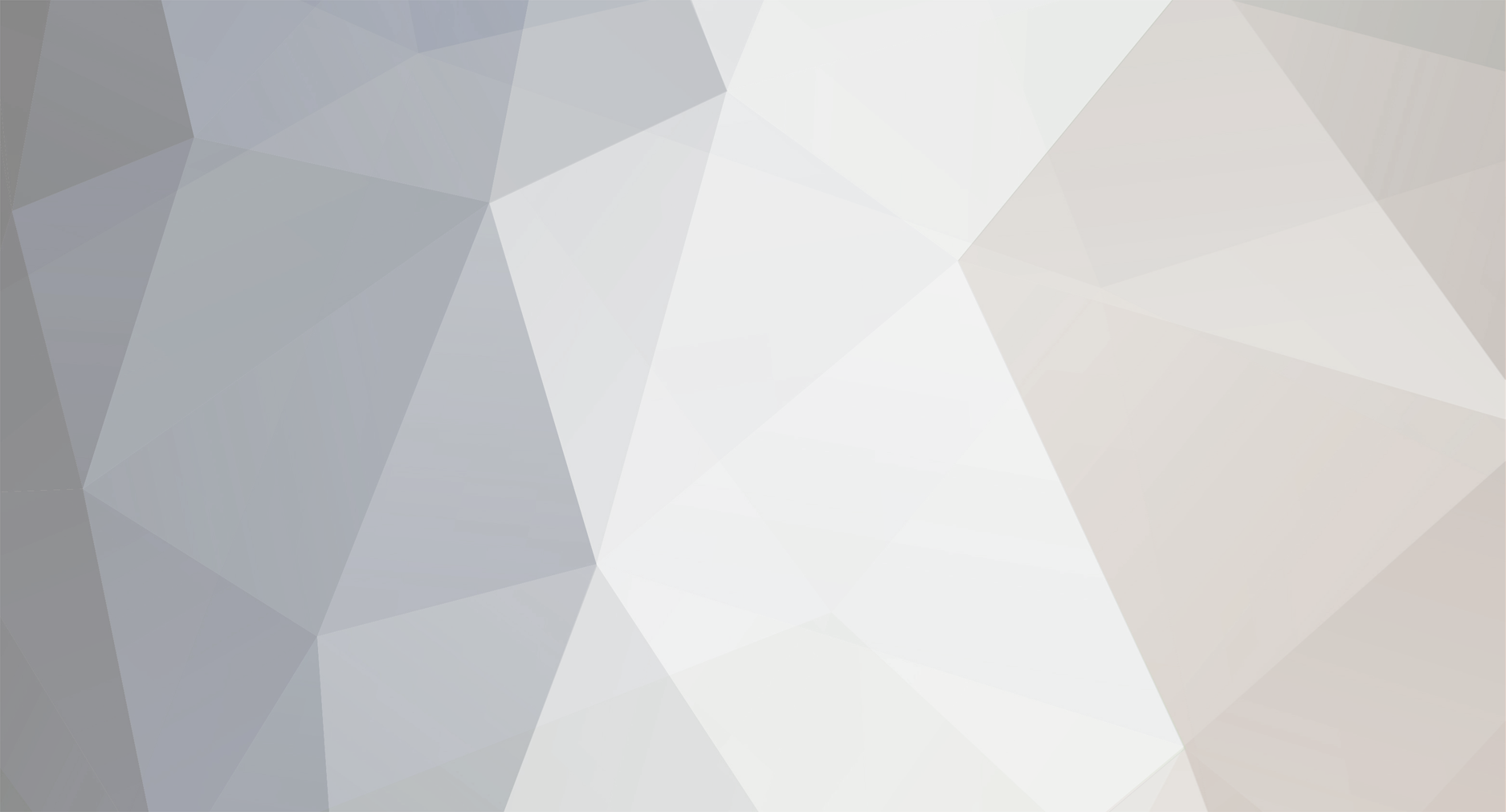
Mahngiel
-
Posts
1,068 -
Joined
-
Last visited
Posts posted by Mahngiel
-
-
Depending on how you arrive at "Ford Explorer" being the make/model, you can do this two ways. You can either carry over the make and model in the query string ($_GET) or you can make hidden form fields.
-
-
jrw4 last visited these forums in december of 2009. i don't think he'll be responding to your help.
-
With being a professional yourself, and notwithstanding the benefits of learning to build a foundation... given that the code needs to be rewritten anyway (i'm afraid to update from php 5.2 to break this legacy application) should I stick to a framework?
-
you could define it pixel based if you wanted. to get the page contents "separated" from the body of the browser, you could add padding to the body. because elements positioned other than relatively (order of appearance on the page) [floats, absolutes, fixes] remove themselves from the natural flow of the page, you have to encapsulate them together. That lack of encapsulation is why you found this error in the first place, and why putting them into one fixed container fixes the issue.
if your site is going to be fixed width, it needs to start at the top-most parent container. if that width is less than the body width (width of the browser) auto margins will center it accordingly - either vertically or horizontally.
-
on your resume, you've misspelled .htaccess... twice.
other than that, i like the polish you give to your sites.
-
It takes a little while to get used to, especially if you're trying to roll your own. That's one of the reasons why I like to use a solid framework - DI is usually baked right in, and if not, it's usually not hard to find a separate component that can plug right in.
I didn't want to, I'm comfortable in a framework environment where I didn't have to completely understand the mechanics, only that it worked. It has been my introduction into the concepts as I have no formal training, just my own use study. However, I received a project written over 10 years ago in procedural PHP, zero documentation, and everything relying on global.
I figured this would be a great opportunity to get to understand the core foundational principals of Classes and OOP. Initially I used typing / DI to pass the objects (as demonstrated in that book you keep linking to, Kevin), but after taking a look at the core of codeigniter I got the impression you could create a massive singleton-esque class where everything lies within on giant body.
I appreciate the pointers and will take a fresh look at how I want to accomplish my goals.
-
use $.load() to bring up a new html page and post the data from it via ajax to a .php script
-
So, if I understand correctly, instead of loading on large class to grant access to objects, I should instead build a chain that passes the required objects between each other during preload that I would want to use later.
-
I am using your method of creating the salt on the fly withOUT storing the users unique salt in the database. So I'm assuming
$user->salt
will work?
Why on earth would you do that? You would NEVER accomplish finding a pair. The salt method I provided to you earlier generates a random 32 character string. If you do not save this salt, you will never match it.
Lastly, I am getting the
Fatal error: Call to undefined method User_model::get_user() in /Users/michaelsanger/Sites/cl_ci_new/application/controllers/auth.php on line 34
error again.
You didn't add that method to your model
CONTROLLER:
<?php function validate_credentials() { // loading the model with the the second object being the database name? $this->load->model('user_model', 'users');
The second parameter passed to the load object is a reference name. You could have a class called Uber_long_and_retarded_class_name_model and pass 'uber' as the second parameter to use
$this->uber->method()
.CONTROLLER:
<?php // when the user hits submit and enters their info, the following checks takes what they entered and stores it in $data and sends over to the model to run and check the query log the user in and start their session. $login = $this->input->post('submit'); if($login) { $user = $this->users->get_user( array('email' => $this->input->post('email')) ); $query = $this->user_model->validate(); }
a) maybe you retrieved a return on $user, maybe you didn't. You haven't checked.
b) you're not sending anything to your validation model, which it is expecting
CONTROLLER:
<?php if($user) { $data = array( 'email' => $user->email, 'password' => $this->encrypt->sha1($user->salt. $this->encrypt->sha1($this->input->post('password'))) ); $user = $this->users->get_user($data); }
a) now you're checking if your user object returned anything useful. problem is, you don't have a value for
$user->salt
CONTROLLER:
<?php if($query) { $data = array( 'email' => $this->input->post('email'), 'is_logged_in' => true ); $this->session->set_userdata($data); redirect('account/dashboard'); }
a) query will not return because you didn't pass the parameter above
b) why are you setting arrays for your userdata?
$this->session->set_userdata('key', 'value');
echo $this->session->userdata('key');
CONTROLLER:
<?php else { $this->index(); } }
You've provided no output for the user on failure. Why?
MODEL:
// takes the data created by the user from the controller and checks it with the database function validate($data) { $this->output->enable_profiler(TRUE); $query = $this->db->where($data)->get('users', 1); if($query->row()) { return $query->row(); } }
Though this is a valid use, it's not logical. You model should not validate your user, it should only return data and your controller decide if it's valid. Then, your controller can do other actions against that. Your models should have methods that perform tasks. See if you can guess what these do merely by their titles
get_user()
get_users()
delete_user()
add_user()
count_users()
-
need more code. post the JS and the structure that works.
-
when i encompass the data in a div tag is when the javascript doesn't work. Any thoughts on why the javascript doesn't work due to div tags?
Seems to be a loss of scope issue. Your JS is likely looking for a child of body and not body>div
-
Would I use jquery and javascript?
Yes, these sorts of actions require it. jquery is easy for apt individuals to pick up as opposed to vanilla JS. http://jsfiddle.net/Mahngiel/2CfzS/
-
There are a million-and-one tutorials on responsive design out there on the webs. Alistapart gets a lot of linkbacks to it.
-
what does fading have to do with floating?
-
the number is represented in seconds. so divide your number by 60 to get minutes and so on
-
What are you actually trying to do?
The db, session, uri, and smarty classes are going to be used throughout the application, and all rely on each other to handle their jobs. What I wanted to do was simplify the job of having to create new instances and passing them around depending on where the user was.
And example that works:
<?php // need this first $db = new db(); // this requires the db wrapper $session = new Session( $db ); $smarty = new Smarty(); $pageID = $_GET['page']; // send requisite data to uri function to validate everything so the template can be utilized. uri( $sesion, $smarty, $db, $pageID );
and instead, create a preloader that automates the process where the objects are accessible inside of the classes.
-
PHP is a server-side language. That means it gets created before the browser gets to see it and use javascript. This means the problem lies elsewhere in your code.
-
transitions require vendor prefixes (-webkit, -moz, -o, etc). this is because there is no standardized engine rendering method for the CSS property - which means there will be discrepancies on how it will be displayed. For this reason, it is advised that you do not use these properties for anything outside of "play".
-
You are getting there, but your handshakes are funny. I fear there is no way to demonstrate without writing code. Try to follow along as i bounce back and forth between classes.
Controllers/login
<?php // load user model $this->load->model('users_model', 'users'); // catch post $login = $this->input->post('login_button_name'); if( $login ) { $user = $this->users->get_user( array('user_email' => $this->input->post('email')) ); // -- cut to 1
models/user_model
<?php function get_user( $data ) { $query = $this->db->where( $data )->get( 'users', 1 ); if( $query->row() ) return $query->row();
controllers/login
<?php // back to 1 // if your query for a user with the supplied email was returned, then you know a user with that credential exists if( $user ) { // now, using that returned row, grab the salt from it and use it in a second query where you apply the same hash method $data = array( 'user_email' => $user->user_email, 'user_password' => $this->encrypt->sha1( $user->user_salt . $this->encrypt->sha1($this->input->post('password'))) ); // send that new array back to get_user $user = $this->users->get_user( $data );
Now, based on if the last user there is returned, you can check for validated user, send off to a model ( yes, a model here has one of the very rare uses of applying session data ) to apply session data.
Notice how I used the controller for all the logic based off what the model returned. IMO, that is how you properly utilize the MVC method.
-
I would like to build a class that maintains several objects that I will be using regularly across other classes. I've read a few books (several times over) and have a decent understanding on the process. I'm failing to grasp a few issues which are killing the app. This is what I've done (borrowing tips from frameworks):
[*] created a loader to require the classes, open a new instance of it, and then amend it to an array of made classes.
[*] created the base class which owns a static value and method to return said value
That works just fine, classes all get loaded as indicated by get_declared_classes, and print_r'ing $this inside the base class. Now, what I'm trying to figure out is how to access the base's loaded classes from the other loaded classes. Here's some relevant code.
<?php echo '<pre>'; class Base { /* ---------------------------*/ private static $me; protected $classes = array(); function __construct( ) { // create reference self::$me =& $this; // create array of loaded classes $this->classes =& loaded_classes(); print_r( $this->classes ); // create superobject, making sure each class is loaded foreach ($this->classes as $class) { $this->$class =& load_class($class); } print_r($this); } public static function &get_me() { return self::$me; } }
The dumps for this are:
Array ( [db] => db [session] => session [uri] => uri [smarty] => smarty ) Base Object ( [classes:protected] => Array ( [db] => db [session] => session [uri] => uri [smarty] => smarty ) [db] => db Object ( ... ) [session] => Session Object ( ... ) [uri] => URI Object ( ... ) [smarty] => Smarty Object ( ... ) )
In the session object, calling $this only provides the current scope.
<?php class Session { public static $session; // ---------------------------------------------------------------------- public function __construct( ) { $this->session = $_SESSION; $this->user = $this->get_user(); print_r($this); }
Only gives current scope
Session Object ( [session] => Array ( [tracking] => Array ( [language_path] => /home/dev/language/english ) ) [user] => )
If I add
$BASE = Base::get_me();
into the constructor of the Session class, i fatal error with 'class Base not found'. What have I missed in order to have access to the super global with all the loaded classes? -
It's a very simple concept that I understand fully. Just don't know how to write it!
It's not going to do you any good if I wrote it for you. I'm trying to push you in the right direction, even giving you logic flow. All you need to do is implement it. Again, this comes back to what I've been telling you for weeks: separate the concerns between your controllers and models. STOP doing logic in your models with input values. The only thing you should be doing in your model is talking to the database.
Look at your credentials method. You're not doing what you said (which you claim to be in your last post). Instead, you're trying to match with some random $salt variable. Go back to my last post, and use my flow.
-
I guess what I want to test is when the height of a specific element has changed?
The resize event is sent to the window element when the size of the browser window changes:SO provided this plugin, however: http://benalman.com/projects/jquery-resize-plugin/
-
Can you send AJAX requests on other domains? Such as this:
Sure you can, how else do you think you can log into sites like google, twitter, facebook, or any other site through single-sign-on services? You need to know what parameters the receiver expects and what language to communicate to it width.
page layout with CSS
in CSS Help
Posted
http://www.barelyfitz.com/screencast/html-training/css/positioning/
That is a very informative article on understanding positioning.