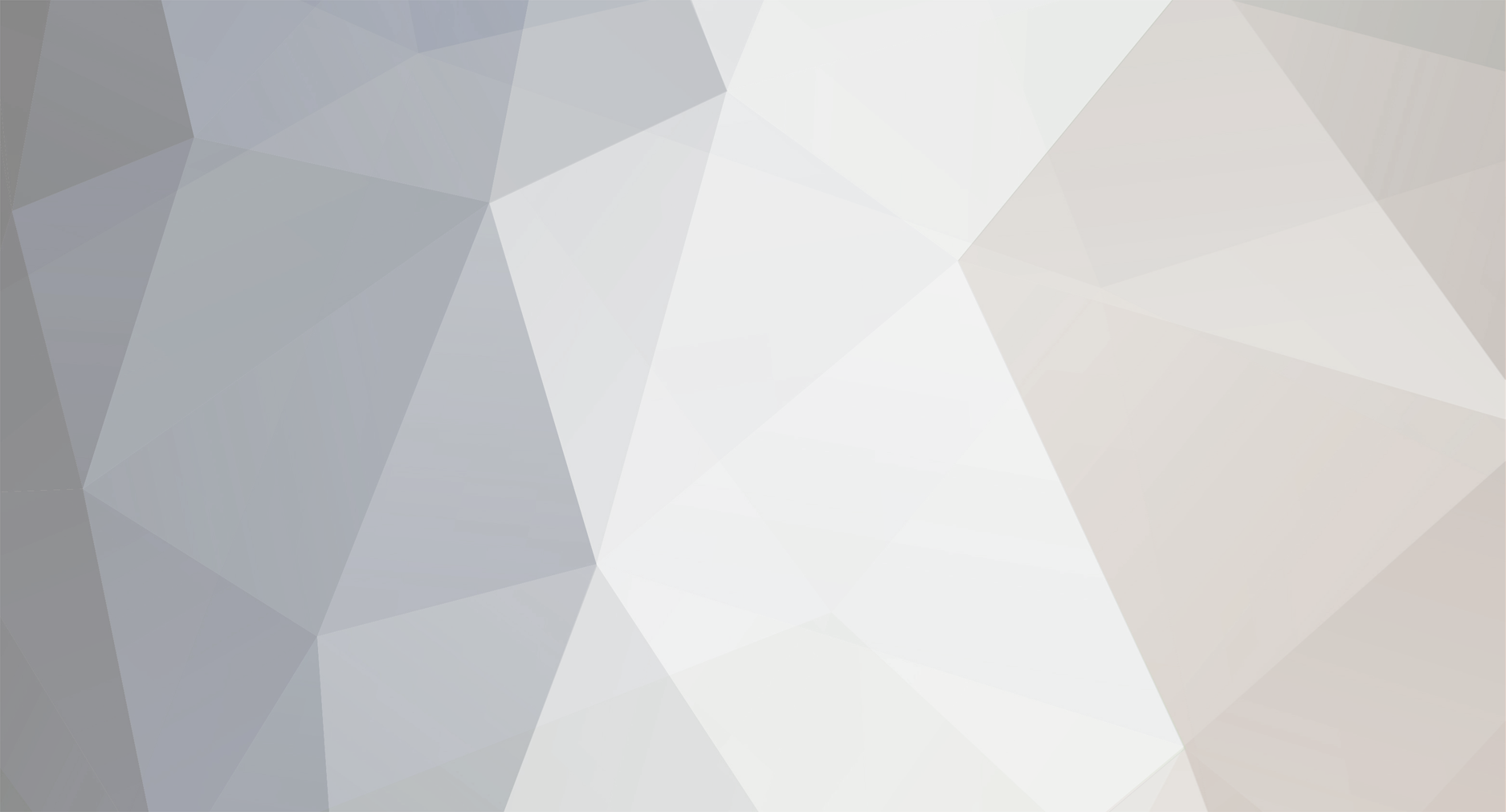
peppericious
-
Posts
124 -
Joined
-
Last visited
Posts posted by peppericious
-
-
I know this should be easy.... However, to validate a 7-digit phone number, I wrote this:
<?php if(!empty($_POST['mobile_number'])) { if(!is_numeric($_POST['mobile_number']) || strlen($_POST['mobile_number']) != 7) { $errors[] = 'Please enter a 7-digit number without spaces or dashes for your <strong>Mobile</strong>.'; } else { $_SESSION['mobile_number'] = $_POST['mobile_number']; } } else { $_SESSION['mobile_number'] = ''; }
... but now I realise that numeric/integer validation won't work because where I am - in Ireland - mobile numbers can start with an initial zero, too. So, the following mobile numbers are possible:
- 0123456
- 0224466
How can I ensure that:
[*]each digit in the mobile number is numeric
[*]an initial zero is possible
[*]there are exactly 7 digits
I suppose I loop through each digit, ensuring that that digit is numeric and break out of the loop if a non-numeric digit is encountered..?
Any suggestions?
TIA
- 0123456
-
Forgot to include the $selected variable in the output
$optionsList .= "<option value='{$value}'{$selected}>{$label}</option>\n";
Thank you very much for your help. Much appreciated.
-
Change
$optionsList = "<option value='{$value}'>{$label}</option>\n";
To
$optionsList .= "<option value='{$value}'>{$label}</option>\n";
Note the '.=' (dot equal) which appends the string to the current value of the variable
Thank you... However, the menu selection is not being retained on submission of the form...
:0(
-
I'm running into a problem....
Using the function as in this example:
//Don't use keys as values $instruments = array('Bassoon', 'Cello', 'Clarinet'); create_options($instruments, false); //Output: // <option value='Bassoon'>Bassoon</option> // <option value='Cello'>Cello</option> // <option value='Clarinet'>Clarinet</option>
... only the last item in the array is being displayed in the menu.
Do I need to amend the function somehow?...
TIA
-
Thank you both for your help.
Psycho, that's a super solution. I'm going to work on it right now. Thanks, too, for your pointers about not echoing with the function. Noted.
-
Some forum users here gave me great help yesterday in working with dropdown menus. In fact, I need quite a few of these dropdown menus in several forms over several pages, so I created several simple arrays and made a function to create the dropdowns.
Here's one of my arrays:
<?php $instruments = array( 'Bassoon', 'Cello', 'Clarinet', 'Double Bass', 'Flute', 'French Horn', 'Oboe', 'Percussion', 'Trombone', 'Trumpet', 'Tuba', 'Viola', 'Violin', 'Other' );
Here's my function:
<?php function create_dropdown($array_name, $array_item) { echo "<select name='$array_item'>\n"; echo "<option value='select'>Select…</option>\n"; foreach( $array_name as $v ) { echo "<option value='$v'>" . $v . "</option>\n"; } echo "</select>\n"; }
... and anywhere I need a dropdown menu, I'm calling it like this:
<?php create_dropdown($instruments, 'instrument');
The dropdown menus, however (in some fairly detailed forms for my local youth orchestra's site), need to be sticky.
How can I modify my function, above, so that the selected value will be retained if there are other form submission errors when the form is submitted?
I have tried endlessly today but to no avail... If you can help while I still have some hair left, I'd be greatly appreciative.
-
Looks like you just need to move the array definition up above the call to in_array(), and that should take care of it. Variables have to be defined before attempting to access them.
.. should've thought of that. Done now and working like a charm. Thanks a lot.... What a forum this is!
-
Here's the whole script... be gentle, I'm an amateur... it's for a site I did up for my local orchestra...
<?php session_start(); // check to ensure user has come from select screen if(!isset($_SESSION['form_started'])) { header('Location: ar_select.php'); exit(); } $formtype = $_SESSION['formtype']; $orch = $_SESSION['orch']; $page_title = "Register/Audition | Musical Experience"; // run validations on all input if(isset($_POST['submit'])) { include('includes/mysqli_connect.php'); $errors = array(); // teacher first if (empty($_POST['mus_teacher_fname'])) { // $errors[] = '<strong>Music Teacher First Name</strong>: you forgot to enter this.'; } else { if (get_magic_quotes_gpc()) { $_SESSION['mus_teacher_fname'] = ucwords(mysqli_real_escape_string($dbc, stripslashes(trim($_POST['mus_teacher_fname'])))); } else { $_SESSION['mus_teacher_fname'] = ucwords(mysqli_real_escape_string($dbc, trim($_POST['mus_teacher_fname']))); } } // teacher last if (empty($_POST['mus_teacher_lname'])) { // $errors[] = '<strong>Music Teacher Last Name</strong>: you forgot to enter this.'; } else { if (get_magic_quotes_gpc()) { $_SESSION['mus_teacher_lname'] = ucwords(mysqli_real_escape_string($dbc, stripslashes(trim($_POST['mus_teacher_lname'])))); } else { $_SESSION['mus_teacher_lname'] = ucwords(mysqli_real_escape_string($dbc, trim($_POST['mus_teacher_lname']))); } } // Examining Body if (empty($_POST['ex_body'])) { // $errors[] = '<strong>Examining Body</strong>: you forgot to enter this.'; } else { if (get_magic_quotes_gpc()) { $_SESSION['ex_body'] = ucwords(mysqli_real_escape_string($dbc, stripslashes(trim($_POST['ex_body'])))); } else { $_SESSION['ex_body'] = ucwords(mysqli_real_escape_string($dbc, trim($_POST['ex_body']))); } } // Musical experience if (empty($_POST['mus_exp'])) { // $errors[] = '<strong>Musical experience</strong>: you forgot to enter this.'; } else { if (get_magic_quotes_gpc()) { $_SESSION['mus_exp'] = ucwords(mysqli_real_escape_string($dbc, stripslashes(trim($_POST['mus_exp'])))); } else { $_SESSION['mus_exp'] = ucwords(mysqli_real_escape_string($dbc, trim($_POST['mus_exp']))); } } // instrument if(isset($_POST['instrument']) && in_array($_POST['instrument'], $instruments)) { $_SESSION['instrument'] = $_POST['instrument']; } else { $errors[] = 'Please select your instrument.'; } // end validations if(empty($errors)) { // get all the session variables and write them to the db //////////////////// } } // close IF submitted conditional include('includes/header.php'); ?> <p><img src="images/demo-images/art1.gif" alt="" width="170" height="220" /></p> </div> <!-- c1 content closer --> </div> <!-- c1 closer --> <div id="c2"> <div class="content"> <p><img src="images/site/reg_banner_3.png" /><p> <h2><?php echo $formtype . " for " . $orch; ?></h2> <p>Fields marked with an asterisk (<span class='red'>*</span>) are required.</p> <?php //////////// // if no errors, say post done if (!empty($errors)) { // If everything's OK. // errors, so report them echo "<div id='error_box'><h2>Oops!…</h2> <ul class='error'>Please fix the following error(s):" ; foreach ($errors as $msg) { // display each error echo "<li>" . $msg . "</li>"; } echo '</ul></div>'; } //////////// ?> <div id='reg_form'> <form id="form1" name="form1" method="post" action=""> <fieldset> <legend>Music related information</legend> <div class='col'> <ul> <li> <label for="music_school">Music School</label> <select name="music_school" id="music_school"> <option value="csm" selected="selected">CSM</option> <option value="abrsm">ABRSM</option> <option value="ccvec">CCVEC</option> <option value="ria">RIA</option> <option value="other">Other</option> </select> <label for="mus_teacher_fname">Music Teacher First Name</label> <input type="text" name="mus_teacher_fname" id="mus_teacher_fname" /> <label for="mus_teacher_lname">Last Name</label> <input type="text" name="mus_teacher_lname" id="mus_teacher_lname" /> <label for="instrument"><span class='red'>*</span>Your main instrument</label> <?php $instruments = array( 'bassoon', 'cello', 'clarinet', 'double bass', 'flute', 'french horn', 'oboe', 'percussion', 'trombone', 'trumpet', 'tuba', 'viola', 'violin', 'other' ); echo "<select name=\"instrument\">\n"; echo "<option value=\"select\">Select One</option>\n"; foreach( $instruments as $v ) { echo "<option value=\"$v\">" . ucfirst($v) . "</option>\n"; } echo "</select>\n"; ?> </li> </ul> </div> <div class='col'> <ul> <li> <p style='font-weight:bold'>Last music exam passed:</p> <label for="grade">Grade</label> <select name="grade" id="grade"> <option value="1" selected="selected">1</option> <option value="2">2</option> <option value="3">3</option> <option value="4">4</option> <option value="5">5</option> <option value="6">6</option> <option value="7">7</option> <option value="8">8</option> </select> <label for="mus_ex_year">Year</label> <select name="mus_ex_year" id="mus_ex_year"> <?php for ($year = date("Y")-10; $year <= date("Y"); $year+= 1) { echo "<option value='" . $year . "'>" . $year . "</option>"; } ?> </select> <label for="ex_body">Examining Body</label> <input type="text" name="ex_body" id="ex_body" /> </li> </ul> </div> <div class='clearfloat'></div> <label for="mus_exp">Do you already have experience of playing in an orchestra or other ensemble?</label> <textarea name="mus_exp" id="mus_exp" ></textarea> </fieldset> <fieldset> <legend>Terms & Conditions</legend> <input type="checkbox" name="t_and_c" id="t_and_c" /> <label for="t_and_c" style="display:inline;">I have read and agree to the <a href="#">Terms & Conditions</a> of Cork Youth Orchestra.</label> </fieldset> <input type="submit" name="submit" id="submit" value="Submit My Application" /> </form> </div> <!-- reg form closer --> </div> <!-- c2 content closer --> </div> <!-- c2 closer --> <?php include('includes/footer.php'); ?>
-
That's very helpful and informative, Pikachu2000...
However, when I put this in my form....
<label for="instrument"><span class='red'>*</span>Your main instrument</label> <?php $instruments = array( 'bassoon', 'cello', 'clarinet', 'double bass', 'flute', 'french horn', 'oboe', 'percussion', 'trombone', 'trumpet', 'tuba', 'viola', 'violin', 'other' ); echo "<select name=\"instrument\">\n"; echo "<option value=\"select\">Select One</option>\n"; foreach( $instruments as $v ) { echo "<option value=\"$v\">" . ucfirst($v) . "</option>\n"; } echo "</select>\n"; ?> </li>
.. and this at the top, with my other validations....
if(isset($_POST['instrument']) && in_array($_POST['instrument'], $instruments)) { $_SESSION['instrument'] = $_POST['instrument']; } else { $errors[] = 'Please select your instrument.'; }
... I get a "Notice: Undefined variable: instruments..." message on line 62, that is this line...
if(isset($_POST['instrument']) && in_array($_POST['instrument'], $instruments)) {
...?
-
if(!isset($_POST['instrument']) || $_POST['instrument'] == "select")
Perfect. Thanks!...
-
I have a dropdown menu in a form where users must select their instrument: here's the dropdown:
<label for="instrument"><span class='red'>*</span>Your main instrument</label> <select name="instrument" id="instrument"> <option value="select" selected="selected">Select your instrument…</option> <option value="bassoon">Bassoon</option> <option value="cello">Cello</option> <option value="clarinet">Clarinet</option> <option value="double_bass">Double Bass</option> <option value="flute">Flute</option> <option value="french_horn">French Horn</option> <option value="oboe">Oboe</option> <option value="percussion">Percussion</option> <option value="trombone">Trombone</option> <option value="trumpet">Trumpet</option> <option value="tuba">Tuba</option> <option value="viola">Viola</option> <option value="violin">Violin</option> <option value="other">Other</option> </select>
I want to validate to ensure that the user has selected an instrument from the list. I was going to do something like this:
// validate for instrument selection if(!isset($_POST['instrument'])) { $serrors[] = 'Please select your instrument.'; } else { $_SESSION['instrument'] = $_POST['instrument']; }
The problem is, the session variable gets set if the user bypasses the menu altogether, leaving the default 'Select your instrument...' option (at the top) as it is.
How can I ensure a selection other than the 'Select your instrument...' at the top of the list?
Thanks in advance.
-
A text field is always set, as defined by isset(), even if it's empty. You can use the empty function to check that it is set, and has a non-false value.
Excellent, thanks. Changed it all to this and it works perfectly.
<?php // ensure that at least one of Mother or Father mobile is entered if(empty($_POST['mother_mobile']) && empty($_POST['father_mobile'])) { $errors[] = 'You must enter a mobile number in at least one of the Mother or Father Mobile fields.'; } else { // if Mother mobile if(!empty($_POST['mother_mobile'])) { if(!is_numeric($_POST['mother_mobile']) || strlen($_POST['mother_mobile']) != 7) { $errors[] = '<strong>Mother mobile</strong>: please enter a 7-digit number without spaces or dashes.'; } else { $_SESSION['mother_mobile'] = $_POST['mother_mobile']; } } // if Father mobile if(!empty($_POST['father_mobile'])) { if(!is_numeric($_POST['father_mobile']) || strlen($_POST['father_mobile']) != 7) { $errors[] = '<strong>Father mobile</strong>: please enter a 7-digit number without spaces or dashes.'; } else { $_SESSION['father_mobile'] = $_POST['father_mobile']; } } }
-
I have 2 fields, one for Mother's mobile, the other for Father's mobile. I want to ensure that at least one of the 2 fields contains a number. This is what I have:
<?php if(!isset($_POST['guardian1_mobile']) && !isset($_POST['guardian2_mobile'])) { // no number entered in either field $errors[] = 'You must enter a mobile number in at least one of the Guardian1 or Guardian2 Mobile fields.'; } else { // if Mother mobile if(!is_numeric($_POST['guardian1_mobile']) || strlen(trim($_POST['guardian1_mobile'])) != 7) { // number must be numeric and exactly 7 digits $errors[] = '<strong>Guardian1 mobile</strong>: please enter a 7-digit number without spaces or dashes.'; } else { $_SESSION['guardian1_mobile'] = $_POST['guardian1_mobile']; } // if Father mobile if(!is_numeric($_POST['guardian2_mobile']) || strlen($_POST['guardian2_mobile']) != 7) { $errors[] = '<strong>Guardian2 mobile</strong>: please enter a 7-digit number without spaces or dashes.'; } else { $_SESSION['guardian2_mobile'] = $_POST['guardian2_mobile']; } }
However, when I leave both fields blank, errors for the 'else' part of the condition are returned ('Please enter a 7-digit number...'). But it should never get as far as the 'else'....
Can anyone tell me what's wrong with my logic in the first line?...
Thanks in advance.
-
I got this nailed down nicely, even though I say so myself, with the following:
<script> $(document).ready(function() { $('.answer').hide(); $('.main h3').toggle( function() { $(this).next('.answer').slideDown(); }, function() { $(this).next('.answer').fadeOut(); } ); // end toggle }); // end ready </script> </head> <body> <div class='main'> <h3>Me voici à Nice.</h3> <div class='answer'> <p>Here I am in Nice.</p> </div> <h3>Je m'amuse bien avec mes amies Sara et Chloë.</h3> <div class='answer'> <p>I'm really having fun with my friends Sara and Chloë.</p> </div> </div> </body>
Wow... this javascript/jQuery combination could be the catalyst for another addiction!...
-
Thanks, I've already figured out now how to target *all* items with a particular class. Still some work to do before I can target individual sentence pairs, but I'm on it...
-
I'm pulling pairs of sentences out of a database using php (for students I'm teaching).
The pairs of sentences are formatted something like this:
<p><span class="sentence">This is the primary sentence (in French).</span><br /><span class="translation">This is a translation of the sentence (in English).</span></p>
I'm already loading jQuery on the page (for other functionality).
How can I script something so that, when I roll over a 'sentence' span, the 'translation' span will fade in to visible, and will then fade out again - to invisible – when I roll off the corresponding 'sentence' span?
(So, to clarify, the 'translation' spans are invisible initially but become visible when the corresponding 'sentence' span is rolled over.)
Is it possible? Am I making sense?...
Be gentle... I'm brand new to javascript...
-
I have a little blog which allows users reply to posts. Replies go into a 'replies' table, awaiting moderation. The table has a moderation_level column. Values in this column are 1, 2 or 3, denoting 'Queued', 'Accepted', 'Rejected', respectively.
moderation_queue.php will display recent replies and allow me to alter the moderation_level value by clicking a radio button.
Each reply on this page will have three radio buttons - 'Queued', 'Accepted' and 'Rejected' - one of which should be selected according to the current value of the moderation_level column in the db. (By default, all replies are initially assigned a value of '1'.)
However I can't figure out how to have the appropriate radio button for each reply selected, or not, according to this value.
Any help greated appreciated. Thanks in advance.
Here's my code on moderation_queue.php:
function get_replies_to_moderate() { global $dbc; $q = "SELECT id, f_blogpost_id, body, f_responder, DATE_FORMAT(created, '%Y') AS year, DATE_FORMAT(created, '%b') AS month, DATE_FORMAT(created, '%d') AS day, DATE_FORMAT(created, '%H:%i') AS time, moderation_level FROM blog_replies WHERE moderation_level = 1 ORDER BY id DESC"; $r = mysqli_query($dbc, $q); if (mysqli_num_rows($r) > 0) { while ($row = mysqli_fetch_array($r, MYSQLI_ASSOC)) { ?> <tr> <td><?php echo $row['id']; ?></td> <td><?php echo $row['f_blogpost_id']; ?></td> <td><?php echo $row['body']; ?></td> <td><?php echo $row['f_responder']; ?></td> <td><?php echo $row['time'] . ' ' . $row['day'] . ' ' . $row['month'] . ' ' . $row['year']; ?></td> <td> <label> <input name="moderation_level" type="radio" value="queued" <?php // not sure what to put here... ?> /> Queued</label> <label> <label> <input name="moderation_level" type="radio" value="accept" <?php // not sure what to put here... ?> /> Accepted</label> <label> <input name="moderation_level" type="radio" value="reject" <?php // not sure what to put here... ?> /> Rejected</label> </td> </tr> <?php } // close WHILE } // close if-posts-exist IF }
-
To get my 'Edit' links to take me to an 'edit_news_item.php' page, I query the database to retrieve an array of info about the particular news item, including its id. Then, I do this:
echo "<p><a href='edit_news_item.php?news_id=" . $row['news_id'] . "'>Edit</a></p>";
That way, the id of the news entry is passed in the URL to the edit_news_item.php page (where another query can pull up whatever data I want to edit in the news entry).
I'm not an expert, but the above works for me.
-
I am looking for someone who would be interested in giving me tutorials in php and mysql - and maybe a bit of jquery/javascript, later - perhaps over Skype?...
I have already built a few sites using html, css, php, mysql, but the functionality of these sites is quite simple and I'd like to be able to do more.
(I also have some other sites which were built for me by a pro several years ago. I am still on very good terms with this developer. However, he has become extremely busy - and is getting busier - and is consequently hard to pin down when I want to make changes to those sites. I would like to be able to make mods to those sites, too, myself over time.)
I know there are many, many tutorials available on the net already, but I really want to discuss specific issues with someone as I proceed with particular tasks I want to accomplish.
At this stage, I don't know how many tutorials I would need. Maybe 6 - 10 one-hour tutorials spread over a month or 6 weeks for starters?...
If you'd be interested in being my tutor, we could talk for a few minutes - over Skype - so that you'd have a better idea of what I want, what I already know, what I'd like to be able to do (having worked with you), and so on. I would be more than happy to pay for the tutorials, via PayPal, or whatever.
I have never had any formal computer training but have taught myself quite a bit about php and mysql over the last year.
It doesn't matter to me where you're based. Keep in mind, however, that I'm in Ireland. So, if you're in the U.S., for example, we would need to take the time difference into account.
If you're interested, please send me a personal message and I'll get back to you. (Please give me some indication, if you can, of what you'd charge me for the tutorials.)
-
I want to add a query to a page to retrieve blog posts from a table I've added to the db . At the top of the page in an include, the following db connector (not made by me) is already in use:
<?php class Database { private $conn; function __construct() { $this->conn = mysql_connect("localhost", "root", "root") or die(mysql_error()); mysql_select_db("thedatabase", $this->conn); } function query($sql) { $result = mysql_query($sql, $this->conn); if(!$result) { die(mysql_error()); } else { return $result; } } } ?>
I've not used classes before, nor have I used functions of the type in the code snippet above so I'll admit I'm fumbling around. If I were using a more basic connector, the query I would run would be something like this:
<?php $q = "SELECT post_id, post_title, post_subtitle, post_body, DATE_FORMAT(post_created, '%M %D %Y') AS created_on, post_ext_link FROM blog ORDER BY post_id DESC LIMIT 3"; $r = @mysqli_query($conn, $q); if (mysqli_num_rows($r) > 0) { while ($row = mysqli_fetch_array($r, MYSQLI_ASSOC)) { echo "<h2>" . $row['post_title'] . "</h2>" . "<h3" . $row['post_subtitle'] . "</h3>" . "<p" . $row['post_body'] . "</p>" . "<p" . $row['created_on'] . "</p>" ; } } else { echo '<p>No posts to display at present.</p>'; } ?>
Could someone kindly oblige me by telling me what changes I must make to the query so that it can make use of the connector already in use on the page? Hope my question makes sense...
All/any help appreciated.
-
Great. Will look into that. Thanks.
-
As a timestamp.
-
To echo this:
Posted on: October 23rd 2011
... I'm querying my db with this:
DATE_FORMAT(creation_date, '%M %D %Y')
What if I wanted to echo this:
23. Oktober, 2011
... that is, to display the date as it would be shown in German?
TIA if you can help.
-
Thanks, everybody, for your help with this. I found a solution, rewriting some code as follows:
while ($row = mysqli_fetch_array($r, MYSQLI_ASSOC)) { $ttl = preg_replace('/(?![^<>]*>)'.preg_quote($keywords,"/").'/i', '<span class="highlight">$0</span>', $row['title']); $subt = preg_replace('/(?![^<>]*>)'.preg_quote($keywords,"/").'/i', '<span class="highlight">$0</span>', $row['subtitle']); $nws = preg_replace('/(?![^<>]*>)'.preg_quote($keywords,"/").'/i', '<span class="highlight">$0</span>', $row['news_entry']); echo "<div class='news_borders_top_bottom'>" . '<h1>' . $ttl . '</h1>' . '<h2>' . $subt . '</h2>' . '<p>' . $nws . '</p>' . '<div class="created_on">Created on: ' . $row['created'] . '</div></div>'; }
Now the search function, as well as the highlighting, works fine.
validating a 7-digit phone number
in PHP Coding Help
Posted
Thanks, guys. Excellent.