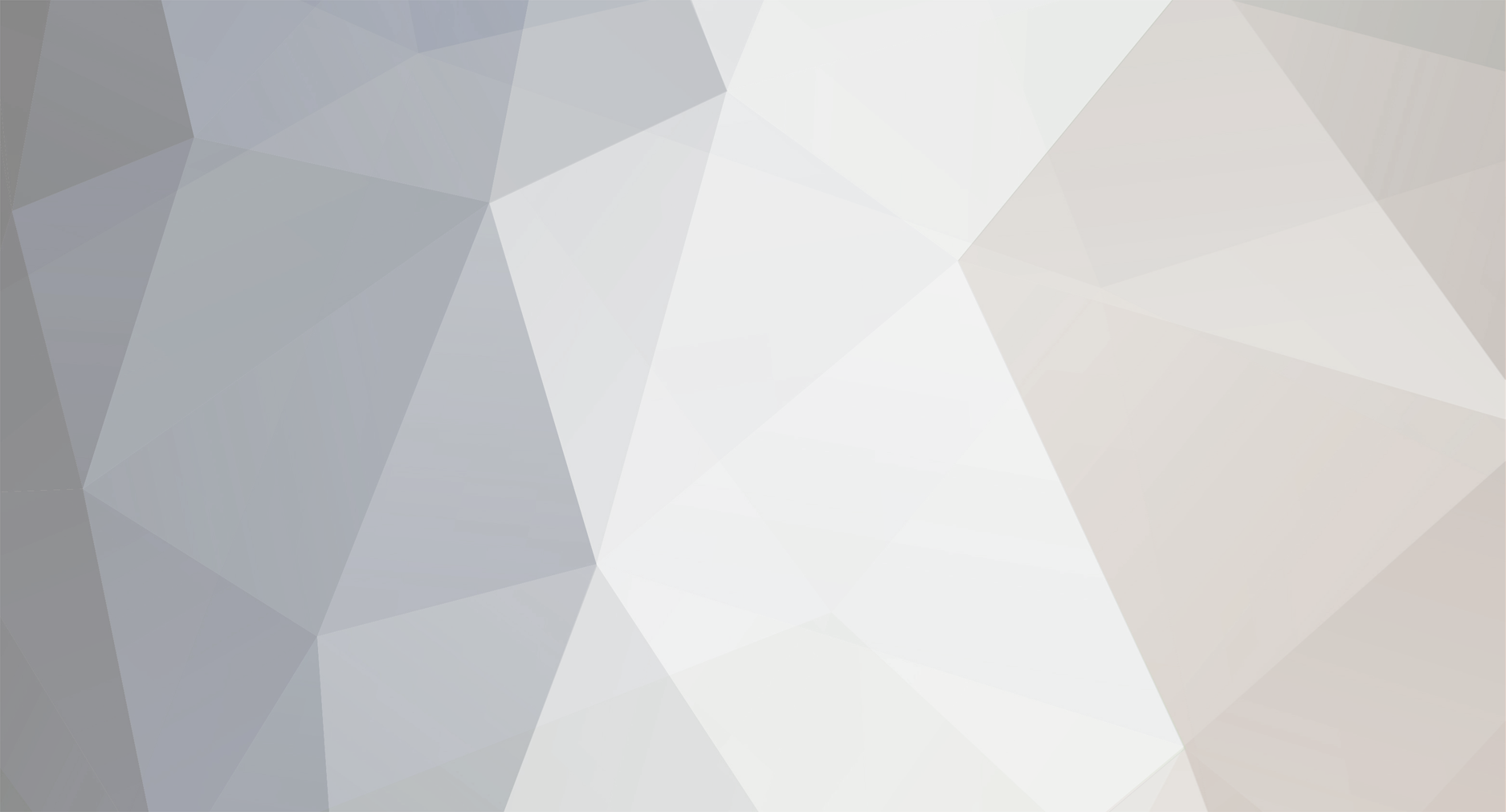
jamesjmann
Members-
Posts
247 -
Joined
-
Last visited
Everything posted by jamesjmann
-
I want to build a captcha from scratch, as I'm all about doing things myself, and I've proposed a nice idea on how I can achieve that, but I'm sort of lost... What I want to do is write a script that will generate a random string of both letters and numbers, with the ability to set a limit on how many characters are generated. Then, I want to store that string within a variable called "random_string". Once the user types in that code, their input would get captured in a separate php file and stored within a variable like so: $captcha_code = $_POST['catpcha_code']; Then, I would write a code to see if the user's input matches the random character string from the html: <?php if ($random_string == $captcha_code) { mail($to, $subject, $body); } else { echo ("The code you entered did not match the captcha. Please go back and try again."); } ?> Is this possible? I haven't yet tried it myself, because I don't know how to generate random strings of characters, but I was just wondering if perhaps I'm on the right track? Any suggestions or advice is appreciated =)
-
lol ok. guess i can try talking to a facebook executive and see what i can weedle out of him haha
-
lol. is that how websites like facebook does it?
-
Is it better to store actual image data in Mysql or just the url to the image on your server? I currently just store the url so when I display images on my page, I do something like this: <a href="$url"><img src="$url"></a> Does anyone know which method big sites like Facebook uses? I'm trying to figure out which method is best, because I'm currently designing a social networking site, and of course, finding a suitable method of storing user data such as this is integral.
-
I put that code right after the <body> tag, because it was conflicting with other php code I have before the <!doctype> tag. I have another question, though. What are the other browser names? For example, $using_ie = (strpos($_SERVER['HTTP_USER_AGENT'], 'Firefox') !== FALSE); for Mozilla Firefox. Is there a list online somewhere with all of the browser's names on it, specific to this kind of code?
-
I want to display my pictures I stored in Mysql in a 4 column, 2 row table WITH pagination. Here's the code I use to display the data currently: //your username $username = "username"; //your password $password = "password"; //your mySQL server $host = "host"; //The name of the database your table is in $database = "database"; //connect, but if there is a problem, display an error message telling why there is a problem $conn = mysql_connect($host,$username,$password) or die("Error connecting to Database!<br>" . mysql_error()); //Choose the database from your mySQL server, but if there is a problem, display an error telling why $db = mysql_select_db($database) or die("Cannot select database!<br>" . mysql_error()); // find out how many rows are in the table $sql = "SELECT COUNT(*) FROM myphotos"; $result = mysql_query($sql, $conn) or trigger_error("SQL", E_USER_ERROR); $r = mysql_fetch_row($result); $numrows = $r[0]; // number of rows to show per page $rowsperpage = 4; // find out total pages $totalpages = ceil($numrows / $rowsperpage); // get the current page or set a default if (isset($_GET['currentpage']) && is_numeric($_GET['currentpage'])) { // cast var as int $currentpage = (int) $_GET['currentpage']; } else { // default page num $currentpage = 1; } // end if // if current page is greater than total pages... if ($currentpage > $totalpages) { // set current page to last page $currentpage = $totalpages; } // end if // if current page is less than first page... if ($currentpage < 1) { // set current page to first page $currentpage = 1; } // end if // the offset of the list, based on current page $offset = ($currentpage - 1) * $rowsperpage; // get the info from the db $sql = "SELECT * FROM myphotos ORDER BY id ASC LIMIT $offset, $rowsperpage"; $result = mysql_query($sql, $conn) or trigger_error("SQL", E_USER_ERROR); /****** build the pagination links ******/ // range of num links to show $range = 3; // if not on page 1, don't show back links if ($currentpage > 1) { // show << link to go back to page 1 echo "<span class=\"pagination\"><a href='{$_SERVER['PHP_SELF']}?currentpage=1'>First</a></span> "; // get previous page num $prevpage = $currentpage - 1; // show < link to go back to 1 page echo " <span class=\"pagination\"><a href='{$_SERVER['PHP_SELF']}?currentpage=$prevpage'>Previous</a></span> "; } // end if // loop to show links to range of pages around current page for ($x = ($currentpage - $range); $x < (($currentpage + $range) + 1); $x++) { // if it's a valid page number... if (($x > 0) && ($x <= $totalpages)) { // if we're on current page... if ($x == $currentpage) { // 'highlight' it but don't make a link echo " <span class=\"paginationDown\"><b>$x</b></span> "; // if not current page... } else { // make it a link echo " <span class=\"pagination\"><a href='{$_SERVER['PHP_SELF']}?currentpage=$x'>$x</a></span> "; } // end else } // end if } // end for // if not on last page, show forward and last page links if ($currentpage != $totalpages) { // get next page $nextpage = $currentpage + 1; // echo forward link for next page echo " <span class=\"pagination\"><a href='{$_SERVER['PHP_SELF']}?currentpage=$nextpage'>Next</a></span> "; // echo forward link for lastpage echo " <span class=\"pagination\"><a href='{$_SERVER['PHP_SELF']}?currentpage=$totalpages'>Last</a></span><br> "; } // end if /****** end build pagination links ******/ // while there are rows to be fetched... while ($list = mysql_fetch_assoc($result)) { extract ($list); // echo data $url = $list['url'];; $title = $list['title']; $description = $list['description']; echo("$title<br><a rel=\"example_group\" title=\"$description\" href=\"$url\"><img src=\"$url\" alt=\"\" width=\"\" height=\"\" class=\"gallery_images\" /></a>"); } // end while /****** build the pagination links ******/ // range of num links to show $range = 3; // if not on page 1, don't show back links if ($currentpage > 1) { // show << link to go back to page 1 echo "<span class=\"pagination\"><a href='{$_SERVER['PHP_SELF']}?currentpage=1'>First</a></span> "; // get previous page num $prevpage = $currentpage - 1; // show < link to go back to 1 page echo " <span class=\"pagination\"><a href='{$_SERVER['PHP_SELF']}?currentpage=$prevpage'>Previous</a></span> "; } // end if // loop to show links to range of pages around current page for ($x = ($currentpage - $range); $x < (($currentpage + $range) + 1); $x++) { // if it's a valid page number... if (($x > 0) && ($x <= $totalpages)) { // if we're on current page... if ($x == $currentpage) { // 'highlight' it but don't make a link echo " <span class=\"paginationDown\"><b>$x</b></span> "; // if not current page... } else { // make it a link echo " <span class=\"pagination\"><a href='{$_SERVER['PHP_SELF']}?currentpage=$x'>$x</a></span> "; } // end else } // end if } // end for // if not on last page, show forward and last page links if ($currentpage != $totalpages) { // get next page $nextpage = $currentpage + 1; // echo forward link for next page echo " <span class=\"pagination\"><a href='{$_SERVER['PHP_SELF']}?currentpage=$nextpage'>Next</a></span> "; // echo forward link for lastpage echo " <span class=\"pagination\"><a href='{$_SERVER['PHP_SELF']}?currentpage=$totalpages'>Last</a></span><br>"; } // end if /****** end build pagination links ******/ The above code just displays the pictures vertically. Here's this code live: http://www.djsmiley.net/gallery/albums/my_photos.php (you can't view the page in IE) Now how would I make it so that the first 8 images in the database display in a 4x2 table, etc.?
-
I do: $using_ie = (strpos($_SERVER['HTTP_USER_AGENT'], 'MSIE') !== FALSE); if ($using_ie) { die("We hate your browser (Internet Explorer), and therefore you don't deserve to use our site.<br /><a href='http://www.google.com/chrome/intl/en/landing_chrome.html?hl=en'>Get Chrome</a>"); } I think it has some flaws though since some browsers have MSIE in the tag, older versions of Opera I think.. Overall I advise against this anyway, I just do it in spite of IE for the time being till I fix my problems with it. If it's styling that's the issue, look into conditional stylesheets. (Meaning, use one stylesheet for IE, and another for other browsers). See, I tried that because I'm using the new CSS3 animations and box shadows, etc. and it's too complicated for me to add multibrowser support for each one, so I decided to just go with this method. I have a couple questions about your code... 1. Do I wrap the entire document (including the !doctype tag) inside the <php> tags? 2. What do you mean some browsers have MSIE in the tag?
-
If a user is using internet explorer, display error "You are not using a browser that is supported. Please reload in Google Chrome or Mozilla Firefox to access content", otherwise display page. Not sure if this is possible with an if else statement, or even php for that matter. Does anyone know what I'm talking about, and if so, any suggestions?
-
so you can't write the html or javascript within the bracket like this? <?php if($variable == "xxxx") { <!-- DO SOME HTML JS OR OTHER CODE HERE --> } ?> Or can you do it both ways?
-
I've seen this, <?php } ?> in a lot of scripts at the very end of the script. What does this piece of code do?
-
It is but now I can't specify the meta tags for the main page. all of the pages with the news articles on them have their own unique tags, but now index.php shows up as "index.php" in the title bar. Here's the whole function: <?php //function for displaying only one item function displayOneItem($id) { global $db; /* query for item */ $query = "SELECT * FROM news WHERE id=$id"; $result = mysql_query ($query); $row = mysql_fetch_assoc($result); /* easier to read variables and * striping out tags */ $title = htmlentities ($row['title']); $news = nl2br (strip_tags ($row['body'], '<a><b><i><u>')); /* if we get no results back, error out */ if (mysql_num_rows ($result) == 0) { echo "This news article does not exist!\n"; return; } echo ("<title>DJSmiley.Net: $title</title>"); echo ("<meta name=\"Description\" content=\"$news\" />"); echo ("<link rel=\"image_src\" href=\"http://www.djsmiley.net/images/Logos/DJ Smiley Logo.jpg\" / >"); /* displays individual article user visits */ echo "<tr><td><strong><h2><a href=\"{$_SERVER['PHP_SELF']}" . "?action=show&id={$row['id']}\">$title</a></h2></strong> <em>posted</em> on {$row['date']} | by <strong>DJ Smiley</strong></td></tr>\n"; echo "<tr><td>"; echo stripslashes($news); echo "</td></tr>\n"; echo "</table>\n"; echo "<br>\n"; echo ("</table>\n<table width=\"100%\" border=\"0\"> <tr> <td width=\"6%\"><a href=\"{$_SERVER['PHP_SELF']}" . "?action=show&id={$row['id']}\"><img src=\"images/Icons/Comment/2.png\" width=\"20\" height=\"20\" class=\"fltlft2\"/></a>$comment_row[0]</td> <td width=\"13%\"><!-- FreeTellaFriend BEGIN --> <a href=\"http://www.freetellafriend.com/tell/\" onclick=\"window.open('http://www.freetellafriend.com/tell/?option=email&heading=Tell+A+Friend&bg=14&url=http%3A%2F%2Fhttp://www.djsmiley.net/index.php?action=show&id={$row['id']}', 'freetellafriend', 'scrollbars=1,menubar=0,width=435,height=500,resizable=1,toolbar=0,location=0,status=0,left='+(screen.width-435)/2+',top='+(screen.height-500)/3);return false;\"><img alt=\"Tell a Friend\" src=\"http://serv1.freetellafriend.com/s14.png\" border=\"0\" /></a> <!-- FreeTellaFriend END --></td> <td width=\"81%\"><span class=\"st_twitter_hcount\" st_url=\"http://www.djsmiley.net/index.php?action=show&id={$row['id']}\" displayText=\"Tweet\"></span><span class=\"st_facebook_hcount\" st_url=\"http://www.djsmiley.net/index.php?action=show&id={$row['id']}\" displayText=\"Share\"></span><span class=\"st_email_hcount\" st_url=\"http://www.djsmiley.net/index.php?action=show&id={$row['id']}\" displayText=\"Email\"></span><span class=\"st_sharethis_hcount\" st_url=\"http://www.djsmiley.net/index.php?action=show&id={$row['id']}\" displayText=\"Share\"></span></td> </tr> </table>"); /* now show the comments */ displayComments($id); } ?> This displays each article echoing their name in the title bar, but how can I reconstruct it (as an if statement, possibly?) so that IF one article is NOT being displayed, the title echos the <title> tag I've specified for index.php?
-
See that's what I tried doing in the beginning but it didn't work cuz I guess the <meta> tag at the very top was getting read first, and after doing some reading I discovered that you have to get rid of the <head> tag at the top in order for the meta tags defined in your script to work
-
I'm pretty sure you didn't. This is what I'm talking about: http://www.codingforums.com/showthread.php?t=129123
-
I understand perfectly what your trying to do. Do you know how I can achieve this, then?
-
Sorry, you've lost me. Again, meta tag data is just data, the same as the rest of your data. Ok, have you ever used the facebook share button? Well, whenever you click it, it takes you to a page that shows a graphic, title, and description of whatever it is you clicked on to share. Now, since I'm using these share buttons for individual articles that are dynamically created, I want the title of the article and description (as in the body), to appear there, because whenever someone clicks on one of my articles, the meta title and description used for the main page shows, which would be "Welcome to my website". Here's the php code that generates my pages; perhaps, this will help you understand a little better: switch($_GET['action']) { case 'show': displayOneItem($_GET['id']); break; case 'all': displayNews(1); break; case 'addcomment': addComment($_GET['id']); break; default: displayNews();
-
I'm not exactly sure what you mean, because since my pages are generated dynamically, I can't exactly go into the header of each one and specify the meta tags using a variable. I would have to use an if statment, I think, like, if $page is true, echo <meta> tags $title $description, etc. Is that right?
-
You know there's a tutorial for pagination right here on phpfreaks. I highly recommend this one because it was very easy to understand and worked for me! http://www.phpfreaks.com/tutorial/basic-pagination
-
How do you give pages that are generated using the _Get method it's own meta tags? Not sure if I'm explaining this properly so allow me to elaborate: When a visitor clicks on an article on my site, they get redirected to a new page (index.php?action=article1) within the original page (index.php). Now, since it's more or less the same page, the meta tags are the same, but I was wondering if there is a way I could make it to where each article has it's own unique tags so when a visitor, for instance, clicks "share on facebook", the title and description of that article appears. Does anyone know what I'm talking about? If so, is there a way to do this?
-
Nevermind. Putting news_id before "user" and "'$id'" before "'{$_POST['name]}'", solved that last issue. Thanks to everyone who helped!
-
ok i don't know what you did, but that definitely worked! now the problem im having is the comments i post now aren't being displayed BECAUSE, they are not being assigned the news article id relative to whatever article i post on. for example... after testing it this popped up in my database: id - 13 news_id - 0 name - random text i entered comment - random text i entered time - time i posted it
-
it has 5 columns. the comment id itself the id of the news article so it knows where to display each comment the name the user inputs the comment the user inputs and the time of the comment the second table is for the news articles id title user date body url btw, here's the link to the script im talking about. perhaps you guys can try it out yourself so you can have a better understanding on what's going on here? http://djsmiley.net/index.php?action=show&id=1
-
Here is the addComment function before: function addComment($id) { global $db; /* insert the comment */ $query = "INSERT INTO comments VALUES('',$id,'{$_POST['name']}','{$_POST['comment']}')"; mysql_query($query); echo "<h1>Success!</h1>Your comment has been posted!<br><a href=\"{$_SERVER['PHP_SELF']}" . "?action=show&id=$id\">Return To Previous Page</a>\n"; } Here's the function modified with your suggestion: function addComment($id) { global $db; /* insert the comment */ $query = "INSERT INTO comments VALUES('',$id,'{$_POST['name']}','{$_POST['comment']}')"; $result = mysql_query ($query); if (!$result) { echo 'Error: ' . mysql_error(); } else { echo "<h1>Success!</h1>Your comment has been posted!<br><a href=\"{$_SERVER['PHP_SELF']}" . "?action=show&id=$id\">Return To Previous Page</a>\n"; } } Now, here's the error I got when I submitted the form: Error: Column count doesn't match value count at row 1
-
That's not my problem. My database is displaying EVERYTHING correctly, as it should. But the form doesn't work. When I complete the form and hit the submit button, I get taken to a "comment successful" page, as is supposed to happen, but the data does not get inserted into my database.
-
The original script is at the link at the very top of my first post
-
Which line is '29'?