http://www.djsmiley.net/displaynews.php <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <meta http-equiv="Content-Type" content="text/html; charset=utf-8" /> <title>Display News</title> </head> <body> <? include("dbconnect.php"); //include the file to connect to the database $getnews = mysql_query("SELECT * FROM mynews ORDER BY id DESC"); //query the database for all of the news while($r=mysql_fetch_array($getnews)){ //while there are rows in the table extract($r); //remove the $r so its just $variable echo("<hr> <font size=3>$title added on $date</font><br> <font size=1>Posted by $user</font><br> <font size=2>$message</font><p>"); } ?> </body> </html>
-
This is with the code you gave me. <?php include("dbconnect.php"); //include the file that connects to the database if(!empty($title)) { //if the title is not empty, than do this function $title = addslashes($_POST['title']); $user = addslashes($_POST['user']); $message = addslashes($_POST['message']); $url = addslashes($_POST['url']); $time = addslashes($_POST['time']); $type = addslashes($_POST['type']); $sql = "INSERT INTO mynews (id, title, user, message, url, time, type) VALUES ('NULL', '$title','$user','$message','$url','$time','$type')"; //Insert all of your information into the mynews table in your database $query = mysql_query($sql) or die("Cannot query the database.<br>" . mysql_error()); echo "Database Updated."; } else { //However, if the title variable is empty, than do this function ?> <form name="news" method="post" action="<?php echo $PHP_SELF; ?>"> <h1>Post New Article</h1> <p>Please fill out all of the following fields:</p> <table width="100%" border="0" cellpadding="0" cellspacing="0"> <tr> <td class="cmsNewsformText">Type*:</td> <td><font size="1"> <input name="type" type="text" class="cmsNewforms" size="50" /> </font></td> </tr> <tr> <td width="109" class="cmsNewsformText">News Topic/Title*: </td> <td width="471"> <font size="1"> <input name="title" type="text" class="cmsNewforms" value="enter title of article" size="50"> </font></td> </tr> <tr> <td width="109" class="cmsNewsformText">Username*:</td> <td width="471"> <font size="1"> <input name="user" type="text" class="cmsNewforms" value="enter username" size="50"> </font></td> </tr> <tr> <td class="cmsNewsformText">Url*:</td> <td><font size="1"> <input name="url" type="text" class="cmsNewforms" value="enter url of news article" size="50" /> </font></td> </tr> <tr> <td width="109" class="cmsNewsformText">Message*:</td> <td width="471"> <font size="1"> <textarea name="message" cols=43 rows=10 class="cmsNewforms">enter body of article </textarea> </font></td> </tr> </table> <p> <font size="1"> <input name="Submit" type="submit" class="Button1" value="Submit"> </font> </p> </form> <?php } //end this function ?>
-
Here's the url to the form http://www.djsmiley.net/postnews.php
-
That didn't work. It's still just refreshing the page and doesn't seem to be talking to my database at all.
-
I realized I just posted this, but I added a whole more info regarding the scripts I'm using and when i tried to update the last post, it wouldn't let me. And I'm talking as basic as you can go! I'm trying to implement a commenting system on my website for news articles I post, and I've only gotten as far as creating the script to connect to my database (I use mysql). I've tried every open source script online for this app and I've not been able to get any one to work within my website. They'll work on separate blank html pages, but I can't figure out how to put the code into my existing pages to go with each article. As a result, I've resorted to creating my own script for the comment box system, which shouldn't be too difficult to make. If anyone could possibly describe a step by step process on how this can be done I would be incredibly grateful! Just note that I don't want to use ajax, jscript or any other languages like that, just PHP. The second thing I want to discuss is my News Content Management System. I used an open source script for this, and it's really basic and easy to understand. I use it because I prefer it to manually typing in an article in dreamweaver (this gets really annoying and I had to find out the hard way). So, I turned to the CMS to help make posting news articles easier. The only problem I have with this script is the form page, as you'll see upon scrolling down. Here's the code I use to connect to my database: <?php $username = "commentboxacp"; //your username $password = "enter password here"; //your password $host = "enter host name here"; //your mySQL server $database = "commentboxacp"; //The name of the database your table is in mysql_connect($host,$username,$password) or die("Error connecting to Database!<br>" . mysql_error()); //connect, but if there is a problem, display an error message telling why there is a problem mysql_select_db($database) or die("Cannot select database!<br>" . mysql_error()); //Choose the database from your mySQL server, but if there is a problem, display an error telling why ?> Of course, I put the password and hostname in when I upload the script but I removed it here for obvious reasons. Now, this next script I inserted into my home page (index.php). <? include("cms/news/dbconnect.php"); //include the file to connect to the database $getnews = mysql_query("SELECT * FROM mynews ORDER BY id DESC"); //query the database for all of the news while($r=mysql_fetch_array($getnews)){ //while there are rows in the table extract($r); //remove the $r so its just $variable echo("<hr> <font size=4>$type: </font><font size=3>$title</font><br> <font size=1><em>Posted by $user</em></font><font size=1> | added on $time</font><br><br> <font size=2>$message</font><br><br> <font size=2><a href=cms/news/index.php>Read More - $url</a></font><br> <!--beginning of comment box--> <!--end of comment box--><p>"); } ?> When I put this on my home page, whatever's stored in my database will replace the "$" variables (that's what I think they're called) in the html format I created. This works great. The only problem I have with it is that I don't know how to assign the text css classes or insert divs to contain the articles. I also need a code for pagination, once the number of news articles I post exceeds a certain number, like "10". Also, notice that I put this right after the variables that display the information for the articles. <!--beginning of comment box--> <!--end of comment box--><p>"); Is this where I would put the html comment box? Assuming so, every time a new article is added to the database, a comment box would appear right underneath it. How would I assign it a unique id so that it'll only display comments related to the specific news article? Now, here's the cms form that doesn't work (I have NO IDEA what the problem is here). The way it's scripted, an error message should pop up if the database wasn't queried. When I test it, the database doesn't update, but no error message is displayed. <?php include("dbconnect.php"); //include the file that connects to the database if(!empty($title)) { //if the title is not empty, than do this function $title = addslashes($title); $user = addslashes($user); $message = addslashes($message); $url = addslashes($url); $time = addslashes($time); $type = addslashes($type); $sql = "INSERT INTO mynews (id, title, user, message, url, time, type) VALUES ('NULL', '$title','$user','$message','$url','$time','$type')"; //Insert all of your information into the mynews table in your database $query = mysql_query($sql) or die("Cannot query the database.<br>" . mysql_error()); echo "Database Updated."; } else { //However, if the title variable is empty, than do this function ?> <form name="news" method="post" action="<?php echo $PHP_SELF; ?>"> <h1>Post New Article</h1> <p>Please fill out all of the following fields:</p> <table width="100%" border="0" cellpadding="0" cellspacing="0"> <tr> <td class="cmsNewsformText">Type*:</td> <td><font size="1"> <input name="type" type="text" class="cmsNewforms" size="50" /> </font></td> </tr> <tr> <td width="109" class="cmsNewsformText">News Topic/Title*: </td> <td width="471"> <font size="1"> <input name="title" type="text" class="cmsNewforms" value="enter title of article" size="50"> </font></td> </tr> <tr> <td width="109" class="cmsNewsformText">Username*:</td> <td width="471"> <font size="1"> <input name="user" type="text" class="cmsNewforms" value="enter username" size="50"> </font></td> </tr> <tr> <td class="cmsNewsformText">Url*:</td> <td><font size="1"> <input name="url" type="text" class="cmsNewforms" value="enter url of news article" size="50" /> </font></td> </tr> <tr> <td width="109" class="cmsNewsformText">Message*:</td> <td width="471"> <font size="1"> <textarea name="message" cols=43 rows=10 class="cmsNewforms">enter body of article </textarea> </font></td> </tr> </table> <p> <font size="1"> <input name="Submit" type="submit" class="Button1" value="Submit"> </font> </p> </form> <?php } //end this function ?> The way this script it supposed to work is to take the information put in the fields and return it to the database. The script on the home page would then run through the database and pull that information to display. The problem though is that the information isn't getting sent. This is the only script out of the three that doesn't work. Help?
-
And I'm talking as basic as you can go! I'm trying to implement a commenting system on my website for news articles I post, and I've only gotten as far as creating the script to connect to my database (I use mysql). I've tried every open source script online for this app and I've not been able to get any one to work within my website. They'll work on separate blank html pages, but I can't figure out how to put the code into my existing pages, which is a huge problem. As a result, I've resorted to creating my own script, which shouldn't be too difficult to make. If anyone could possibly describe a step by step process on how this can be done I would be incredibly grateful! Just note that I don't want to use ajax, jscript or any other languages like that, just PHP. Another question I have, is how would I organize my database to include all news articles, and comments with a news article "id"? Would I need to put both in separate tables and just assign each comment with the uniqe id associated with whatever news article? And the last thing, I want pagination for my news. I have it to where if I insert a news article column into my table "mynews", it'll show up on the home page, but how and where would I insert a pagination code to make the articles "paginate"?
-
Hi, I'm creating a website for my music, and I wanted to design the layout for my website in photoshop, using the "save for webs & devices. I've been using free layouts online, because my "html approach" with designing sites sucks, and as I want my website to be completely original, I decided to switch over to photoshop. Now my question here is after you slice up the image in photoshop, how do you create the actual website in dreamweaver? Do you have to manually create the div containers for the graphics, or does photoshop do this for you? I'm confused!!! Sorry, if I posted this in the wrong place. I would imagine this has to do with css, because you use css to customize the website, right?