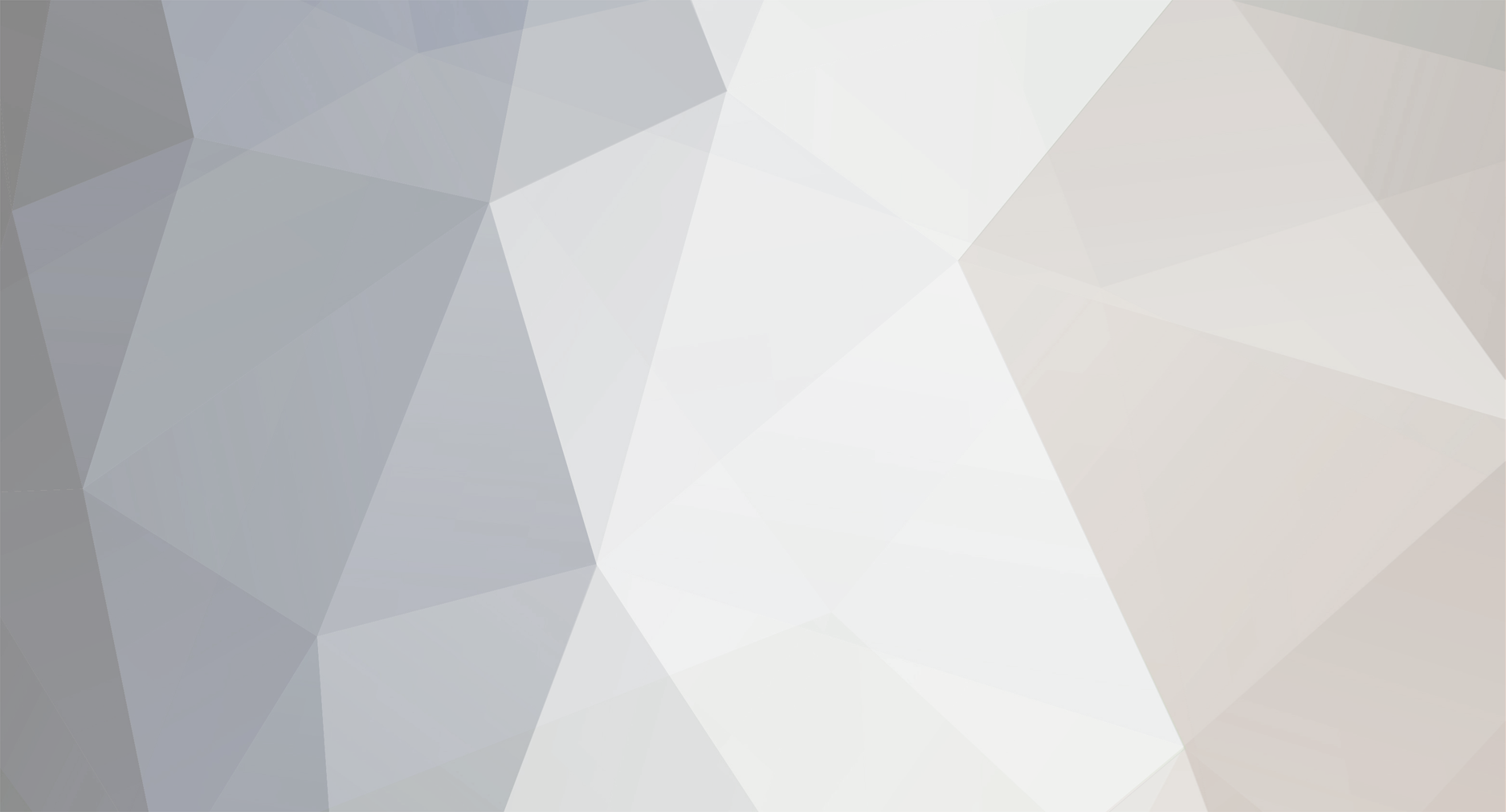
DeadxBeat
Members-
Posts
11 -
Joined
-
Last visited
Never
Everything posted by DeadxBeat
-
Should my error class make use of static functions that every class can use or when an error occurs should I instantiate a new object containing the errors needed to be displayed? Also, if I go the static route should I just run something like Error::display_errors() once on the index page where content should go or should i have it call display_errors at the end of class function that needs it.
-
So sorry, overlooked that $z = $_GET['z']; // Get z variable from URL $x = array("Example 1", "Example 2", "Example 3"); // Set array(you should probably do this dynamically later on) echo "x[z] = " . $x[$z]; // Will display whatever z is echo "<br />"l echo "x[0] = " . $x[0]; // Will display Example 1 echo "<br />"; // Line break for clarity echo "x[1] = " . $x[1]; // Will display Example 2 echo "<br />"; echo "x[2] = " . $x[2]; // Will display Example 3 Please understand that this is just the logic of the code, you will want to check to see if $_GET['z'] is set and check to see that the index "z" is in the array before echo'ing it out.
-
Use an array to store your data. $z = $_GET['z']; // Get z variable from URL $x = array("Example 1", "Example 2", "Example 3"); // Set array(you should probably do this dynamically later on) echo "x[0] = " . $x[0]; // Will display Example 1 echo "<br />"; // Line break for clarity echo "x[1] = " . $x[1]; // Will display Example 2 echo "<br />"; echo "x[2] = " . $x[2]; // Will display Example 3
-
This is my alpha module class that I finished yesterday and works well except Im still trying to figure out a way to include css from individual modules into the main css of the site when those modules are loaded. I documented a little bit, basically I call loadMods() from the _module class on the index page in the nav bar where modules will go( Modules for my cms will be like Polls, Latest Posts, Friends, etc..) <?php class _module { // Class for all things module related private $modlist = null; // Variable containing list of all modules private function getFolders() { // Function to put all directory names in the module folder into a class property array $base_dir = "./modules/"; // Path to modules directory $bad = array(".",".."); // Exclude these "directories" $result = scandir($base_dir); // Scan the directory and put all results into array $dir = array_diff($result, $bad); // Remove the directories that are to be excluded chdir($base_dir); // Make the current working directory the module directory foreach($dir as $test) { // Loop through contents of modules directory if(is_dir($test) == true){ // If the current content is a directory then... $this->modlist[] = $test; // Add directory to global array } } chdir("../"); // Go back to root directory } // End function getFolders() private function updateMods() { // Function to add new modules into database $this->getFolders(); // Get all the directories within the module directory foreach($this->modlist as $module) { // Loop through contents and add new folders to database if they dont exist // TODO REMINDER: Make this so it only adds the folder if the module.php file resides inside folder $sql = "SELECT * FROM cmsModules WHERE name='" . $module . "' LIMIT 1"; $result = mysql_query($sql) or die(mysql_error()); if(mysql_num_rows($result) == 0) { $sql = "INSERT INTO cmsModules VALUES (null, '$module', 0)"; mysql_query($sql) or die(mysql_error()); } } } // End function updateMods() public function loadMods() { // Load the module and display to page $this->updateMods(); // Update the database of modules first $module_base_dir = './modules/'; $module_ext = '.module.php'; $sql = "SELECT * FROM cmsModules WHERE enabled=1"; // Only select enabled modules $result = mysql_query($sql) or die(mysql_error()); while($row = mysql_fetch_assoc($result)) { $module = $row['name']; $module_base_file = $module . $module_ext; if(file_exists($module_base_dir . $module . '/' . $module_base_file)) { // Make sure file exists then... include_once($module_base_dir . $module . '/' . $module_base_file); // Include file once $moduleClass = new $module; // Instantiate modules class if(method_exists($moduleClass, 'loadMod')) { $moduleClass->loadMod(); // Run modules load mechanism } else { echo 'Error: Incorrectly formatted module.'; } } else { echo 'Error: Module ' . $module . ' does not exist<br />'; } } } // End construct } ?> Any constructive feedback on ways to improve this would be greatly appreciated.
-
Content Management System design with OOP
DeadxBeat replied to DeadxBeat's topic in Application Design
Alright, so just so I can clarify here is an example of what im doing. Here is my class file: <?php require_once '_db.php'; require_once '_news.php'; require_once '_cms.php'; require_once '_user.php'; require_once '_admin.php'; require_once '_module.php'; $_db = new _db(); $_cms = new _cms(); $_news = new _news(); $_mods = new _module(); ?> then lets say my _news.php file looks like this: <?php class _news { public function displayNews() { // display news } } ?> then lets say my _cms.php file looks like this: <?php class _cms { public function getPage($p = $_GET['p']) { if (isset($p)) { // display page function } else { $news = new _news(); $news->displayNews(); } } } ?> This would be ok? -
Content Management System design with OOP
DeadxBeat replied to DeadxBeat's topic in Application Design
No template, I want this all to be very basic and structured by myself. What I meant by me being stuck is that I can't decide what functions should go where. For example, right now in my main cms class i have a function called displayNews() and then in my news class is addNews() and remNews(). I'm not sure if I should put displayNews in the cms class or the news class because if i put it in the news class then I would have to create a new news object inside my main cms class and I assume that is bad practice. So my question now, is it viable / good practice to call another classes function from within a different class? -
Content Management System design with OOP
DeadxBeat replied to DeadxBeat's topic in Application Design
Would appreciate a reply from anyone with experience. -
Wasn't scanning for files, just directories. Anywho, I figure it out using a combo of scandir and is_dir and some array functions.
-
Ok...so I'm trying to code a module loading system and I've got it working to where if I name the modules in a database it will load them from their correct folders in the module folder. Now I want to take it a step further and run a construct in my module class that will put the directories in the module folder into an array and then automatically add them to a database if they aren't in there and also remove the items from the database whose folders no longer exist to prevent from trying to load a module with no files. Really, all I need to know is the most efficient way to scan a directory that is given and put the names of all folders only(no files) into an array. Any help would be greatly appreciated, I have looked at scandir() and such but I need to be given the context in how to use it the way I want to, also how to exclude the files and the . and .. from the listing
-
Are you displaying the results in a table? If you are then you could just use a counter in your loop that retrieves the data. $maxrows = 3; // max number of rows per column $count = 0; // counter for loop echo ' '; // start table echo ' '; // start first column in table loop { // whatever kind of loop you decide to use if($count == $maxrows){ // if the $counter variable equals max rows per column var the $count = 0; // set $count back to 0 echo ' '; // close your column, start a new one } $count++; // increment $count by 1 echo ' '; //new row } // close loop
-
Hello Everyone, First off, I read the sticky, this is not a question about OOP but rather a question about design with OOP in mind. With that said, lets get to it. I'm a programmer with a good understanding of PHP and how to use it, I'm relatively new to using OOP but picked up on it quick. I'm trying to develop a content management system using OOP instead of just using an include file with a bunch of unorginized functions. My question is a matter of opinion, I'm just looking for ideas on what classes I should make and for what. So far, I've written the following classes down on a piece of paper: Database Class(Load db variables, connect to db) User Class(Add users, remove users, verify users, load user variables, session initiation) News Class(Add news, delete news, modify news) Admin Class(Modify User permissions) Module Class(add module, remove module) CMS Class(Display Navigation, Display News, Display Modules) Do these seem like adequite classes with good functions also what else would you recommend adding to make a basic CMS. The thing I'm really stuck on is if I should use CMS Class to display everything or if i should include things like Display News in the news class and display modules in the module class. Any ideas / sugguestions would be greatly appreciated. Thanks, Daniel.